Preventing list elements from being reused in an algorithm
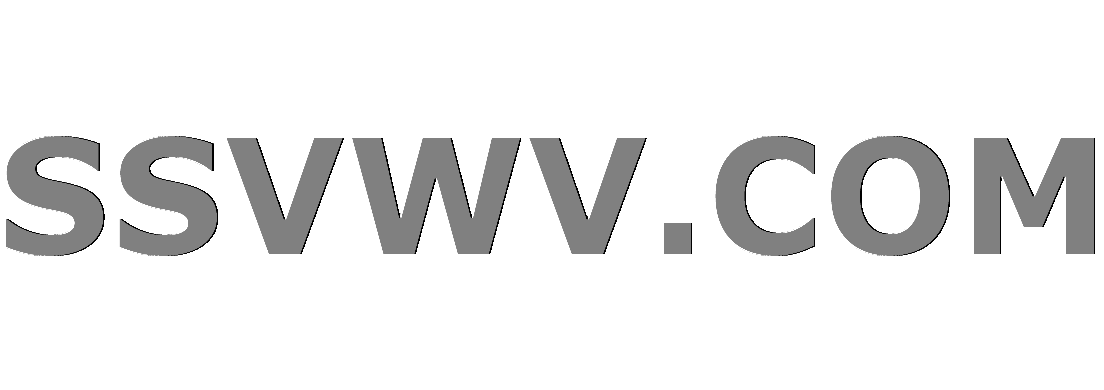
Multi tool use
I;m trying to sort rgb values based on a greedy algorithm that is meant to work by getting a random colour, check the distance between itself and another colour, and then when it finds the one with the shortest distance (euclidean) to itself, append that onto the new array/list.
I'm getting an error concerning the line col[l] not in colouringArray. The point of this line is to make sure colours that are appended to the new list aren't used again.
python
def greedy(cols):
for i in range(len(cols)):
col = [eval(j) for j in cols[i]]
cols[i] = np.array(col)
greedyResult = #what will contain the result
colourArray =
resultIn =
randomColour = random.choice(cols) #make a random choice of colours
colourArray.append(randomColour)
greedyResult.append(colourArray)
colouringArray = greedyResult
resultIn.append(randomColour)
print(colouringArray)
for i in range(25 - 1):
shortestDist = 25
closestColour = ''
for l in range(0,25):
currPosition = colouringArray[-1]
checkPosition = cols[l]
distance = euc_distance(currPosition,checkPosition)
print(colouringArray)
print(colours[l])
if cols[l] not in colouringArray:
if distance < shortestDist:
shortestDist = distance
closestColour = cols[l]
colouringArray.append(closestColour)
print(colouringArray)
return colouringArray
def euc_distance(x, y):
maths = np.sum((x-y)**2)**0.5
print('x', x)
print('y', y)
return maths
Error
if cols[l] not in colouringArray:
ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all()
RGB values being loaded in from just a .txt file
0.9664535356921388 0.4407325991753527 0.007491470058587191
0.9109759624491242 0.939268997363764 0.5822275730589491
0.6715634814879851 0.08393822683708396 0.7664809327917963
0.23680977536311776 0.030814021726609964 0.7887727172362835
0.3460889655971231 0.6232814750391685 0.6158156951036152
0.14855463870828756 0.18309064740993164 0.11441296968868764
0.014618780486909122 0.48675154060475834 0.9649015609162157
0.06456228097718608 0.5410881855511303 0.46589855900830957
0.6014634495610515 0.08892882999066232 0.5790026861873665
0.26958550381944824 0.5564325605562156 0.6446342341782827
0.48103637136651844 0.35523914744298335 0.249152121361209
0.9335154980423467 0.45338801947649354 0.5301612069115903
0.019299566309716853 0.5081019257797922 0.005780237417743139
0.14376842759559538 0.47282692534740633 0.3773474407725964
0.05417519864614284 0.5875285081310694 0.1640032237419612
0.5573302374414681 0.1442457216019083 0.9373070846962247
0.7709799715197749 0.9569331223494054 0.14122776441649953
0.3053927082876986 0.03958962422796164 0.27678369479169207
0.8065125051156038 0.177343035278254 0.15457051471078964
0.9547186557023949 0.154551400089043 0.8338892941512318
0.04106280627180747 0.38618352833582825 0.3495923019636925
0.3417077927504538 0.8164630567142304 0.47593490813172823
0.7828902895224036 0.4708401253088451 0.8173440068581418
0.8815675986556064 0.43959639767963965 0.781063597792481
0.8147411602576974 0.29567891564250737 0.12387667430690597
python
add a comment |
I;m trying to sort rgb values based on a greedy algorithm that is meant to work by getting a random colour, check the distance between itself and another colour, and then when it finds the one with the shortest distance (euclidean) to itself, append that onto the new array/list.
