Dart Future HttpCLientRequest Returns Null
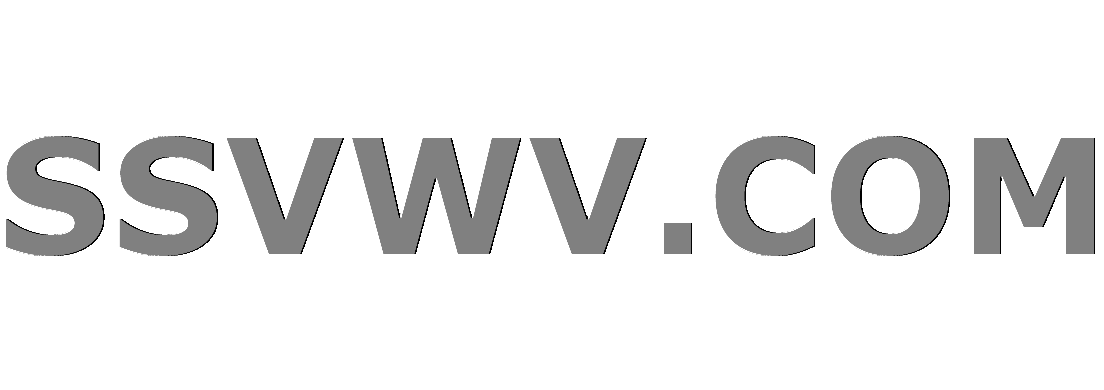
Multi tool use
up vote
0
down vote
favorite
The class Requests set up an HttpClientRequest. The method getTeamsJsonForRequest is supposed to return the JSON response. However, the variable 'return' is not being assigned properly I assum. The print 'CONTS' in the .then response successfully prints the correct response, but printing 'myres' sections says result is null. Not sure why result is not being assigned in the response.transform section.
class Requests
static Future getTeamsJsonForRequest(String reqPath) async
var result;
HttpClient myhttp = new HttpClient();
String path = '/api/v3' + reqPath;
myhttp.get('www.thebluealliance.com', 80, path)
.then((HttpClientRequest request)
request.headers.set("accept", "application/json");
request.headers.set("X-TBA-Auth-Key", "XXXXX");
return request.close();
)
.then((HttpClientResponse response)
response.transform(utf8.decoder).transform(json.decoder).listen((conts)
print('CONTS: ' + conts.toString());
result = json.decode(conts).toString();
);
);
print('myres: ' + result.toString());
return result;
json http

add a comment |
up vote
0
down vote
favorite
The class Requests set up an HttpClientRequest. The method getTeamsJsonForRequest is supposed to return the JSON response. However, the variable 'return' is not being assigned properly I assum. The print 'CONTS' in the .then response successfully prints the correct response, but printing 'myres' sections says result is null. Not sure why result is not being assigned in the response.transform section.
class Requests
static Future getTeamsJsonForRequest(String reqPath) async
var result;
HttpClient myhttp = new HttpClient();
String path = '/api/v3' + reqPath;
myhttp.get('www.thebluealliance.com', 80, path)
.then((HttpClientRequest request)
request.headers.set("accept", "application/json");
request.headers.set("X-TBA-Auth-Key", "XXXXX");
return request.close();
)
.then((HttpClientResponse response)
response.transform(utf8.decoder).transform(json.decoder).listen((conts)
print('CONTS: ' + conts.toString());
result = json.decode(conts).toString();
);
);
print('myres: ' + result.toString());
return result;
json http

add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
The class Requests set up an HttpClientRequest. The method getTeamsJsonForRequest is supposed to return the JSON response. However, the variable 'return' is not being assigned properly I assum. The print 'CONTS' in the .then response successfully prints the correct response, but printing 'myres' sections says result is null. Not sure why result is not being assigned in the response.transform section.
class Requests
static Future getTeamsJsonForRequest(String reqPath) async
var result;
HttpClient myhttp = new HttpClient();
String path = '/api/v3' + reqPath;
myhttp.get('www.thebluealliance.com', 80, path)
.then((HttpClientRequest request)
request.headers.set("accept", "application/json");
request.headers.set("X-TBA-Auth-Key", "XXXXX");
return request.close();
)
.then((HttpClientResponse response)
response.transform(utf8.decoder).transform(json.decoder).listen((conts)
print('CONTS: ' + conts.toString());
result = json.decode(conts).toString();
);
);
print('myres: ' + result.toString());
return result;
json http

