Laravel 5: How to use 'where' or 'orderBy' using eloquent?
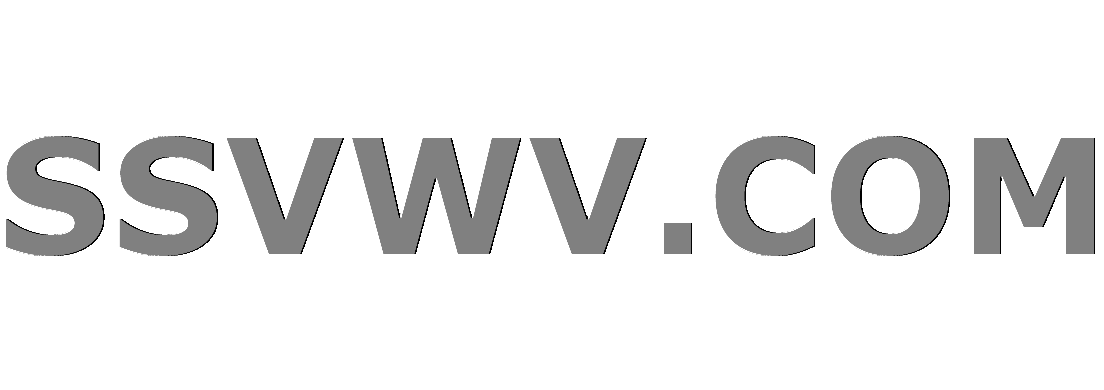
Multi tool use
First things first, this is not a duplicate of Laravel 4: how to "order by" using Eloquent ORM
I have this model User, then I have this other model Idea. I'm writting a view with the details of the user, and I want to post all the ideas the user has suggested. Now, the ideas have a status, the first status is "DRAFT", that means only the user who posted the idea can review it, the idea should not be shown to other users looking at the profile.
Using eloquent I could do something like:
@foreach($user->idea as $idea)
...display details...
@endforeach
My problem is that this approach would cycle through all ideas AND in the order where they were introduced. I can add an @if
to show only what I want, like
@foreach($user->idea as $idea)
@if($idea->status !='DRAFT')
...show the idea...
@endif
@endforeach
But I don't think it's the smart way to do it, I'd like to do something like
$ideas = $user->idea->where('status', '<>', 'DRAFT')
and then cycle through the $ideas
variable, or at least, I'd like somtehing like
$ideas = $user->idea->orderBy('created_at', 'desc')
But I have no idea on how to do it.
php laravel eloquent
add a comment |
First things first, this is not a duplicate of Laravel 4: how to "order by" using Eloquent ORM
I have this model User, then I have this other model Idea. I'm writting a view with the details of the user, and I want to post all the ideas the user has suggested. Now, the ideas have a status, the first status is "DRAFT", that means only the user who posted the idea can review it, the idea should not be shown to other users looking at the profile.
Using eloquent I could do something like:
@foreach($user->idea as $idea)
...display details...
@endforeach
My problem is that this approach would cycle through all ideas AND in the order where they were introduced. I can add an @if
to show only what I want, like
@foreach($user->idea as $idea)
@if($idea->status !='DRAFT')
...show the idea...
@endif
@endforeach
But I don't think it's the smart way to do it, I'd like to do something like
$ideas = $user->idea->where('status', '<>', 'DRAFT')
and then cycle through the $ideas
variable, or at least, I'd like somtehing like
$ideas = $user->idea->orderBy('created_at', 'desc')
But I have no idea on how to do it.
php laravel eloquent
add a comment |
First things first, this is not a duplicate of Laravel 4: how to "order by" using Eloquent ORM
I have this model User, then I have this other model Idea. I'm writting a view with the details of the user, and I want to post all the ideas the user has suggested. Now, the ideas have a status, the first status is "DRAFT", that means only the user who posted the idea can review it, the idea should not be shown to other users looking at the profile.
Using eloquent I could do something like:
@foreach($user->idea as $idea)
...display details...
@endforeach
My problem is that this approach would cycle through all ideas AND in the order where they were introduced. I can add an @if
to show only what I want, like
@foreach($user->idea as $idea)
@if($idea->status !='DRAFT')
...show the idea...
@endif
@endforeach
But I don't think it's the smart way to do it, I'd like to do something like
$ideas = $user->idea->where('status', '<>', 'DRAFT')
and then cycle through the $ideas
variable, or at least, I'd like somtehing like
$ideas = $user->idea->orderBy('created_at', 'desc')
But I have no idea on how to do it.
php laravel eloquent
First things first, this is not a duplicate of Laravel 4: how to "order by" using Eloquent ORM
I have this model User, then I have this other model Idea. I'm writting a view with the details of the user, and I want to post all the ideas the user has suggested. Now, the ideas have a status, the first status is "DRAFT", that means only the user who posted the idea can review it, the idea should not be shown to other users looking at the profile.
Using eloquent I could do something like:
@foreach($user->idea as $idea)
...display details...
@endforeach
My problem is that this approach would cycle through all ideas AND in the order where they were introduced. I can add an @if
to show only what I want, like
@foreach($user->idea as $idea)
@if($idea->status !='DRAFT')
...show the idea...
@endif
@endforeach
But I don't think it's the smart way to do it, I'd like to do something like
$ideas = $user->idea->where('status', '<>', 'DRAFT')
and then cycle through the $ideas
variable, or at least, I'd like somtehing like
$ideas = $user->idea->orderBy('created_at', 'desc')
But I have no idea on how to do it.
php laravel eloquent
php laravel eloquent
asked Nov 14 '18 at 19:05
luisferluisfer
377419
377419
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
You can do it like this:
$ideas = $user->idea()
->where('status', '<>', 'DRAFT')
->orderBy('created_at', 'desc')
->get();
When you use ->idea()
it will start a query.
