using spring boot app as resource server and google oauth2 as authorization server
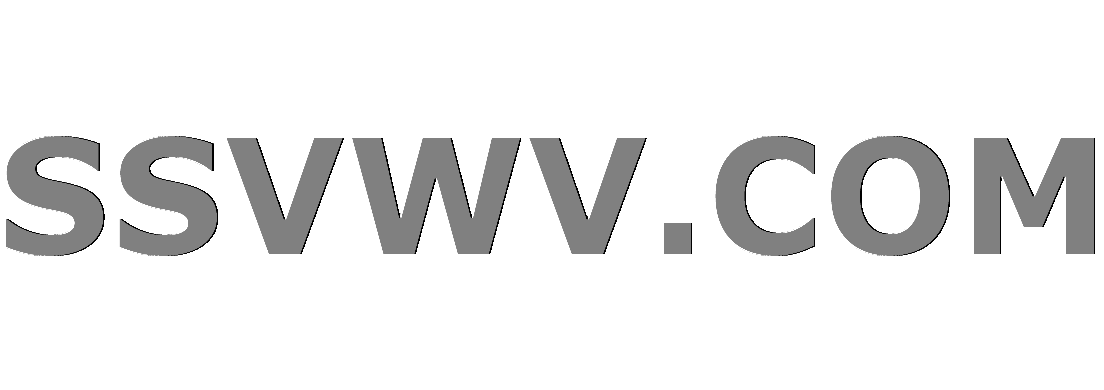
Multi tool use
I have a web app using angular6 for frontend and spring boot for the rest apis.
My angular app is running on localhost:4200 and is able to authenticate successfully with google and I can see authToken and idToken. (I used angularx-social-login plugin in angular)
Now, I want to use this auth token to secure my spring boot api server running at localhost:8080 as well.
I am trying to do similar to (image source)
Here client is my angular app, resource server is spring boot application and authorization server is google
I tried using EnableResourceServer annotation and many different approaches but nothing seems to work. It always gives me invalid token by checking in memory token store and doesn't even try to validate with google for the right token.
I am using the same client-id and secret for the oauth2 configuration in spring app and angular app.
How can I make spring boot application validate the token with google for every request?
My application.properties
server.servlet.context-path=/api
spring.h2.console.enabled=true
spring.datasource.url=jdbc:h2:file:~/h2/testdb
logging.level.org.springframework.web=TRACE
logging.level.org.springframework.security=TRACE
logging.level.org.hibernate=TRACE
security.oauth2.resource.filter-order = 3
spring.security.oauth2.client.registration.google.client-id=#google-client-id
spring.security.oauth2.client.registration.google.client-secret=#google-client-secret
security.oauth2.resource.user-info-uri= https://www.googleapis.com/oauth2/v3/userinfo # URI of the user endpoint.
Here is my resource server configuration class
@Configuration
@EnableResourceServer
public class OAuth2ResourceServerConfig extends ResourceServerConfigurerAdapter
private static final String RESOURCE_ID = "rest_api";
@Override
public void configure(ResourceServerSecurityConfigurer resources)
resources.resourceId(RESOURCE_ID).stateless(false);
@Override
public void configure(HttpSecurity http) throws Exception
http
.requestMatcher(new RequestHeaderRequestMatcher("Authorization"))
.authorizeRequests().anyRequest().fullyAuthenticated();
@EnableGlobalMethodSecurity(prePostEnabled = true)
protected static class GlobalSecurityConfiguration extends GlobalMethodSecurityConfiguration
@Override
protected MethodSecurityExpressionHandler createExpressionHandler()
return new OAuth2MethodSecurityExpressionHandler();

add a comment |
I have a web app using angular6 for frontend and spring boot for the rest apis.
My angular app is running on localhost:4200 and is able to authenticate successfully with google and I can see authToken and idToken. (I used angularx-social-login plugin in angular)
Now, I want to use this auth token to secure my spring boot api server running at localhost:8080 as well.
I am trying to do similar to (image source)
Here client is my angular app, resource server is spring boot application and authorization server is google
I tried using EnableResourceServer annotation and many different approaches but nothing seems to work. It always gives me invalid token by checking in memory token store and doesn't even try to validate with google for the right token.
I am using the same client-id and secret for the oauth2 configuration in spring app and angular app.
How can I make spring boot application validate the token with google for every request?
My application.properties
server.servlet.context-path=/api
spring.h2.console.enabled=true
spring.datasource.url=jdbc:h2:file:~/h2/testdb
logging.level.org.springframework.web=TRACE
logging.level.org.springframework.security=TRACE
logging.level.org.hibernate=TRACE
security.oauth2.resource.filter-order = 3
spring.security.oauth2.client.registration.google.client-id=#google-client-id
spring.security.oauth2.client.registration.google.client-secret=#google-client-secret
security.oauth2.resource.user-info-uri= https://www.googleapis.com/oauth2/v3/userinfo # URI of the user endpoint.
Here is my resource server configuration class
@Configuration
@EnableResourceServer
public class OAuth2ResourceServerConfig extends ResourceServerConfigurerAdapter
private static final String RESOURCE_ID = "rest_api";
@Override
public void configure(ResourceServerSecurityConfigurer resources)
resources.resourceId(RESOURCE_ID).stateless(false);
@Override
public void configure(HttpSecurity http) throws Exception
http
.requestMatcher(new RequestHeaderRequestMatcher("Authorization"))
.authorizeRequests().anyRequest().fullyAuthenticated();
@EnableGlobalMethodSecurity(prePostEnabled = true)
protected static class GlobalSecurityConfiguration extends GlobalMethodSecurityConfiguration
@Override
protected MethodSecurityExpressionHandler createExpressionHandler()
return new OAuth2MethodSecurityExpressionHandler();

