How can I get the string with more lowercase characters from a given List?
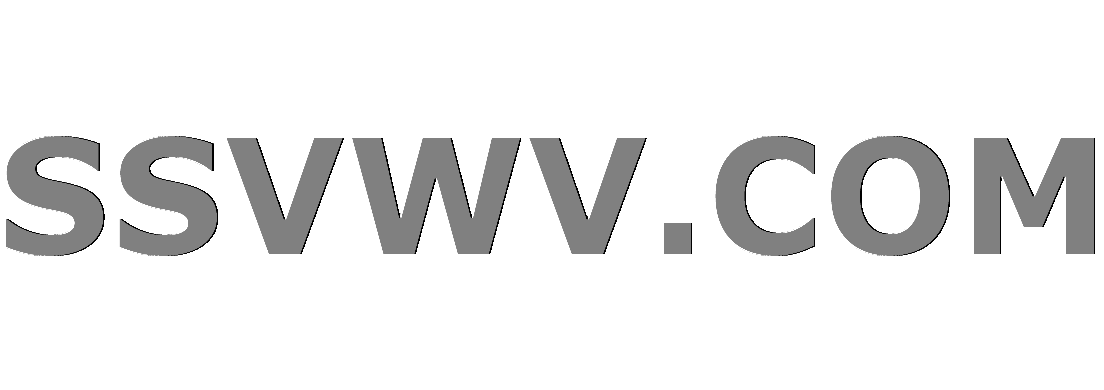
Multi tool use
up vote
1
down vote
favorite
How can I get the string with more lowercase characters from a given List<String>
?
I've already done it in a functional way, using Java 10 Streams, but I want to do it in an iterative way, using while
loops. But I have no idea how to do it.
This is my functional code.
public static Optional<String> stringSearched (List<String> pl)
return pl.stream()
.max(Comparator.comparing(x->x.chars()
.filter(Character::iLowerCase)
.count()
));
java while-loop java-stream
New contributor
Iván Martín Jiménez is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
1
down vote
favorite
How can I get the string with more lowercase characters from a given List<String>
?
I've already done it in a functional way, using Java 10 Streams, but I want to do it in an iterative way, using while
loops. But I have no idea how to do it.
This is my functional code.
public static Optional<String> stringSearched (List<String> pl)
return pl.stream()
.max(Comparator.comparing(x->x.chars()
.filter(Character::iLowerCase)
.count()
));
java while-loop java-stream
New contributor
Iván Martín Jiménez is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
What have you tried already?
– Glitcher
yesterday
Well, I tried to do it with Lists and foreach loop, and from this, use a while loop. But I am in the Engineering Dregee, so teachers want the exercise just as they do it, and I get stucked. Last year we used it Java 8 Streams and I am very confident with then, but we did not study while loops in depth.
– Iván Martín Jiménez
yesterday
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
How can I get the string with more lowercase characters from a given List<String>
?
I've already done it in a functional way, using Java 10 Streams, but I want to do it in an iterative way, using while
loops. But I have no idea how to do it.
This is my functional code.
public static Optional<String> stringSearched (List<String> pl)
return pl.stream()
.max(Comparator.comparing(x->x.chars()
.filter(Character::iLowerCase)
.count()
));
java while-loop java-stream
New contributor
Iván Martín Jiménez is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
How can I get the string with more lowercase characters from a given List<String>
?
I've already done it in a functional way, using Java 10 Streams, but I want to do it in an iterative way, using while
loops. But I have no idea how to do it.
This is my functional code.
public static Optional<String> stringSearched (List<String> pl)
return pl.stream()
.max(Comparator.comparing(x->x.chars()
.filter(Character::iLowerCase)
.count()
));
java while-loop java-stream
java while-loop java-stream
New contributor
Iván Martín Jiménez is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Iván Martín Jiménez is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited yesterday
Stefan Zobel
2,32021827
2,32021827
New contributor
Iván Martín Jiménez is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked yesterday


Iván Martín Jiménez
62
62
New contributor
Iván Martín Jiménez is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Iván Martín Jiménez is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Iván Martín Jiménez is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
What have you tried already?
– Glitcher
yesterday
Well, I tried to do it with Lists and foreach loop, and from this, use a while loop. But I am in the Engineering Dregee, so teachers want the exercise just as they do it, and I get stucked. Last year we used it Java 8 Streams and I am very confident with then, but we did not study while loops in depth.
– Iván Martín Jiménez
yesterday
add a comment |
What have you tried already?
