Split an array in python using indexes from a list
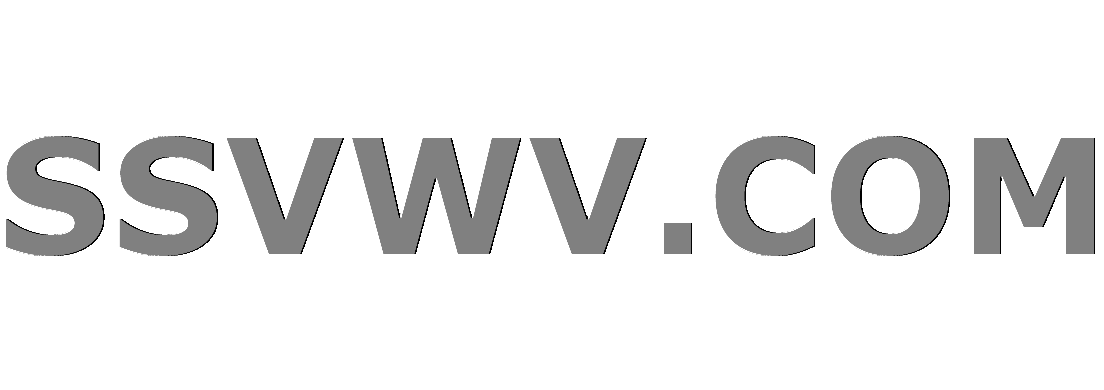
Multi tool use
up vote
-2
down vote
favorite
I have a 2d array of size 3 by 7 in numpy:
[[1 2 3 4 5 6 7]
[4 5 6 7 8 9 0]
[2 3 4 5 6 7 8]]
I also have a list that contains indexes of splitting points:
[1, 3]
Now, I want to split the array using the indexes in the list such that I get:
[[1 2]
[4 5]
[2 3]]
[[ 2 3 4]
[5 6 7]
[3 4 5]]
[[ 4 5 6 7]
[7 8 9 0]
[5 6 7 8]]
How can I do this in python?
python python-3.x list numpy indexing
add a comment |
up vote
-2
down vote
favorite
I have a 2d array of size 3 by 7 in numpy:
[[1 2 3 4 5 6 7]
[4 5 6 7 8 9 0]
[2 3 4 5 6 7 8]]
I also have a list that contains indexes of splitting points:
[1, 3]
Now, I want to split the array using the indexes in the list such that I get:
[[1 2]
[4 5]
[2 3]]
[[ 2 3 4]
[5 6 7]
[3 4 5]]
[[ 4 5 6 7]
[7 8 9 0]
[5 6 7 8]]
How can I do this in python?
python python-3.x list numpy indexing
add a comment |
up vote
-2
down vote
favorite
up vote
-2
down vote
favorite
I have a 2d array of size 3 by 7 in numpy:
[[1 2 3 4 5 6 7]
[4 5 6 7 8 9 0]
[2 3 4 5 6 7 8]]
I also have a list that contains indexes of splitting points:
[1, 3]
Now, I want to split the array using the indexes in the list such that I get:
[[1 2]
[4 5]
[2 3]]
[[ 2 3 4]
[5 6 7]
[3 4 5]]
[[ 4 5 6 7]
[7 8 9 0]
[5 6 7 8]]
How can I do this in python?
python python-3.x list numpy indexing
I have a 2d array of size 3 by 7 in numpy:
[[1 2 3 4 5 6 7]
[4 5 6 7 8 9 0]
[2 3 4 5 6 7 8]]
I also have a list that contains indexes of splitting points:
[1, 3]
Now, I want to split the array using the indexes in the list such that I get:
[[1 2]
[4 5]
[2 3]]
[[ 2 3 4]
[5 6 7]
[3 4 5]]
[[ 4 5 6 7]
[7 8 9 0]
[5 6 7 8]]
How can I do this in python?
python python-3.x list numpy indexing
python python-3.x list numpy indexing
edited 2 days ago


