Function that takes a string and returns a list of 8-character strings
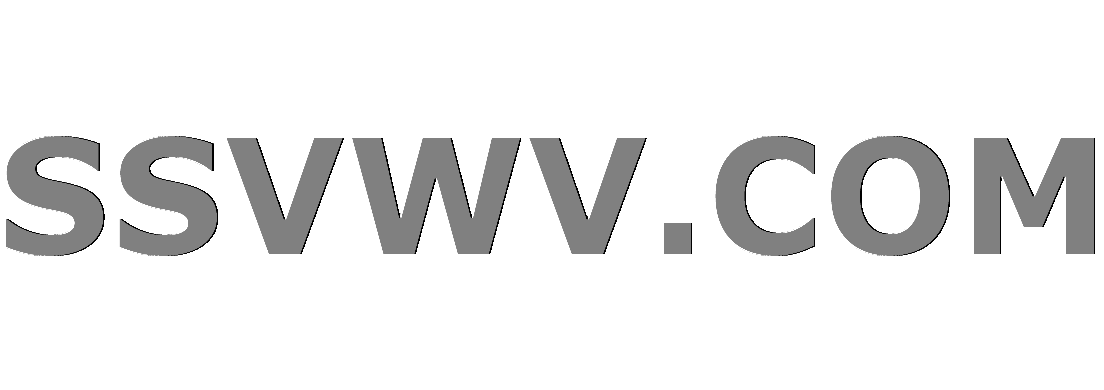
Multi tool use
up vote
3
down vote
favorite
I'm very new to programming. I need to create a function that takes any string and a fill character and returns a list of 8 character strings.
For example:
>>>segmentString("Hello, World!","-")
['Hello, W', 'orld!---']
As you can see, the string has 13 characters. The function splits into 2 strings, where the first contains 8 characters, and the second contains 5 characters and 3 fill characters.
So far, I've figured out how to do this for strings less than 8 characters, but not for more than 8 characters, where the string is split up, which is what I am stuck on.
def segmentString(s1,x):
while len(s1)<8:
s1+=x
return s1
How do you split a string up?
python string
add a comment |
up vote
3
down vote
favorite
I'm very new to programming. I need to create a function that takes any string and a fill character and returns a list of 8 character strings.
For example:
>>>segmentString("Hello, World!","-")
['Hello, W', 'orld!---']
As you can see, the string has 13 characters. The function splits into 2 strings, where the first contains 8 characters, and the second contains 5 characters and 3 fill characters.
So far, I've figured out how to do this for strings less than 8 characters, but not for more than 8 characters, where the string is split up, which is what I am stuck on.
def segmentString(s1,x):
while len(s1)<8:
s1+=x
return s1
How do you split a string up?
python string
add a comment |
up vote
3
down vote
favorite
up vote
3
down vote
favorite
I'm very new to programming. I need to create a function that takes any string and a fill character and returns a list of 8 character strings.
For example:
>>>segmentString("Hello, World!","-")
['Hello, W', 'orld!---']
As you can see, the string has 13 characters. The function splits into 2 strings, where the first contains 8 characters, and the second contains 5 characters and 3 fill characters.
So far, I've figured out how to do this for strings less than 8 characters, but not for more than 8 characters, where the string is split up, which is what I am stuck on.
def segmentString(s1,x):
while len(s1)<8:
s1+=x
return s1
How do you split a string up?
python string
I'm very new to programming. I need to create a function that takes any string and a fill character and returns a list of 8 character strings.
For example:
>>>segmentString("Hello, World!","-")
['Hello, W', 'orld!---']
As you can see, the string has 13 characters. The function splits into 2 strings, where the first contains 8 characters, and the second contains 5 characters and 3 fill characters.
So far, I've figured out how to do this for strings less than 8 characters, but not for more than 8 characters, where the string is split up, which is what I am stuck on.
def segmentString(s1,x):
while len(s1)<8:
s1+=x
return s1
How do you split a string up?
python string
python string
asked Nov 10 at 1:28


hello
314
314
add a comment |
add a comment |
5 Answers
5
active
oldest
votes
up vote
3
down vote
accepted
You can use a slightly modified version of this chunking recipe:
def chunks(L, n, char):
for i in range(0, len(L), n):
yield L[i:i + n].ljust(n, char)
res = list(chunks('Hello, World!', 8, '-'))
['Hello, W', 'orld!---']
str.ljust
performs the necessary padding.