– Glitcher
yesterday
Well, I tried to do it with Lists and foreach loop, and from this, use a while loop. But I am in the Engineering Dregee, so teachers want the exercise just as they do it, and I get stucked. Last year we used it Java 8 Streams and I am very confident with then, but we did not study while loops in depth.
– Iván Martín Jiménez
yesterday
What have you tried already?
– Glitcher
yesterday
What have you tried already?
– Glitcher
yesterday
Well, I tried to do it with Lists and foreach loop, and from this, use a while loop. But I am in the Engineering Dregee, so teachers want the exercise just as they do it, and I get stucked. Last year we used it Java 8 Streams and I am very confident with then, but we did not study while loops in depth.
– Iván Martín Jiménez
yesterday
Well, I tried to do it with Lists and foreach loop, and from this, use a while loop. But I am in the Engineering Dregee, so teachers want the exercise just as they do it, and I get stucked. Last year we used it Java 8 Streams and I am very confident with then, but we did not study while loops in depth.
– Iván Martín Jiménez
yesterday
add a comment |
3 Answers
3
active
oldest
votes
up vote
2
down vote
import java.util.ArrayList;
import java.util.List;
public class Something
public static void main(String args)
ArrayList<String> list = new ArrayList<String>();
list.add("LOSER");
list.add("wInner");
list.add("sECONDpLACE");
System.out.println(findMoreLowerCase(list));
private static String findMoreLowerCase(final List<String> list)
String moreLowerCase = ""; // Tracks the current string with less lowercase
int nrOfLowerCase=0; // Tracks the nr of lowercase for that string
int current; // Used in the for loop
for(String word: list)
current = countLowerCase(word);
if(current > nrOfLowerCase)
// Found a string with more lowercase, update
nrOfLowerCase = current;
moreLowerCase = word;
return moreLowerCase;
private static int countLowerCase(String s)
// Scroll each character and count
int result = 0;
for (char letter : s.toCharArray())
if (Character.isLowerCase(letter))
++result;
;
return result;
That way is very intuitive, but I need to code it with while loops. I´ll see if I can do it from this code. Thank you so much, very helpful :)
– Iván Martín Jiménez
yesterday
Sorry, I just read "loops". Anyway, it should be trivial moving from for loops to while loops, and it can help you understand it better. Let me know if you have problems.
– Tu.ma
yesterday
1
No problem! I will try by my own anyways. Like I said, thanks a lot.
– Iván Martín Jiménez
yesterday
No problem, if you manage to solve please consider marking the answer.
– Tu.ma
yesterday
add a comment |
up vote
0
down vote
The easiest way would to be to iterate over all your words and replace non lower case letters (with a regular expression such as [^a-z]) with empty strings, and then get the length of the string.
The longest string is the one that has the most lower case letters.
add a comment |
up vote
0
down vote
private String moreLowerCase(final List<String> stringList)
String moreLowerCase = "";
final Iterator<String> iterator = stringList.iterator();
while (iterator.hasNext())
final String current = iterator.next().replaceAll("[^a-z]", "");
if (current.length() > moreLowerCase.length())
moreLowerCase = current;
return moreLowerCase;
New contributor
Volodymyr Strontsitskyi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
import java.util.ArrayList;
import java.util.List;
public class Something
public static void main(String args)
ArrayList<String> list = new ArrayList<String>();
list.add("LOSER");
list.add("wInner");
list.add("sECONDpLACE");
System.out.println(findMoreLowerCase(list));
private static String findMoreLowerCase(final List<String> list)
String moreLowerCase = ""; // Tracks the current string with less lowercase
int nrOfLowerCase=0; // Tracks the nr of lowercase for that string
int current; // Used in the for loop
for(String word: list)
current = countLowerCase(word);
if(current > nrOfLowerCase)
// Found a string with more lowercase, update
nrOfLowerCase = current;
moreLowerCase = word;
return moreLowerCase;
private static int countLowerCase(String s)
// Scroll each character and count
int result = 0;
for (char letter : s.toCharArray())
if (Character.isLowerCase(letter))
++result;
;
return result;
That way is very intuitive, but I need to code it with while loops. I´ll see if I can do it from this code. Thank you so much, very helpful :)
– Iván Martín Jiménez
yesterday
Sorry, I just read "loops". Anyway, it should be trivial moving from for loops to while loops, and it can help you understand it better. Let me know if you have problems.
– Tu.ma
yesterday
1
No problem! I will try by my own anyways. Like I said, thanks a lot.
– Iván Martín Jiménez
yesterday
No problem, if you manage to solve please consider marking the answer.
