How to use lodash's _sortBy() to alphabetically sort this list with a custom requirement?
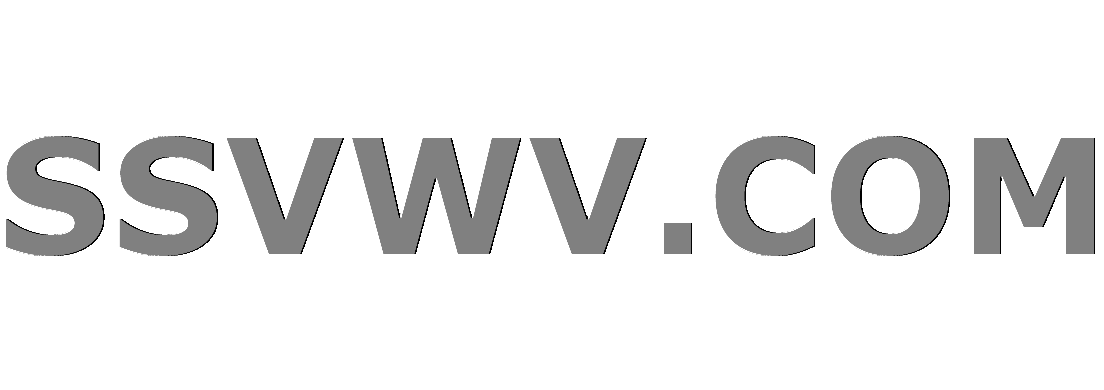
Multi tool use
up vote
0
down vote
favorite
I have the following array of objects:
const myList = [
id: 1, title: '[A] Animal Bite - F - Not Pregnant' ,
id: 2, title: '[P] Sinus Pain - M' ,
id: 3, title: '[A] Animal Bite - F - Pregnant' ,
id: 4, title: 'Check up male' ,
id: 5, title: '[A] Animal Bite - M' ,
id: 6, title: 'Duration' ,
id: 7, title: '[P] Skin Injury - F - Not Pregnant' ,
id: 8, title: '[P] Skin Injury - M' ,
id: 9, title: 'Emergency Screening'
]
After doing:
_.sortBy(myList, 'title');
I get:
Check up male
Duration
Emergency Screening
[A] Animal Bite - F - Not Pregnant
[A] Animal Bite - F - Pregnant
[A] Animal Bite - M
[P] Sinus Pain - M
[P] Skin Injury - F - Not Pregnant
[P] Skin Injury - M
It looks good except I want the items without [A] or [P] to be at the bottom instead of the top. So like this instead:
[A] Animal Bite - F - Not Pregnant
[A] Animal Bite - F - Pregnant
[A] Animal Bite - M
[P] Sinus Pain - M
[P] Skin Injury - F - Not Pregnant
[P] Skin Injury - M
Check up male
Duration
Emergency Screening
How to achieve this?
javascript underscore.js lodash
add a comment |
up vote
0
down vote
favorite
I have the following array of objects:
const myList = [
id: 1, title: '[A] Animal Bite - F - Not Pregnant' ,
id: 2, title: '[P] Sinus Pain - M' ,
id: 3, title: '[A] Animal Bite - F - Pregnant' ,
id: 4, title: 'Check up male' ,
id: 5, title: '[A] Animal Bite - M' ,
id: 6, title: 'Duration' ,
id: 7, title: '[P] Skin Injury - F - Not Pregnant' ,
id: 8, title: '[P] Skin Injury - M' ,
id: 9, title: 'Emergency Screening'
]
After doing:
_.sortBy(myList, 'title');
I get:
Check up male
Duration
Emergency Screening
[A] Animal Bite - F - Not Pregnant
[A] Animal Bite - F - Pregnant
[A] Animal Bite - M
[P] Sinus Pain - M
[P] Skin Injury - F - Not Pregnant
[P] Skin Injury - M
It looks good except I want the items without [A] or [P] to be at the bottom instead of the top. So like this instead:
[A] Animal Bite - F - Not Pregnant
[A] Animal Bite - F - Pregnant
[A] Animal Bite - M
[P] Sinus Pain - M
[P] Skin Injury - F - Not Pregnant
[P] Skin Injury - M
Check up male
Duration
Emergency Screening
How to achieve this?
