Reading file of 1's and 0's one at a time
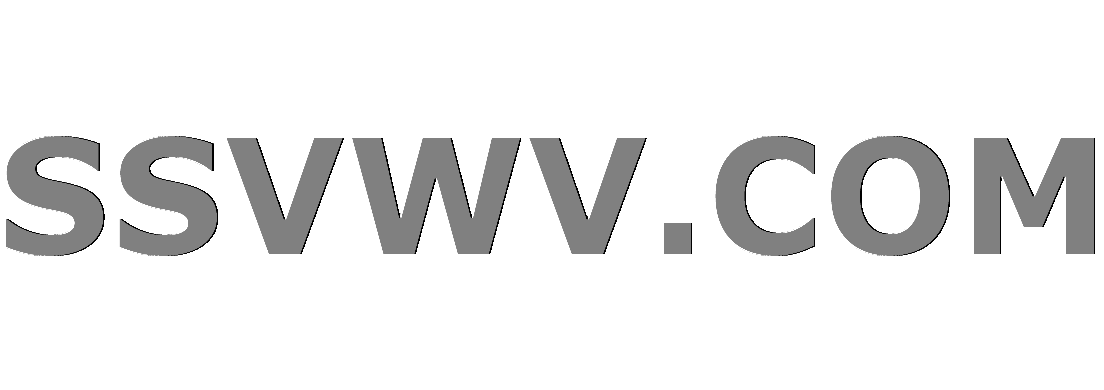
Multi tool use
up vote
0
down vote
favorite
In C++ I am trying to read from the following file into an array:
0000000000
0000000000
0000000000
0001110000
0001010000
0001110000
0000000000
0000000000
0000000000
0000000000
I am using the following to put each int into an array:
X = (int* )malloc(n*n*sizeof(int));
for (int i = 0; i<(n*n); i++)
j = read.get();
if (isdigit(j))
*(X+i) = j - '0';
But when I output to array to a file I get the following output:
0000000000
0000000000
0000000000
0000001110
0000000101
0000000011
1000000000
0000000000
0000000000
0000000000
And I don't believe there is anything wrong with how I am outputting my array:
for (int i = 0; i<(n*n); i++)
write << *(X+i);
if (((1+i) % n) == 0)
write << endl;
I tried reading with the following but it didn't seem to work:
for (int i = 0; i<(n*n); i++)
read >> *(X+i);
c++ file
add a comment |
up vote
0
down vote
favorite
In C++ I am trying to read from the following file into an array:
0000000000
0000000000
0000000000
0001110000
0001010000
0001110000
0000000000
0000000000
0000000000
0000000000
I am using the following to put each int into an array:
X = (int* )malloc(n*n*sizeof(int));
for (int i = 0; i<(n*n); i++)
j = read.get();
if (isdigit(j))
*(X+i) = j - '0';
But when I output to array to a file I get the following output:
0000000000
0000000000
0000000000
0000001110
0000000101
0000000011
1000000000
0000000000
0000000000
0000000000
And I don't believe there is anything wrong with how I am outputting my array:
for (int i = 0; i<(n*n); i++)
write << *(X+i);
if (((1+i) % n) == 0)
write << endl;
I tried reading with the following but it didn't seem to work:
for (int i = 0; i<(n*n); i++)
read >> *(X+i);
c++ file
2
Don't usemalloc()
in C++, usenew
. Even better, usestd::vector
instead of C-style arrays.
– Barmar
Nov 9 at 23:38
It is not recommended to usemalloc()
inC++
. You should generally usenew
or (better) a container likestd::vector
orstd::array
.
– Galik
Nov 9 at 23:38
The problem is in your read loop, on handling (or not handling) non-digits. The correspondingint
in the buffer is left uninitialised, but the writing code assumes something is stored there. The result (accessing an uninitialisedint
in the write loop) is undefined behaviour.
– Peter
Nov 9 at 23:42
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
In C++ I am trying to read from the following file into an array:
0000000000
0000000000
0000000000
0001110000
0001010000
0001110000
0000000000
0000000000
0000000000
0000000000
I am using the following to put each int into an array:
X = (int* )malloc(n*n*sizeof(int));
for (int i = 0; i<(n*n); i++)
j = read.get();
if (isdigit(j))
*(X+i) = j - '0';
But when I output to array to a file I get the following output:
0000000000
0000000000
0000000000
0000001110
0000000101
0000000011
1000000000
0000000000
0000000000
0000000000
And I don't believe there is anything wrong with how I am outputting my array:
for (int i = 0; i<(n*n); i++)
write << *(X+i);
if (((1+i) % n) == 0)
write << endl;
I tried reading with the following but it didn't seem to work:
for (int i = 0; i<(n*n); i++)
read >> *(X+i);
c++ file
In C++ I am trying to read from the following file into an array:
0000000000
0000000000
0000000000
0001110000
0001010000
0001110000
0000000000
0000000000
0000000000
0000000000
I am using the following to put each int into an array:
X = (int* )malloc(n*n*sizeof(int));
for (int i = 0; i<(n*n); i++)
j = read.get();
if (isdigit(j))
*(X+i) = j - '0';
But when I output to array to a file I get the following output:
0000000000
0000000000
0000000000
0000001110
0000000101
0000000011
1000000000
0000000000
0000000000
0000000000
And I don't believe there is anything wrong with how I am outputting my array:
for (int i = 0; i<(n*n); i++)
write << *(X+i);
if (((1+i) % n) == 0)
write << endl;
I tried reading with the following but it didn't seem to work:
for (int i = 0; i<(n*n); i++)
read >> *(X+i);
c++ file
c++ file
edited Nov 9 at 23:37


Galik
33.1k34674
33.1k34674
asked Nov 9 at 23:36
bob
91
91
2
Don't usemalloc()
in C++, usenew
. Even better, usestd::vector
instead of C-style arrays.
