How to convert numbers to words python 3
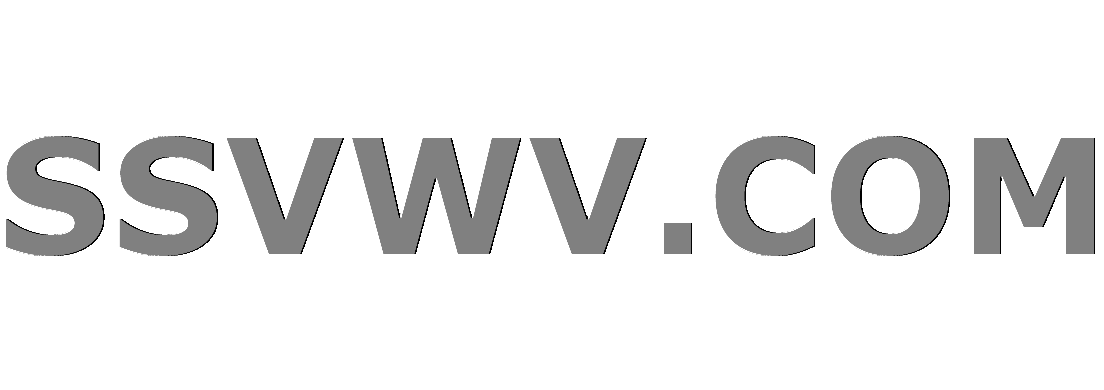
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty height:90px;width:728px;box-sizing:border-box;
I have an issue when trying to convert numbers to words: it crashes when I enter 80, or if i enter 100 it produces "one hundred two hundred". Can someone help me fix my code.
number = input("Enter a value: ")
ones = ["", "one ", "two ","three ", "four", "five ", "six ", "seven ",
"eight ", "nine "]
teens = ["ten ", "eleven ", "twelve ", "thirteen ", "fourteen ", "fifteen ",
"sixteen ", "seventeen ", "eighteen ", "nineteen "]
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy
", "eighty ", "ninety "]
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four
hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred",
"nine hundred"]
word = ""
change = len(number)
while change > 0:
if number == "0":
word ="zero"
break
elif change > 1 and number[change - 2] == "1":
for i in range(0,10):
if number[change - 1] == str(i):
word = teens[i] + word
else:
for i in range(0,10):
if number[change - 1] == str(i):
word = ones[i] + word
if change > 1:
for i in range(0,10):
if number[change - 2] == str(i):
word = decades[i] + word
if change > 2:
for i in range(0,10):
if number[change - 3] == str(i):
word = hundreds[i] + word
change = change - 3
print(word)
python python-3.x for-loop
add a comment |
I have an issue when trying to convert numbers to words: it crashes when I enter 80, or if i enter 100 it produces "one hundred two hundred". Can someone help me fix my code.
number = input("Enter a value: ")
ones = ["", "one ", "two ","three ", "four", "five ", "six ", "seven ",
"eight ", "nine "]
teens = ["ten ", "eleven ", "twelve ", "thirteen ", "fourteen ", "fifteen ",
"sixteen ", "seventeen ", "eighteen ", "nineteen "]
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy
", "eighty ", "ninety "]
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four
hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred",
"nine hundred"]
word = ""
change = len(number)
while change > 0:
if number == "0":
word ="zero"
break
elif change > 1 and number[change - 2] == "1":
for i in range(0,10):
if number[change - 1] == str(i):
word = teens[i] + word
else:
for i in range(0,10):
if number[change - 1] == str(i):
word = ones[i] + word
if change > 1:
for i in range(0,10):
if number[change - 2] == str(i):
word = decades[i] + word
if change > 2:
for i in range(0,10):
if number[change - 3] == str(i):
word = hundreds[i] + word
change = change - 3
print(word)
python python-3.x for-loop
add a comment |
I have an issue when trying to convert numbers to words: it crashes when I enter 80, or if i enter 100 it produces "one hundred two hundred". Can someone help me fix my code.