– Tu.ma
yesterday
add a comment |
up vote
2
down vote
import java.util.ArrayList;
import java.util.List;
public class Something
public static void main(String args)
ArrayList<String> list = new ArrayList<String>();
list.add("LOSER");
list.add("wInner");
list.add("sECONDpLACE");
System.out.println(findMoreLowerCase(list));
private static String findMoreLowerCase(final List<String> list)
String moreLowerCase = ""; // Tracks the current string with less lowercase
int nrOfLowerCase=0; // Tracks the nr of lowercase for that string
int current; // Used in the for loop
for(String word: list)
current = countLowerCase(word);
if(current > nrOfLowerCase)
// Found a string with more lowercase, update
nrOfLowerCase = current;
moreLowerCase = word;
return moreLowerCase;
private static int countLowerCase(String s)
// Scroll each character and count
int result = 0;
for (char letter : s.toCharArray())
if (Character.isLowerCase(letter))
++result;
;
return result;
That way is very intuitive, but I need to code it with while loops. I´ll see if I can do it from this code. Thank you so much, very helpful :)
– Iván Martín Jiménez
yesterday
Sorry, I just read "loops". Anyway, it should be trivial moving from for loops to while loops, and it can help you understand it better. Let me know if you have problems.
– Tu.ma
yesterday
1
No problem! I will try by my own anyways. Like I said, thanks a lot.
– Iván Martín Jiménez
yesterday
No problem, if you manage to solve please consider marking the answer.
– Tu.ma
yesterday
add a comment |
up vote
2
down vote
up vote
2
down vote
import java.util.ArrayList;
import java.util.List;
public class Something
public static void main(String args)
ArrayList<String> list = new ArrayList<String>();
list.add("LOSER");
list.add("wInner");
list.add("sECONDpLACE");
System.out.println(findMoreLowerCase(list));
private static String findMoreLowerCase(final List<String> list)
String moreLowerCase = ""; // Tracks the current string with less lowercase
int nrOfLowerCase=0; // Tracks the nr of lowercase for that string
int current; // Used in the for loop
for(String word: list)
current = countLowerCase(word);
if(current > nrOfLowerCase)
// Found a string with more lowercase, update
nrOfLowerCase = current;
moreLowerCase = word;
return moreLowerCase;
private static int countLowerCase(String s)
// Scroll each character and count
int result = 0;
for (char letter : s.toCharArray())
if (Character.isLowerCase(letter))
++result;
;
return result;
import java.util.ArrayList;
import java.util.List;
public class Something
public static void main(String args)
ArrayList<String> list = new ArrayList<String>();
list.add("LOSER");
list.add("wInner");
list.add("sECONDpLACE");
System.out.println(findMoreLowerCase(list));
private static String findMoreLowerCase(final List<String> list)
String moreLowerCase = ""; // Tracks the current string with less lowercase
int nrOfLowerCase=0; // Tracks the nr of lowercase for that string
int current; // Used in the for loop
for(String word: list)
current = countLowerCase(word);
if(current > nrOfLowerCase)
// Found a string with more lowercase, update
nrOfLowerCase = current;
moreLowerCase = word;
return moreLowerCase;
private static int countLowerCase(String s)
// Scroll each character and count
int result = 0;
for (char letter : s.toCharArray())
if (Character.isLowerCase(letter))
++result;
;
return result;
answered yesterday
Tu.ma
358111
358111
That way is very intuitive, but I need to code it with while loops. I´ll see if I can do it from this code. Thank you so much, very helpful :)
– Iván Martín Jiménez
yesterday
Sorry, I just read "loops". Anyway, it should be trivial moving from for loops to while loops, and it can help you understand it better. Let me know if you have problems.
– Tu.ma
yesterday
1
No problem! I will try by my own anyways. Like I said, thanks a lot.
– Iván Martín Jiménez
yesterday
No problem, if you manage to solve please consider marking the answer.
– Tu.ma
yesterday
add a comment |
That way is very intuitive, but I need to code it with while loops. I´ll see if I can do it from this code. Thank you so much, very helpful :)
– Iván Martín Jiménez
yesterday
Sorry, I just read "loops". Anyway, it should be trivial moving from for loops to while loops, and it can help you understand it better. Let me know if you have problems.
– Tu.ma
yesterday
1
No problem! I will try by my own anyways. Like I said, thanks a lot.
– Iván Martín Jiménez
yesterday
No problem, if you manage to solve please consider marking the answer.
