What is faster? Firing a query within a loop or looping through an array?
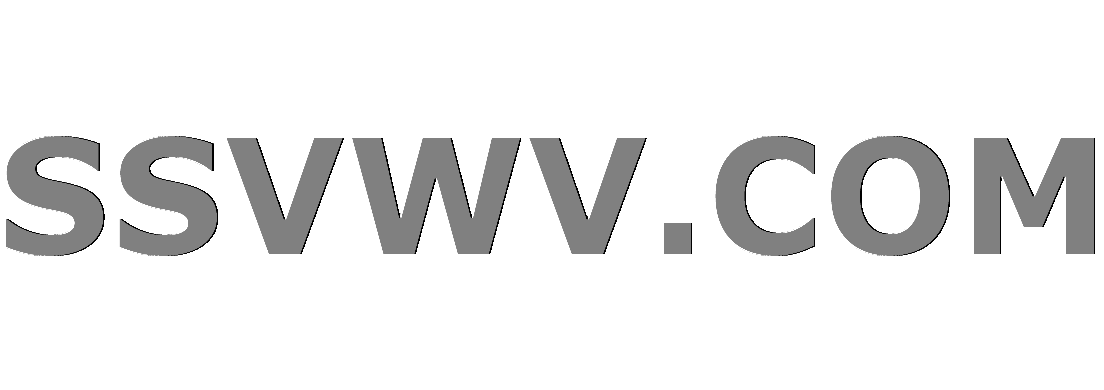
Multi tool use
I was just asking myself what is faster: I have a for loop where I have to check a certain table column against my loop variable.
Now I have two options: Within the loop I fire a query for each iteration and check my results. The second option is to fetch all the rows I need, and within my for-loop I run a foreach-loop and check if I have a valid match between my desired field and my loop variable.
It's a bit hard to explain but I just let the code speak. I don't use real code here within the loops but fictional function calls by way of illustration.
Option 1: Querying the database for each iteration.
<?php
// Option 1:
for($i = 0; $i < 5; $i++)
$query_result = query_database_where_field_equals($i);
if($query_result)
echo "Here is something";
else
echo "Here is nothing";
// Option 2:
$results = query_database_and_get_all_results();
for($i = 0; $i < 5; $i++)
foreach($results AS $result)
if($result['desired_field'] == $i)
echo "Here is something";
else
echo "Here is nothing";
?>
Both ways will work, but I'm pretty sure it's a worse practice to fire queries within a loop in a performant point of view.
But using a foreach loop within the for-loop also seems to be a bit odd to me.
So what is the prefered way of doing this?
Edit - Added information
I have a for loop that needs to run several times. The amount is static (e.g. 5) and does not depend on any records in the database. Each iteration represents a position.
I have a database table which might store information about what a position contains.
Example:
positions (id, position)
So an example record might be:
positions (1, 2)
That means that I have something on position 2. What I want to do in my loop basically is, to check whether I got a record for that position or not. Expected output would be:
Here is nothing
Here is nothing
Here is something
Here is nothing
Here is nothing
php mysql performance nested-loops
add a comment |
I was just asking myself what is faster: I have a for loop where I have to check a certain table column against my loop variable.
Now I have two options: Within the loop I fire a query for each iteration and check my results. The second option is to fetch all the rows I need, and within my for-loop I run a foreach-loop and check if I have a valid match between my desired field and my loop variable.
It's a bit hard to explain but I just let the code speak. I don't use real code here within the loops but fictional function calls by way of illustration.
Option 1: Querying the database for each iteration.
<?php
// Option 1:
for($i = 0; $i < 5; $i++)
$query_result = query_database_where_field_equals($i);
if($query_result)
echo "Here is something";
else
echo "Here is nothing";
// Option 2:
$results = query_database_and_get_all_results();
for($i = 0; $i < 5; $i++)
foreach($results AS $result)
if($result['desired_field'] == $i)
echo "Here is something";
else
echo "Here is nothing";
?>
Both ways will work, but I'm pretty sure it's a worse practice to fire queries within a loop in a performant point of view.
But using a foreach loop within the for-loop also seems to be a bit odd to me.
So what is the prefered way of doing this?