javascript underscore.js lodash
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have the following array of objects:
const myList = [
id: 1, title: '[A] Animal Bite - F - Not Pregnant' ,
id: 2, title: '[P] Sinus Pain - M' ,
id: 3, title: '[A] Animal Bite - F - Pregnant' ,
id: 4, title: 'Check up male' ,
id: 5, title: '[A] Animal Bite - M' ,
id: 6, title: 'Duration' ,
id: 7, title: '[P] Skin Injury - F - Not Pregnant' ,
id: 8, title: '[P] Skin Injury - M' ,
id: 9, title: 'Emergency Screening'
]
After doing:
_.sortBy(myList, 'title');
I get:
Check up male
Duration
Emergency Screening
[A] Animal Bite - F - Not Pregnant
[A] Animal Bite - F - Pregnant
[A] Animal Bite - M
[P] Sinus Pain - M
[P] Skin Injury - F - Not Pregnant
[P] Skin Injury - M
It looks good except I want the items without [A] or [P] to be at the bottom instead of the top. So like this instead:
[A] Animal Bite - F - Not Pregnant
[A] Animal Bite - F - Pregnant
[A] Animal Bite - M
[P] Sinus Pain - M
[P] Skin Injury - F - Not Pregnant
[P] Skin Injury - M
Check up male
Duration
Emergency Screening
How to achieve this?
javascript underscore.js lodash
I have the following array of objects:
const myList = [
id: 1, title: '[A] Animal Bite - F - Not Pregnant' ,
id: 2, title: '[P] Sinus Pain - M' ,
id: 3, title: '[A] Animal Bite - F - Pregnant' ,
id: 4, title: 'Check up male' ,
id: 5, title: '[A] Animal Bite - M' ,
id: 6, title: 'Duration' ,
id: 7, title: '[P] Skin Injury - F - Not Pregnant' ,
id: 8, title: '[P] Skin Injury - M' ,
id: 9, title: 'Emergency Screening'
]
After doing:
_.sortBy(myList, 'title');
I get:
Check up male
Duration
Emergency Screening
[A] Animal Bite - F - Not Pregnant
[A] Animal Bite - F - Pregnant
[A] Animal Bite - M
[P] Sinus Pain - M
[P] Skin Injury - F - Not Pregnant
[P] Skin Injury - M
It looks good except I want the items without [A] or [P] to be at the bottom instead of the top. So like this instead:
[A] Animal Bite - F - Not Pregnant
[A] Animal Bite - F - Pregnant
[A] Animal Bite - M
[P] Sinus Pain - M
[P] Skin Injury - F - Not Pregnant
[P] Skin Injury - M
Check up male
Duration
Emergency Screening
How to achieve this?
javascript underscore.js lodash
javascript underscore.js lodash
asked Nov 9 at 21:36


TK123
10.4k36113156
10.4k36113156
add a comment |
add a comment |
5 Answers
5
active
oldest
votes
up vote
1
down vote
accepted
Use localeCompare
with numeric: false
option in the sort function:
const list = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ]
const r = list.sort((a,b) => a.title.localeCompare(b.title, 0, numeric: false))
console.log(r)
Another way you can also get the same result is via the caseFirst: lower'
option parameter as noted (and explained) by @xehpuk
asnwer:
const list = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ]
const r = list.sort((a,b) => a.title.localeCompare(b.title, 0, caseFirst: 'lower'))
console.log(r)
You also do not need lodash for this if ES6 is an option.
add a comment |
up vote
1
down vote
lodash's sortBy
may take list of comparators. So you can just declare "words don't start from square bracket go later" and inside the "group" sort by title
_.sortBy(myList, [
item => !item.title.startsWith("["),
'title'
]);
And with orderBy
you even can specify ordering in more readable(and flexible) way:
_.orderBy(myList, [
item => item.title.startsWith("["),
'title'
], ['desc', 'asc']);
[UPD] with startsWith
mentioned by @Ele it looks even better
add a comment |
up vote
1
down vote
If you really want to use lodash, you can compare the titles in lower-case:
_.sortBy(myList, item => item.title.toLowerCase());
This works because the code unit of the lower-case characters (97 - 122) is greater than the one of [
(91). This would also have the benefit of comparing the titles case-insensitively.
add a comment |
up vote
1
down vote
This is an alternative using the function Array.prototype.sort()
Assuming the there is max one [
in the string.
const myList = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ];
myList.sort((a, b) =>
if (a.title.startsWith("[") && b.title.startsWith("["))
return a.title.substring(1).localeCompare(b.title.substring(1));
return a.title.localeCompare(b.title);
);
console.log(myList);
.as-console-wrapper max-height: 100% !important; top: 0;
Your comparator doesn't sort the titles not starting with[
. It just moves them to the end.
