Read access violation when attempting strcpy from file buffer to char array
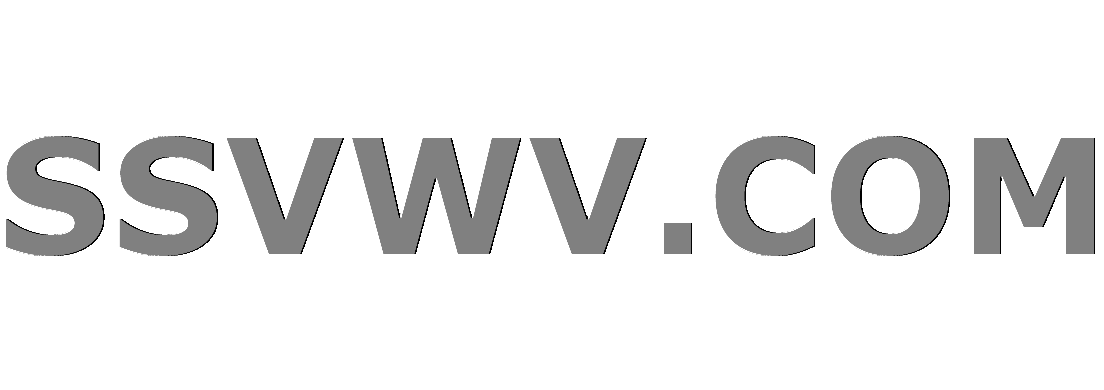
Multi tool use
I've been working on an assignment to implement hashing. In it, I read through a text file called "proteins". The problem occurs when I try to copy it to another char array. Visual Studio throws a read access violation.
#include <iostream>
#include <fstream>
using namespace std;
struct arrayelement
char protein[30];
int count;
;
arrayelement proteins[40];
int main()
char buffer[30];
// open source file
ifstream fin("proteins.txt");
if (!fin) cerr << "Input file could not be openedn"; exit(1);
// loop through strings in file
while (fin >> buffer)
int index = ((buffer[0] - 65) + (2 * (buffer[strlen(buffer)-1] - 65)) % 40);
while (true)
if (proteins[index].protein == buffer) // Found
proteins[index].count++;
break;
if (proteins[index].protein[0] == 0) // Empty
strcpy(proteins[index].protein, buffer); // <-- The error in question
proteins[index].count++;
break;
index++; // Collision
// close file
fin.close();
for (int i = 0; i <= 40; i++)
cout << proteins[i].protein << "t" << proteins[i].count << "n";
c++ arrays file char strcpy
add a comment |
I've been working on an assignment to implement hashing. In it, I read through a text file called "proteins". The problem occurs when I try to copy it to another char array. Visual Studio throws a read access violation.
#include <iostream>
#include <fstream>
using namespace std;
struct arrayelement
char protein[30];
int count;
;
arrayelement proteins[40];
int main()
char buffer[30];
// open source file
ifstream fin("proteins.txt");
if (!fin) cerr << "Input file could not be openedn"; exit(1);
// loop through strings in file
while (fin >> buffer)
int index = ((buffer[0] - 65) + (2 * (buffer[strlen(buffer)-1] - 65)) % 40);
while (true)
if (proteins[index].protein == buffer) // Found
proteins[index].count++;
break;
if (proteins[index].protein[0] == 0) // Empty
strcpy(proteins[index].protein, buffer); // <-- The error in question
proteins[index].count++;
break;
index++; // Collision
// close file
fin.close();
for (int i = 0; i <= 40; i++)
cout << proteins[i].protein << "t" << proteins[i].count << "n";
c++ arrays file char strcpy
Did you try debug this? index may be at 40 when the error occurs.
– Matthieu Brucher
Nov 13 '18 at 13:40
Also usestd::string
and astd::vector<arrayelement>
.
– Matthieu Brucher
Nov 13 '18 at 13:40
2
proteins[index].protein == buffer
This is not how you want to compare char arrays.
