How can I fill a rotated rectangle over an image?
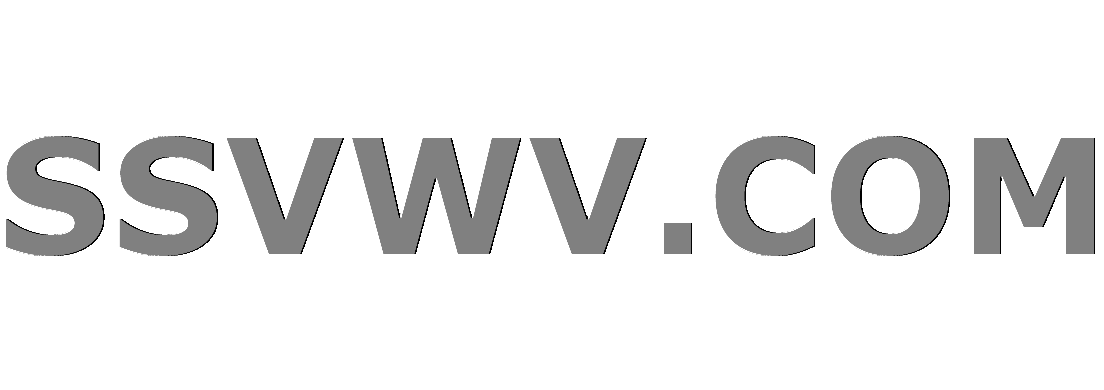
Multi tool use
How can i fill a rotated rectangle? I can only fill a rectangle that is not rotated and it works fine. But i recently need to fill a rotated rectangle on any degree.
This is my code
using (ImageMagick.MagickImage img = new ImageMagick.MagickImage())
img.Read(image.FullPath);
foreach (var item in ListofCoordinates)
ImageMagick.DrawableFillColor fillColor = new ImageMagick.DrawableFillColor(System.Drawing.Color.LightGray);
var d = int.Parse(Math.Round(((item.Rotation * img.Density.X) / 96)).ToString());
var x = int.Parse(Math.Round(((item.X * img.Density.X) / 96)).ToString());
var y = int.Parse(Math.Round(((item.Y * img.Density.X) / 96)).ToString());
var w = int.Parse(Math.Round(((item.Width * img.Density.X) / 96)).ToString());
var h = int.Parse(Math.Round(((item.Height * img.Density.X) / 96)).ToString());
var r = new System.Drawing.Rectangle(x, y, w, h);
ImageMagick.DrawableRectangle rect = new ImageMagick.DrawableRectangle(r);
img.Draw(fillColor, rect);
img.Write(System.IO.Path.Combine(OutputPath, image.FileName));
This is a sample of what i should achieve
This is the sample image
this is the First picture
This is the output
This is the second picture which is the output
c# imagemagick drawing drawrectangle
add a comment |
How can i fill a rotated rectangle? I can only fill a rectangle that is not rotated and it works fine. But i recently need to fill a rotated rectangle on any degree.
This is my code
using (ImageMagick.MagickImage img = new ImageMagick.MagickImage())
img.Read(image.FullPath);
foreach (var item in ListofCoordinates)
ImageMagick.DrawableFillColor fillColor = new ImageMagick.DrawableFillColor(System.Drawing.Color.LightGray);
var d = int.Parse(Math.Round(((item.Rotation * img.Density.X) / 96)).ToString());
var x = int.Parse(Math.Round(((item.X * img.Density.X) / 96)).ToString());
var y = int.Parse(Math.Round(((item.Y * img.Density.X) / 96)).ToString());
var w = int.Parse(Math.Round(((item.Width * img.Density.X) / 96)).ToString());
var h = int.Parse(Math.Round(((item.Height * img.Density.X) / 96)).ToString());
var r = new System.Drawing.Rectangle(x, y, w, h);
ImageMagick.DrawableRectangle rect = new ImageMagick.DrawableRectangle(r);
img.Draw(fillColor, rect);
img.Write(System.IO.Path.Combine(OutputPath, image.FileName));
This is a sample of what i should achieve
This is the sample image
this is the First picture
This is the output
This is the second picture which is the output
c# imagemagick drawing drawrectangle
add a comment |
How can i fill a rotated rectangle? I can only fill a rectangle that is not rotated and it works fine. But i recently need to fill a rotated rectangle on any degree.
