FileNotFound Exception When Trying To Read File Using getServletContext().getRealPath()
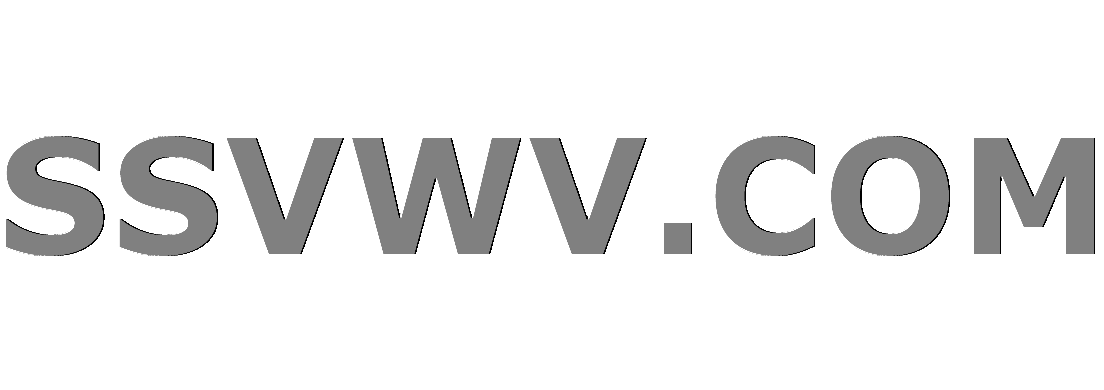
Multi tool use
up vote
0
down vote
favorite
My program is able to create and write to a file using the path-file:
private static final String PUBLIC_KEY_FILE = "WebContent/Config/MyPublic.key";
private static final String PRIVATE_KEY_FILE = "WebContent/Config/MyPrivate.key";
And the correct files are generated within the folders according to the path declared above.
But then a FileNotFoundException error occurs when I try to read from those files using the path files:
private String REAL_PUBLIC_PATH = getServletContext().getRealPath("/WebContent/Config/MyPublic.key");
private String REAL_PRIVATE_PATH = getServletContext().getRealPath("/WebContent/Config/MyPrivate.key");
This is the lines of code that is causing the error:
PublicKey pubKey = readPublicKeyFromFile(this.REAL_PUBLIC_PATH);
PrivateKey privKey = readPrivateKeyFromFile(this.REAL_PRIVATE_PATH);
Which is tied to the variables REAL_PUBLIC_PATH
and REAL_PUBLIC_PATH
. Meaning there's something wrong with how the path file is being read.
My folder structure goes:
> MyProject
>Web-Content
>Config
>MyPublic.key
>MyPrivate.key
>WEB-INF
>META-INF
My full class code is below for context:
public class RSAEncryptionHelper extends HttpServlet
private static final String PUBLIC_KEY_FILE = "WebContent/Config/MyPublic.key";
private static final String PRIVATE_KEY_FILE = "WebContent/Config/MyPrivate.key";
private String REAL_PUBLIC_PATH = getServletContext().getRealPath("/WebContent/Config/MyPublic.key");
private String REAL_PRIVATE_PATH = getServletContext().getRealPath("/WebContent/Config/MyPrivate.key");
public static void main(String args) throws IOException
try
System.out.println("--GENERATE PUBLIC and PRIVATE KEY --");
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
keyPairGenerator.initialize(2048); //1024 for normal securities
KeyPair keyPair = keyPairGenerator.generateKeyPair();
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
System.out.println("n--PULLING OUT PARAMETERS WHICH MAKES KEYPAIR--n");
KeyFactory keyFactory = KeyFactory.getInstance("RSA");
RSAPublicKeySpec rsaPubKeySpec = keyFactory.getKeySpec(publicKey, RSAPublicKeySpec.class);
RSAPrivateKeySpec rsaPrivKeySpec = keyFactory.getKeySpec(privateKey, RSAPrivateKeySpec.class);
System.out.println("n--SAVING PUBLIC KEY AND PRIVATE KEY TO FILES--n");
RSAEncryptionHelper rsaObj = new RSAEncryptionHelper();
rsaObj.saveKeys(PUBLIC_KEY_FILE, rsaPubKeySpec.getModulus(), rsaPubKeySpec.getPublicExponent());
rsaObj.saveKeys(PRIVATE_KEY_FILE, rsaPrivKeySpec.getModulus(), rsaPrivKeySpec.getPrivateExponent());
catch (NoSuchAlgorithmException
private void saveKeys(String fileName, BigInteger mod, BigInteger exp) throws IOException
FileOutputStream fos = null;
ObjectOutputStream oos = null;
try
System.out.println("Generating: " + fileName + "...");
fos = new FileOutputStream(fileName);
oos = new ObjectOutputStream(new BufferedOutputStream(fos));
oos.writeObject(mod);
oos.writeObject(exp);
System.out.println(fileName + "generated successfully");;
catch (Exception e)
e.printStackTrace();
finally
if (oos!=null)
oos.close();
if (fos!= null)
fos.close();
public PublicKey readPublicKeyFromFile(String fileName) throws IOException
FileInputStream fis = null;
ObjectInputStream ois = null;
PublicKey publicKey = null;
try
fis = new FileInputStream(new File(fileName));
ois = new ObjectInputStream(fis);
BigInteger modulus = (BigInteger) ois.readObject();
