jQuery Ajax - how to get response data in error
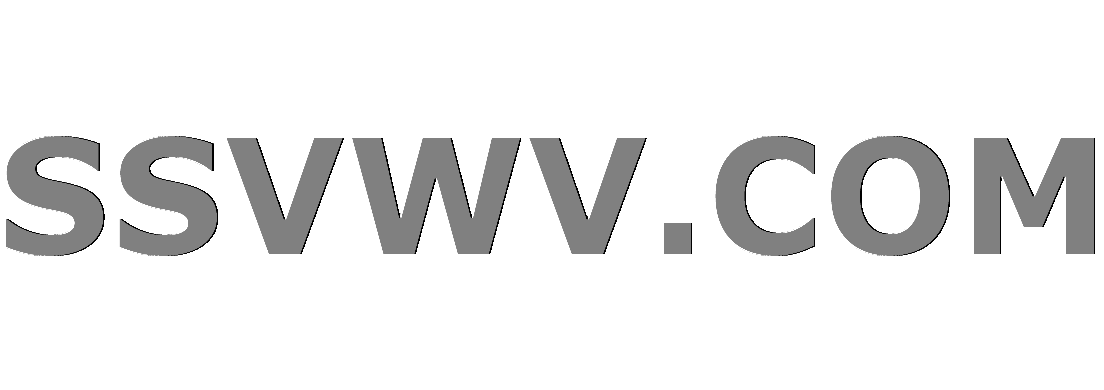
Multi tool use
up vote
46
down vote
favorite
I have a simple web application.
I've created the server REST API so it will return a response with HTTP code and a JSON (or XML) object with more details: application code (specific to scenario, message that describe what happened etc.).
So, for example if a client send a Register request and the password is too short, the response HTTP code will be 400 (Bad Request), and the response data will be: appCode : 1020 , message : "Password is too short"
.
In jQuery I'm using the "ajax" function to create a POST request. When the server returns something different from HTTP code 200 (OK), jQuery defines it as "error".
The error handler can get 3 parameters: jqXHR, textStatus, errorThrown.
Ho can I get the JSON object that sent by the server in error case?
Edit:
1) Here is my JS code:
function register (userName, password)
var postData = ;
postData["userName"] = userName;
postData["password"] = password;
$.ajax (
dataType: "json",
type: "POST",
url: "<server>/rest/register",
data: postData,
success: function(data)
showResultSucceed(data);
hideWaitingDone();
,
error: function (jqXHR, textStatus, errorThrown)
showResultFailed(jqXHR.responseText);
hideWaitingFail();
)
2) When looking at Firebug console, it seems like the response is empty.
When invoking the same request by using REST testing tool, I get a response with JSON object it it.
What am I doing wrong?
javascript jquery
add a comment |
up vote
46
down vote
favorite
I have a simple web application.
I've created the server REST API so it will return a response with HTTP code and a JSON (or XML) object with more details: application code (specific to scenario, message that describe what happened etc.).
So, for example if a client send a Register request and the password is too short, the response HTTP code will be 400 (Bad Request), and the response data will be: appCode : 1020 , message : "Password is too short"
.
In jQuery I'm using the "ajax" function to create a POST request. When the server returns something different from HTTP code 200 (OK), jQuery defines it as "error".
The error handler can get 3 parameters: jqXHR, textStatus, errorThrown.
Ho can I get the JSON object that sent by the server in error case?
Edit:
1) Here is my JS code:
function register (userName, password)
var postData = ;
postData["userName"] = userName;
postData["password"] = password;
$.ajax (
dataType: "json",
type: "POST",
url: "<server>/rest/register",
data: postData,
success: function(data)
showResultSucceed(data);
hideWaitingDone();
,
error: function (jqXHR, textStatus, errorThrown)
showResultFailed(jqXHR.responseText);
hideWaitingFail();
)
2) When looking at Firebug console, it seems like the response is empty.
When invoking the same request by using REST testing tool, I get a response with JSON object it it.
What am I doing wrong?
javascript jquery
1
In case you don't make it as far down as the accepted answer; there is nothing wrong with this code, the problem lies elsewhere.
– Tony
Jan 16 '13 at 21:22
For other future readers, I had a similar problem as well, and while the cross domain issue was completely irrelevant to my case, it turned out the problem was server side. (It seems the server code was broken in such a way that some combination of HTTP headers likeAccept
made it fail to return the correct response body on a 400 error.)
