Passing variables between methods in Python?
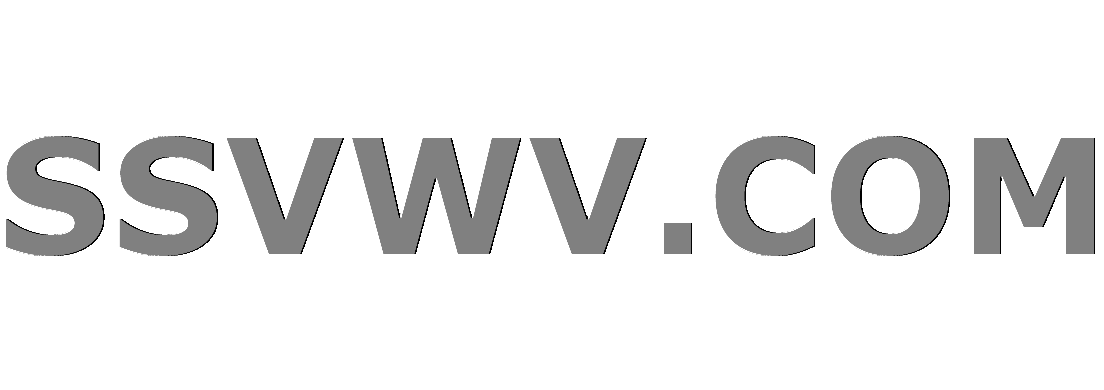
Multi tool use
up vote
2
down vote
favorite
I have a class and two methods. One method gets input from the user and stores it in two variables, x and y. I want another method that accepts an input so adds that input to x and y. When I run calculate(z) for some number z, it gives me errors saying the global variables x and y aren't defined. Obviously this means that the calculate method isn't getting access to x and y from getinput(). What am I doing wrong?
class simpleclass (object):
def getinput (self):
x = input("input value for x: ")
y = input("input value for y: ")
def calculate (self, z):
print x+y+z
python
add a comment |
up vote
2
down vote
favorite
I have a class and two methods. One method gets input from the user and stores it in two variables, x and y. I want another method that accepts an input so adds that input to x and y. When I run calculate(z) for some number z, it gives me errors saying the global variables x and y aren't defined. Obviously this means that the calculate method isn't getting access to x and y from getinput(). What am I doing wrong?
class simpleclass (object):
def getinput (self):
x = input("input value for x: ")
y = input("input value for y: ")
def calculate (self, z):
print x+y+z
python
1
useraw_input
– Karoly Horvath
Mar 1 '12 at 16:33
@yi_H : could you please explain whyraw_input()
would be preferred overinput()
?
– Li-aung Yip
Mar 2 '12 at 6:06
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I have a class and two methods. One method gets input from the user and stores it in two variables, x and y. I want another method that accepts an input so adds that input to x and y. When I run calculate(z) for some number z, it gives me errors saying the global variables x and y aren't defined. Obviously this means that the calculate method isn't getting access to x and y from getinput(). What am I doing wrong?
class simpleclass (object):
def getinput (self):
x = input("input value for x: ")
y = input("input value for y: ")
def calculate (self, z):
print x+y+z
python
I have a class and two methods. One method gets input from the user and stores it in two variables, x and y. I want another method that accepts an input so adds that input to x and y. When I run calculate(z) for some number z, it gives me errors saying the global variables x and y aren't defined. Obviously this means that the calculate method isn't getting access to x and y from getinput(). What am I doing wrong?
class simpleclass (object):
def getinput (self):
x = input("input value for x: ")
y = input("input value for y: ")
def calculate (self, z):
print x+y+z
python
python
asked Mar 1 '12 at 16:31
monolith
1612
1612
1
useraw_input
– Karoly Horvath
Mar 1 '12 at 16:33
@yi_H : could you please explain whyraw_input()
would be preferred overinput()
?
– Li-aung Yip
Mar 2 '12 at 6:06
add a comment |
1
useraw_input
– Karoly Horvath
Mar 1 '12 at 16:33
@yi_H : could you please explain whyraw_input()
would be preferred overinput()
?
