The method toString() in the type Object is not applicable for the arguments (Collection)
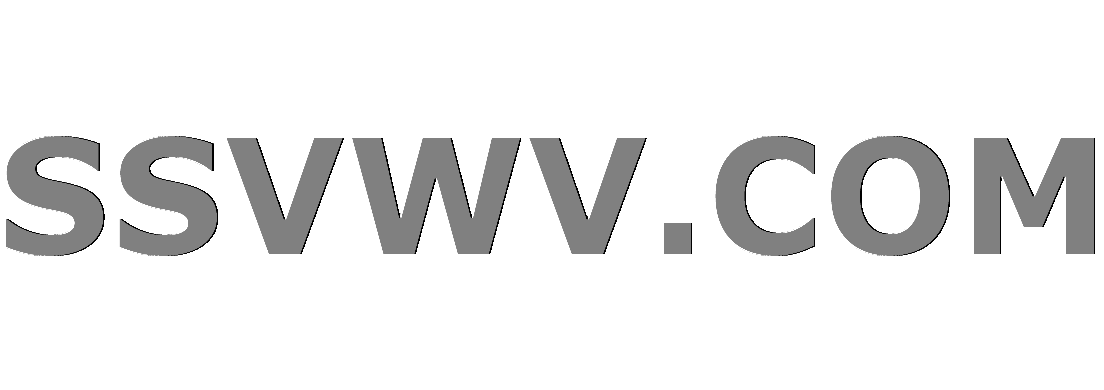
Multi tool use
up vote
-1
down vote
favorite
I'm new to Java and I am trying to write a description class that will return an array of strings from calling describe through my interface.
this line: return Collections.toString(items);
is throwing the error in the title, and I can't understand why.
I know that I need to return type string and that items are not currently a string but I'm new to Java and not sure what to change.
trace error when run: java.lang.Error: Unresolved compilation problem:
The return type is incompatible with Describe.describe()
package uk.ac.uos.assignment;
import java.util.*;
public class Description implements Describe
private Collection<Describe> items;
public Description()
this.items = new ArrayList<>();
public String describe()
return Collections.toString(items);
public void add(Describe d)
items.add(d);
and this is my interface:
package uk.ac.uos.assignment;
interface Describe
String describe();
java
add a comment |
up vote
-1
down vote
favorite
I'm new to Java and I am trying to write a description class that will return an array of strings from calling describe through my interface.
this line: return Collections.toString(items);
is throwing the error in the title, and I can't understand why.
I know that I need to return type string and that items are not currently a string but I'm new to Java and not sure what to change.
trace error when run: java.lang.Error: Unresolved compilation problem:
The return type is incompatible with Describe.describe()
package uk.ac.uos.assignment;
import java.util.*;
public class Description implements Describe
private Collection<Describe> items;
public Description()
this.items = new ArrayList<>();
public String describe()
return Collections.toString(items);
public void add(Describe d)
items.add(d);
and this is my interface:
package uk.ac.uos.assignment;
interface Describe
String describe();
java
Hi Brennan, can you please add the exception stack trace you are getting?
– shriyog
Nov 10 at 15:24
Collections has no static method toString, so the method you are trying to call in your describe function, does not really exist. If you call it as a Object function (return items.toString();), you will get rid of your error, but it isn't still doing what you want.
– Axel M
Nov 10 at 15:50
Please also add your Decribe Interface
– Axel M
Nov 10 at 16:07
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
I'm new to Java and I am trying to write a description class that will return an array of strings from calling describe through my interface.
this line: return Collections.toString(items);
is throwing the error in the title, and I can't understand why.
I know that I need to return type string and that items are not currently a string but I'm new to Java and not sure what to change.
trace error when run: java.lang.Error: Unresolved compilation problem:
The return type is incompatible with Describe.describe()
package uk.ac.uos.assignment;
import java.util.*;
public class Description implements Describe
private Collection<Describe> items;
public Description()
this.items = new ArrayList<>();
public String describe()
return Collections.toString(items);
public void add(Describe d)
items.add(d);
and this is my interface:
package uk.ac.uos.assignment;
interface Describe
String describe();
java
I'm new to Java and I am trying to write a description class that will return an array of strings from calling describe through my interface.
this line: return Collections.toString(items);
is throwing the error in the title, and I can't understand why.
