Django models don't work with Oracle database
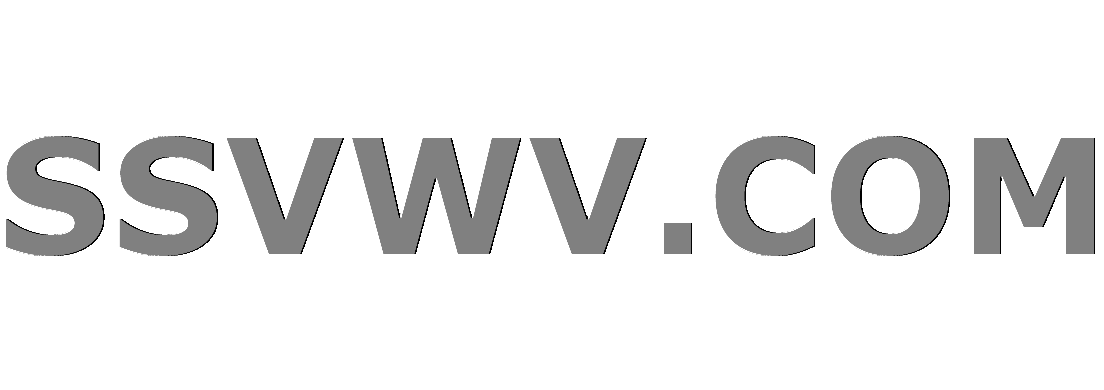
Multi tool use
up vote
1
down vote
favorite
I have several apps with different database connections. They all work fine.
My new app needs an Oracle connection, which is set up in the settings.py
:
'database_oracle' :
'ENGINE' : 'oracle',
'NAME' : 'name',
'USER' : 'user',
'PASSWORD' : 'pw',
'HOST' : 'connection',
'PORT' : 1234,
'SCHMEA' : 'abc',
,
The models in my models.py
have additional meta classes:
class Meta:
db_table = '"abc.table1"'
data_source = 'database_oracle'
data_source_app = 'myapp'
The problem is that
Model1.objects.all()
returns an error: `
django.db.utils.DatabaseError: ORA-00942: table or view does not exist
But if I use the django.db
connection:
from django.db import connections
db_conn = connections['database_oracle']
c = db_conn.cursor()
c.execute("SELECT MAX(date) FROM abc.table1 WHERE key = 1234567").fetchall()
It works perfectly fine.
So I tried different spellings for db_table:
db_table = 'table1'
db_table = '"table1"'
db_table = 'abc.table1'
db_table = '"abc.table1"'
Changes were migrtated every time and this is how my routers.py
look like (the if-statements belong to other apps and other databases):
myapps = ['myapp']
class DataRouter:
def db_for_read(self, model, **hints):
if [...]
elif model._meta.app_label in myapps:
return 'database_oracle'
return None
def db_for_write(self,model, **hints):
if [...]
elif model._meta.app_label in myapps:
return 'database_oracle'
return None
def allow_relation(self, obj1, obj2, **hints):
if [...]
elif obj1._meta.app_label in myapps or obj2._meta.app_label in myapps:
return True
return None
def allow_migrate(self, db, app_label, model_name=None, **hints):
if [...]
elif app_label in myapps:
return db =='database_oracle'
return None
Does anyone have any idea why the models can't connect to my database?
python django oracle django-models
add a comment |
up vote
1
down vote
favorite
I have several apps with different database connections. They all work fine.
My new app needs an Oracle connection, which is set up in the settings.py
:
'database_oracle' :
'ENGINE' : 'oracle',
'NAME' : 'name',
'USER' : 'user',
'PASSWORD' : 'pw',
'HOST' : 'connection',
'PORT' : 1234,
'SCHMEA' : 'abc',
,
The models in my models.py
have additional meta classes:
class Meta:
db_table = '"abc.table1"'
data_source = 'database_oracle'
data_source_app = 'myapp'
The problem is that
Model1.objects.all()
returns an error: `
django.db.utils.DatabaseError: ORA-00942: table or view does not exist
But if I use the django.db
connection:
from django.db import connections
db_conn = connections['database_oracle']
c = db_conn.cursor()
c.execute("SELECT MAX(date) FROM abc.table1 WHERE key = 1234567").fetchall()
It works perfectly fine.