– 0x5453
Nov 13 '18 at 13:44
@0x5453 Oh man, I'm too tired. That did it. After changing that to a strcmp it all runs. Thank you!
– Noctimor
Nov 13 '18 at 13:50
@Noctimor Because you are trying to do C when you are in C++. Use containers....
– Matthieu Brucher
Nov 13 '18 at 13:51
add a comment |
I've been working on an assignment to implement hashing. In it, I read through a text file called "proteins". The problem occurs when I try to copy it to another char array. Visual Studio throws a read access violation.
#include <iostream>
#include <fstream>
using namespace std;
struct arrayelement
char protein[30];
int count;
;
arrayelement proteins[40];
int main()
char buffer[30];
// open source file
ifstream fin("proteins.txt");
if (!fin) cerr << "Input file could not be openedn"; exit(1);
// loop through strings in file
while (fin >> buffer)
int index = ((buffer[0] - 65) + (2 * (buffer[strlen(buffer)-1] - 65)) % 40);
while (true)
if (proteins[index].protein == buffer) // Found
proteins[index].count++;
break;
if (proteins[index].protein[0] == 0) // Empty
strcpy(proteins[index].protein, buffer); // <-- The error in question
proteins[index].count++;
break;
index++; // Collision
// close file
fin.close();
for (int i = 0; i <= 40; i++)
cout << proteins[i].protein << "t" << proteins[i].count << "n";
c++ arrays file char strcpy
I've been working on an assignment to implement hashing. In it, I read through a text file called "proteins". The problem occurs when I try to copy it to another char array. Visual Studio throws a read access violation.
#include <iostream>
#include <fstream>
using namespace std;
struct arrayelement
char protein[30];
int count;
;
arrayelement proteins[40];
int main()
char buffer[30];
// open source file
ifstream fin("proteins.txt");
if (!fin) cerr << "Input file could not be openedn"; exit(1);
// loop through strings in file
while (fin >> buffer)
int index = ((buffer[0] - 65) + (2 * (buffer[strlen(buffer)-1] - 65)) % 40);
while (true)
if (proteins[index].protein == buffer) // Found
proteins[index].count++;
break;
if (proteins[index].protein[0] == 0) // Empty
strcpy(proteins[index].protein, buffer); // <-- The error in question
proteins[index].count++;
break;
index++; // Collision
// close file
fin.close();
for (int i = 0; i <= 40; i++)
cout << proteins[i].protein << "t" << proteins[i].count << "n";
c++ arrays file char strcpy
c++ arrays file char strcpy
asked Nov 13 '18 at 13:39
NoctimorNoctimor
31
31
Did you try debug this? index may be at 40 when the error occurs.
– Matthieu Brucher
Nov 13 '18 at 13:40
Also usestd::string
and astd::vector<arrayelement>
.
– Matthieu Brucher
Nov 13 '18 at 13:40
2
proteins[index].protein == buffer
This is not how you want to compare char arrays.
– 0x5453
Nov 13 '18 at 13:44
@0x5453 Oh man, I'm too tired. That did it. After changing that to a strcmp it all runs. Thank you!
– Noctimor
Nov 13 '18 at 13:50
@Noctimor Because you are trying to do C when you are in C++. Use containers....
– Matthieu Brucher
Nov 13 '18 at 13:51
add a comment |
Did you try debug this? index may be at 40 when the error occurs.
– Matthieu Brucher
Nov 13 '18 at 13:40
Also usestd::string
and astd::vector<arrayelement>
.
– Matthieu Brucher
Nov 13 '18 at 13:40
2
proteins[index].protein == buffer
This is not how you want to compare char arrays.
– 0x5453
Nov 13 '18 at 13:44
@0x5453 Oh man, I'm too tired. That did it. After changing that to a strcmp it all runs. Thank you!
– Noctimor
Nov 13 '18 at 13:50
@Noctimor Because you are trying to do C when you are in C++. Use containers....
