While loop in Bash script?
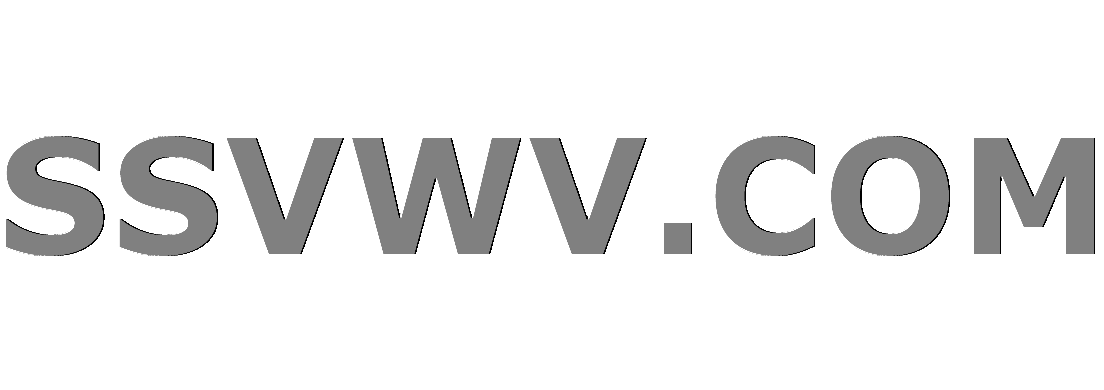
Multi tool use
I'm not used to writing Bash scripts, and Google didn't help in figuring out what is wrong with this script:
#!/bin/bash
while read myline
do
done
echo "Hello"
while read line
do
done
exit 0
The output I get is:
./basic.agi: line 4: syntax error near unexpected token 'done'
./basic.agi: line 4: 'done'
and my bash
version is:
GNU bash, version 3.2.25(1)-release (i686-redhat-linux-gnu)
Thank you.
Edit: The script works OK when the while loop isn't empty.
While I'm at it... I expected to exit the loop when the user typed nothing, ie. simply hit the Enter key, but Bash keeps looping. How can I exit the loop?
while read myline
do
echo $myline
done
echo "Hello"
while read line
do
true
done
exit 0
linux bash
add a comment |
I'm not used to writing Bash scripts, and Google didn't help in figuring out what is wrong with this script:
#!/bin/bash
while read myline
do
done
echo "Hello"
while read line
do
done
exit 0
The output I get is:
./basic.agi: line 4: syntax error near unexpected token 'done'
./basic.agi: line 4: 'done'
and my bash
version is:
GNU bash, version 3.2.25(1)-release (i686-redhat-linux-gnu)
Thank you.
Edit: The script works OK when the while loop isn't empty.
While I'm at it... I expected to exit the loop when the user typed nothing, ie. simply hit the Enter key, but Bash keeps looping. How can I exit the loop?
while read myline
do
echo $myline
done
echo "Hello"
while read line
do
true
done
exit 0
linux bash
1
This simple read-loop works until "end-of-file". You can give an end-of-file by typing control-D.
– Paŭlo Ebermann
Feb 25 '11 at 16:33
add a comment |
I'm not used to writing Bash scripts, and Google didn't help in figuring out what is wrong with this script:
#!/bin/bash
while read myline
do
done
echo "Hello"
while read line
do
done
exit 0
The output I get is:
./basic.agi: line 4: syntax error near unexpected token 'done'
./basic.agi: line 4: 'done'
and my bash
version is:
GNU bash, version 3.2.25(1)-release (i686-redhat-linux-gnu)
Thank you.
Edit: The script works OK when the while loop isn't empty.
While I'm at it... I expected to exit the loop when the user typed nothing, ie. simply hit the Enter key, but Bash keeps looping. How can I exit the loop?
while read myline
do
echo $myline
done
echo "Hello"
while read line
do
true
done
exit 0
linux bash
I'm not used to writing Bash scripts, and Google didn't help in figuring out what is wrong with this script:
#!/bin/bash
while read myline
do
done
echo "Hello"
while read line
do
done
exit 0
The output I get is:
./basic.agi: line 4: syntax error near unexpected token 'done'
./basic.agi: line 4: 'done'
and my bash
version is:
GNU bash, version 3.2.25(1)-release (i686-redhat-linux-gnu)
Thank you.
