Hibernate Fetch is not longer sub-type of Join
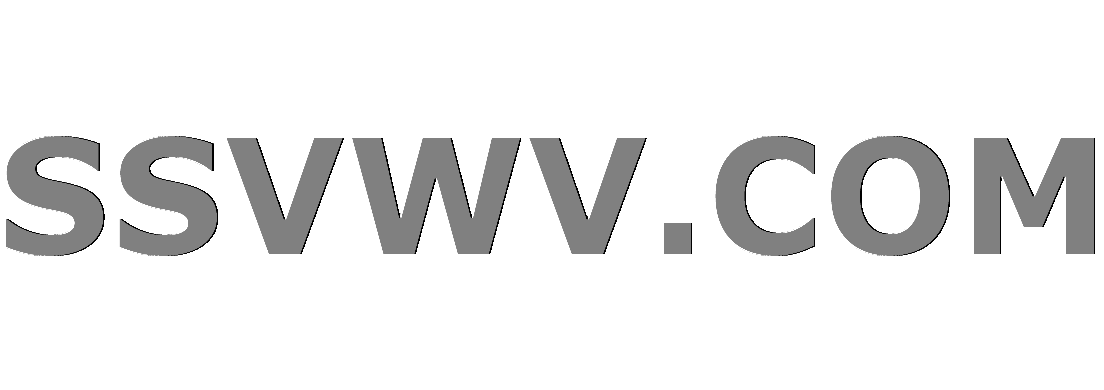
Multi tool use
I'm using Hibernate 5.2.10 with dynamic criteria and find out that Fetch is not longer assignable to Join.
In the next example, I need to fetch group data in the same query, but I also need to use the group's field for sorting or restriction.
CriteriaBuilder cb = session.getCriteriaBuilder();
CriteriaQuery<GroupAssignment> query = cb.createQuery(GroupAssignment.class);
Root<GroupAssignment> root = query.from(GroupAssignment.class);
SingularAttributeJoin<GroupAssignment, Group> groupFetch = (SingularAttributeJoin<GroupAssignment, Group>) root.fetch(GroupAssignment_.group, JoinType.LEFT);
query.orderBy(cb.asc(groupFetch.get(Group_.title)));
I have manually cast Fetch to the SingularAttributeJoin and after that, I can use get method for the ordering purpose, but I'm looking for the right way how I can do that without casting manually.
GroupAssignment class:
@Entity()
@Table(name = "group_assignment")
public class GroupAssignment
@Id
@Column(name = "group_assignment_id")
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
@ManyToOne
@JoinColumn(name = "group_id", nullable = false)
private Group group;
//other fields, getters and setters
GroupAssignment_ class:
@Generated(value = "org.hibernate.jpamodelgen.JPAMetaModelEntityProcessor")
@StaticMetamodel(GroupAssignment.class)
public abstract class GroupAssignment_
public static volatile SingularAttribute<GroupAssignment, Integer> id;
public static volatile SingularAttribute<GroupAssignment, Group> group;
Group class:
@Entity()
@Table(name = "navigation_group")
public class Group
@Id
@Column(name = "group_id")
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
@Column(name = "title")
private String title;
//other fields, getters and setters
Group_ class:
@Generated(value = "org.hibernate.jpamodelgen.JPAMetaModelEntityProcessor")
@StaticMetamodel(Group.class)
public abstract class Group_
public static volatile SingularAttribute<Group, Integer> id;
public static volatile SingularAttribute<Group, String> title;
java hibernate jpa join fetch
|
show 2 more comments
I'm using Hibernate 5.2.10 with dynamic criteria and find out that Fetch is not longer assignable to Join.
In the next example, I need to fetch group data in the same query, but I also need to use the group's field for sorting or restriction.
CriteriaBuilder cb = session.getCriteriaBuilder();
CriteriaQuery<GroupAssignment> query = cb.createQuery(GroupAssignment.class);
Root<GroupAssignment> root = query.from(GroupAssignment.class);
SingularAttributeJoin<GroupAssignment, Group> groupFetch = (SingularAttributeJoin<GroupAssignment, Group>) root.fetch(GroupAssignment_.group, JoinType.LEFT);
query.orderBy(cb.asc(groupFetch.get(Group_.title)));
I have manually cast Fetch to the SingularAttributeJoin and after that, I can use get method for the ordering purpose, but I'm looking for the right way how I can do that without casting manually.
