Django order_by a property
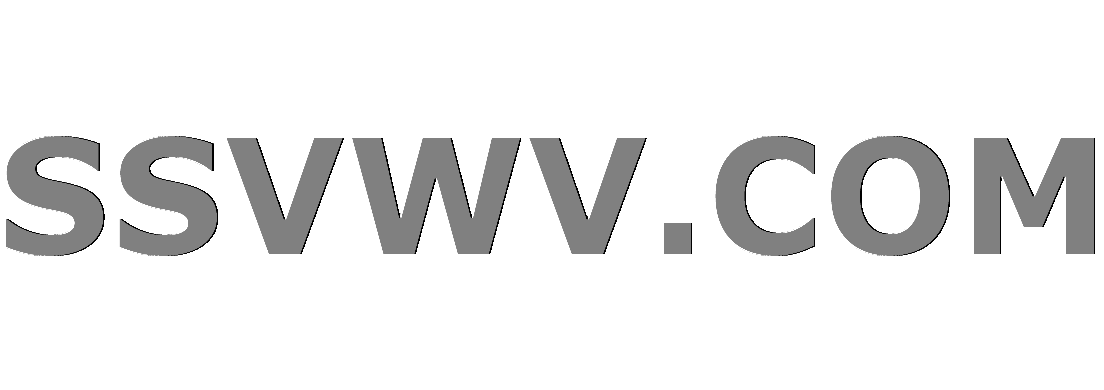
Multi tool use
up vote
6
down vote
favorite
I'm faced with a problem wherein I'm trying to create a QuerySet with the results ordered not by a field on a model, but instead ordered by the result of a value returned by a method on the model.
To wit:
class MyModel(models.Model):
someAttributes = models.TextField(blank=True)
@property
def calculate_rating(self):
<do some calculation and return integer>
Given that, how can I construct a QuerySet that orders the results by the value for each instance as returned by calculate_rating()?
In trying to simply use order_by(), I get an error:
Cannot resolve keyword 'average_rating' into field.
Can anybody provide some ideas?
django django-models
add a comment |
up vote
6
down vote
favorite
I'm faced with a problem wherein I'm trying to create a QuerySet with the results ordered not by a field on a model, but instead ordered by the result of a value returned by a method on the model.
To wit:
class MyModel(models.Model):
someAttributes = models.TextField(blank=True)
@property
def calculate_rating(self):
<do some calculation and return integer>
Given that, how can I construct a QuerySet that orders the results by the value for each instance as returned by calculate_rating()?
In trying to simply use order_by(), I get an error:
Cannot resolve keyword 'average_rating' into field.
Can anybody provide some ideas?
django django-models
possible duplicate of Sorting a Django QuerySet by a property (not a field) of the Model
– Anto
May 8 '14 at 15:48
add a comment |
up vote
6
down vote
favorite
up vote
6
down vote
favorite
I'm faced with a problem wherein I'm trying to create a QuerySet with the results ordered not by a field on a model, but instead ordered by the result of a value returned by a method on the model.
To wit:
class MyModel(models.Model):
someAttributes = models.TextField(blank=True)
@property
def calculate_rating(self):
<do some calculation and return integer>
Given that, how can I construct a QuerySet that orders the results by the value for each instance as returned by calculate_rating()?
In trying to simply use order_by(), I get an error:
Cannot resolve keyword 'average_rating' into field.
Can anybody provide some ideas?
django django-models
I'm faced with a problem wherein I'm trying to create a QuerySet with the results ordered not by a field on a model, but instead ordered by the result of a value returned by a method on the model.
To wit:
class MyModel(models.Model):
someAttributes = models.TextField(blank=True)
@property
def calculate_rating(self):
<do some calculation and return integer>
Given that, how can I construct a QuerySet that orders the results by the value for each instance as returned by calculate_rating()?
In trying to simply use order_by(), I get an error:
Cannot resolve keyword 'average_rating' into field.