Edit: The script works OK when the while loop isn't empty.
While I'm at it... I expected to exit the loop when the user typed nothing, ie. simply hit the Enter key, but Bash keeps looping. How can I exit the loop?
while read myline
do
echo $myline
done
echo "Hello"
while read line
do
true
done
exit 0
linux bash
linux bash
edited Sep 3 '15 at 8:27


paxdiablo
641k17412621681
641k17412621681
asked Feb 24 '11 at 12:07
GulbaharGulbahar
2,264175380
2,264175380
1
This simple read-loop works until "end-of-file". You can give an end-of-file by typing control-D.
– Paŭlo Ebermann
Feb 25 '11 at 16:33
add a comment |
1
This simple read-loop works until "end-of-file". You can give an end-of-file by typing control-D.
– Paŭlo Ebermann
Feb 25 '11 at 16:33
1
1
This simple read-loop works until "end-of-file". You can give an end-of-file by typing control-D.
– Paŭlo Ebermann
Feb 25 '11 at 16:33
This simple read-loop works until "end-of-file". You can give an end-of-file by typing control-D.
– Paŭlo Ebermann
Feb 25 '11 at 16:33
add a comment |
6 Answers
6
active
oldest
votes
You can't have an empty loop. If you want a placeholder, just use true
.
#!/bin/bash
while read myline
do
true
done
or, more likely, do something useful with the input:
#!/bin/bash
while read myline
do
echo "You entered [$line]"
done
As for your second question, on how to exit the loop when the user just presses ENTER with nothing else, you can do something:
#!/bin/bash
read line
while [[ "$line" != "" ]] ; do
echo "You entered [$line]"
read line
done
Thanks, that solved the issue.
– Gulbahar
Feb 24 '11 at 12:23
Thanks again. Next time, I'll post a new question instead.
– Gulbahar
Feb 24 '11 at 12:35
while :; do :; done
work (:
is a synonym fortrue
)
– Fixee
Aug 29 '14 at 1:42
add a comment |
When you are using the read
command in a while loop it need input:
echo "Hello" | while read line ; do echo $line ; done
or using several lines:
echo "Hello" | while read line
do
echo $line
done
add a comment |
What are you trying to do? From the look of it it seems that you are trying to read into a variable?
This is done by simply stating read the value can then be found inside of $
ex:
read myvar
echo $myvar
As other have stated the trouble with the loop is that it is empty which is not allowed.
add a comment |
This is a way to emulate a do
while
loop in Bash, It always executes once and does the test at the end.
while
read -r line
[[ $line ]]
do
:
done
When an empty line is entered, the loop exits. The variable will be empty at that point, but you could set another variable to its value to preserve it, if needed.
while
save=$line
read -r line
[[ $line ]]
do
:
done
Dennis, did you mean to initline
with something other than''
? It appears to me that the loop will never start. My understanding is that until is simply the negative condition version of while in that it doesn't guarantee a minimum execution of one iteration. I may be wrong but the bash manpage seems to indicate that's the case as well.
– paxdiablo
Jul 22 '13 at 22:53
@paxdiablo: You are correct. Other languages have a properdo
loop with a test at the end, but Bash does not. My answer here shows how to emulate ado
while
loop in Bash. I've updated this answer to match that one.
– Dennis Williamson
Jul 23 '13 at 0:14
add a comment |
If you are looking to create a menu with choices as a infinite loop (with the choice to break out)
PS3='Please enter your choice: '
options=("hello" "date" "quit")
select opt in "$options[@]"
do
case $opt in
"hello") echo "world";;
"date") echo $(date);;
"quit")
break;;
*) echo "invalid option";;
esac
done
PS3 is described here
add a comment |
If you type help while
in bash you'll get an explanation of the command.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f5104426%2fwhile-loop-in-bash-script%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can't have an empty loop. If you want a placeholder, just use true
.
#!/bin/bash
while read myline
do
true
done
or, more likely, do something useful with the input:
#!/bin/bash
while read myline
do
echo "You entered [$line]"
done
As for your second question, on how to exit the loop when the user just presses ENTER with nothing else, you can do something:
#!/bin/bash
read line
while [[ "$line" != "" ]] ; do
echo "You entered [$line]"
read line
done
Thanks, that solved the issue.
