C# Winforms: Disable tracking of a binded EF object to avoid context updates till “Update” is pressed
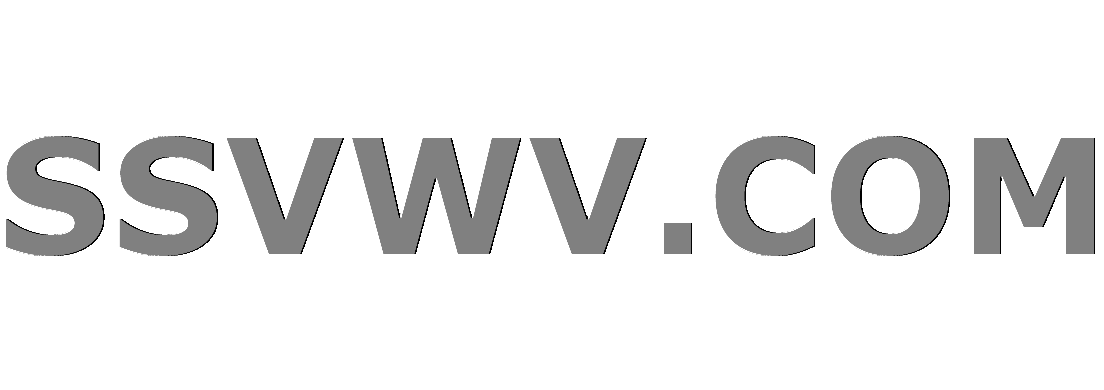
Multi tool use
up vote
0
down vote
favorite
So, I have a form with a list of categories binded to a DataGridView
, and when I double-click on one of the rows, a new form with details of the category is open, and also allow the user to edit or remove the selected category.
Here is the code of both forms:
FrmCategoryList.cs
public partial class FrmCategoryList : Form
private readonly DbContext dbContext;
public FrmCategoryList()
InitializeComponent();
dbContext = new DbContext();
private void FrmCategoryList_Load(object sender, EventArgs e)
FillGrid();
private void txtSearch_TextChanged(object sender, EventArgs e)
FillGrid();
private void dgvCategories_CellDoubleClick(object sender, DataGridViewCellEventArgs e)
var grid = (DataGridView)sender;
if (e.RowIndex >= 0)
var category = (Category)grid.Rows[e.RowIndex].DataBoundItem;
var frmCategoryView = new FrmCategoryView(category.Id, dbContext);
if (frmCategoryView.ShowDialog() == DialogResult.OK)
FillGrid();
private void btnNew_Click(object sender, EventArgs e)
var frmCategoryView = new FrmCategoryView(0, dbContext);
if (frmCategoryView.ShowDialog() == DialogResult.OK)
FillGrid();
private void btnClose_Click(object sender, EventArgs e)
Close();
private void FillGrid()
categoryBinding.DataSource = dbContext.Categories.Where(p => p.Description.StartsWith(txtSearch.Text)).ToList();
private void FrmCategoryList_FormClosing(object sender, FormClosingEventArgs e)
dbContext?.Dispose();
FrmCategoryView.cs
public partial class FrmCategoryView : Form
private readonly DbContext dbContext;
private Category category;
public FrmCategoryView(int categoryId, DbContext dbContext)
InitializeComponent();
this.dbContext = dbContext;
if (categoryId == 0)
category = new Category();
btnSave.Visible = true;
else
category = dbContexto.Categories.Include(c => c.Products).FirstOrDefault(c => c.Id == categoryId);
btnUpdate.Visible = true;
btnDelete.Visible = true;
private void FrmCategoryView_Load(object sender, EventArgs e)
txtDescription.DataBindings.Add("Text", category, "Description");
private void btnSave_Click(object sender, EventArgs e)
if (category.Validate(dbContext))
dbContext.Categories.Add(category);
dbContext.SaveChanges();
MessageBox.Show("Category added!", "Info", MessageBoxButtons.OK, MessageBoxIcon.Information);
DialogResult = DialogResult.OK;
Close();
private void btnUpdate_Click(object sender, EventArgs e)
if (category.Validate(dbContext))
dbContext.Categories.Attach(category);
dbContext.Entry(category).State = EntityState.Modified;
dbContext.SaveChanges();
MessageBox.Show("Category updated!", "Info", MessageBoxButtons.OK, MessageBoxIcon.Information);
DialogResult = DialogResult.OK;
Close();
private void btnDelete_Click(object sender, EventArgs e)
if (MessageBox.Show("¿Are you sure to delete this category?", "Confirmation", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes)
if (category.Products.Count > 0)
MessageBox.Show("You cannot delete a category with dependencies.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
dbContext.Categories.Remove(category);
dbContext.SaveChanges();
MessageBox.Show("Category deleted!.", "Info", MessageBoxButtons.OK, MessageBoxIcon.Information);
DialogResult = DialogResult.OK;
Close();
private void btnClose_Click(object sender, EventArgs e)
DialogResult = DialogResult.Cancel;
Close();
So, the problem comes when I edit some field of a category, and then close the form without pressing the Update button. The context isn't disposed until the FrmCategoryList
is closed, and the category object from FrmCategoryView
is binded to the text, so the context is updated but changes are not saved to the database. So, if I open other category and actually update it, the previous change will be saved too when calling SaveChanges()
because the context keep track of the changes that the user "discard".