– jpmc26
Sep 5 '15 at 19:31
add a comment |
up vote
46
down vote
favorite
up vote
46
down vote
favorite
I have a simple web application.
I've created the server REST API so it will return a response with HTTP code and a JSON (or XML) object with more details: application code (specific to scenario, message that describe what happened etc.).
So, for example if a client send a Register request and the password is too short, the response HTTP code will be 400 (Bad Request), and the response data will be: appCode : 1020 , message : "Password is too short"
.
In jQuery I'm using the "ajax" function to create a POST request. When the server returns something different from HTTP code 200 (OK), jQuery defines it as "error".
The error handler can get 3 parameters: jqXHR, textStatus, errorThrown.
Ho can I get the JSON object that sent by the server in error case?
Edit:
1) Here is my JS code:
function register (userName, password)
var postData = ;
postData["userName"] = userName;
postData["password"] = password;
$.ajax (
dataType: "json",
type: "POST",
url: "<server>/rest/register",
data: postData,
success: function(data)
showResultSucceed(data);
hideWaitingDone();
,
error: function (jqXHR, textStatus, errorThrown)
showResultFailed(jqXHR.responseText);
hideWaitingFail();
)
2) When looking at Firebug console, it seems like the response is empty.
When invoking the same request by using REST testing tool, I get a response with JSON object it it.
What am I doing wrong?
javascript jquery
I have a simple web application.
I've created the server REST API so it will return a response with HTTP code and a JSON (or XML) object with more details: application code (specific to scenario, message that describe what happened etc.).
So, for example if a client send a Register request and the password is too short, the response HTTP code will be 400 (Bad Request), and the response data will be: appCode : 1020 , message : "Password is too short"
.
In jQuery I'm using the "ajax" function to create a POST request. When the server returns something different from HTTP code 200 (OK), jQuery defines it as "error".
The error handler can get 3 parameters: jqXHR, textStatus, errorThrown.
Ho can I get the JSON object that sent by the server in error case?
Edit:
1) Here is my JS code:
function register (userName, password)
var postData = ;
postData["userName"] = userName;
postData["password"] = password;
$.ajax (
dataType: "json",
type: "POST",
url: "<server>/rest/register",
data: postData,
success: function(data)
showResultSucceed(data);
hideWaitingDone();
,
error: function (jqXHR, textStatus, errorThrown)
showResultFailed(jqXHR.responseText);
hideWaitingFail();
)
2) When looking at Firebug console, it seems like the response is empty.
When invoking the same request by using REST testing tool, I get a response with JSON object it it.
What am I doing wrong?
javascript jquery
javascript jquery
edited Jan 31 '12 at 8:04
asked Jan 30 '12 at 16:02
Roy Tsabari
96851539
96851539
1
In case you don't make it as far down as the accepted answer; there is nothing wrong with this code, the problem lies elsewhere.
– Tony
Jan 16 '13 at 21:22
For other future readers, I had a similar problem as well, and while the cross domain issue was completely irrelevant to my case, it turned out the problem was server side. (It seems the server code was broken in such a way that some combination of HTTP headers likeAccept
made it fail to return the correct response body on a 400 error.)
– jpmc26
Sep 5 '15 at 19:31
add a comment |
1
In case you don't make it as far down as the accepted answer; there is nothing wrong with this code, the problem lies elsewhere.
– Tony
Jan 16 '13 at 21:22
For other future readers, I had a similar problem as well, and while the cross domain issue was completely irrelevant to my case, it turned out the problem was server side. (It seems the server code was broken in such a way that some combination of HTTP headers likeAccept
made it fail to return the correct response body on a 400 error.)
– jpmc26
Sep 5 '15 at 19:31
1
1
In case you don't make it as far down as the accepted answer; there is nothing wrong with this code, the problem lies elsewhere.
– Tony
Jan 16 '13 at 21:22
In case you don't make it as far down as the accepted answer; there is nothing wrong with this code, the problem lies elsewhere.