BigInteger exponent = (BigInteger) ois.readObject();
//Get Public Key
RSAPublicKeySpec rsaPublicKeySpec = new RSAPublicKeySpec(modulus, exponent);
KeyFactory fact = KeyFactory.getInstance("RSA");
publicKey = fact.generatePublic(rsaPublicKeySpec);
return publicKey;
catch (IOException e)
e.printStackTrace();
catch (ClassNotFoundException e)
e.printStackTrace();
catch (NoSuchAlgorithmException e)
e.printStackTrace();
catch (InvalidKeySpecException e)
e.printStackTrace();
finally
if (ois != null)
ois.close();
if (fis != null)
fis.close();
return publicKey;
public PrivateKey readPrivateKeyFromFile(String fileName) throws IOException
FileInputStream fis = null;
ObjectInputStream ois = null;
PrivateKey privateKey = null;
try
fis = new FileInputStream(new File(fileName));
ois = new ObjectInputStream(fis);
BigInteger modulus = (BigInteger) ois.readObject();
BigInteger exponent = (BigInteger) ois.readObject();
//Get Public Key
RSAPrivateKeySpec rsaPrivateKeySpec = new RSAPrivateKeySpec(modulus, exponent);
KeyFactory fact = KeyFactory.getInstance("RSA");
privateKey = fact.generatePrivate(rsaPrivateKeySpec);
return privateKey;
catch (IOException e)
e.printStackTrace();
catch (ClassNotFoundException e)
e.printStackTrace();
catch (NoSuchAlgorithmException e)
e.printStackTrace();
catch (InvalidKeySpecException e)
e.printStackTrace();
finally
if (ois != null)
ois.close();
if (fis != null)
fis.close();
return privateKey;
public byte encryptData(String data) throws IOException
System.out.println("n--ENCRYPTION STARTED--");
System.out.println("Data Before Encryption: " + data);
byte dataToEncrypt = data.getBytes();
byte encryptedData = null;
try
PublicKey pubKey = readPublicKeyFromFile(this.REAL_PUBLIC_PATH);
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, pubKey);
encryptedData = cipher.doFinal(dataToEncrypt);
System.out.println("Encrypted Data: " + encryptedData);
catch (IOException e)
e.printStackTrace();
catch (NoSuchAlgorithmException e)
e.printStackTrace();
catch (InvalidKeyException e)
e.printStackTrace();
catch (IllegalBlockSizeException e)
e.printStackTrace();
catch (BadPaddingException e)
e.printStackTrace();
catch (NoSuchPaddingException e)
e.printStackTrace();
System.out.println("--ENCRYPTION COMPLETED--");
return encryptedData;
public String decryptData(byte data) throws IOException
System.out.println("n--DECRYPTION STARTED--");
byte decryptedData = null;
String decData = "";
try
PrivateKey privateKey = readPrivateKeyFromFile(this.REAL_PRIVATE_PATH);
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.DECRYPT_MODE, privateKey);
decryptedData = cipher.doFinal(data);
decData = new String(decryptedData);
System.out.println("Decrypted Data: " + decData);
return decData;
catch (IOException e)
e.printStackTrace();
catch (NoSuchAlgorithmException e)
e.printStackTrace();
catch (NoSuchPaddingException e)
e.printStackTrace();
catch (InvalidKeyException e)
e.printStackTrace();
catch ( IllegalBlockSizeException e)
e.printStackTrace();
catch (BadPaddingException e)
e.printStackTrace();
System.out.println("--DECRYPTION COMPLETED--");
return decData;
java jsp servlets filepath filenotfoundexception
add a comment |
up vote
0
down vote
favorite
My program is able to create and write to a file using the path-file:
private static final String PUBLIC_KEY_FILE = "WebContent/Config/MyPublic.key";
private static final String PRIVATE_KEY_FILE = "WebContent/Config/MyPrivate.key";
And the correct files are generated within the folders according to the path declared above.
