Read tuple from text-file and plot to graph
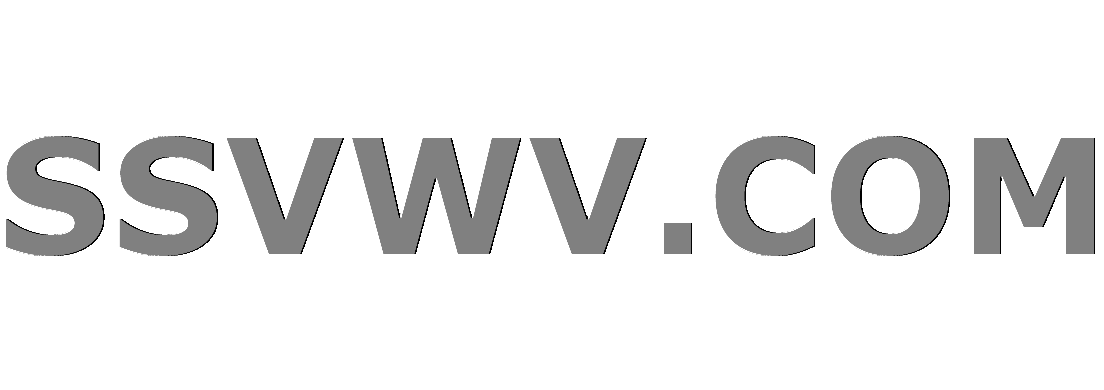
Multi tool use
I have a textfile with tuples of integer data, which I want to plot in a simple graph.
The textfile ("test.txt") looks like this. All tuples are separated by tabs.
Textfile (test.txt)
(1,2) (1,3) (2,8) (3,12) (5,82) (...)
R-Code
m = read.table('test.txt', header = FALSE, sep='t')
plot(log(m[,1]), log(m[,2]))
As a result I'm getting
Error in Math.factor(m[,1]): 'log' not meaningful for factors
What I can understand is, that my data-tuples are not read in as numeric values but as factors. Accordingly the 'log'-operator is not able to operate on these (factor-)values.
So my idea has been to convert factors to numeric, but I'm failing in converting the data. Furthermore I'm not sure if this is the solution for my problem.
What I would like to have is a 2d-graph with (x,y)-values as axis.
Maybe someone has an idea how to handle it.
--- EDIT ---
library(readtext)
library(ggplot2)
DATA_DIR <- system.file("extdata/", package = "readtext")
mytab = readtext(paste0(DATA_DIR, "/hlra/*"))
# readtext object consisting of 1 document and 0 docvars.
# # data.frame [1 x 2]
# doc_id text
# <chr> <chr>
# 1 sample_tuple_file.txt ""(1,2), (1,"..."
mytuple = strsplit(mytab$text, ', ')
mytuple = mytuple[[1]]
substring(mytuple[1], 2, 2) # get x value
substring(mytuple[1], 4, 4) # get y value
x = c()
y = c()
for (i in 1:length(mytuple))
my_x = substring(mytuple[i], 2, 2)
my_y = substring(mytuple[i], 4, 4)
x <- c(x, my_x)
y <- c(y, my_y)
rm(my_x)
rm(my_y)
mydata = data.frame(x = x, y = y)
ggplot(data = mydata, aes(x = x, y = y)) +
geom_point()
So, I've tested the Code above. But it's not working for all datas. The resulting plot just the first value. I've reorganised my text-file, so every single tuple ends with a comma and tab.
plot from code above
I'm completly new to R so I'm sure I'm missing some obviously mistakes in my code. By the way I had to change some code to get it run in R-Studio (added missing library (ggplot2 and readtext) and change file-directory.
r text-files factors
add a comment |
I have a textfile with tuples of integer data, which I want to plot in a simple graph.
The textfile ("test.txt") looks like this. All tuples are separated by tabs.
Textfile (test.txt)
(1,2) (1,3) (2,8) (3,12) (5,82) (...)
R-Code
m = read.table('test.txt', header = FALSE, sep='t')
plot(log(m[,1]), log(m[,2]))
As a result I'm getting
Error in Math.factor(m[,1]): 'log' not meaningful for factors
What I can understand is, that my data-tuples are not read in as numeric values but as factors. Accordingly the 'log'-operator is not able to operate on these (factor-)values.
So my idea has been to convert factors to numeric, but I'm failing in converting the data. Furthermore I'm not sure if this is the solution for my problem.
