Swift 3 - Saving images to Core Data
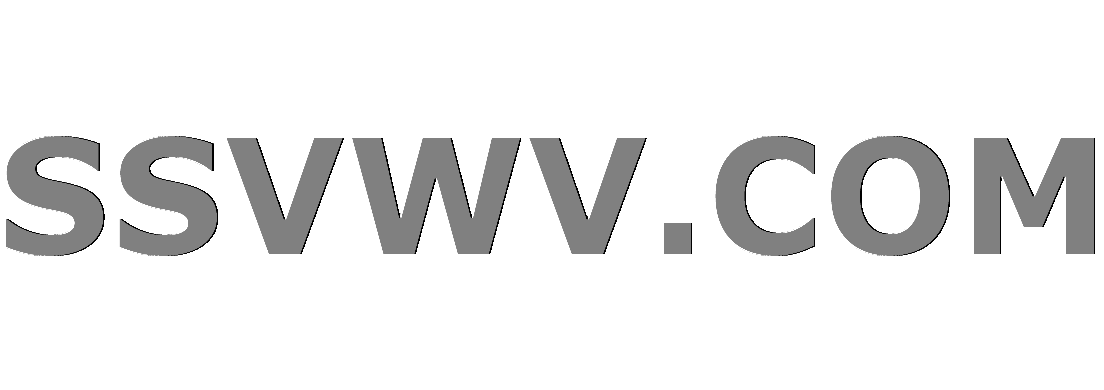
Multi tool use
For some reason I can't figure out how to save images to core data and fetch them again. I have a feeling it's something about my types but have a look:
I get my data from an api call to my server. It returns a base64 string.
Here is where I get the data:
updateAccessTokenOnly(newAccessToken: aToken!)
saveImageToDB(brandName: imageBrandName, image: data! )
Here I save it to my DB:
func saveImageToDB(brandName: String, image: Data)
dropImages()tableDropped in
let managedContext = getContext()
let entity = NSEntityDescription.entity(forEntityName: "CoffeeShopImage", in: managedContext)!
let CSI = NSManagedObject(entity: entity, insertInto: managedContext)
CSI.setValue(image, forKey: "image")
CSI.setValue(brandName, forKey: "brandName")
do
try managedContext.save()
print("saved!")
catch let error as NSError
print("Could not save. (error), (error.userInfo)")
then to fetch it:
func getImageFromDB(callback: @escaping (_ image: UIImage)-> ())
let fetchRequest: NSFetchRequest<NSManagedObject> = NSFetchRequest(entityName: "CoffeeShopImage")
do
let searchResults = try getContext().fetch(fetchRequest)
for images in searchResults
print("vi når her ned i get image")
if (images.value(forKey: "brandName")! as! String == "Baresso")
print(images.value(forKey: "brandName")! as! String)
let image: Data = images.value(forKey: "image")! as! Data
let decodedimage = UIImage(data: image)
callback(decodedimage!)
catch
print("Error with request: (error)")
Full error log:
https://docs.google.com/document/d/1gSXE64Sxtzo81eBSjv4bnBjBnnmG4MX2tuvNtnuJDIM/edit?usp=sharing
Hope someone can help. Thanks in advance!
UPDATED
So I uninstalled the app and then the code above worked. However the pictures come out blue? (yes I've checked that the pictures sent from the database are correct).
Any solution?
ios core-data swift3 base64
add a comment |
For some reason I can't figure out how to save images to core data and fetch them again. I have a feeling it's something about my types but have a look:
I get my data from an api call to my server. It returns a base64 string.
Here is where I get the data:
updateAccessTokenOnly(newAccessToken: aToken!)
saveImageToDB(brandName: imageBrandName, image: data! )
Here I save it to my DB:
func saveImageToDB(brandName: String, image: Data)
dropImages()tableDropped in
let managedContext = getContext()
let entity = NSEntityDescription.entity(forEntityName: "CoffeeShopImage", in: managedContext)!
let CSI = NSManagedObject(entity: entity, insertInto: managedContext)
CSI.setValue(image, forKey: "image")
CSI.setValue(brandName, forKey: "brandName")
do
try managedContext.save()
print("saved!")