– Tony
Jan 16 '13 at 21:22
For other future readers, I had a similar problem as well, and while the cross domain issue was completely irrelevant to my case, it turned out the problem was server side. (It seems the server code was broken in such a way that some combination of HTTP headers like
Accept
made it fail to return the correct response body on a 400 error.)– jpmc26
Sep 5 '15 at 19:31
For other future readers, I had a similar problem as well, and while the cross domain issue was completely irrelevant to my case, it turned out the problem was server side. (It seems the server code was broken in such a way that some combination of HTTP headers like
Accept
made it fail to return the correct response body on a 400 error.)– jpmc26
Sep 5 '15 at 19:31
add a comment |
4 Answers
4
active
oldest
votes
up vote
35
down vote
Here's an example of how you get JSON data on error:
$.ajax(
url: '/path/to/script.php',
data: 'my':'data',
type: 'POST'
).fail(function($xhr)
var data = $xhr.responseJSON;
console.log(data);
);
From the docs:
If json is specified, the response is parsed using jQuery.parseJSON before being passed, as an object, to the success handler. The parsed JSON object is made available through the responseJSON property of the jqXHR object.
Otherwise, if responseJSON
is not available, you can try $.parseJSON($xhr.responseText)
.
"$.parseJSON($xhr.responseText)" is the key!. If instead of using ".fail" you use error: function(xmlHTTP, status, httperror) $.parseJSON(xmlHTTP.responseText);
– Shondeslitch
Jan 10 '17 at 11:42
add a comment |
up vote
25
down vote
directly from the docs
The jQuery XMLHttpRequest (jqXHR) object returned by $.ajax() as of
jQuery 1.5 is a superset of the browser's native XMLHttpRequest
object. For example, it contains responseText and responseXML
properties, as well as a getResponseHeader()
so use the jqXRH argument and get the responseText
property off it.
In the link above, look for the section entitled
The jqXHR Object
1
I read that. the responseText is empty
– Roy Tsabari
Jan 30 '12 at 16:11
@gdoron added the link and section to look for
– hvgotcodes
Jan 30 '12 at 16:13
@tibo, then show us your code. Something is off. Might be best to pause execution in your handler with firebug or webkit and just look at the properties of the arguments. It's gotta be there somewhere...
– hvgotcodes
Jan 30 '12 at 16:14
I just added my code to the question. Can you help?
– Roy Tsabari
Jan 31 '12 at 8:05
without actually digging in, I'm not sure at this point. Are you sure the response body is empty in firebug? Are you sure the url is correct? Are you sure the error handler is being called?
– hvgotcodes
Jan 31 '12 at 12:59
|
show 1 more comment
up vote
1
down vote
I also faced same problem when i was using multipart/form-data. At first I thought multipart/form-data created this mess, but later i found the proper solution.
1) JS code before:
var jersey_url = "http://localhost:8098/final/rest/addItem/upload";
var ans = $.ajax(
type: 'POST',
enctype: 'multipart/form-data',
url: jersey_url,
data: formData,
dataType: "json",
processData: false,
contentType: false
success : funtion(data)
var temp = JSON.parse(data);
console.log("SUCCESS : ", temp.message);
error : funtion($xhr,textStatus,errorThrown)
console.log("ERROR : ", errorThrown);
console.log("ERROR : ", $xhr);
console.log("ERROR : ", textStatus);
);
Here when error occurred, it showed me this in console :-
Error :
Error : abort : f(e), always : f(), .... , responseJSON :""message":"failed""
Error : error
Thus i came to know that we have to use $xhr.responseJSON to get the string message which we sent from rest api.
2) modified/working error funtion:
error : funtion($xhr,textStatus,errorThrown)
var string= $xhr.responseJSON;
var json_object= JSON.parse(string);
console.log("ERROR : ", json_object.message);
Thus will output "Error : failed" on console.
add a comment |
up vote
-3
down vote
accepted
After spending so much time on this problem, I found the problem.
The page is under the URL: www.mydomain.com/register
The REST api is under the URL: server.mydomain.com/rest
Seems like this kind of POST is not so simple.
I'm going to search more information to understand this issue better (if you have more information please share it with me).
When putting the REST API under www.mydomain.com/rest - everything is working fine.
2
You could accept one of the other answers.
– robsch
Apr 5 '17 at 15:06
add a comment |
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
35
down vote
Here's an example of how you get JSON data on error:
$.ajax(
url: '/path/to/script.php',
data: 'my':'data',
type: 'POST'
).fail(function($xhr)
var data = $xhr.responseJSON;
console.log(data);
);
From the docs:
If json is specified, the response is parsed using jQuery.parseJSON before being passed, as an object, to the success handler. The parsed JSON object is made available through the responseJSON property of the jqXHR object.
