Understanding the symbols in scheme
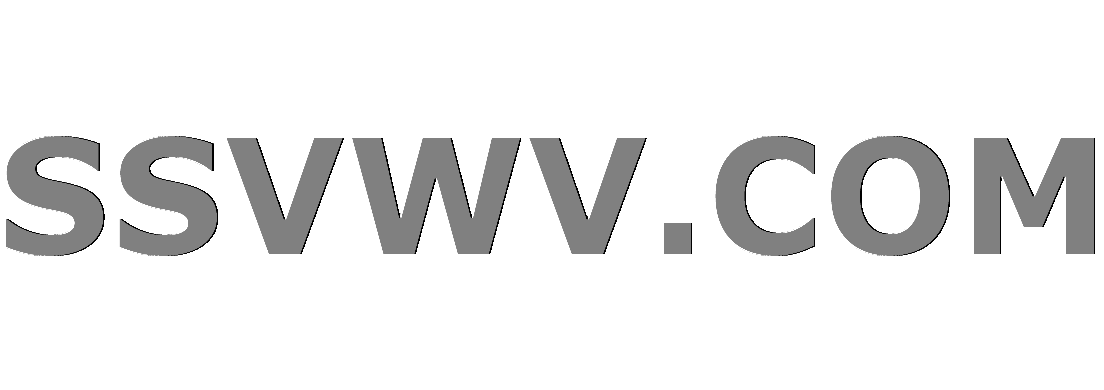
Multi tool use
up vote
-1
down vote
favorite
Can you help me understand why there are 7 symbols (including duplicates) in the value of the following expression ?
'('a ',(string->symbol "b") 'c))
scheme
add a comment |
up vote
-1
down vote
favorite
Can you help me understand why there are 7 symbols (including duplicates) in the value of the following expression ?
'('a ',(string->symbol "b") 'c))
scheme
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
Can you help me understand why there are 7 symbols (including duplicates) in the value of the following expression ?
'('a ',(string->symbol "b") 'c))
scheme
Can you help me understand why there are 7 symbols (including duplicates) in the value of the following expression ?
'('a ',(string->symbol "b") 'c))
scheme
scheme
edited Nov 9 at 20:36
Graham
3,316123558
3,316123558
asked Nov 9 at 19:42
Shir Cohen
6
6
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
You can write a function that checks whether elements are symbols.
Pair the element with its "symbolness" for clarity:
(define (sym-check ls)
(cond ((null? ls) '())
((not (pair? ls)) (cons ls (symbol? ls)))
((pair? (car ls)) (cons (map sym-check (car ls)) (sym-check (cdr ls))))
(else (cons (sym-check (car ls)) (sym-check (cdr ls))))))
> (sym-check '('a ',(string->symbol "b") 'c))
'(((quote . #t) (a . #t)) ((quote . #t)
((unquote . #t)
((string->symbol . #t) ("b" . #f))))
((quote . #t) (c . #t)))
and you get seven #t
s.
Note that'
and ,
are "shorthand" for the symbols quote
and unquote
,
> (quote (unquote (string->symbol "b")))
',(string->symbol "b")
and that using list
instead of quoting gives a very different result:
> (sym-check (list 'a ',(string->symbol "b") 'c))
'((a . #t) ((unquote . #t) ((string->symbol . #t) ("b" . #f))) (c . #t))
which is the same as leaving quoting out of the quoted list:
> (sym-check '(a ,(string->symbol "b") c))
'((a . #t) ((unquote . #t) ((string->symbol . #t) ("b" . #f))) (c . #t))
add a comment |
up vote
1
down vote
'('a ',(string->symbol "b") 'c))
evaluates to the following structure:
((quote a)
(quote (unquote (string->symbol "b")))
(quote c))
I can count 7 so yes. It is 7 symbols in the result of evaluating the quoted expression.
Scheme system has a reader that translates 'x
to (quote x)
and ,
, `
, and ,@
corresponds to forms with quasiquote
, unquote
, and unquote-splicing
. That means that the code `(a b c ,d e f)
becomes (quasiquote (a b c (unquote d) e f))
. Then the macos in the implementation translates it into (list* 'a 'b 'c d '(e f))
or a similar expression that does the same. However with '`(a b c ,d e f)
just becomes the value (quasiquote (a b c (unquote d) e f))
since that is the expression that was quoted. Scheme doesn't expand into normal quote expression.
Usually quote characters inside quoted datum is a bug. Beginners doesn't understand the purpose and thin it needs to be everywhere. But really you only need the outer quote. (+ 4 5)
is 9
and '(+ 4 5)
is (+ 4 5)
. See the difference?
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
You can write a function that checks whether elements are symbols.
