How can I fix “ValueError: ”“ needs to have a value for field ”klant“ before this many-to-many relationship can be used.”
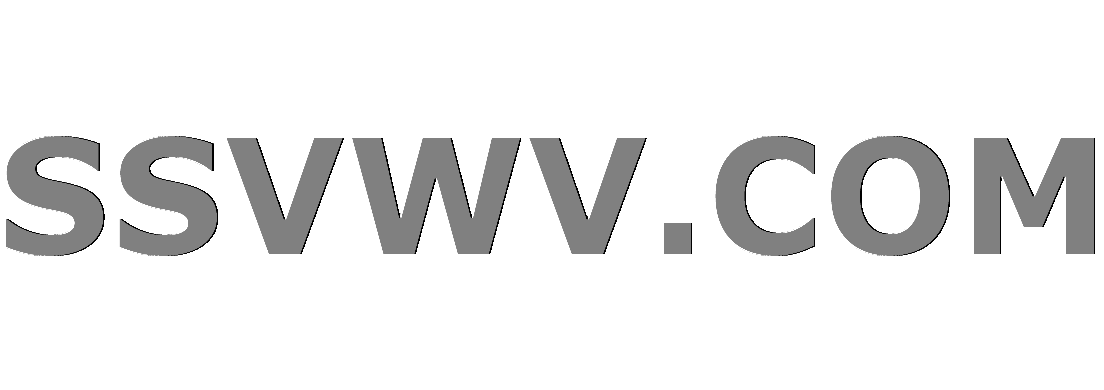
Multi tool use
Here are the imports:
from django.db import models
from datetime import datetime, timedelta
from django.contrib.auth.models import User
This is the first class I defined. It is the status of the action (Actie) and it has a status-id and a status-name with a max_length attribute of 5 (todo, doing, done)
class Status(models.Model):
id = models.IntegerField(primary_key=True)
status_naam = models.CharField(max_length=5, default='todo')
def __str__(self):
return str(self.id) + " - " + self.status_naam
This is the second class Klant wich means customer. It has an id, a customer-name and users from the customer wich is a ManyToManyField referring to users from the the User-table django gives me.
class Klant(models.Model):
id = models.IntegerField(primary_key=True)
klant_naam = models.CharField(max_length=100, default='-')
klant_gebruiker = models.ManyToManyField(User)
def __str__(self):
return str(self.id) + " - " + self.klant_naam
This is the class Actie (Action or the action the user determines) wich has an id, an action-name, a action-status wich refers to the table Status here above, an action-publish-date, an ending-date (the deadline) and a customer-id wich refers to Klant.
class Actie(models.Model):
id = models.IntegerField(primary_key=True)
actie_naam = models.CharField(max_length=150, default='-')
actie_status = models.ForeignKey(Status, default=1)
actie_aanmaakdatum = models.DateTimeField(default=datetime.now())
actie_einddatum = models.DateTimeField(default=datetime.now() + timedelta(days=1))
actie_klant = models.ForeignKey(Klant, default=1)
def __str__(self):
return str(self.id) + " - " + self.actie_naam
This is what I'm doing in the shell:
(InteractiveConsole)
>>> from MyApp.models import User, Klant
>>> klant1 = Klant.objects.create(klant_naam='aCustomer')
>>> user1 = User.objects.get(username='peterdevries')
>>> klant1.klant_gebruiker.add(user1)
Traceback (most recent call last):
File "C:shell.py", line 69, in handle
self.run_shell(shell=options['interface'])
File "C:shell.py", line 61, in run_shell
raise ImportError
ImportError
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "<console>", line 1, in <module>
File "C:related_descriptors.py", line 468, in __get__
return self.related_manager_cls(instance)
File "C:related_descriptors.py", line 751, in __init__
(instance, self.source_field_name))
ValueError: "<Klant: None - aCustomer>" needs to have a value for field "klant" before this many-to-many relationship can be used.
>>>
So my question now is what do I have to do to fix this ValueError?
python django shell django-models revitpythonshell
add a comment |
Here are the imports:
from django.db import models
from datetime import datetime, timedelta
from django.contrib.auth.models import User
This is the first class I defined. It is the status of the action (Actie) and it has a status-id and a status-name with a max_length attribute of 5 (todo, doing, done)
class Status(models.Model):
id = models.IntegerField(primary_key=True)
status_naam = models.CharField(max_length=5, default='todo')
def __str__(self):
return str(self.id) + " - " + self.status_naam
This is the second class Klant wich means customer. It has an id, a customer-name and users from the customer wich is a ManyToManyField referring to users from the the User-table django gives me.
class Klant(models.Model):
id = models.IntegerField(primary_key=True)
klant_naam = models.CharField(max_length=100, default='-')
klant_gebruiker = models.ManyToManyField(User)
def __str__(self):
return str(self.id) + " - " + self.klant_naam
This is the class Actie (Action or the action the user determines) wich has an id, an action-name, a action-status wich refers to the table Status here above, an action-publish-date, an ending-date (the deadline) and a customer-id wich refers to Klant.