1
Easier way to write the padding step:yield x.ljust(n, char)
. No need to perform the calculations for required padding yourself over and over. And because it doesn't even need to know the original length ofx
, you could one-line the body of the loop toyield L[i:i+n].ljust(n, char)
– ShadowRanger
Nov 10 at 1:56
@ShadowRanger, Good point, forgot aboutstr.ljust
!
– jpp
Nov 10 at 1:56
add a comment |
up vote
1
down vote
A slightly modified version of @jpp's answer is to first pad the string with your fill character, so that the length is cleanly divisible by 8:
def segmentString(s, c):
s = s + c * (8 - len(s) % 8)
return [s[i:i+8] for i in range(0, len(s), 8)]
>>> segmentString("Hello, World!","-")
['Hello, W', 'orld!---']
And if you needed the size to be variable, you can just add in a size argument:
def segmentString(s, c, size):
s = s + c * (size - len(s) % size)
return [s[i:i+size] for i in range(0,len(s),size)]
>>> segmentString("Hello, World!","-",4)
['Hell', 'o, W', 'orld', '!---']
add a comment |
up vote
1
down vote
Yet another possibility:
def segmentString(s1, x):
ret_list =
while len(s1) > 8:
ret_list.append(s1[:8])
s1 = s1[8:]
if len(s1) > 0:
ret_list.append(s1 + x*(8-len(s1)))
return ret_list
add a comment |
up vote
0
down vote
I am also new to programming but I think you could use this...
Word = "Hello, World!---"
print([Word[i:i+x] for i in range(0, len(Word), x)])
Just change x to whatever value you want..
Check out https://www.google.co.in/amp/s/www.geeksforgeeks.org/python-string-split/amp/
Hope it helps.
I think that the issue is that the word is not"Hello, World!---"
, but"Hello, World!"
, and it needs to be split into 8 character chunks, with-
as a filler when the last chunk is not 8 characters long
– sacul
Nov 10 at 1:53
Still do not understand at all..
– Toolazytothinkofaname
Nov 10 at 2:01
add a comment |
up vote
0
down vote
Here is the simple answer that you can understand that solves your problem with few simple ifs:
a= 'abcdefghijklmdddn'
def segmentString(stuff):
c=
i=0
hold=""
for letter in stuff:
i+=1
hold+=letter
if i%8==0:
c.append(hold)
hold=""
c.append(hold)
if hold=="":
print(c)
else:
if hold==c[-1]:
print(c)
else:
c.append(hold)
print(c)
add a comment |
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
3
down vote
accepted
You can use a slightly modified version of this chunking recipe:
def chunks(L, n, char):
for i in range(0, len(L), n):
yield L[i:i + n].ljust(n, char)
res = list(chunks('Hello, World!', 8, '-'))
['Hello, W', 'orld!---']
str.ljust
performs the necessary padding.
1
Easier way to write the padding step:yield x.ljust(n, char)
. No need to perform the calculations for required padding yourself over and over. And because it doesn't even need to know the original length ofx
, you could one-line the body of the loop toyield L[i:i+n].ljust(n, char)
– ShadowRanger
Nov 10 at 1:56
@ShadowRanger, Good point, forgot aboutstr.ljust
!
– jpp
Nov 10 at 1:56
add a comment |
up vote
3
down vote
accepted
You can use a slightly modified version of this chunking recipe:
def chunks(L, n, char):
for i in range(0, len(L), n):
yield L[i:i + n].ljust(n, char)
res = list(chunks('Hello, World!', 8, '-'))
['Hello, W', 'orld!---']
str.ljust
performs the necessary padding.