How can I do to solve this? I could re-initialize the context in FrmCategoryView
, but then the changes will not be in FrmCategoryList
context in case I actually save something, and I don't know how to force EF to check database even for already tracked objects.
Also, maybe there is some way to detach the object from the context, and then re-attach it when Update button is pressed. I tried with AsNoTracking() but an error happens when trying to re-attach on Update: it's duplicated because an instance of that object was created in the list form.
Sorry for my bad english, I hope you understand!
EDIT:
Now I tried to set the Entity State of category to Detached right after getting it using the Id in FrmCategoryView
constructor, with:
dbContext.Entry(category).State = EntityState.Detached;
It doesn't work the first time I close the view form: the grid row get updated because the context is updated, even when the category
entry is set as Detached. But the next onces, it doesn't happend. It works like it should work. The changes I make to category
aren't tracked by the context until I re-attach it and save changes on Update button.
c# winforms entity-framework
add a comment |
up vote
0
down vote
favorite
So, I have a form with a list of categories binded to a DataGridView
, and when I double-click on one of the rows, a new form with details of the category is open, and also allow the user to edit or remove the selected category.
Here is the code of both forms:
FrmCategoryList.cs
public partial class FrmCategoryList : Form
private readonly DbContext dbContext;
public FrmCategoryList()
InitializeComponent();
dbContext = new DbContext();
private void FrmCategoryList_Load(object sender, EventArgs e)
FillGrid();
private void txtSearch_TextChanged(object sender, EventArgs e)
FillGrid();
private void dgvCategories_CellDoubleClick(object sender, DataGridViewCellEventArgs e)
var grid = (DataGridView)sender;
if (e.RowIndex >= 0)
var category = (Category)grid.Rows[e.RowIndex].DataBoundItem;
var frmCategoryView = new FrmCategoryView(category.Id, dbContext);
if (frmCategoryView.ShowDialog() == DialogResult.OK)
FillGrid();
private void btnNew_Click(object sender, EventArgs e)
var frmCategoryView = new FrmCategoryView(0, dbContext);
if (frmCategoryView.ShowDialog() == DialogResult.OK)
FillGrid();
private void btnClose_Click(object sender, EventArgs e)
Close();
private void FillGrid()
categoryBinding.DataSource = dbContext.Categories.Where(p => p.Description.StartsWith(txtSearch.Text)).ToList();
private void FrmCategoryList_FormClosing(object sender, FormClosingEventArgs e)
dbContext?.Dispose();
FrmCategoryView.cs
public partial class FrmCategoryView : Form
private readonly DbContext dbContext;
private Category category;
public FrmCategoryView(int categoryId, DbContext dbContext)
InitializeComponent();
this.dbContext = dbContext;
if (categoryId == 0)
category = new Category();
btnSave.Visible = true;
else
category = dbContexto.Categories.Include(c => c.Products).FirstOrDefault(c => c.Id == categoryId);
btnUpdate.Visible = true;
btnDelete.Visible = true;
private void FrmCategoryView_Load(object sender, EventArgs e)
txtDescription.DataBindings.Add("Text", category, "Description");
private void btnSave_Click(object sender, EventArgs e)
if (category.Validate(dbContext))
dbContext.Categories.Add(category);
dbContext.SaveChanges();
MessageBox.Show("Category added!", "Info", MessageBoxButtons.OK, MessageBoxIcon.Information);
DialogResult = DialogResult.OK;
Close();
private void btnUpdate_Click(object sender, EventArgs e)
if (category.Validate(dbContext))
dbContext.Categories.Attach(category);
dbContext.Entry(category).State = EntityState.Modified;
dbContext.SaveChanges();
MessageBox.Show("Category updated!", "Info", MessageBoxButtons.OK, MessageBoxIcon.Information);
DialogResult = DialogResult.OK;
Close();
private void btnDelete_Click(object sender, EventArgs e)
if (MessageBox.Show("¿Are you sure to delete this category?", "Confirmation", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes)
if (category.Products.Count > 0)
MessageBox.Show("You cannot delete a category with dependencies.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
dbContext.Categories.Remove(category);
dbContext.SaveChanges();
MessageBox.Show("Category deleted!.", "Info", MessageBoxButtons.OK, MessageBoxIcon.Information);
DialogResult = DialogResult.OK;