GroupAssignment class:
@Entity()
@Table(name = "group_assignment")
public class GroupAssignment
@Id
@Column(name = "group_assignment_id")
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
@ManyToOne
@JoinColumn(name = "group_id", nullable = false)
private Group group;
//other fields, getters and setters
GroupAssignment_ class:
@Generated(value = "org.hibernate.jpamodelgen.JPAMetaModelEntityProcessor")
@StaticMetamodel(GroupAssignment.class)
public abstract class GroupAssignment_
public static volatile SingularAttribute<GroupAssignment, Integer> id;
public static volatile SingularAttribute<GroupAssignment, Group> group;
Group class:
@Entity()
@Table(name = "navigation_group")
public class Group
@Id
@Column(name = "group_id")
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
@Column(name = "title")
private String title;
//other fields, getters and setters
Group_ class:
@Generated(value = "org.hibernate.jpamodelgen.JPAMetaModelEntityProcessor")
@StaticMetamodel(Group.class)
public abstract class Group_
public static volatile SingularAttribute<Group, Integer> id;
public static volatile SingularAttribute<Group, String> title;
java hibernate jpa join fetch
Can you put the code where you try to cast?
– michaeak
Nov 13 '18 at 12:20
It is already present in the original description (casting to the SingularAttributeJoin)
– Deplake
Nov 13 '18 at 12:22
Is thisCriteriaBuilder
the Hibernate-own CriteriaBuilder? Because there is ajavax.persistence.criteria.CriteriaBuilder
which is quite well documented
– michaeak
Nov 13 '18 at 12:26
Yes, it is javax.persistence.criteria.CriteriaBuilder
– Deplake
Nov 13 '18 at 12:30
Ok, good. Is it possible to post the Group, GroupAssignment, Group_ and GroupAssignment_ classes?
– michaeak
Nov 13 '18 at 12:36
|
show 2 more comments
I'm using Hibernate 5.2.10 with dynamic criteria and find out that Fetch is not longer assignable to Join.
In the next example, I need to fetch group data in the same query, but I also need to use the group's field for sorting or restriction.
CriteriaBuilder cb = session.getCriteriaBuilder();
CriteriaQuery<GroupAssignment> query = cb.createQuery(GroupAssignment.class);
Root<GroupAssignment> root = query.from(GroupAssignment.class);
SingularAttributeJoin<GroupAssignment, Group> groupFetch = (SingularAttributeJoin<GroupAssignment, Group>) root.fetch(GroupAssignment_.group, JoinType.LEFT);
query.orderBy(cb.asc(groupFetch.get(Group_.title)));
I have manually cast Fetch to the SingularAttributeJoin and after that, I can use get method for the ordering purpose, but I'm looking for the right way how I can do that without casting manually.
GroupAssignment class:
@Entity()
@Table(name = "group_assignment")
public class GroupAssignment
@Id
@Column(name = "group_assignment_id")
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
@ManyToOne
@JoinColumn(name = "group_id", nullable = false)
private Group group;
//other fields, getters and setters
GroupAssignment_ class:
@Generated(value = "org.hibernate.jpamodelgen.JPAMetaModelEntityProcessor")
@StaticMetamodel(GroupAssignment.class)
public abstract class GroupAssignment_
public static volatile SingularAttribute<GroupAssignment, Integer> id;
public static volatile SingularAttribute<GroupAssignment, Group> group;
Group class:
@Entity()
@Table(name = "navigation_group")
public class Group
@Id
@Column(name = "group_id")
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
@Column(name = "title")
private String title;
//other fields, getters and setters
Group_ class:
@Generated(value = "org.hibernate.jpamodelgen.JPAMetaModelEntityProcessor")
@StaticMetamodel(Group.class)
public abstract class Group_
public static volatile SingularAttribute<Group, Integer> id;
public static volatile SingularAttribute<Group, String> title;
java hibernate jpa join fetch
I'm using Hibernate 5.2.10 with dynamic criteria and find out that Fetch is not longer assignable to Join.
In the next example, I need to fetch group data in the same query, but I also need to use the group's field for sorting or restriction.
CriteriaBuilder cb = session.getCriteriaBuilder();
CriteriaQuery<GroupAssignment> query = cb.createQuery(GroupAssignment.class);
Root<GroupAssignment> root = query.from(GroupAssignment.class);
SingularAttributeJoin<GroupAssignment, Group> groupFetch = (SingularAttributeJoin<GroupAssignment, Group>) root.fetch(GroupAssignment_.group, JoinType.LEFT);
query.orderBy(cb.asc(groupFetch.get(Group_.title)));
I have manually cast Fetch to the SingularAttributeJoin and after that, I can use get method for the ordering purpose, but I'm looking for the right way how I can do that without casting manually.