What I would like to have is a 2d-graph with (x,y)-values as axis.
Maybe someone has an idea how to handle it.
--- EDIT ---
library(readtext)
library(ggplot2)
DATA_DIR <- system.file("extdata/", package = "readtext")
mytab = readtext(paste0(DATA_DIR, "/hlra/*"))
# readtext object consisting of 1 document and 0 docvars.
# # data.frame [1 x 2]
# doc_id text
# <chr> <chr>
# 1 sample_tuple_file.txt ""(1,2), (1,"..."
mytuple = strsplit(mytab$text, ', ')
mytuple = mytuple[[1]]
substring(mytuple[1], 2, 2) # get x value
substring(mytuple[1], 4, 4) # get y value
x = c()
y = c()
for (i in 1:length(mytuple))
my_x = substring(mytuple[i], 2, 2)
my_y = substring(mytuple[i], 4, 4)
x <- c(x, my_x)
y <- c(y, my_y)
rm(my_x)
rm(my_y)
mydata = data.frame(x = x, y = y)
ggplot(data = mydata, aes(x = x, y = y)) +
geom_point()
So, I've tested the Code above. But it's not working for all datas. The resulting plot just the first value. I've reorganised my text-file, so every single tuple ends with a comma and tab.
plot from code above
I'm completly new to R so I'm sure I'm missing some obviously mistakes in my code. By the way I had to change some code to get it run in R-Studio (added missing library (ggplot2 and readtext) and change file-directory.
r text-files factors
add a comment |
I have a textfile with tuples of integer data, which I want to plot in a simple graph.
The textfile ("test.txt") looks like this. All tuples are separated by tabs.
Textfile (test.txt)
(1,2) (1,3) (2,8) (3,12) (5,82) (...)
R-Code
m = read.table('test.txt', header = FALSE, sep='t')
plot(log(m[,1]), log(m[,2]))
As a result I'm getting
Error in Math.factor(m[,1]): 'log' not meaningful for factors
What I can understand is, that my data-tuples are not read in as numeric values but as factors. Accordingly the 'log'-operator is not able to operate on these (factor-)values.
So my idea has been to convert factors to numeric, but I'm failing in converting the data. Furthermore I'm not sure if this is the solution for my problem.
What I would like to have is a 2d-graph with (x,y)-values as axis.
Maybe someone has an idea how to handle it.
--- EDIT ---
library(readtext)
library(ggplot2)
DATA_DIR <- system.file("extdata/", package = "readtext")
mytab = readtext(paste0(DATA_DIR, "/hlra/*"))
# readtext object consisting of 1 document and 0 docvars.
# # data.frame [1 x 2]
# doc_id text
# <chr> <chr>
# 1 sample_tuple_file.txt ""(1,2), (1,"..."
mytuple = strsplit(mytab$text, ', ')
mytuple = mytuple[[1]]
substring(mytuple[1], 2, 2) # get x value
substring(mytuple[1], 4, 4) # get y value
x = c()
y = c()
for (i in 1:length(mytuple))
my_x = substring(mytuple[i], 2, 2)
my_y = substring(mytuple[i], 4, 4)
x <- c(x, my_x)
y <- c(y, my_y)
rm(my_x)
rm(my_y)
mydata = data.frame(x = x, y = y)
ggplot(data = mydata, aes(x = x, y = y)) +
geom_point()
So, I've tested the Code above. But it's not working for all datas. The resulting plot just the first value. I've reorganised my text-file, so every single tuple ends with a comma and tab.
plot from code above
I'm completly new to R so I'm sure I'm missing some obviously mistakes in my code. By the way I had to change some code to get it run in R-Studio (added missing library (ggplot2 and readtext) and change file-directory.
r text-files factors
I have a textfile with tuples of integer data, which I want to plot in a simple graph.
The textfile ("test.txt") looks like this. All tuples are separated by tabs.
Textfile (test.txt)
(1,2) (1,3) (2,8) (3,12) (5,82) (...)
R-Code
m = read.table('test.txt', header = FALSE, sep='t')
plot(log(m[,1]), log(m[,2]))
As a result I'm getting
Error in Math.factor(m[,1]): 'log' not meaningful for factors
What I can understand is, that my data-tuples are not read in as numeric values but as factors. Accordingly the 'log'-operator is not able to operate on these (factor-)values.