Close();
private void btnClose_Click(object sender, EventArgs e)
DialogResult = DialogResult.Cancel;
Close();
So, the problem comes when I edit some field of a category, and then close the form without pressing the Update button. The context isn't disposed until the FrmCategoryList
is closed, and the category object from FrmCategoryView
is binded to the text, so the context is updated but changes are not saved to the database. So, if I open other category and actually update it, the previous change will be saved too when calling SaveChanges()
because the context keep track of the changes that the user "discard".
How can I do to solve this? I could re-initialize the context in FrmCategoryView
, but then the changes will not be in FrmCategoryList
context in case I actually save something, and I don't know how to force EF to check database even for already tracked objects.
Also, maybe there is some way to detach the object from the context, and then re-attach it when Update button is pressed. I tried with AsNoTracking() but an error happens when trying to re-attach on Update: it's duplicated because an instance of that object was created in the list form.
Sorry for my bad english, I hope you understand!
EDIT:
Now I tried to set the Entity State of category to Detached right after getting it using the Id in FrmCategoryView
constructor, with:
dbContext.Entry(category).State = EntityState.Detached;
It doesn't work the first time I close the view form: the grid row get updated because the context is updated, even when the category
entry is set as Detached. But the next onces, it doesn't happend. It works like it should work. The changes I make to category
aren't tracked by the context until I re-attach it and save changes on Update button.
c# winforms entity-framework
Don't use the same instance of context for edit form.
– Reza Aghaei
Nov 11 at 0:17
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
So, I have a form with a list of categories binded to a DataGridView
, and when I double-click on one of the rows, a new form with details of the category is open, and also allow the user to edit or remove the selected category.
Here is the code of both forms:
FrmCategoryList.cs
public partial class FrmCategoryList : Form
private readonly DbContext dbContext;
public FrmCategoryList()
InitializeComponent();
dbContext = new DbContext();
private void FrmCategoryList_Load(object sender, EventArgs e)
FillGrid();
private void txtSearch_TextChanged(object sender, EventArgs e)
FillGrid();
private void dgvCategories_CellDoubleClick(object sender, DataGridViewCellEventArgs e)
var grid = (DataGridView)sender;
if (e.RowIndex >= 0)
var category = (Category)grid.Rows[e.RowIndex].DataBoundItem;
var frmCategoryView = new FrmCategoryView(category.Id, dbContext);
if (frmCategoryView.ShowDialog() == DialogResult.OK)
FillGrid();
private void btnNew_Click(object sender, EventArgs e)
var frmCategoryView = new FrmCategoryView(0, dbContext);
if (frmCategoryView.ShowDialog() == DialogResult.OK)
FillGrid();
private void btnClose_Click(object sender, EventArgs e)
Close();
private void FillGrid()
categoryBinding.DataSource = dbContext.Categories.Where(p => p.Description.StartsWith(txtSearch.Text)).ToList();
private void FrmCategoryList_FormClosing(object sender, FormClosingEventArgs e)
dbContext?.Dispose();
FrmCategoryView.cs
public partial class FrmCategoryView : Form
private readonly DbContext dbContext;
private Category category;
public FrmCategoryView(int categoryId, DbContext dbContext)
InitializeComponent();
this.dbContext = dbContext;
if (categoryId == 0)
category = new Category();
btnSave.Visible = true;
else
category = dbContexto.Categories.Include(c => c.Products).FirstOrDefault(c => c.Id == categoryId);
btnUpdate.Visible = true;
btnDelete.Visible = true;
private void FrmCategoryView_Load(object sender, EventArgs e)
txtDescription.DataBindings.Add("Text", category, "Description");
private void btnSave_Click(object sender, EventArgs e)
if (category.Validate(dbContext))
dbContext.Categories.Add(category);
dbContext.SaveChanges();
MessageBox.Show("Category added!", "Info", MessageBoxButtons.OK, MessageBoxIcon.Information);
DialogResult = DialogResult.OK;
Close();
private void btnUpdate_Click(object sender, EventArgs e)
if (category.Validate(dbContext))
dbContext.Categories.Attach(category);