1
Easier way to write the padding step:yield x.ljust(n, char)
. No need to perform the calculations for required padding yourself over and over. And because it doesn't even need to know the original length ofx
, you could one-line the body of the loop toyield L[i:i+n].ljust(n, char)
– ShadowRanger
Nov 10 at 1:56
@ShadowRanger, Good point, forgot aboutstr.ljust
!
– jpp
Nov 10 at 1:56
add a comment |
up vote
3
down vote
accepted
up vote
3
down vote
accepted
You can use a slightly modified version of this chunking recipe:
def chunks(L, n, char):
for i in range(0, len(L), n):
yield L[i:i + n].ljust(n, char)
res = list(chunks('Hello, World!', 8, '-'))
['Hello, W', 'orld!---']
str.ljust
performs the necessary padding.
You can use a slightly modified version of this chunking recipe:
def chunks(L, n, char):
for i in range(0, len(L), n):
yield L[i:i + n].ljust(n, char)
res = list(chunks('Hello, World!', 8, '-'))
['Hello, W', 'orld!---']
str.ljust
performs the necessary padding.
edited Nov 10 at 1:58
answered Nov 10 at 1:33


jpp
85.2k194897
85.2k194897
1
Easier way to write the padding step:yield x.ljust(n, char)
. No need to perform the calculations for required padding yourself over and over. And because it doesn't even need to know the original length ofx
, you could one-line the body of the loop toyield L[i:i+n].ljust(n, char)
– ShadowRanger
Nov 10 at 1:56
@ShadowRanger, Good point, forgot aboutstr.ljust
!
– jpp
Nov 10 at 1:56
add a comment |
1
Easier way to write the padding step:yield x.ljust(n, char)
. No need to perform the calculations for required padding yourself over and over. And because it doesn't even need to know the original length ofx
, you could one-line the body of the loop toyield L[i:i+n].ljust(n, char)
– ShadowRanger
Nov 10 at 1:56
@ShadowRanger, Good point, forgot aboutstr.ljust
!
– jpp
Nov 10 at 1:56
1
1
Easier way to write the padding step:
yield x.ljust(n, char)
. No need to perform the calculations for required padding yourself over and over. And because it doesn't even need to know the original length of x
, you could one-line the body of the loop to yield L[i:i+n].ljust(n, char)
– ShadowRanger
Nov 10 at 1:56
Easier way to write the padding step:
yield x.ljust(n, char)
. No need to perform the calculations for required padding yourself over and over. And because it doesn't even need to know the original length of x
, you could one-line the body of the loop to yield L[i:i+n].ljust(n, char)
– ShadowRanger
Nov 10 at 1:56
@ShadowRanger, Good point, forgot about
str.ljust
!– jpp
Nov 10 at 1:56
@ShadowRanger, Good point, forgot about
str.ljust
!– jpp
Nov 10 at 1:56
add a comment |
up vote
1
down vote
A slightly modified version of @jpp's answer is to first pad the string with your fill character, so that the length is cleanly divisible by 8:
def segmentString(s, c):
s = s + c * (8 - len(s) % 8)
return [s[i:i+8] for i in range(0, len(s), 8)]
>>> segmentString("Hello, World!","-")
['Hello, W', 'orld!---']
And if you needed the size to be variable, you can just add in a size argument:
def segmentString(s, c, size):
s = s + c * (size - len(s) % size)
return [s[i:i+size] for i in range(0,len(s),size)]
>>> segmentString("Hello, World!","-",4)
['Hell', 'o, W', 'orld', '!---']
add a comment |
up vote
1
down vote
A slightly modified version of @jpp's answer is to first pad the string with your fill character, so that the length is cleanly divisible by 8:
def segmentString(s, c):
s = s + c * (8 - len(s) % 8)
return [s[i:i+8] for i in range(0, len(s), 8)]
>>> segmentString("Hello, World!","-")
['Hello, W', 'orld!---']
And if you needed the size to be variable, you can just add in a size argument:
def segmentString(s, c, size):
s = s + c * (size - len(s) % size)