catch let error as NSError
print("Could not save. (error), (error.userInfo)")
then to fetch it:
func getImageFromDB(callback: @escaping (_ image: UIImage)-> ())
let fetchRequest: NSFetchRequest<NSManagedObject> = NSFetchRequest(entityName: "CoffeeShopImage")
do
let searchResults = try getContext().fetch(fetchRequest)
for images in searchResults
print("vi når her ned i get image")
if (images.value(forKey: "brandName")! as! String == "Baresso")
print(images.value(forKey: "brandName")! as! String)
let image: Data = images.value(forKey: "image")! as! Data
let decodedimage = UIImage(data: image)
callback(decodedimage!)
catch
print("Error with request: (error)")
Full error log:
https://docs.google.com/document/d/1gSXE64Sxtzo81eBSjv4bnBjBnnmG4MX2tuvNtnuJDIM/edit?usp=sharing
Hope someone can help. Thanks in advance!
UPDATED
So I uninstalled the app and then the code above worked. However the pictures come out blue? (yes I've checked that the pictures sent from the database are correct).
Any solution?
ios core-data swift3 base64
2
Better approach would be storing images inDocumentDirectory
orLibrary
and store the path to image in CoreData as string. Storing images (as Binary Data or Transformable) in CoreData will make encoding/decoding a heavy operation.
– viral
Feb 27 '17 at 11:33
add a comment |
For some reason I can't figure out how to save images to core data and fetch them again. I have a feeling it's something about my types but have a look:
I get my data from an api call to my server. It returns a base64 string.
Here is where I get the data:
updateAccessTokenOnly(newAccessToken: aToken!)
saveImageToDB(brandName: imageBrandName, image: data! )
Here I save it to my DB:
func saveImageToDB(brandName: String, image: Data)
dropImages()tableDropped in
let managedContext = getContext()
let entity = NSEntityDescription.entity(forEntityName: "CoffeeShopImage", in: managedContext)!
let CSI = NSManagedObject(entity: entity, insertInto: managedContext)
CSI.setValue(image, forKey: "image")
CSI.setValue(brandName, forKey: "brandName")
do
try managedContext.save()
print("saved!")
catch let error as NSError
print("Could not save. (error), (error.userInfo)")
then to fetch it:
func getImageFromDB(callback: @escaping (_ image: UIImage)-> ())
let fetchRequest: NSFetchRequest<NSManagedObject> = NSFetchRequest(entityName: "CoffeeShopImage")
do
let searchResults = try getContext().fetch(fetchRequest)
for images in searchResults
print("vi når her ned i get image")
if (images.value(forKey: "brandName")! as! String == "Baresso")
print(images.value(forKey: "brandName")! as! String)
let image: Data = images.value(forKey: "image")! as! Data
let decodedimage = UIImage(data: image)
callback(decodedimage!)
catch
print("Error with request: (error)")
Full error log:
https://docs.google.com/document/d/1gSXE64Sxtzo81eBSjv4bnBjBnnmG4MX2tuvNtnuJDIM/edit?usp=sharing
Hope someone can help. Thanks in advance!
UPDATED
So I uninstalled the app and then the code above worked. However the pictures come out blue? (yes I've checked that the pictures sent from the database are correct).
Any solution?
ios core-data swift3 base64
For some reason I can't figure out how to save images to core data and fetch them again. I have a feeling it's something about my types but have a look:
I get my data from an api call to my server. It returns a base64 string.
Here is where I get the data:
updateAccessTokenOnly(newAccessToken: aToken!)
saveImageToDB(brandName: imageBrandName, image: data! )
Here I save it to my DB:
func saveImageToDB(brandName: String, image: Data)
dropImages()tableDropped in
let managedContext = getContext()
let entity = NSEntityDescription.entity(forEntityName: "CoffeeShopImage", in: managedContext)!
let CSI = NSManagedObject(entity: entity, insertInto: managedContext)
CSI.setValue(image, forKey: "image")
CSI.setValue(brandName, forKey: "brandName")
do
try managedContext.save()
print("saved!")
catch let error as NSError
print("Could not save. (error), (error.userInfo)")
then to fetch it:
func getImageFromDB(callback: @escaping (_ image: UIImage)-> ())
let fetchRequest: NSFetchRequest<NSManagedObject> = NSFetchRequest(entityName: "CoffeeShopImage")
do
let searchResults = try getContext().fetch(fetchRequest)
for images in searchResults
print("vi når her ned i get image")
if (images.value(forKey: "brandName")! as! String == "Baresso")
print(images.value(forKey: "brandName")! as! String)
let image: Data = images.value(forKey: "image")! as! Data
let decodedimage = UIImage(data: image)
callback(decodedimage!)
catch
print("Error with request: (error)")
Full error log:
https://docs.google.com/document/d/1gSXE64Sxtzo81eBSjv4bnBjBnnmG4MX2tuvNtnuJDIM/edit?usp=sharing
Hope someone can help. Thanks in advance!
