ImageMagick White to Transparent Background While Maintaining White Object
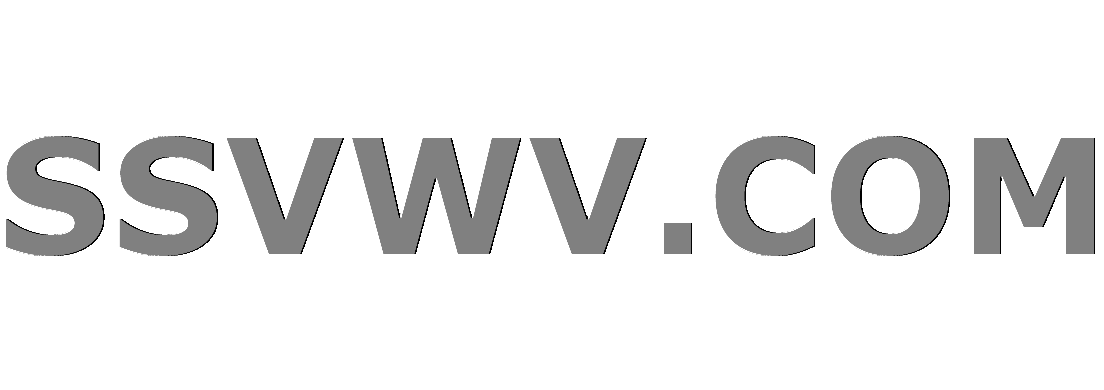
Multi tool use
I am using PHP's ImageMagick to turn the white background of an image, transparent. I pass the image URL to this PHP script and it returns the image.
<?php
# grab the remote image url
$imgUrl = $_GET['img'];
# create new ImageMagick object
$im = new Imagick($imgUrl);
# remove extra white space
$im->clipImage(0);
# convert white background to transparent
$im->paintTransparentImage($im->getImageBackgroundColor(), 0, 3000);
# resize image --- passing 0 as width invokes proportional scaling
$im->resizeImage(0, 200, Imagick::FILTER_LANCZOS, 1);
# set resulting image format as png
$im->setImageFormat('png');
# set header type as PNG image
header('Content-Type: image/png');
# output the new image
echo $im->getImageBlob();
These transformations work pretty well. However, if I have an image with a white object, it doesn't work very well as a result of passing a fuzz value to paintTransparentImage(); which is how I am cleaning up jagged edges.
Here's an example of the result, note the white sofa:
If I don't pass a fuzz value, then I get a proper cut, but i'm left with messy edges:
I have attempted to use resizeImage() in an effort to achieve what's called 'spatial anti-aliasing' (blow up the image really big -> use paintTransparentImage() -> shrink image back down), but I didn't notice any significant changes.
Is there something I can do to better handle these really white images? I've played about with trimImage() and edgeImage(), but I'm not able to get the results i'm after.
Worst case scenario, (though not ideal), is there a means of identifying if an image contains some percentage of a particular colour? IE. if image contains >90% white pixels then I could run paintTransparentImage() with a fuzz value of 0 instead of 3000 which would at least give me a proper cut.
Thanks.
php imagemagick
add a comment |
I am using PHP's ImageMagick to turn the white background of an image, transparent. I pass the image URL to this PHP script and it returns the image.
<?php
# grab the remote image url
$imgUrl = $_GET['img'];
# create new ImageMagick object
$im = new Imagick($imgUrl);
# remove extra white space
$im->clipImage(0);
# convert white background to transparent
$im->paintTransparentImage($im->getImageBackgroundColor(), 0, 3000);
# resize image --- passing 0 as width invokes proportional scaling
$im->resizeImage(0, 200, Imagick::FILTER_LANCZOS, 1);
# set resulting image format as png
$im->setImageFormat('png');
# set header type as PNG image
header('Content-Type: image/png');
# output the new image
echo $im->getImageBlob();
These transformations work pretty well. However, if I have an image with a white object, it doesn't work very well as a result of passing a fuzz value to paintTransparentImage(); which is how I am cleaning up jagged edges.
Here's an example of the result, note the white sofa:
If I don't pass a fuzz value, then I get a proper cut, but i'm left with messy edges:
I have attempted to use resizeImage() in an effort to achieve what's called 'spatial anti-aliasing' (blow up the image really big -> use paintTransparentImage() -> shrink image back down), but I didn't notice any significant changes.