GroupAssignment class:
@Entity()
@Table(name = "group_assignment")
public class GroupAssignment
@Id
@Column(name = "group_assignment_id")
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
@ManyToOne
@JoinColumn(name = "group_id", nullable = false)
private Group group;
//other fields, getters and setters
GroupAssignment_ class:
@Generated(value = "org.hibernate.jpamodelgen.JPAMetaModelEntityProcessor")
@StaticMetamodel(GroupAssignment.class)
public abstract class GroupAssignment_
public static volatile SingularAttribute<GroupAssignment, Integer> id;
public static volatile SingularAttribute<GroupAssignment, Group> group;
Group class:
@Entity()
@Table(name = "navigation_group")
public class Group
@Id
@Column(name = "group_id")
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Integer id;
@Column(name = "title")
private String title;
//other fields, getters and setters
Group_ class:
@Generated(value = "org.hibernate.jpamodelgen.JPAMetaModelEntityProcessor")
@StaticMetamodel(Group.class)
public abstract class Group_
public static volatile SingularAttribute<Group, Integer> id;
public static volatile SingularAttribute<Group, String> title;
java hibernate jpa join fetch
java hibernate jpa join fetch
edited Nov 13 '18 at 17:54


michaeak
779315
779315
asked Oct 2 '18 at 14:48
DeplakeDeplake
385419
385419
Can you put the code where you try to cast?
– michaeak
Nov 13 '18 at 12:20
It is already present in the original description (casting to the SingularAttributeJoin)
– Deplake
Nov 13 '18 at 12:22
Is thisCriteriaBuilder
the Hibernate-own CriteriaBuilder? Because there is ajavax.persistence.criteria.CriteriaBuilder
which is quite well documented
– michaeak
Nov 13 '18 at 12:26
Yes, it is javax.persistence.criteria.CriteriaBuilder
– Deplake
Nov 13 '18 at 12:30
Ok, good. Is it possible to post the Group, GroupAssignment, Group_ and GroupAssignment_ classes?
– michaeak
Nov 13 '18 at 12:36
|
show 2 more comments
Can you put the code where you try to cast?
– michaeak
Nov 13 '18 at 12:20
It is already present in the original description (casting to the SingularAttributeJoin)
– Deplake
Nov 13 '18 at 12:22
Is thisCriteriaBuilder
the Hibernate-own CriteriaBuilder? Because there is ajavax.persistence.criteria.CriteriaBuilder
which is quite well documented
– michaeak
Nov 13 '18 at 12:26
Yes, it is javax.persistence.criteria.CriteriaBuilder
– Deplake
Nov 13 '18 at 12:30
Ok, good. Is it possible to post the Group, GroupAssignment, Group_ and GroupAssignment_ classes?
– michaeak
Nov 13 '18 at 12:36
Can you put the code where you try to cast?
– michaeak
Nov 13 '18 at 12:20
Can you put the code where you try to cast?
– michaeak
Nov 13 '18 at 12:20
It is already present in the original description (casting to the SingularAttributeJoin)
– Deplake
Nov 13 '18 at 12:22
It is already present in the original description (casting to the SingularAttributeJoin)
– Deplake
Nov 13 '18 at 12:22
Is this
CriteriaBuilder
the Hibernate-own CriteriaBuilder? Because there is a javax.persistence.criteria.CriteriaBuilder
which is quite well documented– michaeak
Nov 13 '18 at 12:26
Is this
CriteriaBuilder
the Hibernate-own CriteriaBuilder? Because there is a javax.persistence.criteria.CriteriaBuilder
which is quite well documented– michaeak
Nov 13 '18 at 12:26
Yes, it is javax.persistence.criteria.CriteriaBuilder
– Deplake
Nov 13 '18 at 12:30
Yes, it is javax.persistence.criteria.CriteriaBuilder
– Deplake
Nov 13 '18 at 12:30
Ok, good. Is it possible to post the Group, GroupAssignment, Group_ and GroupAssignment_ classes?
– michaeak
Nov 13 '18 at 12:36
Ok, good. Is it possible to post the Group, GroupAssignment, Group_ and GroupAssignment_ classes?
– michaeak
Nov 13 '18 at 12:36
|
show 2 more comments
1 Answer
1
active
oldest
votes
I think that javax.persistence.criteria.FetchParent may solve your problem:
FetchParent<GroupAssignment, Group> groupFetch = root.fetch(GroupAssignment_.group, JoinType.LEFT);
1
I don't see how FetchParent can solve my issue. It is actually a superclass of Fetch, so it has even less public methods then Fetch...