return [s[i:i+size] for i in range(0,len(s),size)]
>>> segmentString("Hello, World!","-",4)
['Hell', 'o, W', 'orld', '!---']
add a comment |
up vote
1
down vote
up vote
1
down vote
A slightly modified version of @jpp's answer is to first pad the string with your fill character, so that the length is cleanly divisible by 8:
def segmentString(s, c):
s = s + c * (8 - len(s) % 8)
return [s[i:i+8] for i in range(0, len(s), 8)]
>>> segmentString("Hello, World!","-")
['Hello, W', 'orld!---']
And if you needed the size to be variable, you can just add in a size argument:
def segmentString(s, c, size):
s = s + c * (size - len(s) % size)
return [s[i:i+size] for i in range(0,len(s),size)]
>>> segmentString("Hello, World!","-",4)
['Hell', 'o, W', 'orld', '!---']
A slightly modified version of @jpp's answer is to first pad the string with your fill character, so that the length is cleanly divisible by 8:
def segmentString(s, c):
s = s + c * (8 - len(s) % 8)
return [s[i:i+8] for i in range(0, len(s), 8)]
>>> segmentString("Hello, World!","-")
['Hello, W', 'orld!---']
And if you needed the size to be variable, you can just add in a size argument:
def segmentString(s, c, size):
s = s + c * (size - len(s) % size)
return [s[i:i+size] for i in range(0,len(s),size)]
>>> segmentString("Hello, World!","-",4)
['Hell', 'o, W', 'orld', '!---']
answered Nov 10 at 1:48


sacul
27.7k41639
27.7k41639
add a comment |
add a comment |
up vote
1
down vote
Yet another possibility:
def segmentString(s1, x):
ret_list =
while len(s1) > 8:
ret_list.append(s1[:8])
s1 = s1[8:]
if len(s1) > 0:
ret_list.append(s1 + x*(8-len(s1)))
return ret_list
add a comment |
up vote
1
down vote
Yet another possibility:
def segmentString(s1, x):
ret_list =
while len(s1) > 8:
ret_list.append(s1[:8])
s1 = s1[8:]
if len(s1) > 0:
ret_list.append(s1 + x*(8-len(s1)))
return ret_list
add a comment |
up vote
1
down vote
up vote
1
down vote
Yet another possibility:
def segmentString(s1, x):
ret_list =
while len(s1) > 8:
ret_list.append(s1[:8])
s1 = s1[8:]
if len(s1) > 0:
ret_list.append(s1 + x*(8-len(s1)))
return ret_list
Yet another possibility:
def segmentString(s1, x):
ret_list =
while len(s1) > 8:
ret_list.append(s1[:8])
s1 = s1[8:]
if len(s1) > 0:
ret_list.append(s1 + x*(8-len(s1)))
return ret_list
answered Nov 10 at 1:53


John Anderson
2,0581312
2,0581312
add a comment |
add a comment |
up vote
0
down vote
I am also new to programming but I think you could use this...
Word = "Hello, World!---"
print([Word[i:i+x] for i in range(0, len(Word), x)])
Just change x to whatever value you want..
Check out https://www.google.co.in/amp/s/www.geeksforgeeks.org/python-string-split/amp/
Hope it helps.
I think that the issue is that the word is not"Hello, World!---"
, but"Hello, World!"
, and it needs to be split into 8 character chunks, with-
as a filler when the last chunk is not 8 characters long
– sacul
Nov 10 at 1:53
Still do not understand at all..
– Toolazytothinkofaname
Nov 10 at 2:01
add a comment |
up vote
0
down vote
I am also new to programming but I think you could use this...
Word = "Hello, World!---"
print([Word[i:i+x] for i in range(0, len(Word), x)])
Just change x to whatever value you want..
Check out https://www.google.co.in/amp/s/www.geeksforgeeks.org/python-string-split/amp/
Hope it helps.
I think that the issue is that the word is not"Hello, World!---"
, but"Hello, World!"
, and it needs to be split into 8 character chunks, with-
as a filler when the last chunk is not 8 characters long
– sacul
Nov 10 at 1:53
Still do not understand at all..
– Toolazytothinkofaname
Nov 10 at 2:01
add a comment |
up vote
0
down vote
up vote
0
down vote
I am also new to programming but I think you could use this...
