average is outputting as inf
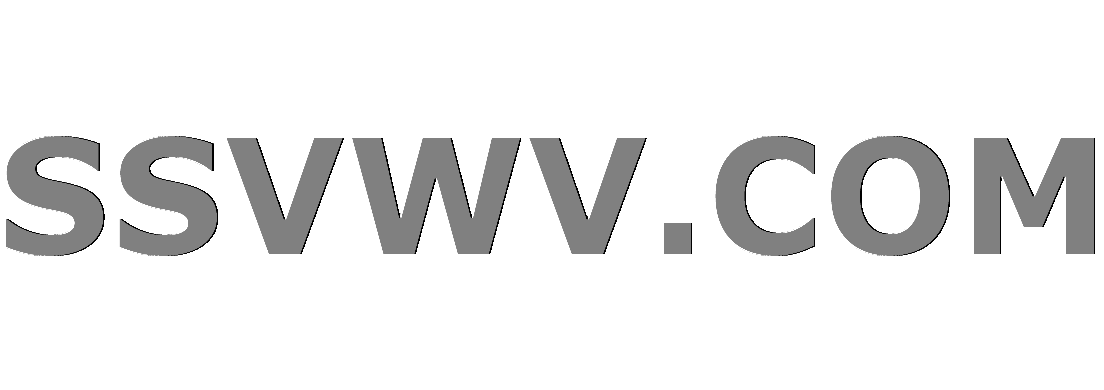
Multi tool use
I am trying to calculate the average for a class from scores given using a data file that was given.
The formula I'm using is grade_Average = sum / i;
The data file that was given is :
Joe Johnson 89
Susie Caldwell 67
Matt Baker 100
Alex Anderson 87
Perry Dixon 55
The output I am getting is
Johnson,Joe B
Caldwell,Susie D
Baker,Matt A
Anderson,Alex B
Dixon,Perry F
Class average inf
I am not sure if I have the formula wrong or if the formula is in the wrong place.
#include <iostream>
#include <string>
#include <fstream>
#include <iomanip>
using namespace std;
int main()
// Variable declarations:
string fName[10];
string lName[10];
float grade_Average;
string file;
string name;
int scores[10];
float sum = 0;
char grade;
int i = 0;
ifstream din;
// Function body:
cout << "Enter the name of the file. " << endl;
cin >> file;
din.open(file.c_str());
if (!din)
cout << " Cannot open the input file. Please try again." << endl;
return 0;
cout << setw(10) << setfill(' ') << "Name" <<setw(20)<<setfill(' ')<< "Grade" << endl;
while (!din.eof())
din >> fName[i];
din >> lName[i];
din >> scores[i];
sum = sum + scores[i];
switch (static_cast<int> (scores[i]/10))
case 0:
case 1:
case 2:
case 3:
case 4:
case 5:
grade = 'F';
break;
case 6:
grade = 'D';
break;
case 7:
grade = 'C';
break;
case 8:
grade = 'B';
break;
case 9:
grade = 'A';
break;
case 10:
grade = 'A';
break;
default:
cout << "Invalid score." << endl;
i++;
name = lName[i] + ',' + fName[i];
cout << setw(10) << setfill(' ') << name << setw(20) << setfill(' ')<<(" ") << grade << endl;
grade_Average = sum / i;
cout << "Class average " << grade_Average << endl;
din.close();
return 0;
c++ arrays while-loop
add a comment |
I am trying to calculate the average for a class from scores given using a data file that was given.
The formula I'm using is grade_Average = sum / i;
The data file that was given is :
Joe Johnson 89
Susie Caldwell 67
Matt Baker 100
Alex Anderson 87
Perry Dixon 55
The output I am getting is
Johnson,Joe B
Caldwell,Susie D
Baker,Matt A
Anderson,Alex B
Dixon,Perry F
Class average inf
I am not sure if I have the formula wrong or if the formula is in the wrong place.