But then a FileNotFoundException error occurs when I try to read from those files using the path files:
private String REAL_PUBLIC_PATH = getServletContext().getRealPath("/WebContent/Config/MyPublic.key");
private String REAL_PRIVATE_PATH = getServletContext().getRealPath("/WebContent/Config/MyPrivate.key");
This is the lines of code that is causing the error:
PublicKey pubKey = readPublicKeyFromFile(this.REAL_PUBLIC_PATH);
PrivateKey privKey = readPrivateKeyFromFile(this.REAL_PRIVATE_PATH);
Which is tied to the variables REAL_PUBLIC_PATH
and REAL_PUBLIC_PATH
. Meaning there's something wrong with how the path file is being read.
My folder structure goes:
> MyProject
>Web-Content
>Config
>MyPublic.key
>MyPrivate.key
>WEB-INF
>META-INF
My full class code is below for context:
public class RSAEncryptionHelper extends HttpServlet
private static final String PUBLIC_KEY_FILE = "WebContent/Config/MyPublic.key";
private static final String PRIVATE_KEY_FILE = "WebContent/Config/MyPrivate.key";
private String REAL_PUBLIC_PATH = getServletContext().getRealPath("/WebContent/Config/MyPublic.key");
private String REAL_PRIVATE_PATH = getServletContext().getRealPath("/WebContent/Config/MyPrivate.key");
public static void main(String args) throws IOException
try
System.out.println("--GENERATE PUBLIC and PRIVATE KEY --");
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
keyPairGenerator.initialize(2048); //1024 for normal securities
KeyPair keyPair = keyPairGenerator.generateKeyPair();
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
System.out.println("n--PULLING OUT PARAMETERS WHICH MAKES KEYPAIR--n");
KeyFactory keyFactory = KeyFactory.getInstance("RSA");
RSAPublicKeySpec rsaPubKeySpec = keyFactory.getKeySpec(publicKey, RSAPublicKeySpec.class);
RSAPrivateKeySpec rsaPrivKeySpec = keyFactory.getKeySpec(privateKey, RSAPrivateKeySpec.class);
System.out.println("n--SAVING PUBLIC KEY AND PRIVATE KEY TO FILES--n");
RSAEncryptionHelper rsaObj = new RSAEncryptionHelper();
rsaObj.saveKeys(PUBLIC_KEY_FILE, rsaPubKeySpec.getModulus(), rsaPubKeySpec.getPublicExponent());
rsaObj.saveKeys(PRIVATE_KEY_FILE, rsaPrivKeySpec.getModulus(), rsaPrivKeySpec.getPrivateExponent());
catch (NoSuchAlgorithmException
private void saveKeys(String fileName, BigInteger mod, BigInteger exp) throws IOException
FileOutputStream fos = null;
ObjectOutputStream oos = null;
try
System.out.println("Generating: " + fileName + "...");
fos = new FileOutputStream(fileName);
oos = new ObjectOutputStream(new BufferedOutputStream(fos));
oos.writeObject(mod);
oos.writeObject(exp);
System.out.println(fileName + "generated successfully");;
catch (Exception e)
e.printStackTrace();
finally
if (oos!=null)
oos.close();
if (fos!= null)
fos.close();
public PublicKey readPublicKeyFromFile(String fileName) throws IOException
FileInputStream fis = null;
ObjectInputStream ois = null;
PublicKey publicKey = null;
try
fis = new FileInputStream(new File(fileName));
ois = new ObjectInputStream(fis);
BigInteger modulus = (BigInteger) ois.readObject();
BigInteger exponent = (BigInteger) ois.readObject();
//Get Public Key
RSAPublicKeySpec rsaPublicKeySpec = new RSAPublicKeySpec(modulus, exponent);
KeyFactory fact = KeyFactory.getInstance("RSA");
publicKey = fact.generatePublic(rsaPublicKeySpec);
return publicKey;
catch (IOException e)
e.printStackTrace();
catch (ClassNotFoundException e)
e.printStackTrace();
catch (NoSuchAlgorithmException e)
e.printStackTrace();
catch (InvalidKeySpecException e)
e.printStackTrace();
finally
if (ois != null)
ois.close();
if (fis != null)
fis.close();
return publicKey;
