How can I get the name of the module where a Table instance was declared?
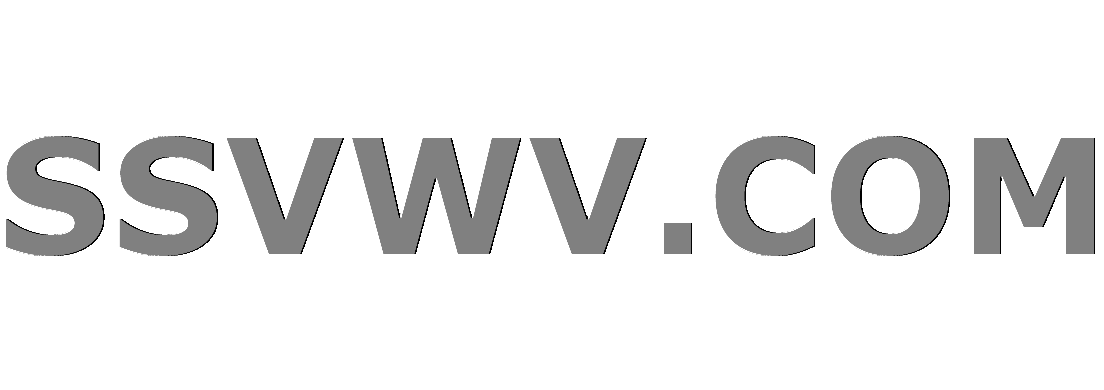
Multi tool use
I have a sqlalchemy table defined like so
from sqlalchemy.sql.schema import Table
my_table = Table(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True),
...
)
I am trying to inspect this instance to get the module where it was created. I have tried using sqlalchemy.inspect(my_table).__module__
, my_table.__module__
, and inspect.getmodule(my_table)
however, all three return sqlalchemy.sql.schema
which is the module where Table
is defined rather than where my_table
is defined.
How can I retrieve the name of the module where I have instantiated my_table
?
python sqlalchemy
add a comment |
I have a sqlalchemy table defined like so
from sqlalchemy.sql.schema import Table
my_table = Table(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True),
...
)
I am trying to inspect this instance to get the module where it was created. I have tried using sqlalchemy.inspect(my_table).__module__
, my_table.__module__
, and inspect.getmodule(my_table)
however, all three return sqlalchemy.sql.schema
which is the module where Table
is defined rather than where my_table
is defined.
How can I retrieve the name of the module where I have instantiated my_table
?
python sqlalchemy
add a comment |
I have a sqlalchemy table defined like so
from sqlalchemy.sql.schema import Table
my_table = Table(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True),
...
)
I am trying to inspect this instance to get the module where it was created. I have tried using sqlalchemy.inspect(my_table).__module__
, my_table.__module__
, and inspect.getmodule(my_table)
however, all three return sqlalchemy.sql.schema
which is the module where Table
is defined rather than where my_table
is defined.
How can I retrieve the name of the module where I have instantiated my_table
?
python sqlalchemy
I have a sqlalchemy table defined like so
from sqlalchemy.sql.schema import Table
my_table = Table(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True),
...
)
I am trying to inspect this instance to get the module where it was created. I have tried using sqlalchemy.inspect(my_table).__module__
, my_table.__module__
, and inspect.getmodule(my_table)
however, all three return sqlalchemy.sql.schema
which is the module where Table
is defined rather than where my_table
is defined.
How can I retrieve the name of the module where I have instantiated my_table
?
python sqlalchemy
python sqlalchemy
edited Nov 15 '18 at 1:48
SuperShoot
1,855721
1,855721
asked Nov 14 '18 at 14:48
RainbaconRainbacon
60011522
60011522
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
You could add the functionality by subclassing Table
. In SQLAlchemy, Table
specifically overrides Table.__init__()
to make it a no-op:
def __init__(self, *args, **kw):
"""Constructor for :class:`~.schema.Table`.
This method is a no-op. See the top-level
documentation for :class:`~.schema.Table`
for constructor arguments.