number = input("Enter a value: ")
ones = ["", "one ", "two ","three ", "four", "five ", "six ", "seven ",
"eight ", "nine "]
teens = ["ten ", "eleven ", "twelve ", "thirteen ", "fourteen ", "fifteen ",
"sixteen ", "seventeen ", "eighteen ", "nineteen "]
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy
", "eighty ", "ninety "]
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four
hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred",
"nine hundred"]
word = ""
change = len(number)
while change > 0:
if number == "0":
word ="zero"
break
elif change > 1 and number[change - 2] == "1":
for i in range(0,10):
if number[change - 1] == str(i):
word = teens[i] + word
else:
for i in range(0,10):
if number[change - 1] == str(i):
word = ones[i] + word
if change > 1:
for i in range(0,10):
if number[change - 2] == str(i):
word = decades[i] + word
if change > 2:
for i in range(0,10):
if number[change - 3] == str(i):
word = hundreds[i] + word
change = change - 3
print(word)
python python-3.x for-loop
I have an issue when trying to convert numbers to words: it crashes when I enter 80, or if i enter 100 it produces "one hundred two hundred". Can someone help me fix my code.
number = input("Enter a value: ")
ones = ["", "one ", "two ","three ", "four", "five ", "six ", "seven ",
"eight ", "nine "]
teens = ["ten ", "eleven ", "twelve ", "thirteen ", "fourteen ", "fifteen ",
"sixteen ", "seventeen ", "eighteen ", "nineteen "]
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy
", "eighty ", "ninety "]
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four
hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred",
"nine hundred"]
word = ""
change = len(number)
while change > 0:
if number == "0":
word ="zero"
break
elif change > 1 and number[change - 2] == "1":
for i in range(0,10):
if number[change - 1] == str(i):
word = teens[i] + word
else:
for i in range(0,10):
if number[change - 1] == str(i):
word = ones[i] + word
if change > 1:
for i in range(0,10):
if number[change - 2] == str(i):
word = decades[i] + word
if change > 2:
for i in range(0,10):
if number[change - 3] == str(i):
word = hundreds[i] + word
change = change - 3
print(word)
python python-3.x for-loop
python python-3.x for-loop
edited Nov 15 '18 at 10:38
WhiteFlower
asked Nov 15 '18 at 10:18
WhiteFlowerWhiteFlower
124
124
add a comment |
add a comment |
5 Answers
5
active
oldest
votes
This should work: word2number
And an example from their docs: print(w2n.word_to_num('one hundred thirty-five'))
I don't think OP wants to use a library.
– Vineeth Sai
Nov 15 '18 at 10:24
@Yasen I do not want to use a library for this applications, thanks though
– WhiteFlower
Nov 15 '18 at 10:31
add a comment |
Just this if statements will work (note that's all you need to make it work):
if len(number)==1:
print(ones[int(number)])
elif len(number)==2 and number[0]=='1':
print(teens[int(number[-1])])
elif len(number)==2 and number[0]!='1':
print(decades[int(number[0])-1])
elif len(number)==3:
print(hundreds[int(number[0])])
Note that have to add commas in decades
and hundreds
, so copy the below, then replace with the clipboard:
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety "]
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred",
2
Thanks for the help. I have tried this it works only for certain cases like 80, 10, 5 etc but if i try 153 or 86 etc it just returns "one hundred" or "eighty".
– WhiteFlower
Nov 15 '18 at 10:37
add a comment |
You have missed a comma between "seventy" and "eighty" in decades. You have to change decades to the following:
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety"]
The same mistake is there in hunderds list as well. Use this new list:
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred", "nine hundred"]
Nevertheless, your implementation has lot of mistakes.
Thanks for that. However, when I enter 80, it is returning 90 and I am unsure as to why if you could help.
