Dictionary.ForEach method
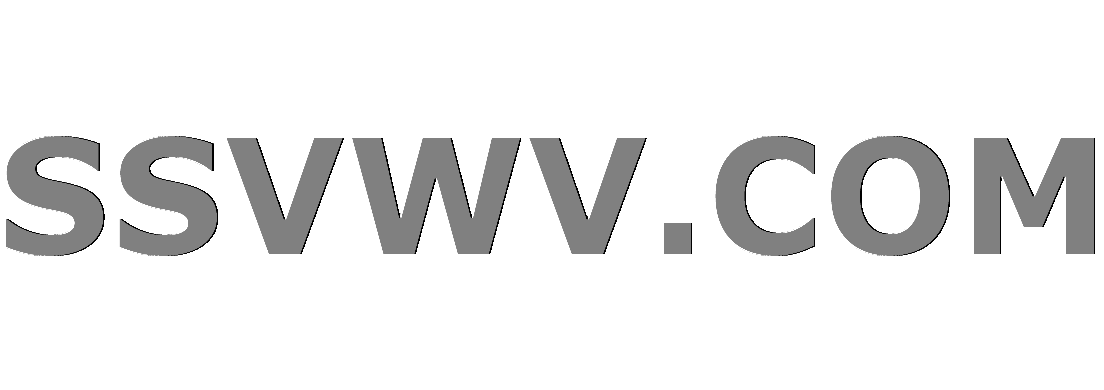
Multi tool use
I wanna declare new extension method which similar to List.ForEach Method.
What I wanna archive:
var dict = new Dictionary<string, string>()
"K1", "V1" ,
"K2", "V2" ,
"K3", "V3" ,
;
dict.ForEach((x, y) =>
Console.WriteLine($"(Key: x, value: y)");
);
How can I do that?
c#
add a comment |
I wanna declare new extension method which similar to List.ForEach Method.
What I wanna archive:
var dict = new Dictionary<string, string>()
"K1", "V1" ,
"K2", "V2" ,
"K3", "V3" ,
;
dict.ForEach((x, y) =>
Console.WriteLine($"(Key: x, value: y)");
);
How can I do that?
c#
add a comment |
I wanna declare new extension method which similar to List.ForEach Method.
What I wanna archive:
var dict = new Dictionary<string, string>()
"K1", "V1" ,
"K2", "V2" ,
"K3", "V3" ,
;
dict.ForEach((x, y) =>
Console.WriteLine($"(Key: x, value: y)");
);
How can I do that?
c#
I wanna declare new extension method which similar to List.ForEach Method.
What I wanna archive:
var dict = new Dictionary<string, string>()
"K1", "V1" ,
"K2", "V2" ,
"K3", "V3" ,
;
dict.ForEach((x, y) =>
Console.WriteLine($"(Key: x, value: y)");
);
How can I do that?
c#
c#
asked Dec 22 '15 at 13:24


FooFoo
1
1
add a comment |
add a comment |
4 Answers
4
active
oldest
votes
You can write an extension method easily:
public static class LinqExtensions
public static void ForEach<TKey, TValue>(this Dictionary<TKey, TValue> dictionary, Action<TKey, TValue> invoke)
foreach(var kvp in dictionary)
invoke(kvp.Key, kvp.Value);
Using like this:
dict.ForEach((x, y) =>
Console.WriteLine($"(Key: x, value: y)");
);
Produces
Key: K1, value: V1
Key: K2, value: V2
Key: K3, value: V3
add a comment |
Try the following:
var dict = new Dictionary<string, string>()
"K1", "V1" ,
"K2", "V2" ,
"K3", "V3" ,
;
foreach(KeyValuePair<string, string> myData in dict )
// Do something with myData.Value or myData.Key
This is the extension method:
public static void ForEach<TKey, TValue>(this Dictionary<TKey, TValue> dictionary, Action<TKey, TValue> invokeMe)
foreach(var keyValue in dictionary)
invokeMe(keyValue.Key, keyValue.Value);
No need to use lambda expression inside the loop?
– Foo
Dec 22 '15 at 13:29
what exactly you want to achieve here? i mean any adddelete things @HappyCoding?
– Neel
Dec 22 '15 at 13:32
Your answer is not matched. I've shown pseudocode above
– Foo
Dec 22 '15 at 13:35
sorry i missed extension method part. updated now @HappyCoding
– Neel
Dec 22 '15 at 13:37
add a comment |
1: Extension methods must be declared in a non-nested, non-generic, static class.
