Render_template second time after page is already loaded
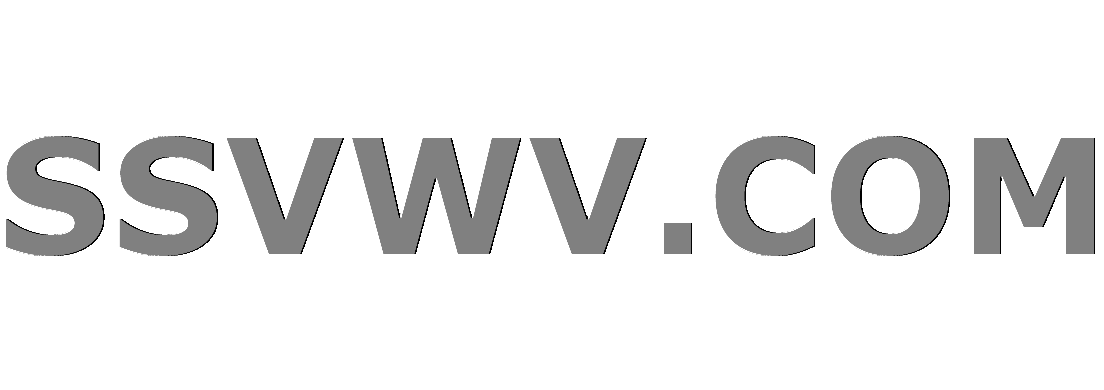
Multi tool use
up vote
1
down vote
favorite
I am trying to query a database through my website and dynamically add columns with the results using Jinja templates. I am using flask and on my views function. I am rendering the values like this
return render_template('query.html',my_list=my_list )
The thing is that when I load the page the user hasn't queried the database yet, so my_list is empty. The user queries the database by pressing a button on the html page and making an ajax request through jquery. My question now is if its possible to return my_list using jinja templates even after the page is already loaded, meaning I have to return render_template a second time (after submit button) to get the values for my_list.
p.s. I prefer not to use json parsing.
Here is some sample code. I query the database with the values of intranetID of column SubmitterID and platform of column Platforms to return the whole entry to the database with the values of the rest of the columns as well.
@app.route('/querydbvalues',methods=['POST', 'GET'])
def querydbvalues():
if request.method == 'POST' or request.method == 'GET':
results = models.mydatabase.query.filter_by(SubmitterID=qIntranetID,Platforms=qPlatform).all()
my_list = [i.user for i in results]
return render_template('query.html',my_list=my_list )
and on my html page
% for n in my_list %
<li>n</li>
% endfor %
on the Javascript file when you press the button i make the ajax request like this
$("#SearchDatabase").click(function()
var tmp = document.getElementById("qIntranetID").value;
var tmp2 = document.getElementById("qPlatform").value;
jQuery.ajax(
dataType: "json",
url:"/querydbvalues", //tell the script where to send requests
data:text:tmp,text2:tmp2,
type:'GET',
contentType: 'application/json',
success: function(results)
//do something
);
);
python ajax flask rendering jinja2
add a comment |
up vote
1
down vote
favorite
I am trying to query a database through my website and dynamically add columns with the results using Jinja templates. I am using flask and on my views function. I am rendering the values like this
return render_template('query.html',my_list=my_list )
The thing is that when I load the page the user hasn't queried the database yet, so my_list is empty. The user queries the database by pressing a button on the html page and making an ajax request through jquery. My question now is if its possible to return my_list using jinja templates even after the page is already loaded, meaning I have to return render_template a second time (after submit button) to get the values for my_list.
p.s. I prefer not to use json parsing.
Here is some sample code. I query the database with the values of intranetID of column SubmitterID and platform of column Platforms to return the whole entry to the database with the values of the rest of the columns as well.
@app.route('/querydbvalues',methods=['POST', 'GET'])
def querydbvalues():
if request.method == 'POST' or request.method == 'GET':
results = models.mydatabase.query.filter_by(SubmitterID=qIntranetID,Platforms=qPlatform).all()
my_list = [i.user for i in results]
return render_template('query.html',my_list=my_list )
and on my html page
% for n in my_list %
<li>n</li>
% endfor %
on the Javascript file when you press the button i make the ajax request like this
$("#SearchDatabase").click(function()
var tmp = document.getElementById("qIntranetID").value;
var tmp2 = document.getElementById("qPlatform").value;
jQuery.ajax(
dataType: "json",
url:"/querydbvalues", //tell the script where to send requests
data:text:tmp,text2:tmp2,
type:'GET',
contentType: 'application/json',
success: function(results)
//do something
);
);
python ajax flask rendering jinja2
You don't need to check the request method. Flask will only route GET and POST requests to that endpoint.