– WhiteFlower
Nov 15 '18 at 10:33
2
Note:hundreds
list also has the same problem...
– U9-Forward
Nov 15 '18 at 10:33
@WhiteFlower Use: word = decades[i-1] + word
– Ernest S Kirubakaran
Nov 15 '18 at 10:36
@U9-Forward updated my answer
– Ernest S Kirubakaran
Nov 15 '18 at 10:38
@ErnestSKirubakaran Thanks, I have updated the comma mistakes. I have tried implementing this, however when i enter 95 it returns "five".
– WhiteFlower
Nov 15 '18 at 10:40
|
show 1 more comment
I think that for making your example working you just need to make sure that each change
digit is accessing the correct value in your lists. That's not the case for your decades
lists, where you missed a blank string for the teens, that you're correctly treating as a separate case.
So to make your code work just change:
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety "]
to this:
decades = ["", "", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety "]
add a comment |
For this implementation, you should always have 10 elements in the lists. For example, in decades you should have some string representing the values between 10-20 even if its not used because the indexing of the array would be wrong otherwise.
Still this code has a lot of mistakes.
Try this.
number = input("Enter a value: ")
ones = ["", "one ", "two ","three ", "four", "five ", "six ", "seven ", "eight ", "nine "]
teens = ["ten ", "eleven ", "twelve ", "thirteen ", "fourteen ", "fifteen ", "sixteen ", "seventeen ", "eighteen ", "nineteen "]
decades = ["", "onety", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy ", "eighty ", "ninety "]
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred", "nine hundred"]
word = ""
change = len(number)
while change > 0:
if number == "0":
word ="zero"
break
elif change > 1 and number[change - 2] == "1":
for i in range(0,10):
if number[change - 1] == str(i):
word = teens[i] + word
if change == 2:
break
else:
for i in range(0,10):
if number[change - 1] == str(i):
word = ones[i] + word
if change > 1 and number[change-2]!="1":
for i in range(0,10):
if number[change - 2] == str(i):
word = decades[i] + word
if change > 2:
for i in range(0,10):
if number[change - 3] == str(i):
word = hundreds[i] + word
change = change - 3
print(word)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53317174%2fhow-to-convert-numbers-to-words-python-3%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
This should work: word2number
And an example from their docs: print(w2n.word_to_num('one hundred thirty-five'))
I don't think OP wants to use a library.
– Vineeth Sai
Nov 15 '18 at 10:24
@Yasen I do not want to use a library for this applications, thanks though
– WhiteFlower
Nov 15 '18 at 10:31
add a comment |
This should work: word2number
And an example from their docs: print(w2n.word_to_num('one hundred thirty-five'))
I don't think OP wants to use a library.
– Vineeth Sai
Nov 15 '18 at 10:24
@Yasen I do not want to use a library for this applications, thanks though
– WhiteFlower
Nov 15 '18 at 10:31
add a comment |
This should work: word2number
And an example from their docs: print(w2n.word_to_num('one hundred thirty-five'))
This should work: word2number
And an example from their docs: print(w2n.word_to_num('one hundred thirty-five'))
answered Nov 15 '18 at 10:23
YasenYasen
1,559816
1,559816
I don't think OP wants to use a library.
– Vineeth Sai
Nov 15 '18 at 10:24
@Yasen I do not want to use a library for this applications, thanks though
– WhiteFlower
Nov 15 '18 at 10:31
add a comment |
I don't think OP wants to use a library.
– Vineeth Sai
Nov 15 '18 at 10:24
@Yasen I do not want to use a library for this applications, thanks though
– WhiteFlower
Nov 15 '18 at 10:31
I don't think OP wants to use a library.
– Vineeth Sai
Nov 15 '18 at 10:24
I don't think OP wants to use a library.