So my idea has been to convert factors to numeric, but I'm failing in converting the data. Furthermore I'm not sure if this is the solution for my problem.
What I would like to have is a 2d-graph with (x,y)-values as axis.
Maybe someone has an idea how to handle it.
--- EDIT ---
library(readtext)
library(ggplot2)
DATA_DIR <- system.file("extdata/", package = "readtext")
mytab = readtext(paste0(DATA_DIR, "/hlra/*"))
# readtext object consisting of 1 document and 0 docvars.
# # data.frame [1 x 2]
# doc_id text
# <chr> <chr>
# 1 sample_tuple_file.txt ""(1,2), (1,"..."
mytuple = strsplit(mytab$text, ', ')
mytuple = mytuple[[1]]
substring(mytuple[1], 2, 2) # get x value
substring(mytuple[1], 4, 4) # get y value
x = c()
y = c()
for (i in 1:length(mytuple))
my_x = substring(mytuple[i], 2, 2)
my_y = substring(mytuple[i], 4, 4)
x <- c(x, my_x)
y <- c(y, my_y)
rm(my_x)
rm(my_y)
mydata = data.frame(x = x, y = y)
ggplot(data = mydata, aes(x = x, y = y)) +
geom_point()
So, I've tested the Code above. But it's not working for all datas. The resulting plot just the first value. I've reorganised my text-file, so every single tuple ends with a comma and tab.
plot from code above
I'm completly new to R so I'm sure I'm missing some obviously mistakes in my code. By the way I had to change some code to get it run in R-Studio (added missing library (ggplot2 and readtext) and change file-directory.
r text-files factors
r text-files factors
edited Nov 15 '18 at 13:30
Krid Reyem
asked Nov 13 '18 at 13:39
Krid ReyemKrid Reyem
73
73
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Assuming you have this kind of text file:
(1,2) (1,3) (2,8) (3,12) (5,82) (...)
I recommend using read_text
. Work out with vectors and strings to prepare data in the format that's ready for plotting.
#if packages aren't yet included in R import them by using R-console
#Command: install.packages("package-name")
#import library "readtext"
library(readtext)
#install library "ggplot2"
library(ggplot2)
#get directory from "readtext"-package which is in my case...
#C:Usersyour_nameDocumentsRwin-library3.5readtextextdatayour_folder
DATA_DIR <- system.file("extdata/", package = "readtext")
#the textfile you want to plot should be in folder "your_folder"
mytab = readtext(paste0(DATA_DIR, "your_folder/*")
# readtext object consisting of 1 document and 0 docvars.
# # data.frame [1 x 2]
# doc_id text
# <chr> <chr>
# 1 sample_tuple_file.txt ""(1,2), (1,"..."
mytuple = strsplit(mytab$text, 't')
mytuple = mytuple[[1]]
substring(mytuple[1], 2, 2) # get x value
substring(mytuple[1], 4, 4) # get y value
x = c()
y = c()
for (i in 1:length(mytuple))
my_x = substring(mytuple[i], 2, 2)
my_y = substring(mytuple[i], 4, 4)
x <- c(x, my_x)
y <- c(y, my_y)
rm(my_x)
rm(my_y)
mydata = data.frame(x = x, y = y)
ggplot(data = mydata, aes(x = x, y = y)) +
geom_point()
Code is not working for me. Not sure if I'm making any mistakes. See my edited question on top
– Krid Reyem
Nov 15 '18 at 13:33
The input structure may be slightly different. How about tryingmytuple = strsplit(mytab$text, ' ')
?
– kon_u
Nov 15 '18 at 13:52
mytuple = strsplit(mytab$text, 't')
worked perfectly. Make sense to me after rethinking my input structure with tuples seperated by tabs ('t').
– Krid Reyem
Nov 15 '18 at 14:14
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282317%2fread-tuple-from-text-file-and-plot-to-graph%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Assuming you have this kind of text file:
(1,2) (1,3) (2,8) (3,12) (5,82) (...)
I recommend using read_text
. Work out with vectors and strings to prepare data in the format that's ready for plotting.
#if packages aren't yet included in R import them by using R-console
#Command: install.packages("package-name")
#import library "readtext"
library(readtext)
#install library "ggplot2"
library(ggplot2)
#get directory from "readtext"-package which is in my case...