UPDATED
So I uninstalled the app and then the code above worked. However the pictures come out blue? (yes I've checked that the pictures sent from the database are correct).
Any solution?
ios core-data swift3 base64
ios core-data swift3 base64
edited Feb 28 '17 at 7:53
Steffen L.
asked Feb 27 '17 at 11:01


Steffen L.Steffen L.
94213
94213
2
Better approach would be storing images inDocumentDirectory
orLibrary
and store the path to image in CoreData as string. Storing images (as Binary Data or Transformable) in CoreData will make encoding/decoding a heavy operation.
– viral
Feb 27 '17 at 11:33
add a comment |
2
Better approach would be storing images inDocumentDirectory
orLibrary
and store the path to image in CoreData as string. Storing images (as Binary Data or Transformable) in CoreData will make encoding/decoding a heavy operation.
– viral
Feb 27 '17 at 11:33
2
2
Better approach would be storing images in
DocumentDirectory
or Library
and store the path to image in CoreData as string. Storing images (as Binary Data or Transformable) in CoreData will make encoding/decoding a heavy operation.– viral
Feb 27 '17 at 11:33
Better approach would be storing images in
DocumentDirectory
or Library
and store the path to image in CoreData as string. Storing images (as Binary Data or Transformable) in CoreData will make encoding/decoding a heavy operation.– viral
Feb 27 '17 at 11:33
add a comment |
3 Answers
3
active
oldest
votes
replace
let image: Data = images.value(forKey: "image")! as! Data
let dataDecoded : Data = Data(base64Encoded: image, options: )!
let decodedimage = UIImage(data: dataDecoded)
with
let image: Data = images.value(forKey: "image")! as! Data
let decodedimage = UIImage(data: image)
Base64 is a way to to convert data to a string. There is no reason to use it here. You already have the data from the database you just want to convert it to a UIImage.
also change
let image = data?.base64EncodedData()
saveImageToDB(brandName: imageBrandName, image: image!)
to
saveImageToDB(brandName: imageBrandName, image: data!)
base64EncodedData
is turning the data from image data into a utf-8 encoded based64encoded string. There is no reason for that.
You should get the base64 encoded string from server, convert it to data and then you never need base64 again. Read and write data to your database, and after you read it convert it to a UIImage. Base64 is an encoding method to transfer data. If you are not talking to the server there is no reason to use base64.
1
Hey Jon, my new best friend hehe. It seems that my error I get is from saving. I tried saving it as a string instead of data. Which seemed to save alright, however when I fetched my images they came out as blue squares instead of, well images. But at the moment, it won't save with the code above.
– Steffen L.
Feb 27 '17 at 11:28
Should I infact store the data as a String instead of Binary Data? Or?
– Steffen L.
Feb 27 '17 at 11:30
Hi Jon, I made the changes you suggested, however I am still getting the error on save. I have updated the code and also put the whole error log.
– Steffen L.
Feb 27 '17 at 16:27
Which means I can't even test the fetch, because it won't save.
– Steffen L.
Feb 27 '17 at 16:32
The error "Can't find or automatically infer mapping model for migration" means that you need to delete you app and reinstall.
– Jon Rose
Feb 28 '17 at 6:36
|
show 8 more comments
After the suggested corrections from Jon Rose all I needed was to add
.withRenderingMode(.alwaysOriginal)
to where I was showing my picture and the code worked.
add a comment |
Saving Image:
guard let managedObjectContext = managedObjectContext else return
// Create User
let user = User(context: managedObjectContext)
// Configure User
user.name = "name"
user.about = "about"
user.address = "Address"
user.age = 30
if let img = UIImage(named: "dog.png")
let data = img.pngData() as NSData?
user.image = data
Fetching Image:
// Create Fetch Request
let fetchRequest: NSFetchRequest<User> = User.fetchRequest()
// Configure Fetch Request
fetchRequest.sortDescriptors = [NSSortDescriptor(key: "name", ascending: true)]
let users = try! managedContext.fetch(fetchRequest)
let user: User = users.first as! User
if let imageData = user?.image
imgView.image = UIImage(data: imageData as Data)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f42484089%2fswift-3-saving-images-to-core-data%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
replace
let image: Data = images.value(forKey: "image")! as! Data
let dataDecoded : Data = Data(base64Encoded: image, options: )!