I know that I need to return type string and that items are not currently a string but I'm new to Java and not sure what to change.
trace error when run: java.lang.Error: Unresolved compilation problem:
The return type is incompatible with Describe.describe()
package uk.ac.uos.assignment;
import java.util.*;
public class Description implements Describe
private Collection<Describe> items;
public Description()
this.items = new ArrayList<>();
public String describe()
return Collections.toString(items);
public void add(Describe d)
items.add(d);
and this is my interface:
package uk.ac.uos.assignment;
interface Describe
String describe();
java
java
edited Nov 10 at 16:17
asked Nov 10 at 15:12
Brennan Minns
12
12
Hi Brennan, can you please add the exception stack trace you are getting?
– shriyog
Nov 10 at 15:24
Collections has no static method toString, so the method you are trying to call in your describe function, does not really exist. If you call it as a Object function (return items.toString();), you will get rid of your error, but it isn't still doing what you want.
– Axel M
Nov 10 at 15:50
Please also add your Decribe Interface
– Axel M
Nov 10 at 16:07
add a comment |
Hi Brennan, can you please add the exception stack trace you are getting?
– shriyog
Nov 10 at 15:24
Collections has no static method toString, so the method you are trying to call in your describe function, does not really exist. If you call it as a Object function (return items.toString();), you will get rid of your error, but it isn't still doing what you want.
– Axel M
Nov 10 at 15:50
Please also add your Decribe Interface
– Axel M
Nov 10 at 16:07
Hi Brennan, can you please add the exception stack trace you are getting?
– shriyog
Nov 10 at 15:24
Hi Brennan, can you please add the exception stack trace you are getting?
– shriyog
Nov 10 at 15:24
Collections has no static method toString, so the method you are trying to call in your describe function, does not really exist. If you call it as a Object function (return items.toString();), you will get rid of your error, but it isn't still doing what you want.
– Axel M
Nov 10 at 15:50
Collections has no static method toString, so the method you are trying to call in your describe function, does not really exist. If you call it as a Object function (return items.toString();), you will get rid of your error, but it isn't still doing what you want.
– Axel M
Nov 10 at 15:50
Please also add your Decribe Interface
– Axel M
Nov 10 at 16:07
Please also add your Decribe Interface
– Axel M
Nov 10 at 16:07
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
Now, in the "describe()" method, I would suggest you do the following:
1) Create an empty String;
2) Iterate through the Collection and add every element, as a string, to the empty string you have created;
3) Return the String.
The basic algorithm I described above, now here's somewhat of an implementation:
public String describe()
StringBuilder y = new StringBuilder();
items.forEach(i -> y.append(i.toString()));
return y.toString();
Note: For this to work, your project must be set to use Java 8 or newer. If it is not, you will need to do a classical iteration through the Collection and then append each element to the StringBuilder.
Note 2: Your "Description" class must have its "toString()" method implemented. Before implementing it though, you should use an @Override annotation, just above the method:
@Override
public String toString()...
add a comment |
up vote
0
down vote
What I understand is that you want a String
array returning the String
representation of each of those items in your Collection<Describe> items
.
Iterate over the collection and call the toString()
method of every Describe
item provided that the Describe
class has its own implementation of toString
.
This would get you a String for each of those items in your collection, collect them and return at the end.
public String describe()
List<String> descriptions = new ArrayList<String>(items.size());
for(Describe item: items)
descriptions.add(item.toString());
return descriptions.toArray(new String[items.size()]);
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Now, in the "describe()" method, I would suggest you do the following:
1) Create an empty String;
2) Iterate through the Collection and add every element, as a string, to the empty string you have created;
3) Return the String.
The basic algorithm I described above, now here's somewhat of an implementation:
public String describe()
StringBuilder y = new StringBuilder();
items.forEach(i -> y.append(i.toString()));
return y.toString();
Note: For this to work, your project must be set to use Java 8 or newer. If it is not, you will need to do a classical iteration through the Collection and then append each element to the StringBuilder.
Note 2: Your "Description" class must have its "toString()" method implemented. Before implementing it though, you should use an @Override annotation, just above the method:
@Override
public String toString()...
add a comment |
up vote
0
down vote
Now, in the "describe()" method, I would suggest you do the following:
1) Create an empty String;
2) Iterate through the Collection and add every element, as a string, to the empty string you have created;
3) Return the String.
The basic algorithm I described above, now here's somewhat of an implementation:
public String describe()
StringBuilder y = new StringBuilder();
items.forEach(i -> y.append(i.toString()));
return y.toString();
Note: For this to work, your project must be set to use Java 8 or newer. If it is not, you will need to do a classical iteration through the Collection and then append each element to the StringBuilder.
