MVC Controller Shopping Cart adding to a list
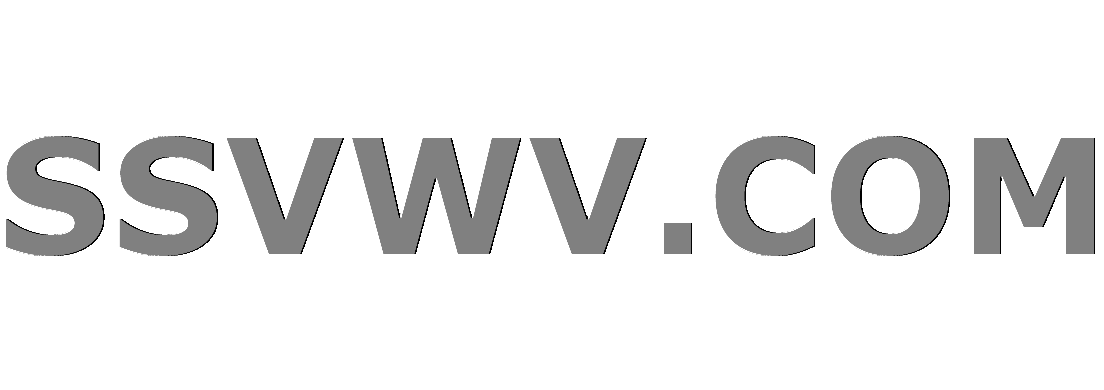
Multi tool use
up vote
1
down vote
favorite
I'm trying to make a shopping cart and I'm having trouble with adding an item to the shopping cart.
I'm trying to add items to the carts, but it seems that it is refreshing itself everytime I call the ShoppingCart part of the controller.
HomeController.cs
using System;
using System.Collections.Generic;
using System.Data;
using System.Data.Entity;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using ANON_Capstone.Models;
namespace ANON_Capstone.Controllers
public class HomeController : Controller
private UsersContext db = new UsersContext();
Carts carts = new Carts();
public ActionResult Index()
return View();
public ActionResult About()
return View();
public ActionResult Contact()
return View();
public ActionResult Courses()
return View(db.Courses.ToList());
public ActionResult ShoppingCart(string className, string classPrice, string classID, string classDesc)
if (carts.CartModels == null)
carts.CartModels = new List<CartModel>();
CartModel cart = new CartModel();
cart.className = className;
cart.classPrice = Convert.ToDouble(classPrice);
cart.classID = Convert.ToInt32(classID);
cart.classDesc = classDesc;
//if (className != null)
//
// carts.CartModels.Add(new CartModel() className = className, classPrice = Convert.ToDouble(classPrice), classID = Convert.ToInt32(classID), classDesc = classDesc );
//
if (className != null)
carts.CartModels.Add(new CartModel() className = className, classPrice = Convert.ToDouble(classPrice), classID = Convert.ToInt32(classID), classDesc = classDesc );
//Session["Cart"] = cart;
//ViewBag.Name = Session["Cart"] as ANON_Capstone.Models.Carts;
//ViewData["Cart"] = cart;
Console.WriteLine();
return View(carts);
Courses.cshtml
@model IEnumerable<ANON_Capstone.Models.Course>
@if (!User.Identity.IsAuthenticated)
@section featured
<section class="featured">
<div class="content-wrapper">
<h2 class="text-center">Please Login or Create an Account to make a Purchase!</h2>
</div>
</section>
<div class="row">
<div class="col-lg-9">
<h2><strong>Courses</strong></h2><br />
@foreach (var item in Model)
<div class="col-md-4 col-xs-6 col-s-8 col-lg-4">
@using (Html.BeginForm("ShoppingCart", "Home", FormMethod.Post))
<img src="~/Images/party.gif" style="width: 175px" class="img-responsive" />
<h2>@item.className</h2>
<p>$@item.classPrice -- @item.maxStudent spots left! </p>
<input type="text" name="className" value="@item.className" hidden="hidden" />
<input type="text" name="classPrice" value="@item.classPrice" hidden="hidden" />
<input type="text" name="classID" value="@item.ClassID" hidden="hidden" />
<input type="hidden" name="classDesc" value="@item.classDesc" />
if (User.Identity.IsAuthenticated)
<input class="btn btn-default" type="submit" name="btnConfirm" value="Add to Shopping Cart" />
<br />
</div>
</div>
</div>
ShoppingCart.cshtml
@model ANON_Capstone.Models.Carts
@
ViewBag.Title = "Shopping Cart";
<h2>Shopping Cart</h2>
@using (Html.BeginForm("ValidateCommand", "PayPal"))
<div class="row">
<div class="col-lg-9">
@if (Model.CartModels.Count != 0)
<table border="1">
<tr>
<th>Course Image</th>
<th>Course Name</th>
<th>Course Desc</th>
<th>Course Price</th>
</tr>
@foreach (var item in Model.CartModels)
<tr>
<td><img src="~/Images/party.gif" style="width: 175px" class="img-responsive" /></td>
<td>@item.className</td>
<td>@item.classDesc</td>
<td>@item.classPrice</td>
</tr>
</table>
<input class="btn btn-default" type="submit" name="btnConfirm" value="Check Out with Paypal" />
else
<text>Your shopping cart is currently empty</text>
</div>
</div>
Carts.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data.Entity;
namespace ANON_Capstone.Models
public class Carts
public List<CartModel> CartModels get; set;
public double CartTotal get; set;
CartModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data.Entity;
namespace ANON_Capstone.Models
public class CartModel
public string className get; set;
public double classPrice get; set;
public int classID get; set;
public string classDesc get; set;
c# list asp.net-mvc-4 shopping-cart
add a comment |
up vote
1
down vote
