UIBezierPath - draw multiple rects for a single path
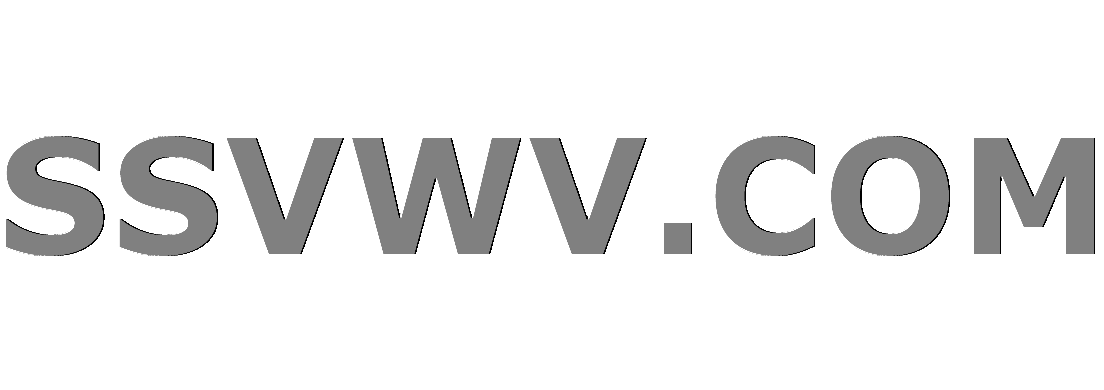
Multi tool use
I can draw a single rect with :
let roundedRect = UIBezierPath(roundedRect: rect, cornerRadius: 50)
with this I can not add a new rect to the same path. (I need multiple rects to a single layer)
I can also just draw a rect by moving the point :
path.move(to: CGPoint(x:point1.x-rectWidth/2.0,y:point1.y) )
path.addLine(to: CGPoint(x: point1.x-rectWidth/2.0, y: point2.y))
path.addLine(to: CGPoint(x: point1.x+rectWidth/2.0, y: point2.y))
path.addLine(to: CGPoint(x: point1.x+rectWidth/2.0, y: point1.y))
path.addLine(to: CGPoint(x:point1.x-rectWidth/2.0,y:point1.y))
which will not be a rounded rect.
Which approach can I use to get a rounded rect ?
swift uibezierpath
add a comment |
I can draw a single rect with :
let roundedRect = UIBezierPath(roundedRect: rect, cornerRadius: 50)
with this I can not add a new rect to the same path. (I need multiple rects to a single layer)
I can also just draw a rect by moving the point :
path.move(to: CGPoint(x:point1.x-rectWidth/2.0,y:point1.y) )
path.addLine(to: CGPoint(x: point1.x-rectWidth/2.0, y: point2.y))
path.addLine(to: CGPoint(x: point1.x+rectWidth/2.0, y: point2.y))
path.addLine(to: CGPoint(x: point1.x+rectWidth/2.0, y: point1.y))
path.addLine(to: CGPoint(x:point1.x-rectWidth/2.0,y:point1.y))
which will not be a rounded rect.
Which approach can I use to get a rounded rect ?
swift uibezierpath
1
Why not create multipleCAShapeLayers
? Like views, layers have sublayers.
– dfd
Nov 12 '18 at 4:10
@dfd, the OP never said anything about layers. We don't know if that's what they're doing with their paths.
– Duncan C
Nov 12 '18 at 4:38
1
@DuncanC The question states "I need multiple rects to a single layer".
– rmaddy
Nov 12 '18 at 5:38
So it does. I missed that bit.
– Duncan C
Nov 12 '18 at 12:40
add a comment |
I can draw a single rect with :
let roundedRect = UIBezierPath(roundedRect: rect, cornerRadius: 50)
with this I can not add a new rect to the same path. (I need multiple rects to a single layer)
I can also just draw a rect by moving the point :
path.move(to: CGPoint(x:point1.x-rectWidth/2.0,y:point1.y) )
path.addLine(to: CGPoint(x: point1.x-rectWidth/2.0, y: point2.y))
path.addLine(to: CGPoint(x: point1.x+rectWidth/2.0, y: point2.y))
path.addLine(to: CGPoint(x: point1.x+rectWidth/2.0, y: point1.y))
path.addLine(to: CGPoint(x:point1.x-rectWidth/2.0,y:point1.y))
which will not be a rounded rect.
