How to use an initializer_list to create a C++ of structures?
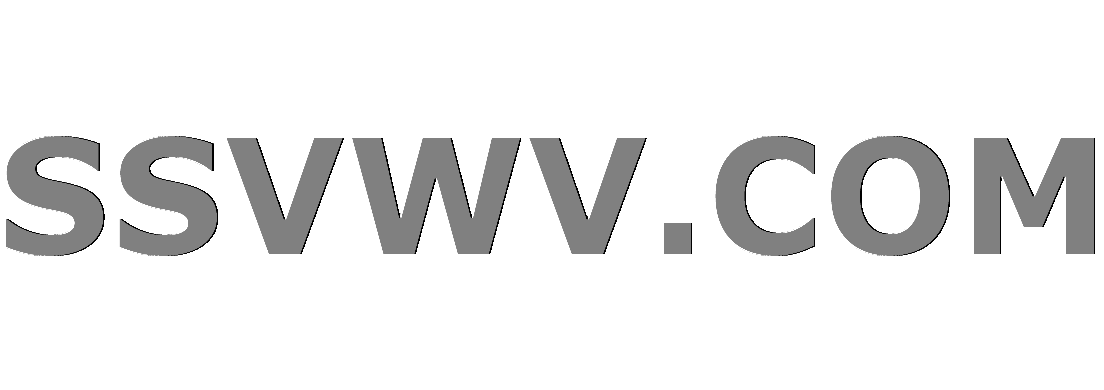
Multi tool use
up vote
1
down vote
favorite
The following code works as expected to initialize a vector of structs:
#include <array>
struct node
std::string name;
std::string value;
;
const std::vector<node> reqFields (
"query", tmpEmail ,
"firstname", firstName ,
"lastname", lastName
);
I want to optimize my code a bit to use a C++ 11 array instead, given that my data is static. However, the following won't compile:
const std::array<node, 3>(
"query", tmpEmail ,
"firstname", firstName ,
"lastname", lastName
);
What is the right syntax to initialize the array? or maybe this is something that Visual Studio 15 has trouble with?
c++ c++11 visual-c++
add a comment |
up vote
1
down vote
favorite
The following code works as expected to initialize a vector of structs:
#include <array>
struct node
std::string name;
std::string value;
;
const std::vector<node> reqFields (
"query", tmpEmail ,
"firstname", firstName ,
"lastname", lastName
);
I want to optimize my code a bit to use a C++ 11 array instead, given that my data is static. However, the following won't compile:
const std::array<node, 3>(
"query", tmpEmail ,
"firstname", firstName ,
"lastname", lastName
);
What is the right syntax to initialize the array? or maybe this is something that Visual Studio 15 has trouble with?
c++ c++11 visual-c++
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
The following code works as expected to initialize a vector of structs:
#include <array>
struct node
std::string name;
std::string value;
;
const std::vector<node> reqFields (
"query", tmpEmail ,
"firstname", firstName ,
"lastname", lastName
);
I want to optimize my code a bit to use a C++ 11 array instead, given that my data is static. However, the following won't compile:
const std::array<node, 3>(
"query", tmpEmail ,
"firstname", firstName ,
"lastname", lastName
);
What is the right syntax to initialize the array? or maybe this is something that Visual Studio 15 has trouble with?
c++ c++11 visual-c++
The following code works as expected to initialize a vector of structs:
#include <array>
struct node
std::string name;
std::string value;
;
const std::vector<node> reqFields (
"query", tmpEmail ,
"firstname", firstName ,
"lastname", lastName
);
I want to optimize my code a bit to use a C++ 11 array instead, given that my data is static. However, the following won't compile:
const std::array<node, 3>(
"query", tmpEmail ,
"firstname", firstName ,
"lastname", lastName
);
What is the right syntax to initialize the array? or maybe this is something that Visual Studio 15 has trouble with?
c++ c++11 visual-c++
c++ c++11 visual-c++
edited Nov 10 at 0:04
Shafik Yaghmour
123k23309511
123k23309511
asked Nov 9 at 23:56
ferchor2003
636
636
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
std::vector has a constructor that takes initializer_list
:
vector( std::initializer_list<T> init,
const Allocator& alloc = Allocator() );
but std::array is an aggregate and follows the rules of aggregate initialization .
So you need to switch from ()
to
const std::array<node, 3> reqFields
"query", "tmp" ,
"firstname", "firstName" ,
"lastname", "lastName"
;
see it live on godbolt.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
std::vector has a constructor that takes initializer_list
:
vector( std::initializer_list<T> init,
const Allocator& alloc = Allocator() );
but std::array is an aggregate and follows the rules of aggregate initialization .
So you need to switch from ()
to
const std::array<node, 3> reqFields
"query", "tmp" ,
"firstname", "firstName" ,
"lastname", "lastName"
;
see it live on godbolt.
add a comment |
up vote
2
down vote
accepted
std::vector has a constructor that takes initializer_list
:
vector( std::initializer_list<T> init,
const Allocator& alloc = Allocator() );
but std::array is an aggregate and follows the rules of aggregate initialization .
So you need to switch from ()
to
const std::array<node, 3> reqFields
"query", "tmp" ,
"firstname", "firstName" ,
"lastname", "lastName"
;
see it live on godbolt.
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
std::vector has a constructor that takes initializer_list
:
vector( std::initializer_list<T> init,
const Allocator& alloc = Allocator() );
but std::array is an aggregate and follows the rules of aggregate initialization .
So you need to switch from ()
to
const std::array<node, 3> reqFields
"query", "tmp" ,
"firstname", "firstName" ,
"lastname", "lastName"
;
see it live on godbolt.
std::vector has a constructor that takes initializer_list
:
vector( std::initializer_list<T> init,
const Allocator& alloc = Allocator() );
but std::array is an aggregate and follows the rules of aggregate initialization .
So you need to switch from ()
to
const std::array<node, 3> reqFields
"query", "tmp" ,
"firstname", "firstName" ,
"lastname", "lastName"
;
see it live on godbolt.
edited Nov 10 at 13:36
answered Nov 10 at 0:00
Shafik Yaghmour
123k23309511
123k23309511
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53234758%2fhow-to-use-an-initializer-list-to-create-a-c-array-of-structures%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8rbLaEepStrWPrMNRm7KWrvKjAysOVl5g4kT NqoZB8wCGP2otE jX0oTA19VjY40ROoHaw3PaI,VQplnMWv9d9NWv,0