#include <iostream>
#include <string>
#include <fstream>
#include <iomanip>
using namespace std;
int main()
// Variable declarations:
string fName[10];
string lName[10];
float grade_Average;
string file;
string name;
int scores[10];
float sum = 0;
char grade;
int i = 0;
ifstream din;
// Function body:
cout << "Enter the name of the file. " << endl;
cin >> file;
din.open(file.c_str());
if (!din)
cout << " Cannot open the input file. Please try again." << endl;
return 0;
cout << setw(10) << setfill(' ') << "Name" <<setw(20)<<setfill(' ')<< "Grade" << endl;
while (!din.eof())
din >> fName[i];
din >> lName[i];
din >> scores[i];
sum = sum + scores[i];
switch (static_cast<int> (scores[i]/10))
case 0:
case 1:
case 2:
case 3:
case 4:
case 5:
grade = 'F';
break;
case 6:
grade = 'D';
break;
case 7:
grade = 'C';
break;
case 8:
grade = 'B';
break;
case 9:
grade = 'A';
break;
case 10:
grade = 'A';
break;
default:
cout << "Invalid score." << endl;
i++;
name = lName[i] + ',' + fName[i];
cout << setw(10) << setfill(' ') << name << setw(20) << setfill(' ')<<(" ") << grade << endl;
grade_Average = sum / i;
cout << "Class average " << grade_Average << endl;
din.close();
return 0;
c++ arrays while-loop
stackoverflow.com/questions/5605125/…, and use a debugger.
– user657267
Nov 11 at 6:16
Definitely use a debugger if you do c++ :)
– L.C.
Nov 11 at 6:19
i
is only incremented if an invalid score is entered. Which means it will have a value of zero if all scores are valid. Then it is used in the statementgrade_average = sum/i
wheresum
andgrade_average
are of typefloat
. With IEEE floating point format (not guaranteed, but probably what you have) division by zero results in an infinity.
– Peter
Nov 11 at 9:03
add a comment |
I am trying to calculate the average for a class from scores given using a data file that was given.
The formula I'm using is grade_Average = sum / i;
The data file that was given is :
Joe Johnson 89
Susie Caldwell 67
Matt Baker 100
Alex Anderson 87
Perry Dixon 55
The output I am getting is
Johnson,Joe B
Caldwell,Susie D
Baker,Matt A
Anderson,Alex B
Dixon,Perry F
Class average inf
I am not sure if I have the formula wrong or if the formula is in the wrong place.
#include <iostream>
#include <string>
#include <fstream>
#include <iomanip>
using namespace std;
int main()
// Variable declarations:
string fName[10];
string lName[10];
float grade_Average;
string file;
string name;
int scores[10];
float sum = 0;
char grade;
int i = 0;
ifstream din;
// Function body:
cout << "Enter the name of the file. " << endl;
cin >> file;
din.open(file.c_str());
if (!din)
cout << " Cannot open the input file. Please try again." << endl;
return 0;
cout << setw(10) << setfill(' ') << "Name" <<setw(20)<<setfill(' ')<< "Grade" << endl;
while (!din.eof())
din >> fName[i];
din >> lName[i];
din >> scores[i];
sum = sum + scores[i];
switch (static_cast<int> (scores[i]/10))
case 0:
case 1:
case 2:
case 3:
case 4:
case 5:
grade = 'F';
break;
case 6:
grade = 'D';
break;
case 7:
grade = 'C';
break;
case 8:
grade = 'B';
break;
case 9:
grade = 'A';
break;
case 10:
grade = 'A';
break;
default:
cout << "Invalid score." << endl;
i++;
name = lName[i] + ',' + fName[i];
cout << setw(10) << setfill(' ') << name << setw(20) << setfill(' ')<<(" ") << grade << endl;
grade_Average = sum / i;
cout << "Class average " << grade_Average << endl;
din.close();
return 0;
c++ arrays while-loop
I am trying to calculate the average for a class from scores given using a data file that was given.
