Julia @distributed: subsequent code run before all workers finish
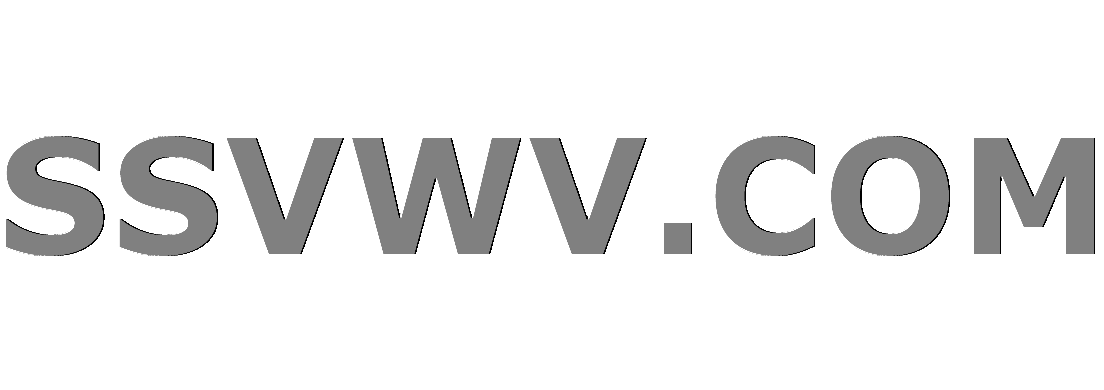
Multi tool use
I have been headbutting on a wall for a few days around this code:
using Distributed
using SharedArrays
# Dimension size
M=10;
N=100;
z_ijw = zeros(Float64,M,N,M)
z_ijw_tmp = SharedArrayFloat64(M*M*N)
i2s = CartesianIndices(z_ijw)
@distributed for iall=1:(M*M*N)
# get index
i=i2s[iall][1]
j=i2s[iall][2]
w=i2s[iall][3]
# Assign function value
z_ijw_tmp[iall]=sqrt(i+j+w) # Any random function would do
end
# Print the last element of the array
println(z_ijw_tmp[end])
println(z_ijw_tmp[end])
println(z_ijw_tmp[end])
The first printed out number is always 0, the second number is either 0 or 10.95... (sqrt of 120, which is correct). The 3rd is either 0 or 10.95 (if the 2nd is 0)
So it appears that the print code (@mainthread?) is allowed to run before all the workers finish. Is there anyway for the print code to run properly the first time (without a wait command)
Without multiple println, I thought it was a problem with scope and spend a few days reading about it @.@
asynchronous julia-lang distributed
add a comment |
I have been headbutting on a wall for a few days around this code:
using Distributed
using SharedArrays
# Dimension size
M=10;
N=100;
z_ijw = zeros(Float64,M,N,M)
z_ijw_tmp = SharedArrayFloat64(M*M*N)
i2s = CartesianIndices(z_ijw)
@distributed for iall=1:(M*M*N)
# get index
i=i2s[iall][1]
j=i2s[iall][2]
w=i2s[iall][3]
# Assign function value
z_ijw_tmp[iall]=sqrt(i+j+w) # Any random function would do
end
# Print the last element of the array
println(z_ijw_tmp[end])
println(z_ijw_tmp[end])
println(z_ijw_tmp[end])
The first printed out number is always 0, the second number is either 0 or 10.95... (sqrt of 120, which is correct). The 3rd is either 0 or 10.95 (if the 2nd is 0)
So it appears that the print code (@mainthread?) is allowed to run before all the workers finish. Is there anyway for the print code to run properly the first time (without a wait command)
Without multiple println, I thought it was a problem with scope and spend a few days reading about it @.@
asynchronous julia-lang distributed
add a comment |
I have been headbutting on a wall for a few days around this code:
using Distributed
using SharedArrays
# Dimension size
M=10;
N=100;
z_ijw = zeros(Float64,M,N,M)
z_ijw_tmp = SharedArrayFloat64(M*M*N)
i2s = CartesianIndices(z_ijw)
@distributed for iall=1:(M*M*N)
# get index
i=i2s[iall][1]
j=i2s[iall][2]
w=i2s[iall][3]
# Assign function value
z_ijw_tmp[iall]=sqrt(i+j+w) # Any random function would do
end
# Print the last element of the array
println(z_ijw_tmp[end])
println(z_ijw_tmp[end])
println(z_ijw_tmp[end])
The first printed out number is always 0, the second number is either 0 or 10.95... (sqrt of 120, which is correct). The 3rd is either 0 or 10.95 (if the 2nd is 0)
So it appears that the print code (@mainthread?) is allowed to run before all the workers finish. Is there anyway for the print code to run properly the first time (without a wait command)
Without multiple println, I thought it was a problem with scope and spend a few days reading about it @.@
asynchronous julia-lang distributed
I have been headbutting on a wall for a few days around this code:
using Distributed
using SharedArrays
# Dimension size
M=10;
N=100;
z_ijw = zeros(Float64,M,N,M)
z_ijw_tmp = SharedArrayFloat64(M*M*N)
i2s = CartesianIndices(z_ijw)
@distributed for iall=1:(M*M*N)
# get index
i=i2s[iall][1]
j=i2s[iall][2]
w=i2s[iall][3]
# Assign function value
z_ijw_tmp[iall]=sqrt(i+j+w) # Any random function would do
end
# Print the last element of the array
println(z_ijw_tmp[end])
println(z_ijw_tmp[end])
println(z_ijw_tmp[end])
The first printed out number is always 0, the second number is either 0 or 10.95... (sqrt of 120, which is correct). The 3rd is either 0 or 10.95 (if the 2nd is 0)
So it appears that the print code (@mainthread?) is allowed to run before all the workers finish. Is there anyway for the print code to run properly the first time (without a wait command)
Without multiple println, I thought it was a problem with scope and spend a few days reading about it @.@
asynchronous julia-lang distributed
asynchronous julia-lang distributed
edited Nov 12 '18 at 14:44
Huy Tran
asked Nov 12 '18 at 14:08
Huy TranHuy Tran
112
112
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
@distributed
with a reducer function, i.e. @distributed (+)
, will be synced, whereas @distributed
without a reducer function will be started asynchronously.
