How to input negative numbers into a list
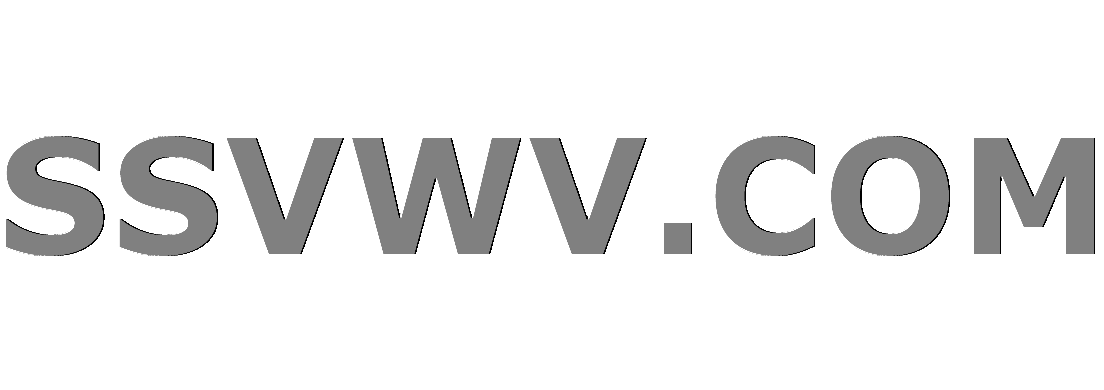
Multi tool use
up vote
0
down vote
favorite
I require some help since whenever I input a negative number the list it interprets it as a separate element so once it gets to sorting it puts all the negative symbols at the beginning. The end goal of the code is to sort 2 merged lists without using the default sort functions. Also if there is a better way to get rid of spaces in a list I would appreciate it, since at the moment I have to convert the list to a string and replace/strip the extra elements that the spaces cause.
list1 = list(input())
list2 = list(input())
mergelist = list1 + list2
print(mergelist)
def bubble_sort(X):
nums = list(X)
for i in range(len(X)):
for j in range(i+1, len(X)):
if X[j] < X[i]:
X[j], X[i] = X[i], X[j]
return X
mergelist = bubble_sort(mergelist)
strmergelist = str(mergelist)
strmergelist = strmergelist.replace("'", '')
strmergelist = strmergelist.replace(",", '')
strmergelist = strmergelist.strip('')
strmergelist = strmergelist.strip()
print(strmergelist)
The output for lists with no negatives is:
1 2 3 4 4 5 5
However with negatives it becomes:
- - - - 1 2 3 3 4 4 5
and my first print function to just check the merging of the lists looks like this when I input any negatives (ignore the spaces since I attempt to remove them later):
['1', ' ', '-', '2', ' ', '3', '3', ' ', '-', '4', ' ', '-', '4', ' ', '-', '5']
python whitespace negative-number
add a comment |
up vote
0
down vote
favorite
I require some help since whenever I input a negative number the list it interprets it as a separate element so once it gets to sorting it puts all the negative symbols at the beginning. The end goal of the code is to sort 2 merged lists without using the default sort functions. Also if there is a better way to get rid of spaces in a list I would appreciate it, since at the moment I have to convert the list to a string and replace/strip the extra elements that the spaces cause.
list1 = list(input())
list2 = list(input())
mergelist = list1 + list2
print(mergelist)
def bubble_sort(X):
nums = list(X)
for i in range(len(X)):
for j in range(i+1, len(X)):
if X[j] < X[i]:
X[j], X[i] = X[i], X[j]
return X
mergelist = bubble_sort(mergelist)
strmergelist = str(mergelist)
strmergelist = strmergelist.replace("'", '')
strmergelist = strmergelist.replace(",", '')
strmergelist = strmergelist.strip('')
strmergelist = strmergelist.strip()
print(strmergelist)
The output for lists with no negatives is:
1 2 3 4 4 5 5
However with negatives it becomes:
- - - - 1 2 3 3 4 4 5
and my first print function to just check the merging of the lists looks like this when I input any negatives (ignore the spaces since I attempt to remove them later):
['1', ' ', '-', '2', ' ', '3', '3', ' ', '-', '4', ' ', '-', '4', ' ', '-', '5']
python whitespace negative-number
2
What format are you supposed to be enteringlist1
andlist2
in... for instance... If you're entering-1 2 -3
... you probably want to be splitting on spaces and then converting each word into an integer before sorting... That way you're not having to strip things/remove brackets etc... You want to be working with lists of numbers - not individual characters...
