center a table in tkinter
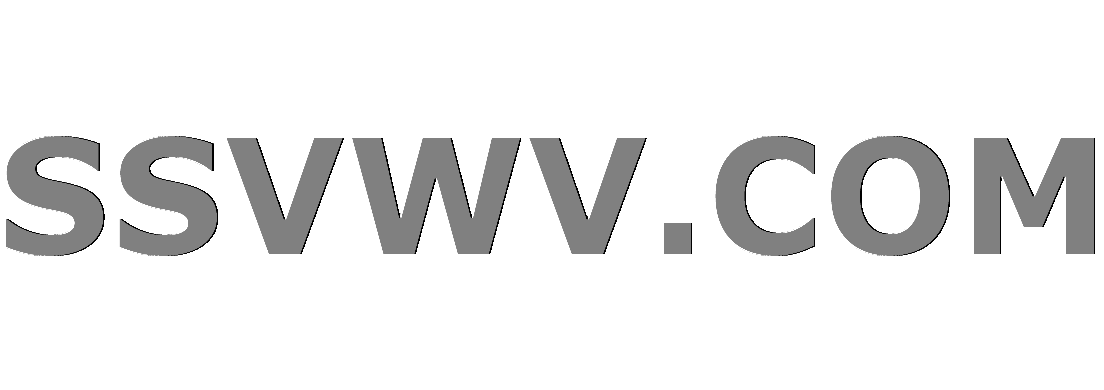
Multi tool use
I am tkintering with tkinter :) and trying to center the "StackQuestion" table.
I do not know what is the option to center horizontally, and or vertically the table inside of the receiving container.
The first class, Class StackQuestion defines how the general windows works and how to switch from container to container. The second, define the table and its content.
I have tried adding different colours to the different containers in order to check which container is active and thereof to try to center everything that is inside the later, without much success.
edit : in order not to have lots of code here I have deleted all the other classes and duplicate "Test" in "for F in (Test, Test):" in order for the code to run on your computer.
#utf8
#python3
import tkinter as tk
from tkinter import *
from tkinter import ttk
class StackQuestion(tk.Tk):
def __init__(self, *args, **kwargs):
tk.Tk.__init__(self, *args, **kwargs)
container = ttk.Frame(self)
container.grid()
#windows resize management
container.grid_rowconfigure(0, weight=1)
container.grid_columnconfigure(0, weight=1)
self.frames =
for F in (Test, Test):
frame = F(container, self)
self.frames[F] = frame
frame.grid(row=1, column=1, sticky='nsew')
self.show_frame(Test)
def show_frame(self, cont):
frame = self.frames[cont]
frame.tkraise()
class Test(tk.Frame):
def __init__(self, parent, controller):
tk.Frame.__init__(self, parent)
label = tk.Label(self, text = 'StackQuestion')
label.grid(row=0, column=0, columnspan=2)
questions = ['Question 1', 'Question 2' , 'Question 3']
row_number = 1
column_number = 0
Tree = ttk.Treeview(self, show ='headings')
Tree['columns'] = ('Author', 'Subject', 'Date', 'Question')
Tree.heading('Author', text = 'Author')
Tree.heading('Subject', text = 'Subject')
Tree.heading('Date', text = 'Date')
Tree.heading('Question', text = 'Question')
for value in questions :
Tree.insert('', 'end', value, text = value)
Tree.set(value, 'Author', value)
Tree.grid()
app = StackQuestion()
app.geometry('1080x700')
app.configure(background='grey')
app.mainloop()
python python-3.x tkinter
add a comment |
I am tkintering with tkinter :) and trying to center the "StackQuestion" table.
I do not know what is the option to center horizontally, and or vertically the table inside of the receiving container.
The first class, Class StackQuestion defines how the general windows works and how to switch from container to container. The second, define the table and its content.
I have tried adding different colours to the different containers in order to check which container is active and thereof to try to center everything that is inside the later, without much success.
edit : in order not to have lots of code here I have deleted all the other classes and duplicate "Test" in "for F in (Test, Test):" in order for the code to run on your computer.