let decodedimage = UIImage(data: dataDecoded)
with
let image: Data = images.value(forKey: "image")! as! Data
let decodedimage = UIImage(data: image)
Base64 is a way to to convert data to a string. There is no reason to use it here. You already have the data from the database you just want to convert it to a UIImage.
also change
let image = data?.base64EncodedData()
saveImageToDB(brandName: imageBrandName, image: image!)
to
saveImageToDB(brandName: imageBrandName, image: data!)
base64EncodedData
is turning the data from image data into a utf-8 encoded based64encoded string. There is no reason for that.
You should get the base64 encoded string from server, convert it to data and then you never need base64 again. Read and write data to your database, and after you read it convert it to a UIImage. Base64 is an encoding method to transfer data. If you are not talking to the server there is no reason to use base64.
1
Hey Jon, my new best friend hehe. It seems that my error I get is from saving. I tried saving it as a string instead of data. Which seemed to save alright, however when I fetched my images they came out as blue squares instead of, well images. But at the moment, it won't save with the code above.
– Steffen L.
Feb 27 '17 at 11:28
Should I infact store the data as a String instead of Binary Data? Or?
– Steffen L.
Feb 27 '17 at 11:30
Hi Jon, I made the changes you suggested, however I am still getting the error on save. I have updated the code and also put the whole error log.
– Steffen L.
Feb 27 '17 at 16:27
Which means I can't even test the fetch, because it won't save.
– Steffen L.
Feb 27 '17 at 16:32
The error "Can't find or automatically infer mapping model for migration" means that you need to delete you app and reinstall.
– Jon Rose
Feb 28 '17 at 6:36
|
show 8 more comments
replace
let image: Data = images.value(forKey: "image")! as! Data
let dataDecoded : Data = Data(base64Encoded: image, options: )!
let decodedimage = UIImage(data: dataDecoded)
with
let image: Data = images.value(forKey: "image")! as! Data
let decodedimage = UIImage(data: image)
Base64 is a way to to convert data to a string. There is no reason to use it here. You already have the data from the database you just want to convert it to a UIImage.
also change
let image = data?.base64EncodedData()
saveImageToDB(brandName: imageBrandName, image: image!)
to
saveImageToDB(brandName: imageBrandName, image: data!)
base64EncodedData
is turning the data from image data into a utf-8 encoded based64encoded string. There is no reason for that.
You should get the base64 encoded string from server, convert it to data and then you never need base64 again. Read and write data to your database, and after you read it convert it to a UIImage. Base64 is an encoding method to transfer data. If you are not talking to the server there is no reason to use base64.
1
Hey Jon, my new best friend hehe. It seems that my error I get is from saving. I tried saving it as a string instead of data. Which seemed to save alright, however when I fetched my images they came out as blue squares instead of, well images. But at the moment, it won't save with the code above.
– Steffen L.
Feb 27 '17 at 11:28
Should I infact store the data as a String instead of Binary Data? Or?
– Steffen L.
Feb 27 '17 at 11:30
Hi Jon, I made the changes you suggested, however I am still getting the error on save. I have updated the code and also put the whole error log.
– Steffen L.
Feb 27 '17 at 16:27
Which means I can't even test the fetch, because it won't save.
– Steffen L.
Feb 27 '17 at 16:32
The error "Can't find or automatically infer mapping model for migration" means that you need to delete you app and reinstall.
– Jon Rose
Feb 28 '17 at 6:36
|
show 8 more comments
replace
let image: Data = images.value(forKey: "image")! as! Data
let dataDecoded : Data = Data(base64Encoded: image, options: )!
let decodedimage = UIImage(data: dataDecoded)
with
let image: Data = images.value(forKey: "image")! as! Data
let decodedimage = UIImage(data: image)
Base64 is a way to to convert data to a string. There is no reason to use it here. You already have the data from the database you just want to convert it to a UIImage.
also change
let image = data?.base64EncodedData()
saveImageToDB(brandName: imageBrandName, image: image!)
to
saveImageToDB(brandName: imageBrandName, image: data!)
base64EncodedData
is turning the data from image data into a utf-8 encoded based64encoded string. There is no reason for that.