Which approach can I use to get a rounded rect ?
swift uibezierpath
I can draw a single rect with :
let roundedRect = UIBezierPath(roundedRect: rect, cornerRadius: 50)
with this I can not add a new rect to the same path. (I need multiple rects to a single layer)
I can also just draw a rect by moving the point :
path.move(to: CGPoint(x:point1.x-rectWidth/2.0,y:point1.y) )
path.addLine(to: CGPoint(x: point1.x-rectWidth/2.0, y: point2.y))
path.addLine(to: CGPoint(x: point1.x+rectWidth/2.0, y: point2.y))
path.addLine(to: CGPoint(x: point1.x+rectWidth/2.0, y: point1.y))
path.addLine(to: CGPoint(x:point1.x-rectWidth/2.0,y:point1.y))
which will not be a rounded rect.
Which approach can I use to get a rounded rect ?
swift uibezierpath
swift uibezierpath
asked Nov 12 '18 at 3:49


CurneliousCurnelious
3,825750102
3,825750102
1
Why not create multipleCAShapeLayers
? Like views, layers have sublayers.
– dfd
Nov 12 '18 at 4:10
@dfd, the OP never said anything about layers. We don't know if that's what they're doing with their paths.
– Duncan C
Nov 12 '18 at 4:38
1
@DuncanC The question states "I need multiple rects to a single layer".
– rmaddy
Nov 12 '18 at 5:38
So it does. I missed that bit.
– Duncan C
Nov 12 '18 at 12:40
add a comment |
1
Why not create multipleCAShapeLayers
? Like views, layers have sublayers.
– dfd
Nov 12 '18 at 4:10
@dfd, the OP never said anything about layers. We don't know if that's what they're doing with their paths.
– Duncan C
Nov 12 '18 at 4:38
1
@DuncanC The question states "I need multiple rects to a single layer".
– rmaddy
Nov 12 '18 at 5:38
So it does. I missed that bit.
– Duncan C
Nov 12 '18 at 12:40
1
1
Why not create multiple
CAShapeLayers
? Like views, layers have sublayers.– dfd
Nov 12 '18 at 4:10
Why not create multiple
CAShapeLayers
? Like views, layers have sublayers.– dfd
Nov 12 '18 at 4:10
@dfd, the OP never said anything about layers. We don't know if that's what they're doing with their paths.
– Duncan C
Nov 12 '18 at 4:38
@dfd, the OP never said anything about layers. We don't know if that's what they're doing with their paths.
– Duncan C
Nov 12 '18 at 4:38
1
1
@DuncanC The question states "I need multiple rects to a single layer".
– rmaddy
Nov 12 '18 at 5:38
@DuncanC The question states "I need multiple rects to a single layer".
– rmaddy
Nov 12 '18 at 5:38
So it does. I missed that bit.
– Duncan C
Nov 12 '18 at 12:40
So it does. I missed that bit.
– Duncan C
Nov 12 '18 at 12:40
add a comment |
1 Answer
1
active
oldest
votes
Building a rounded rect yourself is somewhat tricky. I suggest creating rounded rect paths using the UIBezierPath initializer init(roundedRect:cornerRadius:)
You can use append(_:)
to append rounded rect paths to another path:
var path = UIBezierPath() //Create an empty path
//Add a rounded rect to the path
path.append(UIBezierPath(roundedRect: rect1, cornerRadius: radius1))
//Add another rounded rect to the path
path.append(UIBezierPath(roundedRect: rect2, cornerRadius: radius2))
//Lather, rinse, repeat.
path.append(UIBezierPath(roundedRect: rect3, cornerRadius: radius3))
Edit:
To animate all of your rectangles drawing from the bottom, like an ink-jet printer, create a CAShapeLayer that's the same size as your view, install a zero-height rectangle at the bottom, and make that layer the mask layer for your view's layer. Then create a CABasicAnimation of the rectangle growing to the full height of the view.
So you create multiple instances of a bezier, and extract their paths to a single one. Is it ok with performance (maybe dumb to ask)
– Curnelious
Nov 12 '18 at 5:05
@Curnelious performance-wise which part are you worried about?
– inokey
Nov 12 '18 at 5:39
Thanks, with this I can not find a way to animate it. Do you know maybe how to animate the drawing from the bottom ? could make it work with CBAnimation
– Curnelious
Nov 12 '18 at 6:28
What sort of animation do you want? Drawing one rect at a time, and drawing each rect instantly? Or do you want to "paint" up from the bottom, like an ink-jet printer?
– Duncan C
Nov 12 '18 at 12:39
1
See the edit to my answer. You could use a mask layer with a rectangle shape that grows from zero height to the full height of the view.