– Gulbahar
Feb 24 '11 at 12:23
Thanks again. Next time, I'll post a new question instead.
– Gulbahar
Feb 24 '11 at 12:35
while :; do :; done
work (:
is a synonym fortrue
)
– Fixee
Aug 29 '14 at 1:42
add a comment |
You can't have an empty loop. If you want a placeholder, just use true
.
#!/bin/bash
while read myline
do
true
done
or, more likely, do something useful with the input:
#!/bin/bash
while read myline
do
echo "You entered [$line]"
done
As for your second question, on how to exit the loop when the user just presses ENTER with nothing else, you can do something:
#!/bin/bash
read line
while [[ "$line" != "" ]] ; do
echo "You entered [$line]"
read line
done
Thanks, that solved the issue.
– Gulbahar
Feb 24 '11 at 12:23
Thanks again. Next time, I'll post a new question instead.
– Gulbahar
Feb 24 '11 at 12:35
while :; do :; done
work (:
is a synonym fortrue
)
– Fixee
Aug 29 '14 at 1:42
add a comment |
You can't have an empty loop. If you want a placeholder, just use true
.
#!/bin/bash
while read myline
do
true
done
or, more likely, do something useful with the input:
#!/bin/bash
while read myline
do
echo "You entered [$line]"
done
As for your second question, on how to exit the loop when the user just presses ENTER with nothing else, you can do something:
#!/bin/bash
read line
while [[ "$line" != "" ]] ; do
echo "You entered [$line]"
read line
done
You can't have an empty loop. If you want a placeholder, just use true
.
#!/bin/bash
while read myline
do
true
done
or, more likely, do something useful with the input:
#!/bin/bash
while read myline
do
echo "You entered [$line]"
done
As for your second question, on how to exit the loop when the user just presses ENTER with nothing else, you can do something:
#!/bin/bash
read line
while [[ "$line" != "" ]] ; do
echo "You entered [$line]"
read line
done
edited Sep 3 '15 at 8:23
answered Feb 24 '11 at 12:10


paxdiablopaxdiablo
641k17412621681
641k17412621681
Thanks, that solved the issue.
– Gulbahar
Feb 24 '11 at 12:23
Thanks again. Next time, I'll post a new question instead.
– Gulbahar
Feb 24 '11 at 12:35
while :; do :; done
work (:
is a synonym fortrue
)
– Fixee
Aug 29 '14 at 1:42
add a comment |
Thanks, that solved the issue.
– Gulbahar
Feb 24 '11 at 12:23
Thanks again. Next time, I'll post a new question instead.
– Gulbahar
Feb 24 '11 at 12:35
while :; do :; done
work (:
is a synonym fortrue
)
– Fixee
Aug 29 '14 at 1:42
Thanks, that solved the issue.
– Gulbahar
Feb 24 '11 at 12:23
Thanks, that solved the issue.
– Gulbahar
Feb 24 '11 at 12:23
Thanks again. Next time, I'll post a new question instead.
– Gulbahar
Feb 24 '11 at 12:35
Thanks again. Next time, I'll post a new question instead.
– Gulbahar
Feb 24 '11 at 12:35
while :; do :; done
work (:
is a synonym for true
)– Fixee
Aug 29 '14 at 1:42
while :; do :; done
work (:
is a synonym for true
)– Fixee
Aug 29 '14 at 1:42
add a comment |
When you are using the read
command in a while loop it need input:
echo "Hello" | while read line ; do echo $line ; done
or using several lines:
echo "Hello" | while read line
do
echo $line
done
add a comment |
When you are using the read
command in a while loop it need input:
echo "Hello" | while read line ; do echo $line ; done
or using several lines:
echo "Hello" | while read line
do
echo $line
done
add a comment |
When you are using the read
command in a while loop it need input:
echo "Hello" | while read line ; do echo $line ; done
or using several lines:
echo "Hello" | while read line
do
echo $line
done
When you are using the read
command in a while loop it need input:
echo "Hello" | while read line ; do echo $line ; done
or using several lines:
echo "Hello" | while read line
do
echo $line
done
answered Feb 24 '11 at 12:11
Anders ZommarinAnders Zommarin
4,75311921
4,75311921
add a comment |
add a comment |
What are you trying to do? From the look of it it seems that you are trying to read into a variable?
