How to find related table/view name to use for from statement by using a value in SQL Server
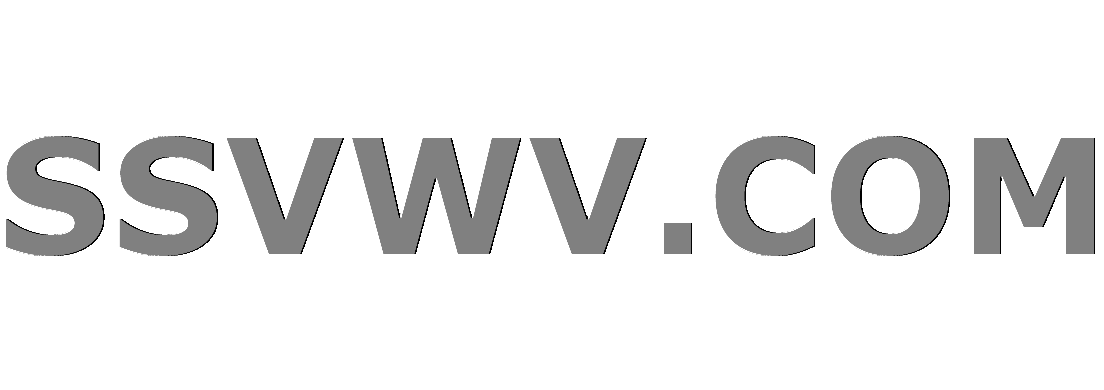
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty height:90px;width:728px;box-sizing:border-box;
I have a table X in SQL Server which includes a column called Type varchar(10)
with 15 potential values: AAA, BBB1, BBB2, CCC, ...
, and another column called IfExists bit
.
In the same DB, I have 15 different views, a view for each Type
, including X_ID
as well. Examples of view names:
View_ReportingAAA
View_ReportingBBB1
View_ReportingBBB2
View_ReportingCCC
....
Usually what I do is to see the Type
of the record by using its X_ID
provided to me in Table X
and use a select query to the related view to get some data.
What I need to do is now to create a trigger for Table X
to set 1 for the value of IfExists
field if there is a record in the related view for the record id X_ID
.
My question is: What is the best way to find and use the related view name for this purpose by using a value of a field in the main table?
I am using the code below for this, but I am not sure if it is the most efficient way to handle this. Any help or advice would be appreciated.
declare @ifExists int = 0;
declare @sql nvarchar(max) = 'set @ifExists = (Select count(*) from View_Reporting';
declare @tablename nvarchar(max) = (Select Type from X where X_ID = @X_ID)
set @sql = @sql + @tablename + ' where V_xid = @X_ID)';
EXECUTE sp_executesql @sql
if (@ifExists > 0)
begin
update X set IfExists = 1 where X_ID = @X_ID
end
sql

add a comment |
I have a table X in SQL Server which includes a column called Type varchar(10)
with 15 potential values: AAA, BBB1, BBB2, CCC, ...
, and another column called IfExists bit
.
In the same DB, I have 15 different views, a view for each Type
, including X_ID
as well. Examples of view names:
View_ReportingAAA
View_ReportingBBB1
View_ReportingBBB2
View_ReportingCCC
....
Usually what I do is to see the Type
of the record by using its X_ID
provided to me in Table X
and use a select query to the related view to get some data.
What I need to do is now to create a trigger for Table X
to set 1 for the value of IfExists
field if there is a record in the related view for the record id X_ID
.
My question is: What is the best way to find and use the related view name for this purpose by using a value of a field in the main table?
I am using the code below for this, but I am not sure if it is the most efficient way to handle this. Any help or advice would be appreciated.
declare @ifExists int = 0;
declare @sql nvarchar(max) = 'set @ifExists = (Select count(*) from View_Reporting';
declare @tablename nvarchar(max) = (Select Type from X where X_ID = @X_ID)
set @sql = @sql + @tablename + ' where V_xid = @X_ID)';
EXECUTE sp_executesql @sql
if (@ifExists > 0)
begin
update X set IfExists = 1 where X_ID = @X_ID
end
sql