– Duncan C
Nov 13 '18 at 11:07
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53255720%2fuibezierpath-draw-multiple-rects-for-a-single-path%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Building a rounded rect yourself is somewhat tricky. I suggest creating rounded rect paths using the UIBezierPath initializer init(roundedRect:cornerRadius:)
You can use append(_:)
to append rounded rect paths to another path:
var path = UIBezierPath() //Create an empty path
//Add a rounded rect to the path
path.append(UIBezierPath(roundedRect: rect1, cornerRadius: radius1))
//Add another rounded rect to the path
path.append(UIBezierPath(roundedRect: rect2, cornerRadius: radius2))
//Lather, rinse, repeat.
path.append(UIBezierPath(roundedRect: rect3, cornerRadius: radius3))
Edit:
To animate all of your rectangles drawing from the bottom, like an ink-jet printer, create a CAShapeLayer that's the same size as your view, install a zero-height rectangle at the bottom, and make that layer the mask layer for your view's layer. Then create a CABasicAnimation of the rectangle growing to the full height of the view.
So you create multiple instances of a bezier, and extract their paths to a single one. Is it ok with performance (maybe dumb to ask)
– Curnelious
Nov 12 '18 at 5:05
@Curnelious performance-wise which part are you worried about?
– inokey
Nov 12 '18 at 5:39
Thanks, with this I can not find a way to animate it. Do you know maybe how to animate the drawing from the bottom ? could make it work with CBAnimation
– Curnelious
Nov 12 '18 at 6:28
What sort of animation do you want? Drawing one rect at a time, and drawing each rect instantly? Or do you want to "paint" up from the bottom, like an ink-jet printer?
– Duncan C
Nov 12 '18 at 12:39
1
See the edit to my answer. You could use a mask layer with a rectangle shape that grows from zero height to the full height of the view.
– Duncan C
Nov 13 '18 at 11:07
|
show 1 more comment
Building a rounded rect yourself is somewhat tricky. I suggest creating rounded rect paths using the UIBezierPath initializer init(roundedRect:cornerRadius:)
You can use append(_:)
to append rounded rect paths to another path:
var path = UIBezierPath() //Create an empty path
//Add a rounded rect to the path
path.append(UIBezierPath(roundedRect: rect1, cornerRadius: radius1))
//Add another rounded rect to the path
path.append(UIBezierPath(roundedRect: rect2, cornerRadius: radius2))
//Lather, rinse, repeat.
path.append(UIBezierPath(roundedRect: rect3, cornerRadius: radius3))
Edit:
To animate all of your rectangles drawing from the bottom, like an ink-jet printer, create a CAShapeLayer that's the same size as your view, install a zero-height rectangle at the bottom, and make that layer the mask layer for your view's layer. Then create a CABasicAnimation of the rectangle growing to the full height of the view.
So you create multiple instances of a bezier, and extract their paths to a single one. Is it ok with performance (maybe dumb to ask)
– Curnelious
Nov 12 '18 at 5:05
@Curnelious performance-wise which part are you worried about?
– inokey
Nov 12 '18 at 5:39
Thanks, with this I can not find a way to animate it. Do you know maybe how to animate the drawing from the bottom ? could make it work with CBAnimation
– Curnelious
Nov 12 '18 at 6:28
What sort of animation do you want? Drawing one rect at a time, and drawing each rect instantly? Or do you want to "paint" up from the bottom, like an ink-jet printer?
– Duncan C
Nov 12 '18 at 12:39
1
See the edit to my answer. You could use a mask layer with a rectangle shape that grows from zero height to the full height of the view.
– Duncan C
Nov 13 '18 at 11:07
|
show 1 more comment
Building a rounded rect yourself is somewhat tricky. I suggest creating rounded rect paths using the UIBezierPath initializer init(roundedRect:cornerRadius:)
You can use append(_:)
to append rounded rect paths to another path:
var path = UIBezierPath() //Create an empty path
//Add a rounded rect to the path
path.append(UIBezierPath(roundedRect: rect1, cornerRadius: radius1))
//Add another rounded rect to the path
path.append(UIBezierPath(roundedRect: rect2, cornerRadius: radius2))
//Lather, rinse, repeat.
path.append(UIBezierPath(roundedRect: rect3, cornerRadius: radius3))
Edit:
To animate all of your rectangles drawing from the bottom, like an ink-jet printer, create a CAShapeLayer that's the same size as your view, install a zero-height rectangle at the bottom, and make that layer the mask layer for your view's layer. Then create a CABasicAnimation of the rectangle growing to the full height of the view.