The class Requests set up an HttpClientRequest. The method getTeamsJsonForRequest is supposed to return the JSON response. However, the variable 'return' is not being assigned properly I assum. The print 'CONTS' in the .then response successfully prints the correct response, but printing 'myres' sections says result is null. Not sure why result is not being assigned in the response.transform section.
class Requests
static Future getTeamsJsonForRequest(String reqPath) async
var result;
HttpClient myhttp = new HttpClient();
String path = '/api/v3' + reqPath;
myhttp.get('www.thebluealliance.com', 80, path)
.then((HttpClientRequest request)
request.headers.set("accept", "application/json");
request.headers.set("X-TBA-Auth-Key", "XXXXX");
return request.close();
)
.then((HttpClientResponse response)
response.transform(utf8.decoder).transform(json.decoder).listen((conts)
print('CONTS: ' + conts.toString());
result = json.decode(conts).toString();
);
);
print('myres: ' + result.toString());
return result;
json http

json http

asked Nov 9 at 20:43
yzet00
166318
166318
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
accepted
Short answer: avoid using Future.then
inside an async
method.
Your print
is executing before the response comes back. https://www.dartlang.org/tutorials/language/futures#async-await
Without an await
any work that is done asynchronously will happen after subsequent statements in this function are executed.
Here is how I'd write this:
Future<String> teamsJsonForRequest(String requestPath) async
var client = new HttpClient();
var path = '/api/v3$requestPath';
var request = (await client.get('www.thebluealliance.com', 80, path))
..headers.set("accept", "application/json")
..headers.set("X-TBA-Auth-Key", "XXXXX");
var response = await request.close();
var result =
await response.transform(utf8.decoder).transform(json.decoder).single;
print('myres: $result');
return result;
add a comment |
up vote
0
down vote
In
.then((HttpClientResponse response)
response.transform(utf8.decoder).transform(json.decoder).listen((conts)
print('CONTS: ' + conts.toString());
result = json.decode(conts).toString();
);
);
print('myres: ' + result.toString());
return result;
this line
result = json.decode(conts).toString();
is executed muuuch later than this line
return result;
The request to the server is sent and then return result;
is executed.
The then(...)
part is executed when the response from the server arrives.
Change the code to
class Requests
static Future getTeamsJsonForRequest(String reqPath) async
HttpClient myhttp = new HttpClient();
String path = '/api/v3' + reqPath;
return myhttp.get('www.thebluealliance.com', 80, path)
.then((HttpClientRequest request)
request.headers.set("accept", "application/json");
request.headers.set("X-TBA-Auth-Key", "XXXXX");
return request.close();
)
.then((HttpClientResponse response)
response.transform(utf8.decoder).transform(json.decoder).listen((conts)
print('CONTS: ' + conts.toString());
var result = json.decode(conts).toString();
print('myres: ' + result.toString());
return result;
);
);
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Short answer: avoid using Future.then
inside an async
method.
Your print
is executing before the response comes back. https://www.dartlang.org/tutorials/language/futures#async-await
Without an await
any work that is done asynchronously will happen after subsequent statements in this function are executed.
Here is how I'd write this:
Future<String> teamsJsonForRequest(String requestPath) async
var client = new HttpClient();
var path = '/api/v3$requestPath';
var request = (await client.get('www.thebluealliance.com', 80, path))
..headers.set("accept", "application/json")
..headers.set("X-TBA-Auth-Key", "XXXXX");
var response = await request.close();
var result =
await response.transform(utf8.decoder).transform(json.decoder).single;
print('myres: $result');
return result;
add a comment |
up vote
0
down vote
accepted
Short answer: avoid using Future.then
inside an async
method.
Your print
is executing before the response comes back. https://www.dartlang.org/tutorials/language/futures#async-await
Without an await
any work that is done asynchronously will happen after subsequent statements in this function are executed.
Here is how I'd write this:
Future<String> teamsJsonForRequest(String requestPath) async
var client = new HttpClient();
var path = '/api/v3$requestPath';
var request = (await client.get('www.thebluealliance.com', 80, path))
..headers.set("accept", "application/json")
..headers.set("X-TBA-Auth-Key", "XXXXX");
var response = await request.close();
var result =
await response.transform(utf8.decoder).transform(json.decoder).single;
print('myres: $result');
return result;
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Short answer: avoid using Future.then
inside an async
method.
Your print
is executing before the response comes back. https://www.dartlang.org/tutorials/language/futures#async-await
Without an await
any work that is done asynchronously will happen after subsequent statements in this function are executed.
Here is how I'd write this:
Future<String> teamsJsonForRequest(String requestPath) async
var client = new HttpClient();
var path = '/api/v3$requestPath';
var request = (await client.get('www.thebluealliance.com', 80, path))
..headers.set("accept", "application/json")
..headers.set("X-TBA-Auth-Key", "XXXXX");
var response = await request.close();
var result =
await response.transform(utf8.decoder).transform(json.decoder).single;
print('myres: $result');
return result;
Short answer: avoid using Future.then
inside an async
method.
Your print