favorite
I'm trying to make a shopping cart and I'm having trouble with adding an item to the shopping cart.
I'm trying to add items to the carts, but it seems that it is refreshing itself everytime I call the ShoppingCart part of the controller.
HomeController.cs
using System;
using System.Collections.Generic;
using System.Data;
using System.Data.Entity;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using ANON_Capstone.Models;
namespace ANON_Capstone.Controllers
public class HomeController : Controller
private UsersContext db = new UsersContext();
Carts carts = new Carts();
public ActionResult Index()
return View();
public ActionResult About()
return View();
public ActionResult Contact()
return View();
public ActionResult Courses()
return View(db.Courses.ToList());
public ActionResult ShoppingCart(string className, string classPrice, string classID, string classDesc)
if (carts.CartModels == null)
carts.CartModels = new List<CartModel>();
CartModel cart = new CartModel();
cart.className = className;
cart.classPrice = Convert.ToDouble(classPrice);
cart.classID = Convert.ToInt32(classID);
cart.classDesc = classDesc;
//if (className != null)
//
// carts.CartModels.Add(new CartModel() className = className, classPrice = Convert.ToDouble(classPrice), classID = Convert.ToInt32(classID), classDesc = classDesc );
//
if (className != null)
carts.CartModels.Add(new CartModel() className = className, classPrice = Convert.ToDouble(classPrice), classID = Convert.ToInt32(classID), classDesc = classDesc );
//Session["Cart"] = cart;
//ViewBag.Name = Session["Cart"] as ANON_Capstone.Models.Carts;
//ViewData["Cart"] = cart;
Console.WriteLine();
return View(carts);
Courses.cshtml
@model IEnumerable<ANON_Capstone.Models.Course>
@if (!User.Identity.IsAuthenticated)
@section featured
<section class="featured">
<div class="content-wrapper">
<h2 class="text-center">Please Login or Create an Account to make a Purchase!</h2>
</div>
</section>
<div class="row">
<div class="col-lg-9">
<h2><strong>Courses</strong></h2><br />
@foreach (var item in Model)
<div class="col-md-4 col-xs-6 col-s-8 col-lg-4">
@using (Html.BeginForm("ShoppingCart", "Home", FormMethod.Post))
<img src="~/Images/party.gif" style="width: 175px" class="img-responsive" />
<h2>@item.className</h2>
<p>$@item.classPrice -- @item.maxStudent spots left! </p>
<input type="text" name="className" value="@item.className" hidden="hidden" />
<input type="text" name="classPrice" value="@item.classPrice" hidden="hidden" />
<input type="text" name="classID" value="@item.ClassID" hidden="hidden" />
<input type="hidden" name="classDesc" value="@item.classDesc" />
if (User.Identity.IsAuthenticated)
<input class="btn btn-default" type="submit" name="btnConfirm" value="Add to Shopping Cart" />
<br />
</div>
</div>
</div>
ShoppingCart.cshtml
@model ANON_Capstone.Models.Carts
@
ViewBag.Title = "Shopping Cart";
<h2>Shopping Cart</h2>
@using (Html.BeginForm("ValidateCommand", "PayPal"))
<div class="row">
<div class="col-lg-9">
@if (Model.CartModels.Count != 0)
<table border="1">
<tr>
<th>Course Image</th>
<th>Course Name</th>
<th>Course Desc</th>
<th>Course Price</th>
</tr>
@foreach (var item in Model.CartModels)
<tr>
<td><img src="~/Images/party.gif" style="width: 175px" class="img-responsive" /></td>
<td>@item.className</td>
<td>@item.classDesc</td>
<td>@item.classPrice</td>
</tr>
</table>
<input class="btn btn-default" type="submit" name="btnConfirm" value="Check Out with Paypal" />
else
<text>Your shopping cart is currently empty</text>
</div>
</div>
Carts.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data.Entity;
namespace ANON_Capstone.Models
public class Carts
public List<CartModel> CartModels get; set;
public double CartTotal get; set;
CartModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data.Entity;
namespace ANON_Capstone.Models
public class CartModel
public string className get; set;
public double classPrice get; set;
public int classID get; set;
public string classDesc get; set;
c# list asp.net-mvc-4 shopping-cart
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm trying to make a shopping cart and I'm having trouble with adding an item to the shopping cart.