Building a rounded rect yourself is somewhat tricky. I suggest creating rounded rect paths using the UIBezierPath initializer init(roundedRect:cornerRadius:)
You can use append(_:)
to append rounded rect paths to another path:
var path = UIBezierPath() //Create an empty path
//Add a rounded rect to the path
path.append(UIBezierPath(roundedRect: rect1, cornerRadius: radius1))
//Add another rounded rect to the path
path.append(UIBezierPath(roundedRect: rect2, cornerRadius: radius2))
//Lather, rinse, repeat.
path.append(UIBezierPath(roundedRect: rect3, cornerRadius: radius3))
Edit:
To animate all of your rectangles drawing from the bottom, like an ink-jet printer, create a CAShapeLayer that's the same size as your view, install a zero-height rectangle at the bottom, and make that layer the mask layer for your view's layer. Then create a CABasicAnimation of the rectangle growing to the full height of the view.
edited Nov 13 '18 at 11:06
answered Nov 12 '18 at 4:37
Duncan CDuncan C
92.2k13114196
92.2k13114196
So you create multiple instances of a bezier, and extract their paths to a single one. Is it ok with performance (maybe dumb to ask)
– Curnelious
Nov 12 '18 at 5:05
@Curnelious performance-wise which part are you worried about?
– inokey
Nov 12 '18 at 5:39
Thanks, with this I can not find a way to animate it. Do you know maybe how to animate the drawing from the bottom ? could make it work with CBAnimation
– Curnelious
Nov 12 '18 at 6:28
What sort of animation do you want? Drawing one rect at a time, and drawing each rect instantly? Or do you want to "paint" up from the bottom, like an ink-jet printer?
– Duncan C
Nov 12 '18 at 12:39
1
See the edit to my answer. You could use a mask layer with a rectangle shape that grows from zero height to the full height of the view.
– Duncan C
Nov 13 '18 at 11:07
|
show 1 more comment
So you create multiple instances of a bezier, and extract their paths to a single one. Is it ok with performance (maybe dumb to ask)
– Curnelious
Nov 12 '18 at 5:05
@Curnelious performance-wise which part are you worried about?
– inokey
Nov 12 '18 at 5:39
Thanks, with this I can not find a way to animate it. Do you know maybe how to animate the drawing from the bottom ? could make it work with CBAnimation
– Curnelious
Nov 12 '18 at 6:28
What sort of animation do you want? Drawing one rect at a time, and drawing each rect instantly? Or do you want to "paint" up from the bottom, like an ink-jet printer?
– Duncan C
Nov 12 '18 at 12:39
1
See the edit to my answer. You could use a mask layer with a rectangle shape that grows from zero height to the full height of the view.
– Duncan C
Nov 13 '18 at 11:07
So you create multiple instances of a bezier, and extract their paths to a single one. Is it ok with performance (maybe dumb to ask)
– Curnelious
Nov 12 '18 at 5:05
So you create multiple instances of a bezier, and extract their paths to a single one. Is it ok with performance (maybe dumb to ask)
– Curnelious
Nov 12 '18 at 5:05
@Curnelious performance-wise which part are you worried about?
– inokey
Nov 12 '18 at 5:39
@Curnelious performance-wise which part are you worried about?
– inokey
Nov 12 '18 at 5:39
Thanks, with this I can not find a way to animate it. Do you know maybe how to animate the drawing from the bottom ? could make it work with CBAnimation
– Curnelious
Nov 12 '18 at 6:28
Thanks, with this I can not find a way to animate it. Do you know maybe how to animate the drawing from the bottom ? could make it work with CBAnimation
– Curnelious
Nov 12 '18 at 6:28
What sort of animation do you want? Drawing one rect at a time, and drawing each rect instantly? Or do you want to "paint" up from the bottom, like an ink-jet printer?
– Duncan C
Nov 12 '18 at 12:39
What sort of animation do you want? Drawing one rect at a time, and drawing each rect instantly? Or do you want to "paint" up from the bottom, like an ink-jet printer?
– Duncan C
Nov 12 '18 at 12:39
1
1
See the edit to my answer. You could use a mask layer with a rectangle shape that grows from zero height to the full height of the view.
– Duncan C
Nov 13 '18 at 11:07
See the edit to my answer. You could use a mask layer with a rectangle shape that grows from zero height to the full height of the view.
– Duncan C
Nov 13 '18 at 11:07
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53255720%2fuibezierpath-draw-multiple-rects-for-a-single-path%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
A yquE9ndjnlI 3s8XjSi2b,8Iyp5Ln 3pBNTi iiZFC2W0UaOe0I0,UumrRgCP,N2lf0NKd
1
Why not create multiple
CAShapeLayers
? Like views, layers have sublayers.– dfd
Nov 12 '18 at 4:10
@dfd, the OP never said anything about layers. We don't know if that's what they're doing with their paths.
– Duncan C
Nov 12 '18 at 4:38
1
@DuncanC The question states "I need multiple rects to a single layer".
– rmaddy
Nov 12 '18 at 5:38
So it does. I missed that bit.
– Duncan C
Nov 12 '18 at 12:40