This is done by simply stating read the value can then be found inside of $
ex:
read myvar
echo $myvar
As other have stated the trouble with the loop is that it is empty which is not allowed.
add a comment |
What are you trying to do? From the look of it it seems that you are trying to read into a variable?
This is done by simply stating read the value can then be found inside of $
ex:
read myvar
echo $myvar
As other have stated the trouble with the loop is that it is empty which is not allowed.
add a comment |
What are you trying to do? From the look of it it seems that you are trying to read into a variable?
This is done by simply stating read the value can then be found inside of $
ex:
read myvar
echo $myvar
As other have stated the trouble with the loop is that it is empty which is not allowed.
What are you trying to do? From the look of it it seems that you are trying to read into a variable?
This is done by simply stating read the value can then be found inside of $
ex:
read myvar
echo $myvar
As other have stated the trouble with the loop is that it is empty which is not allowed.
answered Feb 24 '11 at 12:12
SedrikSedrik
1,2681317
1,2681317
add a comment |
add a comment |
This is a way to emulate a do
while
loop in Bash, It always executes once and does the test at the end.
while
read -r line
[[ $line ]]
do
:
done
When an empty line is entered, the loop exits. The variable will be empty at that point, but you could set another variable to its value to preserve it, if needed.
while
save=$line
read -r line
[[ $line ]]
do
:
done
Dennis, did you mean to initline
with something other than''
? It appears to me that the loop will never start. My understanding is that until is simply the negative condition version of while in that it doesn't guarantee a minimum execution of one iteration. I may be wrong but the bash manpage seems to indicate that's the case as well.
– paxdiablo
Jul 22 '13 at 22:53
@paxdiablo: You are correct. Other languages have a properdo
loop with a test at the end, but Bash does not. My answer here shows how to emulate ado
while
loop in Bash. I've updated this answer to match that one.
– Dennis Williamson
Jul 23 '13 at 0:14
add a comment |
This is a way to emulate a do
while
loop in Bash, It always executes once and does the test at the end.
while
read -r line
[[ $line ]]
do
:
done
When an empty line is entered, the loop exits. The variable will be empty at that point, but you could set another variable to its value to preserve it, if needed.
while
save=$line
read -r line
[[ $line ]]
do
:
done
Dennis, did you mean to initline
with something other than''
? It appears to me that the loop will never start. My understanding is that until is simply the negative condition version of while in that it doesn't guarantee a minimum execution of one iteration. I may be wrong but the bash manpage seems to indicate that's the case as well.
– paxdiablo
Jul 22 '13 at 22:53
@paxdiablo: You are correct. Other languages have a properdo
loop with a test at the end, but Bash does not. My answer here shows how to emulate ado
while
loop in Bash. I've updated this answer to match that one.
– Dennis Williamson
Jul 23 '13 at 0:14
add a comment |
This is a way to emulate a do
while
loop in Bash, It always executes once and does the test at the end.
while
read -r line
[[ $line ]]
do
:
done
When an empty line is entered, the loop exits. The variable will be empty at that point, but you could set another variable to its value to preserve it, if needed.
while
save=$line
read -r line
[[ $line ]]
do
:
done
This is a way to emulate a do
while
loop in Bash, It always executes once and does the test at the end.
while
read -r line
[[ $line ]]
do
:
done
When an empty line is entered, the loop exits. The variable will be empty at that point, but you could set another variable to its value to preserve it, if needed.
while
save=$line
read -r line
[[ $line ]]
do
:
done
edited Jul 23 '13 at 0:14
answered Feb 24 '11 at 15:51
Dennis WilliamsonDennis Williamson
244k64309379
244k64309379
Dennis, did you mean to initline
with something other than''
? It appears to me that the loop will never start. My understanding is that until is simply the negative condition version of while in that it doesn't guarantee a minimum execution of one iteration. I may be wrong but the bash manpage seems to indicate that's the case as well.
