Use 'any' to tell you true/false, if a list contains some value(s) divisible by 42
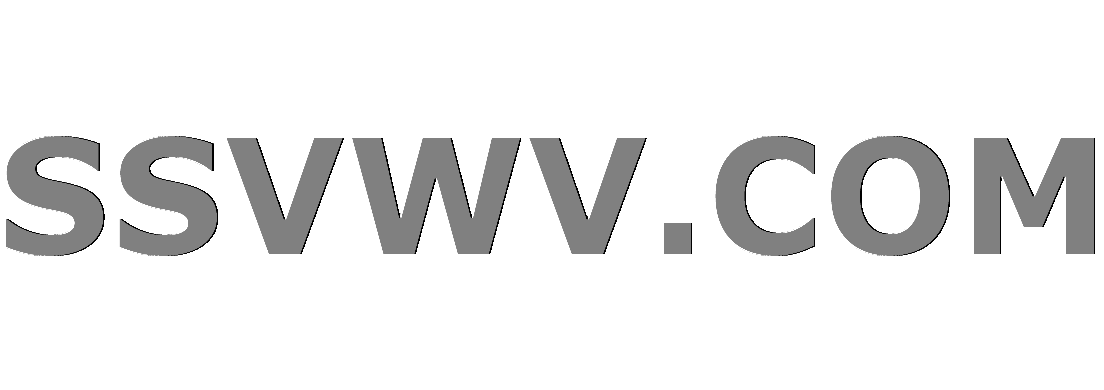
Multi tool use
Having fun working through a Haskell tutorial...
One problem posed is as you see in the subject line... this is one I'd really like to figure out, but I'm at a loss... I've used any
like so:
ghci >any (==55) [15,25,35,45,55,65,75,85,95]
True
ghci >any (==55) [15,25,35,45,54,65,75,85,95]
False
ghci >all even [2,4,6,8]
True
ghci >all even [1,3,5,7,9]
False
and it seems like checking if a list element is divisible by 42 or not, should be fairly easy...
I mean, you would check if any of the numbers in the list are n `mod` 42 == 0
, right?
But how do you state that in the expression? Or do you need to write a 'helper' function?
haskell
add a comment |
Having fun working through a Haskell tutorial...
One problem posed is as you see in the subject line... this is one I'd really like to figure out, but I'm at a loss... I've used any
like so:
ghci >any (==55) [15,25,35,45,55,65,75,85,95]
True
ghci >any (==55) [15,25,35,45,54,65,75,85,95]
False
ghci >all even [2,4,6,8]
True
ghci >all even [1,3,5,7,9]
False
and it seems like checking if a list element is divisible by 42 or not, should be fairly easy...
I mean, you would check if any of the numbers in the list are n `mod` 42 == 0
, right?
But how do you state that in the expression? Or do you need to write a 'helper' function?
haskell
1
Without a helper definition, the most straightforward way would be with an anonymous function (a "lambda").
– duplode
Nov 13 '18 at 3:41
Holy cow, thank you everyone... i had actually thought of the lambda way later, but couldn't figure out putting together the function for some reason... but once i see it, I'm like, "that makes total sense".
– Stormy
Nov 14 '18 at 6:14
add a comment |
Having fun working through a Haskell tutorial...
One problem posed is as you see in the subject line... this is one I'd really like to figure out, but I'm at a loss... I've used any
like so:
ghci >any (==55) [15,25,35,45,55,65,75,85,95]
True
ghci >any (==55) [15,25,35,45,54,65,75,85,95]
False
ghci >all even [2,4,6,8]
True
ghci >all even [1,3,5,7,9]
False
and it seems like checking if a list element is divisible by 42 or not, should be fairly easy...
I mean, you would check if any of the numbers in the list are n `mod` 42 == 0
, right?
But how do you state that in the expression? Or do you need to write a 'helper' function?
haskell
Having fun working through a Haskell tutorial...
One problem posed is as you see in the subject line... this is one I'd really like to figure out, but I'm at a loss... I've used any
like so:
ghci >any (==55) [15,25,35,45,55,65,75,85,95]
True
ghci >any (==55) [15,25,35,45,54,65,75,85,95]
False
ghci >all even [2,4,6,8]
True
ghci >all even [1,3,5,7,9]
False
and it seems like checking if a list element is divisible by 42 or not, should be fairly easy...
I mean, you would check if any of the numbers in the list are n `mod` 42 == 0
, right?
But how do you state that in the expression? Or do you need to write a 'helper' function?
haskell
haskell
edited Nov 13 '18 at 3:39


duplode
22.9k44885
22.9k44885
asked Nov 13 '18 at 3:35
StormyStormy
673
673
1
Without a helper definition, the most straightforward way would be with an anonymous function (a "lambda").