"""
# __init__ is overridden to prevent __new__ from
# calling the superclass constructor.
The key being that it does not invoke super().__init__()
, so that sqlalchemy can take command of instantiation and whatever you do, that needs to be maintained.
from sqlalchemy.sql.schema import Table
class MyTable(Table):
def __init__(self, *args, **kwargs):
self._where_am_i = __file__
my_table = MyTable(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True)
)
In this case, MyTable.__init__()
is still blocking the superclass constructor, but it also adds an attribute to the instance which will be the name of the module that the class is instantiated within. I specifically chose an obscure attribute name (_where_am_i
) that is unlikely to be overwritten by sqlalchemy and using __file__
returns the path of the module (but you can make that anything you want).
I tested that inserts and selects still work:
import logging
from sqlalchemy.sql import select
logging.getLogger('sqlalchemy.engine').setLevel(logging.INFO)
logging.basicConfig(level=logging.INFO)
Base.metadata.drop_all(engine)
Base.metadata.create_all(engine)
conn = engine.connect()
conn.execute(my_table.insert(), ["my_id": i for i in range(1, 6)])
s = select([my_table])
result = conn.execute(s)
for row in result:
print(row)
# (1,)
# (2,)
# (3,)
# (4,)
# (5,)
And instantiation location:
print(my_table._where_am_i) # 53302898.py (that's the name of my module).
External module:
# external_module.py
from sqlalchemy_app import Base
from sqlalchemy.sql.schema import Table
from sqlalchemy import Column, BigInteger
metadata = Base.metadata
class MyTable(Table):
def __init__(self, *args, **kwargs):
self._where_am_i = __file__
my_table = MyTable(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True)
)
And:
# 53302898.py
from external_module import my_table
if __name__ == '__main__':
print(my_table._where_am_i) # prints C:Userspeter_000OneDrivegittestexternal_module.py
Note how it returned the relative file path in the first test and the absolute file path in the external module test. You can read about that here: Python __file__ attribute absolute or relative? but you can make that _where_am_i
attribute return whatever you need to suit your application.
EDIT
The above solution requires subclassing the Table
class inside the module where instances are formed, otherwise it will peg the module where the Class is instantiated, not the instances. If you only want to subclass Table
once in your project you'd need to pass the location to the constructor.
This works:
class MyTable(Table):
def __init__(self, *args, _where_am_i=None, **kwargs):
self._where_am_i = _where_am_i
...but you get a warning upon instantiation:
SAWarning: Can't validate argument '_where_am_i'; can't locate any SQLAlchemy dialect named '_where'
.
To avoid that, you'd have to override sqlalchemy's alternate constructor, Table._init()
, strip out the location parameter and then delegate back up the chain:
class MyTable(Table):
def _init(self, *args, _where_am_i=None, **kwargs):
self._where_am_i = _where_am_i
super()._init(*args, **kwargs)
Import from external module:
# 53302898.py
from external_module import MyTable
my_table = MyTable(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True),
_where_am_i = __file__
)
if __name__ == '__main__':
print(my_table._where_am_i) # 53302898.py
All above tests still pass.
Unfortunately, this solution doesn't scale well. If I putMyTable
into a separate file so that I can share it between several tables, it gets the location of that file rather than the file where the table was instantiated.
– Rainbacon
Nov 15 '18 at 14:32
Then your only option is to pass it in at instantiation time. See edit.
– SuperShoot
Nov 15 '18 at 19:38
add a comment |
You can't. If you refer to the Python documentation index, you see that there are three entries for __module__
: one for a class attribute, one for a function attribute, and one for a method attribute. Only those types of objects have the module in which they were declared recorded. my_table
is none of these; it's just an instance of the Table
class, so the only __module__
you can find on it is Table.__module__
.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53302898%2fhow-can-i-get-the-name-of-the-module-where-a-table-instance-was-declared%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You could add the functionality by subclassing Table
. In SQLAlchemy, Table
specifically overrides Table.__init__()
to make it a no-op:
def __init__(self, *args, **kw):
"""Constructor for :class:`~.schema.Table`.
This method is a no-op. See the top-level
documentation for :class:`~.schema.Table`
for constructor arguments.