I'm trying to add items to the carts, but it seems that it is refreshing itself everytime I call the ShoppingCart part of the controller.
HomeController.cs
using System;
using System.Collections.Generic;
using System.Data;
using System.Data.Entity;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using ANON_Capstone.Models;
namespace ANON_Capstone.Controllers
public class HomeController : Controller
private UsersContext db = new UsersContext();
Carts carts = new Carts();
public ActionResult Index()
return View();
public ActionResult About()
return View();
public ActionResult Contact()
return View();
public ActionResult Courses()
return View(db.Courses.ToList());
public ActionResult ShoppingCart(string className, string classPrice, string classID, string classDesc)
if (carts.CartModels == null)
carts.CartModels = new List<CartModel>();
CartModel cart = new CartModel();
cart.className = className;
cart.classPrice = Convert.ToDouble(classPrice);
cart.classID = Convert.ToInt32(classID);
cart.classDesc = classDesc;
//if (className != null)
//
// carts.CartModels.Add(new CartModel() className = className, classPrice = Convert.ToDouble(classPrice), classID = Convert.ToInt32(classID), classDesc = classDesc );
//
if (className != null)
carts.CartModels.Add(new CartModel() className = className, classPrice = Convert.ToDouble(classPrice), classID = Convert.ToInt32(classID), classDesc = classDesc );
//Session["Cart"] = cart;
//ViewBag.Name = Session["Cart"] as ANON_Capstone.Models.Carts;
//ViewData["Cart"] = cart;
Console.WriteLine();
return View(carts);
Courses.cshtml
@model IEnumerable<ANON_Capstone.Models.Course>
@if (!User.Identity.IsAuthenticated)
@section featured
<section class="featured">
<div class="content-wrapper">
<h2 class="text-center">Please Login or Create an Account to make a Purchase!</h2>
</div>
</section>
<div class="row">
<div class="col-lg-9">
<h2><strong>Courses</strong></h2><br />
@foreach (var item in Model)
<div class="col-md-4 col-xs-6 col-s-8 col-lg-4">
@using (Html.BeginForm("ShoppingCart", "Home", FormMethod.Post))
<img src="~/Images/party.gif" style="width: 175px" class="img-responsive" />
<h2>@item.className</h2>
<p>$@item.classPrice -- @item.maxStudent spots left! </p>
<input type="text" name="className" value="@item.className" hidden="hidden" />
<input type="text" name="classPrice" value="@item.classPrice" hidden="hidden" />
<input type="text" name="classID" value="@item.ClassID" hidden="hidden" />
<input type="hidden" name="classDesc" value="@item.classDesc" />
if (User.Identity.IsAuthenticated)
<input class="btn btn-default" type="submit" name="btnConfirm" value="Add to Shopping Cart" />
<br />
</div>
</div>
</div>
ShoppingCart.cshtml
@model ANON_Capstone.Models.Carts
@
ViewBag.Title = "Shopping Cart";
<h2>Shopping Cart</h2>
@using (Html.BeginForm("ValidateCommand", "PayPal"))
<div class="row">
<div class="col-lg-9">
@if (Model.CartModels.Count != 0)
<table border="1">
<tr>
<th>Course Image</th>
<th>Course Name</th>
<th>Course Desc</th>
<th>Course Price</th>
</tr>
@foreach (var item in Model.CartModels)
<tr>
<td><img src="~/Images/party.gif" style="width: 175px" class="img-responsive" /></td>
<td>@item.className</td>
<td>@item.classDesc</td>
<td>@item.classPrice</td>
</tr>
</table>
<input class="btn btn-default" type="submit" name="btnConfirm" value="Check Out with Paypal" />
else
<text>Your shopping cart is currently empty</text>
</div>
</div>
Carts.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data.Entity;
namespace ANON_Capstone.Models
public class Carts
public List<CartModel> CartModels get; set;
public double CartTotal get; set;
CartModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data.Entity;
namespace ANON_Capstone.Models
public class CartModel
public string className get; set;
public double classPrice get; set;
public int classID get; set;
public string classDesc get; set;
c# list asp.net-mvc-4 shopping-cart
I'm trying to make a shopping cart and I'm having trouble with adding an item to the shopping cart.