is executing before the response comes back. https://www.dartlang.org/tutorials/language/futures#async-await
Without an await
any work that is done asynchronously will happen after subsequent statements in this function are executed.
Here is how I'd write this:
Future<String> teamsJsonForRequest(String requestPath) async
var client = new HttpClient();
var path = '/api/v3$requestPath';
var request = (await client.get('www.thebluealliance.com', 80, path))
..headers.set("accept", "application/json")
..headers.set("X-TBA-Auth-Key", "XXXXX");
var response = await request.close();
var result =
await response.transform(utf8.decoder).transform(json.decoder).single;
print('myres: $result');
return result;
answered Nov 9 at 21:49
Nate Bosch
58929
58929
add a comment |
add a comment |
up vote
0
down vote
In
.then((HttpClientResponse response)
response.transform(utf8.decoder).transform(json.decoder).listen((conts)
print('CONTS: ' + conts.toString());
result = json.decode(conts).toString();
);
);
print('myres: ' + result.toString());
return result;
this line
result = json.decode(conts).toString();
is executed muuuch later than this line
return result;
The request to the server is sent and then return result;
is executed.
The then(...)
part is executed when the response from the server arrives.
Change the code to
class Requests
static Future getTeamsJsonForRequest(String reqPath) async
HttpClient myhttp = new HttpClient();
String path = '/api/v3' + reqPath;
return myhttp.get('www.thebluealliance.com', 80, path)
.then((HttpClientRequest request)
request.headers.set("accept", "application/json");
request.headers.set("X-TBA-Auth-Key", "XXXXX");
return request.close();
)
.then((HttpClientResponse response)
response.transform(utf8.decoder).transform(json.decoder).listen((conts)
print('CONTS: ' + conts.toString());
var result = json.decode(conts).toString();
print('myres: ' + result.toString());
return result;
);
);
add a comment |
up vote
0
down vote
In
.then((HttpClientResponse response)
response.transform(utf8.decoder).transform(json.decoder).listen((conts)
print('CONTS: ' + conts.toString());
result = json.decode(conts).toString();
);
);
print('myres: ' + result.toString());
return result;
this line
result = json.decode(conts).toString();
is executed muuuch later than this line
return result;
The request to the server is sent and then return result;
is executed.
The then(...)
part is executed when the response from the server arrives.
Change the code to
class Requests
static Future getTeamsJsonForRequest(String reqPath) async
HttpClient myhttp = new HttpClient();
String path = '/api/v3' + reqPath;
return myhttp.get('www.thebluealliance.com', 80, path)
.then((HttpClientRequest request)
request.headers.set("accept", "application/json");
request.headers.set("X-TBA-Auth-Key", "XXXXX");
return request.close();
)
.then((HttpClientResponse response)
response.transform(utf8.decoder).transform(json.decoder).listen((conts)
print('CONTS: ' + conts.toString());
var result = json.decode(conts).toString();
print('myres: ' + result.toString());
return result;
);
);
add a comment |
up vote
0
down vote
up vote
0
down vote
In
.then((HttpClientResponse response)
response.transform(utf8.decoder).transform(json.decoder).listen((conts)
print('CONTS: ' + conts.toString());
result = json.decode(conts).toString();
);
);
print('myres: ' + result.toString());
return result;
this line
result = json.decode(conts).toString();
is executed muuuch later than this line
return result;
The request to the server is sent and then return result;
is executed.
The then(...)
part is executed when the response from the server arrives.
Change the code to
class Requests
static Future getTeamsJsonForRequest(String reqPath) async
HttpClient myhttp = new HttpClient();
String path = '/api/v3' + reqPath;
return myhttp.get('www.thebluealliance.com', 80, path)
.then((HttpClientRequest request)
request.headers.set("accept", "application/json");
request.headers.set("X-TBA-Auth-Key", "XXXXX");
return request.close();
)
.then((HttpClientResponse response)
response.transform(utf8.decoder).transform(json.decoder).listen((conts)
print('CONTS: ' + conts.toString());
var result = json.decode(conts).toString();
print('myres: ' + result.toString());
return result;
);
);
In
.then((HttpClientResponse response)
response.transform(utf8.decoder).transform(json.decoder).listen((conts)
print('CONTS: ' + conts.toString());
result = json.decode(conts).toString();
);
);
print('myres: ' + result.toString());
return result;
this line
result = json.decode(conts).toString();
is executed muuuch later than this line
return result;
The request to the server is sent and then return result;
is executed.
The then(...)
part is executed when the response from the server arrives.
Change the code to
class Requests
static Future getTeamsJsonForRequest(String reqPath) async
HttpClient myhttp = new HttpClient();
String path = '/api/v3' + reqPath;
return myhttp.get('www.thebluealliance.com', 80, path)
.then((HttpClientRequest request)
request.headers.set("accept", "application/json");
request.headers.set("X-TBA-Auth-Key", "XXXXX");
return request.close();
)
.then((HttpClientResponse response)
response.transform(utf8.decoder).transform(json.decoder).listen((conts)
print('CONTS: ' + conts.toString());
var result = json.decode(conts).toString();
print('myres: ' + result.toString());
return result;
);
);
answered Nov 9 at 21:50


Günter Zöchbauer
306k63901849
306k63901849
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53233008%2fdart-future-httpclientrequest-returns-null%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
oLzZn7,h6E,Kt,w9X9xweiKGq,330Bt0IcX3 SpPO rxrNTLJcf2fQt,gB VIDQrAC,TKULDWp gbMoCt89M7HW7,kHyM9o3p,AEh,VX,7J1FhBdl