#utf8
#python3
import tkinter as tk
from tkinter import *
from tkinter import ttk
class StackQuestion(tk.Tk):
def __init__(self, *args, **kwargs):
tk.Tk.__init__(self, *args, **kwargs)
container = ttk.Frame(self)
container.grid()
#windows resize management
container.grid_rowconfigure(0, weight=1)
container.grid_columnconfigure(0, weight=1)
self.frames =
for F in (Test, Test):
frame = F(container, self)
self.frames[F] = frame
frame.grid(row=1, column=1, sticky='nsew')
self.show_frame(Test)
def show_frame(self, cont):
frame = self.frames[cont]
frame.tkraise()
class Test(tk.Frame):
def __init__(self, parent, controller):
tk.Frame.__init__(self, parent)
label = tk.Label(self, text = 'StackQuestion')
label.grid(row=0, column=0, columnspan=2)
questions = ['Question 1', 'Question 2' , 'Question 3']
row_number = 1
column_number = 0
Tree = ttk.Treeview(self, show ='headings')
Tree['columns'] = ('Author', 'Subject', 'Date', 'Question')
Tree.heading('Author', text = 'Author')
Tree.heading('Subject', text = 'Subject')
Tree.heading('Date', text = 'Date')
Tree.heading('Question', text = 'Question')
for value in questions :
Tree.insert('', 'end', value, text = value)
Tree.set(value, 'Author', value)
Tree.grid()
app = StackQuestion()
app.geometry('1080x700')
app.configure(background='grey')
app.mainloop()
python python-3.x tkinter
1
If you replacecontainer.grid()
withcontainer.pack()
it automatically centers it...
– toti08
Nov 14 '18 at 11:12
1
However you could also consider changing the geometry of your app and make it fit your table.
– toti08
Nov 14 '18 at 11:12
add a comment |
I am tkintering with tkinter :) and trying to center the "StackQuestion" table.
I do not know what is the option to center horizontally, and or vertically the table inside of the receiving container.
The first class, Class StackQuestion defines how the general windows works and how to switch from container to container. The second, define the table and its content.
I have tried adding different colours to the different containers in order to check which container is active and thereof to try to center everything that is inside the later, without much success.
edit : in order not to have lots of code here I have deleted all the other classes and duplicate "Test" in "for F in (Test, Test):" in order for the code to run on your computer.
#utf8
#python3
import tkinter as tk
from tkinter import *
from tkinter import ttk
class StackQuestion(tk.Tk):
def __init__(self, *args, **kwargs):
tk.Tk.__init__(self, *args, **kwargs)
container = ttk.Frame(self)
container.grid()
#windows resize management
container.grid_rowconfigure(0, weight=1)
container.grid_columnconfigure(0, weight=1)
self.frames =
for F in (Test, Test):
frame = F(container, self)
self.frames[F] = frame
frame.grid(row=1, column=1, sticky='nsew')
self.show_frame(Test)
def show_frame(self, cont):
frame = self.frames[cont]
frame.tkraise()
class Test(tk.Frame):
def __init__(self, parent, controller):
tk.Frame.__init__(self, parent)
label = tk.Label(self, text = 'StackQuestion')
label.grid(row=0, column=0, columnspan=2)
questions = ['Question 1', 'Question 2' , 'Question 3']
row_number = 1
column_number = 0
Tree = ttk.Treeview(self, show ='headings')
Tree['columns'] = ('Author', 'Subject', 'Date', 'Question')
Tree.heading('Author', text = 'Author')
Tree.heading('Subject', text = 'Subject')
Tree.heading('Date', text = 'Date')
Tree.heading('Question', text = 'Question')
for value in questions :
Tree.insert('', 'end', value, text = value)
Tree.set(value, 'Author', value)
Tree.grid()
app = StackQuestion()
app.geometry('1080x700')
app.configure(background='grey')
app.mainloop()
python python-3.x tkinter
I am tkintering with tkinter :) and trying to center the "StackQuestion" table.
I do not know what is the option to center horizontally, and or vertically the table inside of the receiving container.
The first class, Class StackQuestion defines how the general windows works and how to switch from container to container. The second, define the table and its content.
I have tried adding different colours to the different containers in order to check which container is active and thereof to try to center everything that is inside the later, without much success.
edit : in order not to have lots of code here I have deleted all the other classes and duplicate "Test" in "for F in (Test, Test):" in order for the code to run on your computer.