I'm trying to add items to the carts, but it seems that it is refreshing itself everytime I call the ShoppingCart part of the controller.
HomeController.cs
using System;
using System.Collections.Generic;
using System.Data;
using System.Data.Entity;
using System.Linq;
using System.Web;
using System.Web.Mvc;
using ANON_Capstone.Models;
namespace ANON_Capstone.Controllers
public class HomeController : Controller
private UsersContext db = new UsersContext();
Carts carts = new Carts();
public ActionResult Index()
return View();
public ActionResult About()
return View();
public ActionResult Contact()
return View();
public ActionResult Courses()
return View(db.Courses.ToList());
public ActionResult ShoppingCart(string className, string classPrice, string classID, string classDesc)
if (carts.CartModels == null)
carts.CartModels = new List<CartModel>();
CartModel cart = new CartModel();
cart.className = className;
cart.classPrice = Convert.ToDouble(classPrice);
cart.classID = Convert.ToInt32(classID);
cart.classDesc = classDesc;
//if (className != null)
//
// carts.CartModels.Add(new CartModel() className = className, classPrice = Convert.ToDouble(classPrice), classID = Convert.ToInt32(classID), classDesc = classDesc );
//
if (className != null)
carts.CartModels.Add(new CartModel() className = className, classPrice = Convert.ToDouble(classPrice), classID = Convert.ToInt32(classID), classDesc = classDesc );
//Session["Cart"] = cart;
//ViewBag.Name = Session["Cart"] as ANON_Capstone.Models.Carts;
//ViewData["Cart"] = cart;
Console.WriteLine();
return View(carts);
Courses.cshtml
@model IEnumerable<ANON_Capstone.Models.Course>
@if (!User.Identity.IsAuthenticated)
@section featured
<section class="featured">
<div class="content-wrapper">
<h2 class="text-center">Please Login or Create an Account to make a Purchase!</h2>
</div>
</section>
<div class="row">
<div class="col-lg-9">
<h2><strong>Courses</strong></h2><br />
@foreach (var item in Model)
<div class="col-md-4 col-xs-6 col-s-8 col-lg-4">
@using (Html.BeginForm("ShoppingCart", "Home", FormMethod.Post))
<img src="~/Images/party.gif" style="width: 175px" class="img-responsive" />
<h2>@item.className</h2>
<p>$@item.classPrice -- @item.maxStudent spots left! </p>
<input type="text" name="className" value="@item.className" hidden="hidden" />
<input type="text" name="classPrice" value="@item.classPrice" hidden="hidden" />
<input type="text" name="classID" value="@item.ClassID" hidden="hidden" />
<input type="hidden" name="classDesc" value="@item.classDesc" />
if (User.Identity.IsAuthenticated)
<input class="btn btn-default" type="submit" name="btnConfirm" value="Add to Shopping Cart" />
<br />
</div>
</div>
</div>
ShoppingCart.cshtml
@model ANON_Capstone.Models.Carts
@
ViewBag.Title = "Shopping Cart";
<h2>Shopping Cart</h2>
@using (Html.BeginForm("ValidateCommand", "PayPal"))
<div class="row">
<div class="col-lg-9">
@if (Model.CartModels.Count != 0)
<table border="1">
<tr>
<th>Course Image</th>
<th>Course Name</th>
<th>Course Desc</th>
<th>Course Price</th>
</tr>
@foreach (var item in Model.CartModels)
<tr>
<td><img src="~/Images/party.gif" style="width: 175px" class="img-responsive" /></td>
<td>@item.className</td>
<td>@item.classDesc</td>
<td>@item.classPrice</td>
</tr>
</table>
<input class="btn btn-default" type="submit" name="btnConfirm" value="Check Out with Paypal" />
else
<text>Your shopping cart is currently empty</text>
</div>
</div>
Carts.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data.Entity;
namespace ANON_Capstone.Models
public class Carts
public List<CartModel> CartModels get; set;
public double CartTotal get; set;
CartModel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Data.Entity;
namespace ANON_Capstone.Models
public class CartModel
public string className get; set;
public double classPrice get; set;
public int classID get; set;
public string classDesc get; set;
c# list asp.net-mvc-4 shopping-cart
c# list asp.net-mvc-4 shopping-cart
asked Nov 19 '13 at 15:04
Young-kyu Q Han
551212
551212
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
You are just creating new instances of Carts and CartsModel on every page call rather than loading them from the database.