"""
# __init__ is overridden to prevent __new__ from
# calling the superclass constructor.
The key being that it does not invoke super().__init__()
, so that sqlalchemy can take command of instantiation and whatever you do, that needs to be maintained.
from sqlalchemy.sql.schema import Table
class MyTable(Table):
def __init__(self, *args, **kwargs):
self._where_am_i = __file__
my_table = MyTable(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True)
)
In this case, MyTable.__init__()
is still blocking the superclass constructor, but it also adds an attribute to the instance which will be the name of the module that the class is instantiated within. I specifically chose an obscure attribute name (_where_am_i
) that is unlikely to be overwritten by sqlalchemy and using __file__
returns the path of the module (but you can make that anything you want).
I tested that inserts and selects still work:
import logging
from sqlalchemy.sql import select
logging.getLogger('sqlalchemy.engine').setLevel(logging.INFO)
logging.basicConfig(level=logging.INFO)
Base.metadata.drop_all(engine)
Base.metadata.create_all(engine)
conn = engine.connect()
conn.execute(my_table.insert(), ["my_id": i for i in range(1, 6)])
s = select([my_table])
result = conn.execute(s)
for row in result:
print(row)
# (1,)
# (2,)
# (3,)
# (4,)
# (5,)
And instantiation location:
print(my_table._where_am_i) # 53302898.py (that's the name of my module).
External module:
# external_module.py
from sqlalchemy_app import Base
from sqlalchemy.sql.schema import Table
from sqlalchemy import Column, BigInteger
metadata = Base.metadata
class MyTable(Table):
def __init__(self, *args, **kwargs):
self._where_am_i = __file__
my_table = MyTable(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True)
)
And:
# 53302898.py
from external_module import my_table
if __name__ == '__main__':
print(my_table._where_am_i) # prints C:Userspeter_000OneDrivegittestexternal_module.py
Note how it returned the relative file path in the first test and the absolute file path in the external module test. You can read about that here: Python __file__ attribute absolute or relative? but you can make that _where_am_i
attribute return whatever you need to suit your application.
EDIT
The above solution requires subclassing the Table
class inside the module where instances are formed, otherwise it will peg the module where the Class is instantiated, not the instances. If you only want to subclass Table
once in your project you'd need to pass the location to the constructor.
This works:
class MyTable(Table):
def __init__(self, *args, _where_am_i=None, **kwargs):
self._where_am_i = _where_am_i
...but you get a warning upon instantiation:
SAWarning: Can't validate argument '_where_am_i'; can't locate any SQLAlchemy dialect named '_where'
.
To avoid that, you'd have to override sqlalchemy's alternate constructor, Table._init()
, strip out the location parameter and then delegate back up the chain:
class MyTable(Table):
def _init(self, *args, _where_am_i=None, **kwargs):
self._where_am_i = _where_am_i
super()._init(*args, **kwargs)
Import from external module:
# 53302898.py
from external_module import MyTable
my_table = MyTable(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True),
_where_am_i = __file__
)
if __name__ == '__main__':
print(my_table._where_am_i) # 53302898.py
All above tests still pass.
Unfortunately, this solution doesn't scale well. If I putMyTable
into a separate file so that I can share it between several tables, it gets the location of that file rather than the file where the table was instantiated.
– Rainbacon
Nov 15 '18 at 14:32
Then your only option is to pass it in at instantiation time. See edit.
– SuperShoot
Nov 15 '18 at 19:38
add a comment |
You could add the functionality by subclassing Table
. In SQLAlchemy, Table
specifically overrides Table.__init__()
to make it a no-op:
def __init__(self, *args, **kw):
"""Constructor for :class:`~.schema.Table`.
This method is a no-op. See the top-level
documentation for :class:`~.schema.Table`
for constructor arguments.
"""
# __init__ is overridden to prevent __new__ from
# calling the superclass constructor.
The key being that it does not invoke super().__init__()
, so that sqlalchemy can take command of instantiation and whatever you do, that needs to be maintained.
from sqlalchemy.sql.schema import Table
class MyTable(Table):
def __init__(self, *args, **kwargs):
self._where_am_i = __file__
my_table = MyTable(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True)
)
In this case, MyTable.__init__()
is still blocking the superclass constructor, but it also adds an attribute to the instance which will be the name of the module that the class is instantiated within. I specifically chose an obscure attribute name (_where_am_i
) that is unlikely to be overwritten by sqlalchemy and using __file__
returns the path of the module (but you can make that anything you want).