public PrivateKey readPrivateKeyFromFile(String fileName) throws IOException
FileInputStream fis = null;
ObjectInputStream ois = null;
PrivateKey privateKey = null;
try
fis = new FileInputStream(new File(fileName));
ois = new ObjectInputStream(fis);
BigInteger modulus = (BigInteger) ois.readObject();
BigInteger exponent = (BigInteger) ois.readObject();
//Get Public Key
RSAPrivateKeySpec rsaPrivateKeySpec = new RSAPrivateKeySpec(modulus, exponent);
KeyFactory fact = KeyFactory.getInstance("RSA");
privateKey = fact.generatePrivate(rsaPrivateKeySpec);
return privateKey;
catch (IOException e)
e.printStackTrace();
catch (ClassNotFoundException e)
e.printStackTrace();
catch (NoSuchAlgorithmException e)
e.printStackTrace();
catch (InvalidKeySpecException e)
e.printStackTrace();
finally
if (ois != null)
ois.close();
if (fis != null)
fis.close();
return privateKey;
public byte encryptData(String data) throws IOException
System.out.println("n--ENCRYPTION STARTED--");
System.out.println("Data Before Encryption: " + data);
byte dataToEncrypt = data.getBytes();
byte encryptedData = null;
try
PublicKey pubKey = readPublicKeyFromFile(this.REAL_PUBLIC_PATH);
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, pubKey);
encryptedData = cipher.doFinal(dataToEncrypt);
System.out.println("Encrypted Data: " + encryptedData);
catch (IOException e)
e.printStackTrace();
catch (NoSuchAlgorithmException e)
e.printStackTrace();
catch (InvalidKeyException e)
e.printStackTrace();
catch (IllegalBlockSizeException e)
e.printStackTrace();
catch (BadPaddingException e)
e.printStackTrace();
catch (NoSuchPaddingException e)
e.printStackTrace();
System.out.println("--ENCRYPTION COMPLETED--");
return encryptedData;
public String decryptData(byte data) throws IOException
System.out.println("n--DECRYPTION STARTED--");
byte decryptedData = null;
String decData = "";
try
PrivateKey privateKey = readPrivateKeyFromFile(this.REAL_PRIVATE_PATH);
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.DECRYPT_MODE, privateKey);
decryptedData = cipher.doFinal(data);
decData = new String(decryptedData);
System.out.println("Decrypted Data: " + decData);
return decData;
catch (IOException e)
e.printStackTrace();
catch (NoSuchAlgorithmException e)
e.printStackTrace();
catch (NoSuchPaddingException e)
e.printStackTrace();
catch (InvalidKeyException e)
e.printStackTrace();
catch ( IllegalBlockSizeException e)
e.printStackTrace();
catch (BadPaddingException e)
e.printStackTrace();
System.out.println("--DECRYPTION COMPLETED--");
return decData;
java jsp servlets filepath filenotfoundexception
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
My program is able to create and write to a file using the path-file:
private static final String PUBLIC_KEY_FILE = "WebContent/Config/MyPublic.key";
private static final String PRIVATE_KEY_FILE = "WebContent/Config/MyPrivate.key";
And the correct files are generated within the folders according to the path declared above.
But then a FileNotFoundException error occurs when I try to read from those files using the path files:
private String REAL_PUBLIC_PATH = getServletContext().getRealPath("/WebContent/Config/MyPublic.key");
private String REAL_PRIVATE_PATH = getServletContext().getRealPath("/WebContent/Config/MyPrivate.key");
This is the lines of code that is causing the error:
PublicKey pubKey = readPublicKeyFromFile(this.REAL_PUBLIC_PATH);
PrivateKey privKey = readPrivateKeyFromFile(this.REAL_PRIVATE_PATH);
Which is tied to the variables REAL_PUBLIC_PATH
and REAL_PUBLIC_PATH
. Meaning there's something wrong with how the path file is being read.