– xehpuk
Nov 9 at 22:12
add a comment |
up vote
0
down vote
You could move the brackets parts to top by checking the strings, then takes the local compare result.
const
startsWithBrackets = s => /^[.+]/.test(s),
myList = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ];
myList.sort(( title: a , title: b ) =>
startsWithBrackets(b) - startsWithBrackets(a) || a.localeCompare(b));
console.log(myList);
.as-console-wrapper max-height: 100% !important; top: 0;
add a comment |
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
Use localeCompare
with numeric: false
option in the sort function:
const list = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ]
const r = list.sort((a,b) => a.title.localeCompare(b.title, 0, numeric: false))
console.log(r)
Another way you can also get the same result is via the caseFirst: lower'
option parameter as noted (and explained) by @xehpuk
asnwer:
const list = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ]
const r = list.sort((a,b) => a.title.localeCompare(b.title, 0, caseFirst: 'lower'))
console.log(r)
You also do not need lodash for this if ES6 is an option.
add a comment |
up vote
1
down vote
accepted
Use localeCompare
with numeric: false
option in the sort function:
const list = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ]
const r = list.sort((a,b) => a.title.localeCompare(b.title, 0, numeric: false))
console.log(r)
Another way you can also get the same result is via the caseFirst: lower'
option parameter as noted (and explained) by @xehpuk
asnwer:
const list = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ]
const r = list.sort((a,b) => a.title.localeCompare(b.title, 0, caseFirst: 'lower'))
console.log(r)
You also do not need lodash for this if ES6 is an option.
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
Use localeCompare
with numeric: false
option in the sort function:
const list = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ]
const r = list.sort((a,b) => a.title.localeCompare(b.title, 0, numeric: false))
console.log(r)
Another way you can also get the same result is via the caseFirst: lower'
option parameter as noted (and explained) by @xehpuk
asnwer:
const list = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ]
const r = list.sort((a,b) => a.title.localeCompare(b.title, 0, caseFirst: 'lower'))
console.log(r)
You also do not need lodash for this if ES6 is an option.
Use localeCompare
with numeric: false
option in the sort function:
const list = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ]
const r = list.sort((a,b) => a.title.localeCompare(b.title, 0, numeric: false))
console.log(r)
Another way you can also get the same result is via the caseFirst: lower'
option parameter as noted (and explained) by @xehpuk
asnwer:
const list = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ]
const r = list.sort((a,b) => a.title.localeCompare(b.title, 0, caseFirst: 'lower'))
console.log(r)
You also do not need lodash for this if ES6 is an option.
const list = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ]
const r = list.sort((a,b) => a.title.localeCompare(b.title, 0, numeric: false))
console.log(r)
const list = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ]
const r = list.sort((a,b) => a.title.localeCompare(b.title, 0, numeric: false))
console.log(r)
const list = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ]
const r = list.sort((a,b) => a.title.localeCompare(b.title, 0, caseFirst: 'lower'))
console.log(r)
const list = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ]
const r = list.sort((a,b) => a.title.localeCompare(b.title, 0, caseFirst: 'lower'))
console.log(r)
edited Nov 15 at 1:24
answered Nov 9 at 21:56


Akrion
7,19111222
7,19111222
add a comment |
add a comment |
up vote
1
down vote
lodash's sortBy
may take list of comparators. So you can just declare "words don't start from square bracket go later" and inside the "group" sort by title
_.sortBy(myList, [
item => !item.title.startsWith("["),
'title'
]);
And with orderBy
you even can specify ordering in more readable(and flexible) way:
_.orderBy(myList, [
item => item.title.startsWith("["),
'title'
], ['desc', 'asc']);
[UPD] with startsWith
mentioned by @Ele it looks even better
add a comment |
up vote
1
down vote
lodash's sortBy
may take list of comparators. So you can just declare "words don't start from square bracket go later" and inside the "group" sort by title
_.sortBy(myList, [
item => !item.title.startsWith("["),
'title'
]);
And with orderBy