I tested that inserts and selects still work:
import logging
from sqlalchemy.sql import select
logging.getLogger('sqlalchemy.engine').setLevel(logging.INFO)
logging.basicConfig(level=logging.INFO)
Base.metadata.drop_all(engine)
Base.metadata.create_all(engine)
conn = engine.connect()
conn.execute(my_table.insert(), ["my_id": i for i in range(1, 6)])
s = select([my_table])
result = conn.execute(s)
for row in result:
print(row)
# (1,)
# (2,)
# (3,)
# (4,)
# (5,)
And instantiation location:
print(my_table._where_am_i) # 53302898.py (that's the name of my module).
External module:
# external_module.py
from sqlalchemy_app import Base
from sqlalchemy.sql.schema import Table
from sqlalchemy import Column, BigInteger
metadata = Base.metadata
class MyTable(Table):
def __init__(self, *args, **kwargs):
self._where_am_i = __file__
my_table = MyTable(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True)
)
And:
# 53302898.py
from external_module import my_table
if __name__ == '__main__':
print(my_table._where_am_i) # prints C:Userspeter_000OneDrivegittestexternal_module.py
Note how it returned the relative file path in the first test and the absolute file path in the external module test. You can read about that here: Python __file__ attribute absolute or relative? but you can make that _where_am_i
attribute return whatever you need to suit your application.
EDIT
The above solution requires subclassing the Table
class inside the module where instances are formed, otherwise it will peg the module where the Class is instantiated, not the instances. If you only want to subclass Table
once in your project you'd need to pass the location to the constructor.
This works:
class MyTable(Table):
def __init__(self, *args, _where_am_i=None, **kwargs):
self._where_am_i = _where_am_i
...but you get a warning upon instantiation:
SAWarning: Can't validate argument '_where_am_i'; can't locate any SQLAlchemy dialect named '_where'
.
To avoid that, you'd have to override sqlalchemy's alternate constructor, Table._init()
, strip out the location parameter and then delegate back up the chain:
class MyTable(Table):
def _init(self, *args, _where_am_i=None, **kwargs):
self._where_am_i = _where_am_i
super()._init(*args, **kwargs)
Import from external module:
# 53302898.py
from external_module import MyTable
my_table = MyTable(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True),
_where_am_i = __file__
)
if __name__ == '__main__':
print(my_table._where_am_i) # 53302898.py
All above tests still pass.
Unfortunately, this solution doesn't scale well. If I putMyTable
into a separate file so that I can share it between several tables, it gets the location of that file rather than the file where the table was instantiated.
– Rainbacon
Nov 15 '18 at 14:32
Then your only option is to pass it in at instantiation time. See edit.
– SuperShoot
Nov 15 '18 at 19:38
add a comment |
You could add the functionality by subclassing Table
. In SQLAlchemy, Table
specifically overrides Table.__init__()
to make it a no-op:
def __init__(self, *args, **kw):
"""Constructor for :class:`~.schema.Table`.
This method is a no-op. See the top-level
documentation for :class:`~.schema.Table`
for constructor arguments.
"""
# __init__ is overridden to prevent __new__ from
# calling the superclass constructor.
The key being that it does not invoke super().__init__()
, so that sqlalchemy can take command of instantiation and whatever you do, that needs to be maintained.
from sqlalchemy.sql.schema import Table
class MyTable(Table):
def __init__(self, *args, **kwargs):
self._where_am_i = __file__
my_table = MyTable(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True)
)
In this case, MyTable.__init__()
is still blocking the superclass constructor, but it also adds an attribute to the instance which will be the name of the module that the class is instantiated within. I specifically chose an obscure attribute name (_where_am_i
) that is unlikely to be overwritten by sqlalchemy and using __file__
returns the path of the module (but you can make that anything you want).
I tested that inserts and selects still work:
import logging
from sqlalchemy.sql import select
logging.getLogger('sqlalchemy.engine').setLevel(logging.INFO)
logging.basicConfig(level=logging.INFO)
Base.metadata.drop_all(engine)
Base.metadata.create_all(engine)
conn = engine.connect()
conn.execute(my_table.insert(), ["my_id": i for i in range(1, 6)])
s = select([my_table])
result = conn.execute(s)
for row in result:
print(row)
# (1,)
# (2,)
# (3,)
# (4,)
# (5,)
And instantiation location:
print(my_table._where_am_i) # 53302898.py (that's the name of my module).