This is my code
using (ImageMagick.MagickImage img = new ImageMagick.MagickImage())
img.Read(image.FullPath);
foreach (var item in ListofCoordinates)
ImageMagick.DrawableFillColor fillColor = new ImageMagick.DrawableFillColor(System.Drawing.Color.LightGray);
var d = int.Parse(Math.Round(((item.Rotation * img.Density.X) / 96)).ToString());
var x = int.Parse(Math.Round(((item.X * img.Density.X) / 96)).ToString());
var y = int.Parse(Math.Round(((item.Y * img.Density.X) / 96)).ToString());
var w = int.Parse(Math.Round(((item.Width * img.Density.X) / 96)).ToString());
var h = int.Parse(Math.Round(((item.Height * img.Density.X) / 96)).ToString());
var r = new System.Drawing.Rectangle(x, y, w, h);
ImageMagick.DrawableRectangle rect = new ImageMagick.DrawableRectangle(r);
img.Draw(fillColor, rect);
img.Write(System.IO.Path.Combine(OutputPath, image.FileName));
This is a sample of what i should achieve
This is the sample image
this is the First picture
This is the output
This is the second picture which is the output
c# imagemagick drawing drawrectangle
How can i fill a rotated rectangle? I can only fill a rectangle that is not rotated and it works fine. But i recently need to fill a rotated rectangle on any degree.
This is my code
using (ImageMagick.MagickImage img = new ImageMagick.MagickImage())
img.Read(image.FullPath);
foreach (var item in ListofCoordinates)
ImageMagick.DrawableFillColor fillColor = new ImageMagick.DrawableFillColor(System.Drawing.Color.LightGray);
var d = int.Parse(Math.Round(((item.Rotation * img.Density.X) / 96)).ToString());
var x = int.Parse(Math.Round(((item.X * img.Density.X) / 96)).ToString());
var y = int.Parse(Math.Round(((item.Y * img.Density.X) / 96)).ToString());
var w = int.Parse(Math.Round(((item.Width * img.Density.X) / 96)).ToString());
var h = int.Parse(Math.Round(((item.Height * img.Density.X) / 96)).ToString());
var r = new System.Drawing.Rectangle(x, y, w, h);
ImageMagick.DrawableRectangle rect = new ImageMagick.DrawableRectangle(r);
img.Draw(fillColor, rect);
img.Write(System.IO.Path.Combine(OutputPath, image.FileName));
This is a sample of what i should achieve
This is the sample image
this is the First picture
This is the output
This is the second picture which is the output
c# imagemagick drawing drawrectangle
c# imagemagick drawing drawrectangle
edited Nov 12 '18 at 7:15


John
11.7k31938
11.7k31938
asked Nov 12 '18 at 6:56


WagJohnWagJohn
12
12
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Well, while you could use ImageMagic, the .Net libraries are sufficient.
There are few ways to draw shape like rotated rectangle.