you even can specify ordering in more readable(and flexible) way:
_.orderBy(myList, [
item => item.title.startsWith("["),
'title'
], ['desc', 'asc']);
[UPD] with startsWith
mentioned by @Ele it looks even better
add a comment |
up vote
1
down vote
up vote
1
down vote
lodash's sortBy
may take list of comparators. So you can just declare "words don't start from square bracket go later" and inside the "group" sort by title
_.sortBy(myList, [
item => !item.title.startsWith("["),
'title'
]);
And with orderBy
you even can specify ordering in more readable(and flexible) way:
_.orderBy(myList, [
item => item.title.startsWith("["),
'title'
], ['desc', 'asc']);
[UPD] with startsWith
mentioned by @Ele it looks even better
lodash's sortBy
may take list of comparators. So you can just declare "words don't start from square bracket go later" and inside the "group" sort by title
_.sortBy(myList, [
item => !item.title.startsWith("["),
'title'
]);
And with orderBy
you even can specify ordering in more readable(and flexible) way:
_.orderBy(myList, [
item => item.title.startsWith("["),
'title'
], ['desc', 'asc']);
[UPD] with startsWith
mentioned by @Ele it looks even better
edited Nov 9 at 22:05
answered Nov 9 at 21:59
skyboyer
2,93811028
2,93811028
add a comment |
add a comment |
up vote
1
down vote
If you really want to use lodash, you can compare the titles in lower-case:
_.sortBy(myList, item => item.title.toLowerCase());
This works because the code unit of the lower-case characters (97 - 122) is greater than the one of [
(91). This would also have the benefit of comparing the titles case-insensitively.
add a comment |
up vote
1
down vote
If you really want to use lodash, you can compare the titles in lower-case:
_.sortBy(myList, item => item.title.toLowerCase());
This works because the code unit of the lower-case characters (97 - 122) is greater than the one of [
(91). This would also have the benefit of comparing the titles case-insensitively.
add a comment |
up vote
1
down vote
up vote
1
down vote
If you really want to use lodash, you can compare the titles in lower-case:
_.sortBy(myList, item => item.title.toLowerCase());
This works because the code unit of the lower-case characters (97 - 122) is greater than the one of [
(91). This would also have the benefit of comparing the titles case-insensitively.
If you really want to use lodash, you can compare the titles in lower-case:
_.sortBy(myList, item => item.title.toLowerCase());
This works because the code unit of the lower-case characters (97 - 122) is greater than the one of [
(91). This would also have the benefit of comparing the titles case-insensitively.
answered Nov 9 at 22:07


xehpuk
4,1282335
4,1282335
add a comment |
add a comment |
up vote
1
down vote
This is an alternative using the function Array.prototype.sort()
Assuming the there is max one [
in the string.
const myList = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ];
myList.sort((a, b) =>
if (a.title.startsWith("[") && b.title.startsWith("["))
return a.title.substring(1).localeCompare(b.title.substring(1));
return a.title.localeCompare(b.title);
);
console.log(myList);
.as-console-wrapper max-height: 100% !important; top: 0;
Your comparator doesn't sort the titles not starting with[
. It just moves them to the end.
– xehpuk
Nov 9 at 22:12
add a comment |
up vote
1
down vote
This is an alternative using the function Array.prototype.sort()
Assuming the there is max one [
in the string.
const myList = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ];
myList.sort((a, b) =>
if (a.title.startsWith("[") && b.title.startsWith("["))
return a.title.substring(1).localeCompare(b.title.substring(1));
return a.title.localeCompare(b.title);
);
console.log(myList);
.as-console-wrapper max-height: 100% !important; top: 0;
Your comparator doesn't sort the titles not starting with[
. It just moves them to the end.
– xehpuk
Nov 9 at 22:12
add a comment |
up vote
1
down vote
up vote
1
down vote
This is an alternative using the function Array.prototype.sort()
Assuming the there is max one [
in the string.
const myList = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ];
myList.sort((a, b) =>
if (a.title.startsWith("[") && b.title.startsWith("["))
return a.title.substring(1).localeCompare(b.title.substring(1));
return a.title.localeCompare(b.title);
);
console.log(myList);
.as-console-wrapper max-height: 100% !important; top: 0;
This is an alternative using the function Array.prototype.sort()
Assuming the there is max one [
in the string.