– Jon Clements♦
Nov 10 at 19:59
1
so I'm guessinglist1 = [int(n) for n in input().split()]
and the same forlist2
is probably going to sort you out here...
– Jon Clements♦
Nov 10 at 20:00
Those are string. Wouldn't a negative number list be [-1,-2] ?
– QHarr
Nov 10 at 20:01
He's getting the numbers from an Input, but I cannot see that he translates the input into Integers, therefore even if the splitting of the variables were correct,"-1" < "-2"
would theTrue
, because it's looking at the ASCII-value of the characters.
– Hampus Larsson
Nov 10 at 20:04
Why are you callingstr
on a list? Just do" ".join(mergelist)
.
– Aviv Shai
Nov 10 at 20:05
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I require some help since whenever I input a negative number the list it interprets it as a separate element so once it gets to sorting it puts all the negative symbols at the beginning. The end goal of the code is to sort 2 merged lists without using the default sort functions. Also if there is a better way to get rid of spaces in a list I would appreciate it, since at the moment I have to convert the list to a string and replace/strip the extra elements that the spaces cause.
list1 = list(input())
list2 = list(input())
mergelist = list1 + list2
print(mergelist)
def bubble_sort(X):
nums = list(X)
for i in range(len(X)):
for j in range(i+1, len(X)):
if X[j] < X[i]:
X[j], X[i] = X[i], X[j]
return X
mergelist = bubble_sort(mergelist)
strmergelist = str(mergelist)
strmergelist = strmergelist.replace("'", '')
strmergelist = strmergelist.replace(",", '')
strmergelist = strmergelist.strip('')
strmergelist = strmergelist.strip()
print(strmergelist)
The output for lists with no negatives is:
1 2 3 4 4 5 5
However with negatives it becomes:
- - - - 1 2 3 3 4 4 5
and my first print function to just check the merging of the lists looks like this when I input any negatives (ignore the spaces since I attempt to remove them later):
['1', ' ', '-', '2', ' ', '3', '3', ' ', '-', '4', ' ', '-', '4', ' ', '-', '5']
python whitespace negative-number
I require some help since whenever I input a negative number the list it interprets it as a separate element so once it gets to sorting it puts all the negative symbols at the beginning. The end goal of the code is to sort 2 merged lists without using the default sort functions. Also if there is a better way to get rid of spaces in a list I would appreciate it, since at the moment I have to convert the list to a string and replace/strip the extra elements that the spaces cause.
list1 = list(input())
list2 = list(input())
mergelist = list1 + list2
print(mergelist)
def bubble_sort(X):
nums = list(X)
for i in range(len(X)):
for j in range(i+1, len(X)):
if X[j] < X[i]:
X[j], X[i] = X[i], X[j]
return X
mergelist = bubble_sort(mergelist)
strmergelist = str(mergelist)
strmergelist = strmergelist.replace("'", '')
strmergelist = strmergelist.replace(",", '')
strmergelist = strmergelist.strip('')
strmergelist = strmergelist.strip()
print(strmergelist)
The output for lists with no negatives is:
1 2 3 4 4 5 5
However with negatives it becomes:
- - - - 1 2 3 3 4 4 5
and my first print function to just check the merging of the lists looks like this when I input any negatives (ignore the spaces since I attempt to remove them later):
['1', ' ', '-', '2', ' ', '3', '3', ' ', '-', '4', ' ', '-', '4', ' ', '-', '5']
python whitespace negative-number
python whitespace negative-number
asked Nov 10 at 19:53


Rostovne
31
31
2
What format are you supposed to be enteringlist1
andlist2
in... for instance... If you're entering-1 2 -3
... you probably want to be splitting on spaces and then converting each word into an integer before sorting... That way you're not having to strip things/remove brackets etc... You want to be working with lists of numbers - not individual characters...