Word = "Hello, World!---"
print([Word[i:i+x] for i in range(0, len(Word), x)])
Just change x to whatever value you want..
Check out https://www.google.co.in/amp/s/www.geeksforgeeks.org/python-string-split/amp/
Hope it helps.
I am also new to programming but I think you could use this...
Word = "Hello, World!---"
print([Word[i:i+x] for i in range(0, len(Word), x)])
Just change x to whatever value you want..
Check out https://www.google.co.in/amp/s/www.geeksforgeeks.org/python-string-split/amp/
Hope it helps.
edited Nov 10 at 1:54


sacul
27.7k41639
27.7k41639
answered Nov 10 at 1:50
Toolazytothinkofaname
112
112
I think that the issue is that the word is not"Hello, World!---"
, but"Hello, World!"
, and it needs to be split into 8 character chunks, with-
as a filler when the last chunk is not 8 characters long
– sacul
Nov 10 at 1:53
Still do not understand at all..
– Toolazytothinkofaname
Nov 10 at 2:01
add a comment |
I think that the issue is that the word is not"Hello, World!---"
, but"Hello, World!"
, and it needs to be split into 8 character chunks, with-
as a filler when the last chunk is not 8 characters long
– sacul
Nov 10 at 1:53
Still do not understand at all..
– Toolazytothinkofaname
Nov 10 at 2:01
I think that the issue is that the word is not
"Hello, World!---"
, but "Hello, World!"
, and it needs to be split into 8 character chunks, with -
as a filler when the last chunk is not 8 characters long– sacul
Nov 10 at 1:53
I think that the issue is that the word is not
"Hello, World!---"
, but "Hello, World!"
, and it needs to be split into 8 character chunks, with -
as a filler when the last chunk is not 8 characters long– sacul
Nov 10 at 1:53
Still do not understand at all..
– Toolazytothinkofaname
Nov 10 at 2:01
Still do not understand at all..
– Toolazytothinkofaname
Nov 10 at 2:01
add a comment |
up vote
0
down vote
Here is the simple answer that you can understand that solves your problem with few simple ifs:
a= 'abcdefghijklmdddn'
def segmentString(stuff):
c=
i=0
hold=""
for letter in stuff:
i+=1
hold+=letter
if i%8==0:
c.append(hold)
hold=""
c.append(hold)
if hold=="":
print(c)
else:
if hold==c[-1]:
print(c)
else:
c.append(hold)
print(c)
add a comment |
up vote
0
down vote
Here is the simple answer that you can understand that solves your problem with few simple ifs:
a= 'abcdefghijklmdddn'
def segmentString(stuff):
c=
i=0
hold=""
for letter in stuff:
i+=1
hold+=letter
if i%8==0:
c.append(hold)
hold=""
c.append(hold)
if hold=="":
print(c)
else:
if hold==c[-1]:
print(c)
else:
c.append(hold)
print(c)
add a comment |
up vote
0
down vote
up vote
0
down vote
Here is the simple answer that you can understand that solves your problem with few simple ifs:
a= 'abcdefghijklmdddn'
def segmentString(stuff):
c=
i=0
hold=""
for letter in stuff:
i+=1
hold+=letter
if i%8==0:
c.append(hold)
hold=""
c.append(hold)
if hold=="":
print(c)
else:
if hold==c[-1]:
print(c)
else:
c.append(hold)
print(c)
Here is the simple answer that you can understand that solves your problem with few simple ifs:
a= 'abcdefghijklmdddn'
def segmentString(stuff):
c=
i=0
hold=""
for letter in stuff:
i+=1
hold+=letter
if i%8==0:
c.append(hold)
hold=""
c.append(hold)
if hold=="":
print(c)
else:
if hold==c[-1]:
print(c)
else:
c.append(hold)
print(c)
answered Nov 10 at 2:05


Marko Maksimovic
46
46
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53235256%2ffunction-that-takes-a-string-and-returns-a-list-of-8-character-strings%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
QSWUjfyanuAF62Gw0,RwJ7XMd9CAedKwkCb UQNQaDV