For more information about how to query a relationship: https://laravel.com/docs/5.7/eloquent-relationships#querying-relations
Thanks a lot! I missed the first () and the get(). Duh!
– luisfer
Nov 14 '18 at 19:37
@luisfer No problem! Have a nice day. :)
– Chin Leung
Nov 14 '18 at 19:38
add a comment |
You can user where clause like this
DB::table('products_to_categories')
->where('categories_id', $id )
->update([
'categories_id' => $unassigned_cat_id
]);
and you can use orderBy clause like this
DB::table('products_to_categories')
->where('categories_id', $id )
->orderBy('subId','DESC');
Thanks! I was looking not to use the query builder, but that's perfect also, thanks!
– luisfer
Nov 19 '18 at 23:36
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53307148%2flaravel-5-how-to-use-where-or-orderby-using-eloquent%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can do it like this:
$ideas = $user->idea()
->where('status', '<>', 'DRAFT')
->orderBy('created_at', 'desc')
->get();
When you use ->idea()
it will start a query.
For more information about how to query a relationship: https://laravel.com/docs/5.7/eloquent-relationships#querying-relations
Thanks a lot! I missed the first () and the get(). Duh!
– luisfer
Nov 14 '18 at 19:37
@luisfer No problem! Have a nice day. :)
– Chin Leung
Nov 14 '18 at 19:38
add a comment |
You can do it like this:
$ideas = $user->idea()
->where('status', '<>', 'DRAFT')
->orderBy('created_at', 'desc')
->get();
When you use ->idea()
it will start a query.
For more information about how to query a relationship: https://laravel.com/docs/5.7/eloquent-relationships#querying-relations
Thanks a lot! I missed the first () and the get(). Duh!
– luisfer
Nov 14 '18 at 19:37
@luisfer No problem! Have a nice day. :)
– Chin Leung
Nov 14 '18 at 19:38
add a comment |
You can do it like this:
$ideas = $user->idea()
->where('status', '<>', 'DRAFT')
->orderBy('created_at', 'desc')
->get();
When you use ->idea()
it will start a query.
For more information about how to query a relationship: https://laravel.com/docs/5.7/eloquent-relationships#querying-relations
You can do it like this:
$ideas = $user->idea()
->where('status', '<>', 'DRAFT')
->orderBy('created_at', 'desc')
->get();
When you use ->idea()
it will start a query.
For more information about how to query a relationship: https://laravel.com/docs/5.7/eloquent-relationships#querying-relations
edited Nov 14 '18 at 19:11
answered Nov 14 '18 at 19:10


Chin LeungChin Leung
7,32521033
7,32521033
Thanks a lot! I missed the first () and the get(). Duh!
– luisfer
Nov 14 '18 at 19:37
@luisfer No problem! Have a nice day. :)
– Chin Leung
Nov 14 '18 at 19:38
add a comment |
Thanks a lot! I missed the first () and the get(). Duh!
– luisfer
Nov 14 '18 at 19:37
@luisfer No problem! Have a nice day. :)
– Chin Leung
Nov 14 '18 at 19:38
Thanks a lot! I missed the first () and the get(). Duh!
– luisfer
Nov 14 '18 at 19:37
Thanks a lot! I missed the first () and the get(). Duh!
– luisfer
Nov 14 '18 at 19:37
@luisfer No problem! Have a nice day. :)
– Chin Leung
Nov 14 '18 at 19:38
@luisfer No problem! Have a nice day. :)
– Chin Leung
Nov 14 '18 at 19:38
add a comment |
You can user where clause like this
DB::table('products_to_categories')
->where('categories_id', $id )
->update([
'categories_id' => $unassigned_cat_id
]);
and you can use orderBy clause like this
DB::table('products_to_categories')
->where('categories_id', $id )
->orderBy('subId','DESC');
Thanks! I was looking not to use the query builder, but that's perfect also, thanks!
– luisfer
Nov 19 '18 at 23:36
add a comment |
You can user where clause like this
DB::table('products_to_categories')
->where('categories_id', $id )
->update([
'categories_id' => $unassigned_cat_id
]);
and you can use orderBy clause like this
DB::table('products_to_categories')
->where('categories_id', $id )
->orderBy('subId','DESC');
Thanks! I was looking not to use the query builder, but that's perfect also, thanks!
– luisfer
Nov 19 '18 at 23:36
add a comment |
You can user where clause like this
DB::table('products_to_categories')
->where('categories_id', $id )
->update([
'categories_id' => $unassigned_cat_id
]);
and you can use orderBy clause like this
DB::table('products_to_categories')
->where('categories_id', $id )
->orderBy('subId','DESC');
You can user where clause like this
DB::table('products_to_categories')
->where('categories_id', $id )
->update([
'categories_id' => $unassigned_cat_id
]);
and you can use orderBy clause like this
DB::table('products_to_categories')
->where('categories_id', $id )
->orderBy('subId','DESC');
answered Nov 15 '18 at 8:45


Zeeshan TanveerZeeshan Tanveer
313
313
Thanks! I was looking not to use the query builder, but that's perfect also, thanks!
– luisfer
Nov 19 '18 at 23:36
add a comment |
Thanks! I was looking not to use the query builder, but that's perfect also, thanks!
– luisfer
Nov 19 '18 at 23:36
Thanks! I was looking not to use the query builder, but that's perfect also, thanks!
– luisfer
Nov 19 '18 at 23:36
Thanks! I was looking not to use the query builder, but that's perfect also, thanks!
– luisfer
Nov 19 '18 at 23:36
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53307148%2flaravel-5-how-to-use-where-or-orderby-using-eloquent%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
dr5T,xNdP,taEZFg