– Li-aung Yip
Mar 2 '12 at 6:06
1
1
use
raw_input
– Karoly Horvath
Mar 1 '12 at 16:33
use
raw_input
– Karoly Horvath
Mar 1 '12 at 16:33
@yi_H : could you please explain why
raw_input()
would be preferred over input()
?– Li-aung Yip
Mar 2 '12 at 6:06
@yi_H : could you please explain why
raw_input()
would be preferred over input()
?– Li-aung Yip
Mar 2 '12 at 6:06
add a comment |
5 Answers
5
active
oldest
votes
up vote
12
down vote
These need to be instance variables:
class simpleclass(object):
def __init__(self):
self.x = None
self.y = None
def getinput (self):
self.x = input("input value for x: ")
self.y = input("input value for y: ")
def calculate (self, z):
print self.x+self.y+z
1
+1 for most correct answer illustrating use of the __init__() method.
– Li-aung Yip
Mar 1 '12 at 16:38
add a comment |
up vote
5
down vote
You want to use self.x
and self.y
. Like so:
class simpleclass (object):
def getinput (self):
self.x = input("input value for x: ")
self.y = input("input value for y: ")
def calculate (self, z):
print self.x+self.y+z
@DSM: D'oh! Good catch. Edited to fix.
– Li-aung Yip
Mar 1 '12 at 16:37
add a comment |
up vote
2
down vote
Inside classes, there's a variable called self
you can use:
class Example(object):
def getinput(self):
self.x = input("input value for x: ")
def calculate(self, z):
print self.x + z
add a comment |
up vote
2
down vote
x
and y
are local variables. they get destroyed when you move out from the scope of that function.
class simpleclass (object):
def getinput (self):
self.x = raw_input("input value for x: ")
self.y = raw_input("input value for y: ")
def calculate (self, z):
print int(self.x)+int(self.y)+z
Probably want to get a number from the string at some point if you're switching to raw_input.
– DSM
Mar 1 '12 at 16:36
@DSM: right. thx
– Karoly Horvath
Mar 1 '12 at 16:40
add a comment |
up vote
0
down vote
class myClass(object):
def __init__(self):
def helper(self, jsonInputFile):
values = jsonInputFile['values']
ip = values['ip']
username = values['user']
password = values['password']
return values, ip, username, password
def checkHostname(self, jsonInputFile):
values, ip, username, password = self.helper
print values
print '---------'
print ip
print username
print password
the init method initializes the class.
the helper function just holds some variables/data/attributes and releases them to other methods when you call it. Here jsonInputFile is some json.
the checkHostname is a method written to log into some device/server and check the hostname but it needs ip, username and password for that and that is provided by calling the helper method.
add a comment |
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
12
down vote
These need to be instance variables:
class simpleclass(object):
def __init__(self):
self.x = None
self.y = None
def getinput (self):
self.x = input("input value for x: ")
self.y = input("input value for y: ")
def calculate (self, z):
print self.x+self.y+z
1
+1 for most correct answer illustrating use of the __init__() method.
– Li-aung Yip
Mar 1 '12 at 16:38
add a comment |
up vote
12
down vote
These need to be instance variables:
class simpleclass(object):
def __init__(self):
self.x = None
self.y = None
def getinput (self):
self.x = input("input value for x: ")
self.y = input("input value for y: ")
def calculate (self, z):
print self.x+self.y+z
1
+1 for most correct answer illustrating use of the __init__() method.
– Li-aung Yip
Mar 1 '12 at 16:38
add a comment |
up vote
12
down vote
up vote
12
down vote
These need to be instance variables:
class simpleclass(object):
def __init__(self):
self.x = None
self.y = None
def getinput (self):
self.x = input("input value for x: ")
self.y = input("input value for y: ")
def calculate (self, z):
print self.x+self.y+z
These need to be instance variables:
class simpleclass(object):
def __init__(self):
self.x = None
self.y = None
def getinput (self):
self.x = input("input value for x: ")
self.y = input("input value for y: ")
def calculate (self, z):
print self.x+self.y+z
answered Mar 1 '12 at 16:33
turtlebender
1,7271316
1,7271316
1
+1 for most correct answer illustrating use of the __init__() method.
– Li-aung Yip
Mar 1 '12 at 16:38
add a comment |
1
+1 for most correct answer illustrating use of the __init__() method.
– Li-aung Yip
Mar 1 '12 at 16:38
1
1
+1 for most correct answer illustrating use of the __init__() method.