dbContext.Entry(category).State = EntityState.Modified;
dbContext.SaveChanges();
MessageBox.Show("Category updated!", "Info", MessageBoxButtons.OK, MessageBoxIcon.Information);
DialogResult = DialogResult.OK;
Close();
private void btnDelete_Click(object sender, EventArgs e)
if (MessageBox.Show("¿Are you sure to delete this category?", "Confirmation", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes)
if (category.Products.Count > 0)
MessageBox.Show("You cannot delete a category with dependencies.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
dbContext.Categories.Remove(category);
dbContext.SaveChanges();
MessageBox.Show("Category deleted!.", "Info", MessageBoxButtons.OK, MessageBoxIcon.Information);
DialogResult = DialogResult.OK;
Close();
private void btnClose_Click(object sender, EventArgs e)
DialogResult = DialogResult.Cancel;
Close();
So, the problem comes when I edit some field of a category, and then close the form without pressing the Update button. The context isn't disposed until the FrmCategoryList
is closed, and the category object from FrmCategoryView
is binded to the text, so the context is updated but changes are not saved to the database. So, if I open other category and actually update it, the previous change will be saved too when calling SaveChanges()
because the context keep track of the changes that the user "discard".
How can I do to solve this? I could re-initialize the context in FrmCategoryView
, but then the changes will not be in FrmCategoryList
context in case I actually save something, and I don't know how to force EF to check database even for already tracked objects.
Also, maybe there is some way to detach the object from the context, and then re-attach it when Update button is pressed. I tried with AsNoTracking() but an error happens when trying to re-attach on Update: it's duplicated because an instance of that object was created in the list form.
Sorry for my bad english, I hope you understand!
EDIT:
Now I tried to set the Entity State of category to Detached right after getting it using the Id in FrmCategoryView
constructor, with:
dbContext.Entry(category).State = EntityState.Detached;
It doesn't work the first time I close the view form: the grid row get updated because the context is updated, even when the category
entry is set as Detached. But the next onces, it doesn't happend. It works like it should work. The changes I make to category
aren't tracked by the context until I re-attach it and save changes on Update button.
c# winforms entity-framework
So, I have a form with a list of categories binded to a DataGridView
, and when I double-click on one of the rows, a new form with details of the category is open, and also allow the user to edit or remove the selected category.
Here is the code of both forms:
FrmCategoryList.cs
public partial class FrmCategoryList : Form
private readonly DbContext dbContext;
public FrmCategoryList()
InitializeComponent();
dbContext = new DbContext();
private void FrmCategoryList_Load(object sender, EventArgs e)
FillGrid();
private void txtSearch_TextChanged(object sender, EventArgs e)
FillGrid();
private void dgvCategories_CellDoubleClick(object sender, DataGridViewCellEventArgs e)
var grid = (DataGridView)sender;
if (e.RowIndex >= 0)
var category = (Category)grid.Rows[e.RowIndex].DataBoundItem;
var frmCategoryView = new FrmCategoryView(category.Id, dbContext);
if (frmCategoryView.ShowDialog() == DialogResult.OK)
FillGrid();
private void btnNew_Click(object sender, EventArgs e)
var frmCategoryView = new FrmCategoryView(0, dbContext);
if (frmCategoryView.ShowDialog() == DialogResult.OK)
FillGrid();
private void btnClose_Click(object sender, EventArgs e)
Close();
private void FillGrid()
categoryBinding.DataSource = dbContext.Categories.Where(p => p.Description.StartsWith(txtSearch.Text)).ToList();
private void FrmCategoryList_FormClosing(object sender, FormClosingEventArgs e)
dbContext?.Dispose();
FrmCategoryView.cs
public partial class FrmCategoryView : Form
private readonly DbContext dbContext;
private Category category;
public FrmCategoryView(int categoryId, DbContext dbContext)
InitializeComponent();
this.dbContext = dbContext;
if (categoryId == 0)
category = new Category();
btnSave.Visible = true;
else
category = dbContexto.Categories.Include(c => c.Products).FirstOrDefault(c => c.Id == categoryId);
btnUpdate.Visible = true;
btnDelete.Visible = true;