Can anybody provide some ideas?
django django-models
django django-models
asked May 1 '13 at 16:45
Michael Place
2,00111416
2,00111416
possible duplicate of Sorting a Django QuerySet by a property (not a field) of the Model
– Anto
May 8 '14 at 15:48
add a comment |
possible duplicate of Sorting a Django QuerySet by a property (not a field) of the Model
– Anto
May 8 '14 at 15:48
possible duplicate of Sorting a Django QuerySet by a property (not a field) of the Model
– Anto
May 8 '14 at 15:48
possible duplicate of Sorting a Django QuerySet by a property (not a field) of the Model
– Anto
May 8 '14 at 15:48
add a comment |
3 Answers
3
active
oldest
votes
up vote
4
down vote
accepted
There is no way to do this. One thing you can do is to create a separate database field for that model and save the calculated rating in it. You can probably override the save method of the model and do the calculations there, after that you can only refer to the value.
You can also sort the returned QuerySet
using Python sorted
. Take into account that the sorting approach using the built-in sorted function increases a lot the computational complexity and it's not a good idea to use such code in production.
For more information you can check this answer: https://stackoverflow.com/a/981802/1869597
add a comment |
up vote
10
down vote
order_by
is for database stuff. Try to use sorted
instead:
sorted(MyModel.objects.all(), key=lambda m: m.calculate_rating)
add a comment |
up vote
0
down vote
The best way to achieve what you want, is by using the Case
and When
statements. This allows you to keep the final result in QuerySet
instead of list ;)
ratings_tuples = [(r.id, r.calc_rating) for r in MyModel.objects.all()]
s = sorted(ratings_tuples, key = lambda x: x[1]) # Sort by second tuple elem
So here is our sorted list s
that holds IDs and ratings of the elements in a sorted way
pk_list = [idx for idx, rating in ratings_list]
from django.db.models import Case, When
preserved = Case(*[When(pk=pk, then=pos) for pos, pk in enumerate(pk_list)])
queryset = MyModel.objects.filter(pk__in=pk_list).order_by(preserved)
Now, using Case
and When
statements, we are able to do the queryset sorting itself by the IDs from the list we already have. (Django Docs for details).
Had a similar situation and needed a solution. So found some details on this post https://stackoverflow.com/a/37648265/4271452, it works.
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
4
down vote
accepted
There is no way to do this. One thing you can do is to create a separate database field for that model and save the calculated rating in it. You can probably override the save method of the model and do the calculations there, after that you can only refer to the value.
You can also sort the returned QuerySet
using Python sorted
. Take into account that the sorting approach using the built-in sorted function increases a lot the computational complexity and it's not a good idea to use such code in production.
For more information you can check this answer: https://stackoverflow.com/a/981802/1869597
add a comment |
up vote
4
down vote
accepted
There is no way to do this. One thing you can do is to create a separate database field for that model and save the calculated rating in it. You can probably override the save method of the model and do the calculations there, after that you can only refer to the value.
You can also sort the returned QuerySet
using Python sorted
. Take into account that the sorting approach using the built-in sorted function increases a lot the computational complexity and it's not a good idea to use such code in production.
For more information you can check this answer: https://stackoverflow.com/a/981802/1869597
add a comment |
up vote
4
down vote
accepted
up vote
4
down vote
accepted
There is no way to do this. One thing you can do is to create a separate database field for that model and save the calculated rating in it. You can probably override the save method of the model and do the calculations there, after that you can only refer to the value.
You can also sort the returned QuerySet
using Python sorted
. Take into account that the sorting approach using the built-in sorted function increases a lot the computational complexity and it's not a good idea to use such code in production.
For more information you can check this answer: https://stackoverflow.com/a/981802/1869597
There is no way to do this. One thing you can do is to create a separate database field for that model and save the calculated rating in it. You can probably override the save method of the model and do the calculations there, after that you can only refer to the value.
You can also sort the returned QuerySet
using Python sorted
. Take into account that the sorting approach using the built-in sorted function increases a lot the computational complexity and it's not a good idea to use such code in production.