1) Draw 4 points (you could draw any shape this way, not only rectangle), rotate it
C#
Dim Img As Image = New Bitmap(Me.Picturebox1.Width, Me.Picturebox1.Height)
Dim g As Graphics = Graphics.FromImage(Img)
Dim bgBrush As SolidBrush = New SolidBrush(Color.LightSlateGray)
Dim shPen As Pen = New Pen(Color.Black)
Dim pts() As Point
pts = New Point(150, 100), ' 1
New Point(200, 100), ' 2
New Point(150, 200), ' 3
New Point(200, 200) ' 4
Dim pth As New GraphicsPath
pth = ShapeData(0)
g.FillPath(bgBrush, pth) ' fill
g.DrawPath(shPen, pth) ' border
Dim Angle As Int16 = 30
Dim RotMatrix As New Matrix ' rotate matrix to put the shape back to initial coordinates (with bottom left point as reference)
RotMatrix.Rotate(Angle, MatrixOrder.Append) ' Rotate the pattern to the desired angle
RotMatrix.TransformPoints(pts) ' carry out matrix transformation over the shape points
VB.NET
Image Img = new Bitmap(this.Picturebox1.Width, this.Picturebox1.Height);
Graphics g = Graphics.FromImage(Img);
SolidBrush bgBrush = new SolidBrush(Color.LightSlateGray);
Pen shPen = new Pen(Color.Black);
Point pts;
pts = new new Point(150, 100), new Point(200, 100), new Point(150, 200), new Point(200, 200) ; // 4
GraphicsPath pth = new GraphicsPath();
pth = ShapeData(0);
g.FillPath(bgBrush, pth); // fill
g.DrawPath(shPen, pth); // border
Int16 Angle = 30;
Matrix RotMatrix = new Matrix(); // rotate matrix to put the shape back to initial coordinates (with bottom left point as reference)
RotMatrix.Rotate(Angle, MatrixOrder.Append); // Rotate the pattern to the desired angle
RotMatrix.TransformPoints(pts); // carry out matrix transformation over the shape points
2) Draw 4 points already rotated
C#
Dim Img As Image = New Bitmap(Me.Picturebox1.Width, Me.Picturebox1.Height)
Dim g As Graphics = Graphics.FromImage(Img)
Dim bgBrush As SolidBrush = New SolidBrush(Color.LightSlateGray)
Dim shPen As Pen = New Pen(Color.Black)
Dim pts() As Point
pts = New Point(150, 100), ' 1 - rotated coordinates
New Point(210, 110), ' 2 - rotated coordinates
New Point(140, 200), ' 3 - rotated coordinates
New Point(200, 210) ' 4 - rotated coordinates
Dim pth As New GraphicsPath
pth = ShapeData(0)
g.FillPath(bgBrush, pth) ' fill
g.DrawPath(shPen, pth) ' border
VB.NET
Image Img = new Bitmap(this.Picturebox1.Width, this.Picturebox1.Height);
Graphics g = Graphics.FromImage(Img);
SolidBrush bgBrush = new SolidBrush(Color.LightSlateGray);
Pen shPen = new Pen(Color.Black);
Point pts;
pts = new new Point(150, 100), new Point(210, 110), new Point(140, 200), new Point(200, 210) ; // 4
GraphicsPath pth = new GraphicsPath();
pth = ShapeData(0);
g.FillPath(bgBrush, pth); // fill
g.DrawPath(shPen, pth); // border
3) Draw rectangle and rotate graphics. This might be little bit tricky.
C#
Image Img = new Bitmap(this.Picturebox1.Width, this.Picturebox1.Height);
Graphics g = Graphics.FromImage(Img);
SolidBrush bgBrush = new SolidBrush(Color.LightSlateGray);
Pen shPen = new Pen(Color.Black);
Int16 dDimX = 75;
Int16 dDimY = 150;
Int16 dLocX = 200;
Int16 dLocY = 100;
Rectangle rect = new Rectangle(dLocX, dLocY, dDimX, dDimY);
g.RotateTransform(30);
VB.NET
Dim Img As Image = New Bitmap(Me.Picturebox1.Width, Me.Picturebox1.Height)
Dim g As Graphics = Graphics.FromImage(Img)
Dim bgBrush As SolidBrush = New SolidBrush(Color.LightSlateGray)
Dim shPen As Pen = New Pen(Color.Black)
Dim dDimX As Int16 = 75
Dim dDimY As Int16 = 150
Dim dLocX As Int16 = 200
Dim dLocY As Int16 = 100
Dim rect As Rectangle = New Rectangle(dLocX, dLocY, dDimX, dDimY)
g.RotateTransform(30)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53257195%2fhow-can-i-fill-a-rotated-rectangle-over-an-image%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Well, while you could use ImageMagic, the .Net libraries are sufficient.