Is there something I can do to better handle these really white images? I've played about with trimImage() and edgeImage(), but I'm not able to get the results i'm after.
Worst case scenario, (though not ideal), is there a means of identifying if an image contains some percentage of a particular colour? IE. if image contains >90% white pixels then I could run paintTransparentImage() with a fuzz value of 0 instead of 3000 which would at least give me a proper cut.
Thanks.
php imagemagick
Solved. Edited post to show solution.
– Crayons
Apr 21 '17 at 15:38
add a comment |
I am using PHP's ImageMagick to turn the white background of an image, transparent. I pass the image URL to this PHP script and it returns the image.
<?php
# grab the remote image url
$imgUrl = $_GET['img'];
# create new ImageMagick object
$im = new Imagick($imgUrl);
# remove extra white space
$im->clipImage(0);
# convert white background to transparent
$im->paintTransparentImage($im->getImageBackgroundColor(), 0, 3000);
# resize image --- passing 0 as width invokes proportional scaling
$im->resizeImage(0, 200, Imagick::FILTER_LANCZOS, 1);
# set resulting image format as png
$im->setImageFormat('png');
# set header type as PNG image
header('Content-Type: image/png');
# output the new image
echo $im->getImageBlob();
These transformations work pretty well. However, if I have an image with a white object, it doesn't work very well as a result of passing a fuzz value to paintTransparentImage(); which is how I am cleaning up jagged edges.
Here's an example of the result, note the white sofa:
If I don't pass a fuzz value, then I get a proper cut, but i'm left with messy edges:
I have attempted to use resizeImage() in an effort to achieve what's called 'spatial anti-aliasing' (blow up the image really big -> use paintTransparentImage() -> shrink image back down), but I didn't notice any significant changes.
Is there something I can do to better handle these really white images? I've played about with trimImage() and edgeImage(), but I'm not able to get the results i'm after.
Worst case scenario, (though not ideal), is there a means of identifying if an image contains some percentage of a particular colour? IE. if image contains >90% white pixels then I could run paintTransparentImage() with a fuzz value of 0 instead of 3000 which would at least give me a proper cut.
Thanks.
php imagemagick
I am using PHP's ImageMagick to turn the white background of an image, transparent. I pass the image URL to this PHP script and it returns the image.
<?php
# grab the remote image url
$imgUrl = $_GET['img'];
# create new ImageMagick object
$im = new Imagick($imgUrl);
# remove extra white space
$im->clipImage(0);
# convert white background to transparent
$im->paintTransparentImage($im->getImageBackgroundColor(), 0, 3000);
# resize image --- passing 0 as width invokes proportional scaling
$im->resizeImage(0, 200, Imagick::FILTER_LANCZOS, 1);
# set resulting image format as png
$im->setImageFormat('png');
# set header type as PNG image
header('Content-Type: image/png');
# output the new image
echo $im->getImageBlob();
These transformations work pretty well. However, if I have an image with a white object, it doesn't work very well as a result of passing a fuzz value to paintTransparentImage(); which is how I am cleaning up jagged edges.
Here's an example of the result, note the white sofa:
If I don't pass a fuzz value, then I get a proper cut, but i'm left with messy edges:
I have attempted to use resizeImage() in an effort to achieve what's called 'spatial anti-aliasing' (blow up the image really big -> use paintTransparentImage() -> shrink image back down), but I didn't notice any significant changes.
Is there something I can do to better handle these really white images? I've played about with trimImage() and edgeImage(), but I'm not able to get the results i'm after.
Worst case scenario, (though not ideal), is there a means of identifying if an image contains some percentage of a particular colour? IE. if image contains >90% white pixels then I could run paintTransparentImage() with a fuzz value of 0 instead of 3000 which would at least give me a proper cut.
Thanks.
php imagemagick
php imagemagick
edited Nov 14 '18 at 5:49
Crayons
asked Apr 21 '17 at 13:49


CrayonsCrayons
166113
166113
Solved. Edited post to show solution.
– Crayons
Apr 21 '17 at 15:38
add a comment |
Solved. Edited post to show solution.
– Crayons
Apr 21 '17 at 15:38
Solved. Edited post to show solution.
– Crayons
Apr 21 '17 at 15:38
Solved. Edited post to show solution.
– Crayons
Apr 21 '17 at 15:38
add a comment |
1 Answer
1
active
oldest
votes
SOLUTION:
Replace white background with some other colour first, then change that colour to transparent.