– Matthieu Brucher
Nov 13 '18 at 13:51
Did you try debug this? index may be at 40 when the error occurs.
– Matthieu Brucher
Nov 13 '18 at 13:40
Did you try debug this? index may be at 40 when the error occurs.
– Matthieu Brucher
Nov 13 '18 at 13:40
Also use
std::string
and a std::vector<arrayelement>
.– Matthieu Brucher
Nov 13 '18 at 13:40
Also use
std::string
and a std::vector<arrayelement>
.– Matthieu Brucher
Nov 13 '18 at 13:40
2
2
proteins[index].protein == buffer
This is not how you want to compare char arrays.– 0x5453
Nov 13 '18 at 13:44
proteins[index].protein == buffer
This is not how you want to compare char arrays.– 0x5453
Nov 13 '18 at 13:44
@0x5453 Oh man, I'm too tired. That did it. After changing that to a strcmp it all runs. Thank you!
– Noctimor
Nov 13 '18 at 13:50
@0x5453 Oh man, I'm too tired. That did it. After changing that to a strcmp it all runs. Thank you!
– Noctimor
Nov 13 '18 at 13:50
@Noctimor Because you are trying to do C when you are in C++. Use containers....
– Matthieu Brucher
Nov 13 '18 at 13:51
@Noctimor Because you are trying to do C when you are in C++. Use containers....
– Matthieu Brucher
Nov 13 '18 at 13:51
add a comment |
1 Answer
1
active
oldest
votes
If you get more than 30 chars here:
while (fin >> buffer) {
... or if index >= 40 here:
strcpy(proteins[index].protein, buffer);
... the program will probably crash (Undefined behavior). Also, these char*
's will not be pointing at the same address, so the comparison will fail:
proteins[index].protein == buffer
Yep, it was the comparison that did me in. After rewriting it, everything runs. Part of the code here is given per the assignment -- the examples we're working with are guaranteed not to be above 30, and the index given is double what's needed, to practice collision resolution. Otherwise I'd absolutely clean that stuff up too. Thanks!
– Noctimor
Nov 13 '18 at 13:53
Great! Note: The index formula will result in 91 if the input string is"z"
. It'll become:index = 57 + 114 % 40
=>57 + (114 % 40)
– Ted Lyngmo
Nov 13 '18 at 14:10
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282303%2fread-access-violation-when-attempting-strcpy-from-file-buffer-to-char-array%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you get more than 30 chars here:
while (fin >> buffer) {
... or if index >= 40 here:
strcpy(proteins[index].protein, buffer);
... the program will probably crash (Undefined behavior). Also, these char*
's will not be pointing at the same address, so the comparison will fail:
proteins[index].protein == buffer
Yep, it was the comparison that did me in. After rewriting it, everything runs. Part of the code here is given per the assignment -- the examples we're working with are guaranteed not to be above 30, and the index given is double what's needed, to practice collision resolution. Otherwise I'd absolutely clean that stuff up too. Thanks!
– Noctimor
Nov 13 '18 at 13:53
Great! Note: The index formula will result in 91 if the input string is"z"
. It'll become:index = 57 + 114 % 40
=>57 + (114 % 40)
– Ted Lyngmo
Nov 13 '18 at 14:10
add a comment |
If you get more than 30 chars here:
while (fin >> buffer) {
... or if index >= 40 here:
strcpy(proteins[index].protein, buffer);
... the program will probably crash (Undefined behavior). Also, these char*
's will not be pointing at the same address, so the comparison will fail:
proteins[index].protein == buffer
Yep, it was the comparison that did me in. After rewriting it, everything runs. Part of the code here is given per the assignment -- the examples we're working with are guaranteed not to be above 30, and the index given is double what's needed, to practice collision resolution. Otherwise I'd absolutely clean that stuff up too. Thanks!
