Keras model predicting experience from hours
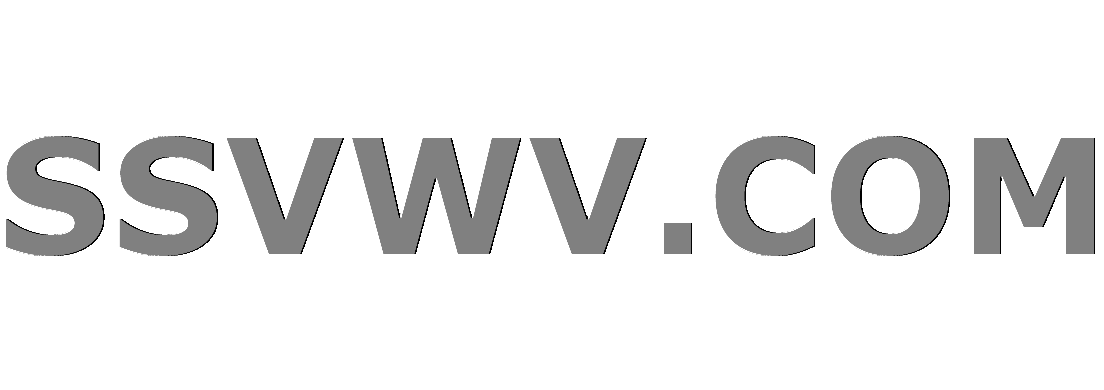
Multi tool use
I am very new to Keras, neural networks and machine learning having just started to learn yesterday. I decided to try predicting the experience over an hour (0 to 23) (for a game and my own generated data-set) that a user would earn. Currently running what I have the predictions seem to be very low and very poor. I have tried a relu activation, which produced predictions all to be zero and from a bit of research, LeakyReLU.
This is the code I have for the prediction model so far:
from keras.models import Sequential
from keras.layers import Dense
from keras.layers import LeakyReLU
import numpy
numpy.random.seed(7)
dataset = numpy.loadtxt("experience.csv", delimiter=",")
X = dataset[: ,0]
Y = dataset[: ,1]
model = Sequential()
model.add(Dense(12, input_dim = 1, activation=LeakyReLU(0.3)))
model.add(Dense(8, activation=LeakyReLU(0.3)))
model.add(Dense(1, activation=LeakyReLU(0.3)))
model.compile(loss = 'mean_absolute_error', optimizer='adam', metrics = ['accuracy'])
model.fit(X, Y, epochs=120, batch_size=10, verbose = 0)
predictions = model.predict(X)
rounded = [round(x[0]) for x in predictions]
print(rounded)
I have also tried playing around with the hidden levels of the network, but honestly have no idea how many there should be or a good way to justify an amount.
If it helps here is the data-set I have been using:
https://raw.githubusercontent.com/NightShadeII/xpPredictor/master/experience.csv
Thankyou for any help
python tensorflow keras
add a comment |
I am very new to Keras, neural networks and machine learning having just started to learn yesterday. I decided to try predicting the experience over an hour (0 to 23) (for a game and my own generated data-set) that a user would earn. Currently running what I have the predictions seem to be very low and very poor. I have tried a relu activation, which produced predictions all to be zero and from a bit of research, LeakyReLU.
This is the code I have for the prediction model so far:
from keras.models import Sequential
from keras.layers import Dense
from keras.layers import LeakyReLU
import numpy
numpy.random.seed(7)
dataset = numpy.loadtxt("experience.csv", delimiter=",")
X = dataset[: ,0]
Y = dataset[: ,1]
model = Sequential()
model.add(Dense(12, input_dim = 1, activation=LeakyReLU(0.3)))
model.add(Dense(8, activation=LeakyReLU(0.3)))
model.add(Dense(1, activation=LeakyReLU(0.3)))
model.compile(loss = 'mean_absolute_error', optimizer='adam', metrics = ['accuracy'])
model.fit(X, Y, epochs=120, batch_size=10, verbose = 0)
predictions = model.predict(X)
rounded = [round(x[0]) for x in predictions]
print(rounded)
I have also tried playing around with the hidden levels of the network, but honestly have no idea how many there should be or a good way to justify an amount.