class Actie(models.Model):
id = models.IntegerField(primary_key=True)
actie_naam = models.CharField(max_length=150, default='-')
actie_status = models.ForeignKey(Status, default=1)
actie_aanmaakdatum = models.DateTimeField(default=datetime.now())
actie_einddatum = models.DateTimeField(default=datetime.now() + timedelta(days=1))
actie_klant = models.ForeignKey(Klant, default=1)
def __str__(self):
return str(self.id) + " - " + self.actie_naam
This is what I'm doing in the shell:
(InteractiveConsole)
>>> from MyApp.models import User, Klant
>>> klant1 = Klant.objects.create(klant_naam='aCustomer')
>>> user1 = User.objects.get(username='peterdevries')
>>> klant1.klant_gebruiker.add(user1)
Traceback (most recent call last):
File "C:shell.py", line 69, in handle
self.run_shell(shell=options['interface'])
File "C:shell.py", line 61, in run_shell
raise ImportError
ImportError
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "<console>", line 1, in <module>
File "C:related_descriptors.py", line 468, in __get__
return self.related_manager_cls(instance)
File "C:related_descriptors.py", line 751, in __init__
(instance, self.source_field_name))
ValueError: "<Klant: None - aCustomer>" needs to have a value for field "klant" before this many-to-many relationship can be used.
>>>
So my question now is what do I have to do to fix this ValueError?
python django shell django-models revitpythonshell
add a comment |
Here are the imports:
from django.db import models
from datetime import datetime, timedelta
from django.contrib.auth.models import User
This is the first class I defined. It is the status of the action (Actie) and it has a status-id and a status-name with a max_length attribute of 5 (todo, doing, done)
class Status(models.Model):
id = models.IntegerField(primary_key=True)
status_naam = models.CharField(max_length=5, default='todo')
def __str__(self):
return str(self.id) + " - " + self.status_naam
This is the second class Klant wich means customer. It has an id, a customer-name and users from the customer wich is a ManyToManyField referring to users from the the User-table django gives me.
class Klant(models.Model):
id = models.IntegerField(primary_key=True)
klant_naam = models.CharField(max_length=100, default='-')
klant_gebruiker = models.ManyToManyField(User)
def __str__(self):
return str(self.id) + " - " + self.klant_naam
This is the class Actie (Action or the action the user determines) wich has an id, an action-name, a action-status wich refers to the table Status here above, an action-publish-date, an ending-date (the deadline) and a customer-id wich refers to Klant.
class Actie(models.Model):
id = models.IntegerField(primary_key=True)
actie_naam = models.CharField(max_length=150, default='-')
actie_status = models.ForeignKey(Status, default=1)
actie_aanmaakdatum = models.DateTimeField(default=datetime.now())
actie_einddatum = models.DateTimeField(default=datetime.now() + timedelta(days=1))
actie_klant = models.ForeignKey(Klant, default=1)
def __str__(self):
return str(self.id) + " - " + self.actie_naam
This is what I'm doing in the shell:
(InteractiveConsole)
>>> from MyApp.models import User, Klant
>>> klant1 = Klant.objects.create(klant_naam='aCustomer')
>>> user1 = User.objects.get(username='peterdevries')
>>> klant1.klant_gebruiker.add(user1)
Traceback (most recent call last):
File "C:shell.py", line 69, in handle
self.run_shell(shell=options['interface'])
File "C:shell.py", line 61, in run_shell
raise ImportError
ImportError
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "<console>", line 1, in <module>
File "C:related_descriptors.py", line 468, in __get__
return self.related_manager_cls(instance)
File "C:related_descriptors.py", line 751, in __init__
(instance, self.source_field_name))
ValueError: "<Klant: None - aCustomer>" needs to have a value for field "klant" before this many-to-many relationship can be used.
>>>
So my question now is what do I have to do to fix this ValueError?
python django shell django-models revitpythonshell
Here are the imports:
from django.db import models
from datetime import datetime, timedelta
from django.contrib.auth.models import User
This is the first class I defined. It is the status of the action (Actie) and it has a status-id and a status-name with a max_length attribute of 5 (todo, doing, done)
class Status(models.Model):
id = models.IntegerField(primary_key=True)
status_naam = models.CharField(max_length=5, default='todo')
def __str__(self):
return str(self.id) + " - " + self.status_naam
This is the second class Klant wich means customer. It has an id, a customer-name and users from the customer wich is a ManyToManyField referring to users from the the User-table django gives me.
class Klant(models.Model):
id = models.IntegerField(primary_key=True)
klant_naam = models.CharField(max_length=100, default='-')
klant_gebruiker = models.ManyToManyField(User)
def __str__(self):
return str(self.id) + " - " + self.klant_naam
This is the class Actie (Action or the action the user determines) wich has an id, an action-name, a action-status wich refers to the table Status here above, an action-publish-date, an ending-date (the deadline) and a customer-id wich refers to Klant.