Otherwise, if responseJSON
is not available, you can try $.parseJSON($xhr.responseText)
.
"$.parseJSON($xhr.responseText)" is the key!. If instead of using ".fail" you use error: function(xmlHTTP, status, httperror) $.parseJSON(xmlHTTP.responseText);
– Shondeslitch
Jan 10 '17 at 11:42
add a comment |
up vote
35
down vote
Here's an example of how you get JSON data on error:
$.ajax(
url: '/path/to/script.php',
data: 'my':'data',
type: 'POST'
).fail(function($xhr)
var data = $xhr.responseJSON;
console.log(data);
);
From the docs:
If json is specified, the response is parsed using jQuery.parseJSON before being passed, as an object, to the success handler. The parsed JSON object is made available through the responseJSON property of the jqXHR object.
Otherwise, if responseJSON
is not available, you can try $.parseJSON($xhr.responseText)
.
"$.parseJSON($xhr.responseText)" is the key!. If instead of using ".fail" you use error: function(xmlHTTP, status, httperror) $.parseJSON(xmlHTTP.responseText);
– Shondeslitch
Jan 10 '17 at 11:42
add a comment |
up vote
35
down vote
up vote
35
down vote
Here's an example of how you get JSON data on error:
$.ajax(
url: '/path/to/script.php',
data: 'my':'data',
type: 'POST'
).fail(function($xhr)
var data = $xhr.responseJSON;
console.log(data);
);
From the docs:
If json is specified, the response is parsed using jQuery.parseJSON before being passed, as an object, to the success handler. The parsed JSON object is made available through the responseJSON property of the jqXHR object.
Otherwise, if responseJSON
is not available, you can try $.parseJSON($xhr.responseText)
.
Here's an example of how you get JSON data on error:
$.ajax(
url: '/path/to/script.php',
data: 'my':'data',
type: 'POST'
).fail(function($xhr)
var data = $xhr.responseJSON;
console.log(data);
);
From the docs:
If json is specified, the response is parsed using jQuery.parseJSON before being passed, as an object, to the success handler. The parsed JSON object is made available through the responseJSON property of the jqXHR object.
Otherwise, if responseJSON
is not available, you can try $.parseJSON($xhr.responseText)
.
edited Apr 27 '15 at 15:49
answered Dec 1 '14 at 23:16
mpen
120k166636932
120k166636932
"$.parseJSON($xhr.responseText)" is the key!. If instead of using ".fail" you use error: function(xmlHTTP, status, httperror) $.parseJSON(xmlHTTP.responseText);
– Shondeslitch
Jan 10 '17 at 11:42
add a comment |
"$.parseJSON($xhr.responseText)" is the key!. If instead of using ".fail" you use error: function(xmlHTTP, status, httperror) $.parseJSON(xmlHTTP.responseText);
– Shondeslitch
Jan 10 '17 at 11:42
"$.parseJSON($xhr.responseText)" is the key!. If instead of using ".fail" you use error: function(xmlHTTP, status, httperror) $.parseJSON(xmlHTTP.responseText);
– Shondeslitch
Jan 10 '17 at 11:42
"$.parseJSON($xhr.responseText)" is the key!. If instead of using ".fail" you use error: function(xmlHTTP, status, httperror) $.parseJSON(xmlHTTP.responseText);
– Shondeslitch
Jan 10 '17 at 11:42
add a comment |
up vote
25
down vote
directly from the docs
The jQuery XMLHttpRequest (jqXHR) object returned by $.ajax() as of
jQuery 1.5 is a superset of the browser's native XMLHttpRequest
object. For example, it contains responseText and responseXML
properties, as well as a getResponseHeader()
so use the jqXRH argument and get the responseText
property off it.
In the link above, look for the section entitled
The jqXHR Object
1
I read that. the responseText is empty
– Roy Tsabari
Jan 30 '12 at 16:11
@gdoron added the link and section to look for
– hvgotcodes
Jan 30 '12 at 16:13
@tibo, then show us your code. Something is off. Might be best to pause execution in your handler with firebug or webkit and just look at the properties of the arguments. It's gotta be there somewhere...
– hvgotcodes
Jan 30 '12 at 16:14
I just added my code to the question. Can you help?