– Jon Clements♦
Nov 10 at 19:59
1
so I'm guessinglist1 = [int(n) for n in input().split()]
and the same forlist2
is probably going to sort you out here...
– Jon Clements♦
Nov 10 at 20:00
Those are string. Wouldn't a negative number list be [-1,-2] ?
– QHarr
Nov 10 at 20:01
He's getting the numbers from an Input, but I cannot see that he translates the input into Integers, therefore even if the splitting of the variables were correct,"-1" < "-2"
would theTrue
, because it's looking at the ASCII-value of the characters.
– Hampus Larsson
Nov 10 at 20:04
Why are you callingstr
on a list? Just do" ".join(mergelist)
.
– Aviv Shai
Nov 10 at 20:05
add a comment |
2
What format are you supposed to be enteringlist1
andlist2
in... for instance... If you're entering-1 2 -3
... you probably want to be splitting on spaces and then converting each word into an integer before sorting... That way you're not having to strip things/remove brackets etc... You want to be working with lists of numbers - not individual characters...
– Jon Clements♦
Nov 10 at 19:59
1
so I'm guessinglist1 = [int(n) for n in input().split()]
and the same forlist2
is probably going to sort you out here...
– Jon Clements♦
Nov 10 at 20:00
Those are string. Wouldn't a negative number list be [-1,-2] ?
– QHarr
Nov 10 at 20:01
He's getting the numbers from an Input, but I cannot see that he translates the input into Integers, therefore even if the splitting of the variables were correct,"-1" < "-2"
would theTrue
, because it's looking at the ASCII-value of the characters.
– Hampus Larsson
Nov 10 at 20:04
Why are you callingstr
on a list? Just do" ".join(mergelist)
.
– Aviv Shai
Nov 10 at 20:05
2
2
What format are you supposed to be entering
list1
and list2
in... for instance... If you're entering -1 2 -3
... you probably want to be splitting on spaces and then converting each word into an integer before sorting... That way you're not having to strip things/remove brackets etc... You want to be working with lists of numbers - not individual characters...– Jon Clements♦
Nov 10 at 19:59
What format are you supposed to be entering
list1
and list2
in... for instance... If you're entering -1 2 -3
... you probably want to be splitting on spaces and then converting each word into an integer before sorting... That way you're not having to strip things/remove brackets etc... You want to be working with lists of numbers - not individual characters...– Jon Clements♦
Nov 10 at 19:59
1
1
so I'm guessing
list1 = [int(n) for n in input().split()]
and the same for list2
is probably going to sort you out here...– Jon Clements♦
Nov 10 at 20:00
so I'm guessing
list1 = [int(n) for n in input().split()]
and the same for list2
is probably going to sort you out here...– Jon Clements♦
Nov 10 at 20:00
Those are string. Wouldn't a negative number list be [-1,-2] ?
– QHarr
Nov 10 at 20:01
Those are string. Wouldn't a negative number list be [-1,-2] ?
– QHarr
Nov 10 at 20:01
He's getting the numbers from an Input, but I cannot see that he translates the input into Integers, therefore even if the splitting of the variables were correct,
"-1" < "-2"
would the True
, because it's looking at the ASCII-value of the characters.– Hampus Larsson
Nov 10 at 20:04
He's getting the numbers from an Input, but I cannot see that he translates the input into Integers, therefore even if the splitting of the variables were correct,
"-1" < "-2"
would the True
, because it's looking at the ASCII-value of the characters.– Hampus Larsson
Nov 10 at 20:04
Why are you calling
str
on a list? Just do " ".join(mergelist)
.– Aviv Shai
Nov 10 at 20:05
Why are you calling
str
on a list? Just do " ".join(mergelist)
.– Aviv Shai
Nov 10 at 20:05
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
list()
doesn't parse a string to a list of integers, it turns an iterable of items into a list of items.
To read a list from the console, try something like:
def read_list():
"""
read a list of integers from stdin
"""
return list(map(int, input().split()))
list1 = read_list()
list2 = read_list()
input.split()
reads one line of user input and will separate it by whitespace - basically to words.
int()
can convert a string to an integer.
map(int, ...)
returns an iterable which applies int()
to each "word" of the user input.
