How to add image to canvas
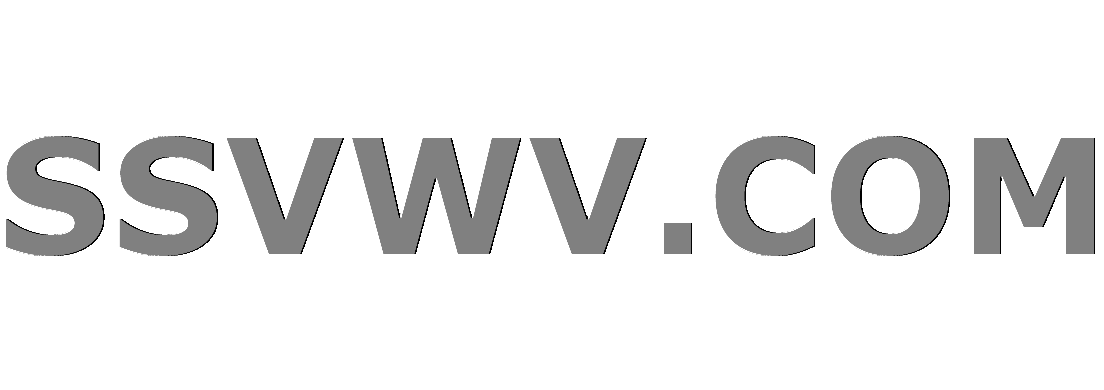
Multi tool use
up vote
82
down vote
favorite
I'm experimenting a bit with the new canvas element in HTML.
I simply want to add an image to the canvas but it doesn't work for some reason.
I have the following code:
HTML
<canvas id="viewport"></canvas>
CSS
canvas#viewport border: 1px solid white; width: 900px;
JS
var canvas = document.getElementById('viewport'),
context = canvas.getContext('2d');
make_base();
function make_base()
base_image = new Image();
base_image.src = 'img/base.png';
context.drawImage(base_image, 100, 100);
The image exists and I get no JavaScript errors. The image just doesn't display.
It must be something really simple I've missed...
javascript html5 canvas
add a comment |
up vote
82
down vote
favorite
I'm experimenting a bit with the new canvas element in HTML.
I simply want to add an image to the canvas but it doesn't work for some reason.
I have the following code:
HTML
<canvas id="viewport"></canvas>
CSS
canvas#viewport border: 1px solid white; width: 900px;
JS
var canvas = document.getElementById('viewport'),
context = canvas.getContext('2d');
make_base();
function make_base()
base_image = new Image();
base_image.src = 'img/base.png';
context.drawImage(base_image, 100, 100);
The image exists and I get no JavaScript errors. The image just doesn't display.
It must be something really simple I've missed...
javascript html5 canvas
add a comment |
up vote
82
down vote
favorite
up vote
82
down vote
favorite
I'm experimenting a bit with the new canvas element in HTML.
I simply want to add an image to the canvas but it doesn't work for some reason.
I have the following code:
HTML
<canvas id="viewport"></canvas>
CSS
canvas#viewport border: 1px solid white; width: 900px;
JS
var canvas = document.getElementById('viewport'),
context = canvas.getContext('2d');
make_base();
function make_base()
base_image = new Image();
base_image.src = 'img/base.png';
context.drawImage(base_image, 100, 100);
The image exists and I get no JavaScript errors. The image just doesn't display.
It must be something really simple I've missed...
javascript html5 canvas
I'm experimenting a bit with the new canvas element in HTML.
I simply want to add an image to the canvas but it doesn't work for some reason.
I have the following code:
HTML
<canvas id="viewport"></canvas>
CSS
canvas#viewport border: 1px solid white; width: 900px;
JS
var canvas = document.getElementById('viewport'),
context = canvas.getContext('2d');
make_base();
function make_base()
base_image = new Image();
base_image.src = 'img/base.png';
context.drawImage(base_image, 100, 100);
The image exists and I get no JavaScript errors. The image just doesn't display.