Edit - Added information
I have a for loop that needs to run several times. The amount is static (e.g. 5) and does not depend on any records in the database. Each iteration represents a position.
I have a database table which might store information about what a position contains.
Example:
positions (id, position)
So an example record might be:
positions (1, 2)
That means that I have something on position 2. What I want to do in my loop basically is, to check whether I got a record for that position or not. Expected output would be:
Here is nothing
Here is nothing
Here is something
Here is nothing
Here is nothing
php mysql performance nested-loops
add a comment |
I was just asking myself what is faster: I have a for loop where I have to check a certain table column against my loop variable.
Now I have two options: Within the loop I fire a query for each iteration and check my results. The second option is to fetch all the rows I need, and within my for-loop I run a foreach-loop and check if I have a valid match between my desired field and my loop variable.
It's a bit hard to explain but I just let the code speak. I don't use real code here within the loops but fictional function calls by way of illustration.
Option 1: Querying the database for each iteration.
<?php
// Option 1:
for($i = 0; $i < 5; $i++)
$query_result = query_database_where_field_equals($i);
if($query_result)
echo "Here is something";
else
echo "Here is nothing";
// Option 2:
$results = query_database_and_get_all_results();
for($i = 0; $i < 5; $i++)
foreach($results AS $result)
if($result['desired_field'] == $i)
echo "Here is something";
else
echo "Here is nothing";
?>
Both ways will work, but I'm pretty sure it's a worse practice to fire queries within a loop in a performant point of view.
But using a foreach loop within the for-loop also seems to be a bit odd to me.
So what is the prefered way of doing this?
Edit - Added information
I have a for loop that needs to run several times. The amount is static (e.g. 5) and does not depend on any records in the database. Each iteration represents a position.
I have a database table which might store information about what a position contains.
Example:
positions (id, position)
So an example record might be:
positions (1, 2)
That means that I have something on position 2. What I want to do in my loop basically is, to check whether I got a record for that position or not. Expected output would be:
Here is nothing
Here is nothing
Here is something
Here is nothing
Here is nothing
php mysql performance nested-loops
I was just asking myself what is faster: I have a for loop where I have to check a certain table column against my loop variable.
Now I have two options: Within the loop I fire a query for each iteration and check my results. The second option is to fetch all the rows I need, and within my for-loop I run a foreach-loop and check if I have a valid match between my desired field and my loop variable.
It's a bit hard to explain but I just let the code speak. I don't use real code here within the loops but fictional function calls by way of illustration.
Option 1: Querying the database for each iteration.
<?php
// Option 1:
for($i = 0; $i < 5; $i++)
$query_result = query_database_where_field_equals($i);
if($query_result)
echo "Here is something";
else
echo "Here is nothing";
// Option 2:
$results = query_database_and_get_all_results();
for($i = 0; $i < 5; $i++)
foreach($results AS $result)
if($result['desired_field'] == $i)
echo "Here is something";
else
echo "Here is nothing";
?>
Both ways will work, but I'm pretty sure it's a worse practice to fire queries within a loop in a performant point of view.
But using a foreach loop within the for-loop also seems to be a bit odd to me.
So what is the prefered way of doing this?
Edit - Added information
I have a for loop that needs to run several times. The amount is static (e.g. 5) and does not depend on any records in the database. Each iteration represents a position.
I have a database table which might store information about what a position contains.
Example:
positions (id, position)
So an example record might be:
positions (1, 2)
That means that I have something on position 2. What I want to do in my loop basically is, to check whether I got a record for that position or not. Expected output would be:
Here is nothing
Here is nothing
Here is something
Here is nothing
Here is nothing
php mysql performance nested-loops
php mysql performance nested-loops
edited Nov 12 '18 at 4:42
Cœur
17.5k9104145
17.5k9104145
asked Jun 19 '13 at 16:00
thplthpl
4,46422043
4,46422043
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
Both previous answers are correct.
@Euantorano: Code iteration is preferable over querying. You'll avoid DB access which is slower and you'll avoid overloading the DB.
Still, as Slaks said, you could add the WHERE condition and that would reduce your array. Improving performance at the loop for checking existence.
Finally I would suggest to get a single bucle to index the results, so you can just check if the results are set.