– Li-aung Yip
Mar 1 '12 at 16:38
+1 for most correct answer illustrating use of the __init__() method.
– Li-aung Yip
Mar 1 '12 at 16:38
add a comment |
up vote
5
down vote
You want to use self.x
and self.y
. Like so:
class simpleclass (object):
def getinput (self):
self.x = input("input value for x: ")
self.y = input("input value for y: ")
def calculate (self, z):
print self.x+self.y+z
@DSM: D'oh! Good catch. Edited to fix.
– Li-aung Yip
Mar 1 '12 at 16:37
add a comment |
up vote
5
down vote
You want to use self.x
and self.y
. Like so:
class simpleclass (object):
def getinput (self):
self.x = input("input value for x: ")
self.y = input("input value for y: ")
def calculate (self, z):
print self.x+self.y+z
@DSM: D'oh! Good catch. Edited to fix.
– Li-aung Yip
Mar 1 '12 at 16:37
add a comment |
up vote
5
down vote
up vote
5
down vote
You want to use self.x
and self.y
. Like so:
class simpleclass (object):
def getinput (self):
self.x = input("input value for x: ")
self.y = input("input value for y: ")
def calculate (self, z):
print self.x+self.y+z
You want to use self.x
and self.y
. Like so:
class simpleclass (object):
def getinput (self):
self.x = input("input value for x: ")
self.y = input("input value for y: ")
def calculate (self, z):
print self.x+self.y+z
answered Mar 1 '12 at 16:32
Li-aung Yip
9,38142241
9,38142241
@DSM: D'oh! Good catch. Edited to fix.
– Li-aung Yip
Mar 1 '12 at 16:37
add a comment |
@DSM: D'oh! Good catch. Edited to fix.
– Li-aung Yip
Mar 1 '12 at 16:37
@DSM: D'oh! Good catch. Edited to fix.
– Li-aung Yip
Mar 1 '12 at 16:37
@DSM: D'oh! Good catch. Edited to fix.
– Li-aung Yip
Mar 1 '12 at 16:37
add a comment |
up vote
2
down vote
Inside classes, there's a variable called self
you can use:
class Example(object):
def getinput(self):
self.x = input("input value for x: ")
def calculate(self, z):
print self.x + z
add a comment |
up vote
2
down vote
Inside classes, there's a variable called self
you can use:
class Example(object):
def getinput(self):
self.x = input("input value for x: ")
def calculate(self, z):
print self.x + z
add a comment |
up vote
2
down vote
up vote
2
down vote
Inside classes, there's a variable called self
you can use:
class Example(object):
def getinput(self):
self.x = input("input value for x: ")
def calculate(self, z):
print self.x + z
Inside classes, there's a variable called self
you can use:
class Example(object):
def getinput(self):
self.x = input("input value for x: ")
def calculate(self, z):
print self.x + z
answered Mar 1 '12 at 16:33
Brendan Long
39.2k10113152
39.2k10113152
add a comment |
add a comment |
up vote
2
down vote
x
and y
are local variables. they get destroyed when you move out from the scope of that function.
class simpleclass (object):
def getinput (self):
self.x = raw_input("input value for x: ")
self.y = raw_input("input value for y: ")
def calculate (self, z):
print int(self.x)+int(self.y)+z
Probably want to get a number from the string at some point if you're switching to raw_input.
– DSM
Mar 1 '12 at 16:36
@DSM: right. thx
– Karoly Horvath
Mar 1 '12 at 16:40
add a comment |
up vote
2
down vote
x
and y
are local variables. they get destroyed when you move out from the scope of that function.
class simpleclass (object):
def getinput (self):
self.x = raw_input("input value for x: ")
self.y = raw_input("input value for y: ")
def calculate (self, z):
print int(self.x)+int(self.y)+z
Probably want to get a number from the string at some point if you're switching to raw_input.
