Constructor in class cannot be applied to give types
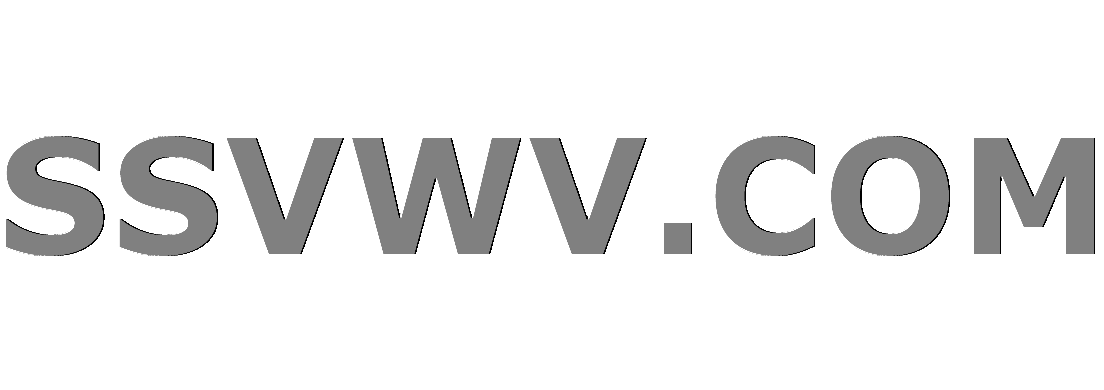
Multi tool use
up vote
0
down vote
favorite
I am attempting to create multiple classes that inherit from the same superclass. My first class worked perfectly. When I attempted a second one I got an error message I haven't seen before. I'm new at this so I'm not sure how to solve the issue.
This is the class
class Employee extends Person
double salary;
private java.util.Date dateCreated;
public Employee()
public Employee(String name, String address, String phone_number, double salary)
super(name, address, phone_number);
this.salary = salary;
public String getDate()
java.util.Date date = new java.util.Date();
return date.toString();
public double getSalary()
return this.salary;
public String toString()
return("Employee object:n" + super.toString() + "n" + "Salary:t$" + getSalary() + "n" + "Date Hired: " + getDate());
I am attempting to call the method this way
Employee employee = new Employee (name, address, number, salary);
System.out.println(employee.toString());
I am getting this error message:
testPerson.java:21: error: constructor Employee in class Employee cannot be applied to given types;
Employee employee = new Employee (name, address, number, salary);
^
required: no arguments
found: String,String,String,double
reason: actual and formal argument lists differ in length
1 error
Here is TestPerson class
public class TestPerson
public static void main(String args)
Scanner input = new Scanner(System.in);
System.out.println("Please enter your name: ");
String name = input.next();
System.out.println("Please enter your address: ");
String address = input.next();
System.out.println("Please enter your phone number: ");
String number = input.next();
System.out.println("Please enter your yearly salary: ");
double salary = input.nextDouble();
Employee employee = new Employee (name, address, number, salary);
System.out.println(employee.toString());
This is the parent class to clear it up. I could be doing something wrong that I'm not catching.
import java.util.*;
public class Person
private String name;
private String address;
private String phone_number;
public Person()
public Person(String name, String address, String phone_number)
this.name = name;
this.address = address;
this.phone_number = phone_number;
public String getName()
return this.name;
public String getAddress()
return this.address;
public String getPhone()
return this.phone_number;
public String toString()
return ("Person object:n" + "Name:tt" + getName() + "n" + "Address:t" + getAddress() + "n" + "Phone#:t" + getPhone());
java inheritance methods constructor extends
|
show 6 more comments
up vote
0
down vote
favorite
I am attempting to create multiple classes that inherit from the same superclass. My first class worked perfectly. When I attempted a second one I got an error message I haven't seen before. I'm new at this so I'm not sure how to solve the issue.
This is the class
class Employee extends Person
double salary;
private java.util.Date dateCreated;
public Employee()
public Employee(String name, String address, String phone_number, double salary)
super(name, address, phone_number);
this.salary = salary;
public String getDate()
java.util.Date date = new java.util.Date();
return date.toString();
public double getSalary()
return this.salary;
public String toString()
return("Employee object:n" + super.toString() + "n" + "Salary:t$" + getSalary() + "n" + "Date Hired: " + getDate());
I am attempting to call the method this way
Employee employee = new Employee (name, address, number, salary);
System.out.println(employee.toString());
I am getting this error message:
testPerson.java:21: error: constructor Employee in class Employee cannot be applied to given types;
Employee employee = new Employee (name, address, number, salary);
^
required: no arguments
found: String,String,String,double
reason: actual and formal argument lists differ in length
1 error
Here is TestPerson class
public class TestPerson
public static void main(String args)
Scanner input = new Scanner(System.in);
System.out.println("Please enter your name: ");
String name = input.next();
System.out.println("Please enter your address: ");
String address = input.next();
System.out.println("Please enter your phone number: ");
String number = input.next();
System.out.println("Please enter your yearly salary: ");
double salary = input.nextDouble();
Employee employee = new Employee (name, address, number, salary);
System.out.println(employee.toString());
This is the parent class to clear it up. I could be doing something wrong that I'm not catching.