There are few ways to draw shape like rotated rectangle.
1) Draw 4 points (you could draw any shape this way, not only rectangle), rotate it
C#
Dim Img As Image = New Bitmap(Me.Picturebox1.Width, Me.Picturebox1.Height)
Dim g As Graphics = Graphics.FromImage(Img)
Dim bgBrush As SolidBrush = New SolidBrush(Color.LightSlateGray)
Dim shPen As Pen = New Pen(Color.Black)
Dim pts() As Point
pts = New Point(150, 100), ' 1
New Point(200, 100), ' 2
New Point(150, 200), ' 3
New Point(200, 200) ' 4
Dim pth As New GraphicsPath
pth = ShapeData(0)
g.FillPath(bgBrush, pth) ' fill
g.DrawPath(shPen, pth) ' border
Dim Angle As Int16 = 30
Dim RotMatrix As New Matrix ' rotate matrix to put the shape back to initial coordinates (with bottom left point as reference)
RotMatrix.Rotate(Angle, MatrixOrder.Append) ' Rotate the pattern to the desired angle
RotMatrix.TransformPoints(pts) ' carry out matrix transformation over the shape points
VB.NET
Image Img = new Bitmap(this.Picturebox1.Width, this.Picturebox1.Height);
Graphics g = Graphics.FromImage(Img);
SolidBrush bgBrush = new SolidBrush(Color.LightSlateGray);
Pen shPen = new Pen(Color.Black);
Point pts;
pts = new new Point(150, 100), new Point(200, 100), new Point(150, 200), new Point(200, 200) ; // 4
GraphicsPath pth = new GraphicsPath();
pth = ShapeData(0);
g.FillPath(bgBrush, pth); // fill
g.DrawPath(shPen, pth); // border
Int16 Angle = 30;
Matrix RotMatrix = new Matrix(); // rotate matrix to put the shape back to initial coordinates (with bottom left point as reference)
RotMatrix.Rotate(Angle, MatrixOrder.Append); // Rotate the pattern to the desired angle
RotMatrix.TransformPoints(pts); // carry out matrix transformation over the shape points
2) Draw 4 points already rotated
C#
Dim Img As Image = New Bitmap(Me.Picturebox1.Width, Me.Picturebox1.Height)
Dim g As Graphics = Graphics.FromImage(Img)
Dim bgBrush As SolidBrush = New SolidBrush(Color.LightSlateGray)
Dim shPen As Pen = New Pen(Color.Black)
Dim pts() As Point
pts = New Point(150, 100), ' 1 - rotated coordinates
New Point(210, 110), ' 2 - rotated coordinates
New Point(140, 200), ' 3 - rotated coordinates
New Point(200, 210) ' 4 - rotated coordinates
Dim pth As New GraphicsPath
pth = ShapeData(0)
g.FillPath(bgBrush, pth) ' fill
g.DrawPath(shPen, pth) ' border
VB.NET
Image Img = new Bitmap(this.Picturebox1.Width, this.Picturebox1.Height);
Graphics g = Graphics.FromImage(Img);
SolidBrush bgBrush = new SolidBrush(Color.LightSlateGray);
Pen shPen = new Pen(Color.Black);
Point pts;
pts = new new Point(150, 100), new Point(210, 110), new Point(140, 200), new Point(200, 210) ; // 4
GraphicsPath pth = new GraphicsPath();
pth = ShapeData(0);
g.FillPath(bgBrush, pth); // fill
g.DrawPath(shPen, pth); // border
3) Draw rectangle and rotate graphics. This might be little bit tricky.
