Post file array with PHP curl
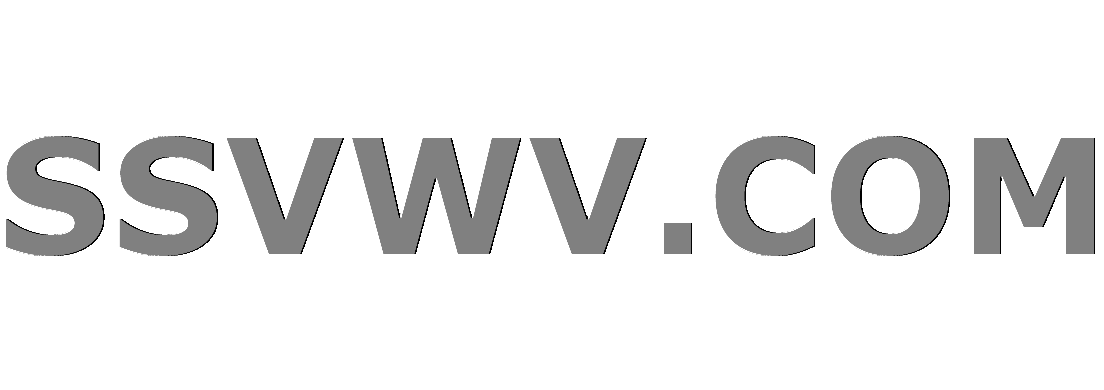
Multi tool use
up vote
0
down vote
favorite
I want to post an array of files to a server using PHP and curl. In HTML it looks as follows:
<form method="POST" type="multipart/form-data">
<input type="text" name="fieldx" value="123"/>
<input type="file" name="localfile"/>
<input type="file" name="localfile"/>
</form>
The same should be done with CURL:
$postParameters['fieldx'] = "123";
$postParameters['localfile'] = array("fulllocalfilepath1", "fulllocalfilepath2");
$request = curl_init('http://server.abc');
curl_setopt($request, CURLOPT_POST, true);
curl_setopt(
$request,
CURLOPT_POSTFIELDS,
$postParameters
);
curl_setopt($request, CURLOPT_RETURNTRANSFER, true);
$rawData = curl_exec($request);
curl_close($request);
But the server does not receive the localfile
array when using CURL. What is the correct command for sending the files?
Regards,
php curl http-post multipartform-data php-curl
add a comment |
up vote
0
down vote
favorite
I want to post an array of files to a server using PHP and curl. In HTML it looks as follows:
<form method="POST" type="multipart/form-data">
<input type="text" name="fieldx" value="123"/>
<input type="file" name="localfile"/>
<input type="file" name="localfile"/>
</form>
The same should be done with CURL:
$postParameters['fieldx'] = "123";
$postParameters['localfile'] = array("fulllocalfilepath1", "fulllocalfilepath2");
$request = curl_init('http://server.abc');
curl_setopt($request, CURLOPT_POST, true);
curl_setopt(
$request,
CURLOPT_POSTFIELDS,
$postParameters
);
curl_setopt($request, CURLOPT_RETURNTRANSFER, true);
$rawData = curl_exec($request);
curl_close($request);
But the server does not receive the localfile
array when using CURL. What is the correct command for sending the files?
Regards,
php curl http-post multipartform-data php-curl
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I want to post an array of files to a server using PHP and curl. In HTML it looks as follows:
<form method="POST" type="multipart/form-data">
<input type="text" name="fieldx" value="123"/>
<input type="file" name="localfile"/>
<input type="file" name="localfile"/>
</form>
The same should be done with CURL:
$postParameters['fieldx'] = "123";
$postParameters['localfile'] = array("fulllocalfilepath1", "fulllocalfilepath2");
$request = curl_init('http://server.abc');
curl_setopt($request, CURLOPT_POST, true);
curl_setopt(
$request,
CURLOPT_POSTFIELDS,
$postParameters
);
curl_setopt($request, CURLOPT_RETURNTRANSFER, true);
$rawData = curl_exec($request);
curl_close($request);
But the server does not receive the localfile
array when using CURL. What is the correct command for sending the files?
Regards,
php curl http-post multipartform-data php-curl
I want to post an array of files to a server using PHP and curl. In HTML it looks as follows:
<form method="POST" type="multipart/form-data">
<input type="text" name="fieldx" value="123"/>
<input type="file" name="localfile"/>
<input type="file" name="localfile"/>
</form>
The same should be done with CURL:
$postParameters['fieldx'] = "123";
$postParameters['localfile'] = array("fulllocalfilepath1", "fulllocalfilepath2");
$request = curl_init('http://server.abc');
curl_setopt($request, CURLOPT_POST, true);
curl_setopt(
$request,
CURLOPT_POSTFIELDS,
$postParameters
);
curl_setopt($request, CURLOPT_RETURNTRANSFER, true);
$rawData = curl_exec($request);
curl_close($request);
But the server does not receive the localfile
array when using CURL. What is the correct command for sending the files?