const myList = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ];
myList.sort((a, b) =>
if (a.title.startsWith("[") && b.title.startsWith("["))
return a.title.substring(1).localeCompare(b.title.substring(1));
return a.title.localeCompare(b.title);
);
console.log(myList);
.as-console-wrapper max-height: 100% !important; top: 0;
const myList = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ];
myList.sort((a, b) =>
if (a.title.startsWith("[") && b.title.startsWith("["))
return a.title.substring(1).localeCompare(b.title.substring(1));
return a.title.localeCompare(b.title);
);
console.log(myList);
.as-console-wrapper max-height: 100% !important; top: 0;
const myList = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ];
myList.sort((a, b) =>
if (a.title.startsWith("[") && b.title.startsWith("["))
return a.title.substring(1).localeCompare(b.title.substring(1));
return a.title.localeCompare(b.title);
);
console.log(myList);
.as-console-wrapper max-height: 100% !important; top: 0;
edited Nov 9 at 22:20
answered Nov 9 at 21:42


Ele
22.3k42044
22.3k42044
Your comparator doesn't sort the titles not starting with[
. It just moves them to the end.
– xehpuk
Nov 9 at 22:12
add a comment |
Your comparator doesn't sort the titles not starting with[
. It just moves them to the end.
– xehpuk
Nov 9 at 22:12
Your comparator doesn't sort the titles not starting with
[
. It just moves them to the end.– xehpuk
Nov 9 at 22:12
Your comparator doesn't sort the titles not starting with
[
. It just moves them to the end.– xehpuk
Nov 9 at 22:12
add a comment |
up vote
0
down vote
You could move the brackets parts to top by checking the strings, then takes the local compare result.
const
startsWithBrackets = s => /^[.+]/.test(s),
myList = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ];
myList.sort(( title: a , title: b ) =>
startsWithBrackets(b) - startsWithBrackets(a) || a.localeCompare(b));
console.log(myList);
.as-console-wrapper max-height: 100% !important; top: 0;
add a comment |
up vote
0
down vote
You could move the brackets parts to top by checking the strings, then takes the local compare result.
const
startsWithBrackets = s => /^[.+]/.test(s),
myList = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ];
myList.sort(( title: a , title: b ) =>
startsWithBrackets(b) - startsWithBrackets(a) || a.localeCompare(b));
console.log(myList);
.as-console-wrapper max-height: 100% !important; top: 0;
add a comment |
up vote
0
down vote
up vote
0
down vote
You could move the brackets parts to top by checking the strings, then takes the local compare result.
const
startsWithBrackets = s => /^[.+]/.test(s),
myList = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ];
myList.sort(( title: a , title: b ) =>
startsWithBrackets(b) - startsWithBrackets(a) || a.localeCompare(b));
console.log(myList);
.as-console-wrapper max-height: 100% !important; top: 0;
You could move the brackets parts to top by checking the strings, then takes the local compare result.
const
startsWithBrackets = s => /^[.+]/.test(s),
myList = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ];
myList.sort(( title: a , title: b ) =>
startsWithBrackets(b) - startsWithBrackets(a) || a.localeCompare(b));
console.log(myList);
.as-console-wrapper max-height: 100% !important; top: 0;
const
startsWithBrackets = s => /^[.+]/.test(s),
myList = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ];
myList.sort(( title: a , title: b ) =>
startsWithBrackets(b) - startsWithBrackets(a) || a.localeCompare(b));
console.log(myList);
.as-console-wrapper max-height: 100% !important; top: 0;
const
startsWithBrackets = s => /^[.+]/.test(s),
myList = [ id: 1, title: '[A] Animal Bite - F - Not Pregnant' , id: 2, title: '[P] Sinus Pain - M' , id: 3, title: '[A] Animal Bite - F - Pregnant' , id: 4, title: 'Check up male' , id: 5, title: '[A] Animal Bite - M' , id: 6, title: 'Duration' , id: 7, title: '[P] Skin Injury - F - Not Pregnant' , id: 8, title: '[P] Skin Injury - M' , id: 9, title: 'Emergency Screening' ];
myList.sort(( title: a , title: b ) =>
startsWithBrackets(b) - startsWithBrackets(a) || a.localeCompare(b));
console.log(myList);
.as-console-wrapper max-height: 100% !important; top: 0;
answered Nov 9 at 22:15


Nina Scholz
170k1382146
170k1382146
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53233552%2fhow-to-use-lodashs-sortby-to-alphabetically-sort-this-list-with-a-custom-req%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vhoETAKvBdjeZgyH