Two libs at once
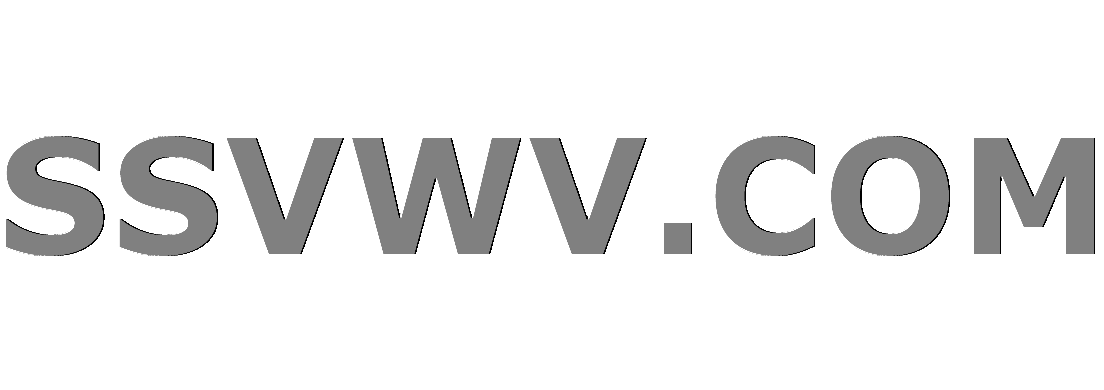
Multi tool use
Hey guys I am trying to make something like a keylogger I am currently using the pynput library but the problem is the program always executes the last thing I have imported for example if I import mouse listener first it doesn't catch the keyboard or the other way around so is there a way I can use both libraries at the same time?
from pynput.keyboard import Key, Listener
from pynput.mouse import Listener
def on_press(key):
print('0 pressed'.format(
key))
def on_release(key):
print('0 release'.format(
key))
def on_move(x, y):
print('Pointer moved to 0'.format(
(x, y)))
def on_click(x, y, button, pressed):
print('0 at 1'.format(
'Pressed' if pressed else 'Released',
(x, y)))
def on_scroll(x, y, dx, dy):
print('Scrolled 0'.format(
(x, y))),
# Collect events until released
with Listener(on_move=on_move, on_click=on_click, on_scroll=on_scroll, on_press=on_press, on_release=on_release) as listener:
listener.join()
python
add a comment |
Hey guys I am trying to make something like a keylogger I am currently using the pynput library but the problem is the program always executes the last thing I have imported for example if I import mouse listener first it doesn't catch the keyboard or the other way around so is there a way I can use both libraries at the same time?
from pynput.keyboard import Key, Listener
from pynput.mouse import Listener
def on_press(key):
print('0 pressed'.format(
key))
def on_release(key):
print('0 release'.format(
key))
def on_move(x, y):
print('Pointer moved to 0'.format(
(x, y)))
def on_click(x, y, button, pressed):
print('0 at 1'.format(
'Pressed' if pressed else 'Released',
(x, y)))
def on_scroll(x, y, dx, dy):
print('Scrolled 0'.format(
(x, y))),
# Collect events until released
with Listener(on_move=on_move, on_click=on_click, on_scroll=on_scroll, on_press=on_press, on_release=on_release) as listener:
listener.join()
python
add a comment |
Hey guys I am trying to make something like a keylogger I am currently using the pynput library but the problem is the program always executes the last thing I have imported for example if I import mouse listener first it doesn't catch the keyboard or the other way around so is there a way I can use both libraries at the same time?
from pynput.keyboard import Key, Listener
from pynput.mouse import Listener
def on_press(key):
print('0 pressed'.format(
key))
def on_release(key):
print('0 release'.format(
key))
def on_move(x, y):
print('Pointer moved to 0'.format(
(x, y)))
def on_click(x, y, button, pressed):
print('0 at 1'.format(
'Pressed' if pressed else 'Released',
(x, y)))
def on_scroll(x, y, dx, dy):
print('Scrolled 0'.format(
(x, y))),
# Collect events until released
with Listener(on_move=on_move, on_click=on_click, on_scroll=on_scroll, on_press=on_press, on_release=on_release) as listener:
listener.join()
python
Hey guys I am trying to make something like a keylogger I am currently using the pynput library but the problem is the program always executes the last thing I have imported for example if I import mouse listener first it doesn't catch the keyboard or the other way around so is there a way I can use both libraries at the same time?