If it helps here is the data-set I have been using:
https://raw.githubusercontent.com/NightShadeII/xpPredictor/master/experience.csv
Thankyou for any help
python tensorflow keras
add a comment |
I am very new to Keras, neural networks and machine learning having just started to learn yesterday. I decided to try predicting the experience over an hour (0 to 23) (for a game and my own generated data-set) that a user would earn. Currently running what I have the predictions seem to be very low and very poor. I have tried a relu activation, which produced predictions all to be zero and from a bit of research, LeakyReLU.
This is the code I have for the prediction model so far:
from keras.models import Sequential
from keras.layers import Dense
from keras.layers import LeakyReLU
import numpy
numpy.random.seed(7)
dataset = numpy.loadtxt("experience.csv", delimiter=",")
X = dataset[: ,0]
Y = dataset[: ,1]
model = Sequential()
model.add(Dense(12, input_dim = 1, activation=LeakyReLU(0.3)))
model.add(Dense(8, activation=LeakyReLU(0.3)))
model.add(Dense(1, activation=LeakyReLU(0.3)))
model.compile(loss = 'mean_absolute_error', optimizer='adam', metrics = ['accuracy'])
model.fit(X, Y, epochs=120, batch_size=10, verbose = 0)
predictions = model.predict(X)
rounded = [round(x[0]) for x in predictions]
print(rounded)
I have also tried playing around with the hidden levels of the network, but honestly have no idea how many there should be or a good way to justify an amount.
If it helps here is the data-set I have been using:
https://raw.githubusercontent.com/NightShadeII/xpPredictor/master/experience.csv
Thankyou for any help
python tensorflow keras
I am very new to Keras, neural networks and machine learning having just started to learn yesterday. I decided to try predicting the experience over an hour (0 to 23) (for a game and my own generated data-set) that a user would earn. Currently running what I have the predictions seem to be very low and very poor. I have tried a relu activation, which produced predictions all to be zero and from a bit of research, LeakyReLU.
This is the code I have for the prediction model so far:
from keras.models import Sequential
from keras.layers import Dense
from keras.layers import LeakyReLU
import numpy
numpy.random.seed(7)
dataset = numpy.loadtxt("experience.csv", delimiter=",")
X = dataset[: ,0]
Y = dataset[: ,1]
model = Sequential()
model.add(Dense(12, input_dim = 1, activation=LeakyReLU(0.3)))
model.add(Dense(8, activation=LeakyReLU(0.3)))
model.add(Dense(1, activation=LeakyReLU(0.3)))
model.compile(loss = 'mean_absolute_error', optimizer='adam', metrics = ['accuracy'])
model.fit(X, Y, epochs=120, batch_size=10, verbose = 0)
predictions = model.predict(X)
rounded = [round(x[0]) for x in predictions]
print(rounded)
I have also tried playing around with the hidden levels of the network, but honestly have no idea how many there should be or a good way to justify an amount.
If it helps here is the data-set I have been using:
https://raw.githubusercontent.com/NightShadeII/xpPredictor/master/experience.csv
Thankyou for any help
python tensorflow keras
python tensorflow keras
asked Nov 15 '18 at 6:35


NightShadeNightShade
11410
11410
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Looking at your data it does not seem like a classification problem.
You have two options:
-> Look at the second column and bucket them depending on the ranges and make classes that can be predicted, for instance: 0, 1, 2 etc. Now it tries to train but does not have enough examples for millions of classes that it thinks you are trying to predict.
-> If you want real valued output and not classes, try using linear regression.
I tried separating them into classes of [0 - 100,000), [100,000- 200,000)... etc. and changed the outputs to [1, 0, 0...0], [0, 1, 0, ... 0], etc. however the model does not really seem to improve and gets stuck at an accuracy of 85% from the start and a loss of 0.55. Do you think you could elaborate on why this does not work I am a bit confused. Really this was just an exercise and something that does seem more suited for linear regression
– NightShade
Nov 18 '18 at 11:01
@NightShade I am a bit confused what are you asking here. Did you try linear regression, does that work better and why? or are you asking how to improve the NN, in that case you could you update the code that you are using, I will have a look at it
– power.puffed
Nov 19 '18 at 10:39
Sorry If I was unclear, essentially, I want to try predicting the data as an exercise using a NN rather than linear regression although it is not of the most importance. What I am saying is I tried bucketing the second column into ranges and tried it as a classification problem however that does not seem to help much, and the model does not seem to improve. I will share what I have for now: github.com/NightShadeII/xpPredictor
– NightShade
Nov 19 '18 at 23:28
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53313712%2fkeras-model-predicting-experience-from-hours%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Looking at your data it does not seem like a classification problem.