I'm getting an error concerning the line col[l] not in colouringArray. The point of this line is to make sure colours that are appended to the new list aren't used again.
python
def greedy(cols):
for i in range(len(cols)):
col = [eval(j) for j in cols[i]]
cols[i] = np.array(col)
greedyResult = #what will contain the result
colourArray =
resultIn =
randomColour = random.choice(cols) #make a random choice of colours
colourArray.append(randomColour)
greedyResult.append(colourArray)
colouringArray = greedyResult
resultIn.append(randomColour)
print(colouringArray)
for i in range(25 - 1):
shortestDist = 25
closestColour = ''
for l in range(0,25):
currPosition = colouringArray[-1]
checkPosition = cols[l]
distance = euc_distance(currPosition,checkPosition)
print(colouringArray)
print(colours[l])
if cols[l] not in colouringArray:
if distance < shortestDist:
shortestDist = distance
closestColour = cols[l]
colouringArray.append(closestColour)
print(colouringArray)
return colouringArray
def euc_distance(x, y):
maths = np.sum((x-y)**2)**0.5
print('x', x)
print('y', y)
return maths
Error
if cols[l] not in colouringArray:
ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all()
RGB values being loaded in from just a .txt file
0.9664535356921388 0.4407325991753527 0.007491470058587191
0.9109759624491242 0.939268997363764 0.5822275730589491
0.6715634814879851 0.08393822683708396 0.7664809327917963
0.23680977536311776 0.030814021726609964 0.7887727172362835
0.3460889655971231 0.6232814750391685 0.6158156951036152
0.14855463870828756 0.18309064740993164 0.11441296968868764
0.014618780486909122 0.48675154060475834 0.9649015609162157
0.06456228097718608 0.5410881855511303 0.46589855900830957
0.6014634495610515 0.08892882999066232 0.5790026861873665
0.26958550381944824 0.5564325605562156 0.6446342341782827
0.48103637136651844 0.35523914744298335 0.249152121361209
0.9335154980423467 0.45338801947649354 0.5301612069115903
0.019299566309716853 0.5081019257797922 0.005780237417743139
0.14376842759559538 0.47282692534740633 0.3773474407725964
0.05417519864614284 0.5875285081310694 0.1640032237419612
0.5573302374414681 0.1442457216019083 0.9373070846962247
0.7709799715197749 0.9569331223494054 0.14122776441649953
0.3053927082876986 0.03958962422796164 0.27678369479169207
0.8065125051156038 0.177343035278254 0.15457051471078964
0.9547186557023949 0.154551400089043 0.8338892941512318
0.04106280627180747 0.38618352833582825 0.3495923019636925
0.3417077927504538 0.8164630567142304 0.47593490813172823
0.7828902895224036 0.4708401253088451 0.8173440068581418
0.8815675986556064 0.43959639767963965 0.781063597792481
0.8147411602576974 0.29567891564250737 0.12387667430690597
python
add a comment |
I;m trying to sort rgb values based on a greedy algorithm that is meant to work by getting a random colour, check the distance between itself and another colour, and then when it finds the one with the shortest distance (euclidean) to itself, append that onto the new array/list.
I'm getting an error concerning the line col[l] not in colouringArray. The point of this line is to make sure colours that are appended to the new list aren't used again.
python
def greedy(cols):
for i in range(len(cols)):
col = [eval(j) for j in cols[i]]
cols[i] = np.array(col)
greedyResult = #what will contain the result
colourArray =
resultIn =
randomColour = random.choice(cols) #make a random choice of colours
colourArray.append(randomColour)
greedyResult.append(colourArray)
colouringArray = greedyResult
resultIn.append(randomColour)
print(colouringArray)
for i in range(25 - 1):
shortestDist = 25
closestColour = ''
for l in range(0,25):
currPosition = colouringArray[-1]
checkPosition = cols[l]
distance = euc_distance(currPosition,checkPosition)
print(colouringArray)
print(colours[l])
if cols[l] not in colouringArray:
if distance < shortestDist:
shortestDist = distance
closestColour = cols[l]
colouringArray.append(closestColour)
print(colouringArray)
return colouringArray
def euc_distance(x, y):
maths = np.sum((x-y)**2)**0.5
print('x', x)
print('y', y)
return maths
Error
if cols[l] not in colouringArray:
ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all()
RGB values being loaded in from just a .txt file
0.9664535356921388 0.4407325991753527 0.007491470058587191
0.9109759624491242 0.939268997363764 0.5822275730589491
0.6715634814879851 0.08393822683708396 0.7664809327917963
0.23680977536311776 0.030814021726609964 0.7887727172362835
0.3460889655971231 0.6232814750391685 0.6158156951036152