class Actie(models.Model):
id = models.IntegerField(primary_key=True)
actie_naam = models.CharField(max_length=150, default='-')
actie_status = models.ForeignKey(Status, default=1)
actie_aanmaakdatum = models.DateTimeField(default=datetime.now())
actie_einddatum = models.DateTimeField(default=datetime.now() + timedelta(days=1))
actie_klant = models.ForeignKey(Klant, default=1)
def __str__(self):
return str(self.id) + " - " + self.actie_naam
This is what I'm doing in the shell:
(InteractiveConsole)
>>> from MyApp.models import User, Klant
>>> klant1 = Klant.objects.create(klant_naam='aCustomer')
>>> user1 = User.objects.get(username='peterdevries')
>>> klant1.klant_gebruiker.add(user1)
Traceback (most recent call last):
File "C:shell.py", line 69, in handle
self.run_shell(shell=options['interface'])
File "C:shell.py", line 61, in run_shell
raise ImportError
ImportError
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "<console>", line 1, in <module>
File "C:related_descriptors.py", line 468, in __get__
return self.related_manager_cls(instance)
File "C:related_descriptors.py", line 751, in __init__
(instance, self.source_field_name))
ValueError: "<Klant: None - aCustomer>" needs to have a value for field "klant" before this many-to-many relationship can be used.
>>>
So my question now is what do I have to do to fix this ValueError?
python django shell django-models revitpythonshell
python django shell django-models revitpythonshell
asked Nov 14 '18 at 11:47


E. ArslanE. Arslan
245
245
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Your models look like they were autogenerated via inspectdb
. The problem is that all your primary keys are IntegerFields. This means that Django does not know that the database will give them a value automatically, so does not update the field on creation with that value - and therefore can't create the many-to-many relation, which requires inserting pks from both sides into the linking table.
You could change the fields to AutoField, but the simplest solution is just to delete those definitions altogether - Django will automatically set the primary key to an AutoField named id
if another pk is not defined.
Thank you, it worked. I changed all the IntegerFields to AutoField with 'primary_key=True' as argument.
– E. Arslan
Nov 14 '18 at 15:24
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53299529%2fhow-can-i-fix-valueerror-klant-none-acustomer-needs-to-have-a-value-for%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your models look like they were autogenerated via inspectdb
. The problem is that all your primary keys are IntegerFields. This means that Django does not know that the database will give them a value automatically, so does not update the field on creation with that value - and therefore can't create the many-to-many relation, which requires inserting pks from both sides into the linking table.
You could change the fields to AutoField, but the simplest solution is just to delete those definitions altogether - Django will automatically set the primary key to an AutoField named id
if another pk is not defined.
Thank you, it worked. I changed all the IntegerFields to AutoField with 'primary_key=True' as argument.
– E. Arslan
Nov 14 '18 at 15:24
add a comment |
Your models look like they were autogenerated via inspectdb
. The problem is that all your primary keys are IntegerFields. This means that Django does not know that the database will give them a value automatically, so does not update the field on creation with that value - and therefore can't create the many-to-many relation, which requires inserting pks from both sides into the linking table.
You could change the fields to AutoField, but the simplest solution is just to delete those definitions altogether - Django will automatically set the primary key to an AutoField named id
if another pk is not defined.
Thank you, it worked. I changed all the IntegerFields to AutoField with 'primary_key=True' as argument.
– E. Arslan
Nov 14 '18 at 15:24
add a comment |
Your models look like they were autogenerated via inspectdb
. The problem is that all your primary keys are IntegerFields. This means that Django does not know that the database will give them a value automatically, so does not update the field on creation with that value - and therefore can't create the many-to-many relation, which requires inserting pks from both sides into the linking table.
You could change the fields to AutoField, but the simplest solution is just to delete those definitions altogether - Django will automatically set the primary key to an AutoField named id
if another pk is not defined.
Your models look like they were autogenerated via inspectdb
. The problem is that all your primary keys are IntegerFields. This means that Django does not know that the database will give them a value automatically, so does not update the field on creation with that value - and therefore can't create the many-to-many relation, which requires inserting pks from both sides into the linking table.
You could change the fields to AutoField, but the simplest solution is just to delete those definitions altogether - Django will automatically set the primary key to an AutoField named id
if another pk is not defined.
answered Nov 14 '18 at 11:52
Daniel RosemanDaniel Roseman
454k41588645
454k41588645
Thank you, it worked. I changed all the IntegerFields to AutoField with 'primary_key=True' as argument.
– E. Arslan
Nov 14 '18 at 15:24
add a comment |
Thank you, it worked. I changed all the IntegerFields to AutoField with 'primary_key=True' as argument.
– E. Arslan
Nov 14 '18 at 15:24
Thank you, it worked. I changed all the IntegerFields to AutoField with 'primary_key=True' as argument.
– E. Arslan
Nov 14 '18 at 15:24
Thank you, it worked. I changed all the IntegerFields to AutoField with 'primary_key=True' as argument.
– E. Arslan
Nov 14 '18 at 15:24
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53299529%2fhow-can-i-fix-valueerror-klant-none-acustomer-needs-to-have-a-value-for%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hZwfogu36YgjUY SVNOsE0GRNLcouBQxb TrKVbRm4 zdo8XqMrpZsCWa98O,GK8tMm3i MZYP4MIW7gv39qXjX87Ny3BddakHV 3