2: The 1st parameter must be annotated with the this
keyword.
public static class DictionaryExtensions
public static void ForEach<TKey, TValue>(
this Dictionary<TKey, TValue> dictionary,
Action<TKey, TValue> action)
foreach (KeyValuePair<TKey, TValue> pair in dictionary)
action(pair.Key, pair.Value);
This method can then be called as if it were a regular instance method:
dict.ForEach((key, value) =>
Console.WriteLine($"(Key: key, Value: value)"));
add a comment |
var dic = new Dictionary<string, string>();
dic.Add("hello", "bob");
dic.Foreach(x =>
Console.WriteLine(x.Key + x.Value);
);
public static void Foreach<T, TY>(this Dictionary<T, TY> collection, Action<T, TY> action)
foreach (var kvp in collection)
action.Invoke(kvp.Key, kvp.Value);
1
action.Invoke(kvp.Key, kvp.Value);
can be shortened toaction(kvp.Key, kvp.Value);
– Zdeslav Vojkovic
Dec 22 '15 at 13:34
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f34416931%2fdictionarytkey-tvalue-foreach-method%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can write an extension method easily:
public static class LinqExtensions
public static void ForEach<TKey, TValue>(this Dictionary<TKey, TValue> dictionary, Action<TKey, TValue> invoke)
foreach(var kvp in dictionary)
invoke(kvp.Key, kvp.Value);
Using like this:
dict.ForEach((x, y) =>
Console.WriteLine($"(Key: x, value: y)");
);
Produces
Key: K1, value: V1
Key: K2, value: V2
Key: K3, value: V3
add a comment |
You can write an extension method easily:
public static class LinqExtensions
public static void ForEach<TKey, TValue>(this Dictionary<TKey, TValue> dictionary, Action<TKey, TValue> invoke)
foreach(var kvp in dictionary)
invoke(kvp.Key, kvp.Value);
Using like this:
dict.ForEach((x, y) =>
Console.WriteLine($"(Key: x, value: y)");
);
Produces
Key: K1, value: V1
Key: K2, value: V2
Key: K3, value: V3
add a comment |
You can write an extension method easily:
public static class LinqExtensions
public static void ForEach<TKey, TValue>(this Dictionary<TKey, TValue> dictionary, Action<TKey, TValue> invoke)
foreach(var kvp in dictionary)
invoke(kvp.Key, kvp.Value);
Using like this:
dict.ForEach((x, y) =>
Console.WriteLine($"(Key: x, value: y)");
);
Produces
Key: K1, value: V1
Key: K2, value: V2
Key: K3, value: V3
You can write an extension method easily:
public static class LinqExtensions
public static void ForEach<TKey, TValue>(this Dictionary<TKey, TValue> dictionary, Action<TKey, TValue> invoke)
foreach(var kvp in dictionary)
invoke(kvp.Key, kvp.Value);
Using like this:
dict.ForEach((x, y) =>
Console.WriteLine($"(Key: x, value: y)");
);
Produces
Key: K1, value: V1
Key: K2, value: V2
Key: K3, value: V3
answered Dec 22 '15 at 13:34
Rob♦Rob
23.8k115575
23.8k115575
add a comment |
add a comment |
Try the following:
var dict = new Dictionary<string, string>()
"K1", "V1" ,
"K2", "V2" ,
"K3", "V3" ,
;
foreach(KeyValuePair<string, string> myData in dict )
// Do something with myData.Value or myData.Key
This is the extension method:
public static void ForEach<TKey, TValue>(this Dictionary<TKey, TValue> dictionary, Action<TKey, TValue> invokeMe)
foreach(var keyValue in dictionary)
invokeMe(keyValue.Key, keyValue.Value);
No need to use lambda expression inside the loop?
– Foo
Dec 22 '15 at 13:29
what exactly you want to achieve here? i mean any adddelete things @HappyCoding?
– Neel
Dec 22 '15 at 13:32
Your answer is not matched. I've shown pseudocode above
– Foo
Dec 22 '15 at 13:35
sorry i missed extension method part. updated now @HappyCoding
– Neel
Dec 22 '15 at 13:37
add a comment |
Try the following:
var dict = new Dictionary<string, string>()
"K1", "V1" ,
"K2", "V2" ,
"K3", "V3" ,
;
foreach(KeyValuePair<string, string> myData in dict )
// Do something with myData.Value or myData.Key
This is the extension method:
public static void ForEach<TKey, TValue>(this Dictionary<TKey, TValue> dictionary, Action<TKey, TValue> invokeMe)
foreach(var keyValue in dictionary)
invokeMe(keyValue.Key, keyValue.Value);
No need to use lambda expression inside the loop?