Web-based applications are stateless, they don't remember variables across requests.
You'll need to write some code to persist and load your cart across multiple requests.
Hi, thank you for the answer. In my home controller, I made sure to create an instance of Carts outside the controllers. Does it still instantiate it everytime I visit a page on home controller? If it does, what method should I use to fix it? Thank you
– Young-kyu Q Han
Nov 19 '13 at 21:49
Yes, it is a new instance every time. You need to give your cart an id and save it to database at the end of every call to the ShoppingCart method. You also need to add a CartId parameter to the ShoppingCart method to load the correct cart from the database on every call.
– Jan Van Herck
Nov 21 '13 at 8:05
add a comment |
up vote
0
down vote
The solution is to involve your foreach
in a "IF" block case and you'll escape your foreach
from a new refresh.
@if(Model.CartModels != null)
foreach (var item in Model.CartModels)
<tr>
<td><img src="~/Images/party.gif" style="width: 175px" class="img-responsive" /></td>
<td>@item.className</td>
<td>@item.classDesc</td>
<td>@item.classPrice</td>
</tr>
</table>
<input class="btn btn-default" type="submit" name="btnConfirm" value="Check Out with Paypal" />
else
<text>Your shopping cart is currently empty</text>
}
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
You are just creating new instances of Carts and CartsModel on every page call rather than loading them from the database.
Web-based applications are stateless, they don't remember variables across requests.
You'll need to write some code to persist and load your cart across multiple requests.
Hi, thank you for the answer. In my home controller, I made sure to create an instance of Carts outside the controllers. Does it still instantiate it everytime I visit a page on home controller? If it does, what method should I use to fix it? Thank you
– Young-kyu Q Han
Nov 19 '13 at 21:49
Yes, it is a new instance every time. You need to give your cart an id and save it to database at the end of every call to the ShoppingCart method. You also need to add a CartId parameter to the ShoppingCart method to load the correct cart from the database on every call.
– Jan Van Herck
Nov 21 '13 at 8:05
add a comment |
up vote
0
down vote
You are just creating new instances of Carts and CartsModel on every page call rather than loading them from the database.
Web-based applications are stateless, they don't remember variables across requests.
You'll need to write some code to persist and load your cart across multiple requests.
Hi, thank you for the answer. In my home controller, I made sure to create an instance of Carts outside the controllers. Does it still instantiate it everytime I visit a page on home controller? If it does, what method should I use to fix it? Thank you
– Young-kyu Q Han
Nov 19 '13 at 21:49
Yes, it is a new instance every time. You need to give your cart an id and save it to database at the end of every call to the ShoppingCart method. You also need to add a CartId parameter to the ShoppingCart method to load the correct cart from the database on every call.
– Jan Van Herck
Nov 21 '13 at 8:05
add a comment |
up vote
0
down vote
up vote
0
down vote
You are just creating new instances of Carts and CartsModel on every page call rather than loading them from the database.
Web-based applications are stateless, they don't remember variables across requests.
You'll need to write some code to persist and load your cart across multiple requests.
You are just creating new instances of Carts and CartsModel on every page call rather than loading them from the database.
Web-based applications are stateless, they don't remember variables across requests.
You'll need to write some code to persist and load your cart across multiple requests.
answered Nov 19 '13 at 15:16
Jan Van Herck
1,674913
1,674913
Hi, thank you for the answer. In my home controller, I made sure to create an instance of Carts outside the controllers. Does it still instantiate it everytime I visit a page on home controller? If it does, what method should I use to fix it? Thank you
– Young-kyu Q Han
Nov 19 '13 at 21:49
Yes, it is a new instance every time. You need to give your cart an id and save it to database at the end of every call to the ShoppingCart method. You also need to add a CartId parameter to the ShoppingCart method to load the correct cart from the database on every call.