Pair the element with its "symbolness" for clarity:
(define (sym-check ls)
(cond ((null? ls) '())
((not (pair? ls)) (cons ls (symbol? ls)))
((pair? (car ls)) (cons (map sym-check (car ls)) (sym-check (cdr ls))))
(else (cons (sym-check (car ls)) (sym-check (cdr ls))))))
> (sym-check '('a ',(string->symbol "b") 'c))
'(((quote . #t) (a . #t)) ((quote . #t)
((unquote . #t)
((string->symbol . #t) ("b" . #f))))
((quote . #t) (c . #t)))
and you get seven #t
s.
Note that'
and ,
are "shorthand" for the symbols quote
and unquote
,
> (quote (unquote (string->symbol "b")))
',(string->symbol "b")
and that using list
instead of quoting gives a very different result:
> (sym-check (list 'a ',(string->symbol "b") 'c))
'((a . #t) ((unquote . #t) ((string->symbol . #t) ("b" . #f))) (c . #t))
which is the same as leaving quoting out of the quoted list:
> (sym-check '(a ,(string->symbol "b") c))
'((a . #t) ((unquote . #t) ((string->symbol . #t) ("b" . #f))) (c . #t))
add a comment |
up vote
1
down vote
You can write a function that checks whether elements are symbols.
Pair the element with its "symbolness" for clarity:
(define (sym-check ls)
(cond ((null? ls) '())
((not (pair? ls)) (cons ls (symbol? ls)))
((pair? (car ls)) (cons (map sym-check (car ls)) (sym-check (cdr ls))))
(else (cons (sym-check (car ls)) (sym-check (cdr ls))))))
> (sym-check '('a ',(string->symbol "b") 'c))
'(((quote . #t) (a . #t)) ((quote . #t)
((unquote . #t)
((string->symbol . #t) ("b" . #f))))
((quote . #t) (c . #t)))
and you get seven #t
s.
Note that'
and ,
are "shorthand" for the symbols quote
and unquote
,
> (quote (unquote (string->symbol "b")))
',(string->symbol "b")
and that using list
instead of quoting gives a very different result:
> (sym-check (list 'a ',(string->symbol "b") 'c))
'((a . #t) ((unquote . #t) ((string->symbol . #t) ("b" . #f))) (c . #t))
which is the same as leaving quoting out of the quoted list:
> (sym-check '(a ,(string->symbol "b") c))
'((a . #t) ((unquote . #t) ((string->symbol . #t) ("b" . #f))) (c . #t))
add a comment |
up vote
1
down vote
up vote
1
down vote
You can write a function that checks whether elements are symbols.
Pair the element with its "symbolness" for clarity:
(define (sym-check ls)
(cond ((null? ls) '())
((not (pair? ls)) (cons ls (symbol? ls)))
((pair? (car ls)) (cons (map sym-check (car ls)) (sym-check (cdr ls))))
(else (cons (sym-check (car ls)) (sym-check (cdr ls))))))
> (sym-check '('a ',(string->symbol "b") 'c))
'(((quote . #t) (a . #t)) ((quote . #t)
((unquote . #t)
((string->symbol . #t) ("b" . #f))))
((quote . #t) (c . #t)))
and you get seven #t
s.
Note that'
and ,
are "shorthand" for the symbols quote
and unquote
,
> (quote (unquote (string->symbol "b")))
',(string->symbol "b")
and that using list
instead of quoting gives a very different result:
> (sym-check (list 'a ',(string->symbol "b") 'c))
'((a . #t) ((unquote . #t) ((string->symbol . #t) ("b" . #f))) (c . #t))
which is the same as leaving quoting out of the quoted list:
> (sym-check '(a ,(string->symbol "b") c))
'((a . #t) ((unquote . #t) ((string->symbol . #t) ("b" . #f))) (c . #t))
You can write a function that checks whether elements are symbols.
Pair the element with its "symbolness" for clarity:
(define (sym-check ls)
(cond ((null? ls) '())
((not (pair? ls)) (cons ls (symbol? ls)))
((pair? (car ls)) (cons (map sym-check (car ls)) (sym-check (cdr ls))))
(else (cons (sym-check (car ls)) (sym-check (cdr ls))))))
> (sym-check '('a ',(string->symbol "b") 'c))
'(((quote . #t) (a . #t)) ((quote . #t)
((unquote . #t)
((string->symbol . #t) ("b" . #f))))
((quote . #t) (c . #t)))
and you get seven #t
s.
Note that'
and ,
are "shorthand" for the symbols quote
and unquote
,
> (quote (unquote (string->symbol "b")))
',(string->symbol "b")
and that using list
instead of quoting gives a very different result:
> (sym-check (list 'a ',(string->symbol "b") 'c))
'((a . #t) ((unquote . #t) ((string->symbol . #t) ("b" . #f))) (c . #t))
which is the same as leaving quoting out of the quoted list:
> (sym-check '(a ,(string->symbol "b") c))
'((a . #t) ((unquote . #t) ((string->symbol . #t) ("b" . #f))) (c . #t))
answered Nov 9 at 21:55


molbdnilo
39.8k32152
39.8k32152
add a comment |
add a comment |
up vote
1
down vote
'('a ',(string->symbol "b") 'c))
evaluates to the following structure:
((quote a)
(quote (unquote (string->symbol "b")))
(quote c))
I can count 7 so yes. It is 7 symbols in the result of evaluating the quoted expression.