It must be something really simple I've missed...
javascript html5 canvas
javascript html5 canvas
edited Jun 30 '13 at 12:51
asked May 15 '11 at 21:28


PeeHaa
49.3k41165240
49.3k41165240
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
up vote
172
down vote
accepted
Maybe you should have to wait until the image is loaded before you draw it. Try this instead:
var canvas = document.getElementById('viewport'),
context = canvas.getContext('2d');
make_base();
function make_base()
base_image = new Image();
base_image.src = 'img/base.png';
base_image.onload = function()
context.drawImage(base_image, 0, 0);
I.e. draw the image in the onload callback of the image.
1
Will that work with images fetched over URL?
– swogger
Nov 13 '14 at 12:53
5
@swogger rather thank ask anything, try out. That worked perfectly. Make sure you check first the img size of the source, otherwise will be cropped unless you use full functioncontext.drawImage(base_image, 0, 0, 200, 200);
. That would draw base_img from 0px position, with draw area of 200x200px.
– erm3nda
Feb 16 '15 at 4:52
1
This was exactly my case. I was loading some blob data into canvas usingnew Image
and wondering why it was always showing me a previous image. Turns out even if I'm loading an image from a variable I still have to wait for theonload
to happen. Thank you!
– aexl
Mar 16 '16 at 22:27
How to add image style ?
– Sagar Rawal
Sep 20 '16 at 12:55
add a comment |
up vote
1
down vote
here is the sample code to draw image on canvas-
$("#selectedImage").change(function(e)
var URL = window.URL;
var url = URL.createObjectURL(e.target.files[0]);
img.src = url;
img.onload = function()
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.drawImage(img, 0, 0, 500, 500);
);
In the above code selectedImage is an input control which can be used to browse image on system.
For more details of sample code to draw image on canvas while maintaining the aspect ratio:
http://newapputil.blogspot.in/2016/09/show-image-on-canvas-html5.html
add a comment |
up vote
1
down vote
In my case, I was mistaken the function parameters, which are:
context.drawImage(image, left, top);
context.drawImage(image, left, top, width, height);
If you expect them to be
context.drawImage(image, width, height);
you will place the image just outside the canvas with the same effects as described in the question.
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
172
down vote
accepted
Maybe you should have to wait until the image is loaded before you draw it. Try this instead:
var canvas = document.getElementById('viewport'),
context = canvas.getContext('2d');
make_base();
function make_base()
base_image = new Image();
base_image.src = 'img/base.png';
base_image.onload = function()
context.drawImage(base_image, 0, 0);
I.e. draw the image in the onload callback of the image.
1
Will that work with images fetched over URL?
– swogger
Nov 13 '14 at 12:53
5
@swogger rather thank ask anything, try out. That worked perfectly. Make sure you check first the img size of the source, otherwise will be cropped unless you use full functioncontext.drawImage(base_image, 0, 0, 200, 200);
. That would draw base_img from 0px position, with draw area of 200x200px.
– erm3nda
Feb 16 '15 at 4:52
1
This was exactly my case. I was loading some blob data into canvas usingnew Image
and wondering why it was always showing me a previous image. Turns out even if I'm loading an image from a variable I still have to wait for theonload
to happen. Thank you!
– aexl
Mar 16 '16 at 22:27
How to add image style ?
– Sagar Rawal
Sep 20 '16 at 12:55
add a comment |
up vote
172
down vote
accepted
Maybe you should have to wait until the image is loaded before you draw it. Try this instead:
var canvas = document.getElementById('viewport'),
context = canvas.getContext('2d');
make_base();
function make_base()
base_image = new Image();
base_image.src = 'img/base.png';
base_image.onload = function()
context.drawImage(base_image, 0, 0);
I.e. draw the image in the onload callback of the image.
1
Will that work with images fetched over URL?
– swogger
Nov 13 '14 at 12:53
5
@swogger rather thank ask anything, try out. That worked perfectly. Make sure you check first the img size of the source, otherwise will be cropped unless you use full functioncontext.drawImage(base_image, 0, 0, 200, 200);
. That would draw base_img from 0px position, with draw area of 200x200px.