– dirn
Mar 24 '16 at 22:32
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I am trying to query a database through my website and dynamically add columns with the results using Jinja templates. I am using flask and on my views function. I am rendering the values like this
return render_template('query.html',my_list=my_list )
The thing is that when I load the page the user hasn't queried the database yet, so my_list is empty. The user queries the database by pressing a button on the html page and making an ajax request through jquery. My question now is if its possible to return my_list using jinja templates even after the page is already loaded, meaning I have to return render_template a second time (after submit button) to get the values for my_list.
p.s. I prefer not to use json parsing.
Here is some sample code. I query the database with the values of intranetID of column SubmitterID and platform of column Platforms to return the whole entry to the database with the values of the rest of the columns as well.
@app.route('/querydbvalues',methods=['POST', 'GET'])
def querydbvalues():
if request.method == 'POST' or request.method == 'GET':
results = models.mydatabase.query.filter_by(SubmitterID=qIntranetID,Platforms=qPlatform).all()
my_list = [i.user for i in results]
return render_template('query.html',my_list=my_list )
and on my html page
% for n in my_list %
<li>n</li>
% endfor %
on the Javascript file when you press the button i make the ajax request like this
$("#SearchDatabase").click(function()
var tmp = document.getElementById("qIntranetID").value;
var tmp2 = document.getElementById("qPlatform").value;
jQuery.ajax(
dataType: "json",
url:"/querydbvalues", //tell the script where to send requests
data:text:tmp,text2:tmp2,
type:'GET',
contentType: 'application/json',
success: function(results)
//do something
);
);
python ajax flask rendering jinja2
I am trying to query a database through my website and dynamically add columns with the results using Jinja templates. I am using flask and on my views function. I am rendering the values like this
return render_template('query.html',my_list=my_list )
The thing is that when I load the page the user hasn't queried the database yet, so my_list is empty. The user queries the database by pressing a button on the html page and making an ajax request through jquery. My question now is if its possible to return my_list using jinja templates even after the page is already loaded, meaning I have to return render_template a second time (after submit button) to get the values for my_list.
p.s. I prefer not to use json parsing.
Here is some sample code. I query the database with the values of intranetID of column SubmitterID and platform of column Platforms to return the whole entry to the database with the values of the rest of the columns as well.
@app.route('/querydbvalues',methods=['POST', 'GET'])
def querydbvalues():
if request.method == 'POST' or request.method == 'GET':
results = models.mydatabase.query.filter_by(SubmitterID=qIntranetID,Platforms=qPlatform).all()
my_list = [i.user for i in results]
return render_template('query.html',my_list=my_list )
and on my html page
% for n in my_list %
<li>n</li>
% endfor %
on the Javascript file when you press the button i make the ajax request like this
$("#SearchDatabase").click(function()
var tmp = document.getElementById("qIntranetID").value;
var tmp2 = document.getElementById("qPlatform").value;
jQuery.ajax(
dataType: "json",
url:"/querydbvalues", //tell the script where to send requests
data:text:tmp,text2:tmp2,
type:'GET',
contentType: 'application/json',
success: function(results)
//do something
);
);
python ajax flask rendering jinja2
python ajax flask rendering jinja2
edited Nov 10 at 10:13
Flimzy
36.7k96496
36.7k96496
asked Mar 24 '16 at 18:55
PetrosM
17810
17810
You don't need to check the request method. Flask will only route GET and POST requests to that endpoint.
– dirn
Mar 24 '16 at 22:32
add a comment |
You don't need to check the request method. Flask will only route GET and POST requests to that endpoint.
– dirn
Mar 24 '16 at 22:32
You don't need to check the request method. Flask will only route GET and POST requests to that endpoint.
– dirn
Mar 24 '16 at 22:32
You don't need to check the request method. Flask will only route GET and POST requests to that endpoint.
– dirn
Mar 24 '16 at 22:32
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
The Ajax call is a completely separate request. You can do what you want in that request, including render templates.