C#
Image Img = new Bitmap(this.Picturebox1.Width, this.Picturebox1.Height);
Graphics g = Graphics.FromImage(Img);
SolidBrush bgBrush = new SolidBrush(Color.LightSlateGray);
Pen shPen = new Pen(Color.Black);
Int16 dDimX = 75;
Int16 dDimY = 150;
Int16 dLocX = 200;
Int16 dLocY = 100;
Rectangle rect = new Rectangle(dLocX, dLocY, dDimX, dDimY);
g.RotateTransform(30);
VB.NET
Dim Img As Image = New Bitmap(Me.Picturebox1.Width, Me.Picturebox1.Height)
Dim g As Graphics = Graphics.FromImage(Img)
Dim bgBrush As SolidBrush = New SolidBrush(Color.LightSlateGray)
Dim shPen As Pen = New Pen(Color.Black)
Dim dDimX As Int16 = 75
Dim dDimY As Int16 = 150
Dim dLocX As Int16 = 200
Dim dLocY As Int16 = 100
Dim rect As Rectangle = New Rectangle(dLocX, dLocY, dDimX, dDimY)
g.RotateTransform(30)
add a comment |
Well, while you could use ImageMagic, the .Net libraries are sufficient.
There are few ways to draw shape like rotated rectangle.
1) Draw 4 points (you could draw any shape this way, not only rectangle), rotate it
C#
Dim Img As Image = New Bitmap(Me.Picturebox1.Width, Me.Picturebox1.Height)
Dim g As Graphics = Graphics.FromImage(Img)
Dim bgBrush As SolidBrush = New SolidBrush(Color.LightSlateGray)
Dim shPen As Pen = New Pen(Color.Black)
Dim pts() As Point
pts = New Point(150, 100), ' 1
New Point(200, 100), ' 2
New Point(150, 200), ' 3
New Point(200, 200) ' 4
Dim pth As New GraphicsPath
pth = ShapeData(0)
g.FillPath(bgBrush, pth) ' fill
g.DrawPath(shPen, pth) ' border
Dim Angle As Int16 = 30
Dim RotMatrix As New Matrix ' rotate matrix to put the shape back to initial coordinates (with bottom left point as reference)
RotMatrix.Rotate(Angle, MatrixOrder.Append) ' Rotate the pattern to the desired angle
RotMatrix.TransformPoints(pts) ' carry out matrix transformation over the shape points
VB.NET
Image Img = new Bitmap(this.Picturebox1.Width, this.Picturebox1.Height);
Graphics g = Graphics.FromImage(Img);
SolidBrush bgBrush = new SolidBrush(Color.LightSlateGray);
Pen shPen = new Pen(Color.Black);
Point pts;
pts = new new Point(150, 100), new Point(200, 100), new Point(150, 200), new Point(200, 200) ; // 4
GraphicsPath pth = new GraphicsPath();
pth = ShapeData(0);
g.FillPath(bgBrush, pth); // fill
g.DrawPath(shPen, pth); // border
Int16 Angle = 30;
Matrix RotMatrix = new Matrix(); // rotate matrix to put the shape back to initial coordinates (with bottom left point as reference)
RotMatrix.Rotate(Angle, MatrixOrder.Append); // Rotate the pattern to the desired angle
RotMatrix.TransformPoints(pts); // carry out matrix transformation over the shape points
2) Draw 4 points already rotated
C#
Dim Img As Image = New Bitmap(Me.Picturebox1.Width, Me.Picturebox1.Height)
Dim g As Graphics = Graphics.FromImage(Img)
Dim bgBrush As SolidBrush = New SolidBrush(Color.LightSlateGray)
Dim shPen As Pen = New Pen(Color.Black)
Dim pts() As Point
pts = New Point(150, 100), ' 1 - rotated coordinates
New Point(210, 110), ' 2 - rotated coordinates
New Point(140, 200), ' 3 - rotated coordinates
New Point(200, 210) ' 4 - rotated coordinates
Dim pth As New GraphicsPath
pth = ShapeData(0)
g.FillPath(bgBrush, pth) ' fill
g.DrawPath(shPen, pth) ' border
VB.NET
Image Img = new Bitmap(this.Picturebox1.Width, this.Picturebox1.Height);
Graphics g = Graphics.FromImage(Img);
SolidBrush bgBrush = new SolidBrush(Color.LightSlateGray);
Pen shPen = new Pen(Color.Black);
Point pts;
pts = new new Point(150, 100), new Point(210, 110), new Point(140, 200), new Point(200, 210) ; // 4
GraphicsPath pth = new GraphicsPath();
pth = ShapeData(0);
g.FillPath(bgBrush, pth); // fill
g.DrawPath(shPen, pth); // border
3) Draw rectangle and rotate graphics. This might be little bit tricky.