– Roy Tsabari
Jan 31 '12 at 8:05
without actually digging in, I'm not sure at this point. Are you sure the response body is empty in firebug? Are you sure the url is correct? Are you sure the error handler is being called?
– hvgotcodes
Jan 31 '12 at 12:59
|
show 1 more comment
up vote
25
down vote
directly from the docs
The jQuery XMLHttpRequest (jqXHR) object returned by $.ajax() as of
jQuery 1.5 is a superset of the browser's native XMLHttpRequest
object. For example, it contains responseText and responseXML
properties, as well as a getResponseHeader()
so use the jqXRH argument and get the responseText
property off it.
In the link above, look for the section entitled
The jqXHR Object
1
I read that. the responseText is empty
– Roy Tsabari
Jan 30 '12 at 16:11
@gdoron added the link and section to look for
– hvgotcodes
Jan 30 '12 at 16:13
@tibo, then show us your code. Something is off. Might be best to pause execution in your handler with firebug or webkit and just look at the properties of the arguments. It's gotta be there somewhere...
– hvgotcodes
Jan 30 '12 at 16:14
I just added my code to the question. Can you help?
– Roy Tsabari
Jan 31 '12 at 8:05
without actually digging in, I'm not sure at this point. Are you sure the response body is empty in firebug? Are you sure the url is correct? Are you sure the error handler is being called?
– hvgotcodes
Jan 31 '12 at 12:59
|
show 1 more comment
up vote
25
down vote
up vote
25
down vote
directly from the docs
The jQuery XMLHttpRequest (jqXHR) object returned by $.ajax() as of
jQuery 1.5 is a superset of the browser's native XMLHttpRequest
object. For example, it contains responseText and responseXML
properties, as well as a getResponseHeader()
so use the jqXRH argument and get the responseText
property off it.
In the link above, look for the section entitled
The jqXHR Object
directly from the docs
The jQuery XMLHttpRequest (jqXHR) object returned by $.ajax() as of
jQuery 1.5 is a superset of the browser's native XMLHttpRequest
object. For example, it contains responseText and responseXML
properties, as well as a getResponseHeader()
so use the jqXRH argument and get the responseText
property off it.
In the link above, look for the section entitled
The jqXHR Object
edited Jan 30 '12 at 16:12
answered Jan 30 '12 at 16:05
hvgotcodes
91.4k21166220
91.4k21166220
1
I read that. the responseText is empty
– Roy Tsabari
Jan 30 '12 at 16:11
@gdoron added the link and section to look for
– hvgotcodes
Jan 30 '12 at 16:13
@tibo, then show us your code. Something is off. Might be best to pause execution in your handler with firebug or webkit and just look at the properties of the arguments. It's gotta be there somewhere...
– hvgotcodes
Jan 30 '12 at 16:14
I just added my code to the question. Can you help?
– Roy Tsabari
Jan 31 '12 at 8:05
without actually digging in, I'm not sure at this point. Are you sure the response body is empty in firebug? Are you sure the url is correct? Are you sure the error handler is being called?
– hvgotcodes
Jan 31 '12 at 12:59
|
show 1 more comment
1
I read that. the responseText is empty
– Roy Tsabari
Jan 30 '12 at 16:11
@gdoron added the link and section to look for
– hvgotcodes
Jan 30 '12 at 16:13
@tibo, then show us your code. Something is off. Might be best to pause execution in your handler with firebug or webkit and just look at the properties of the arguments. It's gotta be there somewhere...
– hvgotcodes
Jan 30 '12 at 16:14
I just added my code to the question. Can you help?
– Roy Tsabari
Jan 31 '12 at 8:05
without actually digging in, I'm not sure at this point. Are you sure the response body is empty in firebug? Are you sure the url is correct? Are you sure the error handler is being called?
– hvgotcodes
Jan 31 '12 at 12:59
1
1
I read that. the responseText is empty
– Roy Tsabari
Jan 30 '12 at 16:11
I read that. the responseText is empty
– Roy Tsabari
Jan 30 '12 at 16:11
@gdoron added the link and section to look for
– hvgotcodes
Jan 30 '12 at 16:13
@gdoron added the link and section to look for
– hvgotcodes
Jan 30 '12 at 16:13
@tibo, then show us your code. Something is off. Might be best to pause execution in your handler with firebug or webkit and just look at the properties of the arguments. It's gotta be there somewhere...