private void FrmCategoryView_Load(object sender, EventArgs e)
txtDescription.DataBindings.Add("Text", category, "Description");
private void btnSave_Click(object sender, EventArgs e)
if (category.Validate(dbContext))
dbContext.Categories.Add(category);
dbContext.SaveChanges();
MessageBox.Show("Category added!", "Info", MessageBoxButtons.OK, MessageBoxIcon.Information);
DialogResult = DialogResult.OK;
Close();
private void btnUpdate_Click(object sender, EventArgs e)
if (category.Validate(dbContext))
dbContext.Categories.Attach(category);
dbContext.Entry(category).State = EntityState.Modified;
dbContext.SaveChanges();
MessageBox.Show("Category updated!", "Info", MessageBoxButtons.OK, MessageBoxIcon.Information);
DialogResult = DialogResult.OK;
Close();
private void btnDelete_Click(object sender, EventArgs e)
if (MessageBox.Show("¿Are you sure to delete this category?", "Confirmation", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes)
if (category.Products.Count > 0)
MessageBox.Show("You cannot delete a category with dependencies.", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
return;
dbContext.Categories.Remove(category);
dbContext.SaveChanges();
MessageBox.Show("Category deleted!.", "Info", MessageBoxButtons.OK, MessageBoxIcon.Information);
DialogResult = DialogResult.OK;
Close();
private void btnClose_Click(object sender, EventArgs e)
DialogResult = DialogResult.Cancel;
Close();
So, the problem comes when I edit some field of a category, and then close the form without pressing the Update button. The context isn't disposed until the FrmCategoryList
is closed, and the category object from FrmCategoryView
is binded to the text, so the context is updated but changes are not saved to the database. So, if I open other category and actually update it, the previous change will be saved too when calling SaveChanges()
because the context keep track of the changes that the user "discard".
How can I do to solve this? I could re-initialize the context in FrmCategoryView
, but then the changes will not be in FrmCategoryList
context in case I actually save something, and I don't know how to force EF to check database even for already tracked objects.
Also, maybe there is some way to detach the object from the context, and then re-attach it when Update button is pressed. I tried with AsNoTracking() but an error happens when trying to re-attach on Update: it's duplicated because an instance of that object was created in the list form.
Sorry for my bad english, I hope you understand!
EDIT:
Now I tried to set the Entity State of category to Detached right after getting it using the Id in FrmCategoryView
constructor, with:
dbContext.Entry(category).State = EntityState.Detached;
It doesn't work the first time I close the view form: the grid row get updated because the context is updated, even when the category
entry is set as Detached. But the next onces, it doesn't happend. It works like it should work. The changes I make to category
aren't tracked by the context until I re-attach it and save changes on Update button.
c# winforms entity-framework
c# winforms entity-framework
edited Nov 10 at 6:02
asked Nov 9 at 18:37
Julián Pera
4718
4718
Don't use the same instance of context for edit form.
– Reza Aghaei
Nov 11 at 0:17
add a comment |
Don't use the same instance of context for edit form.
– Reza Aghaei
Nov 11 at 0:17
Don't use the same instance of context for edit form.
– Reza Aghaei
Nov 11 at 0:17
Don't use the same instance of context for edit form.
– Reza Aghaei
Nov 11 at 0:17
add a comment |
2 Answers
2
active
oldest
votes
up vote
1
down vote
The problem here is you have used ShowDialog
. Read the second point in remarks section of below link
https://docs.microsoft.com/en-us/dotnet/api/system.windows.forms.form.close?view=netframework-4.7.2
In this scenario the form is not disposed.
you have displayed the form using ShowDialog. In these cases, you will
need to call Dispose manually to mark all of the form's controls for
garbage collection.
Or as you want Modal dialog, clean up the context by handling closing
event, if you do not have problem with other controls living till you close parent form.
Well, i didn't know that closing a form opened by ShowDialog() doesn't dispose it, but it's not whats causing the problem. Disposing the form do not discard the changes tracked by the context.