The final call to list()
will turn the iterable to a list.
This should handle negative numbers as well.
Additionally, I see that you want to print the resulting list without extra character. I recommend this:
print(' '.join(mergelist))
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53242835%2fhow-to-input-negative-numbers-into-a-list%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
list()
doesn't parse a string to a list of integers, it turns an iterable of items into a list of items.
To read a list from the console, try something like:
def read_list():
"""
read a list of integers from stdin
"""
return list(map(int, input().split()))
list1 = read_list()
list2 = read_list()
input.split()
reads one line of user input and will separate it by whitespace - basically to words.
int()
can convert a string to an integer.
map(int, ...)
returns an iterable which applies int()
to each "word" of the user input.
The final call to list()
will turn the iterable to a list.
This should handle negative numbers as well.
Additionally, I see that you want to print the resulting list without extra character. I recommend this:
print(' '.join(mergelist))
add a comment |
up vote
0
down vote
accepted
list()
doesn't parse a string to a list of integers, it turns an iterable of items into a list of items.
To read a list from the console, try something like:
def read_list():
"""
read a list of integers from stdin
"""
return list(map(int, input().split()))
list1 = read_list()
list2 = read_list()
input.split()
reads one line of user input and will separate it by whitespace - basically to words.
int()
can convert a string to an integer.
map(int, ...)
returns an iterable which applies int()
to each "word" of the user input.
The final call to list()
will turn the iterable to a list.
This should handle negative numbers as well.
Additionally, I see that you want to print the resulting list without extra character. I recommend this:
print(' '.join(mergelist))
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
list()
doesn't parse a string to a list of integers, it turns an iterable of items into a list of items.
To read a list from the console, try something like:
def read_list():
"""
read a list of integers from stdin
"""
return list(map(int, input().split()))
list1 = read_list()
list2 = read_list()
input.split()
reads one line of user input and will separate it by whitespace - basically to words.
int()
can convert a string to an integer.
map(int, ...)
returns an iterable which applies int()
to each "word" of the user input.
The final call to list()
will turn the iterable to a list.
This should handle negative numbers as well.
Additionally, I see that you want to print the resulting list without extra character. I recommend this:
print(' '.join(mergelist))
list()
doesn't parse a string to a list of integers, it turns an iterable of items into a list of items.
To read a list from the console, try something like:
def read_list():
"""
read a list of integers from stdin
"""
return list(map(int, input().split()))
list1 = read_list()
list2 = read_list()
input.split()
reads one line of user input and will separate it by whitespace - basically to words.
int()
can convert a string to an integer.
map(int, ...)
returns an iterable which applies int()
to each "word" of the user input.
The final call to list()
will turn the iterable to a list.
This should handle negative numbers as well.
Additionally, I see that you want to print the resulting list without extra character. I recommend this:
print(' '.join(mergelist))
answered Nov 10 at 20:09
roeen30
43629
43629
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53242835%2fhow-to-input-negative-numbers-into-a-list%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3E XUQ0ukGW 9Xl6KleC1ZOBxMr9z 7ETbl5lMax023 Jv95jLYTtgoxeNVIGx3,sk4h,ChRX,vBK2,Tw jV8
2
What format are you supposed to be entering
list1
andlist2
in... for instance... If you're entering-1 2 -3
... you probably want to be splitting on spaces and then converting each word into an integer before sorting... That way you're not having to strip things/remove brackets etc... You want to be working with lists of numbers - not individual characters...– Jon Clements♦
Nov 10 at 19:59
1
so I'm guessing
list1 = [int(n) for n in input().split()]
and the same forlist2
is probably going to sort you out here...– Jon Clements♦
Nov 10 at 20:00
Those are string. Wouldn't a negative number list be [-1,-2] ?
– QHarr
Nov 10 at 20:01
He's getting the numbers from an Input, but I cannot see that he translates the input into Integers, therefore even if the splitting of the variables were correct,
"-1" < "-2"
would theTrue
, because it's looking at the ASCII-value of the characters.– Hampus Larsson
Nov 10 at 20:04
Why are you calling
str
on a list? Just do" ".join(mergelist)
.– Aviv Shai
Nov 10 at 20:05