from pynput.keyboard import Key, Listener
from pynput.mouse import Listener
def on_press(key):
print('0 pressed'.format(
key))
def on_release(key):
print('0 release'.format(
key))
def on_move(x, y):
print('Pointer moved to 0'.format(
(x, y)))
def on_click(x, y, button, pressed):
print('0 at 1'.format(
'Pressed' if pressed else 'Released',
(x, y)))
def on_scroll(x, y, dx, dy):
print('Scrolled 0'.format(
(x, y))),
# Collect events until released
with Listener(on_move=on_move, on_click=on_click, on_scroll=on_scroll, on_press=on_press, on_release=on_release) as listener:
listener.join()
python
python
edited Nov 14 '18 at 17:16
Daniel Roseman
456k41591648
456k41591648
asked Nov 14 '18 at 17:16


Thomas Arif BaşoğluThomas Arif Başoğlu
155
155
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
You are importing the name Listener
twice. The second time, it will overwrite the previous Listener. I suggest doing something like:
from pynput.keyboard import Listener as KeyboardListener
from pynput.keyboard import Key
from pynput.mouse import Listener as MouseListener
You will have to use MouseListener
and KeyboardListener
to refer to them.
It is still only executing Mouse listener because it is over Keyboard
– Thomas Arif Başoğlu
Nov 14 '18 at 17:39
@ThomasArifBaşoğlu It shouldn't be. What do you mean by executing. Do you mean defining?
– Qwerty
Nov 14 '18 at 17:41
No running them at the same time
– Thomas Arif Başoğlu
Nov 14 '18 at 19:50
with MouseListener(on_move=on_move, on_click=on_click, on_scroll=on_scroll, on_press=on_press, on_release=on_release) as listener: listener.join()
with KeyboardListener(on_press=on_press, on_release=on_release) as klistener: klistener.join()
– Thomas Arif Başoğlu
Nov 14 '18 at 20:24
I want to make them run at the same time @Qwerty
– Thomas Arif Başoğlu
Nov 14 '18 at 20:24
add a comment |
In Python, you can import things like this:
from pynput.keyboard import Key, Listener as keyListener
from pynput.mouse import Listener as mouseListener
When you need to use Listener
from the mouse library, just use mouseListner
instead and when you need to use Listener
from the keyboard library, just use keyListner
instead.
Hope this helps!
add a comment |
from pynput.keyboard import Key, Listener
from pynput.mouse import Listener
The second line overwrites the name Listener
in the global name space. One way to fix this is
from pynput import keyboard, mouse
Now you have to refer to keyboard.Listener
or mouse.Listener
. You will also need to do keyboard.Key
instead of just Key
.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53305556%2ftwo-libs-at-once%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
You are importing the name Listener
twice. The second time, it will overwrite the previous Listener. I suggest doing something like:
from pynput.keyboard import Listener as KeyboardListener
from pynput.keyboard import Key
from pynput.mouse import Listener as MouseListener
You will have to use MouseListener
and KeyboardListener
to refer to them.
It is still only executing Mouse listener because it is over Keyboard
– Thomas Arif Başoğlu
Nov 14 '18 at 17:39
@ThomasArifBaşoğlu It shouldn't be. What do you mean by executing. Do you mean defining?
– Qwerty
Nov 14 '18 at 17:41
No running them at the same time
– Thomas Arif Başoğlu
Nov 14 '18 at 19:50
with MouseListener(on_move=on_move, on_click=on_click, on_scroll=on_scroll, on_press=on_press, on_release=on_release) as listener: listener.join()
with KeyboardListener(on_press=on_press, on_release=on_release) as klistener: klistener.join()
– Thomas Arif Başoğlu
Nov 14 '18 at 20:24
I want to make them run at the same time @Qwerty
– Thomas Arif Başoğlu
Nov 14 '18 at 20:24
add a comment |
You are importing the name Listener
twice. The second time, it will overwrite the previous Listener. I suggest doing something like:
from pynput.keyboard import Listener as KeyboardListener
from pynput.keyboard import Key
from pynput.mouse import Listener as MouseListener
You will have to use MouseListener
and KeyboardListener
to refer to them.