<?php
# get img url
$imgUrl = $_GET['img'];
# create new ImageMagick object from image url
$im = new Imagick($imgUrl);
# replace white background with fuchsia
$im->floodFillPaintImage("rgb(255, 0, 255)", 2500, "rgb(255,255,255)", 0 , 0, false);
#make fuchsia transparent
$im->paintTransparentImage("rgb(255,0,255)", 0, 10);
# resize image --- passing 0 as width invokes proportional scaling
$im->resizeImage(0, 200, Imagick::FILTER_LANCZOS, 1);
# set resulting image format as png
$im->setImageFormat('png');
# set header type as PNG image
header('Content-Type: image/png');
# output the new image
echo $im->getImageBlob();
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f43544467%2fimagemagick-white-to-transparent-background-while-maintaining-white-object%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
SOLUTION:
Replace white background with some other colour first, then change that colour to transparent.
<?php
# get img url
$imgUrl = $_GET['img'];
# create new ImageMagick object from image url
$im = new Imagick($imgUrl);
# replace white background with fuchsia
$im->floodFillPaintImage("rgb(255, 0, 255)", 2500, "rgb(255,255,255)", 0 , 0, false);
#make fuchsia transparent
$im->paintTransparentImage("rgb(255,0,255)", 0, 10);
# resize image --- passing 0 as width invokes proportional scaling
$im->resizeImage(0, 200, Imagick::FILTER_LANCZOS, 1);
# set resulting image format as png
$im->setImageFormat('png');
# set header type as PNG image
header('Content-Type: image/png');
# output the new image
echo $im->getImageBlob();
add a comment |
SOLUTION:
Replace white background with some other colour first, then change that colour to transparent.
<?php
# get img url
$imgUrl = $_GET['img'];
# create new ImageMagick object from image url
$im = new Imagick($imgUrl);
# replace white background with fuchsia
$im->floodFillPaintImage("rgb(255, 0, 255)", 2500, "rgb(255,255,255)", 0 , 0, false);
#make fuchsia transparent
$im->paintTransparentImage("rgb(255,0,255)", 0, 10);
# resize image --- passing 0 as width invokes proportional scaling
$im->resizeImage(0, 200, Imagick::FILTER_LANCZOS, 1);
# set resulting image format as png
$im->setImageFormat('png');
# set header type as PNG image
header('Content-Type: image/png');
# output the new image
echo $im->getImageBlob();
add a comment |
SOLUTION:
Replace white background with some other colour first, then change that colour to transparent.
<?php
# get img url
$imgUrl = $_GET['img'];
# create new ImageMagick object from image url
$im = new Imagick($imgUrl);
# replace white background with fuchsia
$im->floodFillPaintImage("rgb(255, 0, 255)", 2500, "rgb(255,255,255)", 0 , 0, false);
#make fuchsia transparent
$im->paintTransparentImage("rgb(255,0,255)", 0, 10);
# resize image --- passing 0 as width invokes proportional scaling
$im->resizeImage(0, 200, Imagick::FILTER_LANCZOS, 1);
# set resulting image format as png
$im->setImageFormat('png');
# set header type as PNG image
header('Content-Type: image/png');
# output the new image
echo $im->getImageBlob();
SOLUTION:
Replace white background with some other colour first, then change that colour to transparent.
<?php
# get img url
$imgUrl = $_GET['img'];
# create new ImageMagick object from image url
$im = new Imagick($imgUrl);
# replace white background with fuchsia
$im->floodFillPaintImage("rgb(255, 0, 255)", 2500, "rgb(255,255,255)", 0 , 0, false);
#make fuchsia transparent
$im->paintTransparentImage("rgb(255,0,255)", 0, 10);
# resize image --- passing 0 as width invokes proportional scaling
$im->resizeImage(0, 200, Imagick::FILTER_LANCZOS, 1);
# set resulting image format as png
$im->setImageFormat('png');
# set header type as PNG image
header('Content-Type: image/png');
# output the new image
echo $im->getImageBlob();
edited Nov 14 '18 at 5:51
answered Apr 21 '17 at 15:43


CrayonsCrayons
166113
166113
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f43544467%2fimagemagick-white-to-transparent-background-while-maintaining-white-object%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
QDLLzWWJZ3pFo06q2UhPsO
Solved. Edited post to show solution.
– Crayons
Apr 21 '17 at 15:38