C#
Image Img = new Bitmap(this.Picturebox1.Width, this.Picturebox1.Height);
Graphics g = Graphics.FromImage(Img);
SolidBrush bgBrush = new SolidBrush(Color.LightSlateGray);
Pen shPen = new Pen(Color.Black);
Int16 dDimX = 75;
Int16 dDimY = 150;
Int16 dLocX = 200;
Int16 dLocY = 100;
Rectangle rect = new Rectangle(dLocX, dLocY, dDimX, dDimY);
g.RotateTransform(30);
VB.NET
Dim Img As Image = New Bitmap(Me.Picturebox1.Width, Me.Picturebox1.Height)
Dim g As Graphics = Graphics.FromImage(Img)
Dim bgBrush As SolidBrush = New SolidBrush(Color.LightSlateGray)
Dim shPen As Pen = New Pen(Color.Black)
Dim dDimX As Int16 = 75
Dim dDimY As Int16 = 150
Dim dLocX As Int16 = 200
Dim dLocY As Int16 = 100
Dim rect As Rectangle = New Rectangle(dLocX, dLocY, dDimX, dDimY)
g.RotateTransform(30)
add a comment |
Well, while you could use ImageMagic, the .Net libraries are sufficient.
There are few ways to draw shape like rotated rectangle.
1) Draw 4 points (you could draw any shape this way, not only rectangle), rotate it
C#
Dim Img As Image = New Bitmap(Me.Picturebox1.Width, Me.Picturebox1.Height)
Dim g As Graphics = Graphics.FromImage(Img)
Dim bgBrush As SolidBrush = New SolidBrush(Color.LightSlateGray)
Dim shPen As Pen = New Pen(Color.Black)
Dim pts() As Point
pts = New Point(150, 100), ' 1
New Point(200, 100), ' 2
New Point(150, 200), ' 3
New Point(200, 200) ' 4
Dim pth As New GraphicsPath
pth = ShapeData(0)
g.FillPath(bgBrush, pth) ' fill
g.DrawPath(shPen, pth) ' border
Dim Angle As Int16 = 30
Dim RotMatrix As New Matrix ' rotate matrix to put the shape back to initial coordinates (with bottom left point as reference)
RotMatrix.Rotate(Angle, MatrixOrder.Append) ' Rotate the pattern to the desired angle
RotMatrix.TransformPoints(pts) ' carry out matrix transformation over the shape points
VB.NET
Image Img = new Bitmap(this.Picturebox1.Width, this.Picturebox1.Height);
Graphics g = Graphics.FromImage(Img);
SolidBrush bgBrush = new SolidBrush(Color.LightSlateGray);
Pen shPen = new Pen(Color.Black);
Point pts;
pts = new new Point(150, 100), new Point(200, 100), new Point(150, 200), new Point(200, 200) ; // 4
GraphicsPath pth = new GraphicsPath();
pth = ShapeData(0);
g.FillPath(bgBrush, pth); // fill
g.DrawPath(shPen, pth); // border
Int16 Angle = 30;