Hi, thanks for your input. However my problem is that i want to render "my_list" on the same html page without refreshing or changing to another page. And for some reason i cant and thats why i asked for help
– PetrosM
Mar 24 '16 at 19:06
Well that is what Ajax does. You should maybe explain why you're having problems rendering the same page, rather than saying "for some reason I can't".
– Daniel Roseman
Mar 24 '16 at 19:29
i dont have problem rendering the same page. I have problem rendering the value on "my_list" (the second argument i render after the html page) and using those values with jinja template on my webpage.
– PetrosM
Mar 24 '16 at 19:31
You're going to need to show some code.
– Daniel Roseman
Mar 24 '16 at 19:31
1
Hello, first of all thank you for baring with me and still trying to help me. by "return render_template('query.html',my_list=my_list )" i return my results my_list and with jinja templating i can just use the my_list values on my html page like i described on the code above. however when the page is first loaded my_list = and only changes values when the user presses submit and the querying to the database happens. Once i get the values i want to my_list i want to return them again to use with jinja templating. This doesn't happen and i cant return the new values of my_list.
– PetrosM
Mar 25 '16 at 13:40
|
show 2 more comments
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
The Ajax call is a completely separate request. You can do what you want in that request, including render templates.
Hi, thanks for your input. However my problem is that i want to render "my_list" on the same html page without refreshing or changing to another page. And for some reason i cant and thats why i asked for help
– PetrosM
Mar 24 '16 at 19:06
Well that is what Ajax does. You should maybe explain why you're having problems rendering the same page, rather than saying "for some reason I can't".
– Daniel Roseman
Mar 24 '16 at 19:29
i dont have problem rendering the same page. I have problem rendering the value on "my_list" (the second argument i render after the html page) and using those values with jinja template on my webpage.
– PetrosM
Mar 24 '16 at 19:31
You're going to need to show some code.
– Daniel Roseman
Mar 24 '16 at 19:31
1
Hello, first of all thank you for baring with me and still trying to help me. by "return render_template('query.html',my_list=my_list )" i return my results my_list and with jinja templating i can just use the my_list values on my html page like i described on the code above. however when the page is first loaded my_list = and only changes values when the user presses submit and the querying to the database happens. Once i get the values i want to my_list i want to return them again to use with jinja templating. This doesn't happen and i cant return the new values of my_list.
– PetrosM
Mar 25 '16 at 13:40
|
show 2 more comments
up vote
0
down vote
The Ajax call is a completely separate request. You can do what you want in that request, including render templates.
Hi, thanks for your input. However my problem is that i want to render "my_list" on the same html page without refreshing or changing to another page. And for some reason i cant and thats why i asked for help
– PetrosM
Mar 24 '16 at 19:06
Well that is what Ajax does. You should maybe explain why you're having problems rendering the same page, rather than saying "for some reason I can't".
– Daniel Roseman
Mar 24 '16 at 19:29
i dont have problem rendering the same page. I have problem rendering the value on "my_list" (the second argument i render after the html page) and using those values with jinja template on my webpage.
– PetrosM
Mar 24 '16 at 19:31
You're going to need to show some code.
– Daniel Roseman
Mar 24 '16 at 19:31
1
Hello, first of all thank you for baring with me and still trying to help me. by "return render_template('query.html',my_list=my_list )" i return my results my_list and with jinja templating i can just use the my_list values on my html page like i described on the code above. however when the page is first loaded my_list = and only changes values when the user presses submit and the querying to the database happens. Once i get the values i want to my_list i want to return them again to use with jinja templating. This doesn't happen and i cant return the new values of my_list.
– PetrosM
Mar 25 '16 at 13:40
|
show 2 more comments
up vote
0
down vote
up vote
0
down vote
The Ajax call is a completely separate request. You can do what you want in that request, including render templates.
The Ajax call is a completely separate request. You can do what you want in that request, including render templates.
answered Mar 24 '16 at 18:58
Daniel Roseman
441k40573627
441k40573627
Hi, thanks for your input. However my problem is that i want to render "my_list" on the same html page without refreshing or changing to another page. And for some reason i cant and thats why i asked for help
– PetrosM
Mar 24 '16 at 19:06
Well that is what Ajax does. You should maybe explain why you're having problems rendering the same page, rather than saying "for some reason I can't".
– Daniel Roseman
Mar 24 '16 at 19:29
i dont have problem rendering the same page. I have problem rendering the value on "my_list" (the second argument i render after the html page) and using those values with jinja template on my webpage.