import java.util.*;
public class Person
private String name;
private String address;
private String phone_number;
public Person()
public Person(String name, String address, String phone_number)
this.name = name;
this.address = address;
this.phone_number = phone_number;
public String getName()
return this.name;
public String getAddress()
return this.address;
public String getPhone()
return this.phone_number;
public String toString()
return ("Person object:n" + "Name:tt" + getName() + "n" + "Address:t" + getAddress() + "n" + "Phone#:t" + getPhone());
java inheritance methods constructor extends
1
Are you sure that you don't have avoid
in front of the second Employee constructor, the one that takes 4 parameters?
– Hovercraft Full Of Eels
Nov 11 at 3:45
1
@ScottRobinson No you shouldn't. That would cause it to be a method and not a constructor
– GBlodgett
Nov 11 at 3:49
1
No, there shouldn't be avoid
there, but it is a common cause for this type of error. Please show yourtestPerson
class (which should be re-namedTestPerson
)
– Hovercraft Full Of Eels
Nov 11 at 3:50
1
Recompile, rebuild, clean your project within the IDE. Try again.
– Hovercraft Full Of Eels
Nov 11 at 3:58
2
Looks good. Make sure your test doesn’t run against an “old” version of the Employee class, where you didn’t have the four-argument-constructor
– iPirat
Nov 11 at 4:03
|
show 6 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am attempting to create multiple classes that inherit from the same superclass. My first class worked perfectly. When I attempted a second one I got an error message I haven't seen before. I'm new at this so I'm not sure how to solve the issue.
This is the class
class Employee extends Person
double salary;
private java.util.Date dateCreated;
public Employee()
public Employee(String name, String address, String phone_number, double salary)
super(name, address, phone_number);
this.salary = salary;
public String getDate()
java.util.Date date = new java.util.Date();
return date.toString();
public double getSalary()
return this.salary;
public String toString()
return("Employee object:n" + super.toString() + "n" + "Salary:t$" + getSalary() + "n" + "Date Hired: " + getDate());
I am attempting to call the method this way
Employee employee = new Employee (name, address, number, salary);
System.out.println(employee.toString());
I am getting this error message:
testPerson.java:21: error: constructor Employee in class Employee cannot be applied to given types;
Employee employee = new Employee (name, address, number, salary);
^
required: no arguments
found: String,String,String,double
reason: actual and formal argument lists differ in length
1 error
Here is TestPerson class
public class TestPerson
public static void main(String args)
Scanner input = new Scanner(System.in);
System.out.println("Please enter your name: ");
String name = input.next();
System.out.println("Please enter your address: ");
String address = input.next();
System.out.println("Please enter your phone number: ");
String number = input.next();
System.out.println("Please enter your yearly salary: ");
double salary = input.nextDouble();
Employee employee = new Employee (name, address, number, salary);
System.out.println(employee.toString());
This is the parent class to clear it up. I could be doing something wrong that I'm not catching.
import java.util.*;
public class Person
private String name;
private String address;
private String phone_number;
public Person()
public Person(String name, String address, String phone_number)
this.name = name;
this.address = address;
this.phone_number = phone_number;
public String getName()
return this.name;
public String getAddress()
return this.address;
public String getPhone()
return this.phone_number;
public String toString()
return ("Person object:n" + "Name:tt" + getName() + "n" + "Address:t" + getAddress() + "n" + "Phone#:t" + getPhone());
java inheritance methods constructor extends
I am attempting to create multiple classes that inherit from the same superclass. My first class worked perfectly. When I attempted a second one I got an error message I haven't seen before. I'm new at this so I'm not sure how to solve the issue.
