Destroy user session without Devise Rails 5
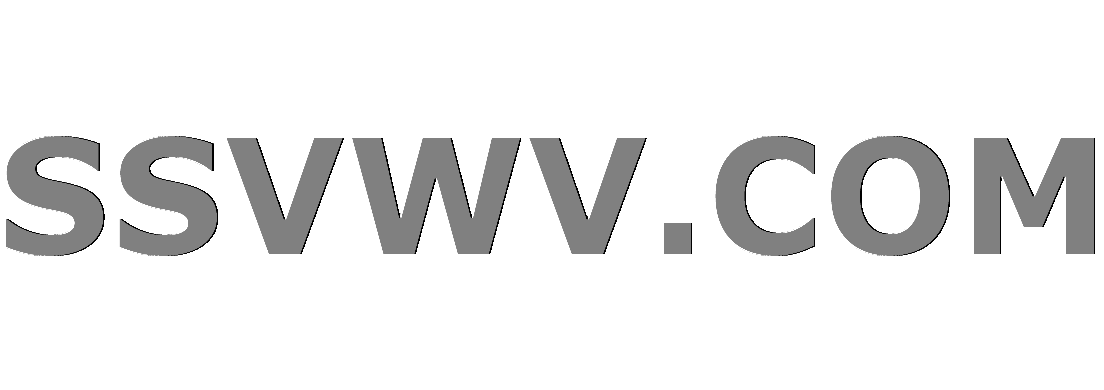
Multi tool use
I will try to do a simple user authorization without Devise.
My session controller has new, create and destroy actions:
def new
@user = User.new
end
def create
@user = User.find_by(name: user_params[:name])
@user ||= User.create(user_params)
if @user.valid? && @user.authenticate(user_params[:password])
session[:user_id] = @user.id
redirect_to tasks_path
end
end
def destroy
reset_session
end
But it doesn't work. How can I destroy a user session?
ruby-on-rails ruby-on-rails-5
add a comment |
I will try to do a simple user authorization without Devise.
My session controller has new, create and destroy actions:
def new
@user = User.new
end
def create
@user = User.find_by(name: user_params[:name])
@user ||= User.create(user_params)
if @user.valid? && @user.authenticate(user_params[:password])
session[:user_id] = @user.id
redirect_to tasks_path
end
end
def destroy
reset_session
end
But it doesn't work. How can I destroy a user session?
ruby-on-rails ruby-on-rails-5
Hi! Could you add some info to your question? What do you mean by 'it doesn't work'? Do you have an error?
– ldeld
Nov 14 '18 at 13:41
I don't have an error. But current_user variable is not be empty or nil after calling a destroy action. In aplication controller I have this method: def current_user @current_user ||= User.find_by(id: session[:user_id]) end
– wannabeaprogrammer
Nov 14 '18 at 13:46
Hi, I think your problem could be on your reset_session method, maybe it's in your helper class. Could you update your answer with its content? You should have a destroy from session hash of your user_id or something similar
– Pietro Allievi
Nov 14 '18 at 15:09
add a comment |
I will try to do a simple user authorization without Devise.
My session controller has new, create and destroy actions:
def new
@user = User.new
end
def create
@user = User.find_by(name: user_params[:name])
@user ||= User.create(user_params)
if @user.valid? && @user.authenticate(user_params[:password])
session[:user_id] = @user.id
redirect_to tasks_path
end
end
def destroy
reset_session
end
But it doesn't work. How can I destroy a user session?
ruby-on-rails ruby-on-rails-5
I will try to do a simple user authorization without Devise.
My session controller has new, create and destroy actions:
def new
@user = User.new
end
def create
@user = User.find_by(name: user_params[:name])
@user ||= User.create(user_params)
if @user.valid? && @user.authenticate(user_params[:password])
session[:user_id] = @user.id
redirect_to tasks_path
end
end
def destroy
reset_session
end
But it doesn't work. How can I destroy a user session?
ruby-on-rails ruby-on-rails-5
ruby-on-rails ruby-on-rails-5
asked Nov 14 '18 at 13:16
wannabeaprogrammerwannabeaprogrammer
82
82
Hi! Could you add some info to your question? What do you mean by 'it doesn't work'? Do you have an error?
– ldeld
Nov 14 '18 at 13:41
I don't have an error. But current_user variable is not be empty or nil after calling a destroy action. In aplication controller I have this method: def current_user @current_user ||= User.find_by(id: session[:user_id]) end
– wannabeaprogrammer
Nov 14 '18 at 13:46
Hi, I think your problem could be on your reset_session method, maybe it's in your helper class. Could you update your answer with its content? You should have a destroy from session hash of your user_id or something similar
– Pietro Allievi
Nov 14 '18 at 15:09
add a comment |
Hi! Could you add some info to your question? What do you mean by 'it doesn't work'? Do you have an error?