– Vineeth Sai
Nov 15 '18 at 10:24
@Yasen I do not want to use a library for this applications, thanks though
– WhiteFlower
Nov 15 '18 at 10:31
@Yasen I do not want to use a library for this applications, thanks though
– WhiteFlower
Nov 15 '18 at 10:31
add a comment |
Just this if statements will work (note that's all you need to make it work):
if len(number)==1:
print(ones[int(number)])
elif len(number)==2 and number[0]=='1':
print(teens[int(number[-1])])
elif len(number)==2 and number[0]!='1':
print(decades[int(number[0])-1])
elif len(number)==3:
print(hundreds[int(number[0])])
Note that have to add commas in decades
and hundreds
, so copy the below, then replace with the clipboard:
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety "]
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred",
2
Thanks for the help. I have tried this it works only for certain cases like 80, 10, 5 etc but if i try 153 or 86 etc it just returns "one hundred" or "eighty".
– WhiteFlower
Nov 15 '18 at 10:37
add a comment |
Just this if statements will work (note that's all you need to make it work):
if len(number)==1:
print(ones[int(number)])
elif len(number)==2 and number[0]=='1':
print(teens[int(number[-1])])
elif len(number)==2 and number[0]!='1':
print(decades[int(number[0])-1])
elif len(number)==3:
print(hundreds[int(number[0])])
Note that have to add commas in decades
and hundreds
, so copy the below, then replace with the clipboard:
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety "]
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred",
2
Thanks for the help. I have tried this it works only for certain cases like 80, 10, 5 etc but if i try 153 or 86 etc it just returns "one hundred" or "eighty".
– WhiteFlower
Nov 15 '18 at 10:37
add a comment |
Just this if statements will work (note that's all you need to make it work):
if len(number)==1:
print(ones[int(number)])
elif len(number)==2 and number[0]=='1':
print(teens[int(number[-1])])
elif len(number)==2 and number[0]!='1':
print(decades[int(number[0])-1])
elif len(number)==3:
print(hundreds[int(number[0])])
Note that have to add commas in decades
and hundreds
, so copy the below, then replace with the clipboard:
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety "]
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred",
Just this if statements will work (note that's all you need to make it work):
if len(number)==1:
print(ones[int(number)])
elif len(number)==2 and number[0]=='1':
print(teens[int(number[-1])])
elif len(number)==2 and number[0]!='1':
print(decades[int(number[0])-1])
elif len(number)==3:
print(hundreds[int(number[0])])
Note that have to add commas in decades
and hundreds
, so copy the below, then replace with the clipboard:
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety "]
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred",
answered Nov 15 '18 at 10:33


U9-ForwardU9-Forward
18.1k51744
18.1k51744
2
Thanks for the help. I have tried this it works only for certain cases like 80, 10, 5 etc but if i try 153 or 86 etc it just returns "one hundred" or "eighty".
– WhiteFlower
Nov 15 '18 at 10:37
add a comment |
2
Thanks for the help. I have tried this it works only for certain cases like 80, 10, 5 etc but if i try 153 or 86 etc it just returns "one hundred" or "eighty".
– WhiteFlower
Nov 15 '18 at 10:37
2
2
Thanks for the help. I have tried this it works only for certain cases like 80, 10, 5 etc but if i try 153 or 86 etc it just returns "one hundred" or "eighty".
– WhiteFlower
Nov 15 '18 at 10:37
Thanks for the help. I have tried this it works only for certain cases like 80, 10, 5 etc but if i try 153 or 86 etc it just returns "one hundred" or "eighty".
– WhiteFlower
Nov 15 '18 at 10:37
add a comment |
You have missed a comma between "seventy" and "eighty" in decades. You have to change decades to the following:
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety"]
The same mistake is there in hunderds list as well. Use this new list:
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred", "nine hundred"]
Nevertheless, your implementation has lot of mistakes.
Thanks for that. However, when I enter 80, it is returning 90 and I am unsure as to why if you could help.
– WhiteFlower
Nov 15 '18 at 10:33
2
Note:hundreds
list also has the same problem...