– Julián Pera
Nov 9 at 23:26
Yes. It will not, You have to take care of the sanity work, may be form closing event could be a better place.
– Naidu
Nov 10 at 0:31
But how? Check the edit I made to the original post.
– Julián Pera
Nov 10 at 1:16
Could you please try the same in form closing event??
– Naidu
Nov 10 at 1:24
Same result. I answered with a solution I found. Give it a look and tell me what you think.
– Julián Pera
Nov 10 at 6:21
add a comment |
up vote
0
down vote
I found a solution.
To recap, this are the changes I made to the original code:
- Added
AsNoTracking()
when filling the grid inFrmCategoryList
. - Detach
category
from the context after getting it using the id inFrmCategoryView
constructor. - Re-attach
category
before saving changes on Update and Delete, with Modified and Deleted states respectively.
After some tests, I didn't find any issue. Any suggestions are appreciated.
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
The problem here is you have used ShowDialog
. Read the second point in remarks section of below link
https://docs.microsoft.com/en-us/dotnet/api/system.windows.forms.form.close?view=netframework-4.7.2
In this scenario the form is not disposed.
you have displayed the form using ShowDialog. In these cases, you will
need to call Dispose manually to mark all of the form's controls for
garbage collection.
Or as you want Modal dialog, clean up the context by handling closing
event, if you do not have problem with other controls living till you close parent form.
Well, i didn't know that closing a form opened by ShowDialog() doesn't dispose it, but it's not whats causing the problem. Disposing the form do not discard the changes tracked by the context.
– Julián Pera
Nov 9 at 23:26
Yes. It will not, You have to take care of the sanity work, may be form closing event could be a better place.
– Naidu
Nov 10 at 0:31
But how? Check the edit I made to the original post.
– Julián Pera
Nov 10 at 1:16
Could you please try the same in form closing event??
– Naidu
Nov 10 at 1:24
Same result. I answered with a solution I found. Give it a look and tell me what you think.
– Julián Pera
Nov 10 at 6:21
add a comment |
up vote
1
down vote
The problem here is you have used ShowDialog
. Read the second point in remarks section of below link
https://docs.microsoft.com/en-us/dotnet/api/system.windows.forms.form.close?view=netframework-4.7.2
In this scenario the form is not disposed.
you have displayed the form using ShowDialog. In these cases, you will
need to call Dispose manually to mark all of the form's controls for
garbage collection.
Or as you want Modal dialog, clean up the context by handling closing
event, if you do not have problem with other controls living till you close parent form.
Well, i didn't know that closing a form opened by ShowDialog() doesn't dispose it, but it's not whats causing the problem. Disposing the form do not discard the changes tracked by the context.
– Julián Pera
Nov 9 at 23:26
Yes. It will not, You have to take care of the sanity work, may be form closing event could be a better place.
– Naidu
Nov 10 at 0:31
But how? Check the edit I made to the original post.
– Julián Pera
Nov 10 at 1:16
Could you please try the same in form closing event??
– Naidu
Nov 10 at 1:24
Same result. I answered with a solution I found. Give it a look and tell me what you think.
– Julián Pera
Nov 10 at 6:21
add a comment |
up vote
1
down vote
up vote
1
down vote
The problem here is you have used ShowDialog
. Read the second point in remarks section of below link
https://docs.microsoft.com/en-us/dotnet/api/system.windows.forms.form.close?view=netframework-4.7.2
In this scenario the form is not disposed.
you have displayed the form using ShowDialog. In these cases, you will
need to call Dispose manually to mark all of the form's controls for
garbage collection.
Or as you want Modal dialog, clean up the context by handling closing
event, if you do not have problem with other controls living till you close parent form.
The problem here is you have used ShowDialog
. Read the second point in remarks section of below link
https://docs.microsoft.com/en-us/dotnet/api/system.windows.forms.form.close?view=netframework-4.7.2
In this scenario the form is not disposed.
you have displayed the form using ShowDialog. In these cases, you will
need to call Dispose manually to mark all of the form's controls for
garbage collection.
Or as you want Modal dialog, clean up the context by handling closing
event, if you do not have problem with other controls living till you close parent form.
edited Nov 9 at 19:00
answered Nov 9 at 18:55


Naidu
4,7973817
4,7973817
Well, i didn't know that closing a form opened by ShowDialog() doesn't dispose it, but it's not whats causing the problem. Disposing the form do not discard the changes tracked by the context.