– erm3nda
Feb 16 '15 at 4:52
1
This was exactly my case. I was loading some blob data into canvas usingnew Image
and wondering why it was always showing me a previous image. Turns out even if I'm loading an image from a variable I still have to wait for theonload
to happen. Thank you!
– aexl
Mar 16 '16 at 22:27
How to add image style ?
– Sagar Rawal
Sep 20 '16 at 12:55
add a comment |
up vote
172
down vote
accepted
up vote
172
down vote
accepted
Maybe you should have to wait until the image is loaded before you draw it. Try this instead:
var canvas = document.getElementById('viewport'),
context = canvas.getContext('2d');
make_base();
function make_base()
base_image = new Image();
base_image.src = 'img/base.png';
base_image.onload = function()
context.drawImage(base_image, 0, 0);
I.e. draw the image in the onload callback of the image.
Maybe you should have to wait until the image is loaded before you draw it. Try this instead:
var canvas = document.getElementById('viewport'),
context = canvas.getContext('2d');
make_base();
function make_base()
base_image = new Image();
base_image.src = 'img/base.png';
base_image.onload = function()
context.drawImage(base_image, 0, 0);
I.e. draw the image in the onload callback of the image.
edited Feb 15 at 21:38
answered May 15 '11 at 21:32
Thomas
6,62242032
6,62242032
1
Will that work with images fetched over URL?
– swogger
Nov 13 '14 at 12:53
5
@swogger rather thank ask anything, try out. That worked perfectly. Make sure you check first the img size of the source, otherwise will be cropped unless you use full functioncontext.drawImage(base_image, 0, 0, 200, 200);
. That would draw base_img from 0px position, with draw area of 200x200px.
– erm3nda
Feb 16 '15 at 4:52
1
This was exactly my case. I was loading some blob data into canvas usingnew Image
and wondering why it was always showing me a previous image. Turns out even if I'm loading an image from a variable I still have to wait for theonload
to happen. Thank you!
– aexl
Mar 16 '16 at 22:27
How to add image style ?
– Sagar Rawal
Sep 20 '16 at 12:55
add a comment |
1
Will that work with images fetched over URL?
– swogger
Nov 13 '14 at 12:53
5
@swogger rather thank ask anything, try out. That worked perfectly. Make sure you check first the img size of the source, otherwise will be cropped unless you use full functioncontext.drawImage(base_image, 0, 0, 200, 200);
. That would draw base_img from 0px position, with draw area of 200x200px.
– erm3nda
Feb 16 '15 at 4:52
1
This was exactly my case. I was loading some blob data into canvas usingnew Image
and wondering why it was always showing me a previous image. Turns out even if I'm loading an image from a variable I still have to wait for theonload
to happen. Thank you!
– aexl
Mar 16 '16 at 22:27
How to add image style ?
– Sagar Rawal
Sep 20 '16 at 12:55
1
1
Will that work with images fetched over URL?
– swogger
Nov 13 '14 at 12:53
Will that work with images fetched over URL?
– swogger
Nov 13 '14 at 12:53
5
5
@swogger rather thank ask anything, try out. That worked perfectly. Make sure you check first the img size of the source, otherwise will be cropped unless you use full function
context.drawImage(base_image, 0, 0, 200, 200);
. That would draw base_img from 0px position, with draw area of 200x200px.– erm3nda
Feb 16 '15 at 4:52
@swogger rather thank ask anything, try out. That worked perfectly. Make sure you check first the img size of the source, otherwise will be cropped unless you use full function
context.drawImage(base_image, 0, 0, 200, 200);
. That would draw base_img from 0px position, with draw area of 200x200px.– erm3nda
Feb 16 '15 at 4:52
1
1
This was exactly my case. I was loading some blob data into canvas using
new Image
and wondering why it was always showing me a previous image. Turns out even if I'm loading an image from a variable I still have to wait for the onload
to happen. Thank you!– aexl
Mar 16 '16 at 22:27
This was exactly my case. I was loading some blob data into canvas using
new Image
and wondering why it was always showing me a previous image. Turns out even if I'm loading an image from a variable I still have to wait for the onload
to happen. Thank you!– aexl
Mar 16 '16 at 22:27
How to add image style ?
