group DataFrame by column value using pandas
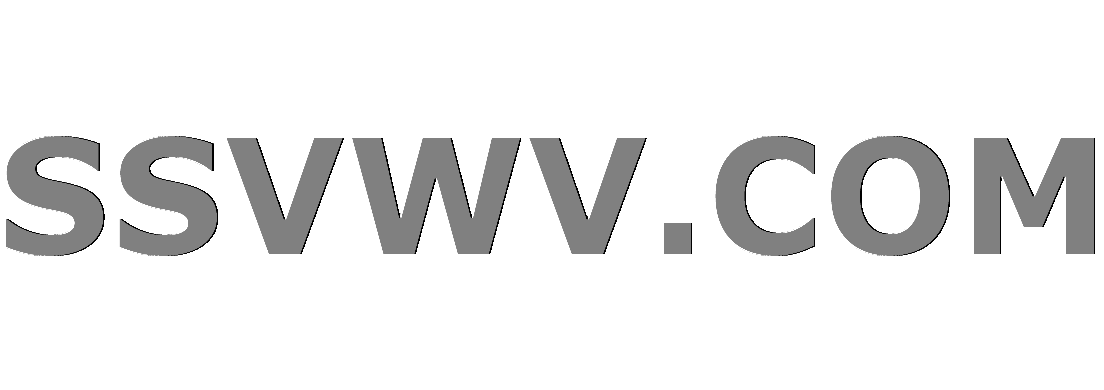
Multi tool use
I have the following (pandas) DataFrame:
b c d e
0 100 369 203 314
1 100 228 784 366
2 200 811 664 202
3 200 531 932 575
I want to iterate its rows but I need to know where the value of b
changed.
I am looking for a way to get this DF content in groups or something like that and then iterate over the rows of each group (with a nested loop and in this way I can get an indication that the b
value did changed):
In the first iteration my new df will be:
b c d e
0 100 369 203 314
1 100 228 784 366
In the second iteration my new df will be:
b c d e
0 200 811 664 202
1 200 531 932 575
python python-2.7 pandas
|
show 3 more comments
I have the following (pandas) DataFrame:
b c d e
0 100 369 203 314
1 100 228 784 366
2 200 811 664 202
3 200 531 932 575
I want to iterate its rows but I need to know where the value of b
changed.
I am looking for a way to get this DF content in groups or something like that and then iterate over the rows of each group (with a nested loop and in this way I can get an indication that the b
value did changed):
In the first iteration my new df will be:
b c d e
0 100 369 203 314
1 100 228 784 366
In the second iteration my new df will be:
b c d e
0 200 811 664 202
1 200 531 932 575
python python-2.7 pandas
1
to clarify: did you want a new df for every single unique value of items in the b column?
– Capn Jack
Nov 13 '18 at 16:14
have you looked into groupby?
– Christian Sloper
Nov 13 '18 at 16:15
I don't really need a new df, if I can get the same result but in other datastructure it will be fine.
– Andy Thomas
Nov 13 '18 at 16:15
d=x: y for x, y in df.groupby('b')
– Wen-Ben
Nov 13 '18 at 16:16
d = dict(tuple(df.groupby('b')))
?
– jezrael
Nov 13 '18 at 16:17
|
show 3 more comments
I have the following (pandas) DataFrame:
b c d e
0 100 369 203 314
1 100 228 784 366
2 200 811 664 202
3 200 531 932 575
I want to iterate its rows but I need to know where the value of b
changed.
I am looking for a way to get this DF content in groups or something like that and then iterate over the rows of each group (with a nested loop and in this way I can get an indication that the b
value did changed):
In the first iteration my new df will be:
b c d e
0 100 369 203 314
1 100 228 784 366
In the second iteration my new df will be:
b c d e
0 200 811 664 202
1 200 531 932 575
python python-2.7 pandas
I have the following (pandas) DataFrame:
b c d e
0 100 369 203 314
1 100 228 784 366
2 200 811 664 202
3 200 531 932 575
I want to iterate its rows but I need to know where the value of b
changed.
I am looking for a way to get this DF content in groups or something like that and then iterate over the rows of each group (with a nested loop and in this way I can get an indication that the b
value did changed):
In the first iteration my new df will be:
b c d e
0 100 369 203 314
1 100 228 784 366
In the second iteration my new df will be:
b c d e
0 200 811 664 202
1 200 531 932 575
python python-2.7 pandas
python python-2.7 pandas
asked Nov 13 '18 at 16:13
Andy ThomasAndy Thomas
4321625
4321625
1
to clarify: did you want a new df for every single unique value of items in the b column?
– Capn Jack
Nov 13 '18 at 16:14
have you looked into groupby?
– Christian Sloper
Nov 13 '18 at 16:15
I don't really need a new df, if I can get the same result but in other datastructure it will be fine.
– Andy Thomas
Nov 13 '18 at 16:15
d=x: y for x, y in df.groupby('b')
– Wen-Ben
Nov 13 '18 at 16:16
d = dict(tuple(df.groupby('b')))
?
– jezrael
Nov 13 '18 at 16:17
|
show 3 more comments
1
to clarify: did you want a new df for every single unique value of items in the b column?
– Capn Jack
Nov 13 '18 at 16:14
have you looked into groupby?
– Christian Sloper
Nov 13 '18 at 16:15
I don't really need a new df, if I can get the same result but in other datastructure it will be fine.
– Andy Thomas
Nov 13 '18 at 16:15
d=x: y for x, y in df.groupby('b')
– Wen-Ben
Nov 13 '18 at 16:16
d = dict(tuple(df.groupby('b')))
?