External module:
# external_module.py
from sqlalchemy_app import Base
from sqlalchemy.sql.schema import Table
from sqlalchemy import Column, BigInteger
metadata = Base.metadata
class MyTable(Table):
def __init__(self, *args, **kwargs):
self._where_am_i = __file__
my_table = MyTable(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True)
)
And:
# 53302898.py
from external_module import my_table
if __name__ == '__main__':
print(my_table._where_am_i) # prints C:Userspeter_000OneDrivegittestexternal_module.py
Note how it returned the relative file path in the first test and the absolute file path in the external module test. You can read about that here: Python __file__ attribute absolute or relative? but you can make that _where_am_i
attribute return whatever you need to suit your application.
EDIT
The above solution requires subclassing the Table
class inside the module where instances are formed, otherwise it will peg the module where the Class is instantiated, not the instances. If you only want to subclass Table
once in your project you'd need to pass the location to the constructor.
This works:
class MyTable(Table):
def __init__(self, *args, _where_am_i=None, **kwargs):
self._where_am_i = _where_am_i
...but you get a warning upon instantiation:
SAWarning: Can't validate argument '_where_am_i'; can't locate any SQLAlchemy dialect named '_where'
.
To avoid that, you'd have to override sqlalchemy's alternate constructor, Table._init()
, strip out the location parameter and then delegate back up the chain:
class MyTable(Table):
def _init(self, *args, _where_am_i=None, **kwargs):
self._where_am_i = _where_am_i
super()._init(*args, **kwargs)
Import from external module:
# 53302898.py
from external_module import MyTable
my_table = MyTable(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True),
_where_am_i = __file__
)
if __name__ == '__main__':
print(my_table._where_am_i) # 53302898.py
All above tests still pass.
You could add the functionality by subclassing Table
. In SQLAlchemy, Table
specifically overrides Table.__init__()
to make it a no-op:
def __init__(self, *args, **kw):
"""Constructor for :class:`~.schema.Table`.
This method is a no-op. See the top-level
documentation for :class:`~.schema.Table`
for constructor arguments.
"""
# __init__ is overridden to prevent __new__ from
# calling the superclass constructor.
The key being that it does not invoke super().__init__()
, so that sqlalchemy can take command of instantiation and whatever you do, that needs to be maintained.
from sqlalchemy.sql.schema import Table
class MyTable(Table):
def __init__(self, *args, **kwargs):
self._where_am_i = __file__
my_table = MyTable(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True)
)
In this case, MyTable.__init__()
is still blocking the superclass constructor, but it also adds an attribute to the instance which will be the name of the module that the class is instantiated within. I specifically chose an obscure attribute name (_where_am_i
) that is unlikely to be overwritten by sqlalchemy and using __file__
returns the path of the module (but you can make that anything you want).
I tested that inserts and selects still work:
import logging
from sqlalchemy.sql import select
logging.getLogger('sqlalchemy.engine').setLevel(logging.INFO)
logging.basicConfig(level=logging.INFO)
Base.metadata.drop_all(engine)
Base.metadata.create_all(engine)
conn = engine.connect()
conn.execute(my_table.insert(), ["my_id": i for i in range(1, 6)])
s = select([my_table])
result = conn.execute(s)
for row in result:
print(row)
# (1,)
# (2,)
# (3,)
# (4,)
# (5,)
And instantiation location:
print(my_table._where_am_i) # 53302898.py (that's the name of my module).
External module:
# external_module.py
from sqlalchemy_app import Base
from sqlalchemy.sql.schema import Table
from sqlalchemy import Column, BigInteger
metadata = Base.metadata
class MyTable(Table):
def __init__(self, *args, **kwargs):
self._where_am_i = __file__
my_table = MyTable(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True)
)
And:
# 53302898.py
from external_module import my_table
if __name__ == '__main__':
print(my_table._where_am_i) # prints C:Userspeter_000OneDrivegittestexternal_module.py
Note how it returned the relative file path in the first test and the absolute file path in the external module test. You can read about that here: Python __file__ attribute absolute or relative? but you can make that _where_am_i
attribute return whatever you need to suit your application.