#utf8
#python3
import tkinter as tk
from tkinter import *
from tkinter import ttk
class StackQuestion(tk.Tk):
def __init__(self, *args, **kwargs):
tk.Tk.__init__(self, *args, **kwargs)
container = ttk.Frame(self)
container.grid()
#windows resize management
container.grid_rowconfigure(0, weight=1)
container.grid_columnconfigure(0, weight=1)
self.frames =
for F in (Test, Test):
frame = F(container, self)
self.frames[F] = frame
frame.grid(row=1, column=1, sticky='nsew')
self.show_frame(Test)
def show_frame(self, cont):
frame = self.frames[cont]
frame.tkraise()
class Test(tk.Frame):
def __init__(self, parent, controller):
tk.Frame.__init__(self, parent)
label = tk.Label(self, text = 'StackQuestion')
label.grid(row=0, column=0, columnspan=2)
questions = ['Question 1', 'Question 2' , 'Question 3']
row_number = 1
column_number = 0
Tree = ttk.Treeview(self, show ='headings')
Tree['columns'] = ('Author', 'Subject', 'Date', 'Question')
Tree.heading('Author', text = 'Author')
Tree.heading('Subject', text = 'Subject')
Tree.heading('Date', text = 'Date')
Tree.heading('Question', text = 'Question')
for value in questions :
Tree.insert('', 'end', value, text = value)
Tree.set(value, 'Author', value)
Tree.grid()
app = StackQuestion()
app.geometry('1080x700')
app.configure(background='grey')
app.mainloop()
python python-3.x tkinter
python python-3.x tkinter
edited Nov 14 '18 at 11:00


martineau
68.8k1091185
68.8k1091185
asked Nov 14 '18 at 10:56
PelicanPelican
6110
6110
1
If you replacecontainer.grid()
withcontainer.pack()
it automatically centers it...
– toti08
Nov 14 '18 at 11:12
1
However you could also consider changing the geometry of your app and make it fit your table.
– toti08
Nov 14 '18 at 11:12
add a comment |
1
If you replacecontainer.grid()
withcontainer.pack()
it automatically centers it...
– toti08
Nov 14 '18 at 11:12
1
However you could also consider changing the geometry of your app and make it fit your table.
– toti08
Nov 14 '18 at 11:12
1
1
If you replace
container.grid()
with container.pack()
it automatically centers it...– toti08
Nov 14 '18 at 11:12
If you replace
container.grid()
with container.pack()
it automatically centers it...– toti08
Nov 14 '18 at 11:12
1
1
However you could also consider changing the geometry of your app and make it fit your table.
– toti08
Nov 14 '18 at 11:12
However you could also consider changing the geometry of your app and make it fit your table.
– toti08
Nov 14 '18 at 11:12
add a comment |
1 Answer
1
active
oldest
votes
I'm not an expert python programmer, in fact I'm still a newbie and I'm tkintering too in order to learn. So I've found this "noob-fix" following the hint by toti08
You can change
container.grid()
with:
container.pack(pady=50)
This will center it horizzontally and give the table a bit of padding top so you can also center it vertically or at least give it a little margin on the border.
Hope this helps until someone more experienced comes along with a solution to your problem :)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53298559%2fcenter-a-table-in-tkinter%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I'm not an expert python programmer, in fact I'm still a newbie and I'm tkintering too in order to learn. So I've found this "noob-fix" following the hint by toti08
You can change
container.grid()
with:
container.pack(pady=50)
This will center it horizzontally and give the table a bit of padding top so you can also center it vertically or at least give it a little margin on the border.
Hope this helps until someone more experienced comes along with a solution to your problem :)
add a comment |
I'm not an expert python programmer, in fact I'm still a newbie and I'm tkintering too in order to learn. So I've found this "noob-fix" following the hint by toti08
You can change
container.grid()
with:
container.pack(pady=50)
This will center it horizzontally and give the table a bit of padding top so you can also center it vertically or at least give it a little margin on the border.
Hope this helps until someone more experienced comes along with a solution to your problem :)
add a comment |
I'm not an expert python programmer, in fact I'm still a newbie and I'm tkintering too in order to learn. So I've found this "noob-fix" following the hint by toti08
You can change
container.grid()
with:
container.pack(pady=50)
This will center it horizzontally and give the table a bit of padding top so you can also center it vertically or at least give it a little margin on the border.
Hope this helps until someone more experienced comes along with a solution to your problem :)
I'm not an expert python programmer, in fact I'm still a newbie and I'm tkintering too in order to learn. So I've found this "noob-fix" following the hint by toti08
You can change
container.grid()
with:
container.pack(pady=50)
This will center it horizzontally and give the table a bit of padding top so you can also center it vertically or at least give it a little margin on the border.
Hope this helps until someone more experienced comes along with a solution to your problem :)
answered Nov 15 '18 at 15:44
John WilverJohn Wilver
185
185
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53298559%2fcenter-a-table-in-tkinter%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
MDTkD nMQ9qMNlurflS54W1tBozG,ERUkA,PDILAQKp3ltq63tvGSpAlOLuzZc2yO1nnNy53tF,x 9d,bbSrm2vxVsrLK,F iD4BR,VI2Ee9
1
If you replace
container.grid()
withcontainer.pack()
it automatically centers it...– toti08
Nov 14 '18 at 11:12
1
However you could also consider changing the geometry of your app and make it fit your table.
– toti08
Nov 14 '18 at 11:12