Putting a @sync
in front of your @distributed
should make the code behave the way you want it to.
This is also noted in the documentation here:
Note that without a reducer function, @distributed executes asynchronously, i.e. it spawns independent tasks on all available workers and returns immediately without waiting for completion. To wait for completion, prefix the call with @sync
Thank you!! I am surprised I this is not common problem for everyone else. All examples are to be applied on REPL (step by step), so the problem only emerges when you run it all in a script.
– Huy Tran
Nov 12 '18 at 17:06
I don't think it's a problem at all. It's well defined and documented behavior.
– crstnbr
Nov 12 '18 at 18:56
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53263892%2fjulia-distributed-subsequent-code-run-before-all-workers-finish%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
@distributed
with a reducer function, i.e. @distributed (+)
, will be synced, whereas @distributed
without a reducer function will be started asynchronously.
Putting a @sync
in front of your @distributed
should make the code behave the way you want it to.
This is also noted in the documentation here:
Note that without a reducer function, @distributed executes asynchronously, i.e. it spawns independent tasks on all available workers and returns immediately without waiting for completion. To wait for completion, prefix the call with @sync
Thank you!! I am surprised I this is not common problem for everyone else. All examples are to be applied on REPL (step by step), so the problem only emerges when you run it all in a script.
– Huy Tran
Nov 12 '18 at 17:06
I don't think it's a problem at all. It's well defined and documented behavior.
– crstnbr
Nov 12 '18 at 18:56
add a comment |
@distributed
with a reducer function, i.e. @distributed (+)
, will be synced, whereas @distributed
without a reducer function will be started asynchronously.
Putting a @sync
in front of your @distributed
should make the code behave the way you want it to.
This is also noted in the documentation here:
Note that without a reducer function, @distributed executes asynchronously, i.e. it spawns independent tasks on all available workers and returns immediately without waiting for completion. To wait for completion, prefix the call with @sync
Thank you!! I am surprised I this is not common problem for everyone else. All examples are to be applied on REPL (step by step), so the problem only emerges when you run it all in a script.
– Huy Tran
Nov 12 '18 at 17:06
I don't think it's a problem at all. It's well defined and documented behavior.
– crstnbr
Nov 12 '18 at 18:56
add a comment |
@distributed
with a reducer function, i.e. @distributed (+)
, will be synced, whereas @distributed
without a reducer function will be started asynchronously.
Putting a @sync
in front of your @distributed
should make the code behave the way you want it to.
This is also noted in the documentation here:
Note that without a reducer function, @distributed executes asynchronously, i.e. it spawns independent tasks on all available workers and returns immediately without waiting for completion. To wait for completion, prefix the call with @sync
@distributed
with a reducer function, i.e. @distributed (+)
, will be synced, whereas @distributed
without a reducer function will be started asynchronously.
Putting a @sync
in front of your @distributed
should make the code behave the way you want it to.
This is also noted in the documentation here:
Note that without a reducer function, @distributed executes asynchronously, i.e. it spawns independent tasks on all available workers and returns immediately without waiting for completion. To wait for completion, prefix the call with @sync
answered Nov 12 '18 at 15:43


crstnbrcrstnbr
3,81411022
3,81411022
Thank you!! I am surprised I this is not common problem for everyone else. All examples are to be applied on REPL (step by step), so the problem only emerges when you run it all in a script.
– Huy Tran
Nov 12 '18 at 17:06
I don't think it's a problem at all. It's well defined and documented behavior.
– crstnbr
Nov 12 '18 at 18:56
add a comment |
Thank you!! I am surprised I this is not common problem for everyone else. All examples are to be applied on REPL (step by step), so the problem only emerges when you run it all in a script.
– Huy Tran
Nov 12 '18 at 17:06
I don't think it's a problem at all. It's well defined and documented behavior.
– crstnbr
Nov 12 '18 at 18:56
Thank you!! I am surprised I this is not common problem for everyone else. All examples are to be applied on REPL (step by step), so the problem only emerges when you run it all in a script.
– Huy Tran
Nov 12 '18 at 17:06
Thank you!! I am surprised I this is not common problem for everyone else. All examples are to be applied on REPL (step by step), so the problem only emerges when you run it all in a script.
– Huy Tran
Nov 12 '18 at 17:06
I don't think it's a problem at all. It's well defined and documented behavior.
– crstnbr
Nov 12 '18 at 18:56
I don't think it's a problem at all. It's well defined and documented behavior.
– crstnbr
Nov 12 '18 at 18:56
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53263892%2fjulia-distributed-subsequent-code-run-before-all-workers-finish%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KJRGAR97Zd7XwIe0FDv mQ,hN40E