jpp
79.8k184695
79.8k184695
asked 2 days ago


mrbright
224
224
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
You can use a list comprehension with slicing, using zip
to extract indices pairwise.
A = np.array([[1, 2, 3, 4, 5, 6, 7],
[4, 5, 6, 7, 8, 9, 0],
[2, 3, 4, 5, 6, 7, 8]])
idx = [1, 3]
idx = [0] + idx + [A.shape[1]]
res = [A[:, start: end+1] for start, end in zip(idx, idx[1:])]
print(*res, sep='n'*2)
[[1 2]
[4 5]
[2 3]]
[[2 3 4]
[5 6 7]
[3 4 5]]
[[4 5 6 7]
[7 8 9 0]
[5 6 7 8]]
Thank you @jpp, It worked
– mrbright
2 days ago
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
You can use a list comprehension with slicing, using zip
to extract indices pairwise.
A = np.array([[1, 2, 3, 4, 5, 6, 7],
[4, 5, 6, 7, 8, 9, 0],
[2, 3, 4, 5, 6, 7, 8]])
idx = [1, 3]
idx = [0] + idx + [A.shape[1]]
res = [A[:, start: end+1] for start, end in zip(idx, idx[1:])]
print(*res, sep='n'*2)
[[1 2]
[4 5]
[2 3]]
[[2 3 4]
[5 6 7]
[3 4 5]]
[[4 5 6 7]
[7 8 9 0]
[5 6 7 8]]
Thank you @jpp, It worked
– mrbright
2 days ago
add a comment |
up vote
2
down vote
accepted
You can use a list comprehension with slicing, using zip
to extract indices pairwise.
A = np.array([[1, 2, 3, 4, 5, 6, 7],
[4, 5, 6, 7, 8, 9, 0],
[2, 3, 4, 5, 6, 7, 8]])
idx = [1, 3]
idx = [0] + idx + [A.shape[1]]
res = [A[:, start: end+1] for start, end in zip(idx, idx[1:])]
print(*res, sep='n'*2)
[[1 2]
[4 5]
[2 3]]
[[2 3 4]
[5 6 7]
[3 4 5]]
[[4 5 6 7]
[7 8 9 0]
[5 6 7 8]]
Thank you @jpp, It worked
– mrbright
2 days ago
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
You can use a list comprehension with slicing, using zip
to extract indices pairwise.
A = np.array([[1, 2, 3, 4, 5, 6, 7],
[4, 5, 6, 7, 8, 9, 0],
[2, 3, 4, 5, 6, 7, 8]])
idx = [1, 3]
idx = [0] + idx + [A.shape[1]]
res = [A[:, start: end+1] for start, end in zip(idx, idx[1:])]
print(*res, sep='n'*2)
[[1 2]
[4 5]
[2 3]]
[[2 3 4]
[5 6 7]
[3 4 5]]
[[4 5 6 7]
[7 8 9 0]
[5 6 7 8]]
You can use a list comprehension with slicing, using zip
to extract indices pairwise.
A = np.array([[1, 2, 3, 4, 5, 6, 7],
[4, 5, 6, 7, 8, 9, 0],
[2, 3, 4, 5, 6, 7, 8]])
idx = [1, 3]
idx = [0] + idx + [A.shape[1]]
res = [A[:, start: end+1] for start, end in zip(idx, idx[1:])]
print(*res, sep='n'*2)
[[1 2]
[4 5]
[2 3]]
[[2 3 4]
[5 6 7]
[3 4 5]]
[[4 5 6 7]
[7 8 9 0]
[5 6 7 8]]
answered 2 days ago


jpp
79.8k184695
79.8k184695
Thank you @jpp, It worked
– mrbright
2 days ago
add a comment |
Thank you @jpp, It worked
– mrbright
2 days ago
Thank you @jpp, It worked
– mrbright
2 days ago
Thank you @jpp, It worked
– mrbright
2 days ago
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53225184%2fsplit-an-array-in-python-using-indexes-from-a-list%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
yyx2 CeNE8tYDoocPwngkzulV0 BnjaJ76my SzObbd8EkDVwbxjmK0oiXmVjf