My folder structure goes:
> MyProject
>Web-Content
>Config
>MyPublic.key
>MyPrivate.key
>WEB-INF
>META-INF
My full class code is below for context:
public class RSAEncryptionHelper extends HttpServlet
private static final String PUBLIC_KEY_FILE = "WebContent/Config/MyPublic.key";
private static final String PRIVATE_KEY_FILE = "WebContent/Config/MyPrivate.key";
private String REAL_PUBLIC_PATH = getServletContext().getRealPath("/WebContent/Config/MyPublic.key");
private String REAL_PRIVATE_PATH = getServletContext().getRealPath("/WebContent/Config/MyPrivate.key");
public static void main(String args) throws IOException
try
System.out.println("--GENERATE PUBLIC and PRIVATE KEY --");
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
keyPairGenerator.initialize(2048); //1024 for normal securities
KeyPair keyPair = keyPairGenerator.generateKeyPair();
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
System.out.println("n--PULLING OUT PARAMETERS WHICH MAKES KEYPAIR--n");
KeyFactory keyFactory = KeyFactory.getInstance("RSA");
RSAPublicKeySpec rsaPubKeySpec = keyFactory.getKeySpec(publicKey, RSAPublicKeySpec.class);
RSAPrivateKeySpec rsaPrivKeySpec = keyFactory.getKeySpec(privateKey, RSAPrivateKeySpec.class);
System.out.println("n--SAVING PUBLIC KEY AND PRIVATE KEY TO FILES--n");
RSAEncryptionHelper rsaObj = new RSAEncryptionHelper();
rsaObj.saveKeys(PUBLIC_KEY_FILE, rsaPubKeySpec.getModulus(), rsaPubKeySpec.getPublicExponent());
rsaObj.saveKeys(PRIVATE_KEY_FILE, rsaPrivKeySpec.getModulus(), rsaPrivKeySpec.getPrivateExponent());
catch (NoSuchAlgorithmException
private void saveKeys(String fileName, BigInteger mod, BigInteger exp) throws IOException
FileOutputStream fos = null;
ObjectOutputStream oos = null;
try
System.out.println("Generating: " + fileName + "...");
fos = new FileOutputStream(fileName);
oos = new ObjectOutputStream(new BufferedOutputStream(fos));
oos.writeObject(mod);
oos.writeObject(exp);
System.out.println(fileName + "generated successfully");;
catch (Exception e)
e.printStackTrace();
finally
if (oos!=null)
oos.close();
if (fos!= null)
fos.close();
public PublicKey readPublicKeyFromFile(String fileName) throws IOException
FileInputStream fis = null;
ObjectInputStream ois = null;
PublicKey publicKey = null;
try
fis = new FileInputStream(new File(fileName));
ois = new ObjectInputStream(fis);
BigInteger modulus = (BigInteger) ois.readObject();
BigInteger exponent = (BigInteger) ois.readObject();
//Get Public Key
RSAPublicKeySpec rsaPublicKeySpec = new RSAPublicKeySpec(modulus, exponent);
KeyFactory fact = KeyFactory.getInstance("RSA");
publicKey = fact.generatePublic(rsaPublicKeySpec);
return publicKey;
catch (IOException e)
e.printStackTrace();
catch (ClassNotFoundException e)
e.printStackTrace();
catch (NoSuchAlgorithmException e)
e.printStackTrace();
catch (InvalidKeySpecException e)
e.printStackTrace();
finally
if (ois != null)
ois.close();
if (fis != null)
fis.close();
return publicKey;
public PrivateKey readPrivateKeyFromFile(String fileName) throws IOException
FileInputStream fis = null;
ObjectInputStream ois = null;
PrivateKey privateKey = null;
try
fis = new FileInputStream(new File(fileName));
ois = new ObjectInputStream(fis);
BigInteger modulus = (BigInteger) ois.readObject();