You should get the base64 encoded string from server, convert it to data and then you never need base64 again. Read and write data to your database, and after you read it convert it to a UIImage. Base64 is an encoding method to transfer data. If you are not talking to the server there is no reason to use base64.
replace
let image: Data = images.value(forKey: "image")! as! Data
let dataDecoded : Data = Data(base64Encoded: image, options: )!
let decodedimage = UIImage(data: dataDecoded)
with
let image: Data = images.value(forKey: "image")! as! Data
let decodedimage = UIImage(data: image)
Base64 is a way to to convert data to a string. There is no reason to use it here. You already have the data from the database you just want to convert it to a UIImage.
also change
let image = data?.base64EncodedData()
saveImageToDB(brandName: imageBrandName, image: image!)
to
saveImageToDB(brandName: imageBrandName, image: data!)
base64EncodedData
is turning the data from image data into a utf-8 encoded based64encoded string. There is no reason for that.
You should get the base64 encoded string from server, convert it to data and then you never need base64 again. Read and write data to your database, and after you read it convert it to a UIImage. Base64 is an encoding method to transfer data. If you are not talking to the server there is no reason to use base64.
edited Feb 27 '17 at 12:07
answered Feb 27 '17 at 11:20
Jon RoseJon Rose
5,73711728
5,73711728
1
Hey Jon, my new best friend hehe. It seems that my error I get is from saving. I tried saving it as a string instead of data. Which seemed to save alright, however when I fetched my images they came out as blue squares instead of, well images. But at the moment, it won't save with the code above.
– Steffen L.
Feb 27 '17 at 11:28
Should I infact store the data as a String instead of Binary Data? Or?
– Steffen L.
Feb 27 '17 at 11:30
Hi Jon, I made the changes you suggested, however I am still getting the error on save. I have updated the code and also put the whole error log.
– Steffen L.
Feb 27 '17 at 16:27
Which means I can't even test the fetch, because it won't save.
– Steffen L.
Feb 27 '17 at 16:32
The error "Can't find or automatically infer mapping model for migration" means that you need to delete you app and reinstall.
– Jon Rose
Feb 28 '17 at 6:36
|
show 8 more comments
1
Hey Jon, my new best friend hehe. It seems that my error I get is from saving. I tried saving it as a string instead of data. Which seemed to save alright, however when I fetched my images they came out as blue squares instead of, well images. But at the moment, it won't save with the code above.
– Steffen L.
Feb 27 '17 at 11:28
Should I infact store the data as a String instead of Binary Data? Or?
– Steffen L.
Feb 27 '17 at 11:30
Hi Jon, I made the changes you suggested, however I am still getting the error on save. I have updated the code and also put the whole error log.
– Steffen L.
Feb 27 '17 at 16:27
Which means I can't even test the fetch, because it won't save.
– Steffen L.
Feb 27 '17 at 16:32
The error "Can't find or automatically infer mapping model for migration" means that you need to delete you app and reinstall.
– Jon Rose
Feb 28 '17 at 6:36
1
1
Hey Jon, my new best friend hehe. It seems that my error I get is from saving. I tried saving it as a string instead of data. Which seemed to save alright, however when I fetched my images they came out as blue squares instead of, well images. But at the moment, it won't save with the code above.
– Steffen L.
Feb 27 '17 at 11:28
Hey Jon, my new best friend hehe. It seems that my error I get is from saving. I tried saving it as a string instead of data. Which seemed to save alright, however when I fetched my images they came out as blue squares instead of, well images. But at the moment, it won't save with the code above.
– Steffen L.
Feb 27 '17 at 11:28
Should I infact store the data as a String instead of Binary Data? Or?
– Steffen L.
Feb 27 '17 at 11:30
Should I infact store the data as a String instead of Binary Data? Or?
– Steffen L.
Feb 27 '17 at 11:30
Hi Jon, I made the changes you suggested, however I am still getting the error on save. I have updated the code and also put the whole error log.
– Steffen L.
Feb 27 '17 at 16:27
Hi Jon, I made the changes you suggested, however I am still getting the error on save. I have updated the code and also put the whole error log.
– Steffen L.
Feb 27 '17 at 16:27
Which means I can't even test the fetch, because it won't save.
– Steffen L.
Feb 27 '17 at 16:32
Which means I can't even test the fetch, because it won't save.
– Steffen L.
Feb 27 '17 at 16:32
The error "Can't find or automatically infer mapping model for migration" means that you need to delete you app and reinstall.