This is the class
class Employee extends Person
double salary;
private java.util.Date dateCreated;
public Employee()
public Employee(String name, String address, String phone_number, double salary)
super(name, address, phone_number);
this.salary = salary;
public String getDate()
java.util.Date date = new java.util.Date();
return date.toString();
public double getSalary()
return this.salary;
public String toString()
return("Employee object:n" + super.toString() + "n" + "Salary:t$" + getSalary() + "n" + "Date Hired: " + getDate());
I am attempting to call the method this way
Employee employee = new Employee (name, address, number, salary);
System.out.println(employee.toString());
I am getting this error message:
testPerson.java:21: error: constructor Employee in class Employee cannot be applied to given types;
Employee employee = new Employee (name, address, number, salary);
^
required: no arguments
found: String,String,String,double
reason: actual and formal argument lists differ in length
1 error
Here is TestPerson class
public class TestPerson
public static void main(String args)
Scanner input = new Scanner(System.in);
System.out.println("Please enter your name: ");
String name = input.next();
System.out.println("Please enter your address: ");
String address = input.next();
System.out.println("Please enter your phone number: ");
String number = input.next();
System.out.println("Please enter your yearly salary: ");
double salary = input.nextDouble();
Employee employee = new Employee (name, address, number, salary);
System.out.println(employee.toString());
This is the parent class to clear it up. I could be doing something wrong that I'm not catching.
import java.util.*;
public class Person
private String name;
private String address;
private String phone_number;
public Person()
public Person(String name, String address, String phone_number)
this.name = name;
this.address = address;
this.phone_number = phone_number;
public String getName()
return this.name;
public String getAddress()
return this.address;
public String getPhone()
return this.phone_number;
public String toString()
return ("Person object:n" + "Name:tt" + getName() + "n" + "Address:t" + getAddress() + "n" + "Phone#:t" + getPhone());
java inheritance methods constructor extends
java inheritance methods constructor extends
edited Nov 11 at 4:10
asked Nov 11 at 3:39
Scott Robinson
11
11
1
Are you sure that you don't have avoid
in front of the second Employee constructor, the one that takes 4 parameters?
– Hovercraft Full Of Eels
Nov 11 at 3:45
1
@ScottRobinson No you shouldn't. That would cause it to be a method and not a constructor
– GBlodgett
Nov 11 at 3:49
1
No, there shouldn't be avoid
there, but it is a common cause for this type of error. Please show yourtestPerson
class (which should be re-namedTestPerson
)
– Hovercraft Full Of Eels
Nov 11 at 3:50
1
Recompile, rebuild, clean your project within the IDE. Try again.
– Hovercraft Full Of Eels
Nov 11 at 3:58
2
Looks good. Make sure your test doesn’t run against an “old” version of the Employee class, where you didn’t have the four-argument-constructor
– iPirat
Nov 11 at 4:03
|
show 6 more comments
1
Are you sure that you don't have avoid
in front of the second Employee constructor, the one that takes 4 parameters?
– Hovercraft Full Of Eels
Nov 11 at 3:45
1
@ScottRobinson No you shouldn't. That would cause it to be a method and not a constructor
– GBlodgett
Nov 11 at 3:49
1
No, there shouldn't be avoid
there, but it is a common cause for this type of error. Please show yourtestPerson
class (which should be re-namedTestPerson
)
– Hovercraft Full Of Eels
Nov 11 at 3:50
1
Recompile, rebuild, clean your project within the IDE. Try again.
– Hovercraft Full Of Eels
Nov 11 at 3:58
2
Looks good. Make sure your test doesn’t run against an “old” version of the Employee class, where you didn’t have the four-argument-constructor
– iPirat
Nov 11 at 4:03
1
1
Are you sure that you don't have a
void
in front of the second Employee constructor, the one that takes 4 parameters?– Hovercraft Full Of Eels
Nov 11 at 3:45
Are you sure that you don't have a
void
in front of the second Employee constructor, the one that takes 4 parameters?– Hovercraft Full Of Eels
Nov 11 at 3:45
1
1
@ScottRobinson No you shouldn't. That would cause it to be a method and not a constructor
– GBlodgett
Nov 11 at 3:49
@ScottRobinson No you shouldn't. That would cause it to be a method and not a constructor
– GBlodgett
Nov 11 at 3:49
1
1
No, there shouldn't be a
void
there, but it is a common cause for this type of error. Please show your testPerson
class (which should be re-named TestPerson
)– Hovercraft Full Of Eels
Nov 11 at 3:50
No, there shouldn't be a
void
there, but it is a common cause for this type of error. Please show your testPerson
class (which should be re-named TestPerson
)– Hovercraft Full Of Eels
Nov 11 at 3:50
1
1
Recompile, rebuild, clean your project within the IDE. Try again.
– Hovercraft Full Of Eels
Nov 11 at 3:58
Recompile, rebuild, clean your project within the IDE. Try again.
– Hovercraft Full Of Eels
Nov 11 at 3:58
2
2
Looks good. Make sure your test doesn’t run against an “old” version of the Employee class, where you didn’t have the four-argument-constructor
– iPirat
Nov 11 at 4:03
Looks good. Make sure your test doesn’t run against an “old” version of the Employee class, where you didn’t have the four-argument-constructor
– iPirat
Nov 11 at 4:03
|
show 6 more comments
1 Answer
1
active
oldest
votes
up vote
0
down vote
I'm following the state of this issue since the beginning now and I found it really interesting so I copied your code and test it locally and surprise like I was expecting ... no single error found.