– Tu.ma
yesterday
That way is very intuitive, but I need to code it with while loops. I´ll see if I can do it from this code. Thank you so much, very helpful :)
– Iván Martín Jiménez
yesterday
That way is very intuitive, but I need to code it with while loops. I´ll see if I can do it from this code. Thank you so much, very helpful :)
– Iván Martín Jiménez
yesterday
Sorry, I just read "loops". Anyway, it should be trivial moving from for loops to while loops, and it can help you understand it better. Let me know if you have problems.
– Tu.ma
yesterday
Sorry, I just read "loops". Anyway, it should be trivial moving from for loops to while loops, and it can help you understand it better. Let me know if you have problems.
– Tu.ma
yesterday
1
1
No problem! I will try by my own anyways. Like I said, thanks a lot.
– Iván Martín Jiménez
yesterday
No problem! I will try by my own anyways. Like I said, thanks a lot.
– Iván Martín Jiménez
yesterday
No problem, if you manage to solve please consider marking the answer.
– Tu.ma
yesterday
No problem, if you manage to solve please consider marking the answer.
– Tu.ma
yesterday
add a comment |
up vote
0
down vote
The easiest way would to be to iterate over all your words and replace non lower case letters (with a regular expression such as [^a-z]) with empty strings, and then get the length of the string.
The longest string is the one that has the most lower case letters.
add a comment |
up vote
0
down vote
The easiest way would to be to iterate over all your words and replace non lower case letters (with a regular expression such as [^a-z]) with empty strings, and then get the length of the string.
The longest string is the one that has the most lower case letters.
add a comment |
up vote
0
down vote
up vote
0
down vote
The easiest way would to be to iterate over all your words and replace non lower case letters (with a regular expression such as [^a-z]) with empty strings, and then get the length of the string.
The longest string is the one that has the most lower case letters.
The easiest way would to be to iterate over all your words and replace non lower case letters (with a regular expression such as [^a-z]) with empty strings, and then get the length of the string.
The longest string is the one that has the most lower case letters.
answered yesterday
npinti
45.8k55481
45.8k55481
add a comment |
add a comment |
up vote
0
down vote
private String moreLowerCase(final List<String> stringList)
String moreLowerCase = "";
final Iterator<String> iterator = stringList.iterator();
while (iterator.hasNext())
final String current = iterator.next().replaceAll("[^a-z]", "");
if (current.length() > moreLowerCase.length())
moreLowerCase = current;
return moreLowerCase;
New contributor
Volodymyr Strontsitskyi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
private String moreLowerCase(final List<String> stringList)
String moreLowerCase = "";
final Iterator<String> iterator = stringList.iterator();
while (iterator.hasNext())
final String current = iterator.next().replaceAll("[^a-z]", "");
if (current.length() > moreLowerCase.length())
moreLowerCase = current;
return moreLowerCase;
New contributor
Volodymyr Strontsitskyi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
up vote
0
down vote
private String moreLowerCase(final List<String> stringList)
String moreLowerCase = "";
final Iterator<String> iterator = stringList.iterator();
while (iterator.hasNext())
final String current = iterator.next().replaceAll("[^a-z]", "");
if (current.length() > moreLowerCase.length())
moreLowerCase = current;
return moreLowerCase;
New contributor
Volodymyr Strontsitskyi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
private String moreLowerCase(final List<String> stringList)
String moreLowerCase = "";
final Iterator<String> iterator = stringList.iterator();
while (iterator.hasNext())
final String current = iterator.next().replaceAll("[^a-z]", "");
if (current.length() > moreLowerCase.length())
moreLowerCase = current;
return moreLowerCase;
New contributor
Volodymyr Strontsitskyi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Volodymyr Strontsitskyi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered yesterday
Volodymyr Strontsitskyi
1
1
New contributor
Volodymyr Strontsitskyi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Volodymyr Strontsitskyi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Volodymyr Strontsitskyi is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
Iván Martín Jiménez is a new contributor. Be nice, and check out our Code of Conduct.
Iván Martín Jiménez is a new contributor. Be nice, and check out our Code of Conduct.
Iván Martín Jiménez is a new contributor. Be nice, and check out our Code of Conduct.
Iván Martín Jiménez is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53224385%2fhow-can-i-get-the-string-with-more-lowercase-characters-from-a-given-list%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Fh X,w uZnY4iPOPMULwW79,PA
What have you tried already?
– Glitcher
yesterday
Well, I tried to do it with Lists and foreach loop, and from this, use a while loop. But I am in the Engineering Dregee, so teachers want the exercise just as they do it, and I get stucked. Last year we used it Java 8 Streams and I am very confident with then, but we did not study while loops in depth.
– Iván Martín Jiménez
yesterday