$res = array();
$results = query_database_and_get_all_results();
for($results as $r)
$res[$result['desired_field']] = $r;
for($i = 0; $i < 5; $i++)
echo (isset($res[i]) ? "Here is something" : "Here is nothing");
This code gives you a constant preformance based on the size of the array + your second bucle.
Depending on your needs a better query could actually bring better performance, but this is based on your specific question.
Hope it helps!
So simple but so effective! That's a solution that "feels right". As I said so simple but I just could not come up with this! :) Thanks
– thpl
Jun 19 '13 at 20:56
add a comment |
The second option is the preferable of the two given options, but knowing some more information about exactly what it is you're looping through and querying might help you get a better answer.
I added some additional information and added the expected output. Thanks :)
– thpl
Jun 19 '13 at 16:15
add a comment |
Neither.
You should put the loop into the query, using WHERE field IN (0, 1, 2, 3, 4)
I think you got me wrong (No surprise. My English is horrible). I need the for-loop since the amount of iteration is fixed/static. Even if there are no records for the given id I want to display data.
– thpl
Jun 19 '13 at 16:19
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f17195930%2fwhat-is-faster-firing-a-query-within-a-loop-or-looping-through-an-array%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Both previous answers are correct.
@Euantorano: Code iteration is preferable over querying. You'll avoid DB access which is slower and you'll avoid overloading the DB.
Still, as Slaks said, you could add the WHERE condition and that would reduce your array. Improving performance at the loop for checking existence.
Finally I would suggest to get a single bucle to index the results, so you can just check if the results are set.
$res = array();
$results = query_database_and_get_all_results();
for($results as $r)
$res[$result['desired_field']] = $r;
for($i = 0; $i < 5; $i++)
echo (isset($res[i]) ? "Here is something" : "Here is nothing");
This code gives you a constant preformance based on the size of the array + your second bucle.
Depending on your needs a better query could actually bring better performance, but this is based on your specific question.
Hope it helps!
So simple but so effective! That's a solution that "feels right". As I said so simple but I just could not come up with this! :) Thanks
– thpl
Jun 19 '13 at 20:56
add a comment |
Both previous answers are correct.
@Euantorano: Code iteration is preferable over querying. You'll avoid DB access which is slower and you'll avoid overloading the DB.
Still, as Slaks said, you could add the WHERE condition and that would reduce your array. Improving performance at the loop for checking existence.
Finally I would suggest to get a single bucle to index the results, so you can just check if the results are set.
$res = array();
$results = query_database_and_get_all_results();
for($results as $r)
$res[$result['desired_field']] = $r;
for($i = 0; $i < 5; $i++)
echo (isset($res[i]) ? "Here is something" : "Here is nothing");
This code gives you a constant preformance based on the size of the array + your second bucle.
Depending on your needs a better query could actually bring better performance, but this is based on your specific question.
Hope it helps!
So simple but so effective! That's a solution that "feels right". As I said so simple but I just could not come up with this! :) Thanks
– thpl
Jun 19 '13 at 20:56
add a comment |
Both previous answers are correct.
@Euantorano: Code iteration is preferable over querying. You'll avoid DB access which is slower and you'll avoid overloading the DB.
Still, as Slaks said, you could add the WHERE condition and that would reduce your array. Improving performance at the loop for checking existence.
Finally I would suggest to get a single bucle to index the results, so you can just check if the results are set.
$res = array();
$results = query_database_and_get_all_results();
for($results as $r)
$res[$result['desired_field']] = $r;
for($i = 0; $i < 5; $i++)
echo (isset($res[i]) ? "Here is something" : "Here is nothing");
This code gives you a constant preformance based on the size of the array + your second bucle.
Depending on your needs a better query could actually bring better performance, but this is based on your specific question.
Hope it helps!
Both previous answers are correct.
@Euantorano: Code iteration is preferable over querying. You'll avoid DB access which is slower and you'll avoid overloading the DB.
Still, as Slaks said, you could add the WHERE condition and that would reduce your array. Improving performance at the loop for checking existence.
Finally I would suggest to get a single bucle to index the results, so you can just check if the results are set.
$res = array();
$results = query_database_and_get_all_results();
for($results as $r)
$res[$result['desired_field']] = $r;
for($i = 0; $i < 5; $i++)
echo (isset($res[i]) ? "Here is something" : "Here is nothing");
This code gives you a constant preformance based on the size of the array + your second bucle.