BigInteger exponent = (BigInteger) ois.readObject();
//Get Public Key
RSAPrivateKeySpec rsaPrivateKeySpec = new RSAPrivateKeySpec(modulus, exponent);
KeyFactory fact = KeyFactory.getInstance("RSA");
privateKey = fact.generatePrivate(rsaPrivateKeySpec);
return privateKey;
catch (IOException e)
e.printStackTrace();
catch (ClassNotFoundException e)
e.printStackTrace();
catch (NoSuchAlgorithmException e)
e.printStackTrace();
catch (InvalidKeySpecException e)
e.printStackTrace();
finally
if (ois != null)
ois.close();
if (fis != null)
fis.close();
return privateKey;
public byte encryptData(String data) throws IOException
System.out.println("n--ENCRYPTION STARTED--");
System.out.println("Data Before Encryption: " + data);
byte dataToEncrypt = data.getBytes();
byte encryptedData = null;
try
PublicKey pubKey = readPublicKeyFromFile(this.REAL_PUBLIC_PATH);
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, pubKey);
encryptedData = cipher.doFinal(dataToEncrypt);
System.out.println("Encrypted Data: " + encryptedData);
catch (IOException e)
e.printStackTrace();
catch (NoSuchAlgorithmException e)
e.printStackTrace();
catch (InvalidKeyException e)
e.printStackTrace();
catch (IllegalBlockSizeException e)
e.printStackTrace();
catch (BadPaddingException e)
e.printStackTrace();
catch (NoSuchPaddingException e)
e.printStackTrace();
System.out.println("--ENCRYPTION COMPLETED--");
return encryptedData;
public String decryptData(byte data) throws IOException
System.out.println("n--DECRYPTION STARTED--");
byte decryptedData = null;
String decData = "";
try
PrivateKey privateKey = readPrivateKeyFromFile(this.REAL_PRIVATE_PATH);
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.DECRYPT_MODE, privateKey);
decryptedData = cipher.doFinal(data);
decData = new String(decryptedData);
System.out.println("Decrypted Data: " + decData);
return decData;
catch (IOException e)
e.printStackTrace();
catch (NoSuchAlgorithmException e)
e.printStackTrace();
catch (NoSuchPaddingException e)
e.printStackTrace();
catch (InvalidKeyException e)
e.printStackTrace();
catch ( IllegalBlockSizeException e)
e.printStackTrace();
catch (BadPaddingException e)
e.printStackTrace();
System.out.println("--DECRYPTION COMPLETED--");
return decData;
java jsp servlets filepath filenotfoundexception
My program is able to create and write to a file using the path-file:
private static final String PUBLIC_KEY_FILE = "WebContent/Config/MyPublic.key";
private static final String PRIVATE_KEY_FILE = "WebContent/Config/MyPrivate.key";
And the correct files are generated within the folders according to the path declared above.
But then a FileNotFoundException error occurs when I try to read from those files using the path files:
private String REAL_PUBLIC_PATH = getServletContext().getRealPath("/WebContent/Config/MyPublic.key");
private String REAL_PRIVATE_PATH = getServletContext().getRealPath("/WebContent/Config/MyPrivate.key");
This is the lines of code that is causing the error:
PublicKey pubKey = readPublicKeyFromFile(this.REAL_PUBLIC_PATH);
PrivateKey privKey = readPrivateKeyFromFile(this.REAL_PRIVATE_PATH);
Which is tied to the variables REAL_PUBLIC_PATH
and REAL_PUBLIC_PATH
. Meaning there's something wrong with how the path file is being read.