You have two options:
-> Look at the second column and bucket them depending on the ranges and make classes that can be predicted, for instance: 0, 1, 2 etc. Now it tries to train but does not have enough examples for millions of classes that it thinks you are trying to predict.
-> If you want real valued output and not classes, try using linear regression.
I tried separating them into classes of [0 - 100,000), [100,000- 200,000)... etc. and changed the outputs to [1, 0, 0...0], [0, 1, 0, ... 0], etc. however the model does not really seem to improve and gets stuck at an accuracy of 85% from the start and a loss of 0.55. Do you think you could elaborate on why this does not work I am a bit confused. Really this was just an exercise and something that does seem more suited for linear regression
– NightShade
Nov 18 '18 at 11:01
@NightShade I am a bit confused what are you asking here. Did you try linear regression, does that work better and why? or are you asking how to improve the NN, in that case you could you update the code that you are using, I will have a look at it
– power.puffed
Nov 19 '18 at 10:39
Sorry If I was unclear, essentially, I want to try predicting the data as an exercise using a NN rather than linear regression although it is not of the most importance. What I am saying is I tried bucketing the second column into ranges and tried it as a classification problem however that does not seem to help much, and the model does not seem to improve. I will share what I have for now: github.com/NightShadeII/xpPredictor
– NightShade
Nov 19 '18 at 23:28
add a comment |
Looking at your data it does not seem like a classification problem.
You have two options:
-> Look at the second column and bucket them depending on the ranges and make classes that can be predicted, for instance: 0, 1, 2 etc. Now it tries to train but does not have enough examples for millions of classes that it thinks you are trying to predict.
-> If you want real valued output and not classes, try using linear regression.
I tried separating them into classes of [0 - 100,000), [100,000- 200,000)... etc. and changed the outputs to [1, 0, 0...0], [0, 1, 0, ... 0], etc. however the model does not really seem to improve and gets stuck at an accuracy of 85% from the start and a loss of 0.55. Do you think you could elaborate on why this does not work I am a bit confused. Really this was just an exercise and something that does seem more suited for linear regression
– NightShade
Nov 18 '18 at 11:01
@NightShade I am a bit confused what are you asking here. Did you try linear regression, does that work better and why? or are you asking how to improve the NN, in that case you could you update the code that you are using, I will have a look at it
– power.puffed
Nov 19 '18 at 10:39
Sorry If I was unclear, essentially, I want to try predicting the data as an exercise using a NN rather than linear regression although it is not of the most importance. What I am saying is I tried bucketing the second column into ranges and tried it as a classification problem however that does not seem to help much, and the model does not seem to improve. I will share what I have for now: github.com/NightShadeII/xpPredictor
– NightShade
Nov 19 '18 at 23:28
add a comment |
Looking at your data it does not seem like a classification problem.
You have two options:
-> Look at the second column and bucket them depending on the ranges and make classes that can be predicted, for instance: 0, 1, 2 etc. Now it tries to train but does not have enough examples for millions of classes that it thinks you are trying to predict.
-> If you want real valued output and not classes, try using linear regression.
Looking at your data it does not seem like a classification problem.
You have two options:
-> Look at the second column and bucket them depending on the ranges and make classes that can be predicted, for instance: 0, 1, 2 etc. Now it tries to train but does not have enough examples for millions of classes that it thinks you are trying to predict.