Matrix RotMatrix = new Matrix(); // rotate matrix to put the shape back to initial coordinates (with bottom left point as reference)
RotMatrix.Rotate(Angle, MatrixOrder.Append); // Rotate the pattern to the desired angle
RotMatrix.TransformPoints(pts); // carry out matrix transformation over the shape points
2) Draw 4 points already rotated
C#
Dim Img As Image = New Bitmap(Me.Picturebox1.Width, Me.Picturebox1.Height)
Dim g As Graphics = Graphics.FromImage(Img)
Dim bgBrush As SolidBrush = New SolidBrush(Color.LightSlateGray)
Dim shPen As Pen = New Pen(Color.Black)
Dim pts() As Point
pts = New Point(150, 100), ' 1 - rotated coordinates
New Point(210, 110), ' 2 - rotated coordinates
New Point(140, 200), ' 3 - rotated coordinates
New Point(200, 210) ' 4 - rotated coordinates
Dim pth As New GraphicsPath
pth = ShapeData(0)
g.FillPath(bgBrush, pth) ' fill
g.DrawPath(shPen, pth) ' border
VB.NET
Image Img = new Bitmap(this.Picturebox1.Width, this.Picturebox1.Height);
Graphics g = Graphics.FromImage(Img);
SolidBrush bgBrush = new SolidBrush(Color.LightSlateGray);
Pen shPen = new Pen(Color.Black);
Point pts;
pts = new new Point(150, 100), new Point(210, 110), new Point(140, 200), new Point(200, 210) ; // 4
GraphicsPath pth = new GraphicsPath();
pth = ShapeData(0);
g.FillPath(bgBrush, pth); // fill
g.DrawPath(shPen, pth); // border
3) Draw rectangle and rotate graphics. This might be little bit tricky.
C#
Image Img = new Bitmap(this.Picturebox1.Width, this.Picturebox1.Height);
Graphics g = Graphics.FromImage(Img);
SolidBrush bgBrush = new SolidBrush(Color.LightSlateGray);
Pen shPen = new Pen(Color.Black);
Int16 dDimX = 75;
Int16 dDimY = 150;
Int16 dLocX = 200;
Int16 dLocY = 100;
Rectangle rect = new Rectangle(dLocX, dLocY, dDimX, dDimY);
g.RotateTransform(30);
VB.NET
Dim Img As Image = New Bitmap(Me.Picturebox1.Width, Me.Picturebox1.Height)
Dim g As Graphics = Graphics.FromImage(Img)
Dim bgBrush As SolidBrush = New SolidBrush(Color.LightSlateGray)
Dim shPen As Pen = New Pen(Color.Black)
Dim dDimX As Int16 = 75
Dim dDimY As Int16 = 150
Dim dLocX As Int16 = 200
Dim dLocY As Int16 = 100
Dim rect As Rectangle = New Rectangle(dLocX, dLocY, dDimX, dDimY)
g.RotateTransform(30)
Well, while you could use ImageMagic, the .Net libraries are sufficient.
There are few ways to draw shape like rotated rectangle.
