Attempting to have scene load with camera position the same as it left off
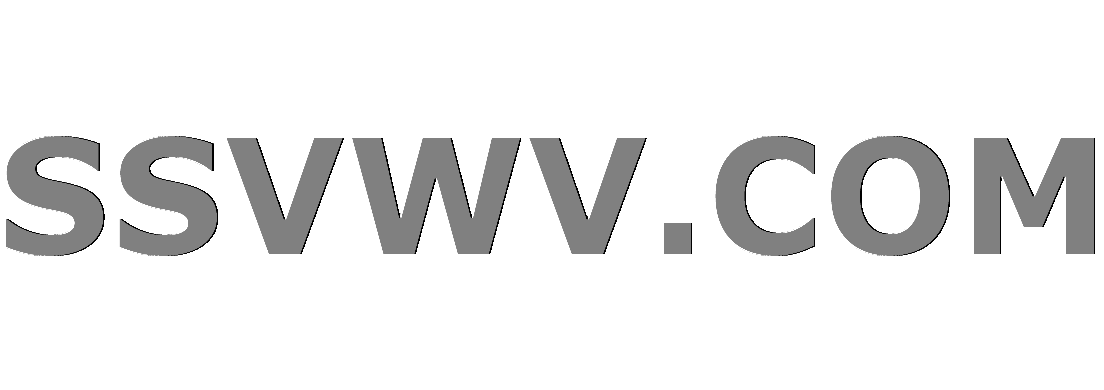
Multi tool use
So basically, think Mario here, I have a world map. A player enters a level, then when the player returns to the Map, I want it to load the camera position the player last left off on. I am having trouble doing this and it keeps resetting back to the original camera position.
Here is what I've tried so far:
I have a WorldManager class with the following code:
private void Start()
highestLevelUnlocked = PlayerPrefsManager.GetHighestLevelUnlocked();
if (highestLevelUnlocked > 0)
rectTransform.offsetMin = new Vector2(PlayerPrefsManager.GetLastLevelViewedLeft(), rectTransform.offsetMin.x);
rectTransform.offsetMax = new Vector2(PlayerPrefsManager.GetLastLevelViewedRight(), rectTransform.offsetMax.x);
else
rectTransform.offsetMin = new Vector2(0, 0);
rectTransform.offsetMax = new Vector2(0, 0);
// Sets the camera coordinates.
public void SetCameraCoordinates()
PlayerPrefsManager.SetLastLevelViewed(Mathf.RoundToInt(rectTransform.offsetMin.x), Mathf.RoundToInt(rectTransform.offsetMax.x));
I'm sure i'm doing something completely wrong but I can't figure it out and was hoping for some insight.
PlayerPrefsManager is static and holds onto player data between scenes.
Thank you!
Edit : I'm using a Scroll View for the player to scroll across the map with mouse/thumbs.
c# unity3d
add a comment |
So basically, think Mario here, I have a world map. A player enters a level, then when the player returns to the Map, I want it to load the camera position the player last left off on. I am having trouble doing this and it keeps resetting back to the original camera position.
Here is what I've tried so far:
I have a WorldManager class with the following code:
private void Start()
highestLevelUnlocked = PlayerPrefsManager.GetHighestLevelUnlocked();
if (highestLevelUnlocked > 0)
rectTransform.offsetMin = new Vector2(PlayerPrefsManager.GetLastLevelViewedLeft(), rectTransform.offsetMin.x);
rectTransform.offsetMax = new Vector2(PlayerPrefsManager.GetLastLevelViewedRight(), rectTransform.offsetMax.x);
else
rectTransform.offsetMin = new Vector2(0, 0);
rectTransform.offsetMax = new Vector2(0, 0);
// Sets the camera coordinates.
public void SetCameraCoordinates()
PlayerPrefsManager.SetLastLevelViewed(Mathf.RoundToInt(rectTransform.offsetMin.x), Mathf.RoundToInt(rectTransform.offsetMax.x));
I'm sure i'm doing something completely wrong but I can't figure it out and was hoping for some insight.
PlayerPrefsManager is static and holds onto player data between scenes.
Thank you!
Edit : I'm using a Scroll View for the player to scroll across the map with mouse/thumbs.
c# unity3d
1
How do yourSetLastLevelViewed
,GetLastLevelViewedLeft
andGetLastLevelViewedRight
look like? I'ld also recommend not to use a static class but rather a ScriptableObject
– derHugo
Nov 14 '18 at 6:04
Thanks for this! I haven't looked into ScriptableObject. I will read up on this now!