– Deplake
Nov 14 '18 at 8:11
You are right. I had to do almost the same, using the FetchParent and manually casting to Join too, but later in the code, so I missed it when I first checked to see how I did. Sorry.
– Sannon Aragão
Nov 14 '18 at 13:40
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f52610873%2fhibernate-fetch-is-not-longer-sub-type-of-join%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think that javax.persistence.criteria.FetchParent may solve your problem:
FetchParent<GroupAssignment, Group> groupFetch = root.fetch(GroupAssignment_.group, JoinType.LEFT);
1
I don't see how FetchParent can solve my issue. It is actually a superclass of Fetch, so it has even less public methods then Fetch...
– Deplake
Nov 14 '18 at 8:11
You are right. I had to do almost the same, using the FetchParent and manually casting to Join too, but later in the code, so I missed it when I first checked to see how I did. Sorry.
– Sannon Aragão
Nov 14 '18 at 13:40
add a comment |
I think that javax.persistence.criteria.FetchParent may solve your problem:
FetchParent<GroupAssignment, Group> groupFetch = root.fetch(GroupAssignment_.group, JoinType.LEFT);
1
I don't see how FetchParent can solve my issue. It is actually a superclass of Fetch, so it has even less public methods then Fetch...
– Deplake
Nov 14 '18 at 8:11
You are right. I had to do almost the same, using the FetchParent and manually casting to Join too, but later in the code, so I missed it when I first checked to see how I did. Sorry.
– Sannon Aragão
Nov 14 '18 at 13:40
add a comment |
I think that javax.persistence.criteria.FetchParent may solve your problem:
FetchParent<GroupAssignment, Group> groupFetch = root.fetch(GroupAssignment_.group, JoinType.LEFT);
I think that javax.persistence.criteria.FetchParent may solve your problem:
FetchParent<GroupAssignment, Group> groupFetch = root.fetch(GroupAssignment_.group, JoinType.LEFT);
answered Nov 13 '18 at 18:38
Sannon AragãoSannon Aragão
58112
58112
1
I don't see how FetchParent can solve my issue. It is actually a superclass of Fetch, so it has even less public methods then Fetch...
– Deplake
Nov 14 '18 at 8:11
You are right. I had to do almost the same, using the FetchParent and manually casting to Join too, but later in the code, so I missed it when I first checked to see how I did. Sorry.
– Sannon Aragão
Nov 14 '18 at 13:40
add a comment |
1
I don't see how FetchParent can solve my issue. It is actually a superclass of Fetch, so it has even less public methods then Fetch...
– Deplake
Nov 14 '18 at 8:11
You are right. I had to do almost the same, using the FetchParent and manually casting to Join too, but later in the code, so I missed it when I first checked to see how I did. Sorry.
– Sannon Aragão
Nov 14 '18 at 13:40
1
1
I don't see how FetchParent can solve my issue. It is actually a superclass of Fetch, so it has even less public methods then Fetch...
– Deplake
Nov 14 '18 at 8:11
I don't see how FetchParent can solve my issue. It is actually a superclass of Fetch, so it has even less public methods then Fetch...
– Deplake
Nov 14 '18 at 8:11
You are right. I had to do almost the same, using the FetchParent and manually casting to Join too, but later in the code, so I missed it when I first checked to see how I did. Sorry.
– Sannon Aragão
Nov 14 '18 at 13:40
You are right. I had to do almost the same, using the FetchParent and manually casting to Join too, but later in the code, so I missed it when I first checked to see how I did. Sorry.
– Sannon Aragão
Nov 14 '18 at 13:40
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f52610873%2fhibernate-fetch-is-not-longer-sub-type-of-join%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
99nVhtOxd,rMjpbc0i2P8Ri
Can you put the code where you try to cast?
– michaeak
Nov 13 '18 at 12:20
It is already present in the original description (casting to the SingularAttributeJoin)
– Deplake
Nov 13 '18 at 12:22
Is this
CriteriaBuilder
the Hibernate-own CriteriaBuilder? Because there is ajavax.persistence.criteria.CriteriaBuilder
which is quite well documented– michaeak
Nov 13 '18 at 12:26
Yes, it is javax.persistence.criteria.CriteriaBuilder
– Deplake
Nov 13 '18 at 12:30
Ok, good. Is it possible to post the Group, GroupAssignment, Group_ and GroupAssignment_ classes?
– michaeak
Nov 13 '18 at 12:36