SQL WITH SELECT How to loop through
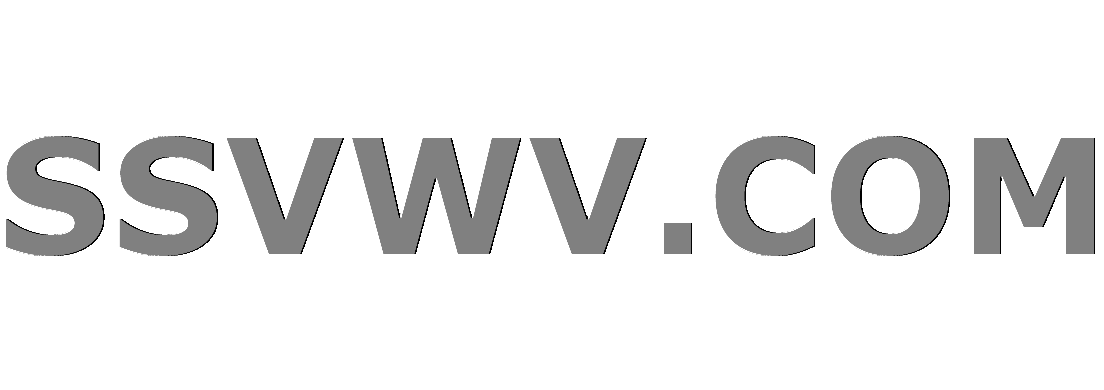
Multi tool use
I am trying to achieve something like this:
I have two large tables and there is no common key between them. Obviously when I want to join them, it is a Cartesian product like m x n rows. I want to avoid that. I will read one record from tableA
and then match it with tableB
all the rows but at least I will have control over how many records I have processed in tableA
.
Currently, I am not knowing how far the query has executed.
My question is can I do that in WITH
statement:
I have taken a simple case say EMP
table from SCOTT/TIGER
example and one record at a time would match with DEPT
table and return DNAME
. I have written this query but is not doing as expected. And how to reference DNAME
from 2nd query:
with emp_rec as (select * from emp),
dept_rec as (select dname
from dept, emp_rec
where dept.deptno in (10, 20) and
dept.deptno = emp_rec.deptno)
select *
from emp_rec
Results which I am expecting is similar to joining EMP and DEPT tables on emp/deptno = dept/deptno. But I want to do it one EMP record at a time without CURSOR or STORED PROCEDURE in pure SQL. Hope I was able to explain what is on my mind.
What is that I am doing wrong, please help.
thank you
Hi,
I have been able to solve my problem. Instead of SELECTING from EMP_REC I changed to DEPT_REC, it worked. Here is the new query:
with emp_rec as (select * from emp),
dept_rec as (select emp_rec.*, dname
from dept, emp_rec
where dept.deptno in (10, 20) and
dept.deptno = emp_rec.deptno)
select *
from dept_rec
thanks
I am able to control both queries separately. And the restriction, that subQueries do not refer to parentQuery's variables, is gone.
sql postgresql-9.6
|
show 1 more comment
I am trying to achieve something like this:
I have two large tables and there is no common key between them. Obviously when I want to join them, it is a Cartesian product like m x n rows. I want to avoid that. I will read one record from tableA
and then match it with tableB
all the rows but at least I will have control over how many records I have processed in tableA
.
Currently, I am not knowing how far the query has executed.
My question is can I do that in WITH
statement:
I have taken a simple case say EMP
table from SCOTT/TIGER
example and one record at a time would match with DEPT
table and return DNAME
. I have written this query but is not doing as expected. And how to reference DNAME
from 2nd query:
with emp_rec as (select * from emp),
dept_rec as (select dname
from dept, emp_rec
where dept.deptno in (10, 20) and
dept.deptno = emp_rec.deptno)
select *
from emp_rec
Results which I am expecting is similar to joining EMP and DEPT tables on emp/deptno = dept/deptno. But I want to do it one EMP record at a time without CURSOR or STORED PROCEDURE in pure SQL. Hope I was able to explain what is on my mind.
What is that I am doing wrong, please help.
thank you
Hi,
I have been able to solve my problem. Instead of SELECTING from EMP_REC I changed to DEPT_REC, it worked. Here is the new query:
with emp_rec as (select * from emp),
dept_rec as (select emp_rec.*, dname
from dept, emp_rec
where dept.deptno in (10, 20) and
dept.deptno = emp_rec.deptno)
select *
from dept_rec
thanks
I am able to control both queries separately. And the restriction, that subQueries do not refer to parentQuery's variables, is gone.
sql postgresql-9.6
3
It would be helpful if you show some input data and expected output..
– Franco Piccolo
Nov 12 '18 at 6:32
Any reason this question was down voted.