– Jon Rose
Feb 28 '17 at 6:36
The error "Can't find or automatically infer mapping model for migration" means that you need to delete you app and reinstall.
– Jon Rose
Feb 28 '17 at 6:36
|
show 8 more comments
After the suggested corrections from Jon Rose all I needed was to add
.withRenderingMode(.alwaysOriginal)
to where I was showing my picture and the code worked.
add a comment |
After the suggested corrections from Jon Rose all I needed was to add
.withRenderingMode(.alwaysOriginal)
to where I was showing my picture and the code worked.
add a comment |
After the suggested corrections from Jon Rose all I needed was to add
.withRenderingMode(.alwaysOriginal)
to where I was showing my picture and the code worked.
After the suggested corrections from Jon Rose all I needed was to add
.withRenderingMode(.alwaysOriginal)
to where I was showing my picture and the code worked.
answered Mar 2 '17 at 9:31


Steffen L.Steffen L.
94213
94213
add a comment |
add a comment |
Saving Image:
guard let managedObjectContext = managedObjectContext else return
// Create User
let user = User(context: managedObjectContext)
// Configure User
user.name = "name"
user.about = "about"
user.address = "Address"
user.age = 30
if let img = UIImage(named: "dog.png")
let data = img.pngData() as NSData?
user.image = data
Fetching Image:
// Create Fetch Request
let fetchRequest: NSFetchRequest<User> = User.fetchRequest()
// Configure Fetch Request
fetchRequest.sortDescriptors = [NSSortDescriptor(key: "name", ascending: true)]
let users = try! managedContext.fetch(fetchRequest)
let user: User = users.first as! User
if let imageData = user?.image
imgView.image = UIImage(data: imageData as Data)
add a comment |
Saving Image:
guard let managedObjectContext = managedObjectContext else return
// Create User
let user = User(context: managedObjectContext)
// Configure User
user.name = "name"
user.about = "about"
user.address = "Address"
user.age = 30
if let img = UIImage(named: "dog.png")
let data = img.pngData() as NSData?
user.image = data
Fetching Image:
// Create Fetch Request
let fetchRequest: NSFetchRequest<User> = User.fetchRequest()
// Configure Fetch Request
fetchRequest.sortDescriptors = [NSSortDescriptor(key: "name", ascending: true)]
let users = try! managedContext.fetch(fetchRequest)
let user: User = users.first as! User
if let imageData = user?.image
imgView.image = UIImage(data: imageData as Data)
add a comment |
Saving Image:
guard let managedObjectContext = managedObjectContext else return
// Create User
let user = User(context: managedObjectContext)
// Configure User
user.name = "name"
user.about = "about"
user.address = "Address"
user.age = 30
if let img = UIImage(named: "dog.png")
let data = img.pngData() as NSData?
user.image = data
Fetching Image:
// Create Fetch Request
let fetchRequest: NSFetchRequest<User> = User.fetchRequest()
// Configure Fetch Request
fetchRequest.sortDescriptors = [NSSortDescriptor(key: "name", ascending: true)]
let users = try! managedContext.fetch(fetchRequest)
let user: User = users.first as! User
if let imageData = user?.image
imgView.image = UIImage(data: imageData as Data)
Saving Image:
guard let managedObjectContext = managedObjectContext else return
// Create User
let user = User(context: managedObjectContext)
// Configure User
user.name = "name"
user.about = "about"
user.address = "Address"
user.age = 30
if let img = UIImage(named: "dog.png")
let data = img.pngData() as NSData?
user.image = data
Fetching Image:
// Create Fetch Request
let fetchRequest: NSFetchRequest<User> = User.fetchRequest()
// Configure Fetch Request
fetchRequest.sortDescriptors = [NSSortDescriptor(key: "name", ascending: true)]
let users = try! managedContext.fetch(fetchRequest)
let user: User = users.first as! User
if let imageData = user?.image
imgView.image = UIImage(data: imageData as Data)
answered Nov 15 '18 at 6:02
Yogendra SinghYogendra Singh
25527
25527
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f42484089%2fswift-3-saving-images-to-core-data%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
w,l iBvZFV
2
Better approach would be storing images in
DocumentDirectory
orLibrary
and store the path to image in CoreData as string. Storing images (as Binary Data or Transformable) in CoreData will make encoding/decoding a heavy operation.– viral
Feb 27 '17 at 11:33