EDIT
The above solution requires subclassing the Table
class inside the module where instances are formed, otherwise it will peg the module where the Class is instantiated, not the instances. If you only want to subclass Table
once in your project you'd need to pass the location to the constructor.
This works:
class MyTable(Table):
def __init__(self, *args, _where_am_i=None, **kwargs):
self._where_am_i = _where_am_i
...but you get a warning upon instantiation:
SAWarning: Can't validate argument '_where_am_i'; can't locate any SQLAlchemy dialect named '_where'
.
To avoid that, you'd have to override sqlalchemy's alternate constructor, Table._init()
, strip out the location parameter and then delegate back up the chain:
class MyTable(Table):
def _init(self, *args, _where_am_i=None, **kwargs):
self._where_am_i = _where_am_i
super()._init(*args, **kwargs)
Import from external module:
# 53302898.py
from external_module import MyTable
my_table = MyTable(
"my_table",
metadata,
Column("my_id", BigInteger(), primary_key=True),
_where_am_i = __file__
)
if __name__ == '__main__':
print(my_table._where_am_i) # 53302898.py
All above tests still pass.
edited Nov 15 '18 at 19:52
answered Nov 15 '18 at 1:12
SuperShootSuperShoot
1,855721
1,855721
Unfortunately, this solution doesn't scale well. If I putMyTable
into a separate file so that I can share it between several tables, it gets the location of that file rather than the file where the table was instantiated.
– Rainbacon
Nov 15 '18 at 14:32
Then your only option is to pass it in at instantiation time. See edit.
– SuperShoot
Nov 15 '18 at 19:38
add a comment |
Unfortunately, this solution doesn't scale well. If I putMyTable
into a separate file so that I can share it between several tables, it gets the location of that file rather than the file where the table was instantiated.
– Rainbacon
Nov 15 '18 at 14:32
Then your only option is to pass it in at instantiation time. See edit.
– SuperShoot
Nov 15 '18 at 19:38
Unfortunately, this solution doesn't scale well. If I put
MyTable
into a separate file so that I can share it between several tables, it gets the location of that file rather than the file where the table was instantiated.– Rainbacon
Nov 15 '18 at 14:32
Unfortunately, this solution doesn't scale well. If I put
MyTable
into a separate file so that I can share it between several tables, it gets the location of that file rather than the file where the table was instantiated.– Rainbacon
Nov 15 '18 at 14:32
Then your only option is to pass it in at instantiation time. See edit.
– SuperShoot
Nov 15 '18 at 19:38
Then your only option is to pass it in at instantiation time. See edit.
– SuperShoot
Nov 15 '18 at 19:38
add a comment |
You can't. If you refer to the Python documentation index, you see that there are three entries for __module__
: one for a class attribute, one for a function attribute, and one for a method attribute. Only those types of objects have the module in which they were declared recorded. my_table
is none of these; it's just an instance of the Table
class, so the only __module__
you can find on it is Table.__module__
.
add a comment |
You can't. If you refer to the Python documentation index, you see that there are three entries for __module__
: one for a class attribute, one for a function attribute, and one for a method attribute. Only those types of objects have the module in which they were declared recorded. my_table
is none of these; it's just an instance of the Table
class, so the only __module__
you can find on it is Table.__module__
.
add a comment |
You can't. If you refer to the Python documentation index, you see that there are three entries for __module__
: one for a class attribute, one for a function attribute, and one for a method attribute. Only those types of objects have the module in which they were declared recorded. my_table
is none of these; it's just an instance of the Table
class, so the only __module__
you can find on it is Table.__module__
.
You can't. If you refer to the Python documentation index, you see that there are three entries for __module__
: one for a class attribute, one for a function attribute, and one for a method attribute. Only those types of objects have the module in which they were declared recorded. my_table
is none of these; it's just an instance of the Table
class, so the only __module__
you can find on it is Table.__module__
.
answered Nov 14 '18 at 20:21
jwodderjwodder
33.8k45684
33.8k45684
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53302898%2fhow-can-i-get-the-name-of-the-module-where-a-table-instance-was-declared%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6GBJBJREcIlJ5uIV,WH gqnCup2GJJ2H,M1nbI3OasIuBkZ7 kZ3ZcySlocHOcGnBNxH9FkYB5eEz cqIxkN1Al gbWz7cbM j