Scheme system has a reader that translates 'x
to (quote x)
and ,
, `
, and ,@
corresponds to forms with quasiquote
, unquote
, and unquote-splicing
. That means that the code `(a b c ,d e f)
becomes (quasiquote (a b c (unquote d) e f))
. Then the macos in the implementation translates it into (list* 'a 'b 'c d '(e f))
or a similar expression that does the same. However with '`(a b c ,d e f)
just becomes the value (quasiquote (a b c (unquote d) e f))
since that is the expression that was quoted. Scheme doesn't expand into normal quote expression.
Usually quote characters inside quoted datum is a bug. Beginners doesn't understand the purpose and thin it needs to be everywhere. But really you only need the outer quote. (+ 4 5)
is 9
and '(+ 4 5)
is (+ 4 5)
. See the difference?
add a comment |
up vote
1
down vote
'('a ',(string->symbol "b") 'c))
evaluates to the following structure:
((quote a)
(quote (unquote (string->symbol "b")))
(quote c))
I can count 7 so yes. It is 7 symbols in the result of evaluating the quoted expression.
Scheme system has a reader that translates 'x
to (quote x)
and ,
, `
, and ,@
corresponds to forms with quasiquote
, unquote
, and unquote-splicing
. That means that the code `(a b c ,d e f)
becomes (quasiquote (a b c (unquote d) e f))
. Then the macos in the implementation translates it into (list* 'a 'b 'c d '(e f))
or a similar expression that does the same. However with '`(a b c ,d e f)
just becomes the value (quasiquote (a b c (unquote d) e f))
since that is the expression that was quoted. Scheme doesn't expand into normal quote expression.
Usually quote characters inside quoted datum is a bug. Beginners doesn't understand the purpose and thin it needs to be everywhere. But really you only need the outer quote. (+ 4 5)
is 9
and '(+ 4 5)
is (+ 4 5)
. See the difference?
add a comment |
up vote
1
down vote
up vote
1
down vote
'('a ',(string->symbol "b") 'c))
evaluates to the following structure:
((quote a)
(quote (unquote (string->symbol "b")))
(quote c))
I can count 7 so yes. It is 7 symbols in the result of evaluating the quoted expression.
Scheme system has a reader that translates 'x
to (quote x)
and ,
, `
, and ,@
corresponds to forms with quasiquote
, unquote
, and unquote-splicing
. That means that the code `(a b c ,d e f)
becomes (quasiquote (a b c (unquote d) e f))
. Then the macos in the implementation translates it into (list* 'a 'b 'c d '(e f))
or a similar expression that does the same. However with '`(a b c ,d e f)
just becomes the value (quasiquote (a b c (unquote d) e f))
since that is the expression that was quoted. Scheme doesn't expand into normal quote expression.
Usually quote characters inside quoted datum is a bug. Beginners doesn't understand the purpose and thin it needs to be everywhere. But really you only need the outer quote. (+ 4 5)
is 9
and '(+ 4 5)
is (+ 4 5)
. See the difference?
'('a ',(string->symbol "b") 'c))
evaluates to the following structure:
((quote a)
(quote (unquote (string->symbol "b")))
(quote c))
I can count 7 so yes. It is 7 symbols in the result of evaluating the quoted expression.
Scheme system has a reader that translates 'x
to (quote x)
and ,
, `
, and ,@
corresponds to forms with quasiquote
, unquote
, and unquote-splicing
. That means that the code `(a b c ,d e f)
becomes (quasiquote (a b c (unquote d) e f))
. Then the macos in the implementation translates it into (list* 'a 'b 'c d '(e f))
or a similar expression that does the same. However with '`(a b c ,d e f)
just becomes the value (quasiquote (a b c (unquote d) e f))
since that is the expression that was quoted. Scheme doesn't expand into normal quote expression.
Usually quote characters inside quoted datum is a bug. Beginners doesn't understand the purpose and thin it needs to be everywhere. But really you only need the outer quote. (+ 4 5)
is 9
and '(+ 4 5)
is (+ 4 5)
. See the difference?
answered Nov 10 at 0:52
Sylwester
33.5k22854
33.5k22854
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53232308%2funderstanding-the-symbols-in-scheme%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LXeNHZz9AnCrPGIZl,u82uAShCF1nRflfRPu,yANbL GOgITw,h 0H,JfAYTzR,OjVPvyyjSn02j Qml,ePRtoZZ