It is still only executing Mouse listener because it is over Keyboard
– Thomas Arif Başoğlu
Nov 14 '18 at 17:39
@ThomasArifBaşoğlu It shouldn't be. What do you mean by executing. Do you mean defining?
– Qwerty
Nov 14 '18 at 17:41
No running them at the same time
– Thomas Arif Başoğlu
Nov 14 '18 at 19:50
with MouseListener(on_move=on_move, on_click=on_click, on_scroll=on_scroll, on_press=on_press, on_release=on_release) as listener: listener.join()
with KeyboardListener(on_press=on_press, on_release=on_release) as klistener: klistener.join()
– Thomas Arif Başoğlu
Nov 14 '18 at 20:24
I want to make them run at the same time @Qwerty
– Thomas Arif Başoğlu
Nov 14 '18 at 20:24
add a comment |
You are importing the name Listener
twice. The second time, it will overwrite the previous Listener. I suggest doing something like:
from pynput.keyboard import Listener as KeyboardListener
from pynput.keyboard import Key
from pynput.mouse import Listener as MouseListener
You will have to use MouseListener
and KeyboardListener
to refer to them.
You are importing the name Listener
twice. The second time, it will overwrite the previous Listener. I suggest doing something like:
from pynput.keyboard import Listener as KeyboardListener
from pynput.keyboard import Key
from pynput.mouse import Listener as MouseListener
You will have to use MouseListener
and KeyboardListener
to refer to them.
answered Nov 14 '18 at 17:23


QwertyQwerty
833619
833619
It is still only executing Mouse listener because it is over Keyboard
– Thomas Arif Başoğlu
Nov 14 '18 at 17:39
@ThomasArifBaşoğlu It shouldn't be. What do you mean by executing. Do you mean defining?
– Qwerty
Nov 14 '18 at 17:41
No running them at the same time
– Thomas Arif Başoğlu
Nov 14 '18 at 19:50
with MouseListener(on_move=on_move, on_click=on_click, on_scroll=on_scroll, on_press=on_press, on_release=on_release) as listener: listener.join()
with KeyboardListener(on_press=on_press, on_release=on_release) as klistener: klistener.join()
– Thomas Arif Başoğlu
Nov 14 '18 at 20:24
I want to make them run at the same time @Qwerty
– Thomas Arif Başoğlu
Nov 14 '18 at 20:24
add a comment |
It is still only executing Mouse listener because it is over Keyboard
– Thomas Arif Başoğlu
Nov 14 '18 at 17:39
@ThomasArifBaşoğlu It shouldn't be. What do you mean by executing. Do you mean defining?
– Qwerty
Nov 14 '18 at 17:41
No running them at the same time
– Thomas Arif Başoğlu
Nov 14 '18 at 19:50
with MouseListener(on_move=on_move, on_click=on_click, on_scroll=on_scroll, on_press=on_press, on_release=on_release) as listener: listener.join()
with KeyboardListener(on_press=on_press, on_release=on_release) as klistener: klistener.join()
– Thomas Arif Başoğlu
Nov 14 '18 at 20:24
I want to make them run at the same time @Qwerty
– Thomas Arif Başoğlu
Nov 14 '18 at 20:24
It is still only executing Mouse listener because it is over Keyboard
– Thomas Arif Başoğlu
Nov 14 '18 at 17:39
It is still only executing Mouse listener because it is over Keyboard
– Thomas Arif Başoğlu
Nov 14 '18 at 17:39
@ThomasArifBaşoğlu It shouldn't be. What do you mean by executing. Do you mean defining?
– Qwerty
Nov 14 '18 at 17:41
@ThomasArifBaşoğlu It shouldn't be. What do you mean by executing. Do you mean defining?