– U9-Forward
Nov 15 '18 at 10:33
@WhiteFlower Use: word = decades[i-1] + word
– Ernest S Kirubakaran
Nov 15 '18 at 10:36
@U9-Forward updated my answer
– Ernest S Kirubakaran
Nov 15 '18 at 10:38
@ErnestSKirubakaran Thanks, I have updated the comma mistakes. I have tried implementing this, however when i enter 95 it returns "five".
– WhiteFlower
Nov 15 '18 at 10:40
|
show 1 more comment
You have missed a comma between "seventy" and "eighty" in decades. You have to change decades to the following:
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety"]
The same mistake is there in hunderds list as well. Use this new list:
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred", "nine hundred"]
Nevertheless, your implementation has lot of mistakes.
Thanks for that. However, when I enter 80, it is returning 90 and I am unsure as to why if you could help.
– WhiteFlower
Nov 15 '18 at 10:33
2
Note:hundreds
list also has the same problem...
– U9-Forward
Nov 15 '18 at 10:33
@WhiteFlower Use: word = decades[i-1] + word
– Ernest S Kirubakaran
Nov 15 '18 at 10:36
@U9-Forward updated my answer
– Ernest S Kirubakaran
Nov 15 '18 at 10:38
@ErnestSKirubakaran Thanks, I have updated the comma mistakes. I have tried implementing this, however when i enter 95 it returns "five".
– WhiteFlower
Nov 15 '18 at 10:40
|
show 1 more comment
You have missed a comma between "seventy" and "eighty" in decades. You have to change decades to the following:
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety"]
The same mistake is there in hunderds list as well. Use this new list:
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred", "nine hundred"]
Nevertheless, your implementation has lot of mistakes.
You have missed a comma between "seventy" and "eighty" in decades. You have to change decades to the following:
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety"]
The same mistake is there in hunderds list as well. Use this new list:
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred", "nine hundred"]
Nevertheless, your implementation has lot of mistakes.
edited Nov 15 '18 at 10:38
answered Nov 15 '18 at 10:31
Ernest S KirubakaranErnest S Kirubakaran
911510
911510
Thanks for that. However, when I enter 80, it is returning 90 and I am unsure as to why if you could help.
– WhiteFlower
Nov 15 '18 at 10:33
2
Note:hundreds
list also has the same problem...
– U9-Forward
Nov 15 '18 at 10:33
@WhiteFlower Use: word = decades[i-1] + word
– Ernest S Kirubakaran
Nov 15 '18 at 10:36
@U9-Forward updated my answer
– Ernest S Kirubakaran
Nov 15 '18 at 10:38
@ErnestSKirubakaran Thanks, I have updated the comma mistakes. I have tried implementing this, however when i enter 95 it returns "five".
– WhiteFlower
Nov 15 '18 at 10:40
|
show 1 more comment
Thanks for that. However, when I enter 80, it is returning 90 and I am unsure as to why if you could help.
– WhiteFlower
Nov 15 '18 at 10:33
2
Note:hundreds
list also has the same problem...
– U9-Forward
Nov 15 '18 at 10:33
@WhiteFlower Use: word = decades[i-1] + word
– Ernest S Kirubakaran
Nov 15 '18 at 10:36
@U9-Forward updated my answer
– Ernest S Kirubakaran
Nov 15 '18 at 10:38
@ErnestSKirubakaran Thanks, I have updated the comma mistakes. I have tried implementing this, however when i enter 95 it returns "five".
– WhiteFlower
Nov 15 '18 at 10:40
Thanks for that. However, when I enter 80, it is returning 90 and I am unsure as to why if you could help.
– WhiteFlower
Nov 15 '18 at 10:33
Thanks for that. However, when I enter 80, it is returning 90 and I am unsure as to why if you could help.