– hvgotcodes
Jan 30 '12 at 16:14
@tibo, then show us your code. Something is off. Might be best to pause execution in your handler with firebug or webkit and just look at the properties of the arguments. It's gotta be there somewhere...
– hvgotcodes
Jan 30 '12 at 16:14
I just added my code to the question. Can you help?
– Roy Tsabari
Jan 31 '12 at 8:05
I just added my code to the question. Can you help?
– Roy Tsabari
Jan 31 '12 at 8:05
without actually digging in, I'm not sure at this point. Are you sure the response body is empty in firebug? Are you sure the url is correct? Are you sure the error handler is being called?
– hvgotcodes
Jan 31 '12 at 12:59
without actually digging in, I'm not sure at this point. Are you sure the response body is empty in firebug? Are you sure the url is correct? Are you sure the error handler is being called?
– hvgotcodes
Jan 31 '12 at 12:59
|
show 1 more comment
up vote
1
down vote
I also faced same problem when i was using multipart/form-data. At first I thought multipart/form-data created this mess, but later i found the proper solution.
1) JS code before:
var jersey_url = "http://localhost:8098/final/rest/addItem/upload";
var ans = $.ajax(
type: 'POST',
enctype: 'multipart/form-data',
url: jersey_url,
data: formData,
dataType: "json",
processData: false,
contentType: false
success : funtion(data)
var temp = JSON.parse(data);
console.log("SUCCESS : ", temp.message);
error : funtion($xhr,textStatus,errorThrown)
console.log("ERROR : ", errorThrown);
console.log("ERROR : ", $xhr);
console.log("ERROR : ", textStatus);
);
Here when error occurred, it showed me this in console :-
Error :
Error : abort : f(e), always : f(), .... , responseJSON :""message":"failed""
Error : error
Thus i came to know that we have to use $xhr.responseJSON to get the string message which we sent from rest api.
2) modified/working error funtion:
error : funtion($xhr,textStatus,errorThrown)
var string= $xhr.responseJSON;
var json_object= JSON.parse(string);
console.log("ERROR : ", json_object.message);
Thus will output "Error : failed" on console.
add a comment |
up vote
1
down vote
I also faced same problem when i was using multipart/form-data. At first I thought multipart/form-data created this mess, but later i found the proper solution.
1) JS code before:
var jersey_url = "http://localhost:8098/final/rest/addItem/upload";
var ans = $.ajax(
type: 'POST',
enctype: 'multipart/form-data',
url: jersey_url,
data: formData,
dataType: "json",
processData: false,
contentType: false
success : funtion(data)
var temp = JSON.parse(data);
console.log("SUCCESS : ", temp.message);
error : funtion($xhr,textStatus,errorThrown)
console.log("ERROR : ", errorThrown);
console.log("ERROR : ", $xhr);
console.log("ERROR : ", textStatus);
);
Here when error occurred, it showed me this in console :-
Error :
Error : abort : f(e), always : f(), .... , responseJSON :""message":"failed""
Error : error
Thus i came to know that we have to use $xhr.responseJSON to get the string message which we sent from rest api.
2) modified/working error funtion:
error : funtion($xhr,textStatus,errorThrown)
var string= $xhr.responseJSON;
var json_object= JSON.parse(string);
console.log("ERROR : ", json_object.message);
Thus will output "Error : failed" on console.
add a comment |
up vote
1
down vote
up vote
1
down vote
I also faced same problem when i was using multipart/form-data. At first I thought multipart/form-data created this mess, but later i found the proper solution.
1) JS code before:
var jersey_url = "http://localhost:8098/final/rest/addItem/upload";
var ans = $.ajax(
type: 'POST',
enctype: 'multipart/form-data',
url: jersey_url,
data: formData,
dataType: "json",
processData: false,
contentType: false
success : funtion(data)
var temp = JSON.parse(data);
console.log("SUCCESS : ", temp.message);
error : funtion($xhr,textStatus,errorThrown)
console.log("ERROR : ", errorThrown);
console.log("ERROR : ", $xhr);
console.log("ERROR : ", textStatus);
);
Here when error occurred, it showed me this in console :-
Error :
Error : abort : f(e), always : f(), .... , responseJSON :""message":"failed""
Error : error
Thus i came to know that we have to use $xhr.responseJSON to get the string message which we sent from rest api.