– Holden
Nov 14 '18 at 6:52
This tutorial is actually a better start point than the docs
– derHugo
Nov 14 '18 at 6:54
add a comment |
So basically, think Mario here, I have a world map. A player enters a level, then when the player returns to the Map, I want it to load the camera position the player last left off on. I am having trouble doing this and it keeps resetting back to the original camera position.
Here is what I've tried so far:
I have a WorldManager class with the following code:
private void Start()
highestLevelUnlocked = PlayerPrefsManager.GetHighestLevelUnlocked();
if (highestLevelUnlocked > 0)
rectTransform.offsetMin = new Vector2(PlayerPrefsManager.GetLastLevelViewedLeft(), rectTransform.offsetMin.x);
rectTransform.offsetMax = new Vector2(PlayerPrefsManager.GetLastLevelViewedRight(), rectTransform.offsetMax.x);
else
rectTransform.offsetMin = new Vector2(0, 0);
rectTransform.offsetMax = new Vector2(0, 0);
// Sets the camera coordinates.
public void SetCameraCoordinates()
PlayerPrefsManager.SetLastLevelViewed(Mathf.RoundToInt(rectTransform.offsetMin.x), Mathf.RoundToInt(rectTransform.offsetMax.x));
I'm sure i'm doing something completely wrong but I can't figure it out and was hoping for some insight.
PlayerPrefsManager is static and holds onto player data between scenes.
Thank you!
Edit : I'm using a Scroll View for the player to scroll across the map with mouse/thumbs.
c# unity3d
So basically, think Mario here, I have a world map. A player enters a level, then when the player returns to the Map, I want it to load the camera position the player last left off on. I am having trouble doing this and it keeps resetting back to the original camera position.
Here is what I've tried so far:
I have a WorldManager class with the following code:
private void Start()
highestLevelUnlocked = PlayerPrefsManager.GetHighestLevelUnlocked();
if (highestLevelUnlocked > 0)
rectTransform.offsetMin = new Vector2(PlayerPrefsManager.GetLastLevelViewedLeft(), rectTransform.offsetMin.x);
rectTransform.offsetMax = new Vector2(PlayerPrefsManager.GetLastLevelViewedRight(), rectTransform.offsetMax.x);
else
rectTransform.offsetMin = new Vector2(0, 0);
rectTransform.offsetMax = new Vector2(0, 0);
// Sets the camera coordinates.
public void SetCameraCoordinates()
PlayerPrefsManager.SetLastLevelViewed(Mathf.RoundToInt(rectTransform.offsetMin.x), Mathf.RoundToInt(rectTransform.offsetMax.x));
I'm sure i'm doing something completely wrong but I can't figure it out and was hoping for some insight.
PlayerPrefsManager is static and holds onto player data between scenes.
Thank you!
Edit : I'm using a Scroll View for the player to scroll across the map with mouse/thumbs.
c# unity3d
c# unity3d
edited Nov 14 '18 at 4:43


John
12.9k32242
12.9k32242
asked Nov 14 '18 at 4:35


HoldenHolden
678
678
1
How do yourSetLastLevelViewed
,GetLastLevelViewedLeft
andGetLastLevelViewedRight
look like? I'ld also recommend not to use a static class but rather a ScriptableObject
– derHugo
Nov 14 '18 at 6:04
Thanks for this! I haven't looked into ScriptableObject. I will read up on this now!
– Holden
Nov 14 '18 at 6:52
This tutorial is actually a better start point than the docs
– derHugo
Nov 14 '18 at 6:54
add a comment |
1
How do yourSetLastLevelViewed
,GetLastLevelViewedLeft
andGetLastLevelViewedRight
look like? I'ld also recommend not to use a static class but rather a ScriptableObject
– derHugo
Nov 14 '18 at 6:04
Thanks for this! I haven't looked into ScriptableObject. I will read up on this now!
– Holden
Nov 14 '18 at 6:52
This tutorial is actually a better start point than the docs
– derHugo
Nov 14 '18 at 6:54
1
1
How do your
SetLastLevelViewed
, GetLastLevelViewedLeft
and GetLastLevelViewedRight
look like? I'ld also recommend not to use a static class but rather a ScriptableObject– derHugo
Nov 14 '18 at 6:04
How do your
SetLastLevelViewed
, GetLastLevelViewedLeft
and GetLastLevelViewedRight
look like? I'ld also recommend not to use a static class but rather a ScriptableObject– derHugo
Nov 14 '18 at 6:04
Thanks for this! I haven't looked into ScriptableObject. I will read up on this now!