It seems that your compiler is trying to use the constructor with no arguments instead of the second one you gived. You should verify your code if this is really this Employee class who is used in testPerson.java.
Best not to post "your code worked for me" as an answer.
– Hovercraft Full Of Eels
Nov 11 at 4:50
this is not a "your code worked for me" answer. I'm advising him to check again his imports and the structure of his project because no one found a single mistake on his code.
– Moussa
Nov 11 at 4:54
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53245636%2fconstructor-in-class-cannot-be-applied-to-give-types%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
I'm following the state of this issue since the beginning now and I found it really interesting so I copied your code and test it locally and surprise like I was expecting ... no single error found.
It seems that your compiler is trying to use the constructor with no arguments instead of the second one you gived. You should verify your code if this is really this Employee class who is used in testPerson.java.
Best not to post "your code worked for me" as an answer.
– Hovercraft Full Of Eels
Nov 11 at 4:50
this is not a "your code worked for me" answer. I'm advising him to check again his imports and the structure of his project because no one found a single mistake on his code.
– Moussa
Nov 11 at 4:54
add a comment |
up vote
0
down vote
I'm following the state of this issue since the beginning now and I found it really interesting so I copied your code and test it locally and surprise like I was expecting ... no single error found.
It seems that your compiler is trying to use the constructor with no arguments instead of the second one you gived. You should verify your code if this is really this Employee class who is used in testPerson.java.
Best not to post "your code worked for me" as an answer.
– Hovercraft Full Of Eels
Nov 11 at 4:50
this is not a "your code worked for me" answer. I'm advising him to check again his imports and the structure of his project because no one found a single mistake on his code.
– Moussa
Nov 11 at 4:54
add a comment |
up vote
0
down vote
up vote
0
down vote
I'm following the state of this issue since the beginning now and I found it really interesting so I copied your code and test it locally and surprise like I was expecting ... no single error found.
It seems that your compiler is trying to use the constructor with no arguments instead of the second one you gived. You should verify your code if this is really this Employee class who is used in testPerson.java.
I'm following the state of this issue since the beginning now and I found it really interesting so I copied your code and test it locally and surprise like I was expecting ... no single error found.
It seems that your compiler is trying to use the constructor with no arguments instead of the second one you gived. You should verify your code if this is really this Employee class who is used in testPerson.java.
answered Nov 11 at 4:35
Moussa
788
788
Best not to post "your code worked for me" as an answer.
– Hovercraft Full Of Eels
Nov 11 at 4:50
this is not a "your code worked for me" answer. I'm advising him to check again his imports and the structure of his project because no one found a single mistake on his code.
– Moussa
Nov 11 at 4:54
add a comment |
Best not to post "your code worked for me" as an answer.
– Hovercraft Full Of Eels
Nov 11 at 4:50
this is not a "your code worked for me" answer. I'm advising him to check again his imports and the structure of his project because no one found a single mistake on his code.
– Moussa
Nov 11 at 4:54
Best not to post "your code worked for me" as an answer.
– Hovercraft Full Of Eels
Nov 11 at 4:50
Best not to post "your code worked for me" as an answer.
– Hovercraft Full Of Eels
Nov 11 at 4:50
this is not a "your code worked for me" answer. I'm advising him to check again his imports and the structure of his project because no one found a single mistake on his code.
– Moussa
Nov 11 at 4:54
this is not a "your code worked for me" answer. I'm advising him to check again his imports and the structure of his project because no one found a single mistake on his code.
– Moussa
Nov 11 at 4:54
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53245636%2fconstructor-in-class-cannot-be-applied-to-give-types%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
GeNl5ozXvVvwP9,fj GSLTqQk56,n278DREWxn HVRz05dcdW bmmNXC9d6vhTfeY3f Lag,xZj Rl7,DTmc
1
Are you sure that you don't have a
void
in front of the second Employee constructor, the one that takes 4 parameters?– Hovercraft Full Of Eels
Nov 11 at 3:45
1
@ScottRobinson No you shouldn't. That would cause it to be a method and not a constructor
– GBlodgett
Nov 11 at 3:49
1
No, there shouldn't be a
void
there, but it is a common cause for this type of error. Please show yourtestPerson
class (which should be re-namedTestPerson
)– Hovercraft Full Of Eels
Nov 11 at 3:50
1
Recompile, rebuild, clean your project within the IDE. Try again.
– Hovercraft Full Of Eels
Nov 11 at 3:58
2
Looks good. Make sure your test doesn’t run against an “old” version of the Employee class, where you didn’t have the four-argument-constructor
– iPirat
Nov 11 at 4:03