Dependency Injection issue : Unable to resolve service
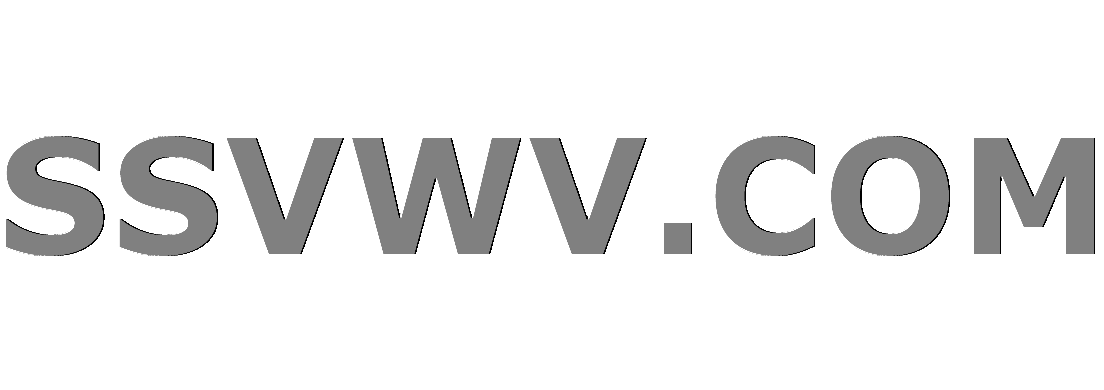
Multi tool use
up vote
-1
down vote
favorite
I'm trying to implement 3-tier concept in my ASP.NET Core MVC application and I'm quite struggling with DI.
Here's a quick representation of solution structure :
- Business
- Common
- User
- Data
- AppDbContext
- Web
- Controllers
- Views
Business, Common, Data and Web are 4 different projects referencing each other when necessary.
Problem is, when I try to access the AppDbContext
inside controllers, I get this error :
InvalidOperationException: Unable to resolve service for type 'com.jds.chat.Data.AppDbContext' while attempting to activate 'com.jds.chat..Web.Controllers.CustomCriMessengerClientBotController'
The AppDbContext.cs
in the Data
project:
public class AppDbContext : DbContext
static string DbContextName = "AppDbConnectionString";
public DbSet<User> Users get; set;
public AppDbContext(DbContextOptions<AppDbContext> options) : base(options)
public AppDbContext(): base()
protected override void OnModelCreating(ModelBuilder modelBuilder)
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
if (!optionsBuilder.IsConfigured)
IConfigurationRoot configuration = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json")
.Build();
var connectionString = configuration
.GetConnectionString(DbContextName);
optionsBuilder.UseSqlServer(connectionString,
x => x.MigrationsAssembly("com.jds.chat..Data"));
public virtual void Commit()
base.SaveChanges();
The Container builder in the Data
project:
public class AutoFacDataModule : Autofac.Module
protected override void Load(ContainerBuilder builder)
builder.RegisterType<AppDbContext>().InstancePerLifetimeScope();
The controller in Web project :
private readonly AppDbContext _dbContext;
public ChatController(AppDbContext dbContext)
_dbContext = dbContext;
public IActionResult Index()
var users = _dbContext.Users.Where(u => u.IsInChat).ToList();
return View();
The startup.cs in web project :
public class Startup
public Startup(IConfiguration configuration)
Configuration = configuration;
public IConfiguration Configuration get;
// This method gets called by the runtime.
// Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
services.Configure<CookiePolicyOptions>(options =>
// This lambda determines whether user consent
// for non-essential cookies is needed for a given request.
options.CheckConsentNeeded = context => true;
options.MinimumSameSitePolicy = SameSiteMode.None;
);
var builder = new ContainerBuilder();
builder.RegisterModule(new AutofacBusinessModule());
builder.RegisterModule(new Cri.Module.Chatbot.
Business.AutofacBusinessModule());
services.AddMvc()
.SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
// This method gets called by the runtime.
// Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
if (env.IsDevelopment())
app.UseDeveloperExceptionPage();
//app.UseDatabaseErrorPage();
else
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseCookiePolicy();
app.UseMvc(routes =>
routes.MapRoute(
name: "default",
template: "controller=Home/action=Index/id?");
);
I'm missing something, but yet I don't see what...
asp.net-mvc asp.net-core dependency-injection
add a comment |
up vote
-1
down vote
favorite
I'm trying to implement 3-tier concept in my ASP.NET Core MVC application and I'm quite struggling with DI.