– PetrosM
Mar 24 '16 at 19:31
You're going to need to show some code.
– Daniel Roseman
Mar 24 '16 at 19:31
1
Hello, first of all thank you for baring with me and still trying to help me. by "return render_template('query.html',my_list=my_list )" i return my results my_list and with jinja templating i can just use the my_list values on my html page like i described on the code above. however when the page is first loaded my_list = and only changes values when the user presses submit and the querying to the database happens. Once i get the values i want to my_list i want to return them again to use with jinja templating. This doesn't happen and i cant return the new values of my_list.
– PetrosM
Mar 25 '16 at 13:40
|
show 2 more comments
Hi, thanks for your input. However my problem is that i want to render "my_list" on the same html page without refreshing or changing to another page. And for some reason i cant and thats why i asked for help
– PetrosM
Mar 24 '16 at 19:06
Well that is what Ajax does. You should maybe explain why you're having problems rendering the same page, rather than saying "for some reason I can't".
– Daniel Roseman
Mar 24 '16 at 19:29
i dont have problem rendering the same page. I have problem rendering the value on "my_list" (the second argument i render after the html page) and using those values with jinja template on my webpage.
– PetrosM
Mar 24 '16 at 19:31
You're going to need to show some code.
– Daniel Roseman
Mar 24 '16 at 19:31
1
Hello, first of all thank you for baring with me and still trying to help me. by "return render_template('query.html',my_list=my_list )" i return my results my_list and with jinja templating i can just use the my_list values on my html page like i described on the code above. however when the page is first loaded my_list = and only changes values when the user presses submit and the querying to the database happens. Once i get the values i want to my_list i want to return them again to use with jinja templating. This doesn't happen and i cant return the new values of my_list.
– PetrosM
Mar 25 '16 at 13:40
Hi, thanks for your input. However my problem is that i want to render "my_list" on the same html page without refreshing or changing to another page. And for some reason i cant and thats why i asked for help
– PetrosM
Mar 24 '16 at 19:06
Hi, thanks for your input. However my problem is that i want to render "my_list" on the same html page without refreshing or changing to another page. And for some reason i cant and thats why i asked for help
– PetrosM
Mar 24 '16 at 19:06
Well that is what Ajax does. You should maybe explain why you're having problems rendering the same page, rather than saying "for some reason I can't".
– Daniel Roseman
Mar 24 '16 at 19:29
Well that is what Ajax does. You should maybe explain why you're having problems rendering the same page, rather than saying "for some reason I can't".
– Daniel Roseman
Mar 24 '16 at 19:29
i dont have problem rendering the same page. I have problem rendering the value on "my_list" (the second argument i render after the html page) and using those values with jinja template on my webpage.
– PetrosM
Mar 24 '16 at 19:31
i dont have problem rendering the same page. I have problem rendering the value on "my_list" (the second argument i render after the html page) and using those values with jinja template on my webpage.
– PetrosM
Mar 24 '16 at 19:31
You're going to need to show some code.
– Daniel Roseman
Mar 24 '16 at 19:31
You're going to need to show some code.
– Daniel Roseman
Mar 24 '16 at 19:31
1
1
Hello, first of all thank you for baring with me and still trying to help me. by "return render_template('query.html',my_list=my_list )" i return my results my_list and with jinja templating i can just use the my_list values on my html page like i described on the code above. however when the page is first loaded my_list = and only changes values when the user presses submit and the querying to the database happens. Once i get the values i want to my_list i want to return them again to use with jinja templating. This doesn't happen and i cant return the new values of my_list.
– PetrosM
Mar 25 '16 at 13:40
Hello, first of all thank you for baring with me and still trying to help me. by "return render_template('query.html',my_list=my_list )" i return my results my_list and with jinja templating i can just use the my_list values on my html page like i described on the code above. however when the page is first loaded my_list = and only changes values when the user presses submit and the querying to the database happens. Once i get the values i want to my_list i want to return them again to use with jinja templating. This doesn't happen and i cant return the new values of my_list.
– PetrosM
Mar 25 '16 at 13:40
|
show 2 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f36207962%2frender-template-second-time-after-page-is-already-loaded%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pt8EwWdRyuDDK,W5YAOsicSx dX0sxIx4onUu90ozUodX2q Xsj9KUEi5gUxevwok2QnXE9qfuM t
You don't need to check the request method. Flask will only route GET and POST requests to that endpoint.
– dirn
Mar 24 '16 at 22:32