add a comment |
I have a web app using angular6 for frontend and spring boot for the rest apis.
My angular app is running on localhost:4200 and is able to authenticate successfully with google and I can see authToken and idToken. (I used angularx-social-login plugin in angular)
Now, I want to use this auth token to secure my spring boot api server running at localhost:8080 as well.
I am trying to do similar to (image source)
Here client is my angular app, resource server is spring boot application and authorization server is google
I tried using EnableResourceServer annotation and many different approaches but nothing seems to work. It always gives me invalid token by checking in memory token store and doesn't even try to validate with google for the right token.
I am using the same client-id and secret for the oauth2 configuration in spring app and angular app.
How can I make spring boot application validate the token with google for every request?
My application.properties
server.servlet.context-path=/api
spring.h2.console.enabled=true
spring.datasource.url=jdbc:h2:file:~/h2/testdb
logging.level.org.springframework.web=TRACE
logging.level.org.springframework.security=TRACE
logging.level.org.hibernate=TRACE
security.oauth2.resource.filter-order = 3
spring.security.oauth2.client.registration.google.client-id=#google-client-id
spring.security.oauth2.client.registration.google.client-secret=#google-client-secret
security.oauth2.resource.user-info-uri= https://www.googleapis.com/oauth2/v3/userinfo # URI of the user endpoint.
Here is my resource server configuration class
@Configuration
@EnableResourceServer
public class OAuth2ResourceServerConfig extends ResourceServerConfigurerAdapter
private static final String RESOURCE_ID = "rest_api";
@Override
public void configure(ResourceServerSecurityConfigurer resources)
resources.resourceId(RESOURCE_ID).stateless(false);
@Override
public void configure(HttpSecurity http) throws Exception
http
.requestMatcher(new RequestHeaderRequestMatcher("Authorization"))
.authorizeRequests().anyRequest().fullyAuthenticated();
@EnableGlobalMethodSecurity(prePostEnabled = true)
protected static class GlobalSecurityConfiguration extends GlobalMethodSecurityConfiguration
@Override
protected MethodSecurityExpressionHandler createExpressionHandler()
return new OAuth2MethodSecurityExpressionHandler();

I have a web app using angular6 for frontend and spring boot for the rest apis.
My angular app is running on localhost:4200 and is able to authenticate successfully with google and I can see authToken and idToken. (I used angularx-social-login plugin in angular)
Now, I want to use this auth token to secure my spring boot api server running at localhost:8080 as well.
I am trying to do similar to (image source)
Here client is my angular app, resource server is spring boot application and authorization server is google
I tried using EnableResourceServer annotation and many different approaches but nothing seems to work. It always gives me invalid token by checking in memory token store and doesn't even try to validate with google for the right token.
I am using the same client-id and secret for the oauth2 configuration in spring app and angular app.
How can I make spring boot application validate the token with google for every request?
My application.properties
server.servlet.context-path=/api
spring.h2.console.enabled=true
spring.datasource.url=jdbc:h2:file:~/h2/testdb
logging.level.org.springframework.web=TRACE
logging.level.org.springframework.security=TRACE
logging.level.org.hibernate=TRACE
security.oauth2.resource.filter-order = 3
spring.security.oauth2.client.registration.google.client-id=#google-client-id
spring.security.oauth2.client.registration.google.client-secret=#google-client-secret
security.oauth2.resource.user-info-uri= https://www.googleapis.com/oauth2/v3/userinfo # URI of the user endpoint.
Here is my resource server configuration class
@Configuration
@EnableResourceServer
public class OAuth2ResourceServerConfig extends ResourceServerConfigurerAdapter
private static final String RESOURCE_ID = "rest_api";
@Override
public void configure(ResourceServerSecurityConfigurer resources)
resources.resourceId(RESOURCE_ID).stateless(false);
@Override
public void configure(HttpSecurity http) throws Exception
http
.requestMatcher(new RequestHeaderRequestMatcher("Authorization"))
.authorizeRequests().anyRequest().fullyAuthenticated();
@EnableGlobalMethodSecurity(prePostEnabled = true)
protected static class GlobalSecurityConfiguration extends GlobalMethodSecurityConfiguration
@Override
protected MethodSecurityExpressionHandler createExpressionHandler()
return new OAuth2MethodSecurityExpressionHandler();


asked Nov 14 '18 at 6:34
Rajani KaruturiRajani Karuturi
2,60012036
2,60012036
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53294357%2fusing-spring-boot-app-as-resource-server-and-google-oauth2-as-authorization-serv%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53294357%2fusing-spring-boot-app-as-resource-server-and-google-oauth2-as-authorization-serv%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DySqtYLU O