– Jan Van Herck
Nov 21 '13 at 8:05
add a comment |
Hi, thank you for the answer. In my home controller, I made sure to create an instance of Carts outside the controllers. Does it still instantiate it everytime I visit a page on home controller? If it does, what method should I use to fix it? Thank you
– Young-kyu Q Han
Nov 19 '13 at 21:49
Yes, it is a new instance every time. You need to give your cart an id and save it to database at the end of every call to the ShoppingCart method. You also need to add a CartId parameter to the ShoppingCart method to load the correct cart from the database on every call.
– Jan Van Herck
Nov 21 '13 at 8:05
Hi, thank you for the answer. In my home controller, I made sure to create an instance of Carts outside the controllers. Does it still instantiate it everytime I visit a page on home controller? If it does, what method should I use to fix it? Thank you
– Young-kyu Q Han
Nov 19 '13 at 21:49
Hi, thank you for the answer. In my home controller, I made sure to create an instance of Carts outside the controllers. Does it still instantiate it everytime I visit a page on home controller? If it does, what method should I use to fix it? Thank you
– Young-kyu Q Han
Nov 19 '13 at 21:49
Yes, it is a new instance every time. You need to give your cart an id and save it to database at the end of every call to the ShoppingCart method. You also need to add a CartId parameter to the ShoppingCart method to load the correct cart from the database on every call.
– Jan Van Herck
Nov 21 '13 at 8:05
Yes, it is a new instance every time. You need to give your cart an id and save it to database at the end of every call to the ShoppingCart method. You also need to add a CartId parameter to the ShoppingCart method to load the correct cart from the database on every call.
– Jan Van Herck
Nov 21 '13 at 8:05
add a comment |
up vote
0
down vote
The solution is to involve your foreach
in a "IF" block case and you'll escape your foreach
from a new refresh.
@if(Model.CartModels != null)
foreach (var item in Model.CartModels)
<tr>
<td><img src="~/Images/party.gif" style="width: 175px" class="img-responsive" /></td>
<td>@item.className</td>
<td>@item.classDesc</td>
<td>@item.classPrice</td>
</tr>
</table>
<input class="btn btn-default" type="submit" name="btnConfirm" value="Check Out with Paypal" />
else
<text>Your shopping cart is currently empty</text>
}
add a comment |
up vote
0
down vote
The solution is to involve your foreach
in a "IF" block case and you'll escape your foreach
from a new refresh.
@if(Model.CartModels != null)
foreach (var item in Model.CartModels)
<tr>
<td><img src="~/Images/party.gif" style="width: 175px" class="img-responsive" /></td>
<td>@item.className</td>
<td>@item.classDesc</td>
<td>@item.classPrice</td>
</tr>
</table>
<input class="btn btn-default" type="submit" name="btnConfirm" value="Check Out with Paypal" />
else
<text>Your shopping cart is currently empty</text>
}
add a comment |
up vote
0
down vote
up vote
0
down vote
The solution is to involve your foreach
in a "IF" block case and you'll escape your foreach
from a new refresh.
@if(Model.CartModels != null)
foreach (var item in Model.CartModels)
<tr>
<td><img src="~/Images/party.gif" style="width: 175px" class="img-responsive" /></td>
<td>@item.className</td>
<td>@item.classDesc</td>
<td>@item.classPrice</td>
</tr>
</table>
<input class="btn btn-default" type="submit" name="btnConfirm" value="Check Out with Paypal" />
else
<text>Your shopping cart is currently empty</text>
}
The solution is to involve your foreach
in a "IF" block case and you'll escape your foreach
from a new refresh.
@if(Model.CartModels != null)
foreach (var item in Model.CartModels)
<tr>
<td><img src="~/Images/party.gif" style="width: 175px" class="img-responsive" /></td>
<td>@item.className</td>
<td>@item.classDesc</td>
<td>@item.classPrice</td>
</tr>
</table>
<input class="btn btn-default" type="submit" name="btnConfirm" value="Check Out with Paypal" />
else
<text>Your shopping cart is currently empty</text>
}
edited Nov 10 at 14:18


Stephen Rauch
27.5k153156
27.5k153156
answered Nov 10 at 13:59
Nilson Martins
11
11
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f20074964%2fmvc-controller-shopping-cart-adding-to-a-list%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ORJ52IMtWFMwKp3B,m RJq48GcdjeD