My folder structure goes:
> MyProject
>Web-Content
>Config
>MyPublic.key
>MyPrivate.key
>WEB-INF
>META-INF
My full class code is below for context:
public class RSAEncryptionHelper extends HttpServlet
private static final String PUBLIC_KEY_FILE = "WebContent/Config/MyPublic.key";
private static final String PRIVATE_KEY_FILE = "WebContent/Config/MyPrivate.key";
private String REAL_PUBLIC_PATH = getServletContext().getRealPath("/WebContent/Config/MyPublic.key");
private String REAL_PRIVATE_PATH = getServletContext().getRealPath("/WebContent/Config/MyPrivate.key");
public static void main(String args) throws IOException
try
System.out.println("--GENERATE PUBLIC and PRIVATE KEY --");
KeyPairGenerator keyPairGenerator = KeyPairGenerator.getInstance("RSA");
keyPairGenerator.initialize(2048); //1024 for normal securities
KeyPair keyPair = keyPairGenerator.generateKeyPair();
PublicKey publicKey = keyPair.getPublic();
PrivateKey privateKey = keyPair.getPrivate();
System.out.println("n--PULLING OUT PARAMETERS WHICH MAKES KEYPAIR--n");
KeyFactory keyFactory = KeyFactory.getInstance("RSA");
RSAPublicKeySpec rsaPubKeySpec = keyFactory.getKeySpec(publicKey, RSAPublicKeySpec.class);
RSAPrivateKeySpec rsaPrivKeySpec = keyFactory.getKeySpec(privateKey, RSAPrivateKeySpec.class);
System.out.println("n--SAVING PUBLIC KEY AND PRIVATE KEY TO FILES--n");
RSAEncryptionHelper rsaObj = new RSAEncryptionHelper();
rsaObj.saveKeys(PUBLIC_KEY_FILE, rsaPubKeySpec.getModulus(), rsaPubKeySpec.getPublicExponent());
rsaObj.saveKeys(PRIVATE_KEY_FILE, rsaPrivKeySpec.getModulus(), rsaPrivKeySpec.getPrivateExponent());
catch (NoSuchAlgorithmException
private void saveKeys(String fileName, BigInteger mod, BigInteger exp) throws IOException
FileOutputStream fos = null;
ObjectOutputStream oos = null;
try
System.out.println("Generating: " + fileName + "...");
fos = new FileOutputStream(fileName);
oos = new ObjectOutputStream(new BufferedOutputStream(fos));
oos.writeObject(mod);
oos.writeObject(exp);
System.out.println(fileName + "generated successfully");;
catch (Exception e)
e.printStackTrace();
finally
if (oos!=null)
oos.close();
if (fos!= null)
fos.close();
public PublicKey readPublicKeyFromFile(String fileName) throws IOException
FileInputStream fis = null;
ObjectInputStream ois = null;
PublicKey publicKey = null;
try
fis = new FileInputStream(new File(fileName));
ois = new ObjectInputStream(fis);
BigInteger modulus = (BigInteger) ois.readObject();
BigInteger exponent = (BigInteger) ois.readObject();
//Get Public Key
RSAPublicKeySpec rsaPublicKeySpec = new RSAPublicKeySpec(modulus, exponent);
KeyFactory fact = KeyFactory.getInstance("RSA");
publicKey = fact.generatePublic(rsaPublicKeySpec);
return publicKey;
catch (IOException e)
e.printStackTrace();
catch (ClassNotFoundException e)
e.printStackTrace();
catch (NoSuchAlgorithmException e)
e.printStackTrace();
catch (InvalidKeySpecException e)
e.printStackTrace();
finally
if (ois != null)
ois.close();
if (fis != null)
fis.close();
return publicKey;
public PrivateKey readPrivateKeyFromFile(String fileName) throws IOException
FileInputStream fis = null;
ObjectInputStream ois = null;
PrivateKey privateKey = null;
try
fis = new FileInputStream(new File(fileName));
ois = new ObjectInputStream(fis);
BigInteger modulus = (BigInteger) ois.readObject();
BigInteger exponent = (BigInteger) ois.readObject();
//Get Public Key
RSAPrivateKeySpec rsaPrivateKeySpec = new RSAPrivateKeySpec(modulus, exponent);
KeyFactory fact = KeyFactory.getInstance("RSA");
privateKey = fact.generatePrivate(rsaPrivateKeySpec);
return privateKey;
catch (IOException e)
e.printStackTrace();
catch (ClassNotFoundException e)
e.printStackTrace();
catch (NoSuchAlgorithmException e)
e.printStackTrace();
catch (InvalidKeySpecException e)
e.printStackTrace();
finally
if (ois != null)
ois.close();
if (fis != null)
fis.close();
return privateKey;
public byte encryptData(String data) throws IOException
System.out.println("n--ENCRYPTION STARTED--");
System.out.println("Data Before Encryption: " + data);
byte dataToEncrypt = data.getBytes();
byte encryptedData = null;
try
PublicKey pubKey = readPublicKeyFromFile(this.REAL_PUBLIC_PATH);
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.ENCRYPT_MODE, pubKey);
encryptedData = cipher.doFinal(dataToEncrypt);
System.out.println("Encrypted Data: " + encryptedData);
catch (IOException e)
e.printStackTrace();
catch (NoSuchAlgorithmException e)
e.printStackTrace();
catch (InvalidKeyException e)
e.printStackTrace();