– Holden
Nov 14 '18 at 6:52
Thanks for this! I haven't looked into ScriptableObject. I will read up on this now!
– Holden
Nov 14 '18 at 6:52
This tutorial is actually a better start point than the docs
– derHugo
Nov 14 '18 at 6:54
This tutorial is actually a better start point than the docs
– derHugo
Nov 14 '18 at 6:54
add a comment |
1 Answer
1
active
oldest
votes
Thanks for anyone who looked at this. I was able to solve the problem by using the following:
private void Start()
lastLevelViewed = PlayerPrefsManager.GetLastLevelViewed();
if(lastLevelViewed > 0)
scrollRect.horizontalScrollbar.value = PlayerPrefsManager.GetLastLevelViewed();
else
scrollRect.horizontalScrollbar.value = 0;
// Sets the camera coordinates.
public void SetCameraCoordinates()
PlayerPrefsManager.SetLastLevelViewed(scrollRect.horizontalScrollbar.value);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53293257%2fattempting-to-have-scene-load-with-camera-position-the-same-as-it-left-off%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for anyone who looked at this. I was able to solve the problem by using the following:
private void Start()
lastLevelViewed = PlayerPrefsManager.GetLastLevelViewed();
if(lastLevelViewed > 0)
scrollRect.horizontalScrollbar.value = PlayerPrefsManager.GetLastLevelViewed();
else
scrollRect.horizontalScrollbar.value = 0;
// Sets the camera coordinates.
public void SetCameraCoordinates()
PlayerPrefsManager.SetLastLevelViewed(scrollRect.horizontalScrollbar.value);
add a comment |
Thanks for anyone who looked at this. I was able to solve the problem by using the following:
private void Start()
lastLevelViewed = PlayerPrefsManager.GetLastLevelViewed();
if(lastLevelViewed > 0)
scrollRect.horizontalScrollbar.value = PlayerPrefsManager.GetLastLevelViewed();
else
scrollRect.horizontalScrollbar.value = 0;
// Sets the camera coordinates.
public void SetCameraCoordinates()
PlayerPrefsManager.SetLastLevelViewed(scrollRect.horizontalScrollbar.value);
add a comment |
Thanks for anyone who looked at this. I was able to solve the problem by using the following:
private void Start()
lastLevelViewed = PlayerPrefsManager.GetLastLevelViewed();
if(lastLevelViewed > 0)
scrollRect.horizontalScrollbar.value = PlayerPrefsManager.GetLastLevelViewed();
else
scrollRect.horizontalScrollbar.value = 0;
// Sets the camera coordinates.
public void SetCameraCoordinates()
PlayerPrefsManager.SetLastLevelViewed(scrollRect.horizontalScrollbar.value);
Thanks for anyone who looked at this. I was able to solve the problem by using the following:
private void Start()
lastLevelViewed = PlayerPrefsManager.GetLastLevelViewed();
if(lastLevelViewed > 0)
scrollRect.horizontalScrollbar.value = PlayerPrefsManager.GetLastLevelViewed();
else
scrollRect.horizontalScrollbar.value = 0;
// Sets the camera coordinates.
public void SetCameraCoordinates()
PlayerPrefsManager.SetLastLevelViewed(scrollRect.horizontalScrollbar.value);
answered Nov 14 '18 at 6:51


HoldenHolden
678
678
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53293257%2fattempting-to-have-scene-load-with-camera-position-the-same-as-it-left-off%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Laaho3Cs4,Vy8HiINkLRjxm,ACG8f3tPRk0,WLnxvGfrHMOQNXo9mvD,rKzM4Mz,l QwnYzL Y,GjZIsfzG ju7o5g0JfcDPiE
1
How do your
SetLastLevelViewed
,GetLastLevelViewedLeft
andGetLastLevelViewedRight
look like? I'ld also recommend not to use a static class but rather a ScriptableObject– derHugo
Nov 14 '18 at 6:04
Thanks for this! I haven't looked into ScriptableObject. I will read up on this now!
– Holden
Nov 14 '18 at 6:52
This tutorial is actually a better start point than the docs
– derHugo
Nov 14 '18 at 6:54