– duplode
Nov 13 '18 at 3:41
Holy cow, thank you everyone... i had actually thought of the lambda way later, but couldn't figure out putting together the function for some reason... but once i see it, I'm like, "that makes total sense".
– Stormy
Nov 14 '18 at 6:14
add a comment |
1
Without a helper definition, the most straightforward way would be with an anonymous function (a "lambda").
– duplode
Nov 13 '18 at 3:41
Holy cow, thank you everyone... i had actually thought of the lambda way later, but couldn't figure out putting together the function for some reason... but once i see it, I'm like, "that makes total sense".
– Stormy
Nov 14 '18 at 6:14
1
1
Without a helper definition, the most straightforward way would be with an anonymous function (a "lambda").
– duplode
Nov 13 '18 at 3:41
Without a helper definition, the most straightforward way would be with an anonymous function (a "lambda").
– duplode
Nov 13 '18 at 3:41
Holy cow, thank you everyone... i had actually thought of the lambda way later, but couldn't figure out putting together the function for some reason... but once i see it, I'm like, "that makes total sense".
– Stormy
Nov 14 '18 at 6:14
Holy cow, thank you everyone... i had actually thought of the lambda way later, but couldn't figure out putting together the function for some reason... but once i see it, I'm like, "that makes total sense".
– Stormy
Nov 14 '18 at 6:14
add a comment |
2 Answers
2
active
oldest
votes
Composing (0==)
and (`mod 42`)
:
f :: [Integer] -> Bool
f = any ((0==).(`mod` 42))
futher reducing parenthesis noise:
f :: [Integer] -> Bool
f = any $ (0==).(`mod` 42)
Helper function:
f :: [Integer] -> Bool
f = any div42
where
div42 n = n `mod` 42 == 0
Stylistically, for this function, either way seems fine. However, say you wanted to check if any values are divisible by 42 OR 52, then utilizing composition may become more obfuscated/complex/futile. Whereas using a helper function keeps things readable: div42Or52 n = n `mod` 42 == 0 || n `mod` 52 == 0
. Note, I've used a where
clause above, but a let in
expression or a lambda are possible alternative ways to structure helper functions.
Ultimately, its up to the developer to balance concise code with understandable code.
2
For point-free code, it often helps readability to factor things into many small, generic, reusable definitions. For example, in this case I might writedivisibleBy d = (== 0) . (`mod` d)
(“to be divisible by d means to be equal to zero modulo d”) and then useany (divisibleBy 42)
. Nothing wrong with using a lambda or auxiliary definition in awhere
clause, though.
– Jon Purdy
Nov 13 '18 at 8:17
1
@JonPurdy, I completely agree. The only challenge is figuring out the order of the arguments.isDivisibleBy x y
sends a different message thanx `isDivisibleBy` y
.
– dfeuer
Nov 13 '18 at 15:35
1
@dfeuer: Yeah, same problem with other names really;divides
with the same definition but used asany (42 `divides`)
makes just as much sense, butdivides 42
is the wrong way around. This is why I prefer functions not be named such that they’re intended to be used infix, because it’s in conflict with using them in prefix; if you do want infix, an operator is preferable. (Besides, I have a personal objection to grave accents being used as quotation characters in a programming language, haha)
– Jon Purdy
Nov 13 '18 at 20:27
add a comment |
You can define function inplace:
any (n -> n `mod` 42 == 0) [1, 2, 42]
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53273415%2fuse-any-to-tell-you-true-false-if-a-list-contains-some-values-divisible-by%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Composing (0==)
and (`mod 42`)
:
f :: [Integer] -> Bool
f = any ((0==).(`mod` 42))
futher reducing parenthesis noise:
f :: [Integer] -> Bool
f = any $ (0==).(`mod` 42)
Helper function:
f :: [Integer] -> Bool
f = any div42
where
div42 n = n `mod` 42 == 0
Stylistically, for this function, either way seems fine. However, say you wanted to check if any values are divisible by 42 OR 52, then utilizing composition may become more obfuscated/complex/futile. Whereas using a helper function keeps things readable: div42Or52 n = n `mod` 42 == 0 || n `mod` 52 == 0
. Note, I've used a where
clause above, but a let in
expression or a lambda are possible alternative ways to structure helper functions.
Ultimately, its up to the developer to balance concise code with understandable code.
2
For point-free code, it often helps readability to factor things into many small, generic, reusable definitions. For example, in this case I might writedivisibleBy d = (== 0) . (`mod` d)
(“to be divisible by d means to be equal to zero modulo d”) and then useany (divisibleBy 42)
. Nothing wrong with using a lambda or auxiliary definition in awhere
clause, though.
– Jon Purdy
Nov 13 '18 at 8:17
1
@JonPurdy, I completely agree. The only challenge is figuring out the order of the arguments.isDivisibleBy x y
sends a different message thanx `isDivisibleBy` y
.