– Foo
Dec 22 '15 at 13:29
what exactly you want to achieve here? i mean any adddelete things @HappyCoding?
– Neel
Dec 22 '15 at 13:32
Your answer is not matched. I've shown pseudocode above
– Foo
Dec 22 '15 at 13:35
sorry i missed extension method part. updated now @HappyCoding
– Neel
Dec 22 '15 at 13:37
add a comment |
Try the following:
var dict = new Dictionary<string, string>()
"K1", "V1" ,
"K2", "V2" ,
"K3", "V3" ,
;
foreach(KeyValuePair<string, string> myData in dict )
// Do something with myData.Value or myData.Key
This is the extension method:
public static void ForEach<TKey, TValue>(this Dictionary<TKey, TValue> dictionary, Action<TKey, TValue> invokeMe)
foreach(var keyValue in dictionary)
invokeMe(keyValue.Key, keyValue.Value);
Try the following:
var dict = new Dictionary<string, string>()
"K1", "V1" ,
"K2", "V2" ,
"K3", "V3" ,
;
foreach(KeyValuePair<string, string> myData in dict )
// Do something with myData.Value or myData.Key
This is the extension method:
public static void ForEach<TKey, TValue>(this Dictionary<TKey, TValue> dictionary, Action<TKey, TValue> invokeMe)
foreach(var keyValue in dictionary)
invokeMe(keyValue.Key, keyValue.Value);
edited Nov 13 '18 at 0:35
Lauren Van Sloun
94911018
94911018
answered Dec 22 '15 at 13:26


NeelNeel
9,93512949
9,93512949
No need to use lambda expression inside the loop?
– Foo
Dec 22 '15 at 13:29
what exactly you want to achieve here? i mean any adddelete things @HappyCoding?
– Neel
Dec 22 '15 at 13:32
Your answer is not matched. I've shown pseudocode above
– Foo
Dec 22 '15 at 13:35
sorry i missed extension method part. updated now @HappyCoding
– Neel
Dec 22 '15 at 13:37
add a comment |
No need to use lambda expression inside the loop?
– Foo
Dec 22 '15 at 13:29
what exactly you want to achieve here? i mean any adddelete things @HappyCoding?
– Neel
Dec 22 '15 at 13:32
Your answer is not matched. I've shown pseudocode above
– Foo
Dec 22 '15 at 13:35
sorry i missed extension method part. updated now @HappyCoding
– Neel
Dec 22 '15 at 13:37
No need to use lambda expression inside the loop?
– Foo
Dec 22 '15 at 13:29
No need to use lambda expression inside the loop?
– Foo
Dec 22 '15 at 13:29
what exactly you want to achieve here? i mean any adddelete things @HappyCoding?
– Neel
Dec 22 '15 at 13:32
what exactly you want to achieve here? i mean any adddelete things @HappyCoding?
– Neel
Dec 22 '15 at 13:32
Your answer is not matched. I've shown pseudocode above
– Foo
Dec 22 '15 at 13:35
Your answer is not matched. I've shown pseudocode above
– Foo
Dec 22 '15 at 13:35
sorry i missed extension method part. updated now @HappyCoding
– Neel
Dec 22 '15 at 13:37
sorry i missed extension method part. updated now @HappyCoding
– Neel
Dec 22 '15 at 13:37
add a comment |
1: Extension methods must be declared in a non-nested, non-generic, static class.
2: The 1st parameter must be annotated with the this
keyword.
public static class DictionaryExtensions
public static void ForEach<TKey, TValue>(
this Dictionary<TKey, TValue> dictionary,
Action<TKey, TValue> action)
foreach (KeyValuePair<TKey, TValue> pair in dictionary)
action(pair.Key, pair.Value);
This method can then be called as if it were a regular instance method:
dict.ForEach((key, value) =>
Console.WriteLine($"(Key: key, Value: value)"));
add a comment |
1: Extension methods must be declared in a non-nested, non-generic, static class.
2: The 1st parameter must be annotated with the this
keyword.
public static class DictionaryExtensions
public static void ForEach<TKey, TValue>(
this Dictionary<TKey, TValue> dictionary,
Action<TKey, TValue> action)
foreach (KeyValuePair<TKey, TValue> pair in dictionary)
action(pair.Key, pair.Value);
This method can then be called as if it were a regular instance method:
dict.ForEach((key, value) =>
Console.WriteLine($"(Key: key, Value: value)"));
add a comment |
1: Extension methods must be declared in a non-nested, non-generic, static class.