So I tried different spellings for db_table:
db_table = 'table1'
db_table = '"table1"'
db_table = 'abc.table1'
db_table = '"abc.table1"'
Changes were migrtated every time and this is how my routers.py
look like (the if-statements belong to other apps and other databases):
myapps = ['myapp']
class DataRouter:
def db_for_read(self, model, **hints):
if [...]
elif model._meta.app_label in myapps:
return 'database_oracle'
return None
def db_for_write(self,model, **hints):
if [...]
elif model._meta.app_label in myapps:
return 'database_oracle'
return None
def allow_relation(self, obj1, obj2, **hints):
if [...]
elif obj1._meta.app_label in myapps or obj2._meta.app_label in myapps:
return True
return None
def allow_migrate(self, db, app_label, model_name=None, **hints):
if [...]
elif app_label in myapps:
return db =='database_oracle'
return None
Does anyone have any idea why the models can't connect to my database?
python django oracle django-models
did you triedModel1.objects.using("database_oracle").all()
?
– dorintufar
Nov 9 at 12:22
1
also try to remove unnecessary double quotes fromdb_table = '"abc.table1"'
inMeta
class
– dorintufar
Nov 9 at 12:23
@dorintufar thanks so far and yes, I tried it but the Traceback is still the same. And I tried the double quotes as Django suggests to use them for oracle dbs: docs.djangoproject.com/en/2.0/ref/models/options
– Andrea
Nov 9 at 13:31
1
You've trieddb_table = '"abc"."table1"'
?
– Will Keeling
Nov 9 at 17:46
@WillKeeling that was the answer :). Thanks so much!
– Andrea
22 hours ago
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have several apps with different database connections. They all work fine.
My new app needs an Oracle connection, which is set up in the settings.py
:
'database_oracle' :
'ENGINE' : 'oracle',
'NAME' : 'name',
'USER' : 'user',
'PASSWORD' : 'pw',
'HOST' : 'connection',
'PORT' : 1234,
'SCHMEA' : 'abc',
,
The models in my models.py
have additional meta classes:
class Meta:
db_table = '"abc.table1"'
data_source = 'database_oracle'
data_source_app = 'myapp'
The problem is that
Model1.objects.all()
returns an error: `
django.db.utils.DatabaseError: ORA-00942: table or view does not exist
But if I use the django.db
connection:
from django.db import connections
db_conn = connections['database_oracle']
c = db_conn.cursor()
c.execute("SELECT MAX(date) FROM abc.table1 WHERE key = 1234567").fetchall()
It works perfectly fine.
So I tried different spellings for db_table:
db_table = 'table1'
db_table = '"table1"'
db_table = 'abc.table1'
db_table = '"abc.table1"'
Changes were migrtated every time and this is how my routers.py
look like (the if-statements belong to other apps and other databases):
myapps = ['myapp']
class DataRouter:
def db_for_read(self, model, **hints):
if [...]
elif model._meta.app_label in myapps:
return 'database_oracle'
return None
def db_for_write(self,model, **hints):
if [...]
elif model._meta.app_label in myapps:
return 'database_oracle'
return None
def allow_relation(self, obj1, obj2, **hints):
if [...]
elif obj1._meta.app_label in myapps or obj2._meta.app_label in myapps:
return True
return None
def allow_migrate(self, db, app_label, model_name=None, **hints):
if [...]
elif app_label in myapps:
return db =='database_oracle'
return None
Does anyone have any idea why the models can't connect to my database?
python django oracle django-models
I have several apps with different database connections. They all work fine.
My new app needs an Oracle connection, which is set up in the settings.py
:
'database_oracle' :
'ENGINE' : 'oracle',
'NAME' : 'name',
'USER' : 'user',
'PASSWORD' : 'pw',
'HOST' : 'connection',
'PORT' : 1234,
'SCHMEA' : 'abc',
,
The models in my models.py
have additional meta classes:
class Meta:
db_table = '"abc.table1"'
data_source = 'database_oracle'
data_source_app = 'myapp'
The problem is that
Model1.objects.all()
returns an error: `
django.db.utils.DatabaseError: ORA-00942: table or view does not exist
But if I use the django.db
connection:
from django.db import connections
db_conn = connections['database_oracle']
c = db_conn.cursor()
c.execute("SELECT MAX(date) FROM abc.table1 WHERE key = 1234567").fetchall()
It works perfectly fine.