0.14855463870828756 0.18309064740993164 0.11441296968868764
0.014618780486909122 0.48675154060475834 0.9649015609162157
0.06456228097718608 0.5410881855511303 0.46589855900830957
0.6014634495610515 0.08892882999066232 0.5790026861873665
0.26958550381944824 0.5564325605562156 0.6446342341782827
0.48103637136651844 0.35523914744298335 0.249152121361209
0.9335154980423467 0.45338801947649354 0.5301612069115903
0.019299566309716853 0.5081019257797922 0.005780237417743139
0.14376842759559538 0.47282692534740633 0.3773474407725964
0.05417519864614284 0.5875285081310694 0.1640032237419612
0.5573302374414681 0.1442457216019083 0.9373070846962247
0.7709799715197749 0.9569331223494054 0.14122776441649953
0.3053927082876986 0.03958962422796164 0.27678369479169207
0.8065125051156038 0.177343035278254 0.15457051471078964
0.9547186557023949 0.154551400089043 0.8338892941512318
0.04106280627180747 0.38618352833582825 0.3495923019636925
0.3417077927504538 0.8164630567142304 0.47593490813172823
0.7828902895224036 0.4708401253088451 0.8173440068581418
0.8815675986556064 0.43959639767963965 0.781063597792481
0.8147411602576974 0.29567891564250737 0.12387667430690597
python
I;m trying to sort rgb values based on a greedy algorithm that is meant to work by getting a random colour, check the distance between itself and another colour, and then when it finds the one with the shortest distance (euclidean) to itself, append that onto the new array/list.
I'm getting an error concerning the line col[l] not in colouringArray. The point of this line is to make sure colours that are appended to the new list aren't used again.
python
def greedy(cols):
for i in range(len(cols)):
col = [eval(j) for j in cols[i]]
cols[i] = np.array(col)
greedyResult = #what will contain the result
colourArray =
resultIn =
randomColour = random.choice(cols) #make a random choice of colours
colourArray.append(randomColour)
greedyResult.append(colourArray)
colouringArray = greedyResult
resultIn.append(randomColour)
print(colouringArray)
for i in range(25 - 1):
shortestDist = 25
closestColour = ''
for l in range(0,25):
currPosition = colouringArray[-1]
checkPosition = cols[l]
distance = euc_distance(currPosition,checkPosition)
print(colouringArray)
print(colours[l])
if cols[l] not in colouringArray:
if distance < shortestDist:
shortestDist = distance
closestColour = cols[l]
colouringArray.append(closestColour)
print(colouringArray)
return colouringArray
def euc_distance(x, y):
maths = np.sum((x-y)**2)**0.5
print('x', x)
print('y', y)
return maths
Error
if cols[l] not in colouringArray:
ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all()
RGB values being loaded in from just a .txt file
0.9664535356921388 0.4407325991753527 0.007491470058587191
0.9109759624491242 0.939268997363764 0.5822275730589491
0.6715634814879851 0.08393822683708396 0.7664809327917963
0.23680977536311776 0.030814021726609964 0.7887727172362835
0.3460889655971231 0.6232814750391685 0.6158156951036152
0.14855463870828756 0.18309064740993164 0.11441296968868764
0.014618780486909122 0.48675154060475834 0.9649015609162157
0.06456228097718608 0.5410881855511303 0.46589855900830957
0.6014634495610515 0.08892882999066232 0.5790026861873665
0.26958550381944824 0.5564325605562156 0.6446342341782827
0.48103637136651844 0.35523914744298335 0.249152121361209
0.9335154980423467 0.45338801947649354 0.5301612069115903
0.019299566309716853 0.5081019257797922 0.005780237417743139
0.14376842759559538 0.47282692534740633 0.3773474407725964
0.05417519864614284 0.5875285081310694 0.1640032237419612
0.5573302374414681 0.1442457216019083 0.9373070846962247
0.7709799715197749 0.9569331223494054 0.14122776441649953
0.3053927082876986 0.03958962422796164 0.27678369479169207
0.8065125051156038 0.177343035278254 0.15457051471078964
0.9547186557023949 0.154551400089043 0.8338892941512318
0.04106280627180747 0.38618352833582825 0.3495923019636925
0.3417077927504538 0.8164630567142304 0.47593490813172823
0.7828902895224036 0.4708401253088451 0.8173440068581418
0.8815675986556064 0.43959639767963965 0.781063597792481
0.8147411602576974 0.29567891564250737 0.12387667430690597
python
python
asked Nov 11 '18 at 19:07
user10463530
add a comment |
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53252189%2fpreventing-list-elements-from-being-reused-in-an-algorithm%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53252189%2fpreventing-list-elements-from-being-reused-in-an-algorithm%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
gVX8nD0O CoBlRgM,Vzb,yArB x nTzxcb n8