Depending on your needs a better query could actually bring better performance, but this is based on your specific question.
Hope it helps!
answered Jun 19 '13 at 18:28
riverinyoriverinyo
15627
15627
So simple but so effective! That's a solution that "feels right". As I said so simple but I just could not come up with this! :) Thanks
– thpl
Jun 19 '13 at 20:56
add a comment |
So simple but so effective! That's a solution that "feels right". As I said so simple but I just could not come up with this! :) Thanks
– thpl
Jun 19 '13 at 20:56
So simple but so effective! That's a solution that "feels right". As I said so simple but I just could not come up with this! :) Thanks
– thpl
Jun 19 '13 at 20:56
So simple but so effective! That's a solution that "feels right". As I said so simple but I just could not come up with this! :) Thanks
– thpl
Jun 19 '13 at 20:56
add a comment |
The second option is the preferable of the two given options, but knowing some more information about exactly what it is you're looping through and querying might help you get a better answer.
I added some additional information and added the expected output. Thanks :)
– thpl
Jun 19 '13 at 16:15
add a comment |
The second option is the preferable of the two given options, but knowing some more information about exactly what it is you're looping through and querying might help you get a better answer.
I added some additional information and added the expected output. Thanks :)
– thpl
Jun 19 '13 at 16:15
add a comment |
The second option is the preferable of the two given options, but knowing some more information about exactly what it is you're looping through and querying might help you get a better answer.
The second option is the preferable of the two given options, but knowing some more information about exactly what it is you're looping through and querying might help you get a better answer.
answered Jun 19 '13 at 16:03
euantoranoeuantorano
90131224
90131224
I added some additional information and added the expected output. Thanks :)
– thpl
Jun 19 '13 at 16:15
add a comment |
I added some additional information and added the expected output. Thanks :)
– thpl
Jun 19 '13 at 16:15
I added some additional information and added the expected output. Thanks :)
– thpl
Jun 19 '13 at 16:15
I added some additional information and added the expected output. Thanks :)
– thpl
Jun 19 '13 at 16:15
add a comment |
Neither.
You should put the loop into the query, using WHERE field IN (0, 1, 2, 3, 4)
I think you got me wrong (No surprise. My English is horrible). I need the for-loop since the amount of iteration is fixed/static. Even if there are no records for the given id I want to display data.
– thpl
Jun 19 '13 at 16:19
add a comment |
Neither.
You should put the loop into the query, using WHERE field IN (0, 1, 2, 3, 4)
I think you got me wrong (No surprise. My English is horrible). I need the for-loop since the amount of iteration is fixed/static. Even if there are no records for the given id I want to display data.
– thpl
Jun 19 '13 at 16:19
add a comment |
Neither.
You should put the loop into the query, using WHERE field IN (0, 1, 2, 3, 4)
Neither.
You should put the loop into the query, using WHERE field IN (0, 1, 2, 3, 4)
answered Jun 19 '13 at 16:02
SLaksSLaks
679k13916281751
679k13916281751
I think you got me wrong (No surprise. My English is horrible). I need the for-loop since the amount of iteration is fixed/static. Even if there are no records for the given id I want to display data.
– thpl
Jun 19 '13 at 16:19
add a comment |
I think you got me wrong (No surprise. My English is horrible). I need the for-loop since the amount of iteration is fixed/static. Even if there are no records for the given id I want to display data.
– thpl
Jun 19 '13 at 16:19
I think you got me wrong (No surprise. My English is horrible). I need the for-loop since the amount of iteration is fixed/static. Even if there are no records for the given id I want to display data.
– thpl
Jun 19 '13 at 16:19
I think you got me wrong (No surprise. My English is horrible). I need the for-loop since the amount of iteration is fixed/static. Even if there are no records for the given id I want to display data.
– thpl
Jun 19 '13 at 16:19
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f17195930%2fwhat-is-faster-firing-a-query-within-a-loop-or-looping-through-an-array%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qyZ 4zdhZN np1N,3abBQ5hP4hjk7WpXklsZX,W9Pr8XEkL7vz8f5 fLKs