So I tried different spellings for db_table:
db_table = 'table1'
db_table = '"table1"'
db_table = 'abc.table1'
db_table = '"abc.table1"'
Changes were migrtated every time and this is how my routers.py
look like (the if-statements belong to other apps and other databases):
myapps = ['myapp']
class DataRouter:
def db_for_read(self, model, **hints):
if [...]
elif model._meta.app_label in myapps:
return 'database_oracle'
return None
def db_for_write(self,model, **hints):
if [...]
elif model._meta.app_label in myapps:
return 'database_oracle'
return None
def allow_relation(self, obj1, obj2, **hints):
if [...]
elif obj1._meta.app_label in myapps or obj2._meta.app_label in myapps:
return True
return None
def allow_migrate(self, db, app_label, model_name=None, **hints):
if [...]
elif app_label in myapps:
return db =='database_oracle'
return None
Does anyone have any idea why the models can't connect to my database?
python django oracle django-models
python django oracle django-models
edited Nov 9 at 12:22


Vineeth Sai
2,09231022
2,09231022
asked Nov 9 at 12:19
Andrea
152
152
did you triedModel1.objects.using("database_oracle").all()
?
– dorintufar
Nov 9 at 12:22
1
also try to remove unnecessary double quotes fromdb_table = '"abc.table1"'
inMeta
class
– dorintufar
Nov 9 at 12:23
@dorintufar thanks so far and yes, I tried it but the Traceback is still the same. And I tried the double quotes as Django suggests to use them for oracle dbs: docs.djangoproject.com/en/2.0/ref/models/options
– Andrea
Nov 9 at 13:31
1
You've trieddb_table = '"abc"."table1"'
?
– Will Keeling
Nov 9 at 17:46
@WillKeeling that was the answer :). Thanks so much!
– Andrea
22 hours ago
add a comment |
did you triedModel1.objects.using("database_oracle").all()
?
– dorintufar
Nov 9 at 12:22
1
also try to remove unnecessary double quotes fromdb_table = '"abc.table1"'
inMeta
class
– dorintufar
Nov 9 at 12:23
@dorintufar thanks so far and yes, I tried it but the Traceback is still the same. And I tried the double quotes as Django suggests to use them for oracle dbs: docs.djangoproject.com/en/2.0/ref/models/options
– Andrea
Nov 9 at 13:31
1
You've trieddb_table = '"abc"."table1"'
?
– Will Keeling
Nov 9 at 17:46
@WillKeeling that was the answer :). Thanks so much!
– Andrea
22 hours ago
did you tried
Model1.objects.using("database_oracle").all()
?– dorintufar
Nov 9 at 12:22
did you tried
Model1.objects.using("database_oracle").all()
?– dorintufar
Nov 9 at 12:22
1
1
also try to remove unnecessary double quotes from
db_table = '"abc.table1"'
in Meta
class– dorintufar
Nov 9 at 12:23
also try to remove unnecessary double quotes from
db_table = '"abc.table1"'
in Meta
class– dorintufar
Nov 9 at 12:23
@dorintufar thanks so far and yes, I tried it but the Traceback is still the same. And I tried the double quotes as Django suggests to use them for oracle dbs: docs.djangoproject.com/en/2.0/ref/models/options
– Andrea
Nov 9 at 13:31
@dorintufar thanks so far and yes, I tried it but the Traceback is still the same. And I tried the double quotes as Django suggests to use them for oracle dbs: docs.djangoproject.com/en/2.0/ref/models/options
– Andrea
Nov 9 at 13:31
1
1
You've tried
db_table = '"abc"."table1"'
?– Will Keeling
Nov 9 at 17:46
You've tried
db_table = '"abc"."table1"'
?– Will Keeling
Nov 9 at 17:46
@WillKeeling that was the answer :). Thanks so much!
– Andrea
22 hours ago
@WillKeeling that was the answer :). Thanks so much!
– Andrea
22 hours ago
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53225609%2fdjango-models-dont-work-with-oracle-database%23new-answer', 'question_page');
);
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
MMB H7lYaOTv5twXpO2zFH 2Y4oE9TpBF5pAOJtR2axCmDTh7LZ7ZOPCq4eCX
did you tried
Model1.objects.using("database_oracle").all()
?– dorintufar
Nov 9 at 12:22
1
also try to remove unnecessary double quotes from
db_table = '"abc.table1"'
inMeta
class– dorintufar
Nov 9 at 12:23
@dorintufar thanks so far and yes, I tried it but the Traceback is still the same. And I tried the double quotes as Django suggests to use them for oracle dbs: docs.djangoproject.com/en/2.0/ref/models/options
– Andrea
Nov 9 at 13:31
1
You've tried
db_table = '"abc"."table1"'
?– Will Keeling
Nov 9 at 17:46
@WillKeeling that was the answer :). Thanks so much!
– Andrea
22 hours ago