– paxdiablo
Jul 22 '13 at 22:53
@paxdiablo: You are correct. Other languages have a properdo
loop with a test at the end, but Bash does not. My answer here shows how to emulate ado
while
loop in Bash. I've updated this answer to match that one.
– Dennis Williamson
Jul 23 '13 at 0:14
add a comment |
Dennis, did you mean to initline
with something other than''
? It appears to me that the loop will never start. My understanding is that until is simply the negative condition version of while in that it doesn't guarantee a minimum execution of one iteration. I may be wrong but the bash manpage seems to indicate that's the case as well.
– paxdiablo
Jul 22 '13 at 22:53
@paxdiablo: You are correct. Other languages have a properdo
loop with a test at the end, but Bash does not. My answer here shows how to emulate ado
while
loop in Bash. I've updated this answer to match that one.
– Dennis Williamson
Jul 23 '13 at 0:14
Dennis, did you mean to init
line
with something other than ''
? It appears to me that the loop will never start. My understanding is that until is simply the negative condition version of while in that it doesn't guarantee a minimum execution of one iteration. I may be wrong but the bash manpage seems to indicate that's the case as well.– paxdiablo
Jul 22 '13 at 22:53
Dennis, did you mean to init
line
with something other than ''
? It appears to me that the loop will never start. My understanding is that until is simply the negative condition version of while in that it doesn't guarantee a minimum execution of one iteration. I may be wrong but the bash manpage seems to indicate that's the case as well.– paxdiablo
Jul 22 '13 at 22:53
@paxdiablo: You are correct. Other languages have a proper
do
loop with a test at the end, but Bash does not. My answer here shows how to emulate a do
while
loop in Bash. I've updated this answer to match that one.– Dennis Williamson
Jul 23 '13 at 0:14
@paxdiablo: You are correct. Other languages have a proper
do
loop with a test at the end, but Bash does not. My answer here shows how to emulate a do
while
loop in Bash. I've updated this answer to match that one.– Dennis Williamson
Jul 23 '13 at 0:14
add a comment |
If you are looking to create a menu with choices as a infinite loop (with the choice to break out)
PS3='Please enter your choice: '
options=("hello" "date" "quit")
select opt in "$options[@]"
do
case $opt in
"hello") echo "world";;
"date") echo $(date);;
"quit")
break;;
*) echo "invalid option";;
esac
done
PS3 is described here
add a comment |
If you are looking to create a menu with choices as a infinite loop (with the choice to break out)
PS3='Please enter your choice: '
options=("hello" "date" "quit")
select opt in "$options[@]"
do
case $opt in
"hello") echo "world";;
"date") echo $(date);;
"quit")
break;;
*) echo "invalid option";;
esac
done
PS3 is described here
add a comment |
If you are looking to create a menu with choices as a infinite loop (with the choice to break out)
PS3='Please enter your choice: '
options=("hello" "date" "quit")
select opt in "$options[@]"
do
case $opt in
"hello") echo "world";;
"date") echo $(date);;
"quit")
break;;
*) echo "invalid option";;
esac
done
PS3 is described here
If you are looking to create a menu with choices as a infinite loop (with the choice to break out)
PS3='Please enter your choice: '
options=("hello" "date" "quit")
select opt in "$options[@]"
do
case $opt in
"hello") echo "world";;
"date") echo $(date);;
"quit")
break;;
*) echo "invalid option";;
esac
done
PS3 is described here
answered Nov 14 '18 at 22:31


BenbentwoBenbentwo
1116
1116
add a comment |
add a comment |
If you type help while
in bash you'll get an explanation of the command.
add a comment |
If you type help while
in bash you'll get an explanation of the command.
add a comment |
If you type help while
in bash you'll get an explanation of the command.
If you type help while
in bash you'll get an explanation of the command.
answered Feb 24 '11 at 12:11
onemasseonemasse
4,47052635
4,47052635
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f5104426%2fwhile-loop-in-bash-script%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
RfzuUU,p6QM9quXtvvmkjt,YVbVAIvyF0XLeZZD3XtqP9SNwSztWg zz
1
This simple read-loop works until "end-of-file". You can give an end-of-file by typing control-D.
– Paŭlo Ebermann
Feb 25 '11 at 16:33