The formula I'm using is grade_Average = sum / i;
The data file that was given is :
Joe Johnson 89
Susie Caldwell 67
Matt Baker 100
Alex Anderson 87
Perry Dixon 55
The output I am getting is
Johnson,Joe B
Caldwell,Susie D
Baker,Matt A
Anderson,Alex B
Dixon,Perry F
Class average inf
I am not sure if I have the formula wrong or if the formula is in the wrong place.
#include <iostream>
#include <string>
#include <fstream>
#include <iomanip>
using namespace std;
int main()
// Variable declarations:
string fName[10];
string lName[10];
float grade_Average;
string file;
string name;
int scores[10];
float sum = 0;
char grade;
int i = 0;
ifstream din;
// Function body:
cout << "Enter the name of the file. " << endl;
cin >> file;
din.open(file.c_str());
if (!din)
cout << " Cannot open the input file. Please try again." << endl;
return 0;
cout << setw(10) << setfill(' ') << "Name" <<setw(20)<<setfill(' ')<< "Grade" << endl;
while (!din.eof())
din >> fName[i];
din >> lName[i];
din >> scores[i];
sum = sum + scores[i];
switch (static_cast<int> (scores[i]/10))
case 0:
case 1:
case 2:
case 3:
case 4:
case 5:
grade = 'F';
break;
case 6:
grade = 'D';
break;
case 7:
grade = 'C';
break;
case 8:
grade = 'B';
break;
case 9:
grade = 'A';
break;
case 10:
grade = 'A';
break;
default:
cout << "Invalid score." << endl;
i++;
name = lName[i] + ',' + fName[i];
cout << setw(10) << setfill(' ') << name << setw(20) << setfill(' ')<<(" ") << grade << endl;
grade_Average = sum / i;
cout << "Class average " << grade_Average << endl;
din.close();
return 0;
c++ arrays while-loop
c++ arrays while-loop
edited Nov 11 at 7:11
bolov
30.4k668128
30.4k668128
asked Nov 11 at 6:11
Wessley Ray Cone
12
12
stackoverflow.com/questions/5605125/…, and use a debugger.
– user657267
Nov 11 at 6:16
Definitely use a debugger if you do c++ :)
– L.C.
Nov 11 at 6:19
i
is only incremented if an invalid score is entered. Which means it will have a value of zero if all scores are valid. Then it is used in the statementgrade_average = sum/i
wheresum
andgrade_average
are of typefloat
. With IEEE floating point format (not guaranteed, but probably what you have) division by zero results in an infinity.
– Peter
Nov 11 at 9:03
add a comment |
stackoverflow.com/questions/5605125/…, and use a debugger.
– user657267
Nov 11 at 6:16
Definitely use a debugger if you do c++ :)
– L.C.
Nov 11 at 6:19
i
is only incremented if an invalid score is entered. Which means it will have a value of zero if all scores are valid. Then it is used in the statementgrade_average = sum/i
wheresum
andgrade_average
are of typefloat
. With IEEE floating point format (not guaranteed, but probably what you have) division by zero results in an infinity.
– Peter
Nov 11 at 9:03
stackoverflow.com/questions/5605125/…, and use a debugger.
– user657267
Nov 11 at 6:16
stackoverflow.com/questions/5605125/…, and use a debugger.
– user657267
Nov 11 at 6:16
Definitely use a debugger if you do c++ :)
– L.C.
Nov 11 at 6:19
Definitely use a debugger if you do c++ :)
– L.C.
Nov 11 at 6:19
i
is only incremented if an invalid score is entered. Which means it will have a value of zero if all scores are valid. Then it is used in the statementgrade_average = sum/i
where sum
andgrade_average
are of type float
. With IEEE floating point format (not guaranteed, but probably what you have) division by zero results in an infinity.– Peter
Nov 11 at 9:03
i
is only incremented if an invalid score is entered. Which means it will have a value of zero if all scores are valid. Then it is used in the statementgrade_average = sum/i
where sum
andgrade_average
are of type float
. With IEEE floating point format (not guaranteed, but probably what you have) division by zero results in an infinity.– Peter
Nov 11 at 9:03
add a comment |
2 Answers
2
active
oldest
votes
Your i++ is inside the default block. The i variable is most probably 0. Either you put i++ outside of the switch block or you put it before every break statement.
add a comment |
i++
is inside the switch
block and in the default
case. It will never be executed for the given input. Therefore throughout the run i
will just be 0
. Dividing by 0
gives you inf
.