– ldeld
Nov 14 '18 at 13:41
I don't have an error. But current_user variable is not be empty or nil after calling a destroy action. In aplication controller I have this method: def current_user @current_user ||= User.find_by(id: session[:user_id]) end
– wannabeaprogrammer
Nov 14 '18 at 13:46
Hi, I think your problem could be on your reset_session method, maybe it's in your helper class. Could you update your answer with its content? You should have a destroy from session hash of your user_id or something similar
– Pietro Allievi
Nov 14 '18 at 15:09
Hi! Could you add some info to your question? What do you mean by 'it doesn't work'? Do you have an error?
– ldeld
Nov 14 '18 at 13:41
Hi! Could you add some info to your question? What do you mean by 'it doesn't work'? Do you have an error?
– ldeld
Nov 14 '18 at 13:41
I don't have an error. But current_user variable is not be empty or nil after calling a destroy action. In aplication controller I have this method: def current_user @current_user ||= User.find_by(id: session[:user_id]) end
– wannabeaprogrammer
Nov 14 '18 at 13:46
I don't have an error. But current_user variable is not be empty or nil after calling a destroy action. In aplication controller I have this method: def current_user @current_user ||= User.find_by(id: session[:user_id]) end
– wannabeaprogrammer
Nov 14 '18 at 13:46
Hi, I think your problem could be on your reset_session method, maybe it's in your helper class. Could you update your answer with its content? You should have a destroy from session hash of your user_id or something similar
– Pietro Allievi
Nov 14 '18 at 15:09
Hi, I think your problem could be on your reset_session method, maybe it's in your helper class. Could you update your answer with its content? You should have a destroy from session hash of your user_id or something similar
– Pietro Allievi
Nov 14 '18 at 15:09
add a comment |
1 Answer
1
active
oldest
votes
Something like this should do it. It really depends on what kind of helpers you have set up. You'll need access to the session variable you created and also the user id, so session.delete(@user.id)
might end up being what you need. Depends on how this is being sent over to the method and what data you have access to (is there a before action or something providing the @user variable or do you need to find it with something like @user = User.find(:params)
where params is the user id.
def destroy
session.delete(:user_id)
@user = nil
end
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53301141%2fdestroy-user-session-without-devise-rails-5%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Something like this should do it. It really depends on what kind of helpers you have set up. You'll need access to the session variable you created and also the user id, so session.delete(@user.id)
might end up being what you need. Depends on how this is being sent over to the method and what data you have access to (is there a before action or something providing the @user variable or do you need to find it with something like @user = User.find(:params)
where params is the user id.
def destroy
session.delete(:user_id)
@user = nil
end
add a comment |
Something like this should do it. It really depends on what kind of helpers you have set up. You'll need access to the session variable you created and also the user id, so session.delete(@user.id)
might end up being what you need. Depends on how this is being sent over to the method and what data you have access to (is there a before action or something providing the @user variable or do you need to find it with something like @user = User.find(:params)
where params is the user id.
def destroy
session.delete(:user_id)
@user = nil
end
add a comment |
Something like this should do it. It really depends on what kind of helpers you have set up. You'll need access to the session variable you created and also the user id, so session.delete(@user.id)
might end up being what you need. Depends on how this is being sent over to the method and what data you have access to (is there a before action or something providing the @user variable or do you need to find it with something like @user = User.find(:params)
where params is the user id.
def destroy
session.delete(:user_id)
@user = nil
end
Something like this should do it. It really depends on what kind of helpers you have set up. You'll need access to the session variable you created and also the user id, so session.delete(@user.id)
might end up being what you need. Depends on how this is being sent over to the method and what data you have access to (is there a before action or something providing the @user variable or do you need to find it with something like @user = User.find(:params)
where params is the user id.
def destroy
session.delete(:user_id)
@user = nil
end
answered Nov 14 '18 at 14:27


Rockwell RiceRockwell Rice
2,22841937
2,22841937
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53301141%2fdestroy-user-session-without-devise-rails-5%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cAH 543trLCLIx1kQi 7r
Hi! Could you add some info to your question? What do you mean by 'it doesn't work'? Do you have an error?
– ldeld
Nov 14 '18 at 13:41
I don't have an error. But current_user variable is not be empty or nil after calling a destroy action. In aplication controller I have this method: def current_user @current_user ||= User.find_by(id: session[:user_id]) end
– wannabeaprogrammer
Nov 14 '18 at 13:46
Hi, I think your problem could be on your reset_session method, maybe it's in your helper class. Could you update your answer with its content? You should have a destroy from session hash of your user_id or something similar
– Pietro Allievi
Nov 14 '18 at 15:09