– jezrael
Nov 13 '18 at 16:17
1
1
to clarify: did you want a new df for every single unique value of items in the b column?
– Capn Jack
Nov 13 '18 at 16:14
to clarify: did you want a new df for every single unique value of items in the b column?
– Capn Jack
Nov 13 '18 at 16:14
have you looked into groupby?
– Christian Sloper
Nov 13 '18 at 16:15
have you looked into groupby?
– Christian Sloper
Nov 13 '18 at 16:15
I don't really need a new df, if I can get the same result but in other datastructure it will be fine.
– Andy Thomas
Nov 13 '18 at 16:15
I don't really need a new df, if I can get the same result but in other datastructure it will be fine.
– Andy Thomas
Nov 13 '18 at 16:15
d=x: y for x, y in df.groupby('b')
– Wen-Ben
Nov 13 '18 at 16:16
d=x: y for x, y in df.groupby('b')
– Wen-Ben
Nov 13 '18 at 16:16
d = dict(tuple(df.groupby('b')))
?– jezrael
Nov 13 '18 at 16:17
d = dict(tuple(df.groupby('b')))
?– jezrael
Nov 13 '18 at 16:17
|
show 3 more comments
1 Answer
1
active
oldest
votes
You can use the groupby method:
from pandas import DataFrame
columns = ["b", "c", "d", "e"]
data = [[100, 369, 203, 314], [100, 228, 784, 366], [200, 811, 664, 202], [200, 531, 932, 575]]
df = DataFrame(data=data,columns=columns)
def split_dfs(df, col):
return [group[1] for group in list(df.groupby(col))]
dfs = split_dfs(df, "b")
for df_group in dfs:
print(df_group)
b c d e
0 100 369 203 314
1 100 228 784 366
b c d e
2 200 811 664 202
3 200 531 932 575
Perfect! thank you.
– Andy Thomas
Nov 14 '18 at 8:56
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53285122%2fgroup-dataframe-by-column-value-using-pandas%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use the groupby method:
from pandas import DataFrame
columns = ["b", "c", "d", "e"]
data = [[100, 369, 203, 314], [100, 228, 784, 366], [200, 811, 664, 202], [200, 531, 932, 575]]
df = DataFrame(data=data,columns=columns)
def split_dfs(df, col):
return [group[1] for group in list(df.groupby(col))]
dfs = split_dfs(df, "b")
for df_group in dfs:
print(df_group)
b c d e
0 100 369 203 314
1 100 228 784 366
b c d e
2 200 811 664 202
3 200 531 932 575
Perfect! thank you.
– Andy Thomas
Nov 14 '18 at 8:56
add a comment |
You can use the groupby method:
from pandas import DataFrame
columns = ["b", "c", "d", "e"]
data = [[100, 369, 203, 314], [100, 228, 784, 366], [200, 811, 664, 202], [200, 531, 932, 575]]
df = DataFrame(data=data,columns=columns)
def split_dfs(df, col):
return [group[1] for group in list(df.groupby(col))]
dfs = split_dfs(df, "b")
for df_group in dfs:
print(df_group)
b c d e
0 100 369 203 314
1 100 228 784 366
b c d e
2 200 811 664 202
3 200 531 932 575
Perfect! thank you.
– Andy Thomas
Nov 14 '18 at 8:56
add a comment |
You can use the groupby method:
from pandas import DataFrame
columns = ["b", "c", "d", "e"]
data = [[100, 369, 203, 314], [100, 228, 784, 366], [200, 811, 664, 202], [200, 531, 932, 575]]
df = DataFrame(data=data,columns=columns)
def split_dfs(df, col):
return [group[1] for group in list(df.groupby(col))]
dfs = split_dfs(df, "b")
for df_group in dfs:
print(df_group)
b c d e
0 100 369 203 314
1 100 228 784 366
b c d e
2 200 811 664 202
3 200 531 932 575
You can use the groupby method:
from pandas import DataFrame
columns = ["b", "c", "d", "e"]
data = [[100, 369, 203, 314], [100, 228, 784, 366], [200, 811, 664, 202], [200, 531, 932, 575]]
df = DataFrame(data=data,columns=columns)
def split_dfs(df, col):
return [group[1] for group in list(df.groupby(col))]
dfs = split_dfs(df, "b")
for df_group in dfs:
print(df_group)
b c d e
0 100 369 203 314
1 100 228 784 366
b c d e
2 200 811 664 202
3 200 531 932 575
edited Nov 14 '18 at 9:08
answered Nov 13 '18 at 16:34
user2921352user2921352
988
988
Perfect! thank you.
– Andy Thomas
Nov 14 '18 at 8:56
add a comment |
Perfect! thank you.
– Andy Thomas
Nov 14 '18 at 8:56
Perfect! thank you.
– Andy Thomas
Nov 14 '18 at 8:56
Perfect! thank you.
– Andy Thomas
Nov 14 '18 at 8:56
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53285122%2fgroup-dataframe-by-column-value-using-pandas%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
bv hBh l3UdWElefa5nIQKWnLfNZLSVdT7UxjaV13xxiBVpMSquo188 aZ709J qr aWbs,lXlMqn060D
1
to clarify: did you want a new df for every single unique value of items in the b column?
– Capn Jack
Nov 13 '18 at 16:14
have you looked into groupby?
– Christian Sloper
Nov 13 '18 at 16:15
I don't really need a new df, if I can get the same result but in other datastructure it will be fine.
– Andy Thomas
Nov 13 '18 at 16:15
d=x: y for x, y in df.groupby('b')
– Wen-Ben
Nov 13 '18 at 16:16
d = dict(tuple(df.groupby('b')))
?– jezrael
Nov 13 '18 at 16:17