– Julián Pera
Nov 9 at 23:26
Yes. It will not, You have to take care of the sanity work, may be form closing event could be a better place.
– Naidu
Nov 10 at 0:31
But how? Check the edit I made to the original post.
– Julián Pera
Nov 10 at 1:16
Could you please try the same in form closing event??
– Naidu
Nov 10 at 1:24
Same result. I answered with a solution I found. Give it a look and tell me what you think.
– Julián Pera
Nov 10 at 6:21
add a comment |
Well, i didn't know that closing a form opened by ShowDialog() doesn't dispose it, but it's not whats causing the problem. Disposing the form do not discard the changes tracked by the context.
– Julián Pera
Nov 9 at 23:26
Yes. It will not, You have to take care of the sanity work, may be form closing event could be a better place.
– Naidu
Nov 10 at 0:31
But how? Check the edit I made to the original post.
– Julián Pera
Nov 10 at 1:16
Could you please try the same in form closing event??
– Naidu
Nov 10 at 1:24
Same result. I answered with a solution I found. Give it a look and tell me what you think.
– Julián Pera
Nov 10 at 6:21
Well, i didn't know that closing a form opened by ShowDialog() doesn't dispose it, but it's not whats causing the problem. Disposing the form do not discard the changes tracked by the context.
– Julián Pera
Nov 9 at 23:26
Well, i didn't know that closing a form opened by ShowDialog() doesn't dispose it, but it's not whats causing the problem. Disposing the form do not discard the changes tracked by the context.
– Julián Pera
Nov 9 at 23:26
Yes. It will not, You have to take care of the sanity work, may be form closing event could be a better place.
– Naidu
Nov 10 at 0:31
Yes. It will not, You have to take care of the sanity work, may be form closing event could be a better place.
– Naidu
Nov 10 at 0:31
But how? Check the edit I made to the original post.
– Julián Pera
Nov 10 at 1:16
But how? Check the edit I made to the original post.
– Julián Pera
Nov 10 at 1:16
Could you please try the same in form closing event??
– Naidu
Nov 10 at 1:24
Could you please try the same in form closing event??
– Naidu
Nov 10 at 1:24
Same result. I answered with a solution I found. Give it a look and tell me what you think.
– Julián Pera
Nov 10 at 6:21
Same result. I answered with a solution I found. Give it a look and tell me what you think.
– Julián Pera
Nov 10 at 6:21
add a comment |
up vote
0
down vote
I found a solution.
To recap, this are the changes I made to the original code:
- Added
AsNoTracking()
when filling the grid inFrmCategoryList
. - Detach
category
from the context after getting it using the id inFrmCategoryView
constructor. - Re-attach
category
before saving changes on Update and Delete, with Modified and Deleted states respectively.
After some tests, I didn't find any issue. Any suggestions are appreciated.
add a comment |
up vote
0
down vote
I found a solution.
To recap, this are the changes I made to the original code:
- Added
AsNoTracking()
when filling the grid inFrmCategoryList
. - Detach
category
from the context after getting it using the id inFrmCategoryView
constructor. - Re-attach
category
before saving changes on Update and Delete, with Modified and Deleted states respectively.
After some tests, I didn't find any issue. Any suggestions are appreciated.
add a comment |
up vote
0
down vote
up vote
0
down vote
I found a solution.
To recap, this are the changes I made to the original code:
- Added
AsNoTracking()
when filling the grid inFrmCategoryList
. - Detach
category
from the context after getting it using the id inFrmCategoryView
constructor. - Re-attach
category
before saving changes on Update and Delete, with Modified and Deleted states respectively.
After some tests, I didn't find any issue. Any suggestions are appreciated.
I found a solution.
To recap, this are the changes I made to the original code:
- Added
AsNoTracking()
when filling the grid inFrmCategoryList
. - Detach
category
from the context after getting it using the id inFrmCategoryView
constructor. - Re-attach
category
before saving changes on Update and Delete, with Modified and Deleted states respectively.
After some tests, I didn't find any issue. Any suggestions are appreciated.
edited Nov 10 at 6:19
answered Nov 10 at 6:02
Julián Pera
4718
4718
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53231560%2fc-sharp-winforms-disable-tracking-of-a-binded-ef-object-to-avoid-context-update%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
bomP664fPe6qK,jjwvLindNZFEJE
Don't use the same instance of context for edit form.
– Reza Aghaei
Nov 11 at 0:17