Update an existing array element with jsonnet
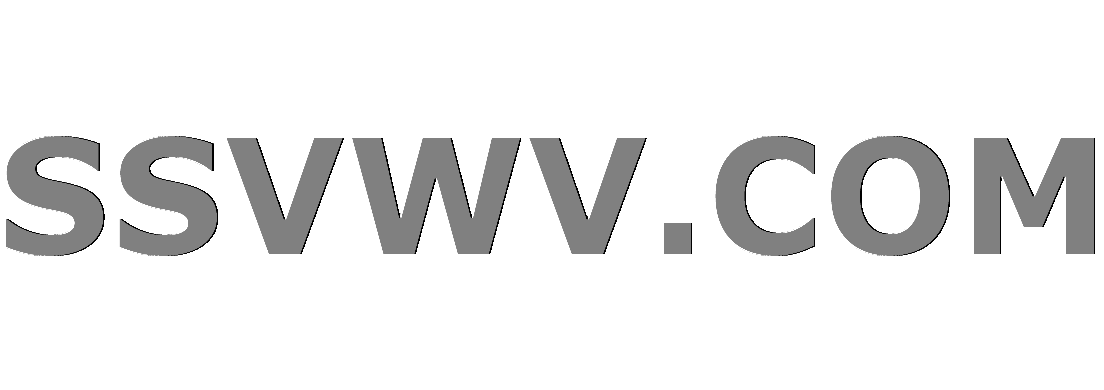
Multi tool use
I am using jsonnet to read in a value which consists of an array. I want to modify the first element in that array to add a value. The data structure looks like this:
"my_value": [
"env": "something"
,
"var": "bar"
]
I want to add a value to my_value[0]
. How can I reference that in jsonnet?
jsonnet
add a comment |
I am using jsonnet to read in a value which consists of an array. I want to modify the first element in that array to add a value. The data structure looks like this:
"my_value": [
"env": "something"
,
"var": "bar"
]
I want to add a value to my_value[0]
. How can I reference that in jsonnet?
jsonnet
add a comment |
I am using jsonnet to read in a value which consists of an array. I want to modify the first element in that array to add a value. The data structure looks like this:
"my_value": [
"env": "something"
,
"var": "bar"
]
I want to add a value to my_value[0]
. How can I reference that in jsonnet?
jsonnet
I am using jsonnet to read in a value which consists of an array. I want to modify the first element in that array to add a value. The data structure looks like this:
"my_value": [
"env": "something"
,
"var": "bar"
]
I want to add a value to my_value[0]
. How can I reference that in jsonnet?
jsonnet
jsonnet
asked Jul 9 '18 at 23:41
jaxxstormjaxxstorm
4,79511731
4,79511731
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
A possible approach using https://jsonnet.org/ref/stdlib.html#mapWithIndex as per below:
$ cat foo.jsonnet
local my_array = [
env: "something",
,
var: "bar",
,
];
local add_by_idx(idx) = (
if idx == 0 then extra: "stuff" else
);
std.mapWithIndex(function(i, v) v + add_by_idx(i), my_array)
$ jsonnet foo.jsonnet
[
"env": "something",
"extra": "stuff"
,
"var": "bar"
]
add a comment |
A IMO better approach: converting the array
to object
for easier overloading, then ab-using the fact that jsonnet handles objects by alpha-sorting its keys:
$ cat foo.jsonnet
local array_a = [
env: "something" ,
var: "bar" ,
];
local array_b = [
,
extra: "stuff" ,
];
// "Standarize" index key string as "%06d"
local _idx(i) = ("%06d" % [i]);
// Convert array [elem1, elem2, ...] to object as 000000: elem0, 000001: elem1, ...