– WhiteFlower
Nov 15 '18 at 10:33
2
2
Note:
hundreds
list also has the same problem...– U9-Forward
Nov 15 '18 at 10:33
Note:
hundreds
list also has the same problem...– U9-Forward
Nov 15 '18 at 10:33
@WhiteFlower Use: word = decades[i-1] + word
– Ernest S Kirubakaran
Nov 15 '18 at 10:36
@WhiteFlower Use: word = decades[i-1] + word
– Ernest S Kirubakaran
Nov 15 '18 at 10:36
@U9-Forward updated my answer
– Ernest S Kirubakaran
Nov 15 '18 at 10:38
@U9-Forward updated my answer
– Ernest S Kirubakaran
Nov 15 '18 at 10:38
@ErnestSKirubakaran Thanks, I have updated the comma mistakes. I have tried implementing this, however when i enter 95 it returns "five".
– WhiteFlower
Nov 15 '18 at 10:40
@ErnestSKirubakaran Thanks, I have updated the comma mistakes. I have tried implementing this, however when i enter 95 it returns "five".
– WhiteFlower
Nov 15 '18 at 10:40
|
show 1 more comment
I think that for making your example working you just need to make sure that each change
digit is accessing the correct value in your lists. That's not the case for your decades
lists, where you missed a blank string for the teens, that you're correctly treating as a separate case.
So to make your code work just change:
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety "]
to this:
decades = ["", "", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety "]
add a comment |
I think that for making your example working you just need to make sure that each change
digit is accessing the correct value in your lists. That's not the case for your decades
lists, where you missed a blank string for the teens, that you're correctly treating as a separate case.
So to make your code work just change:
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety "]
to this:
decades = ["", "", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety "]
add a comment |
I think that for making your example working you just need to make sure that each change
digit is accessing the correct value in your lists. That's not the case for your decades
lists, where you missed a blank string for the teens, that you're correctly treating as a separate case.
So to make your code work just change:
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety "]
to this:
decades = ["", "", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety "]
I think that for making your example working you just need to make sure that each change
digit is accessing the correct value in your lists. That's not the case for your decades
lists, where you missed a blank string for the teens, that you're correctly treating as a separate case.
So to make your code work just change:
decades = ["", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety "]
to this:
decades = ["", "", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy", "eighty ", "ninety "]
answered Nov 15 '18 at 11:01
toti08toti08
1,80941623
1,80941623
add a comment |
add a comment |
For this implementation, you should always have 10 elements in the lists. For example, in decades you should have some string representing the values between 10-20 even if its not used because the indexing of the array would be wrong otherwise.
Still this code has a lot of mistakes.
Try this.
number = input("Enter a value: ")
ones = ["", "one ", "two ","three ", "four", "five ", "six ", "seven ", "eight ", "nine "]
teens = ["ten ", "eleven ", "twelve ", "thirteen ", "fourteen ", "fifteen ", "sixteen ", "seventeen ", "eighteen ", "nineteen "]
decades = ["", "onety", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy ", "eighty ", "ninety "]
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred", "nine hundred"]
word = ""
change = len(number)
while change > 0:
if number == "0":
word ="zero"
break
elif change > 1 and number[change - 2] == "1":
for i in range(0,10):
if number[change - 1] == str(i):
word = teens[i] + word
if change == 2:
break
else:
for i in range(0,10):
if number[change - 1] == str(i):
word = ones[i] + word
if change > 1 and number[change-2]!="1":
for i in range(0,10):
if number[change - 2] == str(i):
word = decades[i] + word
if change > 2:
for i in range(0,10):
if number[change - 3] == str(i):
word = hundreds[i] + word
change = change - 3
print(word)
add a comment |
For this implementation, you should always have 10 elements in the lists. For example, in decades you should have some string representing the values between 10-20 even if its not used because the indexing of the array would be wrong otherwise.
Still this code has a lot of mistakes.