add a comment |
I have a table X in SQL Server which includes a column called Type varchar(10)
with 15 potential values: AAA, BBB1, BBB2, CCC, ...
, and another column called IfExists bit
.
In the same DB, I have 15 different views, a view for each Type
, including X_ID
as well. Examples of view names:
View_ReportingAAA
View_ReportingBBB1
View_ReportingBBB2
View_ReportingCCC
....
Usually what I do is to see the Type
of the record by using its X_ID
provided to me in Table X
and use a select query to the related view to get some data.
What I need to do is now to create a trigger for Table X
to set 1 for the value of IfExists
field if there is a record in the related view for the record id X_ID
.
My question is: What is the best way to find and use the related view name for this purpose by using a value of a field in the main table?
I am using the code below for this, but I am not sure if it is the most efficient way to handle this. Any help or advice would be appreciated.
declare @ifExists int = 0;
declare @sql nvarchar(max) = 'set @ifExists = (Select count(*) from View_Reporting';
declare @tablename nvarchar(max) = (Select Type from X where X_ID = @X_ID)
set @sql = @sql + @tablename + ' where V_xid = @X_ID)';
EXECUTE sp_executesql @sql
if (@ifExists > 0)
begin
update X set IfExists = 1 where X_ID = @X_ID
end
sql

I have a table X in SQL Server which includes a column called Type varchar(10)
with 15 potential values: AAA, BBB1, BBB2, CCC, ...
, and another column called IfExists bit
.
In the same DB, I have 15 different views, a view for each Type
, including X_ID
as well. Examples of view names:
View_ReportingAAA
View_ReportingBBB1
View_ReportingBBB2
View_ReportingCCC
....
Usually what I do is to see the Type
of the record by using its X_ID
provided to me in Table X
and use a select query to the related view to get some data.
What I need to do is now to create a trigger for Table X
to set 1 for the value of IfExists
field if there is a record in the related view for the record id X_ID
.
My question is: What is the best way to find and use the related view name for this purpose by using a value of a field in the main table?
I am using the code below for this, but I am not sure if it is the most efficient way to handle this. Any help or advice would be appreciated.
declare @ifExists int = 0;
declare @sql nvarchar(max) = 'set @ifExists = (Select count(*) from View_Reporting';
declare @tablename nvarchar(max) = (Select Type from X where X_ID = @X_ID)
set @sql = @sql + @tablename + ' where V_xid = @X_ID)';
EXECUTE sp_executesql @sql
if (@ifExists > 0)
begin
update X set IfExists = 1 where X_ID = @X_ID
end
sql

sql

edited Nov 15 '18 at 17:38
marc_s
586k13011281272
586k13011281272
asked Nov 15 '18 at 17:19


Eray BalkanliEray Balkanli
4,65852347
4,65852347
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Maybe this could be easier:
declare @ifExists bit
select @ifExists=count(*) from INFORMATION_SCHEMA.VIEWS
where TABLE_NAME='View_Reporting'+(select [Type] from X where X_ID=@X_ID)
update X set IfExists=@IfExists where X_ID=@X_ID and IfExists<>@IfExists
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "1"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53324796%2fhow-to-find-related-table-view-name-to-use-for-from-statement-by-using-a-value-i%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Maybe this could be easier:
declare @ifExists bit
select @ifExists=count(*) from INFORMATION_SCHEMA.VIEWS
where TABLE_NAME='View_Reporting'+(select [Type] from X where X_ID=@X_ID)
update X set IfExists=@IfExists where X_ID=@X_ID and IfExists<>@IfExists
add a comment |
Maybe this could be easier:
declare @ifExists bit
select @ifExists=count(*) from INFORMATION_SCHEMA.VIEWS
where TABLE_NAME='View_Reporting'+(select [Type] from X where X_ID=@X_ID)
update X set IfExists=@IfExists where X_ID=@X_ID and IfExists<>@IfExists
add a comment |
Maybe this could be easier:
declare @ifExists bit
select @ifExists=count(*) from INFORMATION_SCHEMA.VIEWS
where TABLE_NAME='View_Reporting'+(select [Type] from X where X_ID=@X_ID)
update X set IfExists=@IfExists where X_ID=@X_ID and IfExists<>@IfExists
Maybe this could be easier:
declare @ifExists bit
select @ifExists=count(*) from INFORMATION_SCHEMA.VIEWS
where TABLE_NAME='View_Reporting'+(select [Type] from X where X_ID=@X_ID)
update X set IfExists=@IfExists where X_ID=@X_ID and IfExists<>@IfExists
answered Nov 24 '18 at 21:37


XabiXabi
23126
23126
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53324796%2fhow-to-find-related-table-view-name-to-use-for-from-statement-by-using-a-value-i%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Zi9ZiXkztg,SG1U E r81D5BMgCXbrwAnrHyIwkzXr2