local _to_obj(a) = std.foldl(
function(x, y) x + y,
std.mapWithIndex(function(i, v) [_idx(i)]: v , a),
);
local _to_array(obj) = [obj[k] for k in std.objectFields(obj)];
_to_array(std.mergePatch(_to_obj(array_a), _to_obj(array_b)))
$ jsonnet foo.jsonnet
[
"env": "something"
,
"extra": "stuff",
"var": "bar"
]
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f51255389%2fupdate-an-existing-array-element-with-jsonnet%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
A possible approach using https://jsonnet.org/ref/stdlib.html#mapWithIndex as per below:
$ cat foo.jsonnet
local my_array = [
env: "something",
,
var: "bar",
,
];
local add_by_idx(idx) = (
if idx == 0 then extra: "stuff" else
);
std.mapWithIndex(function(i, v) v + add_by_idx(i), my_array)
$ jsonnet foo.jsonnet
[
"env": "something",
"extra": "stuff"
,
"var": "bar"
]
add a comment |
A possible approach using https://jsonnet.org/ref/stdlib.html#mapWithIndex as per below:
$ cat foo.jsonnet
local my_array = [
env: "something",
,
var: "bar",
,
];
local add_by_idx(idx) = (
if idx == 0 then extra: "stuff" else
);
std.mapWithIndex(function(i, v) v + add_by_idx(i), my_array)
$ jsonnet foo.jsonnet
[
"env": "something",
"extra": "stuff"
,
"var": "bar"
]
add a comment |
A possible approach using https://jsonnet.org/ref/stdlib.html#mapWithIndex as per below:
$ cat foo.jsonnet
local my_array = [
env: "something",
,
var: "bar",
,
];
local add_by_idx(idx) = (
if idx == 0 then extra: "stuff" else
);
std.mapWithIndex(function(i, v) v + add_by_idx(i), my_array)
$ jsonnet foo.jsonnet
[
"env": "something",
"extra": "stuff"
,
"var": "bar"
]
A possible approach using https://jsonnet.org/ref/stdlib.html#mapWithIndex as per below:
$ cat foo.jsonnet
local my_array = [
env: "something",
,
var: "bar",
,
];
local add_by_idx(idx) = (
if idx == 0 then extra: "stuff" else
);
std.mapWithIndex(function(i, v) v + add_by_idx(i), my_array)
$ jsonnet foo.jsonnet
[
"env": "something",
"extra": "stuff"
,
"var": "bar"
]
edited Jul 10 '18 at 0:23
answered Jul 10 '18 at 0:14
jjojjo
5938
5938
add a comment |
add a comment |
A IMO better approach: converting the array
to object
for easier overloading, then ab-using the fact that jsonnet handles objects by alpha-sorting its keys:
$ cat foo.jsonnet
local array_a = [
env: "something" ,
var: "bar" ,
];
local array_b = [
,
extra: "stuff" ,
];
// "Standarize" index key string as "%06d"
local _idx(i) = ("%06d" % [i]);
// Convert array [elem1, elem2, ...] to object as 000000: elem0, 000001: elem1, ...
local _to_obj(a) = std.foldl(
function(x, y) x + y,
std.mapWithIndex(function(i, v) [_idx(i)]: v , a),
);
local _to_array(obj) = [obj[k] for k in std.objectFields(obj)];
_to_array(std.mergePatch(_to_obj(array_a), _to_obj(array_b)))
$ jsonnet foo.jsonnet
[
"env": "something"
,
"extra": "stuff",
"var": "bar"
]
add a comment |
A IMO better approach: converting the array
to object
for easier overloading, then ab-using the fact that jsonnet handles objects by alpha-sorting its keys:
$ cat foo.jsonnet
local array_a = [
env: "something" ,
var: "bar" ,
];
local array_b = [
,
extra: "stuff" ,
];
// "Standarize" index key string as "%06d"
local _idx(i) = ("%06d" % [i]);
// Convert array [elem1, elem2, ...] to object as 000000: elem0, 000001: elem1, ...
local _to_obj(a) = std.foldl(
function(x, y) x + y,
std.mapWithIndex(function(i, v) [_idx(i)]: v , a),
);
local _to_array(obj) = [obj[k] for k in std.objectFields(obj)];
_to_array(std.mergePatch(_to_obj(array_a), _to_obj(array_b)))
$ jsonnet foo.jsonnet
[
"env": "something"
,
"extra": "stuff",
"var": "bar"
]
add a comment |
A IMO better approach: converting the array
to object
for easier overloading, then ab-using the fact that jsonnet handles objects by alpha-sorting its keys:
$ cat foo.jsonnet
local array_a = [
env: "something" ,
var: "bar" ,
];
local array_b = [
,
extra: "stuff" ,
];
// "Standarize" index key string as "%06d"
local _idx(i) = ("%06d" % [i]);
// Convert array [elem1, elem2, ...] to object as 000000: elem0, 000001: elem1, ...
local _to_obj(a) = std.foldl(
function(x, y) x + y,
std.mapWithIndex(function(i, v) [_idx(i)]: v , a),
);
local _to_array(obj) = [obj[k] for k in std.objectFields(obj)];
_to_array(std.mergePatch(_to_obj(array_a), _to_obj(array_b)))
$ jsonnet foo.jsonnet
[
"env": "something"
,
"extra": "stuff",
"var": "bar"
]
A IMO better approach: converting the array
to object
for easier overloading, then ab-using the fact that jsonnet handles objects by alpha-sorting its keys:
$ cat foo.jsonnet
local array_a = [
env: "something" ,
var: "bar" ,
];
local array_b = [
,
extra: "stuff" ,
];
// "Standarize" index key string as "%06d"
local _idx(i) = ("%06d" % [i]);
// Convert array [elem1, elem2, ...] to object as 000000: elem0, 000001: elem1, ...
local _to_obj(a) = std.foldl(
function(x, y) x + y,
std.mapWithIndex(function(i, v) [_idx(i)]: v , a),
);
local _to_array(obj) = [obj[k] for k in std.objectFields(obj)];
_to_array(std.mergePatch(_to_obj(array_a), _to_obj(array_b)))
$ jsonnet foo.jsonnet
[
"env": "something"
,
"extra": "stuff",
"var": "bar"
]
edited Nov 12 '18 at 21:09
answered Nov 12 '18 at 14:22
jjojjo
5938
5938
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f51255389%2fupdate-an-existing-array-element-with-jsonnet%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fJvn0wGtlDpnxLpTDHzQ60M,r4IT 1lNVYble,D8k8sH,LhTSyGtbRq,0tgZBRtzukZuCXWGWyLpJ5EWGJLciMuQVNen2555JNmbIG1z