-> If you want real valued output and not classes, try using linear regression.
edited Nov 15 '18 at 14:43
answered Nov 15 '18 at 14:35


power.puffedpower.puffed
119
119
I tried separating them into classes of [0 - 100,000), [100,000- 200,000)... etc. and changed the outputs to [1, 0, 0...0], [0, 1, 0, ... 0], etc. however the model does not really seem to improve and gets stuck at an accuracy of 85% from the start and a loss of 0.55. Do you think you could elaborate on why this does not work I am a bit confused. Really this was just an exercise and something that does seem more suited for linear regression
– NightShade
Nov 18 '18 at 11:01
@NightShade I am a bit confused what are you asking here. Did you try linear regression, does that work better and why? or are you asking how to improve the NN, in that case you could you update the code that you are using, I will have a look at it
– power.puffed
Nov 19 '18 at 10:39
Sorry If I was unclear, essentially, I want to try predicting the data as an exercise using a NN rather than linear regression although it is not of the most importance. What I am saying is I tried bucketing the second column into ranges and tried it as a classification problem however that does not seem to help much, and the model does not seem to improve. I will share what I have for now: github.com/NightShadeII/xpPredictor
– NightShade
Nov 19 '18 at 23:28
add a comment |
I tried separating them into classes of [0 - 100,000), [100,000- 200,000)... etc. and changed the outputs to [1, 0, 0...0], [0, 1, 0, ... 0], etc. however the model does not really seem to improve and gets stuck at an accuracy of 85% from the start and a loss of 0.55. Do you think you could elaborate on why this does not work I am a bit confused. Really this was just an exercise and something that does seem more suited for linear regression
– NightShade
Nov 18 '18 at 11:01
@NightShade I am a bit confused what are you asking here. Did you try linear regression, does that work better and why? or are you asking how to improve the NN, in that case you could you update the code that you are using, I will have a look at it
– power.puffed
Nov 19 '18 at 10:39
Sorry If I was unclear, essentially, I want to try predicting the data as an exercise using a NN rather than linear regression although it is not of the most importance. What I am saying is I tried bucketing the second column into ranges and tried it as a classification problem however that does not seem to help much, and the model does not seem to improve. I will share what I have for now: github.com/NightShadeII/xpPredictor
– NightShade
Nov 19 '18 at 23:28
I tried separating them into classes of [0 - 100,000), [100,000- 200,000)... etc. and changed the outputs to [1, 0, 0...0], [0, 1, 0, ... 0], etc. however the model does not really seem to improve and gets stuck at an accuracy of 85% from the start and a loss of 0.55. Do you think you could elaborate on why this does not work I am a bit confused. Really this was just an exercise and something that does seem more suited for linear regression
– NightShade
Nov 18 '18 at 11:01
I tried separating them into classes of [0 - 100,000), [100,000- 200,000)... etc. and changed the outputs to [1, 0, 0...0], [0, 1, 0, ... 0], etc. however the model does not really seem to improve and gets stuck at an accuracy of 85% from the start and a loss of 0.55. Do you think you could elaborate on why this does not work I am a bit confused. Really this was just an exercise and something that does seem more suited for linear regression
– NightShade
Nov 18 '18 at 11:01
@NightShade I am a bit confused what are you asking here. Did you try linear regression, does that work better and why? or are you asking how to improve the NN, in that case you could you update the code that you are using, I will have a look at it
– power.puffed
Nov 19 '18 at 10:39
@NightShade I am a bit confused what are you asking here. Did you try linear regression, does that work better and why? or are you asking how to improve the NN, in that case you could you update the code that you are using, I will have a look at it
– power.puffed
Nov 19 '18 at 10:39
Sorry If I was unclear, essentially, I want to try predicting the data as an exercise using a NN rather than linear regression although it is not of the most importance. What I am saying is I tried bucketing the second column into ranges and tried it as a classification problem however that does not seem to help much, and the model does not seem to improve. I will share what I have for now: github.com/NightShadeII/xpPredictor
– NightShade
Nov 19 '18 at 23:28
Sorry If I was unclear, essentially, I want to try predicting the data as an exercise using a NN rather than linear regression although it is not of the most importance. What I am saying is I tried bucketing the second column into ranges and tried it as a classification problem however that does not seem to help much, and the model does not seem to improve. I will share what I have for now: github.com/NightShadeII/xpPredictor
– NightShade
Nov 19 '18 at 23:28
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53313712%2fkeras-model-predicting-experience-from-hours%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
2cYUfB5,g6m51UgM3LPfX0YXIfBN9DU xc0QjO