2) modified/working error funtion:
error : funtion($xhr,textStatus,errorThrown)
var string= $xhr.responseJSON;
var json_object= JSON.parse(string);
console.log("ERROR : ", json_object.message);
Thus will output "Error : failed" on console.
I also faced same problem when i was using multipart/form-data. At first I thought multipart/form-data created this mess, but later i found the proper solution.
1) JS code before:
var jersey_url = "http://localhost:8098/final/rest/addItem/upload";
var ans = $.ajax(
type: 'POST',
enctype: 'multipart/form-data',
url: jersey_url,
data: formData,
dataType: "json",
processData: false,
contentType: false
success : funtion(data)
var temp = JSON.parse(data);
console.log("SUCCESS : ", temp.message);
error : funtion($xhr,textStatus,errorThrown)
console.log("ERROR : ", errorThrown);
console.log("ERROR : ", $xhr);
console.log("ERROR : ", textStatus);
);
Here when error occurred, it showed me this in console :-
Error :
Error : abort : f(e), always : f(), .... , responseJSON :""message":"failed""
Error : error
Thus i came to know that we have to use $xhr.responseJSON to get the string message which we sent from rest api.
2) modified/working error funtion:
error : funtion($xhr,textStatus,errorThrown)
var string= $xhr.responseJSON;
var json_object= JSON.parse(string);
console.log("ERROR : ", json_object.message);
Thus will output "Error : failed" on console.
edited Nov 12 at 4:45
answered Nov 10 at 5:24


Archit Sanghvi
113
113
add a comment |
add a comment |
up vote
-3
down vote
accepted
After spending so much time on this problem, I found the problem.
The page is under the URL: www.mydomain.com/register
The REST api is under the URL: server.mydomain.com/rest
Seems like this kind of POST is not so simple.
I'm going to search more information to understand this issue better (if you have more information please share it with me).
When putting the REST API under www.mydomain.com/rest - everything is working fine.
2
You could accept one of the other answers.
– robsch
Apr 5 '17 at 15:06
add a comment |
up vote
-3
down vote
accepted
After spending so much time on this problem, I found the problem.
The page is under the URL: www.mydomain.com/register
The REST api is under the URL: server.mydomain.com/rest
Seems like this kind of POST is not so simple.
I'm going to search more information to understand this issue better (if you have more information please share it with me).
When putting the REST API under www.mydomain.com/rest - everything is working fine.
2
You could accept one of the other answers.
– robsch
Apr 5 '17 at 15:06
add a comment |
up vote
-3
down vote
accepted
up vote
-3
down vote
accepted
After spending so much time on this problem, I found the problem.
The page is under the URL: www.mydomain.com/register
The REST api is under the URL: server.mydomain.com/rest
Seems like this kind of POST is not so simple.
I'm going to search more information to understand this issue better (if you have more information please share it with me).
When putting the REST API under www.mydomain.com/rest - everything is working fine.
After spending so much time on this problem, I found the problem.
The page is under the URL: www.mydomain.com/register
The REST api is under the URL: server.mydomain.com/rest
Seems like this kind of POST is not so simple.
I'm going to search more information to understand this issue better (if you have more information please share it with me).
When putting the REST API under www.mydomain.com/rest - everything is working fine.
edited Feb 1 '12 at 15:02


Daniel Fischer
161k14262393
161k14262393
answered Feb 1 '12 at 14:27
Roy Tsabari
96851539
96851539
2
You could accept one of the other answers.
– robsch
Apr 5 '17 at 15:06
add a comment |
2
You could accept one of the other answers.
– robsch
Apr 5 '17 at 15:06
2
2
You could accept one of the other answers.
– robsch
Apr 5 '17 at 15:06
You could accept one of the other answers.
– robsch
Apr 5 '17 at 15:06
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f9066790%2fjquery-ajax-how-to-get-response-data-in-error%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7 GQJ5mACi,1fE6XO2K
1
In case you don't make it as far down as the accepted answer; there is nothing wrong with this code, the problem lies elsewhere.
– Tony
Jan 16 '13 at 21:22
For other future readers, I had a similar problem as well, and while the cross domain issue was completely irrelevant to my case, it turned out the problem was server side. (It seems the server code was broken in such a way that some combination of HTTP headers like
Accept
made it fail to return the correct response body on a 400 error.)– jpmc26
Sep 5 '15 at 19:31