– Barmar
Nov 9 at 23:38
It is not recommended to usemalloc()
inC++
. You should generally usenew
or (better) a container likestd::vector
orstd::array
.
– Galik
Nov 9 at 23:38
The problem is in your read loop, on handling (or not handling) non-digits. The correspondingint
in the buffer is left uninitialised, but the writing code assumes something is stored there. The result (accessing an uninitialisedint
in the write loop) is undefined behaviour.
– Peter
Nov 9 at 23:42
add a comment |
2
Don't usemalloc()
in C++, usenew
. Even better, usestd::vector
instead of C-style arrays.
– Barmar
Nov 9 at 23:38
It is not recommended to usemalloc()
inC++
. You should generally usenew
or (better) a container likestd::vector
orstd::array
.
– Galik
Nov 9 at 23:38
The problem is in your read loop, on handling (or not handling) non-digits. The correspondingint
in the buffer is left uninitialised, but the writing code assumes something is stored there. The result (accessing an uninitialisedint
in the write loop) is undefined behaviour.
– Peter
Nov 9 at 23:42
2
2
Don't use
malloc()
in C++, use new
. Even better, use std::vector
instead of C-style arrays.– Barmar
Nov 9 at 23:38
Don't use
malloc()
in C++, use new
. Even better, use std::vector
instead of C-style arrays.– Barmar
Nov 9 at 23:38
It is not recommended to use
malloc()
in C++
. You should generally use new
or (better) a container like std::vector
or std::array
.– Galik
Nov 9 at 23:38
It is not recommended to use
malloc()
in C++
. You should generally use new
or (better) a container like std::vector
or std::array
.– Galik
Nov 9 at 23:38
The problem is in your read loop, on handling (or not handling) non-digits. The corresponding
int
in the buffer is left uninitialised, but the writing code assumes something is stored there. The result (accessing an uninitialised int
in the write loop) is undefined behaviour.– Peter
Nov 9 at 23:42
The problem is in your read loop, on handling (or not handling) non-digits. The corresponding
int
in the buffer is left uninitialised, but the writing code assumes something is stored there. The result (accessing an uninitialised int
in the write loop) is undefined behaviour.– Peter
Nov 9 at 23:42
add a comment |
1 Answer
1
active
oldest
votes
up vote
4
down vote
The problem is that you're incrementing i
even when isdigit(j)
is false. So you're leaving the array elements corresponding to the newlines in the file uninitialized, and also not reading all the digits because you're counting the newlines. You need to put the increment inside the if
.
for (int i = 0; i < n*n; )
char j = read.get();
if (isdigit(j))
X[i++] = j - '0';
BTW, if you're using a pointer as an array, use array syntax X[index]
rather than *(X+index)
. It makes the intent clearer.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
4
down vote
The problem is that you're incrementing i
even when isdigit(j)
is false. So you're leaving the array elements corresponding to the newlines in the file uninitialized, and also not reading all the digits because you're counting the newlines. You need to put the increment inside the if
.
for (int i = 0; i < n*n; )
char j = read.get();
if (isdigit(j))
X[i++] = j - '0';
BTW, if you're using a pointer as an array, use array syntax X[index]
rather than *(X+index)
. It makes the intent clearer.
add a comment |
up vote
4
down vote
The problem is that you're incrementing i
even when isdigit(j)
is false. So you're leaving the array elements corresponding to the newlines in the file uninitialized, and also not reading all the digits because you're counting the newlines. You need to put the increment inside the if
.
for (int i = 0; i < n*n; )
char j = read.get();
if (isdigit(j))
X[i++] = j - '0';
BTW, if you're using a pointer as an array, use array syntax X[index]
rather than *(X+index)
. It makes the intent clearer.
add a comment |
up vote
4
down vote
up vote
4
down vote
The problem is that you're incrementing i
even when isdigit(j)
is false. So you're leaving the array elements corresponding to the newlines in the file uninitialized, and also not reading all the digits because you're counting the newlines. You need to put the increment inside the if
.
for (int i = 0; i < n*n; )
char j = read.get();
if (isdigit(j))
X[i++] = j - '0';
BTW, if you're using a pointer as an array, use array syntax X[index]
rather than *(X+index)
. It makes the intent clearer.
The problem is that you're incrementing i
even when isdigit(j)
is false. So you're leaving the array elements corresponding to the newlines in the file uninitialized, and also not reading all the digits because you're counting the newlines. You need to put the increment inside the if
.
for (int i = 0; i < n*n; )
char j = read.get();
if (isdigit(j))
X[i++] = j - '0';
BTW, if you're using a pointer as an array, use array syntax X[index]
rather than *(X+index)
. It makes the intent clearer.
answered Nov 9 at 23:42
Barmar
413k34239339
413k34239339
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53234607%2freading-file-of-1s-and-0s-one-at-a-time%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
c7DgNC12brum 6I9PVOnj GMcAC6zQsQA AaPrNnnHTMButgd,ptr9
2
Don't use
malloc()
in C++, usenew
. Even better, usestd::vector
instead of C-style arrays.– Barmar
Nov 9 at 23:38
It is not recommended to use
malloc()
inC++
. You should generally usenew
or (better) a container likestd::vector
orstd::array
.– Galik
Nov 9 at 23:38
The problem is in your read loop, on handling (or not handling) non-digits. The corresponding
int
in the buffer is left uninitialised, but the writing code assumes something is stored there. The result (accessing an uninitialisedint
in the write loop) is undefined behaviour.– Peter
Nov 9 at 23:42