– Sagar Rawal
Sep 20 '16 at 12:55
How to add image style ?
– Sagar Rawal
Sep 20 '16 at 12:55
add a comment |
up vote
1
down vote
here is the sample code to draw image on canvas-
$("#selectedImage").change(function(e)
var URL = window.URL;
var url = URL.createObjectURL(e.target.files[0]);
img.src = url;
img.onload = function()
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.drawImage(img, 0, 0, 500, 500);
);
In the above code selectedImage is an input control which can be used to browse image on system.
For more details of sample code to draw image on canvas while maintaining the aspect ratio:
http://newapputil.blogspot.in/2016/09/show-image-on-canvas-html5.html
add a comment |
up vote
1
down vote
here is the sample code to draw image on canvas-
$("#selectedImage").change(function(e)
var URL = window.URL;
var url = URL.createObjectURL(e.target.files[0]);
img.src = url;
img.onload = function()
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.drawImage(img, 0, 0, 500, 500);
);
In the above code selectedImage is an input control which can be used to browse image on system.
For more details of sample code to draw image on canvas while maintaining the aspect ratio:
http://newapputil.blogspot.in/2016/09/show-image-on-canvas-html5.html
add a comment |
up vote
1
down vote
up vote
1
down vote
here is the sample code to draw image on canvas-
$("#selectedImage").change(function(e)
var URL = window.URL;
var url = URL.createObjectURL(e.target.files[0]);
img.src = url;
img.onload = function()
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.drawImage(img, 0, 0, 500, 500);
);
In the above code selectedImage is an input control which can be used to browse image on system.
For more details of sample code to draw image on canvas while maintaining the aspect ratio:
http://newapputil.blogspot.in/2016/09/show-image-on-canvas-html5.html
here is the sample code to draw image on canvas-
$("#selectedImage").change(function(e)
var URL = window.URL;
var url = URL.createObjectURL(e.target.files[0]);
img.src = url;
img.onload = function()
var canvas = document.getElementById("myCanvas");
var ctx = canvas.getContext("2d");
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.drawImage(img, 0, 0, 500, 500);
);
In the above code selectedImage is an input control which can be used to browse image on system.
For more details of sample code to draw image on canvas while maintaining the aspect ratio:
http://newapputil.blogspot.in/2016/09/show-image-on-canvas-html5.html
edited Nov 3 '16 at 9:29
answered Nov 3 '16 at 8:48


nvivekgoyal
167313
167313
add a comment |
add a comment |
up vote
1
down vote
In my case, I was mistaken the function parameters, which are:
context.drawImage(image, left, top);
context.drawImage(image, left, top, width, height);
If you expect them to be
context.drawImage(image, width, height);
you will place the image just outside the canvas with the same effects as described in the question.
add a comment |
up vote
1
down vote
In my case, I was mistaken the function parameters, which are:
context.drawImage(image, left, top);
context.drawImage(image, left, top, width, height);
If you expect them to be
context.drawImage(image, width, height);
you will place the image just outside the canvas with the same effects as described in the question.
add a comment |
up vote
1
down vote
up vote
1
down vote
In my case, I was mistaken the function parameters, which are:
context.drawImage(image, left, top);
context.drawImage(image, left, top, width, height);
If you expect them to be
context.drawImage(image, width, height);
you will place the image just outside the canvas with the same effects as described in the question.
In my case, I was mistaken the function parameters, which are:
context.drawImage(image, left, top);
context.drawImage(image, left, top, width, height);
If you expect them to be
context.drawImage(image, width, height);
you will place the image just outside the canvas with the same effects as described in the question.
answered Nov 7 '17 at 10:08


Marc
8,34923270
8,34923270
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f6011378%2fhow-to-add-image-to-canvas%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
AjV,p eVt EkIrVn2x0qA,aGDiiOOjkH,l1Bc70CT 3miPXt,z,9O5zAim WK,uz3Sq2FN6dY 4imDG6w2ov5kiBG