– BB23850
Nov 12 '18 at 6:59
FWIW I didn't downvote it, but as suggested I would reframe the question with input data and expected outputs
– Franco Piccolo
Nov 12 '18 at 7:01
Franco Piccolo Never mind about up votes and down votes. Great thing is problem solved.
– BB23850
Nov 12 '18 at 7:20
If you have solved your problem, you should create an answer and accept it. Otherwise this question will remain as "unsolved"
– a_horse_with_no_name
Nov 12 '18 at 7:20
|
show 1 more comment
I am trying to achieve something like this:
I have two large tables and there is no common key between them. Obviously when I want to join them, it is a Cartesian product like m x n rows. I want to avoid that. I will read one record from tableA
and then match it with tableB
all the rows but at least I will have control over how many records I have processed in tableA
.
Currently, I am not knowing how far the query has executed.
My question is can I do that in WITH
statement:
I have taken a simple case say EMP
table from SCOTT/TIGER
example and one record at a time would match with DEPT
table and return DNAME
. I have written this query but is not doing as expected. And how to reference DNAME
from 2nd query:
with emp_rec as (select * from emp),
dept_rec as (select dname
from dept, emp_rec
where dept.deptno in (10, 20) and
dept.deptno = emp_rec.deptno)
select *
from emp_rec
Results which I am expecting is similar to joining EMP and DEPT tables on emp/deptno = dept/deptno. But I want to do it one EMP record at a time without CURSOR or STORED PROCEDURE in pure SQL. Hope I was able to explain what is on my mind.
What is that I am doing wrong, please help.
thank you
Hi,
I have been able to solve my problem. Instead of SELECTING from EMP_REC I changed to DEPT_REC, it worked. Here is the new query:
with emp_rec as (select * from emp),
dept_rec as (select emp_rec.*, dname
from dept, emp_rec
where dept.deptno in (10, 20) and
dept.deptno = emp_rec.deptno)
select *
from dept_rec
thanks
I am able to control both queries separately. And the restriction, that subQueries do not refer to parentQuery's variables, is gone.
sql postgresql-9.6
I am trying to achieve something like this:
I have two large tables and there is no common key between them. Obviously when I want to join them, it is a Cartesian product like m x n rows. I want to avoid that. I will read one record from tableA
and then match it with tableB
all the rows but at least I will have control over how many records I have processed in tableA
.
Currently, I am not knowing how far the query has executed.
My question is can I do that in WITH
statement:
I have taken a simple case say EMP
table from SCOTT/TIGER
example and one record at a time would match with DEPT
table and return DNAME
. I have written this query but is not doing as expected. And how to reference DNAME
from 2nd query:
with emp_rec as (select * from emp),
dept_rec as (select dname
from dept, emp_rec
where dept.deptno in (10, 20) and
dept.deptno = emp_rec.deptno)
select *
from emp_rec
Results which I am expecting is similar to joining EMP and DEPT tables on emp/deptno = dept/deptno. But I want to do it one EMP record at a time without CURSOR or STORED PROCEDURE in pure SQL. Hope I was able to explain what is on my mind.
What is that I am doing wrong, please help.
thank you
Hi,
I have been able to solve my problem. Instead of SELECTING from EMP_REC I changed to DEPT_REC, it worked. Here is the new query:
with emp_rec as (select * from emp),
dept_rec as (select emp_rec.*, dname
from dept, emp_rec
where dept.deptno in (10, 20) and
dept.deptno = emp_rec.deptno)
select *
from dept_rec
thanks
I am able to control both queries separately. And the restriction, that subQueries do not refer to parentQuery's variables, is gone.
sql postgresql-9.6
sql postgresql-9.6
edited Nov 12 '18 at 7:18
BB23850
asked Nov 12 '18 at 6:25
BB23850BB23850
237
237
3
It would be helpful if you show some input data and expected output..
– Franco Piccolo
Nov 12 '18 at 6:32
Any reason this question was down voted.
– BB23850
Nov 12 '18 at 6:59
FWIW I didn't downvote it, but as suggested I would reframe the question with input data and expected outputs
– Franco Piccolo
Nov 12 '18 at 7:01
Franco Piccolo Never mind about up votes and down votes. Great thing is problem solved.
– BB23850
Nov 12 '18 at 7:20
If you have solved your problem, you should create an answer and accept it. Otherwise this question will remain as "unsolved"
– a_horse_with_no_name
Nov 12 '18 at 7:20
|
show 1 more comment
3
It would be helpful if you show some input data and expected output..
– Franco Piccolo
Nov 12 '18 at 6:32
Any reason this question was down voted.
– BB23850
Nov 12 '18 at 6:59
FWIW I didn't downvote it, but as suggested I would reframe the question with input data and expected outputs
– Franco Piccolo
Nov 12 '18 at 7:01
Franco Piccolo Never mind about up votes and down votes. Great thing is problem solved.