1) Draw 4 points (you could draw any shape this way, not only rectangle), rotate it
C#
Dim Img As Image = New Bitmap(Me.Picturebox1.Width, Me.Picturebox1.Height)
Dim g As Graphics = Graphics.FromImage(Img)
Dim bgBrush As SolidBrush = New SolidBrush(Color.LightSlateGray)
Dim shPen As Pen = New Pen(Color.Black)
Dim pts() As Point
pts = New Point(150, 100), ' 1
New Point(200, 100), ' 2
New Point(150, 200), ' 3
New Point(200, 200) ' 4
Dim pth As New GraphicsPath
pth = ShapeData(0)
g.FillPath(bgBrush, pth) ' fill
g.DrawPath(shPen, pth) ' border
Dim Angle As Int16 = 30
Dim RotMatrix As New Matrix ' rotate matrix to put the shape back to initial coordinates (with bottom left point as reference)
RotMatrix.Rotate(Angle, MatrixOrder.Append) ' Rotate the pattern to the desired angle
RotMatrix.TransformPoints(pts) ' carry out matrix transformation over the shape points
VB.NET
Image Img = new Bitmap(this.Picturebox1.Width, this.Picturebox1.Height);
Graphics g = Graphics.FromImage(Img);
SolidBrush bgBrush = new SolidBrush(Color.LightSlateGray);
Pen shPen = new Pen(Color.Black);
Point pts;
pts = new new Point(150, 100), new Point(200, 100), new Point(150, 200), new Point(200, 200) ; // 4
GraphicsPath pth = new GraphicsPath();
pth = ShapeData(0);
g.FillPath(bgBrush, pth); // fill
g.DrawPath(shPen, pth); // border
Int16 Angle = 30;
Matrix RotMatrix = new Matrix(); // rotate matrix to put the shape back to initial coordinates (with bottom left point as reference)
RotMatrix.Rotate(Angle, MatrixOrder.Append); // Rotate the pattern to the desired angle
RotMatrix.TransformPoints(pts); // carry out matrix transformation over the shape points
2) Draw 4 points already rotated
C#
Dim Img As Image = New Bitmap(Me.Picturebox1.Width, Me.Picturebox1.Height)
Dim g As Graphics = Graphics.FromImage(Img)
Dim bgBrush As SolidBrush = New SolidBrush(Color.LightSlateGray)
Dim shPen As Pen = New Pen(Color.Black)
Dim pts() As Point
pts = New Point(150, 100), ' 1 - rotated coordinates
New Point(210, 110), ' 2 - rotated coordinates
New Point(140, 200), ' 3 - rotated coordinates
New Point(200, 210) ' 4 - rotated coordinates
Dim pth As New GraphicsPath
pth = ShapeData(0)
g.FillPath(bgBrush, pth) ' fill
g.DrawPath(shPen, pth) ' border
VB.NET
Image Img = new Bitmap(this.Picturebox1.Width, this.Picturebox1.Height);
Graphics g = Graphics.FromImage(Img);
SolidBrush bgBrush = new SolidBrush(Color.LightSlateGray);
Pen shPen = new Pen(Color.Black);
Point pts;
pts = new new Point(150, 100), new Point(210, 110), new Point(140, 200), new Point(200, 210) ; // 4
GraphicsPath pth = new GraphicsPath();
pth = ShapeData(0);
g.FillPath(bgBrush, pth); // fill
g.DrawPath(shPen, pth); // border
3) Draw rectangle and rotate graphics. This might be little bit tricky.
C#
Image Img = new Bitmap(this.Picturebox1.Width, this.Picturebox1.Height);
Graphics g = Graphics.FromImage(Img);
SolidBrush bgBrush = new SolidBrush(Color.LightSlateGray);
Pen shPen = new Pen(Color.Black);
Int16 dDimX = 75;
Int16 dDimY = 150;
Int16 dLocX = 200;
Int16 dLocY = 100;
Rectangle rect = new Rectangle(dLocX, dLocY, dDimX, dDimY);
g.RotateTransform(30);
VB.NET
Dim Img As Image = New Bitmap(Me.Picturebox1.Width, Me.Picturebox1.Height)
Dim g As Graphics = Graphics.FromImage(Img)
Dim bgBrush As SolidBrush = New SolidBrush(Color.LightSlateGray)
Dim shPen As Pen = New Pen(Color.Black)
Dim dDimX As Int16 = 75
Dim dDimY As Int16 = 150
Dim dLocX As Int16 = 200
Dim dLocY As Int16 = 100
Dim rect As Rectangle = New Rectangle(dLocX, dLocY, dDimX, dDimY)
g.RotateTransform(30)
answered Dec 26 '18 at 16:18


Oak_3260548Oak_3260548
568519
568519
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53257195%2fhow-can-i-fill-a-rotated-rectangle-over-an-image%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
MN5vc0wX,iTv