Try this.
number = input("Enter a value: ")
ones = ["", "one ", "two ","three ", "four", "five ", "six ", "seven ", "eight ", "nine "]
teens = ["ten ", "eleven ", "twelve ", "thirteen ", "fourteen ", "fifteen ", "sixteen ", "seventeen ", "eighteen ", "nineteen "]
decades = ["", "onety", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy ", "eighty ", "ninety "]
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred", "nine hundred"]
word = ""
change = len(number)
while change > 0:
if number == "0":
word ="zero"
break
elif change > 1 and number[change - 2] == "1":
for i in range(0,10):
if number[change - 1] == str(i):
word = teens[i] + word
if change == 2:
break
else:
for i in range(0,10):
if number[change - 1] == str(i):
word = ones[i] + word
if change > 1 and number[change-2]!="1":
for i in range(0,10):
if number[change - 2] == str(i):
word = decades[i] + word
if change > 2:
for i in range(0,10):
if number[change - 3] == str(i):
word = hundreds[i] + word
change = change - 3
print(word)
add a comment |
For this implementation, you should always have 10 elements in the lists. For example, in decades you should have some string representing the values between 10-20 even if its not used because the indexing of the array would be wrong otherwise.
Still this code has a lot of mistakes.
Try this.
number = input("Enter a value: ")
ones = ["", "one ", "two ","three ", "four", "five ", "six ", "seven ", "eight ", "nine "]
teens = ["ten ", "eleven ", "twelve ", "thirteen ", "fourteen ", "fifteen ", "sixteen ", "seventeen ", "eighteen ", "nineteen "]
decades = ["", "onety", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy ", "eighty ", "ninety "]
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred", "nine hundred"]
word = ""
change = len(number)
while change > 0:
if number == "0":
word ="zero"
break
elif change > 1 and number[change - 2] == "1":
for i in range(0,10):
if number[change - 1] == str(i):
word = teens[i] + word
if change == 2:
break
else:
for i in range(0,10):
if number[change - 1] == str(i):
word = ones[i] + word
if change > 1 and number[change-2]!="1":
for i in range(0,10):
if number[change - 2] == str(i):
word = decades[i] + word
if change > 2:
for i in range(0,10):
if number[change - 3] == str(i):
word = hundreds[i] + word
change = change - 3
print(word)
For this implementation, you should always have 10 elements in the lists. For example, in decades you should have some string representing the values between 10-20 even if its not used because the indexing of the array would be wrong otherwise.
Still this code has a lot of mistakes.
Try this.
number = input("Enter a value: ")
ones = ["", "one ", "two ","three ", "four", "five ", "six ", "seven ", "eight ", "nine "]
teens = ["ten ", "eleven ", "twelve ", "thirteen ", "fourteen ", "fifteen ", "sixteen ", "seventeen ", "eighteen ", "nineteen "]
decades = ["", "onety", "twenty ", "thirty ", "forty ", "fifty ", "sixty ", "seventy ", "eighty ", "ninety "]
hundreds = ["", "one hundred ", "two hundred ", "three hundred ", "four hundred ", "five hundred", "six hundred ", "seven hundred", "eight hundred", "nine hundred"]
word = ""
change = len(number)
while change > 0:
if number == "0":
word ="zero"
break
elif change > 1 and number[change - 2] == "1":
for i in range(0,10):
if number[change - 1] == str(i):
word = teens[i] + word
if change == 2:
break
else:
for i in range(0,10):
if number[change - 1] == str(i):
word = ones[i] + word
if change > 1 and number[change-2]!="1":
for i in range(0,10):
if number[change - 2] == str(i):
word = decades[i] + word
if change > 2:
for i in range(0,10):
if number[change - 3] == str(i):
word = hundreds[i] + word
change = change - 3
print(word)
answered Nov 15 '18 at 11:03


RakihthaRRRakihthaRR
304313
304313
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53317174%2fhow-to-convert-numbers-to-words-python-3%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uqAxfYey1,o 753CCh82bFlqkXd,ihoelbKP