catch (IllegalBlockSizeException e)
e.printStackTrace();
catch (BadPaddingException e)
e.printStackTrace();
catch (NoSuchPaddingException e)
e.printStackTrace();
System.out.println("--ENCRYPTION COMPLETED--");
return encryptedData;
public String decryptData(byte data) throws IOException
System.out.println("n--DECRYPTION STARTED--");
byte decryptedData = null;
String decData = "";
try
PrivateKey privateKey = readPrivateKeyFromFile(this.REAL_PRIVATE_PATH);
Cipher cipher = Cipher.getInstance("RSA");
cipher.init(Cipher.DECRYPT_MODE, privateKey);
decryptedData = cipher.doFinal(data);
decData = new String(decryptedData);
System.out.println("Decrypted Data: " + decData);
return decData;
catch (IOException e)
e.printStackTrace();
catch (NoSuchAlgorithmException e)
e.printStackTrace();
catch (NoSuchPaddingException e)
e.printStackTrace();
catch (InvalidKeyException e)
e.printStackTrace();
catch ( IllegalBlockSizeException e)
e.printStackTrace();
catch (BadPaddingException e)
e.printStackTrace();
System.out.println("--DECRYPTION COMPLETED--");
return decData;
java jsp servlets filepath filenotfoundexception
java jsp servlets filepath filenotfoundexception
asked Nov 9 at 20:04
Jae Bin
247
247
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
Do your keys already exist before you run the program? if not, then getRealPath to a file that doesn't exist isn't going to work.
But that shouldn't matter because if you can access the files using private static final String PUBLIC_KEY_FILE, why not use that same path to read the files.
So instead of this:
PrivateKey privateKey = readPrivateKeyFromFile(this.REAL_PRIVATE_PATH);
Why not do this?
PrivateKey privateKey = readPrivateKeyFromFile(this.PRIVATE_KEY_FILE);
If you've sucessfully written to a file using PRIVATE_KEY_FILE path, you can also read from the same path.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Do your keys already exist before you run the program? if not, then getRealPath to a file that doesn't exist isn't going to work.
But that shouldn't matter because if you can access the files using private static final String PUBLIC_KEY_FILE, why not use that same path to read the files.
So instead of this:
PrivateKey privateKey = readPrivateKeyFromFile(this.REAL_PRIVATE_PATH);
Why not do this?
PrivateKey privateKey = readPrivateKeyFromFile(this.PRIVATE_KEY_FILE);
If you've sucessfully written to a file using PRIVATE_KEY_FILE path, you can also read from the same path.
add a comment |
up vote
0
down vote
Do your keys already exist before you run the program? if not, then getRealPath to a file that doesn't exist isn't going to work.
But that shouldn't matter because if you can access the files using private static final String PUBLIC_KEY_FILE, why not use that same path to read the files.
So instead of this:
PrivateKey privateKey = readPrivateKeyFromFile(this.REAL_PRIVATE_PATH);
Why not do this?
PrivateKey privateKey = readPrivateKeyFromFile(this.PRIVATE_KEY_FILE);
If you've sucessfully written to a file using PRIVATE_KEY_FILE path, you can also read from the same path.
add a comment |
up vote
0
down vote
up vote
0
down vote
Do your keys already exist before you run the program? if not, then getRealPath to a file that doesn't exist isn't going to work.
But that shouldn't matter because if you can access the files using private static final String PUBLIC_KEY_FILE, why not use that same path to read the files.
So instead of this:
PrivateKey privateKey = readPrivateKeyFromFile(this.REAL_PRIVATE_PATH);
Why not do this?
PrivateKey privateKey = readPrivateKeyFromFile(this.PRIVATE_KEY_FILE);
If you've sucessfully written to a file using PRIVATE_KEY_FILE path, you can also read from the same path.
Do your keys already exist before you run the program? if not, then getRealPath to a file that doesn't exist isn't going to work.
But that shouldn't matter because if you can access the files using private static final String PUBLIC_KEY_FILE, why not use that same path to read the files.
So instead of this:
PrivateKey privateKey = readPrivateKeyFromFile(this.REAL_PRIVATE_PATH);
Why not do this?
PrivateKey privateKey = readPrivateKeyFromFile(this.PRIVATE_KEY_FILE);
If you've sucessfully written to a file using PRIVATE_KEY_FILE path, you can also read from the same path.
answered Nov 9 at 20:21
Justin
37618
37618
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53232603%2ffilenotfound-exception-when-trying-to-read-file-using-getservletcontext-getrea%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vlhyV,hVy6