– dfeuer
Nov 13 '18 at 15:35
1
@dfeuer: Yeah, same problem with other names really;divides
with the same definition but used asany (42 `divides`)
makes just as much sense, butdivides 42
is the wrong way around. This is why I prefer functions not be named such that they’re intended to be used infix, because it’s in conflict with using them in prefix; if you do want infix, an operator is preferable. (Besides, I have a personal objection to grave accents being used as quotation characters in a programming language, haha)
– Jon Purdy
Nov 13 '18 at 20:27
add a comment |
Composing (0==)
and (`mod 42`)
:
f :: [Integer] -> Bool
f = any ((0==).(`mod` 42))
futher reducing parenthesis noise:
f :: [Integer] -> Bool
f = any $ (0==).(`mod` 42)
Helper function:
f :: [Integer] -> Bool
f = any div42
where
div42 n = n `mod` 42 == 0
Stylistically, for this function, either way seems fine. However, say you wanted to check if any values are divisible by 42 OR 52, then utilizing composition may become more obfuscated/complex/futile. Whereas using a helper function keeps things readable: div42Or52 n = n `mod` 42 == 0 || n `mod` 52 == 0
. Note, I've used a where
clause above, but a let in
expression or a lambda are possible alternative ways to structure helper functions.
Ultimately, its up to the developer to balance concise code with understandable code.
2
For point-free code, it often helps readability to factor things into many small, generic, reusable definitions. For example, in this case I might writedivisibleBy d = (== 0) . (`mod` d)
(“to be divisible by d means to be equal to zero modulo d”) and then useany (divisibleBy 42)
. Nothing wrong with using a lambda or auxiliary definition in awhere
clause, though.
– Jon Purdy
Nov 13 '18 at 8:17
1
@JonPurdy, I completely agree. The only challenge is figuring out the order of the arguments.isDivisibleBy x y
sends a different message thanx `isDivisibleBy` y
.
– dfeuer
Nov 13 '18 at 15:35
1
@dfeuer: Yeah, same problem with other names really;divides
with the same definition but used asany (42 `divides`)
makes just as much sense, butdivides 42
is the wrong way around. This is why I prefer functions not be named such that they’re intended to be used infix, because it’s in conflict with using them in prefix; if you do want infix, an operator is preferable. (Besides, I have a personal objection to grave accents being used as quotation characters in a programming language, haha)
– Jon Purdy
Nov 13 '18 at 20:27
add a comment |
Composing (0==)
and (`mod 42`)
:
f :: [Integer] -> Bool
f = any ((0==).(`mod` 42))
futher reducing parenthesis noise:
f :: [Integer] -> Bool
f = any $ (0==).(`mod` 42)
Helper function:
f :: [Integer] -> Bool
f = any div42
where
div42 n = n `mod` 42 == 0
Stylistically, for this function, either way seems fine. However, say you wanted to check if any values are divisible by 42 OR 52, then utilizing composition may become more obfuscated/complex/futile. Whereas using a helper function keeps things readable: div42Or52 n = n `mod` 42 == 0 || n `mod` 52 == 0
. Note, I've used a where
clause above, but a let in
expression or a lambda are possible alternative ways to structure helper functions.
Ultimately, its up to the developer to balance concise code with understandable code.
Composing (0==)
and (`mod 42`)
:
f :: [Integer] -> Bool
f = any ((0==).(`mod` 42))
futher reducing parenthesis noise:
f :: [Integer] -> Bool
f = any $ (0==).(`mod` 42)
Helper function:
f :: [Integer] -> Bool
f = any div42
where
div42 n = n `mod` 42 == 0
Stylistically, for this function, either way seems fine. However, say you wanted to check if any values are divisible by 42 OR 52, then utilizing composition may become more obfuscated/complex/futile. Whereas using a helper function keeps things readable: div42Or52 n = n `mod` 42 == 0 || n `mod` 52 == 0
. Note, I've used a where
clause above, but a let in
expression or a lambda are possible alternative ways to structure helper functions.
Ultimately, its up to the developer to balance concise code with understandable code.
edited Nov 13 '18 at 5:06
answered Nov 13 '18 at 4:07
DavOSDavOS
8261923
8261923
2
For point-free code, it often helps readability to factor things into many small, generic, reusable definitions. For example, in this case I might writedivisibleBy d = (== 0) . (`mod` d)
(“to be divisible by d means to be equal to zero modulo d”) and then useany (divisibleBy 42)
. Nothing wrong with using a lambda or auxiliary definition in awhere
clause, though.
– Jon Purdy
Nov 13 '18 at 8:17
1
@JonPurdy, I completely agree. The only challenge is figuring out the order of the arguments.isDivisibleBy x y
sends a different message thanx `isDivisibleBy` y
.