2: The 1st parameter must be annotated with the this
keyword.
public static class DictionaryExtensions
public static void ForEach<TKey, TValue>(
this Dictionary<TKey, TValue> dictionary,
Action<TKey, TValue> action)
foreach (KeyValuePair<TKey, TValue> pair in dictionary)
action(pair.Key, pair.Value);
This method can then be called as if it were a regular instance method:
dict.ForEach((key, value) =>
Console.WriteLine($"(Key: key, Value: value)"));
1: Extension methods must be declared in a non-nested, non-generic, static class.
2: The 1st parameter must be annotated with the this
keyword.
public static class DictionaryExtensions
public static void ForEach<TKey, TValue>(
this Dictionary<TKey, TValue> dictionary,
Action<TKey, TValue> action)
foreach (KeyValuePair<TKey, TValue> pair in dictionary)
action(pair.Key, pair.Value);
This method can then be called as if it were a regular instance method:
dict.ForEach((key, value) =>
Console.WriteLine($"(Key: key, Value: value)"));
answered Dec 22 '15 at 13:35
Dennis_EDennis_E
7,4581421
7,4581421
add a comment |
add a comment |
var dic = new Dictionary<string, string>();
dic.Add("hello", "bob");
dic.Foreach(x =>
Console.WriteLine(x.Key + x.Value);
);
public static void Foreach<T, TY>(this Dictionary<T, TY> collection, Action<T, TY> action)
foreach (var kvp in collection)
action.Invoke(kvp.Key, kvp.Value);
1
action.Invoke(kvp.Key, kvp.Value);
can be shortened toaction(kvp.Key, kvp.Value);
– Zdeslav Vojkovic
Dec 22 '15 at 13:34
add a comment |
var dic = new Dictionary<string, string>();
dic.Add("hello", "bob");
dic.Foreach(x =>
Console.WriteLine(x.Key + x.Value);
);
public static void Foreach<T, TY>(this Dictionary<T, TY> collection, Action<T, TY> action)
foreach (var kvp in collection)
action.Invoke(kvp.Key, kvp.Value);
1
action.Invoke(kvp.Key, kvp.Value);
can be shortened toaction(kvp.Key, kvp.Value);
– Zdeslav Vojkovic
Dec 22 '15 at 13:34
add a comment |
var dic = new Dictionary<string, string>();
dic.Add("hello", "bob");
dic.Foreach(x =>
Console.WriteLine(x.Key + x.Value);
);
public static void Foreach<T, TY>(this Dictionary<T, TY> collection, Action<T, TY> action)
foreach (var kvp in collection)
action.Invoke(kvp.Key, kvp.Value);
var dic = new Dictionary<string, string>();
dic.Add("hello", "bob");
dic.Foreach(x =>
Console.WriteLine(x.Key + x.Value);
);
public static void Foreach<T, TY>(this Dictionary<T, TY> collection, Action<T, TY> action)
foreach (var kvp in collection)
action.Invoke(kvp.Key, kvp.Value);
edited Nov 13 '18 at 0:41
Lauren Van Sloun
94911018
94911018
answered Dec 22 '15 at 13:31
MacMac
184
184
1
action.Invoke(kvp.Key, kvp.Value);
can be shortened toaction(kvp.Key, kvp.Value);
– Zdeslav Vojkovic
Dec 22 '15 at 13:34
add a comment |
1
action.Invoke(kvp.Key, kvp.Value);
can be shortened toaction(kvp.Key, kvp.Value);
– Zdeslav Vojkovic
Dec 22 '15 at 13:34
1
1
action.Invoke(kvp.Key, kvp.Value);
can be shortened to action(kvp.Key, kvp.Value);
– Zdeslav Vojkovic
Dec 22 '15 at 13:34
action.Invoke(kvp.Key, kvp.Value);
can be shortened to action(kvp.Key, kvp.Value);
– Zdeslav Vojkovic
Dec 22 '15 at 13:34
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f34416931%2fdictionarytkey-tvalue-foreach-method%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ljKhWPl9kG7yQ4UWGVRUqljJUYjd,iOKvPaLI5Bf,Dbk,L,g z 5smhPn Mz,lUqlTkml8pGrr9 VZJH