#C:Usersyour_nameDocumentsRwin-library3.5readtextextdatayour_folder
DATA_DIR <- system.file("extdata/", package = "readtext")
#the textfile you want to plot should be in folder "your_folder"
mytab = readtext(paste0(DATA_DIR, "your_folder/*")
# readtext object consisting of 1 document and 0 docvars.
# # data.frame [1 x 2]
# doc_id text
# <chr> <chr>
# 1 sample_tuple_file.txt ""(1,2), (1,"..."
mytuple = strsplit(mytab$text, 't')
mytuple = mytuple[[1]]
substring(mytuple[1], 2, 2) # get x value
substring(mytuple[1], 4, 4) # get y value
x = c()
y = c()
for (i in 1:length(mytuple))
my_x = substring(mytuple[i], 2, 2)
my_y = substring(mytuple[i], 4, 4)
x <- c(x, my_x)
y <- c(y, my_y)
rm(my_x)
rm(my_y)
mydata = data.frame(x = x, y = y)
ggplot(data = mydata, aes(x = x, y = y)) +
geom_point()
Code is not working for me. Not sure if I'm making any mistakes. See my edited question on top
– Krid Reyem
Nov 15 '18 at 13:33
The input structure may be slightly different. How about tryingmytuple = strsplit(mytab$text, ' ')
?
– kon_u
Nov 15 '18 at 13:52
mytuple = strsplit(mytab$text, 't')
worked perfectly. Make sense to me after rethinking my input structure with tuples seperated by tabs ('t').
– Krid Reyem
Nov 15 '18 at 14:14
add a comment |
Assuming you have this kind of text file:
(1,2) (1,3) (2,8) (3,12) (5,82) (...)
I recommend using read_text
. Work out with vectors and strings to prepare data in the format that's ready for plotting.
#if packages aren't yet included in R import them by using R-console
#Command: install.packages("package-name")
#import library "readtext"
library(readtext)
#install library "ggplot2"
library(ggplot2)
#get directory from "readtext"-package which is in my case...
#C:Usersyour_nameDocumentsRwin-library3.5readtextextdatayour_folder
DATA_DIR <- system.file("extdata/", package = "readtext")
#the textfile you want to plot should be in folder "your_folder"
mytab = readtext(paste0(DATA_DIR, "your_folder/*")
# readtext object consisting of 1 document and 0 docvars.
# # data.frame [1 x 2]
# doc_id text
# <chr> <chr>
# 1 sample_tuple_file.txt ""(1,2), (1,"..."
mytuple = strsplit(mytab$text, 't')
mytuple = mytuple[[1]]
substring(mytuple[1], 2, 2) # get x value
substring(mytuple[1], 4, 4) # get y value
x = c()
y = c()
for (i in 1:length(mytuple))
my_x = substring(mytuple[i], 2, 2)
my_y = substring(mytuple[i], 4, 4)
x <- c(x, my_x)
y <- c(y, my_y)
rm(my_x)
rm(my_y)
mydata = data.frame(x = x, y = y)
ggplot(data = mydata, aes(x = x, y = y)) +
geom_point()
Code is not working for me. Not sure if I'm making any mistakes. See my edited question on top
– Krid Reyem
Nov 15 '18 at 13:33
The input structure may be slightly different. How about tryingmytuple = strsplit(mytab$text, ' ')
?
– kon_u
Nov 15 '18 at 13:52
mytuple = strsplit(mytab$text, 't')
worked perfectly. Make sense to me after rethinking my input structure with tuples seperated by tabs ('t').
– Krid Reyem
Nov 15 '18 at 14:14
add a comment |
Assuming you have this kind of text file:
(1,2) (1,3) (2,8) (3,12) (5,82) (...)
I recommend using read_text
. Work out with vectors and strings to prepare data in the format that's ready for plotting.
#if packages aren't yet included in R import them by using R-console
#Command: install.packages("package-name")
#import library "readtext"
library(readtext)
#install library "ggplot2"
library(ggplot2)
#get directory from "readtext"-package which is in my case...