– Noctimor
Nov 13 '18 at 13:53
Great! Note: The index formula will result in 91 if the input string is"z"
. It'll become:index = 57 + 114 % 40
=>57 + (114 % 40)
– Ted Lyngmo
Nov 13 '18 at 14:10
add a comment |
If you get more than 30 chars here:
while (fin >> buffer) {
... or if index >= 40 here:
strcpy(proteins[index].protein, buffer);
... the program will probably crash (Undefined behavior). Also, these char*
's will not be pointing at the same address, so the comparison will fail:
proteins[index].protein == buffer
If you get more than 30 chars here:
while (fin >> buffer) {
... or if index >= 40 here:
strcpy(proteins[index].protein, buffer);
... the program will probably crash (Undefined behavior). Also, these char*
's will not be pointing at the same address, so the comparison will fail:
proteins[index].protein == buffer
answered Nov 13 '18 at 13:47


Ted LyngmoTed Lyngmo
2,8112317
2,8112317
Yep, it was the comparison that did me in. After rewriting it, everything runs. Part of the code here is given per the assignment -- the examples we're working with are guaranteed not to be above 30, and the index given is double what's needed, to practice collision resolution. Otherwise I'd absolutely clean that stuff up too. Thanks!
– Noctimor
Nov 13 '18 at 13:53
Great! Note: The index formula will result in 91 if the input string is"z"
. It'll become:index = 57 + 114 % 40
=>57 + (114 % 40)
– Ted Lyngmo
Nov 13 '18 at 14:10
add a comment |
Yep, it was the comparison that did me in. After rewriting it, everything runs. Part of the code here is given per the assignment -- the examples we're working with are guaranteed not to be above 30, and the index given is double what's needed, to practice collision resolution. Otherwise I'd absolutely clean that stuff up too. Thanks!
– Noctimor
Nov 13 '18 at 13:53
Great! Note: The index formula will result in 91 if the input string is"z"
. It'll become:index = 57 + 114 % 40
=>57 + (114 % 40)
– Ted Lyngmo
Nov 13 '18 at 14:10
Yep, it was the comparison that did me in. After rewriting it, everything runs. Part of the code here is given per the assignment -- the examples we're working with are guaranteed not to be above 30, and the index given is double what's needed, to practice collision resolution. Otherwise I'd absolutely clean that stuff up too. Thanks!
– Noctimor
Nov 13 '18 at 13:53
Yep, it was the comparison that did me in. After rewriting it, everything runs. Part of the code here is given per the assignment -- the examples we're working with are guaranteed not to be above 30, and the index given is double what's needed, to practice collision resolution. Otherwise I'd absolutely clean that stuff up too. Thanks!
– Noctimor
Nov 13 '18 at 13:53
Great! Note: The index formula will result in 91 if the input string is
"z"
. It'll become: index = 57 + 114 % 40
=> 57 + (114 % 40)
– Ted Lyngmo
Nov 13 '18 at 14:10
Great! Note: The index formula will result in 91 if the input string is
"z"
. It'll become: index = 57 + 114 % 40
=> 57 + (114 % 40)
– Ted Lyngmo
Nov 13 '18 at 14:10
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282303%2fread-access-violation-when-attempting-strcpy-from-file-buffer-to-char-array%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Ma0OJIdqI6XqEqOoHHYroKu7sf sr89RjC0PwJn5Aia3FnOTjjUP5hi6S4RxHzFvY,w67IA QQ,p
Did you try debug this? index may be at 40 when the error occurs.
– Matthieu Brucher
Nov 13 '18 at 13:40
Also use
std::string
and astd::vector<arrayelement>
.– Matthieu Brucher
Nov 13 '18 at 13:40
2
proteins[index].protein == buffer
This is not how you want to compare char arrays.– 0x5453
Nov 13 '18 at 13:44
@0x5453 Oh man, I'm too tired. That did it. After changing that to a strcmp it all runs. Thank you!
– Noctimor
Nov 13 '18 at 13:50
@Noctimor Because you are trying to do C when you are in C++. Use containers....
– Matthieu Brucher
Nov 13 '18 at 13:51