– BB23850
Nov 12 '18 at 7:20
If you have solved your problem, you should create an answer and accept it. Otherwise this question will remain as "unsolved"
– a_horse_with_no_name
Nov 12 '18 at 7:20
3
3
It would be helpful if you show some input data and expected output..
– Franco Piccolo
Nov 12 '18 at 6:32
It would be helpful if you show some input data and expected output..
– Franco Piccolo
Nov 12 '18 at 6:32
Any reason this question was down voted.
– BB23850
Nov 12 '18 at 6:59
Any reason this question was down voted.
– BB23850
Nov 12 '18 at 6:59
FWIW I didn't downvote it, but as suggested I would reframe the question with input data and expected outputs
– Franco Piccolo
Nov 12 '18 at 7:01
FWIW I didn't downvote it, but as suggested I would reframe the question with input data and expected outputs
– Franco Piccolo
Nov 12 '18 at 7:01
Franco Piccolo Never mind about up votes and down votes. Great thing is problem solved.
– BB23850
Nov 12 '18 at 7:20
Franco Piccolo Never mind about up votes and down votes. Great thing is problem solved.
– BB23850
Nov 12 '18 at 7:20
If you have solved your problem, you should create an answer and accept it. Otherwise this question will remain as "unsolved"
– a_horse_with_no_name
Nov 12 '18 at 7:20
If you have solved your problem, you should create an answer and accept it. Otherwise this question will remain as "unsolved"
– a_horse_with_no_name
Nov 12 '18 at 7:20
|
show 1 more comment
1 Answer
1
active
oldest
votes
WITH statement provides multiple queries to interact with one another. This is different from subqueries in FROM clause where each query is independent of other.
Another feature of CTE which became useful here, the final query can call any of the subqueries in WITH clause. I had two subqueries in WITH clause EMP_REC and DEPT_REC. I called DEPT_REC and it worked.
To understand the data in this query please use Oracle's SCOTT/TIGER database which has standard tables EMP (14 rows), DEPT etc.
thanks for bearing with me
bb23850
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53256881%2fsql-with-select-how-to-loop-through%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
WITH statement provides multiple queries to interact with one another. This is different from subqueries in FROM clause where each query is independent of other.
Another feature of CTE which became useful here, the final query can call any of the subqueries in WITH clause. I had two subqueries in WITH clause EMP_REC and DEPT_REC. I called DEPT_REC and it worked.
To understand the data in this query please use Oracle's SCOTT/TIGER database which has standard tables EMP (14 rows), DEPT etc.
thanks for bearing with me
bb23850
add a comment |
WITH statement provides multiple queries to interact with one another. This is different from subqueries in FROM clause where each query is independent of other.
Another feature of CTE which became useful here, the final query can call any of the subqueries in WITH clause. I had two subqueries in WITH clause EMP_REC and DEPT_REC. I called DEPT_REC and it worked.
To understand the data in this query please use Oracle's SCOTT/TIGER database which has standard tables EMP (14 rows), DEPT etc.
thanks for bearing with me
bb23850
add a comment |
WITH statement provides multiple queries to interact with one another. This is different from subqueries in FROM clause where each query is independent of other.
Another feature of CTE which became useful here, the final query can call any of the subqueries in WITH clause. I had two subqueries in WITH clause EMP_REC and DEPT_REC. I called DEPT_REC and it worked.
To understand the data in this query please use Oracle's SCOTT/TIGER database which has standard tables EMP (14 rows), DEPT etc.
thanks for bearing with me
bb23850
WITH statement provides multiple queries to interact with one another. This is different from subqueries in FROM clause where each query is independent of other.
Another feature of CTE which became useful here, the final query can call any of the subqueries in WITH clause. I had two subqueries in WITH clause EMP_REC and DEPT_REC. I called DEPT_REC and it worked.
To understand the data in this query please use Oracle's SCOTT/TIGER database which has standard tables EMP (14 rows), DEPT etc.
thanks for bearing with me
bb23850
answered Nov 12 '18 at 14:12
BB23850BB23850
237
237
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53256881%2fsql-with-select-how-to-loop-through%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
w1ST jRRxRZIghh1qx8NgAHTjZ,HGqm,D2E0GJeezVKgXwYbS4mOyF1mMarhzMfu,lryc
3
It would be helpful if you show some input data and expected output..
– Franco Piccolo
Nov 12 '18 at 6:32
Any reason this question was down voted.
– BB23850
Nov 12 '18 at 6:59
FWIW I didn't downvote it, but as suggested I would reframe the question with input data and expected outputs
– Franco Piccolo
Nov 12 '18 at 7:01
Franco Piccolo Never mind about up votes and down votes. Great thing is problem solved.
– BB23850
Nov 12 '18 at 7:20
If you have solved your problem, you should create an answer and accept it. Otherwise this question will remain as "unsolved"
– a_horse_with_no_name
Nov 12 '18 at 7:20