Here's a quick representation of solution structure :
- Business
- Common
- User
- Data
- AppDbContext
- Web
- Controllers
- Views
Business, Common, Data and Web are 4 different projects referencing each other when necessary.
Problem is, when I try to access the AppDbContext
inside controllers, I get this error :
InvalidOperationException: Unable to resolve service for type 'com.jds.chat.Data.AppDbContext' while attempting to activate 'com.jds.chat..Web.Controllers.CustomCriMessengerClientBotController'
The AppDbContext.cs
in the Data
project:
public class AppDbContext : DbContext
static string DbContextName = "AppDbConnectionString";
public DbSet<User> Users get; set;
public AppDbContext(DbContextOptions<AppDbContext> options) : base(options)
public AppDbContext(): base()
protected override void OnModelCreating(ModelBuilder modelBuilder)
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
if (!optionsBuilder.IsConfigured)
IConfigurationRoot configuration = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json")
.Build();
var connectionString = configuration
.GetConnectionString(DbContextName);
optionsBuilder.UseSqlServer(connectionString,
x => x.MigrationsAssembly("com.jds.chat..Data"));
public virtual void Commit()
base.SaveChanges();
The Container builder in the Data
project:
public class AutoFacDataModule : Autofac.Module
protected override void Load(ContainerBuilder builder)
builder.RegisterType<AppDbContext>().InstancePerLifetimeScope();
The controller in Web project :
private readonly AppDbContext _dbContext;
public ChatController(AppDbContext dbContext)
_dbContext = dbContext;
public IActionResult Index()
var users = _dbContext.Users.Where(u => u.IsInChat).ToList();
return View();
The startup.cs in web project :
public class Startup
public Startup(IConfiguration configuration)
Configuration = configuration;
public IConfiguration Configuration get;
// This method gets called by the runtime.
// Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
services.Configure<CookiePolicyOptions>(options =>
// This lambda determines whether user consent
// for non-essential cookies is needed for a given request.
options.CheckConsentNeeded = context => true;
options.MinimumSameSitePolicy = SameSiteMode.None;
);
var builder = new ContainerBuilder();
builder.RegisterModule(new AutofacBusinessModule());
builder.RegisterModule(new Cri.Module.Chatbot.
Business.AutofacBusinessModule());
services.AddMvc()
.SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
// This method gets called by the runtime.
// Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
if (env.IsDevelopment())
app.UseDeveloperExceptionPage();
//app.UseDatabaseErrorPage();
else
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseCookiePolicy();
app.UseMvc(routes =>
routes.MapRoute(
name: "default",
template: "controller=Home/action=Index/id?");
);
I'm missing something, but yet I don't see what...
asp.net-mvc asp.net-core dependency-injection
Have you registered your DbContext with your DI container?
– Jasen
Nov 9 at 19:21
@Jasen Sorry, forgot inormation in original post. I edited to add the conatiner
– j0w
Nov 9 at 19:32
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
I'm trying to implement 3-tier concept in my ASP.NET Core MVC application and I'm quite struggling with DI.
Here's a quick representation of solution structure :
- Business
- Common
- User
- Data
- AppDbContext
- Web
- Controllers
- Views
Business, Common, Data and Web are 4 different projects referencing each other when necessary.
Problem is, when I try to access the AppDbContext
inside controllers, I get this error :
InvalidOperationException: Unable to resolve service for type 'com.jds.chat.Data.AppDbContext' while attempting to activate 'com.jds.chat..Web.Controllers.CustomCriMessengerClientBotController'
The AppDbContext.cs
in the Data
project:
public class AppDbContext : DbContext
static string DbContextName = "AppDbConnectionString";
public DbSet<User> Users get; set;
public AppDbContext(DbContextOptions<AppDbContext> options) : base(options)
public AppDbContext(): base()
protected override void OnModelCreating(ModelBuilder modelBuilder)
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
if (!optionsBuilder.IsConfigured)
IConfigurationRoot configuration = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json")
.Build();
var connectionString = configuration
.GetConnectionString(DbContextName);
optionsBuilder.UseSqlServer(connectionString,
x => x.MigrationsAssembly("com.jds.chat..Data"));
public virtual void Commit()
base.SaveChanges();
The Container builder in the Data
project:
public class AutoFacDataModule : Autofac.Module
protected override void Load(ContainerBuilder builder)
builder.RegisterType<AppDbContext>().InstancePerLifetimeScope();
The controller in Web project :
private readonly AppDbContext _dbContext;
public ChatController(AppDbContext dbContext)
_dbContext = dbContext;
public IActionResult Index()
var users = _dbContext.Users.Where(u => u.IsInChat).ToList();
return View();
The startup.cs in web project :
public class Startup
public Startup(IConfiguration configuration)
Configuration = configuration;
public IConfiguration Configuration get;
// This method gets called by the runtime.
// Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
services.Configure<CookiePolicyOptions>(options =>
// This lambda determines whether user consent
// for non-essential cookies is needed for a given request.
options.CheckConsentNeeded = context => true;
options.MinimumSameSitePolicy = SameSiteMode.None;
);
var builder = new ContainerBuilder();
builder.RegisterModule(new AutofacBusinessModule());
builder.RegisterModule(new Cri.Module.Chatbot.
Business.AutofacBusinessModule());
services.AddMvc()
.SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
// This method gets called by the runtime.
// Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
if (env.IsDevelopment())
app.UseDeveloperExceptionPage();
//app.UseDatabaseErrorPage();
else
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseCookiePolicy();
app.UseMvc(routes =>
routes.MapRoute(
name: "default",
template: "controller=Home/action=Index/id?");
);
I'm missing something, but yet I don't see what...
asp.net-mvc asp.net-core dependency-injection
I'm trying to implement 3-tier concept in my ASP.NET Core MVC application and I'm quite struggling with DI.
Here's a quick representation of solution structure :
- Business
- Common
- User
- Data
- AppDbContext
- Web
- Controllers
- Views
Business, Common, Data and Web are 4 different projects referencing each other when necessary.
Problem is, when I try to access the AppDbContext
inside controllers, I get this error :
InvalidOperationException: Unable to resolve service for type 'com.jds.chat.Data.AppDbContext' while attempting to activate 'com.jds.chat..Web.Controllers.CustomCriMessengerClientBotController'
The AppDbContext.cs
in the Data
project:
public class AppDbContext : DbContext
static string DbContextName = "AppDbConnectionString";
public DbSet<User> Users get; set;
public AppDbContext(DbContextOptions<AppDbContext> options) : base(options)
public AppDbContext(): base()
protected override void OnModelCreating(ModelBuilder modelBuilder)
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
if (!optionsBuilder.IsConfigured)
IConfigurationRoot configuration = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json")
.Build();
var connectionString = configuration
.GetConnectionString(DbContextName);
optionsBuilder.UseSqlServer(connectionString,
x => x.MigrationsAssembly("com.jds.chat..Data"));
public virtual void Commit()
base.SaveChanges();
The Container builder in the Data
project:
public class AutoFacDataModule : Autofac.Module
protected override void Load(ContainerBuilder builder)
builder.RegisterType<AppDbContext>().InstancePerLifetimeScope();
The controller in Web project :
private readonly AppDbContext _dbContext;
public ChatController(AppDbContext dbContext)
_dbContext = dbContext;
public IActionResult Index()
var users = _dbContext.Users.Where(u => u.IsInChat).ToList();
return View();
The startup.cs in web project :
public class Startup
public Startup(IConfiguration configuration)
Configuration = configuration;
public IConfiguration Configuration get;
// This method gets called by the runtime.
// Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
services.Configure<CookiePolicyOptions>(options =>
// This lambda determines whether user consent
// for non-essential cookies is needed for a given request.
options.CheckConsentNeeded = context => true;
options.MinimumSameSitePolicy = SameSiteMode.None;
);
var builder = new ContainerBuilder();
builder.RegisterModule(new AutofacBusinessModule());
builder.RegisterModule(new Cri.Module.Chatbot.
Business.AutofacBusinessModule());
services.AddMvc()
.SetCompatibilityVersion(CompatibilityVersion.Version_2_1);
// This method gets called by the runtime.
// Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
if (env.IsDevelopment())
app.UseDeveloperExceptionPage();
//app.UseDatabaseErrorPage();
else
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseCookiePolicy();
app.UseMvc(routes =>
routes.MapRoute(
name: "default",
template: "controller=Home/action=Index/id?");
);
I'm missing something, but yet I don't see what...
asp.net-mvc asp.net-core dependency-injection
asp.net-mvc asp.net-core dependency-injection
edited Nov 9 at 21:01
asked Nov 9 at 19:08
j0w
648
648
Have you registered your DbContext with your DI container?