– DSM
Mar 1 '12 at 16:36
@DSM: right. thx
– Karoly Horvath
Mar 1 '12 at 16:40
add a comment |
up vote
2
down vote
up vote
2
down vote
x
and y
are local variables. they get destroyed when you move out from the scope of that function.
class simpleclass (object):
def getinput (self):
self.x = raw_input("input value for x: ")
self.y = raw_input("input value for y: ")
def calculate (self, z):
print int(self.x)+int(self.y)+z
x
and y
are local variables. they get destroyed when you move out from the scope of that function.
class simpleclass (object):
def getinput (self):
self.x = raw_input("input value for x: ")
self.y = raw_input("input value for y: ")
def calculate (self, z):
print int(self.x)+int(self.y)+z
edited Mar 1 '12 at 16:39
answered Mar 1 '12 at 16:34
Karoly Horvath
77.6k1090155
77.6k1090155
Probably want to get a number from the string at some point if you're switching to raw_input.
– DSM
Mar 1 '12 at 16:36
@DSM: right. thx
– Karoly Horvath
Mar 1 '12 at 16:40
add a comment |
Probably want to get a number from the string at some point if you're switching to raw_input.
– DSM
Mar 1 '12 at 16:36
@DSM: right. thx
– Karoly Horvath
Mar 1 '12 at 16:40
Probably want to get a number from the string at some point if you're switching to raw_input.
– DSM
Mar 1 '12 at 16:36
Probably want to get a number from the string at some point if you're switching to raw_input.
– DSM
Mar 1 '12 at 16:36
@DSM: right. thx
– Karoly Horvath
Mar 1 '12 at 16:40
@DSM: right. thx
– Karoly Horvath
Mar 1 '12 at 16:40
add a comment |
up vote
0
down vote
class myClass(object):
def __init__(self):
def helper(self, jsonInputFile):
values = jsonInputFile['values']
ip = values['ip']
username = values['user']
password = values['password']
return values, ip, username, password
def checkHostname(self, jsonInputFile):
values, ip, username, password = self.helper
print values
print '---------'
print ip
print username
print password
the init method initializes the class.
the helper function just holds some variables/data/attributes and releases them to other methods when you call it. Here jsonInputFile is some json.
the checkHostname is a method written to log into some device/server and check the hostname but it needs ip, username and password for that and that is provided by calling the helper method.
add a comment |
up vote
0
down vote
class myClass(object):
def __init__(self):
def helper(self, jsonInputFile):
values = jsonInputFile['values']
ip = values['ip']
username = values['user']
password = values['password']
return values, ip, username, password
def checkHostname(self, jsonInputFile):
values, ip, username, password = self.helper
print values
print '---------'
print ip
print username
print password
the init method initializes the class.
the helper function just holds some variables/data/attributes and releases them to other methods when you call it. Here jsonInputFile is some json.
the checkHostname is a method written to log into some device/server and check the hostname but it needs ip, username and password for that and that is provided by calling the helper method.
add a comment |
up vote
0
down vote
up vote
0
down vote
class myClass(object):
def __init__(self):
def helper(self, jsonInputFile):
values = jsonInputFile['values']
ip = values['ip']
username = values['user']
password = values['password']
return values, ip, username, password
def checkHostname(self, jsonInputFile):
values, ip, username, password = self.helper
print values
print '---------'
print ip
print username
print password
the init method initializes the class.
the helper function just holds some variables/data/attributes and releases them to other methods when you call it. Here jsonInputFile is some json.
the checkHostname is a method written to log into some device/server and check the hostname but it needs ip, username and password for that and that is provided by calling the helper method.
class myClass(object):
def __init__(self):
def helper(self, jsonInputFile):
values = jsonInputFile['values']
ip = values['ip']
username = values['user']
password = values['password']
return values, ip, username, password
def checkHostname(self, jsonInputFile):
values, ip, username, password = self.helper
print values
print '---------'
print ip
print username
print password
the init method initializes the class.
the helper function just holds some variables/data/attributes and releases them to other methods when you call it. Here jsonInputFile is some json.
the checkHostname is a method written to log into some device/server and check the hostname but it needs ip, username and password for that and that is provided by calling the helper method.
answered Jun 28 '17 at 14:07


Gajendra D Ambi
8311013
8311013
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f9520075%2fpassing-variables-between-methods-in-python%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
NRjDxXAQCH 6n07Hn7,lE0,SprL1YNeJvnlGp
1
use
raw_input
– Karoly Horvath
Mar 1 '12 at 16:33
@yi_H : could you please explain why
raw_input()
would be preferred overinput()
?– Li-aung Yip
Mar 2 '12 at 6:06