Note 2: Your "Description" class must have its "toString()" method implemented. Before implementing it though, you should use an @Override annotation, just above the method:
@Override
public String toString()...
add a comment |
up vote
0
down vote
up vote
0
down vote
Now, in the "describe()" method, I would suggest you do the following:
1) Create an empty String;
2) Iterate through the Collection and add every element, as a string, to the empty string you have created;
3) Return the String.
The basic algorithm I described above, now here's somewhat of an implementation:
public String describe()
StringBuilder y = new StringBuilder();
items.forEach(i -> y.append(i.toString()));
return y.toString();
Note: For this to work, your project must be set to use Java 8 or newer. If it is not, you will need to do a classical iteration through the Collection and then append each element to the StringBuilder.
Note 2: Your "Description" class must have its "toString()" method implemented. Before implementing it though, you should use an @Override annotation, just above the method:
@Override
public String toString()...
Now, in the "describe()" method, I would suggest you do the following:
1) Create an empty String;
2) Iterate through the Collection and add every element, as a string, to the empty string you have created;
3) Return the String.
The basic algorithm I described above, now here's somewhat of an implementation:
public String describe()
StringBuilder y = new StringBuilder();
items.forEach(i -> y.append(i.toString()));
return y.toString();
Note: For this to work, your project must be set to use Java 8 or newer. If it is not, you will need to do a classical iteration through the Collection and then append each element to the StringBuilder.
Note 2: Your "Description" class must have its "toString()" method implemented. Before implementing it though, you should use an @Override annotation, just above the method:
@Override
public String toString()...
edited Nov 10 at 15:47
answered Nov 10 at 15:26


Rakirnd
714
714
add a comment |
add a comment |
up vote
0
down vote
What I understand is that you want a String
array returning the String
representation of each of those items in your Collection<Describe> items
.
Iterate over the collection and call the toString()
method of every Describe
item provided that the Describe
class has its own implementation of toString
.
This would get you a String for each of those items in your collection, collect them and return at the end.
public String describe()
List<String> descriptions = new ArrayList<String>(items.size());
for(Describe item: items)
descriptions.add(item.toString());
return descriptions.toArray(new String[items.size()]);
add a comment |
up vote
0
down vote
What I understand is that you want a String
array returning the String
representation of each of those items in your Collection<Describe> items
.
Iterate over the collection and call the toString()
method of every Describe
item provided that the Describe
class has its own implementation of toString
.
This would get you a String for each of those items in your collection, collect them and return at the end.
public String describe()
List<String> descriptions = new ArrayList<String>(items.size());
for(Describe item: items)
descriptions.add(item.toString());
return descriptions.toArray(new String[items.size()]);
add a comment |
up vote
0
down vote
up vote
0
down vote
What I understand is that you want a String
array returning the String
representation of each of those items in your Collection<Describe> items
.
Iterate over the collection and call the toString()
method of every Describe
item provided that the Describe
class has its own implementation of toString
.
This would get you a String for each of those items in your collection, collect them and return at the end.
public String describe()
List<String> descriptions = new ArrayList<String>(items.size());
for(Describe item: items)
descriptions.add(item.toString());
return descriptions.toArray(new String[items.size()]);
What I understand is that you want a String
array returning the String
representation of each of those items in your Collection<Describe> items
.
Iterate over the collection and call the toString()
method of every Describe
item provided that the Describe
class has its own implementation of toString
.
This would get you a String for each of those items in your collection, collect them and return at the end.
public String describe()
List<String> descriptions = new ArrayList<String>(items.size());
for(Describe item: items)
descriptions.add(item.toString());
return descriptions.toArray(new String[items.size()]);
edited Nov 10 at 15:49
answered Nov 10 at 15:15


shriyog
426616
426616
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53240285%2fthe-method-tostring-in-the-type-object-is-not-applicable-for-the-arguments-co%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
RVUjmnsD6T yUaTTk
Hi Brennan, can you please add the exception stack trace you are getting?
– shriyog
Nov 10 at 15:24
Collections has no static method toString, so the method you are trying to call in your describe function, does not really exist. If you call it as a Object function (return items.toString();), you will get rid of your error, but it isn't still doing what you want.
– Axel M
Nov 10 at 15:50
Please also add your Decribe Interface
– Axel M
Nov 10 at 16:07