For more information you can check this answer: https://stackoverflow.com/a/981802/1869597
edited May 23 '17 at 12:25
Community♦
11
11
answered May 1 '13 at 16:59
Jordan Jambazov
2,1651132
2,1651132
add a comment |
add a comment |
up vote
10
down vote
order_by
is for database stuff. Try to use sorted
instead:
sorted(MyModel.objects.all(), key=lambda m: m.calculate_rating)
add a comment |
up vote
10
down vote
order_by
is for database stuff. Try to use sorted
instead:
sorted(MyModel.objects.all(), key=lambda m: m.calculate_rating)
add a comment |
up vote
10
down vote
up vote
10
down vote
order_by
is for database stuff. Try to use sorted
instead:
sorted(MyModel.objects.all(), key=lambda m: m.calculate_rating)
order_by
is for database stuff. Try to use sorted
instead:
sorted(MyModel.objects.all(), key=lambda m: m.calculate_rating)
answered May 1 '13 at 16:55
Alexandre
8901021
8901021
add a comment |
add a comment |
up vote
0
down vote
The best way to achieve what you want, is by using the Case
and When
statements. This allows you to keep the final result in QuerySet
instead of list ;)
ratings_tuples = [(r.id, r.calc_rating) for r in MyModel.objects.all()]
s = sorted(ratings_tuples, key = lambda x: x[1]) # Sort by second tuple elem
So here is our sorted list s
that holds IDs and ratings of the elements in a sorted way
pk_list = [idx for idx, rating in ratings_list]
from django.db.models import Case, When
preserved = Case(*[When(pk=pk, then=pos) for pos, pk in enumerate(pk_list)])
queryset = MyModel.objects.filter(pk__in=pk_list).order_by(preserved)
Now, using Case
and When
statements, we are able to do the queryset sorting itself by the IDs from the list we already have. (Django Docs for details).
Had a similar situation and needed a solution. So found some details on this post https://stackoverflow.com/a/37648265/4271452, it works.
add a comment |
up vote
0
down vote
The best way to achieve what you want, is by using the Case
and When
statements. This allows you to keep the final result in QuerySet
instead of list ;)
ratings_tuples = [(r.id, r.calc_rating) for r in MyModel.objects.all()]
s = sorted(ratings_tuples, key = lambda x: x[1]) # Sort by second tuple elem
So here is our sorted list s
that holds IDs and ratings of the elements in a sorted way
pk_list = [idx for idx, rating in ratings_list]
from django.db.models import Case, When
preserved = Case(*[When(pk=pk, then=pos) for pos, pk in enumerate(pk_list)])
queryset = MyModel.objects.filter(pk__in=pk_list).order_by(preserved)
Now, using Case
and When
statements, we are able to do the queryset sorting itself by the IDs from the list we already have. (Django Docs for details).
Had a similar situation and needed a solution. So found some details on this post https://stackoverflow.com/a/37648265/4271452, it works.
add a comment |
up vote
0
down vote
up vote
0
down vote
The best way to achieve what you want, is by using the Case
and When
statements. This allows you to keep the final result in QuerySet
instead of list ;)
ratings_tuples = [(r.id, r.calc_rating) for r in MyModel.objects.all()]
s = sorted(ratings_tuples, key = lambda x: x[1]) # Sort by second tuple elem
So here is our sorted list s
that holds IDs and ratings of the elements in a sorted way
pk_list = [idx for idx, rating in ratings_list]
from django.db.models import Case, When
preserved = Case(*[When(pk=pk, then=pos) for pos, pk in enumerate(pk_list)])
queryset = MyModel.objects.filter(pk__in=pk_list).order_by(preserved)
Now, using Case
and When
statements, we are able to do the queryset sorting itself by the IDs from the list we already have. (Django Docs for details).
Had a similar situation and needed a solution. So found some details on this post https://stackoverflow.com/a/37648265/4271452, it works.
The best way to achieve what you want, is by using the Case
and When
statements. This allows you to keep the final result in QuerySet
instead of list ;)
ratings_tuples = [(r.id, r.calc_rating) for r in MyModel.objects.all()]
s = sorted(ratings_tuples, key = lambda x: x[1]) # Sort by second tuple elem
So here is our sorted list s
that holds IDs and ratings of the elements in a sorted way
pk_list = [idx for idx, rating in ratings_list]
from django.db.models import Case, When
preserved = Case(*[When(pk=pk, then=pos) for pos, pk in enumerate(pk_list)])
queryset = MyModel.objects.filter(pk__in=pk_list).order_by(preserved)
Now, using Case
and When
statements, we are able to do the queryset sorting itself by the IDs from the list we already have. (Django Docs for details).
Had a similar situation and needed a solution. So found some details on this post https://stackoverflow.com/a/37648265/4271452, it works.
answered Nov 10 at 0:03
Iulian Pinzaru
497
497
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f16322513%2fdjango-order-by-a-property%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5QY PPcabZ4bJcoW3NF8M4ZH8X,UnL6DO5pS98MLJY
possible duplicate of Sorting a Django QuerySet by a property (not a field) of the Model
– Anto
May 8 '14 at 15:48