– Qwerty
Nov 14 '18 at 17:41
No running them at the same time
– Thomas Arif Başoğlu
Nov 14 '18 at 19:50
No running them at the same time
– Thomas Arif Başoğlu
Nov 14 '18 at 19:50
with MouseListener(on_move=on_move, on_click=on_click, on_scroll=on_scroll, on_press=on_press, on_release=on_release) as listener: listener.join()
with KeyboardListener(on_press=on_press, on_release=on_release) as klistener: klistener.join()
– Thomas Arif Başoğlu
Nov 14 '18 at 20:24
with MouseListener(on_move=on_move, on_click=on_click, on_scroll=on_scroll, on_press=on_press, on_release=on_release) as listener: listener.join()
with KeyboardListener(on_press=on_press, on_release=on_release) as klistener: klistener.join()
– Thomas Arif Başoğlu
Nov 14 '18 at 20:24
I want to make them run at the same time @Qwerty
– Thomas Arif Başoğlu
Nov 14 '18 at 20:24
I want to make them run at the same time @Qwerty
– Thomas Arif Başoğlu
Nov 14 '18 at 20:24
add a comment |
In Python, you can import things like this:
from pynput.keyboard import Key, Listener as keyListener
from pynput.mouse import Listener as mouseListener
When you need to use Listener
from the mouse library, just use mouseListner
instead and when you need to use Listener
from the keyboard library, just use keyListner
instead.
Hope this helps!
add a comment |
In Python, you can import things like this:
from pynput.keyboard import Key, Listener as keyListener
from pynput.mouse import Listener as mouseListener
When you need to use Listener
from the mouse library, just use mouseListner
instead and when you need to use Listener
from the keyboard library, just use keyListner
instead.
Hope this helps!
add a comment |
In Python, you can import things like this:
from pynput.keyboard import Key, Listener as keyListener
from pynput.mouse import Listener as mouseListener
When you need to use Listener
from the mouse library, just use mouseListner
instead and when you need to use Listener
from the keyboard library, just use keyListner
instead.
Hope this helps!
In Python, you can import things like this:
from pynput.keyboard import Key, Listener as keyListener
from pynput.mouse import Listener as mouseListener
When you need to use Listener
from the mouse library, just use mouseListner
instead and when you need to use Listener
from the keyboard library, just use keyListner
instead.
Hope this helps!
answered Nov 14 '18 at 17:22


lgwilliamslgwilliams
503518
503518
add a comment |
add a comment |
from pynput.keyboard import Key, Listener
from pynput.mouse import Listener
The second line overwrites the name Listener
in the global name space. One way to fix this is
from pynput import keyboard, mouse
Now you have to refer to keyboard.Listener
or mouse.Listener
. You will also need to do keyboard.Key
instead of just Key
.
add a comment |
from pynput.keyboard import Key, Listener
from pynput.mouse import Listener
The second line overwrites the name Listener
in the global name space. One way to fix this is
from pynput import keyboard, mouse
Now you have to refer to keyboard.Listener
or mouse.Listener
. You will also need to do keyboard.Key
instead of just Key
.
add a comment |
from pynput.keyboard import Key, Listener
from pynput.mouse import Listener
The second line overwrites the name Listener
in the global name space. One way to fix this is
from pynput import keyboard, mouse
Now you have to refer to keyboard.Listener
or mouse.Listener
. You will also need to do keyboard.Key
instead of just Key
.
from pynput.keyboard import Key, Listener
from pynput.mouse import Listener
The second line overwrites the name Listener
in the global name space. One way to fix this is
from pynput import keyboard, mouse
Now you have to refer to keyboard.Listener
or mouse.Listener
. You will also need to do keyboard.Key
instead of just Key
.
answered Nov 14 '18 at 17:23
Code-ApprenticeCode-Apprentice
48.3k1490179
48.3k1490179
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53305556%2ftwo-libs-at-once%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
AkV,pwRb08 lwhZw,sN2a66A0OmY17LvlRCK,FpUJagWDbBYE4YrKEJ Q7EGt