– Jasen
Nov 9 at 19:21
@Jasen Sorry, forgot inormation in original post. I edited to add the conatiner
– j0w
Nov 9 at 19:32
add a comment |
Have you registered your DbContext with your DI container?
– Jasen
Nov 9 at 19:21
@Jasen Sorry, forgot inormation in original post. I edited to add the conatiner
– j0w
Nov 9 at 19:32
Have you registered your DbContext with your DI container?
– Jasen
Nov 9 at 19:21
Have you registered your DbContext with your DI container?
– Jasen
Nov 9 at 19:21
@Jasen Sorry, forgot inormation in original post. I edited to add the conatiner
– j0w
Nov 9 at 19:32
@Jasen Sorry, forgot inormation in original post. I edited to add the conatiner
– j0w
Nov 9 at 19:32
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
You're registering the AppDbContext in the AutoFacDataModule, here:
public class AutoFacDataModule : Autofac.Module
protected override void Load(ContainerBuilder builder)
builder.RegisterType<AppDbContext>().InstancePerLifetimeScope();
But you're not registering that module with Autofac with anywhere in ConfigureServices
. You're registering two other modules:
builder.RegisterModule(new AutofacBusinessModule());
builder.RegisterModule(new Cri.Module.Chatbot.Business.AutofacBusinessModule());
But not the AutodacDataModule
. You need to add something like:
builder.RegisterModule(new AutofacDataModule());
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
You're registering the AppDbContext in the AutoFacDataModule, here:
public class AutoFacDataModule : Autofac.Module
protected override void Load(ContainerBuilder builder)
builder.RegisterType<AppDbContext>().InstancePerLifetimeScope();
But you're not registering that module with Autofac with anywhere in ConfigureServices
. You're registering two other modules:
builder.RegisterModule(new AutofacBusinessModule());
builder.RegisterModule(new Cri.Module.Chatbot.Business.AutofacBusinessModule());
But not the AutodacDataModule
. You need to add something like:
builder.RegisterModule(new AutofacDataModule());
add a comment |
up vote
0
down vote
You're registering the AppDbContext in the AutoFacDataModule, here:
public class AutoFacDataModule : Autofac.Module
protected override void Load(ContainerBuilder builder)
builder.RegisterType<AppDbContext>().InstancePerLifetimeScope();
But you're not registering that module with Autofac with anywhere in ConfigureServices
. You're registering two other modules:
builder.RegisterModule(new AutofacBusinessModule());
builder.RegisterModule(new Cri.Module.Chatbot.Business.AutofacBusinessModule());
But not the AutodacDataModule
. You need to add something like:
builder.RegisterModule(new AutofacDataModule());
add a comment |
up vote
0
down vote
up vote
0
down vote
You're registering the AppDbContext in the AutoFacDataModule, here:
public class AutoFacDataModule : Autofac.Module
protected override void Load(ContainerBuilder builder)
builder.RegisterType<AppDbContext>().InstancePerLifetimeScope();
But you're not registering that module with Autofac with anywhere in ConfigureServices
. You're registering two other modules:
builder.RegisterModule(new AutofacBusinessModule());
builder.RegisterModule(new Cri.Module.Chatbot.Business.AutofacBusinessModule());
But not the AutodacDataModule
. You need to add something like:
builder.RegisterModule(new AutofacDataModule());
You're registering the AppDbContext in the AutoFacDataModule, here:
public class AutoFacDataModule : Autofac.Module
protected override void Load(ContainerBuilder builder)
builder.RegisterType<AppDbContext>().InstancePerLifetimeScope();
But you're not registering that module with Autofac with anywhere in ConfigureServices
. You're registering two other modules:
builder.RegisterModule(new AutofacBusinessModule());
builder.RegisterModule(new Cri.Module.Chatbot.Business.AutofacBusinessModule());
But not the AutodacDataModule
. You need to add something like:
builder.RegisterModule(new AutofacDataModule());
edited Nov 9 at 21:28
answered Nov 9 at 21:23
jmoerdyk
4,49362838
4,49362838
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53231908%2fdependency-injection-issue-unable-to-resolve-service%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
hV,fU0BMbjgtgAOtBf1ki7xXlXAQ
Have you registered your DbContext with your DI container?
– Jasen
Nov 9 at 19:21
@Jasen Sorry, forgot inormation in original post. I edited to add the conatiner
– j0w
Nov 9 at 19:32