Regards,
php curl http-post multipartform-data php-curl
php curl http-post multipartform-data php-curl
asked 2 days ago
Hyndrix
1,71052244
1,71052244
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
Got the solution:
$postParameters['fieldx'] = "123";
$postParameters['localfile[0]'] = new cURLFile("fulllocalfilepath1", "mime", "readable name 1");
$postParameters['localfile[1]'] = new cURLFile("fulllocalfilepath2", "mime", "readable name 2");
$request = curl_init('http://server.abc');
curl_setopt($request, CURLOPT_POST, true);
curl_setopt(
$request,
CURLOPT_POSTFIELDS,
$postParameters
);
curl_setopt($request, CURLOPT_RETURNTRANSFER, true);
$rawData = curl_exec($request);
curl_close($request);
you can also do$postParameters['localfile']=>array((new cURLFile("fulllocalfilepath1", "mime", "readable name 1")), (new cURLFile("fulllocalfilepath2", "mime", "readable name 2")));
– hanshenrik
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Got the solution:
$postParameters['fieldx'] = "123";
$postParameters['localfile[0]'] = new cURLFile("fulllocalfilepath1", "mime", "readable name 1");
$postParameters['localfile[1]'] = new cURLFile("fulllocalfilepath2", "mime", "readable name 2");
$request = curl_init('http://server.abc');
curl_setopt($request, CURLOPT_POST, true);
curl_setopt(
$request,
CURLOPT_POSTFIELDS,
$postParameters
);
curl_setopt($request, CURLOPT_RETURNTRANSFER, true);
$rawData = curl_exec($request);
curl_close($request);
you can also do$postParameters['localfile']=>array((new cURLFile("fulllocalfilepath1", "mime", "readable name 1")), (new cURLFile("fulllocalfilepath2", "mime", "readable name 2")));
– hanshenrik
2 days ago
add a comment |
up vote
0
down vote
Got the solution:
$postParameters['fieldx'] = "123";
$postParameters['localfile[0]'] = new cURLFile("fulllocalfilepath1", "mime", "readable name 1");
$postParameters['localfile[1]'] = new cURLFile("fulllocalfilepath2", "mime", "readable name 2");
$request = curl_init('http://server.abc');
curl_setopt($request, CURLOPT_POST, true);
curl_setopt(
$request,
CURLOPT_POSTFIELDS,
$postParameters
);
curl_setopt($request, CURLOPT_RETURNTRANSFER, true);
$rawData = curl_exec($request);
curl_close($request);
you can also do$postParameters['localfile']=>array((new cURLFile("fulllocalfilepath1", "mime", "readable name 1")), (new cURLFile("fulllocalfilepath2", "mime", "readable name 2")));
– hanshenrik
2 days ago
add a comment |
up vote
0
down vote
up vote
0
down vote
Got the solution:
$postParameters['fieldx'] = "123";
$postParameters['localfile[0]'] = new cURLFile("fulllocalfilepath1", "mime", "readable name 1");
$postParameters['localfile[1]'] = new cURLFile("fulllocalfilepath2", "mime", "readable name 2");
$request = curl_init('http://server.abc');
curl_setopt($request, CURLOPT_POST, true);
curl_setopt(
$request,
CURLOPT_POSTFIELDS,
$postParameters
);
curl_setopt($request, CURLOPT_RETURNTRANSFER, true);
$rawData = curl_exec($request);
curl_close($request);
Got the solution:
$postParameters['fieldx'] = "123";
$postParameters['localfile[0]'] = new cURLFile("fulllocalfilepath1", "mime", "readable name 1");
$postParameters['localfile[1]'] = new cURLFile("fulllocalfilepath2", "mime", "readable name 2");
$request = curl_init('http://server.abc');
curl_setopt($request, CURLOPT_POST, true);
curl_setopt(
$request,
CURLOPT_POSTFIELDS,
$postParameters
);
curl_setopt($request, CURLOPT_RETURNTRANSFER, true);
$rawData = curl_exec($request);
curl_close($request);
answered 2 days ago
Hyndrix
1,71052244
1,71052244
you can also do$postParameters['localfile']=>array((new cURLFile("fulllocalfilepath1", "mime", "readable name 1")), (new cURLFile("fulllocalfilepath2", "mime", "readable name 2")));
– hanshenrik
2 days ago
add a comment |
you can also do$postParameters['localfile']=>array((new cURLFile("fulllocalfilepath1", "mime", "readable name 1")), (new cURLFile("fulllocalfilepath2", "mime", "readable name 2")));
– hanshenrik
2 days ago
you can also do
$postParameters['localfile']=>array((new cURLFile("fulllocalfilepath1", "mime", "readable name 1")), (new cURLFile("fulllocalfilepath2", "mime", "readable name 2")));
– hanshenrik
2 days ago
you can also do
$postParameters['localfile']=>array((new cURLFile("fulllocalfilepath1", "mime", "readable name 1")), (new cURLFile("fulllocalfilepath2", "mime", "readable name 2")));
– hanshenrik
2 days ago
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53225179%2fpost-file-array-with-php-curl%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
kPgdK,4id6w,RNpw lD,UiOi8TXvV 6,Ygz lmIKdk6QBv,M Id Pb,z95DLSZaAsuMJMcSI1,lbX 37qwzwEKJK