#C:Usersyour_nameDocumentsRwin-library3.5readtextextdatayour_folder
DATA_DIR <- system.file("extdata/", package = "readtext")
#the textfile you want to plot should be in folder "your_folder"
mytab = readtext(paste0(DATA_DIR, "your_folder/*")
# readtext object consisting of 1 document and 0 docvars.
# # data.frame [1 x 2]
# doc_id text
# <chr> <chr>
# 1 sample_tuple_file.txt ""(1,2), (1,"..."
mytuple = strsplit(mytab$text, 't')
mytuple = mytuple[[1]]
substring(mytuple[1], 2, 2) # get x value
substring(mytuple[1], 4, 4) # get y value
x = c()
y = c()
for (i in 1:length(mytuple))
my_x = substring(mytuple[i], 2, 2)
my_y = substring(mytuple[i], 4, 4)
x <- c(x, my_x)
y <- c(y, my_y)
rm(my_x)
rm(my_y)
mydata = data.frame(x = x, y = y)
ggplot(data = mydata, aes(x = x, y = y)) +
geom_point()
Assuming you have this kind of text file:
(1,2) (1,3) (2,8) (3,12) (5,82) (...)
I recommend using read_text
. Work out with vectors and strings to prepare data in the format that's ready for plotting.
#if packages aren't yet included in R import them by using R-console
#Command: install.packages("package-name")
#import library "readtext"
library(readtext)
#install library "ggplot2"
library(ggplot2)
#get directory from "readtext"-package which is in my case...
#C:Usersyour_nameDocumentsRwin-library3.5readtextextdatayour_folder
DATA_DIR <- system.file("extdata/", package = "readtext")
#the textfile you want to plot should be in folder "your_folder"
mytab = readtext(paste0(DATA_DIR, "your_folder/*")
# readtext object consisting of 1 document and 0 docvars.
# # data.frame [1 x 2]
# doc_id text
# <chr> <chr>
# 1 sample_tuple_file.txt ""(1,2), (1,"..."
mytuple = strsplit(mytab$text, 't')
mytuple = mytuple[[1]]
substring(mytuple[1], 2, 2) # get x value
substring(mytuple[1], 4, 4) # get y value
x = c()
y = c()
for (i in 1:length(mytuple))
my_x = substring(mytuple[i], 2, 2)
my_y = substring(mytuple[i], 4, 4)
x <- c(x, my_x)
y <- c(y, my_y)
rm(my_x)
rm(my_y)
mydata = data.frame(x = x, y = y)
ggplot(data = mydata, aes(x = x, y = y)) +
geom_point()
edited Nov 16 '18 at 1:19
Krid Reyem
73
73
answered Nov 13 '18 at 15:10
kon_ukon_u
1966
1966
Code is not working for me. Not sure if I'm making any mistakes. See my edited question on top
– Krid Reyem
Nov 15 '18 at 13:33
The input structure may be slightly different. How about tryingmytuple = strsplit(mytab$text, ' ')
?
– kon_u
Nov 15 '18 at 13:52
mytuple = strsplit(mytab$text, 't')
worked perfectly. Make sense to me after rethinking my input structure with tuples seperated by tabs ('t').
– Krid Reyem
Nov 15 '18 at 14:14
add a comment |
Code is not working for me. Not sure if I'm making any mistakes. See my edited question on top
– Krid Reyem
Nov 15 '18 at 13:33
The input structure may be slightly different. How about tryingmytuple = strsplit(mytab$text, ' ')
?
– kon_u
Nov 15 '18 at 13:52
mytuple = strsplit(mytab$text, 't')
worked perfectly. Make sense to me after rethinking my input structure with tuples seperated by tabs ('t').
– Krid Reyem
Nov 15 '18 at 14:14
Code is not working for me. Not sure if I'm making any mistakes. See my edited question on top
– Krid Reyem
Nov 15 '18 at 13:33
Code is not working for me. Not sure if I'm making any mistakes. See my edited question on top
– Krid Reyem
Nov 15 '18 at 13:33
The input structure may be slightly different. How about trying
mytuple = strsplit(mytab$text, ' ')
?– kon_u
Nov 15 '18 at 13:52
The input structure may be slightly different. How about trying
mytuple = strsplit(mytab$text, ' ')
?– kon_u
Nov 15 '18 at 13:52
mytuple = strsplit(mytab$text, 't')
worked perfectly. Make sense to me after rethinking my input structure with tuples seperated by tabs ('t').– Krid Reyem
Nov 15 '18 at 14:14
mytuple = strsplit(mytab$text, 't')
worked perfectly. Make sense to me after rethinking my input structure with tuples seperated by tabs ('t').– Krid Reyem
Nov 15 '18 at 14:14
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282317%2fread-tuple-from-text-file-and-plot-to-graph%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
yjWm2j