– dfeuer
Nov 13 '18 at 15:35
1
@dfeuer: Yeah, same problem with other names really;divides
with the same definition but used asany (42 `divides`)
makes just as much sense, butdivides 42
is the wrong way around. This is why I prefer functions not be named such that they’re intended to be used infix, because it’s in conflict with using them in prefix; if you do want infix, an operator is preferable. (Besides, I have a personal objection to grave accents being used as quotation characters in a programming language, haha)
– Jon Purdy
Nov 13 '18 at 20:27
add a comment |
2
For point-free code, it often helps readability to factor things into many small, generic, reusable definitions. For example, in this case I might writedivisibleBy d = (== 0) . (`mod` d)
(“to be divisible by d means to be equal to zero modulo d”) and then useany (divisibleBy 42)
. Nothing wrong with using a lambda or auxiliary definition in awhere
clause, though.
– Jon Purdy
Nov 13 '18 at 8:17
1
@JonPurdy, I completely agree. The only challenge is figuring out the order of the arguments.isDivisibleBy x y
sends a different message thanx `isDivisibleBy` y
.
– dfeuer
Nov 13 '18 at 15:35
1
@dfeuer: Yeah, same problem with other names really;divides
with the same definition but used asany (42 `divides`)
makes just as much sense, butdivides 42
is the wrong way around. This is why I prefer functions not be named such that they’re intended to be used infix, because it’s in conflict with using them in prefix; if you do want infix, an operator is preferable. (Besides, I have a personal objection to grave accents being used as quotation characters in a programming language, haha)
– Jon Purdy
Nov 13 '18 at 20:27
2
2
For point-free code, it often helps readability to factor things into many small, generic, reusable definitions. For example, in this case I might write
divisibleBy d = (== 0) . (`mod` d)
(“to be divisible by d means to be equal to zero modulo d”) and then use any (divisibleBy 42)
. Nothing wrong with using a lambda or auxiliary definition in a where
clause, though.– Jon Purdy
Nov 13 '18 at 8:17
For point-free code, it often helps readability to factor things into many small, generic, reusable definitions. For example, in this case I might write
divisibleBy d = (== 0) . (`mod` d)
(“to be divisible by d means to be equal to zero modulo d”) and then use any (divisibleBy 42)
. Nothing wrong with using a lambda or auxiliary definition in a where
clause, though.– Jon Purdy
Nov 13 '18 at 8:17
1
1
@JonPurdy, I completely agree. The only challenge is figuring out the order of the arguments.
isDivisibleBy x y
sends a different message than x `isDivisibleBy` y
.– dfeuer
Nov 13 '18 at 15:35
@JonPurdy, I completely agree. The only challenge is figuring out the order of the arguments.
isDivisibleBy x y
sends a different message than x `isDivisibleBy` y
.– dfeuer
Nov 13 '18 at 15:35
1
1
@dfeuer: Yeah, same problem with other names really;
divides
with the same definition but used as any (42 `divides`)
makes just as much sense, but divides 42
is the wrong way around. This is why I prefer functions not be named such that they’re intended to be used infix, because it’s in conflict with using them in prefix; if you do want infix, an operator is preferable. (Besides, I have a personal objection to grave accents being used as quotation characters in a programming language, haha)– Jon Purdy
Nov 13 '18 at 20:27
@dfeuer: Yeah, same problem with other names really;
divides
with the same definition but used as any (42 `divides`)
makes just as much sense, but divides 42
is the wrong way around. This is why I prefer functions not be named such that they’re intended to be used infix, because it’s in conflict with using them in prefix; if you do want infix, an operator is preferable. (Besides, I have a personal objection to grave accents being used as quotation characters in a programming language, haha)– Jon Purdy
Nov 13 '18 at 20:27
add a comment |
You can define function inplace:
any (n -> n `mod` 42 == 0) [1, 2, 42]
add a comment |
You can define function inplace:
any (n -> n `mod` 42 == 0) [1, 2, 42]
add a comment |
You can define function inplace:
any (n -> n `mod` 42 == 0) [1, 2, 42]
You can define function inplace:
any (n -> n `mod` 42 == 0) [1, 2, 42]
answered Nov 13 '18 at 4:12
talextalex
10.9k1648
10.9k1648
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53273415%2fuse-any-to-tell-you-true-false-if-a-list-contains-some-values-divisible-by%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sJyqPZEeL5sg9m4MKopuZRU2yN7N,CqS5D,LIJ8n2c 6LYiTTHU3UzSawzEHVMMT9lxHIxspcNCkail80
1
Without a helper definition, the most straightforward way would be with an anonymous function (a "lambda").
– duplode
Nov 13 '18 at 3:41
Holy cow, thank you everyone... i had actually thought of the lambda way later, but couldn't figure out putting together the function for some reason... but once i see it, I'm like, "that makes total sense".
– Stormy
Nov 14 '18 at 6:14