Your program will also fail if more than 10
entries are given (and the first issue is corrected). You should use std::vector<std::string>
, std::vector<int>
and push_back
instead of the raw arrays of std::string
and int
, if these arrays are needed at all. (For just calculating the average, the individual entries don't really need to be saved.)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53246322%2faverage-is-outputting-as-inf%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your i++ is inside the default block. The i variable is most probably 0. Either you put i++ outside of the switch block or you put it before every break statement.
add a comment |
Your i++ is inside the default block. The i variable is most probably 0. Either you put i++ outside of the switch block or you put it before every break statement.
add a comment |
Your i++ is inside the default block. The i variable is most probably 0. Either you put i++ outside of the switch block or you put it before every break statement.
Your i++ is inside the default block. The i variable is most probably 0. Either you put i++ outside of the switch block or you put it before every break statement.
answered Nov 11 at 6:18
L.C.
19012
19012
add a comment |
add a comment |
i++
is inside the switch
block and in the default
case. It will never be executed for the given input. Therefore throughout the run i
will just be 0
. Dividing by 0
gives you inf
.
Your program will also fail if more than 10
entries are given (and the first issue is corrected). You should use std::vector<std::string>
, std::vector<int>
and push_back
instead of the raw arrays of std::string
and int
, if these arrays are needed at all. (For just calculating the average, the individual entries don't really need to be saved.)
add a comment |
i++
is inside the switch
block and in the default
case. It will never be executed for the given input. Therefore throughout the run i
will just be 0
. Dividing by 0
gives you inf
.
Your program will also fail if more than 10
entries are given (and the first issue is corrected). You should use std::vector<std::string>
, std::vector<int>
and push_back
instead of the raw arrays of std::string
and int
, if these arrays are needed at all. (For just calculating the average, the individual entries don't really need to be saved.)
add a comment |
i++
is inside the switch
block and in the default
case. It will never be executed for the given input. Therefore throughout the run i
will just be 0
. Dividing by 0
gives you inf
.
Your program will also fail if more than 10
entries are given (and the first issue is corrected). You should use std::vector<std::string>
, std::vector<int>
and push_back
instead of the raw arrays of std::string
and int
, if these arrays are needed at all. (For just calculating the average, the individual entries don't really need to be saved.)
i++
is inside the switch
block and in the default
case. It will never be executed for the given input. Therefore throughout the run i
will just be 0
. Dividing by 0
gives you inf
.
Your program will also fail if more than 10
entries are given (and the first issue is corrected). You should use std::vector<std::string>
, std::vector<int>
and push_back
instead of the raw arrays of std::string
and int
, if these arrays are needed at all. (For just calculating the average, the individual entries don't really need to be saved.)
edited Nov 11 at 6:23
answered Nov 11 at 6:17
user10605163
2,238421
2,238421
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53246322%2faverage-is-outputting-as-inf%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UtZCLNmDluj6U,8OJJhnB,2VRpJQ0c8Felo ysCNbHomGFxlWO 26r,m
stackoverflow.com/questions/5605125/…, and use a debugger.
– user657267
Nov 11 at 6:16
Definitely use a debugger if you do c++ :)
– L.C.
Nov 11 at 6:19
i
is only incremented if an invalid score is entered. Which means it will have a value of zero if all scores are valid. Then it is used in the statementgrade_average = sum/i
wheresum
andgrade_average
are of typefloat
. With IEEE floating point format (not guaranteed, but probably what you have) division by zero results in an infinity.– Peter
Nov 11 at 9:03